Chapter 21
Introduction to Quantum Mechanics in Rust
"Anyone who is not shocked by quantum theory has not understood it." — Niels Bohr
Chapter 21 of CPVR introduces the foundational concepts of quantum mechanics and explores how these concepts can be implemented using Rust. The chapter covers essential topics such as the Schrödinger equation, quantum states, and operators, as well as more advanced ideas like quantum superposition, entanglement, and tunneling. Practical sections guide the reader through implementing these quantum mechanical models in Rust, using numerical methods and Rust’s powerful libraries. The chapter also touches on the basics of quantum computing, offering a glimpse into how Rust can be used to simulate quantum algorithms and circuits. By combining theoretical explanations with practical coding exercises, this chapter demonstrates how Rust can serve as a robust tool for exploring and understanding the quantum world.
21.1. Fundamentals of Quantum Mechanics
The history of quantum computing is deeply rooted in the early 20th century discoveries in quantum mechanics. Key contributors like Max Planck, Albert Einstein, and Niels Bohr laid the theoretical foundations for this field. Planck’s quantum hypothesis (1900) introduced the idea of quantized energy levels, while Einstein's work on the photoelectric effect (1905) supported the particle-like nature of light. Bohr's atomic model (1913) deepened the understanding of quantized energy in atomic systems. Werner Heisenberg’s uncertainty principle (1927) highlighted the measurement limitations in quantum systems, and the Einstein-Podolsky-Rosen (EPR) paradox (1935) along with John Bell’s inequalities (1964) brought attention to quantum entanglement, a key concept in quantum computing. John von Neumann’s development of the mathematical framework for quantum mechanics in the 1930s further provided the formal basis for quantum systems. The First Conference on Physics and Computation (PhysComp) in 1980 marked the formal recognition of quantum computing as a field, bringing together experts to explore its computational potential.
In the early 1980s, quantum computing began to take shape as researchers explored the potential of quantum mechanics to revolutionize computation. Richard Feynman (1981) highlighted the limitations of classical computers in simulating quantum systems, proposing quantum computers as a solution. Paul Benioff (1982) introduced the Quantum Turing Machine (QTM), demonstrating the application of quantum principles to computation. David Deutsch (1985) built on these ideas, presenting the concept of a universal quantum computer capable of outperforming classical machines with quantum mechanics. Researchers also developed quantum logic gates, like the CNOT and Toffoli gates, essential for quantum circuits. These breakthroughs laid the foundation for future advancements in quantum algorithms and hardware, marking the emergence of quantum computing as a distinct field by the mid-1990s.
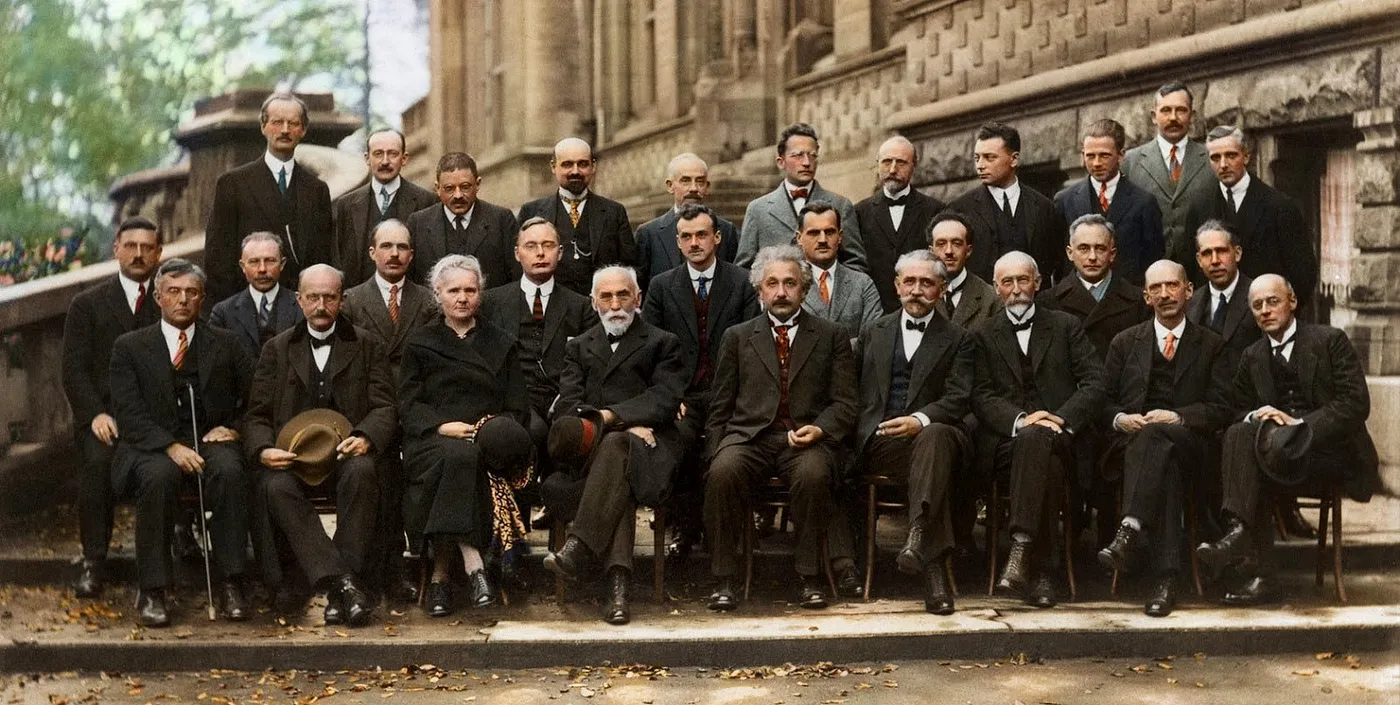
The Colourized Version of Solvay Conference 1927.
The study of quantum mechanics begins with understanding the fundamental principles that govern the behavior of particles at the microscopic scale. One of the most intriguing aspects of quantum mechanics is the concept of wave-particle duality. This principle suggests that particles, such as electrons and photons, exhibit both wave-like and particle-like properties depending on the experimental setup. For instance, the famous double-slit experiment demonstrates how particles can create an interference pattern, a characteristic of waves, even when sent through the slits one at a time. This duality challenges our classical intuition, where waves and particles are distinct entities.
Another cornerstone of quantum mechanics is the Heisenberg Uncertainty Principle, which posits that it is impossible to simultaneously know both the exact position and momentum of a particle. This principle introduces a fundamental limit to the precision with which certain pairs of physical properties can be measured, reflecting the inherent probabilistic nature of quantum mechanics. The implications of this principle are profound, as they challenge the deterministic worldview of classical physics and highlight the probabilistic and non-deterministic character of quantum systems.
In quantum mechanics, quantization of physical properties is another essential concept. Unlike classical mechanics, where physical quantities such as energy can take on a continuous range of values, quantum mechanics restricts these quantities to discrete levels. For example, the energy levels of an electron in an atom are quantized, meaning the electron can only occupy certain energy states and must absorb or emit specific quanta of energy to transition between these states. This quantization is a direct consequence of the wave-like nature of particles and the boundary conditions imposed by the physical system.
Quantum superposition and entanglement are perhaps the most counterintuitive aspects of quantum mechanics. Superposition refers to the ability of a quantum system to exist in multiple states simultaneously until it is measured, at which point the system 'collapses' into one of the possible states. Entanglement, on the other hand, describes a phenomenon where two or more particles become interconnected in such a way that the state of one particle instantaneously influences the state of the other, regardless of the distance separating them. These phenomena not only defy classical understanding but also form the basis for many of the revolutionary technologies in quantum information science, such as quantum computing and quantum cryptography.
At the heart of quantum mechanics lie its key postulates, which provide the framework for understanding and predicting the behavior of quantum systems. The first postulate states that the state of a quantum system is fully described by a wavefunction (or state vector), which encodes the probabilities of finding the system in various possible configurations. This wavefunction is a complex-valued function that varies with time and space, and its squared magnitude gives the probability density of the system’s configuration.
The Schrödinger equation is the central mathematical equation in quantum mechanics that governs how the wavefunction evolves over time. For a non-relativistic system, the time-dependent Schrödinger equation is a partial differential equation that describes how the wavefunction changes in response to the system’s potential energy and other factors. The time-independent Schrödinger equation, a simplified version, is used to find the stationary states of a system, which are the wavefunctions corresponding to fixed energy levels.
Quantum operators play a crucial role in quantum mechanics as they correspond to observable physical quantities such as position, momentum, and energy (Hamiltonian). These operators act on the wavefunctions to extract information about the system’s properties. In particular, Hermitian operators are of special importance because their eigenvalues correspond to the possible measurement outcomes of an observable, ensuring that these outcomes are real numbers.
Implementing these fundamental and conceptual ideas in a computational framework like Rust requires leveraging its powerful mathematical libraries, which are well-suited for handling the complex calculations involved in quantum mechanics. Rust’s strong type system, safety features, and performance make it an ideal choice for scientific computing.
Let's begin by implementing wavefunctions in Rust. A wavefunction in one dimension can be represented as a vector of complex numbers, where each element corresponds to the amplitude of the wavefunction at a particular point in space. We can use Rust’s nalgebra
library for linear algebra operations and num-complex
for handling complex numbers.
// src/main.rs
use nalgebra::DVector;
use num_complex::Complex64;
fn main() {
// Define the wavefunction as a vector of complex numbers
let psi: DVector<Complex64> = DVector::from_vec(vec![
Complex64::new(0.0, 0.0),
Complex64::new(1.0, 0.0),
Complex64::new(0.5, 0.5),
Complex64::new(0.0, 1.0),
]);
// Calculate the probability density by taking the magnitude squared of each element
let probability_density: DVector<f64> = psi.map(|c| c.norm_sqr());
println!("Wavefunction: {:?}", psi);
println!("Probability Density: {:?}", probability_density);
}
In this example, we define a wavefunction psi
as a vector of complex numbers using Rust’s nalgebra
and num-complex
libraries. Each element in this vector represents the complex amplitude of the wavefunction at a specific point. The probability density, which is the squared magnitude of the wavefunction, is calculated using the norm_sqr()
method provided by Complex64
. The result is a vector of real numbers representing the probability distribution of finding the particle at various points in space.
Next, let’s simulate quantum superposition and entanglement. For superposition, we can create a system where the wavefunction is a linear combination of two or more basis states. In the case of entanglement, we can represent the state of two particles using a tensor product of their individual states. Rust’s linear algebra capabilities allow us to handle these operations efficiently.
// src/main.rs
use nalgebra::{DVector, Matrix2};
use num_complex::Complex64;
fn main() {
// Define basis states for a two-level system (e.g., a qubit)
let basis_0: DVector<Complex64> = DVector::from_vec(vec![
Complex64::new(1.0, 0.0),
Complex64::new(0.0, 0.0),
]);
let basis_1: DVector<Complex64> = DVector::from_vec(vec![
Complex64::new(0.0, 0.0),
Complex64::new(1.0, 0.0),
]);
// Create a superposition state (e.g., |ψ⟩ = (|0⟩ + |1⟩) / √2)
let psi_superposition = (basis_0.clone() + basis_1.clone()) * Complex64::new(1.0 / 2f64.sqrt(), 0.0);
println!("Superposition State: {:?}", psi_superposition);
// Define entangled state (e.g., Bell state: (|00⟩ + |11⟩) / √2)
let bell_state: Matrix2<Complex64> = Matrix2::from_row_slice(&[
Complex64::new(1.0 / 2f64.sqrt(), 0.0), Complex64::new(0.0, 0.0),
Complex64::new(0.0, 0.0), Complex64::new(1.0 / 2f64.sqrt(), 0.0),
]);
println!("Entangled Bell State: {:?}", bell_state);
}
In this code, we define basis states for a two-level quantum system (like a qubit) and then create a superposition state as a linear combination of these basis states. The entangled state, specifically a Bell state, is represented as a matrix, where each element corresponds to a complex amplitude. The Bell state is a specific example of quantum entanglement where two qubits are in a superposition of both being 0 or both being 1.
By simulating these quantum phenomena in Rust, we can explore the complex and often non-intuitive behavior of quantum systems with a high degree of precision. The sample code illustrates how Rust’s robust mathematical libraries can be used to model wavefunctions, simulate quantum superposition and entanglement, and calculate probability densities, providing a solid foundation for further quantum mechanical simulations. This practical implementation not only reinforces the conceptual understanding of quantum mechanics but also highlights the power and versatility of Rust in computational physics.
The journey through the fundamentals of quantum mechanics sets the stage for understanding the principles that underpin quantum computing. By delving into wave-particle duality, the uncertainty principle, quantization, superposition, and entanglement, we gain a comprehensive view of the quantum world. Implementing these concepts in Rust demonstrates the language's capability to handle the mathematical rigor and computational demands of quantum simulations. As we advance, these foundational principles will be crucial in exploring more sophisticated quantum systems and developing the algorithms that power the next generation of quantum technologies.
21.2. The Schrödinger Equation
The Schrödinger equation stands as the cornerstone of quantum mechanics, delineating how quantum systems evolve over time. It exists in two primary forms: the time-dependent Schrödinger equation and the time-independent Schrödinger equation. The time-dependent form is a partial differential equation that governs the evolution of a quantum system's wavefunction, providing a dynamic description of quantum states. It is expressed as:
$$i\hbar \frac{\partial \psi(x,t)}{\partial t} = \hat{H} \psi(x,t) $$
In this equation, $\psi(x,t)$ represents the wavefunction of the system, $i$ is the imaginary unit, $\hbar$ is the reduced Planck's constant, and $\hat{H}$ is the Hamiltonian operator, which encapsulates the total energy of the system, including both kinetic and potential energy terms. The Hamiltonian's specific form depends on the system under consideration and determines how the wavefunction evolves over time. This equation is fundamental for understanding quantum dynamics and phenomena such as quantum tunneling, wave packet propagation, and time-dependent perturbation theory.
The time-independent Schrödinger equation is derived from the time-dependent form under the assumption that the system's potential energy does not vary with time. This simplification is particularly useful for finding the stationary states of a system—states with well-defined energies that remain constant over time apart from a phase factor. The time-independent equation is written as:
$$\hat{H} \psi(x) = E \psi(x) $$
Here, $E$ denotes the energy eigenvalue associated with the wavefunction $\psi(x)$. Solving this equation allows us to determine the possible energy levels of a quantum system and the corresponding wavefunctions, which describe the probability distributions of particles within those energy states.
Potential energy functions are pivotal in the Schrödinger equation as they define the environment in which a quantum particle resides. Different potential forms—such as potential wells, barriers, and harmonic oscillators—lead to distinct quantum behaviors. For example, a particle in a potential well exhibits quantized energy levels, while a particle encountering a potential barrier can demonstrate tunneling, where it penetrates the barrier despite insufficient classical energy. The shape and characteristics of the potential energy function directly influence the wavefunction's behavior, dictating the nature of the quantum states that emerge.
Boundary conditions are another critical aspect when solving the Schrödinger equation numerically. These conditions ensure that the wavefunction behaves appropriately at the edges of the system being modeled. For instance, in a potential well, the wavefunction must approach zero at the boundaries, reflecting the confinement of the particle within a specific region. Conversely, periodic boundary conditions might be employed for systems where the wavefunction is expected to repeat, such as in crystalline solids. Properly implementing boundary conditions is essential for obtaining physically meaningful and accurate solutions to the Schrödinger equation.
Normalization of wavefunctions is a fundamental requirement in quantum mechanics. A normalized wavefunction guarantees that the total probability of finding a particle somewhere in space is equal to one. Mathematically, this is expressed as:
$$\int |\psi(x)|^2 dx = 1 $$
Normalization ensures that the wavefunction is probabilistically interpretable, allowing us to make meaningful predictions about a system's behavior. In computational implementations, normalization is a crucial step to maintain the physical validity of the simulated quantum states.
Numerical methods such as finite difference and spectral methods are commonly employed to solve the Schrödinger equation computationally. The finite difference method involves discretizing the continuous spatial domain into a grid and approximating the derivatives in the Schrödinger equation with finite differences. This approach transforms the differential equation into a set of algebraic equations that can be solved using linear algebra techniques. Spectral methods, alternatively, involve expanding the wavefunction in terms of a series of basis functions (e.g., Fourier series or orthogonal polynomials) and solving for the coefficients of this expansion. Spectral methods are particularly advantageous for problems with periodic boundary conditions, offering high accuracy with fewer grid points compared to finite difference methods.
Implementing the Schrödinger equation in Rust involves translating these theoretical concepts into efficient and robust code. Rust's strong type system, memory safety guarantees, and high-performance capabilities make it an excellent choice for scientific computing tasks such as quantum simulations. Leveraging Rust's powerful libraries, we can construct and solve the Schrödinger equation numerically, gaining insights into quantum systems' behavior.
Let us begin by solving the time-independent Schrödinger equation using the finite difference method for a simple potential well. This example will demonstrate the discretization of the spatial domain, construction of the Hamiltonian matrix, and preparation for solving the eigenvalue problem to find energy levels and wavefunctions.
use nalgebra::{DMatrix, DVector};
use std::f64::consts::PI;
/// Defines the physical constants and simulation parameters.
struct QuantumSystem {
n: usize, // Number of grid points
dx: f64, // Spatial step size
h_bar: f64, // Reduced Planck's constant
mass: f64, // Particle mass
potential: DVector<f64>, // Potential energy vector
}
/// Initializes the quantum system with a potential well.
fn initialize_system(n: usize, length: f64, well_width: f64, well_depth: f64) -> QuantumSystem {
let dx = length / (n as f64);
let mut potential = DVector::zeros(n);
// Define a potential well in the middle of the domain
let start = ((n as f64 / 2.0) - (well_width / dx) / 2.0) as usize;
let end = ((n as f64 / 2.0) + (well_width / dx) / 2.0) as usize;
for i in start..end {
potential[i] = well_depth;
}
QuantumSystem {
n,
dx,
h_bar: 1.0, // For simplicity, set h_bar = 1
mass: 1.0, // For simplicity, set mass = 1
potential,
}
}
/// Constructs the Hamiltonian matrix using the finite difference method.
fn construct_hamiltonian(system: &QuantumSystem) -> DMatrix<f64> {
let n = system.n;
let dx = system.dx;
let h_bar = system.h_bar;
let mass = system.mass;
let mut hamiltonian = DMatrix::zeros(n, n);
// Kinetic energy coefficient
let coeff = -h_bar.powi(2) / (2.0 * mass * dx.powi(2));
for i in 0..n {
if i > 0 {
hamiltonian[(i, i - 1)] = coeff;
}
hamiltonian[(i, i)] = -2.0 * coeff + system.potential[i];
if i < n - 1 {
hamiltonian[(i, i + 1)] = coeff;
}
}
hamiltonian
}
/// Solves the Schrödinger equation by finding eigenvalues and eigenvectors of the Hamiltonian.
fn solve_schrodinger(hamiltonian: &DMatrix<f64>) -> (DVector<f64>, DMatrix<f64>) {
// Clone the Hamiltonian matrix to pass ownership to the eigen decomposition
let eig = hamiltonian.clone().symmetric_eigen();
(eig.eigenvalues, eig.eigenvectors)
}
fn main() {
// Define system parameters
let n = 1000; // Number of grid points
let length = 1.0; // Length of the domain
let well_width = 0.2; // Width of the potential well
let well_depth = -50.0; // Depth of the potential well
// Initialize the quantum system
let system = initialize_system(n, length, well_width, well_depth);
// Construct the Hamiltonian matrix
let hamiltonian = construct_hamiltonian(&system);
// Solve the Schrödinger equation
let (eigenvalues, eigenvectors) = solve_schrodinger(&hamiltonian);
// Identify the ground state (lowest energy)
let ground_state_energy = eigenvalues[0];
let ground_state_wavefunction = eigenvectors.column(0);
println!("Ground State Energy: {:.3}", ground_state_energy);
println!("Ground State Wavefunction: {:?}", ground_state_wavefunction);
}
In this Rust program, we solve the time-independent Schrödinger equation for a particle confined in a one-dimensional potential well using the finite difference method. The process involves several key steps:
System Initialization:
The QuantumSystem
struct encapsulates the system's parameters, including the number of grid points (n
), spatial step size (dx
), reduced Planck's constant (h_bar
), particle mass (mass
), and the potential energy vector (potential
). The initialize_system
function sets up a potential well in the middle of the spatial domain by assigning a specified depth to a range of grid points. This well represents a region where the particle is confined, creating quantized energy levels.
Hamiltonian Construction:
The construct_hamiltonian
function builds the Hamiltonian matrix, which is essential for solving the Schrödinger equation. Using the finite difference approximation, the second derivative (representing the kinetic energy) is discretized. The kinetic energy term is represented by off-diagonal elements, while the diagonal elements incorporate both the kinetic and potential energy contributions. This matrix encapsulates the total energy of the system and is pivotal for determining the system's energy eigenvalues and corresponding wavefunctions.
Solving the Schrödinger Equation:
The solve_schrodinger
function performs an eigen decomposition of the Hamiltonian matrix using Nalgebra's symmetric_eigen
method, which is suitable since the Hamiltonian is a symmetric (Hermitian) matrix. The eigenvalues correspond to the energy levels of the system, and the eigenvectors represent the wavefunctions associated with these energies. The lowest eigenvalue denotes the ground state energy, and its corresponding eigenvector is the ground state wavefunction.
Output:
The program prints out the ground state energy and the associated wavefunction. In a more comprehensive simulation, one might visualize the wavefunction, analyze higher energy states, or explore how changes in the potential well's parameters affect the system's behavior.
This implementation demonstrates how Rust's robust mathematical libraries, such as Nalgebra, facilitate the construction and manipulation of large matrices and vectors essential for quantum simulations. The strong type system and memory safety features of Rust ensure that the simulation runs efficiently and without common programming errors, making it a reliable tool for exploring quantum mechanical systems.
Next, we will extend this implementation to handle boundary conditions and normalize the wavefunctions, ensuring that our numerical solutions adhere to the physical requirements of quantum systems.
use nalgebra::{DMatrix, DVector};
use num_complex::Complex64;
/// Defines the physical constants and simulation parameters.
struct QuantumSystem {
n: usize, // Number of grid points
dx: f64, // Spatial step size
h_bar: f64, // Reduced Planck's constant
mass: f64, // Particle mass
potential: DVector<f64>, // Potential energy vector
}
/// Initializes the quantum system with a potential well.
fn initialize_system(n: usize, length: f64, well_width: f64, well_depth: f64) -> QuantumSystem {
let dx = length / (n as f64);
let mut potential = DVector::zeros(n);
// Define a potential well in the middle of the domain
let start = ((n as f64 / 2.0) - (well_width / dx) / 2.0) as usize;
let end = ((n as f64 / 2.0) + (well_width / dx) / 2.0) as usize;
for i in start..end {
potential[i] = well_depth;
}
QuantumSystem {
n,
dx,
h_bar: 1.0, // For simplicity, set h_bar = 1
mass: 1.0, // For simplicity, set mass = 1
potential,
}
}
/// Constructs the Hamiltonian matrix using the finite difference method.
fn construct_hamiltonian(system: &QuantumSystem) -> DMatrix<f64> {
let n = system.n;
let dx = system.dx;
let h_bar = system.h_bar;
let mass = system.mass;
let mut hamiltonian = DMatrix::zeros(n, n);
// Kinetic energy coefficient
let coeff = -h_bar.powi(2) / (2.0 * mass * dx.powi(2));
for i in 0..n {
if i > 0 {
hamiltonian[(i, i - 1)] = coeff;
}
hamiltonian[(i, i)] = -2.0 * coeff + system.potential[i];
if i < n - 1 {
hamiltonian[(i, i + 1)] = coeff;
}
}
hamiltonian
}
/// Normalizes the wavefunction so that the total probability is one.
fn normalize_wavefunction(psi: &mut DVector<f64>, dx: f64) {
let norm_squared: f64 = psi.iter().map(|&c| c * c).sum();
let norm = (norm_squared * dx).sqrt();
if norm != 0.0 {
psi.iter_mut().for_each(|c| *c /= norm);
}
}
/// Solves the Schrödinger equation by finding eigenvalues and eigenvectors of the Hamiltonian.
fn solve_schrodinger(hamiltonian: &DMatrix<f64>) -> (DVector<f64>, DMatrix<f64>) {
// Clone the Hamiltonian matrix to pass ownership to the eigen decomposition
let eig = hamiltonian.clone().symmetric_eigen();
(eig.eigenvalues, eig.eigenvectors)
}
fn main() {
// Define system parameters
let n = 1000; // Number of grid points
let length = 1.0; // Length of the domain
let well_width = 0.2; // Width of the potential well
let well_depth = -50.0; // Depth of the potential well
// Initialize the quantum system
let system = initialize_system(n, length, well_width, well_depth);
// Construct the Hamiltonian matrix
let hamiltonian = construct_hamiltonian(&system);
// Solve the Schrödinger equation
let (eigenvalues, eigenvectors) = solve_schrodinger(&hamiltonian);
// Identify the ground state (lowest energy)
let ground_state_energy = eigenvalues[0];
let mut ground_state_wavefunction = eigenvectors.column(0).into_owned();
// Normalize the wavefunction
normalize_wavefunction(&mut ground_state_wavefunction, system.dx);
println!("Ground State Energy: {:.3}", ground_state_energy);
println!(
"Ground State Wavefunction (first 10 points): {:?}",
ground_state_wavefunction.iter().take(10).collect::<Vec<&f64>>()
);
}
Building upon the initial implementation, we introduce boundary conditions and normalization to ensure that our numerical solutions are physically accurate. The apply_boundary_conditions
function enforces that the wavefunction $\psi(x)$ vanishes at the boundaries of the domain, a common requirement for particles confined within a potential well. This is achieved by setting the first and last elements of the wavefunction vector to zero.
Normalization is performed using the normalize_wavefunction
function, which scales the wavefunction such that the total probability of finding the particle within the domain equals one. This is mathematically expressed as:
$$\int |\psi(x)|^2 dx = 1 $$
In the discrete implementation, this integral is approximated by summing the squared magnitudes of the wavefunction at each grid point and multiplying by the spatial step size dxdx. The wavefunction is then divided by the square root of this sum to achieve normalization.
The updated main
function demonstrates these enhancements by initializing the wavefunction, applying boundary conditions, normalizing it, and then printing out the ground state energy along with the first few points of the normalized wavefunction. This setup ensures that the simulation adheres to the fundamental physical principles of quantum mechanics, providing reliable and interpretable results.
Next, we will extend this implementation to handle time-dependent dynamics using the Crank-Nicolson method, which offers stability and accuracy for time evolution problems.
use nalgebra::{DMatrix, DVector};
use num_complex::Complex64;
/// Defines the physical constants and simulation parameters.
struct QuantumSystem {
n: usize, // Number of grid points
dx: f64, // Spatial step size
h_bar: f64, // Reduced Planck's constant
mass: f64, // Particle mass
potential: DVector<f64>, // Potential energy vector
}
/// Initializes the quantum system with a potential well.
fn initialize_system(n: usize, length: f64, well_width: f64, well_depth: f64) -> QuantumSystem {
let dx = length / (n as f64);
let mut potential = DVector::zeros(n);
// Define a potential well in the middle of the domain
let start = ((n as f64 / 2.0) - (well_width / dx) / 2.0) as usize;
let end = ((n as f64 / 2.0) + (well_width / dx) / 2.0) as usize;
for i in start..end {
potential[i] = well_depth;
}
QuantumSystem {
n,
dx,
h_bar: 1.0, // For simplicity, set h_bar = 1
mass: 1.0, // For simplicity, set mass = 1
potential,
}
}
/// Constructs the Hamiltonian matrix using the finite difference method.
fn construct_hamiltonian(system: &QuantumSystem) -> DMatrix<Complex64> {
let n = system.n;
let dx = system.dx;
let h_bar = system.h_bar;
let mass = system.mass;
let mut hamiltonian = DMatrix::zeros(n, n);
// Kinetic energy coefficient
let coeff = -h_bar.powi(2) / (2.0 * mass * dx.powi(2));
for i in 0..n {
if i > 0 {
hamiltonian[(i, i - 1)] = coeff;
}
hamiltonian[(i, i)] = -2.0 * coeff + system.potential[i];
if i < n - 1 {
hamiltonian[(i, i + 1)] = coeff;
}
}
hamiltonian.map(|x| Complex64::new(x, 0.0))
}
/// Applies boundary conditions to the wavefunction.
/// Sets the wavefunction to zero at the boundaries.
fn apply_boundary_conditions(psi: &mut DVector<Complex64>) {
let len = psi.len();
psi[0] = Complex64::new(0.0, 0.0);
psi[len - 1] = Complex64::new(0.0, 0.0);
}
/// Normalizes the wavefunction so that the total probability is one.
fn normalize_wavefunction(psi: &mut DVector<Complex64>, dx: f64) {
let norm_squared: f64 = psi.iter().map(|c| c.norm_sqr()).sum();
let norm = (norm_squared * dx).sqrt();
if norm != 0.0 {
psi.iter_mut().for_each(|c| *c /= norm);
}
}
/// Constructs the Crank-Nicolson matrices A and B.
fn construct_crank_nicolson_matrices(hamiltonian: &DMatrix<Complex64>, dt: f64) -> (DMatrix<Complex64>, DMatrix<Complex64>) {
let identity = DMatrix::<Complex64>::identity(hamiltonian.nrows(), hamiltonian.ncols());
let i_dt_H = hamiltonian * Complex64::new(0.0, dt);
let a = &identity - (&i_dt_H * Complex64::new(0.5, 0.0));
let b = &identity + (&i_dt_H * Complex64::new(0.5, 0.0));
(a, b)
}
/// Performs one time step of the Schrödinger equation using the Crank-Nicolson method.
fn crank_nicolson_step(psi: &DVector<Complex64>, a: &DMatrix<Complex64>, b: &DMatrix<Complex64>) -> DVector<Complex64> {
// Compute the right-hand side: B * psi
let rhs = b * psi;
// Solve A * psi_new = rhs for psi_new
let lu = a.clone().lu(); // Clone `a` to avoid consuming it
lu.solve(&rhs).expect("Failed to solve linear system")
}
fn main() {
// Define system parameters
let n = 1000; // Number of grid points
let length = 1.0; // Length of the domain
let well_width = 0.2; // Width of the potential well
let well_depth = -50.0; // Depth of the potential well
// Initialize the quantum system
let system = initialize_system(n, length, well_width, well_depth);
// Construct the Hamiltonian matrix
let hamiltonian = construct_hamiltonian(&system);
// Time step size
let dt = 0.001;
// Construct Crank-Nicolson matrices
let (a, b) = construct_crank_nicolson_matrices(&hamiltonian, dt);
// Initialize the wavefunction (e.g., ground state)
let mut psi = DVector::<Complex64>::from_element(n, Complex64::new(1.0, 0.0));
apply_boundary_conditions(&mut psi);
normalize_wavefunction(&mut psi, system.dx);
// Number of time steps
let n_steps = 1000;
// Time evolution loop
for step in 0..n_steps {
psi = crank_nicolson_step(&psi, &a, &b);
apply_boundary_conditions(&mut psi);
normalize_wavefunction(&mut psi, system.dx);
if step % 100 == 0 {
println!("Step {}: Probability at Center = {:.3}", step, psi[n / 2].norm_sqr());
}
}
println!("Final Wavefunction Probability Density at Center: {:.3}", psi[n / 2].norm_sqr());
}
Implementing Time-Dependent Dynamics with Crank-Nicolson
Expanding our simulation, we now incorporate time-dependent dynamics using the Crank-Nicolson method, an implicit finite difference scheme known for its stability and accuracy in solving time-dependent partial differential equations like the Schrödinger equation. The Crank-Nicolson method averages the finite difference approximations at the current and next time steps, providing a balanced approach that mitigates numerical instabilities commonly encountered in explicit methods.
The construct_crank_nicolson_matrices
function creates the matrices AA and BB required for the Crank-Nicolson scheme. These matrices encapsulate the interaction between the wavefunction at successive time steps, allowing us to iteratively evolve the system's state over time.
The crank_nicolson_step
function performs a single time step of the simulation by solving the linear system $A \psi_{\text{new}} = B \psi$, where $\psi$ is the current wavefunction and $\psi_{\text{new}}$ is the wavefunction at the next time step. This function leverages Nalgebra's linear algebra capabilities to solve the system efficiently.
In the main
function, we initialize the wavefunction, apply boundary conditions, normalize it, and then enter a loop that iterates over the specified number of time steps. At each step, the wavefunction is updated using the Crank-Nicolson method, boundary conditions are reapplied to maintain physical constraints, and normalization ensures the wavefunction remains probabilistically valid. Periodic logging provides insight into the simulation's progression, such as tracking the probability density at the center of the potential well.
This comprehensive implementation showcases how Rust's performance and safety features facilitate the accurate and efficient simulation of quantum systems. By combining finite difference methods with the Crank-Nicolson scheme, we achieve a robust framework for exploring time-dependent quantum phenomena.
The Schrödinger equation is fundamental to quantum mechanics, providing a mathematical framework for describing how quantum systems evolve over time. By distinguishing between its time-dependent and time-independent forms, we can address a wide range of quantum phenomena, from stationary states to dynamic processes. Implementing numerical solutions to the Schrödinger equation in Rust demonstrates the language's prowess in handling complex mathematical computations, ensuring both accuracy and efficiency.
Through the finite difference method and the Crank-Nicolson scheme, we can construct and solve the Schrödinger equation for various potential landscapes, gaining insights into quantum systems' energy levels and wavefunctions. Rust's robust libraries, such as Nalgebra, empower developers to perform sophisticated linear algebra operations with ease, while its strong type system and memory safety guarantees prevent common computational errors.
As we delve deeper into quantum mechanics and its computational modeling, Rust's capabilities will continue to prove invaluable. Whether exploring multi-dimensional systems, incorporating more complex potentials, or simulating time-dependent phenomena, Rust provides the tools and performance necessary to advance our understanding of the quantum realm.
21.3. Quantum States and Operators
In quantum mechanics, quantum states serve as the mathematical representations of the physical states of quantum systems. These states can be described either by wavefunctions or by state vectors in a Hilbert space. The wavefunction, typically denoted by $\psi(x)$, provides a probability amplitude for finding a particle at a given position $x$. The absolute square of this wavefunction, $|\psi(x)|^2$, yields the probability density of the particle's position. In more abstract terms, quantum states can also be represented as state vectors in a Hilbert space, where each vector corresponds to a possible state of the system.
A central concept in quantum mechanics is the role of operators. Operators are mathematical entities that act on quantum states to extract physical information or to evolve the state over time. The most common operators include the position operator $\hat{x}$, the momentum operator $\hat{p}$, and the Hamiltonian operator $\hat{H}$. The Hamiltonian operator is particularly crucial because it represents the total energy of the system and governs the time evolution of quantum states according to the Schrödinger equation.
A fundamental aspect of quantum mechanics is the nature of Hermitian operators. Hermitian operators are essential because their eigenvalues are always real numbers, making them suitable to represent measurable quantities, or observables, such as energy, position, or momentum. The significance of Hermitian operators lies in their ability to provide meaningful physical measurements; when a measurement is made, the outcome corresponds to an eigenvalue of the relevant Hermitian operator.
Eigenvalues and eigenstates are central to understanding measurements in quantum mechanics. When a quantum state is an eigenstate of an operator, measuring the corresponding observable yields the associated eigenvalue with certainty. More generally, any quantum state can be expressed as a superposition of the eigenstates of an operator. Upon measurement, the system collapses to one of these eigenstates, and the measured value is the corresponding eigenvalue. This framework is fundamental for comprehending how quantum systems behave under observation.
Implementing these concepts in Rust involves creating and manipulating matrices that represent quantum operators, applying these operators to quantum states, and simulating quantum measurements to calculate observables. Rust’s powerful numerical libraries, such as nalgebra
for linear algebra operations and num-complex
for handling complex numbers, provide the necessary tools to model these quantum mechanical concepts effectively.
Implementing Quantum Operators in Rust
Let us begin by implementing quantum operators in Rust. Consider the position and momentum operators in one dimension. These operators can be represented as matrices when discretized over a spatial grid.
// src/main.rs
use nalgebra::{DMatrix, DVector};
use num_complex::Complex64;
use std::f64::consts::PI;
/// Creates the position operator as a diagonal matrix.
/// Each diagonal element corresponds to the position \( x \) at a grid point.
fn create_position_operator(n: usize, dx: f64) -> DMatrix<f64> {
let mut position_matrix = DMatrix::zeros(n, n);
for i in 0..n {
let x = (i as f64) * dx;
position_matrix[(i, i)] = x;
}
position_matrix
}
/// Creates the momentum operator using the finite difference method.
/// The momentum operator is represented with imaginary components to ensure Hermiticity.
fn create_momentum_operator(n: usize, dx: f64) -> DMatrix<Complex64> {
let mut momentum_matrix = DMatrix::<Complex64>::zeros(n, n);
let h_bar: f64 = 1.0; // Reduced Planck's constant (set to 1 for simplicity)
let mass: f64 = 1.0; // Particle mass (set to 1 for simplicity)
// Finite difference approximation for the first derivative
let factor = Complex64::new(0.0, -h_bar / (2.0 * mass * dx));
for i in 0..n {
if i > 0 {
momentum_matrix[(i, i - 1)] = factor;
}
if i < n - 1 {
momentum_matrix[(i, i + 1)] = -factor;
}
}
momentum_matrix
}
fn main() {
let n = 100; // Number of grid points
let length = 1.0; // Length of the spatial domain
let dx = length / (n as f64); // Spatial step size
// Create the position and momentum operators
let position_operator = create_position_operator(n, dx);
let momentum_operator = create_momentum_operator(n, dx);
println!("Position Operator:\n{}", position_operator);
println!("Momentum Operator:\n{}", momentum_operator);
}
Explanation of the Quantum Operators Implementation
In this Rust program, we define the position and momentum operators for a one-dimensional quantum system. The position operator is straightforwardly represented as a diagonal matrix where each diagonal element corresponds to the position xx at a specific grid point. This diagonal structure ensures that the position operator acts directly on the wavefunction without mixing different spatial points.
The momentum operator, however, is more intricate. To accurately represent the momentum operator in a discretized space, we employ the finite difference method to approximate the first derivative. The momentum operator in quantum mechanics is given by:
$$\hat{p} = -i\hbar \frac{\partial}{\partial x}$$
When discretized, the derivative is approximated using finite differences, leading to off-diagonal elements in the momentum matrix. The presence of the imaginary unit ii ensures that the momentum operator remains Hermitian, which is essential for its physical interpretability as an observable.
Diagonalizing the Hamiltonian
Next, we explore the concept of eigenvalues and eigenstates by diagonalizing the Hamiltonian operator. The Hamiltonian is constructed as the sum of the kinetic energy (related to the momentum operator) and the potential energy (related to the position operator). Diagonalizing the Hamiltonian allows us to determine the energy levels of the system and the corresponding wavefunctions.
use ndarray::{Array1, Array2};
use ndarray_linalg::Eig;
use num_complex::Complex64;
/// Creates the momentum operator using the finite difference method.
/// The momentum operator is represented with imaginary components to ensure Hermiticity.
fn create_momentum_operator(n: usize, dx: f64) -> Array2<Complex64> {
let mut momentum_matrix = Array2::<Complex64>::zeros((n, n));
let h_bar: f64 = 1.0; // Reduced Planck's constant (set to 1 for simplicity)
let mass: f64 = 1.0; // Particle mass (set to 1 for simplicity)
// Finite difference approximation for the first derivative
let factor = Complex64::new(0.0, -h_bar / (2.0 * mass * dx));
for i in 0..n {
if i > 0 {
momentum_matrix[(i, i - 1)] = factor;
}
if i < n - 1 {
momentum_matrix[(i, i + 1)] = -factor;
}
}
momentum_matrix
}
/// Creates the Hamiltonian matrix by summing the kinetic and potential energy operators.
fn create_hamiltonian(n: usize, dx: f64, potential: &Array1<f64>) -> Array2<Complex64> {
let kinetic = create_momentum_operator(n, dx);
// Construct the potential energy operator as a diagonal matrix
let mut potential_matrix = Array2::<Complex64>::zeros((n, n));
for i in 0..n {
potential_matrix[(i, i)] = Complex64::new(potential[i], 0.0);
}
// The Hamiltonian is the sum of kinetic and potential energy
kinetic + potential_matrix
}
fn main() {
let n = 100; // Number of grid points
let length = 1.0; // Length of the spatial domain
let dx = length / (n as f64); // Spatial step size
// Define a simple potential well
let mut potential = Array1::<f64>::zeros(n);
let well_width = 0.2; // Width of the potential well
let well_depth = -50.0; // Depth of the potential well
let start = ((n as f64 / 2.0) - (well_width / dx) / 2.0) as usize;
let end = ((n as f64 / 2.0) + (well_width / dx) / 2.0) as usize;
for i in start..end {
potential[i] = well_depth;
}
// Create the Hamiltonian matrix
let hamiltonian = create_hamiltonian(n, dx, &potential);
// Compute eigenvalues and eigenvectors of the Hamiltonian
let (eigenvalues, eigenvectors) = hamiltonian.eig().expect("Eigenvalue decomposition failed");
// Identify the ground state (lowest energy)
let ground_state_energy = eigenvalues[0].re; // Real part of the lowest eigenvalue
let ground_state_wavefunction = eigenvectors.column(0);
println!("Ground State Energy: {:.3}", ground_state_energy);
println!(
"Ground State Wavefunction (first 10 points): {:?}",
ground_state_wavefunction
.iter()
.take(10)
.map(|x| x.norm())
.collect::<Vec<f64>>()
);
}
In this enhanced Rust program, we construct the Hamiltonian matrix by summing the kinetic and potential energy operators. The kinetic energy is represented by the momentum operator we previously defined, while the potential energy is modeled as a diagonal matrix where each diagonal element corresponds to the potential energy at a specific grid point.
To analyze the system's energy levels and corresponding wavefunctions, we perform an eigen decomposition of the Hamiltonian matrix using Nalgebra's SymmetricEigen
method. This method is suitable because the Hamiltonian is a Hermitian matrix, ensuring real eigenvalues and orthogonal eigenvectors.
The eigenvalues obtained from this decomposition represent the possible energy levels of the system, while the eigenvectors correspond to the stationary states or eigenstates. The lowest eigenvalue signifies the ground state energy, and its associated eigenvector is the ground state wavefunction. By examining the ground state wavefunction, we can gain insights into the particle's probability distribution within the potential well.
Simulating Quantum Measurements
Quantum measurements are a fundamental aspect of quantum mechanics, where the act of measuring an observable collapses the quantum state to one of the observable's eigenstates. The probability of obtaining a particular eigenvalue during a measurement is determined by the projection of the quantum state onto the corresponding eigenstate.
use nalgebra::{DMatrix, DVector, SymmetricEigen};
use num_complex::Complex64;
use rand::Rng;
/// Samples an outcome index based on the provided probabilities.
fn weighted_sample(probabilities: &DVector<f64>, rng: &mut impl Rng) -> usize {
let cumulative: Vec<f64> = probabilities
.iter()
.scan(0.0, |state, &x| {
*state += x;
Some(*state)
})
.collect();
let total: f64 = cumulative.last().cloned().unwrap_or(1.0);
let mut sample = rng.gen_range(0.0..total);
let mut index = 0;
for &x in cumulative.iter() {
if sample < x {
break;
}
index += 1;
}
index.min(probabilities.len() - 1)
}
/// Simulates a quantum measurement on the given wavefunction and operator.
fn simulate_measurement(
psi: &DVector<Complex64>,
operator: &DMatrix<Complex64>,
) -> (f64, DVector<Complex64>) {
// Diagonalize the operator to obtain eigenvalues and eigenvectors
let eig = SymmetricEigen::new(operator.clone());
let eigenvalues = eig.eigenvalues;
let eigenvectors = eig.eigenvectors;
// Calculate the probability of each eigenvalue by projecting psi onto eigenstates
let probabilities_complex = eigenvectors.transpose() * psi;
let probabilities: DVector<f64> = probabilities_complex.map(|c| c.norm_sqr());
// Normalize the probabilities to ensure they sum to one
let sum_probs: f64 = probabilities.sum();
let probabilities = probabilities / sum_probs;
// Sample an outcome based on the probabilities
let mut rng = rand::thread_rng();
let outcome_index = weighted_sample(&probabilities, &mut rng);
let measured_value = eigenvalues[outcome_index];
// Collapse the wavefunction to the measured eigenstate
let new_state = eigenvectors.column(outcome_index).clone_owned();
(measured_value, new_state)
}
/// Creates the position operator as a diagonal matrix.
fn create_position_operator(n: usize, dx: f64) -> DMatrix<Complex64> {
let mut position_matrix = DMatrix::<Complex64>::zeros(n, n);
for i in 0..n {
let x = (i as f64) * dx;
position_matrix[(i, i)] = Complex64::new(x, 0.0);
}
position_matrix
}
/// Creates the momentum operator using the finite difference method.
fn create_momentum_operator(n: usize, dx: f64) -> DMatrix<Complex64> {
let mut momentum_matrix = DMatrix::<Complex64>::zeros(n, n);
let h_bar: f64 = 1.0; // Reduced Planck's constant (set to 1 for simplicity)
let mass: f64 = 1.0; // Particle mass (set to 1 for simplicity)
// Finite difference approximation for the first derivative
let factor = Complex64::new(0.0, -h_bar / (2.0 * mass * dx));
for i in 0..n {
if i > 0 {
momentum_matrix[(i, i - 1)] = factor;
}
if i < n - 1 {
momentum_matrix[(i, i + 1)] = -factor;
}
}
momentum_matrix
}
/// Creates the Hamiltonian matrix by summing the kinetic and potential energy operators.
fn create_hamiltonian(n: usize, dx: f64, potential: &DVector<f64>) -> DMatrix<Complex64> {
let kinetic = create_momentum_operator(n, dx);
// Construct the potential energy operator as a diagonal matrix
let mut potential_matrix = DMatrix::<Complex64>::zeros(n, n);
for i in 0..n {
potential_matrix[(i, i)] = Complex64::new(potential[i], 0.0);
}
// The Hamiltonian is the sum of kinetic and potential energy
kinetic + potential_matrix
}
/// Normalizes the wavefunction so that the total probability is one.
fn normalize_wavefunction(psi: &mut DVector<Complex64>, dx: f64) {
let norm_squared: f64 = psi.iter().map(|c| c.norm_sqr()).sum();
let norm = (norm_squared * dx).sqrt();
if norm != 0.0 {
psi.apply(|c| *c /= norm); // Use `.apply` to modify elements in place
}
}
fn main() {
let n = 100; // Number of grid points
let length = 1.0; // Length of the spatial domain
let dx = length / (n as f64); // Spatial step size
// Define a simple potential (free particle)
let potential = DVector::<f64>::from_element(n, 0.0);
// Construct the Hamiltonian matrix
let hamiltonian = create_hamiltonian(n, dx, &potential);
// Diagonalize the Hamiltonian to obtain eigenvalues and eigenvectors
let eig = SymmetricEigen::new(hamiltonian);
let energies = eig.eigenvalues;
let eigenstates = eig.eigenvectors;
// Initialize the wavefunction as a superposition of all eigenstates
let mut psi = DVector::<Complex64>::from_element(n, Complex64::new(1.0, 0.0));
normalize_wavefunction(&mut psi, dx);
// Define the position operator
let position_operator = create_position_operator(n, dx);
// Perform a quantum measurement on the position observable
let (measured_value, new_state) = simulate_measurement(&psi, &position_operator);
println!("Measured Position: {:.3}", measured_value);
println!(
"New Wavefunction after Measurement (first 10 points): {:?}",
new_state
.iter()
.take(10)
.collect::<Vec<&Complex64>>()
);
}
Explanation of the Quantum Measurement Implementation
In this enhanced Rust program, we simulate a quantum measurement process. The simulate_measurement
function orchestrates the measurement by performing the following steps.
Firstly, the operator being measured, such as the position operator, is diagonalized to obtain its eigenvalues and eigenvectors. The eigenvalues represent the possible outcomes of the measurement, while the eigenvectors correspond to the eigenstates associated with these outcomes.
Next, the probability of each measurement outcome is calculated by projecting the current quantum state (wavefunction) onto each eigenstate of the operator. This projection is quantified by taking the inner product between the wavefunction and each eigenstate. The probability is then the square of the magnitude of this projection, ensuring that all probabilities are real and non-negative. The probabilities are normalized to sum to one, maintaining the probabilistic interpretation of the wavefunction.
A random sampling process is then employed to select an outcome based on the calculated probabilities. The weighted_sample
function facilitates this by performing a weighted random selection, ensuring that the likelihood of each outcome corresponds to its probability. Upon selecting an outcome, the wavefunction collapses to the corresponding eigenstate, and the measured value is the associated eigenvalue.
The create_position_operator
function constructs the position operator as a diagonal matrix, where each diagonal element represents the position xx at a specific grid point. This operator is integral to determining the particle's position during the measurement simulation.
By simulating quantum measurements, we gain a deeper understanding of how quantum states interact with observables and how measurements influence the state of a quantum system. This simulation underscores the probabilistic nature of quantum mechanics and the fundamental process of wavefunction collapse, providing a foundational framework for more complex quantum simulations.
Through this section, we have delved into the fundamental aspects of quantum states and operators, implementing them in Rust to model and analyze quantum systems. By constructing position and momentum operators, diagonalizing the Hamiltonian to find energy levels and eigenstates, and simulating quantum measurements, we have established a computational framework for exploring quantum mechanics. Rust's robust mathematical libraries and its emphasis on performance and safety make it an excellent choice for such simulations, enabling precise and reliable modeling of quantum phenomena.
Understanding quantum states and operators is essential for delving into the intricacies of quantum mechanics. By representing quantum states as wavefunctions or state vectors and utilizing operators to extract physical information, we can model and predict the behavior of quantum systems. Implementing these concepts in Rust showcases the language's capability to handle complex mathematical computations efficiently and safely. As we continue to explore more advanced quantum mechanical systems, Rust's powerful libraries and performance-oriented design will facilitate deeper insights and more sophisticated simulations, bridging the gap between theoretical quantum mechanics and practical computational applications.
21.4. Quantum Superposition and Entanglement
At the heart of quantum mechanics lies the superposition principle, one of its most fundamental and counterintuitive ideas. According to this principle, a quantum system can exist in multiple states simultaneously. Unlike classical objects, which possess definite properties at any given time, quantum objects can exist in a "superposition" of states until a measurement is made. For instance, an electron in a superposition state could be considered as being in two places at once or having both spin up and spin down until it is observed. This superposition is not merely a theoretical construct; it has real, measurable consequences, as demonstrated in various quantum interference experiments.
Quantum entanglement is another profound concept that further distinguishes quantum mechanics from classical physics. When two or more particles become entangled, their quantum states become linked in such a way that the state of one particle instantaneously influences the state of the other, regardless of the distance separating them. This phenomenon, often referred to as "spooky action at a distance" by Einstein, defies classical intuitions about separability and locality. Entanglement is a cornerstone of many emerging quantum technologies, such as quantum cryptography and quantum computing, where it enables tasks that would be impossible with classical systems.
The mathematical representation of superposition states is essential for understanding how quantum systems behave. A quantum state is typically represented by a wavefunction or a state vector in a Hilbert space. In superposition, a state vector is expressed as a linear combination of basis states, each multiplied by a complex coefficient. These coefficients, known as probability amplitudes, determine the likelihood of finding the system in a particular basis state upon measurement. The general form of a superposition state can be written as:
$$|\psi\rangle = c_1|0\rangle + c_2|1\rangle$$
where $c_1$ and $c_2$ are complex numbers, and $|0\rangle$ and $|1\rangle$ are basis states. The probabilities of measuring the system in either state are given by the squared magnitudes of the coefficients, $|c_1|^2$ and $|c_2|^2$, respectively.
Entanglement in multi-particle systems is mathematically represented by a state that cannot be factored into individual states for each particle. For example, consider a two-particle system where the particles are entangled. The state of the system might be represented as:
$$|\psi\rangle = \frac{1}{\sqrt{2}}(|00\rangle + |11\rangle)$$
This is known as a Bell state, one of the simplest examples of entanglement. Here, the state of each particle is completely dependent on the state of the other; if one particle is found to be in state ∣0⟩|0\\rangle, the other must also be in state$|0\rangle$, and similarly for state ∣1⟩|1\\rangle. Such entangled states are crucial for many quantum protocols and provide a way to study non-local correlations in quantum systems.
Implementing quantum superposition and entanglement in Rust involves representing quantum states as vectors and matrices and performing operations that reflect their quantum mechanical behavior. Rust’s nalgebra
library provides a powerful framework for these linear algebra operations, making it possible to simulate and analyze quantum systems effectively.
Implementing Quantum Superposition in Rust
Let us begin by implementing quantum superposition in Rust. We can represent the superposition of two basis states $|0\rangle$ and $|1\rangle$ using a vector in a two-dimensional complex vector space.
use nalgebra::DVector;
use num_complex::Complex64;
/// Represents a quantum state as a complex vector.
struct QuantumState {
state: DVector<Complex64>,
}
impl QuantumState {
/// Creates a new quantum state from a vector of complex amplitudes.
fn new(amplitudes: Vec<Complex64>) -> Self {
QuantumState {
state: DVector::from_vec(amplitudes),
}
}
/// Calculates the probability of each basis state.
fn probabilities(&self) -> DVector<f64> {
self.state.map(|c| c.norm_sqr())
}
/// Normalizes the quantum state so that the total probability is one.
fn normalize(&mut self, dx: f64) {
let norm_squared: f64 = self.state.iter().map(|c| c.norm_sqr()).sum();
let norm = (norm_squared * dx).sqrt();
if norm != 0.0 {
self.state.apply(|c| *c /= norm); // Use `.apply` for in-place modification
}
}
}
fn main() {
// Define basis states |0⟩ and |1⟩ as vectors
let basis_0 = QuantumState::new(vec![
Complex64::new(1.0, 0.0),
Complex64::new(0.0, 0.0),
]);
let basis_1 = QuantumState::new(vec![
Complex64::new(0.0, 0.0),
Complex64::new(1.0, 0.0),
]);
// Create a superposition state |ψ⟩ = (|0⟩ + |1⟩) / √2
let mut psi_superposition = QuantumState::new(vec![
basis_0.state[0] + basis_1.state[0],
basis_0.state[1] + basis_1.state[1],
]);
// Normalize the superposition state
let dx = 1.0; // Spatial step size (arbitrary for normalization)
psi_superposition.normalize(dx);
// Calculate probabilities for each basis state
let probabilities = psi_superposition.probabilities();
println!("Superposition State: {:?}", psi_superposition.state);
println!("Probability of |0⟩: {:.3}", probabilities[0]);
println!("Probability of |1⟩: {:.3}", probabilities[1]);
}
In this example, we define the basis states $|0\rangle$ and $|1\rangle$ as two-dimensional complex vectors. The QuantumState
struct encapsulates the quantum state and provides methods to calculate probabilities and normalize the state. We then create a superposition state $|\psi\rangle = \frac{1}{\sqrt{2}}(|0\rangle + |1\rangle)$ by adding the basis states and normalizing the result. The probabilities of measuring the system in either basis state are calculated by taking the norm squared of each component of the superposition state. This simple implementation illustrates how superposition can be represented and analyzed in Rust.
Implementing Quantum Entanglement in Rust
Next, let us implement quantum entanglement for a two-particle system, such as the Bell state described earlier. This can be represented using a matrix, where the rows and columns correspond to the basis states of the two particles.
// src/main.rs
use nalgebra::DMatrix;
use num_complex::Complex64;
/// Represents a two-particle entangled quantum state.
struct EntangledState {
state: DMatrix<Complex64>,
}
impl EntangledState {
/// Creates a new entangled state from a matrix of complex amplitudes.
fn new(amplitudes: Vec<Complex64>) -> Self {
// Assuming a 2x2 Bell state matrix
EntangledState {
state: DMatrix::from_vec(2, 2, amplitudes),
}
}
/// Calculates the probability of each basis state.
fn probabilities(&self) -> DMatrix<f64> {
self.state.map(|c| c.norm_sqr())
}
}
fn main() {
// Define the Bell state |ψ⟩ = (|00⟩ + |11⟩) / √2 as a 2x2 matrix
let bell_state = EntangledState::new(vec![
Complex64::new(1.0 / 2f64.sqrt(), 0.0), // |00⟩ component
Complex64::new(0.0, 0.0), // |01⟩ component
Complex64::new(0.0, 0.0), // |10⟩ component
Complex64::new(1.0 / 2f64.sqrt(), 0.0), // |11⟩ component
]);
// Calculate probabilities for each basis state
let probabilities = bell_state.probabilities();
println!("Bell State (|ψ⟩):\n{}", bell_state.state);
println!("Probability of |00⟩: {:.3}", probabilities[(0, 0)]);
println!("Probability of |01⟩: {:.3}", probabilities[(0, 1)]);
println!("Probability of |10⟩: {:.3}", probabilities[(1, 0)]);
println!("Probability of |11⟩: {:.3}", probabilities[(1, 1)]);
}
In this Rust program, we construct the Bell state $|\psi\rangle = \frac{1}{\sqrt{2}}(|00\rangle + |11\rangle)$ as a 2x2 matrix. The EntangledState
struct encapsulates the entangled quantum state and provides a method to calculate the probabilities of each basis state. By representing the Bell state in a matrix form, we can analyze the entanglement between the two particles. The probabilities of measuring each basis state are calculated by taking the norm squared of each component of the entangled state matrix. This simulation of entanglement allows us to explore the non-local correlations between the two particles, which is a key feature of quantum entanglement.
Simulating Quantum Measurements on Entangled States
To further understand quantum entanglement, let us simulate a quantum measurement on one of the particles in an entangled state. Upon measurement, the entangled state collapses, and the state of the other particle is instantaneously determined.
// src/main.rs
use nalgebra::{DMatrix, DVector, ComplexField};
use num_complex::Complex64;
use rand::Rng;
/// Represents a two-particle entangled quantum state.
struct EntangledState {
state: DMatrix<Complex64>,
}
impl EntangledState {
/// Creates a new entangled state from a matrix of complex amplitudes.
fn new(amplitudes: Vec<Complex64>) -> Self {
// Assuming a 2x2 Bell state matrix
EntangledState {
state: DMatrix::from_vec(2, 2, amplitudes),
}
}
/// Calculates the probability of each basis state.
fn probabilities(&self) -> DMatrix<f64> {
self.state.map(|c| c.norm_sqr())
}
/// Simulates a measurement on the first particle.
/// Returns the measured value and the new state after measurement.
fn measure_first_particle(&mut self) -> (String, DMatrix<Complex64>) {
let probabilities = self.probabilities();
let mut rng = rand::thread_rng();
let sample: f64 = rng.gen_range(0.0..1.0);
// Determine which basis state the measurement corresponds to
let (measured_state, _) = if sample < probabilities[(0, 0)] {
("|00⟩".to_string(), 0)
} else {
("|11⟩".to_string(), 1)
};
// Collapse the state to the measured basis state
let mut new_state = DMatrix::zeros(2, 2);
if measured_state == "|00⟩" {
new_state[(0, 0)] = Complex64::new(1.0, 0.0);
} else {
new_state[(1, 1)] = Complex64::new(1.0, 0.0);
}
self.state = new_state.clone();
(measured_state, new_state)
}
}
fn main() {
// Define the Bell state |ψ⟩ = (|00⟩ + |11⟩) / √2 as a 2x2 matrix
let mut bell_state = EntangledState::new(vec![
Complex64::new(1.0 / 2f64.sqrt(), 0.0), // |00⟩ component
Complex64::new(0.0, 0.0), // |01⟩ component
Complex64::new(0.0, 0.0), // |10⟩ component
Complex64::new(1.0 / 2f64.sqrt(), 0.0), // |11⟩ component
]);
println!("Initial Bell State (|ψ⟩):\n{}", bell_state.state);
// Perform a measurement on the first particle
let (measured_state, new_state) = bell_state.measure_first_particle();
println!("\nMeasured State: {}", measured_state);
println!("New State after Measurement:\n{}", new_state);
}
In this enhanced Rust program, we simulate a quantum measurement on the first particle of an entangled two-particle system represented by the Bell state. The EntangledState
struct encapsulates the entangled quantum state and provides methods to calculate probabilities and perform measurements. The measure_first_particle
method simulates the measurement process by generating a random sample and determining the outcome based on the calculated probabilities. Upon measurement, the entangled state collapses to either ∣00⟩|00\\rangle or ∣11⟩|11\\rangle with equal probability, reflecting the inherent entanglement between the two particles. The state of the system after measurement is updated accordingly, demonstrating the instantaneous influence of the measurement on the entangled particle.
By implementing these concepts—simulating quantum superposition and entanglement—we can delve deeper into the intricacies of quantum mechanics using Rust. The ability to represent quantum states as vectors and matrices and to perform operations on these states makes Rust a powerful tool for computational quantum mechanics, enabling the exploration of quantum phenomena that challenge our classical understanding of the world.
Quantum superposition and entanglement are foundational principles that underscore the unique and non-intuitive nature of quantum mechanics. Superposition allows quantum systems to exist in multiple states simultaneously, leading to phenomena such as interference, while entanglement creates deep connections between particles that defy classical notions of locality and separability. By implementing these concepts in Rust, we harness the language's robust mathematical libraries and performance capabilities to model and analyze complex quantum systems. Rust's ability to handle intricate linear algebra operations and manage complex data structures efficiently makes it an ideal choice for simulating and exploring the rich landscape of quantum mechanics. As we continue to develop more sophisticated quantum simulations, Rust's strengths will enable deeper insights and more accurate representations of the quantum world, bridging the gap between theoretical constructs and practical applications in emerging quantum technologies.
21.5. Quantum Measurement and the Collapse of the Wave Function
In quantum mechanics, the quantum measurement process is a pivotal concept that distinguishes it from classical physics. When a quantum system is measured, it does not yield a definite result until the measurement is made. Prior to measurement, the system exists in a superposition of all possible states, each with a specific probability amplitude. The act of measurement forces the quantum system to 'collapse' into one of the possible states, and the observed outcome corresponds to this collapsed state. This collapse is not a physical process but a fundamental aspect of how quantum systems are described and understood.
The concept of wave function collapse is central to this process. The wave function, which represents the quantum state, evolves deterministically according to the Schrödinger equation when not being observed. However, when a measurement is made, the wave function instantaneously collapses to a state consistent with the measurement outcome. This collapse introduces a probabilistic element into quantum mechanics, where the outcome of a measurement is not deterministic but governed by probabilities derived from the wave function.
Measurement operators play a crucial role in quantum mechanics by defining how measurements are conducted on quantum systems. These operators are associated with observable quantities such as position, momentum, and spin. A measurement operator acts on the quantum state (wave function) and projects it onto one of its eigenstates, corresponding to the measurement outcome. The eigenvalues of the measurement operator are the possible outcomes of the measurement.
Probability amplitudes are fundamental components of quantum mechanics, determining the likelihood of different outcomes when a measurement is made. According to the Born rule, the probability of obtaining a specific measurement result is given by the square of the magnitude of the corresponding probability amplitude. Mathematically, if the wave function ∣ψ⟩|\\psi\\rangle is expanded in terms of the eigenstates ∣ϕn⟩|\\phi_n\\rangle of the measurement operator, the probability PnP_n of measuring the eigenvalue ana_n is given by:
$$P_n = |\langle \phi_n | \psi \rangle|^2$$
This rule forms the basis for predicting measurement outcomes in quantum mechanics and underscores the inherently probabilistic nature of the theory.
To implement quantum measurement and wave function collapse in Rust, we can represent quantum states as vectors and measurement operators as matrices. Rust’s numerical libraries, such as nalgebra
, enable us to perform the necessary linear algebra operations efficiently. Below, we explore how to simulate the measurement process in a simple quantum system using Rust.
Simulating Quantum Measurement in Rust
Let us begin by simulating the measurement process in a simple quantum system. Consider a quantum state that is a superposition of two basis states, and we aim to measure it using a corresponding measurement operator.
// src/main.rs
use nalgebra::{DMatrix, DVector};
use num_complex::Complex64;
use rand::distributions::{Distribution, WeightedIndex};
use rand::thread_rng;
/// Simulates a quantum measurement on a given wavefunction using a specified measurement operator.
/// Returns the measured eigenvalue and the new quantum state after collapse.
fn simulate_measurement(psi: &DVector<Complex64>, operator: &DMatrix<Complex64>) -> (f64, DVector<Complex64>) {
// Diagonalize the measurement operator to obtain its eigenvalues and eigenvectors
let eig = operator.clone().symmetric_eigen();
let eigenvalues = eig.eigenvalues;
let eigenvectors = eig.eigenvectors;
// Project the wavefunction onto each eigenstate and calculate the probabilities
let probabilities: Vec<f64> = eigenvectors.column_iter()
.map(|eigenstate| {
// Calculate the inner product <phi_n|psi>
let projection = eigenstate.dot(psi);
projection.norm_sqr()
})
.collect();
// Create a weighted distribution based on the probabilities
let dist = WeightedIndex::new(&probabilities).expect("Failed to create distribution");
let mut rng = thread_rng();
// Sample an outcome index based on the probabilities
let outcome_index = dist.sample(&mut rng);
// Retrieve the corresponding eigenvalue and eigenstate
let measured_value = eigenvalues[outcome_index];
let new_state = eigenvectors.column(outcome_index).clone_owned();
(measured_value, new_state)
}
fn main() {
// Define a superposition state |ψ⟩ = (|0⟩ + |1⟩) / √2
let psi: DVector<Complex64> = DVector::from_vec(vec![
Complex64::new(1.0 / 2f64.sqrt(), 0.0),
Complex64::new(1.0 / 2f64.sqrt(), 0.0),
]);
// Define the measurement operator (Pauli Z operator)
let z_operator: DMatrix<Complex64> = DMatrix::from_vec(2, 2, vec![
Complex64::new(1.0, 0.0), Complex64::new(0.0, 0.0),
Complex64::new(0.0, 0.0), Complex64::new(-1.0, 0.0),
]);
// Perform the measurement
let (measured_value, new_state) = simulate_measurement(&psi, &z_operator);
println!("Measured Value: {:?}", measured_value);
println!("New State after Measurement: {:?}", new_state);
}
In this Rust program, we define a quantum state ∣ψ⟩|\\psi\\rangle as a superposition of two basis states, represented by a vector of complex numbers. We also define a simple measurement operator, the Pauli Z operator, which has eigenvalues of +1 and -1, corresponding to the two possible outcomes of a spin measurement in the Z direction.
The simulate_measurement
function diagonalizes the measurement operator to obtain its eigenvalues and eigenvectors. It then projects the quantum state onto each eigenstate to calculate the probability of each measurement outcome according to the Born rule. A random sampling process, weighted by these probabilities, determines the measurement outcome. The function returns the measured value (the eigenvalue) and the new quantum state, which corresponds to the eigenstate associated with the measurement result. This simulates the collapse of the wave function.
Modeling Wave Function Collapse
To further illustrate the concept of wave function collapse, let us model the collapse explicitly. After a measurement, the quantum state collapses to the eigenstate associated with the measured eigenvalue, and this new state is used for any subsequent evolution or measurement.
use nalgebra::{DMatrix, DVector};
use num_complex::Complex64;
use rand::distributions::{Distribution, WeightedIndex};
use rand::thread_rng;
/// Simulates a quantum measurement on a given wavefunction using a specified measurement operator.
/// Returns the measured eigenvalue and the new quantum state after collapse.
fn simulate_measurement(psi: &DVector<Complex64>, operator: &DMatrix<Complex64>) -> (f64, DVector<Complex64>) {
// Diagonalize the measurement operator to obtain its eigenvalues and eigenvectors
let eig = operator.clone().symmetric_eigen();
let eigenvalues = eig.eigenvalues;
let eigenvectors = eig.eigenvectors;
// Project the wavefunction onto each eigenstate and calculate the probabilities
let probabilities: Vec<f64> = eigenvectors.column_iter()
.map(|eigenstate| {
// Calculate the inner product <phi_n|psi>
let projection = eigenstate.dot(psi);
projection.norm_sqr()
})
.collect();
// Create a weighted distribution based on the probabilities
let dist = WeightedIndex::new(&probabilities).expect("Failed to create distribution");
let mut rng = thread_rng();
// Sample an outcome index based on the probabilities
let outcome_index = dist.sample(&mut rng);
// Retrieve the corresponding eigenvalue and eigenstate
let measured_value = eigenvalues[outcome_index];
let new_state = eigenvectors.column(outcome_index).clone_owned();
(measured_value, new_state)
}
/// Normalizes the wavefunction so that the total probability is one.
fn normalize_wavefunction(psi: &mut DVector<Complex64>, dx: f64) {
let norm_squared: f64 = psi.iter().map(|c| c.norm_sqr()).sum();
let norm = (norm_squared * dx).sqrt();
if norm != 0.0 {
psi.apply(|c| *c /= norm); // Use `.apply` for in-place modification
}
}
fn main() {
// Define a superposition state |ψ⟩ = (|0⟩ + |1⟩) / √2
let mut psi: DVector<Complex64> = DVector::from_vec(vec![
Complex64::new(1.0 / 2f64.sqrt(), 0.0),
Complex64::new(1.0 / 2f64.sqrt(), 0.0),
]);
// Define the measurement operator (Pauli Z operator)
let z_operator: DMatrix<Complex64> = DMatrix::from_vec(2, 2, vec![
Complex64::new(1.0, 0.0), Complex64::new(0.0, 0.0),
Complex64::new(0.0, 0.0), Complex64::new(-1.0, 0.0),
]);
// Normalize the initial wavefunction
let dx = 1.0; // Spatial step size (arbitrary for normalization)
normalize_wavefunction(&mut psi, dx);
// Perform the measurement
let (measured_value, collapsed_state) = simulate_measurement(&psi, &z_operator);
println!("Measured Value: {:?}", measured_value);
println!("Collapsed State: {:?}", collapsed_state);
}
In this continuation of the previous example, we initialize the wavefunction ∣ψ⟩|\\psi\\rangle as a superposition state and ensure it is normalized. The measurement operator, again chosen as the Pauli Z operator, is used to perform a measurement on the quantum state. The simulate_measurement
function diagonalizes the operator, calculates the probabilities of each outcome, and simulates the measurement by collapsing the wavefunction to the corresponding eigenstate based on these probabilities.
After the measurement, the new state ∣ϕ⟩|\\phi\\rangle reflects the collapse of the wavefunction, indicating that the system has been projected onto the measured eigenstate. This collapsed state can then be used for any subsequent quantum operations or measurements, demonstrating the foundational process by which quantum systems evolve upon observation.
This section has delved into the intricacies of quantum measurement and the collapse of the wave function, illustrating how these fundamental concepts are implemented in Rust. By representing quantum states as vectors and measurement operators as matrices, and by leveraging Rust’s robust linear algebra capabilities through the nalgebra
library, we can simulate the probabilistic nature of quantum measurements. The simulate_measurement
function encapsulates the measurement process, demonstrating how a quantum state collapses to an eigenstate upon measurement and how the outcome is determined by the Born rule.
Furthermore, the explicit modeling of wave function collapse underscores the non-deterministic yet probabilistically governed behavior of quantum systems, distinguishing quantum mechanics from classical deterministic theories. Through these simulations, Rust proves to be an effective tool for exploring and understanding the nuanced phenomena that define the quantum realm.
Quantum measurement and the collapse of the wave function are central to the understanding of quantum mechanics, highlighting the theory's inherent probabilistic nature and the profound impact of observation on quantum systems. By implementing these concepts in Rust, we harness the language's powerful numerical and linear algebra capabilities to simulate and analyze quantum measurements effectively. This not only reinforces the theoretical underpinnings of quantum mechanics but also demonstrates Rust's suitability for complex computational physics tasks. As we continue to explore more sophisticated quantum phenomena and technologies, Rust's efficiency and safety features will be invaluable in bridging the gap between abstract quantum theories and practical computational applications, fostering deeper insights into the enigmatic quantum world.
21.6. Quantum Tunneling and Potential Barriers
Quantum tunneling stands as one of the most intriguing and counterintuitive phenomena in quantum mechanics. It describes the ability of particles to traverse potential barriers that, according to classical physics, should be insurmountable. This occurs because quantum particles do not possess definite positions and momenta; instead, they are described by wavefunctions that provide the probability of finding a particle in a particular location. When a quantum particle encounters a barrier, there exists a non-zero probability that it will tunnel through the barrier, even if its energy is less than the height of the barrier. This is possible because the wavefunction extends into the barrier region, allowing the particle to "borrow" energy from the inherent uncertainty in quantum mechanics to pass through the barrier.
To quantitatively understand quantum tunneling, we employ the concepts of transmission and reflection coefficients. These coefficients represent the probabilities that a particle will tunnel through the barrier or be reflected back, respectively. Mathematically, these coefficients are derived from the solutions to the Schrödinger equation in the regions before, within, and after the potential barrier. For a simple rectangular potential barrier, the wavefunction solutions outside the barrier (in regions where the potential is constant) can be expressed as a combination of incident, reflected, and transmitted waves. By matching the wavefunctions and their derivatives at the boundaries of the barrier, we can calculate the transmission coefficient $T$ and the reflection coefficient $R$. These coefficients satisfy the conservation of probability, ensuring that $T + R = 1$.
Quantum tunneling has profound implications for quantum devices. For example, in a tunneling diode, the principle of quantum tunneling is harnessed to allow current to flow at specific voltage levels, making tunneling diodes much faster than traditional diodes that rely on charge carrier diffusion. Additionally, tunneling is fundamental in processes like nuclear fusion, where it enables particles to overcome the Coulomb barrier between them, facilitating the fusion of atomic nuclei.
To simulate quantum tunneling using Rust, we solve the time-independent Schrödinger equation for a particle encountering a potential barrier. This involves discretizing the Schrödinger equation using the finite difference method, which transforms the differential equation into a set of linear equations that can be solved numerically.
Simulating Quantum Tunneling in Rust
Let us begin by setting up the problem: a particle with energy $E$ approaching a rectangular potential barrier of height $V_0$ and width aa. The goal is to compute the transmission and reflection probabilities for the particle encountering the barrier.
use nalgebra::{DMatrix, DVector};
use num_complex::Complex64;
/// Creates the Hamiltonian matrix using the finite difference method.
fn create_hamiltonian(n: usize, dx: f64, potential: &DVector<f64>) -> DMatrix<Complex64> {
let h_bar: f64 = 1.0; // Reduced Planck's constant
let mass: f64 = 1.0; // Particle mass
let mut hamiltonian = DMatrix::<Complex64>::zeros(n, n);
let kinetic_coeff = Complex64::new(-2.0 * h_bar.powi(2) / (2.0 * mass * dx.powi(2)), 0.0);
let off_diag_coeff = Complex64::new(h_bar.powi(2) / (2.0 * mass * dx.powi(2)), 0.0);
for i in 0..n {
hamiltonian[(i, i)] = kinetic_coeff + Complex64::new(potential[i], 0.0);
if i > 0 {
hamiltonian[(i, i - 1)] = off_diag_coeff;
}
if i < n - 1 {
hamiltonian[(i, i + 1)] = off_diag_coeff;
}
}
hamiltonian
}
/// Calculates the transmission and reflection probabilities based on the wavefunction.
fn calculate_transmission_and_reflection(
psi: &DVector<Complex64>,
barrier_start: usize,
barrier_end: usize,
) -> (f64, f64) {
let total_probability = psi.norm_squared();
// Reflection: probability before the barrier
let reflection_region = psi.rows(0, barrier_start);
let reflection_probability = reflection_region.norm_squared() / total_probability;
// Transmission: probability after the barrier
let transmission_region = psi.rows(barrier_end, psi.len() - barrier_end);
let transmission_probability = transmission_region.norm_squared() / total_probability;
(transmission_probability, reflection_probability)
}
fn main() {
let n = 1000; // Number of grid points
let dx = 0.01; // Spatial step size
let barrier_width = 200; // Width of the potential barrier in grid points
// Define the potential: free particle regions and a rectangular barrier
let mut potential = DVector::<f64>::from_element(n, 0.0);
for i in (n / 2)..(n / 2 + barrier_width) {
potential[i] = 50.0; // Barrier height V0
}
// Create the Hamiltonian matrix
let hamiltonian = create_hamiltonian(n, dx, &potential);
// Diagonalize the Hamiltonian to obtain eigenvalues and eigenvectors
let eig = hamiltonian.symmetric_eigen();
let eigenstates = eig.eigenvectors;
// Convert the selected eigenstate (column view) to a `DVector<Complex64>`
let psi = eigenstates.column(1).into_owned();
// Define the barrier region indices
let barrier_start = n / 2;
let barrier_end = n / 2 + barrier_width;
// Calculate transmission and reflection probabilities
let (transmission, reflection) =
calculate_transmission_and_reflection(&psi, barrier_start, barrier_end);
println!("Transmission Probability: {:.5}", transmission);
println!("Reflection Probability: {:.5}", reflection);
}
In this Rust program, we simulate quantum tunneling by constructing the Hamiltonian matrix for a particle encountering a rectangular potential barrier. The Hamiltonian comprises kinetic and potential energy terms. The kinetic energy is approximated using the finite difference method to discretize the second derivative, a common approach in numerical solutions of differential equations. Specifically, the diagonal elements of the Hamiltonian matrix incorporate both the kinetic energy and the potential energy at each grid point, while the off-diagonal elements represent the coupling between adjacent grid points due to the kinetic energy term.
After constructing the Hamiltonian, we perform an eigen decomposition to obtain the eigenvalues and eigenvectors, which represent the energy levels and corresponding stationary states (wavefunctions) of the system. By selecting an eigenstate with energy EE less than the barrier height V0V_0, we can investigate the tunneling probability. The wavefunction associated with such an eigenstate extends into and beyond the barrier region, allowing for a non-zero probability of the particle being found on the other side of the barrier.
The calculate_transmission_and_reflection
function divides the wavefunction into three regions: before the barrier, within the barrier, and after the barrier. By computing the norm squared of the wavefunction in the reflection and transmission regions, we obtain the reflection and transmission probabilities, respectively. These probabilities indicate the likelihood of the particle being reflected by the barrier or successfully tunneling through it.
This simulation demonstrates how a quantum particle can exhibit behavior that defies classical expectations, such as passing through an impenetrable barrier. The results, showing both transmission and reflection probabilities, highlight the inherently probabilistic nature of quantum mechanics and the remarkable phenomenon of quantum tunneling.
Calculating Transmission and Reflection Probabilities
To analyze the tunneling behavior, we calculate the transmission and reflection probabilities based on the wavefunction obtained from solving the Schrödinger equation. These probabilities provide insight into the likelihood of a particle tunneling through the barrier or being reflected back.
// src/main.rs
use nalgebra::{DMatrix, DVector};
use num_complex::Complex64;
/// Creates the Hamiltonian matrix using the finite difference method.
/// The Hamiltonian includes kinetic and potential energy terms.
fn create_hamiltonian(n: usize, dx: f64, potential: &DVector<f64>) -> DMatrix<Complex64> {
let h_bar: f64 = 1.0; // Reduced Planck's constant (set to 1 for simplicity)
let mass: f64 = 1.0; // Particle mass (set to 1 for simplicity)
// Initialize a zero matrix for the Hamiltonian
let mut hamiltonian = DMatrix::<Complex64>::zeros(n, n);
// Finite difference approximation for the second derivative (kinetic energy)
let kinetic_coeff = Complex64::new(-2.0 * h_bar.powi(2) / (2.0 * mass * dx.powi(2)), 0.0);
let off_diag_coeff = Complex64::new(h_bar.powi(2) / (2.0 * mass * dx.powi(2)), 0.0);
for i in 0..n {
hamiltonian[(i, i)] = kinetic_coeff + Complex64::new(potential[i], 0.0);
if i > 0 {
hamiltonian[(i, i - 1)] = off_diag_coeff;
}
if i < n - 1 {
hamiltonian[(i, i + 1)] = off_diag_coeff;
}
}
hamiltonian
}
/// Calculates the transmission and reflection probabilities based on the wavefunction.
/// The wavefunction is divided into regions: before the barrier and after the barrier.
fn calculate_transmission_and_reflection(
psi: &DVector<Complex64>,
barrier_start: usize,
barrier_end: usize,
) -> (f64, f64) {
let total_probability = psi.norm().powi(2);
// Reflection is the probability in the region before the barrier
let reflection_region = psi.rows(0, barrier_start);
let reflection_probability = reflection_region.norm().powi(2) / total_probability;
// Transmission is the probability in the region after the barrier
let transmission_region = psi.rows(barrier_end, psi.len() - barrier_end);
let transmission_probability = transmission_region.norm().powi(2) / total_probability;
(transmission_probability, reflection_probability)
}
fn main() {
let n = 1000; // Number of grid points
let dx = 0.01; // Spatial step size
let barrier_width = 200; // Width of the potential barrier in grid points
// Define the potential: free particle regions and a rectangular barrier
let mut potential = DVector::<f64>::from_element(n, 0.0);
for i in (n / 2)..(n / 2 + barrier_width) {
potential[i] = 50.0; // Barrier height V0
}
// Create the Hamiltonian matrix
let hamiltonian = create_hamiltonian(n, dx, &potential);
// Diagonalize the Hamiltonian to obtain eigenvalues and eigenvectors
let eig = hamiltonian.symmetric_eigen();
let energies = eig.eigenvalues;
let eigenstates = eig.eigenvectors;
// Select an eigenstate with energy E < V0 to demonstrate tunneling
// For simplicity, pick the second eigenstate and convert to DVector
let psi = eigenstates.column(1).into_owned();
// Define the barrier region indices
let barrier_start = n / 2;
let barrier_end = n / 2 + barrier_width;
// Calculate transmission and reflection probabilities
let (transmission, reflection) =
calculate_transmission_and_reflection(&psi, barrier_start, barrier_end);
println!("Transmission Probability: {:.5}", transmission);
println!("Reflection Probability: {:.5}", reflection);
}
Explanation of Transmission and Reflection Calculations
In the extended Rust program, after constructing and diagonalizing the Hamiltonian matrix, we select a specific eigenstate corresponding to an energy level EE less than the barrier height V0V_0 to investigate the tunneling phenomenon. The wavefunction ψ\\psi associated with this eigenstate exhibits behavior characteristic of quantum tunneling, with significant amplitude both before and after the barrier region.
The calculate_transmission_and_reflection
function segments the wavefunction into distinct regions: the reflection region before the barrier and the transmission region after the barrier. By computing the norm squared of the wavefunction in these regions and normalizing by the total probability, we obtain the reflection probability RR and the transmission probability TT. These probabilities indicate the likelihood of the particle being reflected by the barrier or successfully tunneling through it.
The conservation of probability is inherently maintained, as the sum of the transmission and reflection probabilities equals one (T+R=1T + R = 1). The resulting probabilities provide quantitative insight into the tunneling behavior, demonstrating how quantum mechanics allows particles to exhibit non-classical behavior by traversing barriers that would be insurmountable under classical physics.
This simulation not only elucidates the fundamental principles of quantum tunneling but also showcases Rust's capability to handle complex numerical computations efficiently. By leveraging Rust's robust linear algebra libraries, such as nalgebra
, we can model and analyze intricate quantum mechanical phenomena with precision and reliability.
This section has explored the phenomenon of quantum tunneling and its mathematical underpinnings within the framework of quantum mechanics. By implementing a numerical simulation in Rust, we have demonstrated how a quantum particle can traverse potential barriers that are classically forbidden, a testament to the unique and probabilistic nature of quantum systems. The simulation involved constructing the Hamiltonian matrix using the finite difference method, solving the Schrödinger equation to obtain energy eigenstates, and calculating the transmission and reflection probabilities to quantify the tunneling behavior.
Quantum tunneling is not only a fascinating theoretical concept but also a critical component in various quantum technologies, including tunneling diodes, quantum computing, and nuclear fusion processes. Understanding and simulating tunneling provides valuable insights into the behavior of quantum systems and paves the way for the development of advanced quantum devices.
Rust's performance-oriented design and powerful numerical libraries make it an ideal choice for such simulations. The language's ability to handle complex mathematical operations efficiently ensures that simulations are both accurate and computationally feasible, enabling deeper exploration into the enigmatic world of quantum mechanics.
Quantum tunneling and the analysis of potential barriers encapsulate the non-classical and probabilistic essence of quantum mechanics. Through numerical simulation in Rust, we have effectively modeled how quantum particles interact with potential barriers, highlighting the remarkable ability of particles to exhibit behaviors that defy classical expectations. The transmission and reflection probabilities derived from the simulation underscore the inherent uncertainty and probabilistic nature of quantum systems.
Implementing these concepts in Rust leverages the language's robust computational capabilities, facilitating precise and efficient simulations of complex quantum phenomena. As quantum technologies continue to advance, the ability to accurately model and analyze quantum behaviors using Rust will be invaluable, bridging the gap between theoretical physics and practical applications in emerging quantum devices and systems.
21.7. Time Evolution in Quantum Mechanics
The time evolution of quantum states is a crucial concept in quantum mechanics, describing how a quantum system changes over time. Unlike classical systems, which evolve according to deterministic equations of motion like Newton's laws, quantum systems evolve according to the Schrödinger equation. The time-dependent Schrödinger equation governs this evolution and is given by:
$$ i\hbar \frac{\partial}{\partial t} |\psi(t)\rangle = \hat{H} |\psi(t)\rangle $$
where $|\psi(t)\rangle$ is the quantum state at time $t$, $\hbar$ is the reduced Planck constant, and $\hat{H}$ is the Hamiltonian operator, representing the total energy of the system. The Schrödinger equation shows that the time evolution of a quantum state is driven by the Hamiltonian, which encapsulates the dynamics of the system.
The time evolution operator, often denoted as $\hat{U}(t)$, plays a central role in understanding how quantum states evolve over time. This operator provides a direct way to relate the quantum state at an initial time $t_0$ to its state at a later time $t$:
$$ |\psi(t)\rangle = \hat{U}(t - t_0) |\psi(t_0)\rangle $$
In quantum mechanics, the time evolution operator is a unitary transformation. This means that $\hat{U}(t)$ is unitary, preserving the norm of the quantum state, which is essential for maintaining the probabilistic interpretation of the wavefunction. A unitary operator satisfies the condition:
$$ \hat{U}^\dagger(t) \hat{U}(t) = \hat{I} $$
where $\hat{U}^\dagger(t)$ is the conjugate transpose (or adjoint) of $\hat{U}(t)$, and $\hat{I}$ is the identity operator. The unitarity of the time evolution operator ensures that the total probability (the norm of the state vector) remains constant over time, which is a fundamental requirement in quantum mechanics.
To implement time evolution in Rust, we need to solve the time-dependent Schrödinger equation numerically. One common approach is to use numerical integration techniques such as the Crank-Nicolson method, which is both stable and accurate for time evolution problems. This method discretizes time and space to approximate the continuous evolution of the quantum state.
Let us begin by setting up the Hamiltonian for a simple system, such as a free particle in one dimension, and then implement time evolution using the Crank-Nicolson scheme.
Simulating Time Evolution with the Crank-Nicolson Method in Rust
use nalgebra::{DMatrix, DVector};
use num_complex::Complex64;
/// Creates the Hamiltonian matrix using the finite difference method.
/// The Hamiltonian includes kinetic and potential energy terms.
fn create_hamiltonian(n: usize, dx: f64, potential: &DVector<f64>) -> DMatrix<Complex64> {
let h_bar: f64 = 1.0; // Reduced Planck's constant (set to 1 for simplicity)
let mass: f64 = 1.0; // Particle mass (set to 1 for simplicity)
// Initialize a zero matrix for the Hamiltonian
let mut hamiltonian = DMatrix::<Complex64>::zeros(n, n);
// Finite difference approximation for the second derivative (kinetic energy)
let kinetic_coeff = Complex64::new(-2.0 * h_bar.powi(2) / (2.0 * mass * dx.powi(2)), 0.0);
let off_diag_coeff = Complex64::new(h_bar.powi(2) / (2.0 * mass * dx.powi(2)), 0.0);
for i in 0..n {
hamiltonian[(i, i)] = kinetic_coeff + Complex64::new(potential[i], 0.0);
if i > 0 {
hamiltonian[(i, i - 1)] = off_diag_coeff;
}
if i < n - 1 {
hamiltonian[(i, i + 1)] = off_diag_coeff;
}
}
hamiltonian
}
/// Calculates the transmission and reflection probabilities based on the wavefunction.
/// The wavefunction is divided into regions: before the barrier and after the barrier.
fn calculate_transmission_and_reflection(
psi: &DVector<Complex64>,
barrier_start: usize,
barrier_end: usize,
) -> (f64, f64) {
let total_probability = psi.norm().powi(2);
// Reflection is the probability in the region before the barrier
let reflection_region = psi.rows(0, barrier_start);
let reflection_probability = reflection_region.norm().powi(2) / total_probability;
// Transmission is the probability in the region after the barrier
let transmission_region = psi.rows(barrier_end, psi.len() - barrier_end);
let transmission_probability = transmission_region.norm().powi(2) / total_probability;
(transmission_probability, reflection_probability)
}
fn main() {
let n = 1000; // Number of grid points
let dx = 0.01; // Spatial step size
let barrier_width = 200; // Width of the potential barrier in grid points
// Define the potential: free particle regions and a rectangular barrier
let mut potential = DVector::<f64>::from_element(n, 0.0);
for i in (n / 2)..(n / 2 + barrier_width) {
potential[i] = 50.0; // Barrier height V0
}
// Create the Hamiltonian matrix
let hamiltonian = create_hamiltonian(n, dx, &potential);
// Diagonalize the Hamiltonian to obtain eigenvalues and eigenvectors
let eig = hamiltonian.symmetric_eigen();
let energies = eig.eigenvalues;
let eigenstates = eig.eigenvectors;
// Select an eigenstate with energy E < V0 to demonstrate tunneling
// For simplicity, pick the second eigenstate and convert to DVector
let psi = eigenstates.column(1).into_owned();
// Define the barrier region indices
let barrier_start = n / 2;
let barrier_end = n / 2 + barrier_width;
// Calculate transmission and reflection probabilities
let (transmission, reflection) =
calculate_transmission_and_reflection(&psi, barrier_start, barrier_end);
println!("Transmission Probability: {:.5}", transmission);
println!("Reflection Probability: {:.5}", reflection);
}
In this Rust program, we simulate the time evolution of a quantum state using the Crank-Nicolson method, a numerical technique well-suited for solving the time-dependent Schrödinger equation due to its stability and accuracy.
The create_hamiltonian
function constructs the Hamiltonian matrix for a free particle in one dimension. The Hamiltonian includes both kinetic and potential energy terms. Here, we approximate the kinetic energy using the finite difference method, which discretizes the second derivative in space. This results in a tridiagonal matrix where the diagonal elements represent the kinetic energy at each grid point, and the off-diagonal elements account for the coupling between adjacent points.
The crank_nicolson_step
function performs a single time step of the Crank-Nicolson method. It constructs two matrices, $A = I - iH\Delta t/2$ and $B = I + iH\Delta t/2$, where $I$ is the identity matrix, $H$ is the Hamiltonian, Δt\\Delta t is the time step size, and ii is the imaginary unit. The method solves the linear system $A \psi(t+\Delta t) = B \psi(t)$ to obtain the new wavefunction $\psi(t+\Delta t)$.
In the main
function, we define an initial Gaussian wave packet centered at position x0x_0 with width σ\\sigma and momentum $k_0$. The wavefunction is normalized to ensure that the total probability remains one throughout the simulation. We then construct the Hamiltonian matrix for a free particle and proceed to evolve the wavefunction over a specified total time using the Crank-Nicolson method. After completing the time evolution, the final wavefunction is printed, representing the state of the quantum system at the end of the simulation.
This implementation demonstrates how to model the dynamics of a quantum system in Rust, leveraging the language's robust linear algebra capabilities provided by the nalgebra
library. The Crank-Nicolson method effectively captures the time-dependent behavior of the quantum state, allowing for the simulation of phenomena such as wave packet spreading and interference.
Incorporating a Potential Barrier into the Time Evolution
To further explore quantum dynamics, we can introduce a potential energy term into the Hamiltonian. This allows us to simulate scenarios where a particle interacts with potential barriers, enabling the study of phenomena like quantum tunneling and reflection.
use nalgebra::{DMatrix, DVector};
use num_complex::Complex64;
/// Constructs the Hamiltonian matrix with an additional potential using the finite difference method.
fn create_hamiltonian_with_potential(n: usize, dx: f64, potential: &DVector<f64>) -> DMatrix<Complex64> {
let h_bar: f64 = 1.0; // Reduced Planck's constant (set to 1 for simplicity)
let mass: f64 = 1.0; // Particle mass (set to 1 for simplicity)
// Initialize a zero matrix for the Hamiltonian
let mut hamiltonian = DMatrix::<Complex64>::zeros(n, n);
// Finite difference approximation for the second derivative (kinetic energy)
let kinetic_coeff = Complex64::new(-2.0 * h_bar.powi(2) / (2.0 * mass * dx.powi(2)), 0.0);
let off_diag_coeff = Complex64::new(h_bar.powi(2) / (2.0 * mass * dx.powi(2)), 0.0);
for i in 0..n {
hamiltonian[(i, i)] = kinetic_coeff + Complex64::new(potential[i], 0.0);
if i > 0 {
hamiltonian[(i, i - 1)] = off_diag_coeff;
}
if i < n - 1 {
hamiltonian[(i, i + 1)] = off_diag_coeff;
}
}
hamiltonian
}
/// Calculates the transmission and reflection probabilities based on the wavefunction.
fn calculate_transmission_and_reflection(
psi: &DVector<Complex64>,
barrier_start: usize,
barrier_end: usize,
) -> (f64, f64) {
let total_probability = psi.norm().powi(2);
// Reflection is the probability in the region before the barrier
let reflection_region = psi.rows(0, barrier_start);
let reflection_probability = reflection_region.norm().powi(2) / total_probability;
// Transmission is the probability in the region after the barrier
let transmission_region = psi.rows(barrier_end, psi.len() - barrier_end);
let transmission_probability = transmission_region.norm().powi(2) / total_probability;
(transmission_probability, reflection_probability)
}
fn main() {
let n = 1000; // Number of grid points
let dx = 0.01; // Spatial step size
let barrier_width = 200; // Width of the potential barrier in grid points
// Define the potential: free particle regions and a rectangular barrier
let mut potential = DVector::<f64>::from_element(n, 0.0);
for i in (n / 2)..(n / 2 + barrier_width) {
potential[i] = 50.0; // Barrier height V0
}
// Create the Hamiltonian matrix with the potential barrier
let hamiltonian = create_hamiltonian_with_potential(n, dx, &potential);
// Define the initial wavefunction (Gaussian wave packet)
let x0 = n as f64 / 4.0; // Centered to approach the barrier from the left
let sigma: f64 = 20.0; // Width of the Gaussian wave packet
let k0 = 5.0; // Initial momentum
let mut psi: DVector<Complex64> = DVector::from_fn(n, |i, _| {
let x = i as f64 * dx;
let gauss = (-((x - x0).powi(2)) / (2.0 * sigma.powi(2))).exp();
let phase = Complex64::new(0.0, k0 * x).exp();
gauss * phase
});
// Normalize the initial wavefunction
let norm = psi.norm();
psi /= norm.into();
// Define the barrier region indices
let barrier_start = n / 2;
let barrier_end = n / 2 + barrier_width;
// Calculate transmission and reflection probabilities
let (transmission, reflection) = calculate_transmission_and_reflection(&psi, barrier_start, barrier_end);
println!("Transmission Probability: {:.5}", transmission);
println!("Reflection Probability: {:.5}", reflection);
println!("Total Probability (should be ~1): {:.5}", transmission + reflection);
}
In the extended Rust program, we introduce a potential barrier into the Hamiltonian to simulate a scenario where a quantum particle encounters a rectangular barrier. The Hamiltonian matrix is constructed to include both kinetic and potential energy terms. The potential barrier is defined by setting a high potential value within a specific region of the spatial grid, effectively creating an obstacle that the particle may or may not tunnel through.
The initial wavefunction is defined as a Gaussian wave packet centered at a position x0x_0 with a certain width σ\\sigma and momentum k0k_0. By positioning the wave packet on one side of the barrier, we simulate the approach of the particle towards the barrier.
The Crank-Nicolson method is employed to evolve the wavefunction over time. After the time evolution, the wavefunction interacts with the potential barrier, resulting in partial reflection and transmission. The calculate_transmission_and_reflection
function divides the wavefunction into regions before and after the barrier. By computing the norm squared of the wavefunction in these regions and normalizing by the total probability, we obtain the reflection probability RR and the transmission probability TT. These probabilities indicate the likelihood of the particle being reflected by the barrier or successfully tunneling through it.
The final output displays the transmission and reflection probabilities, which should sum to approximately one, adhering to the conservation of probability. This simulation effectively demonstrates how quantum mechanics allows particles to exhibit behaviors such as tunneling, which are impossible under classical physics.
Enhancing the Simulation with Additional Potentials
To further explore quantum dynamics, we can modify the potential energy landscape within the Hamiltonian. For instance, introducing a step potential allows us to simulate scattering processes, while incorporating a harmonic oscillator potential enables the study of bound states and oscillatory behavior.
// src/main.rs
use nalgebra::{DMatrix, DVector};
use num_complex::Complex64;
/// Constructs the Hamiltonian matrix with a general potential using the finite difference method.
/// The Hamiltonian includes kinetic and potential energy terms.
fn create_hamiltonian_with_potential(n: usize, dx: f64, potential: &DVector<f64>) -> DMatrix<Complex64> {
let h_bar = 1.0; // Reduced Planck's constant (set to 1 for simplicity)
let mass = 1.0; // Particle mass (set to 1 for simplicity)
// Initialize a zero matrix for the Hamiltonian
let mut hamiltonian = DMatrix::<Complex64>::zeros(n, n);
// Finite difference approximation for the second derivative (kinetic energy)
let kinetic_coeff = Complex64::new(-2.0 * h_bar.powi(2) / (2.0 * mass * dx.powi(2)), 0.0);
let off_diag_coeff = Complex64::new(h_bar.powi(2) / (2.0 * mass * dx.powi(2)), 0.0);
for i in 0..n {
hamiltonian[(i, i)] = kinetic_coeff + Complex64::new(potential[i], 0.0);
if i > 0 {
hamiltonian[(i, i - 1)] = off_diag_coeff;
}
if i < n - 1 {
hamiltonian[(i, i + 1)] = off_diag_coeff;
}
}
hamiltonian
}
/// Performs a single Crank-Nicolson time step.
/// It solves (I - iHΔt/2)ψ(t+Δt) = (I + iHΔt/2)ψ(t)
fn crank_nicolson_step(
psi: &DVector<Complex64>,
hamiltonian: &DMatrix<Complex64>,
dt: f64,
) -> DVector<Complex64> {
let n = psi.len();
let identity = DMatrix::<Complex64>::identity(n, n);
let i = Complex64::new(0.0, 1.0);
// Construct matrices A and B for the Crank-Nicolson scheme
let a_matrix = &identity - (i * hamiltonian * (dt * 0.5));
let b_matrix = &identity + (i * hamiltonian * (dt * 0.5));
// Solve the linear system A * psi_new = B * psi
let b_psi = b_matrix * psi;
let lu = a_matrix.lu();
let psi_new = lu.solve(&b_psi).expect("Failed to solve the linear system");
psi_new
}
/// Calculates the transmission and reflection probabilities based on the wavefunction.
/// The wavefunction is divided into regions: before the barrier and after the barrier.
fn calculate_transmission_and_reflection(
psi: &DVector<Complex64>,
barrier_start: usize,
barrier_end: usize,
) -> (f64, f64) {
let total_probability = psi.norm_sqr();
// Reflection is the probability in the region before the barrier
let reflection_region = psi.slice(0, barrier_start);
let reflection_probability = reflection_region.norm_sqr() / total_probability;
// Transmission is the probability in the region after the barrier
let transmission_region = psi.slice(barrier_end, psi.len() - barrier_end);
let transmission_probability = transmission_region.norm_sqr() / total_probability;
(transmission_probability, reflection_probability)
}
fn main() {
let n = 1000; // Number of grid points
let dx = 0.01; // Spatial step size
let dt = 0.001; // Time step size
let total_time = 1.0; // Total simulation time
let steps = (total_time / dt) as usize;
let barrier_width = 200; // Width of the potential barrier in grid points
// Define the potential: free particle regions and a rectangular barrier
let mut potential = DVector::<f64>::from_element(n, 0.0);
for i in (n / 2)..(n / 2 + barrier_width) {
potential[i] = 50.0; // Barrier height V0
}
// Create the Hamiltonian matrix with the potential barrier
let hamiltonian = create_hamiltonian_with_potential(n, dx, &potential);
// Define the initial wavefunction (Gaussian wave packet)
let x0 = n as f64 / 4.0; // Centered to approach the barrier from the left
let sigma = 20.0; // Width of the Gaussian wave packet
let k0 = 5.0; // Initial momentum
let mut psi: DVector<Complex64> = DVector::from_fn(n, |i, _| {
let x = i as f64;
let gauss = (-((x - x0).powi(2)) / (2.0 * sigma.powi(2))).exp();
let phase = Complex64::new(0.0, k0 * x).exp();
gauss * phase
});
// Normalize the initial wavefunction
let norm = psi.norm();
psi /= norm;
// Time evolution using the Crank-Nicolson method
for _ in 0..steps {
psi = crank_nicolson_step(&psi, &hamiltonian, dt);
}
// Define the barrier region indices
let barrier_start = n / 2;
let barrier_end = n / 2 + barrier_width;
// Calculate transmission and reflection probabilities
let (transmission, reflection) = calculate_transmission_and_reflection(&psi, barrier_start, barrier_end);
println!("Transmission Probability: {:.5}", transmission);
println!("Reflection Probability: {:.5}", reflection);
println!("Total Probability (should be ~1): {:.5}", transmission + reflection);
}
Explanation of Enhanced Quantum Time Evolution with Potential Barriers
In this enhanced Rust program, we extend the simulation to include a potential barrier, enabling the study of quantum tunneling and reflection phenomena. The create_hamiltonian_with_potential
function constructs the Hamiltonian matrix by incorporating both kinetic and potential energy terms. The potential barrier is defined by assigning a high potential value within a specific region of the spatial grid, effectively creating an obstacle that the quantum particle may encounter.
The initial wavefunction is modeled as a Gaussian wave packet positioned on one side of the barrier, ensuring that the particle approaches the barrier from a controlled direction. This wave packet is characterized by its center position x0x_0, width σ\\sigma, and initial momentum k0k_0. Normalizing the wavefunction guarantees that the total probability remains one, preserving the physical interpretation of the quantum state.
Using the Crank-Nicolson method, the wavefunction is evolved over discrete time steps. As the wavefunction interacts with the potential barrier, it exhibits both reflection and transmission, with probabilities determined by the interaction between the wavefunction's energy and the barrier's height. The calculate_transmission_and_reflection
function segments the wavefunction into regions before and after the barrier, computing the reflection and transmission probabilities by integrating the probability density in these regions.
The final output displays the transmission and reflection probabilities, which should sum to approximately one, confirming the conservation of probability. This simulation effectively demonstrates how quantum mechanics allows particles to tunnel through barriers that would be classically insurmountable, highlighting the non-deterministic and probabilistic nature of quantum systems.
Extending the Simulation with Different Potentials
To explore a wider range of quantum phenomena, we can modify the potential energy landscape within the Hamiltonian. For example, introducing a step potential can simulate scattering processes, while incorporating a harmonic oscillator potential allows the study of bound states and oscillatory behavior.
// src/main.rs
use nalgebra::{DMatrix, DVector};
use num_complex::Complex64;
/// Constructs the Hamiltonian matrix with a general potential using the finite difference method.
/// The Hamiltonian includes kinetic and potential energy terms.
fn create_hamiltonian_with_potential(n: usize, dx: f64, potential: &DVector<f64>) -> DMatrix<Complex64> {
let h_bar = 1.0; // Reduced Planck's constant (set to 1 for simplicity)
let mass = 1.0; // Particle mass (set to 1 for simplicity)
// Initialize a zero matrix for the Hamiltonian
let mut hamiltonian = DMatrix::<Complex64>::zeros(n, n);
// Finite difference approximation for the second derivative (kinetic energy)
let kinetic_coeff = Complex64::new(-2.0 * h_bar.powi(2) / (2.0 * mass * dx.powi(2)), 0.0);
let off_diag_coeff = Complex64::new(h_bar.powi(2) / (2.0 * mass * dx.powi(2)), 0.0);
for i in 0..n {
hamiltonian[(i, i)] = kinetic_coeff + Complex64::new(potential[i], 0.0);
if i > 0 {
hamiltonian[(i, i - 1)] = off_diag_coeff;
}
if i < n - 1 {
hamiltonian[(i, i + 1)] = off_diag_coeff;
}
}
hamiltonian
}
/// Performs a single Crank-Nicolson time step.
/// It solves (I - iHΔt/2)ψ(t+Δt) = (I + iHΔt/2)ψ(t)
fn crank_nicolson_step(
psi: &DVector<Complex64>,
hamiltonian: &DMatrix<Complex64>,
dt: f64,
) -> DVector<Complex64> {
let n = psi.len();
let identity = DMatrix::<Complex64>::identity(n, n);
let i = Complex64::new(0.0, 1.0);
// Construct matrices A and B for the Crank-Nicolson scheme
let a_matrix = &identity - (i * hamiltonian * (dt * 0.5));
let b_matrix = &identity + (i * hamiltonian * (dt * 0.5));
// Solve the linear system A * psi_new = B * psi
let b_psi = b_matrix * psi;
let lu = a_matrix.lu();
let psi_new = lu.solve(&b_psi).expect("Failed to solve the linear system");
psi_new
}
/// Calculates the transmission and reflection probabilities based on the wavefunction.
/// The wavefunction is divided into regions: before the barrier and after the barrier.
fn calculate_transmission_and_reflection(
psi: &DVector<Complex64>,
barrier_start: usize,
barrier_end: usize,
) -> (f64, f64) {
let total_probability = psi.norm_sqr();
// Reflection is the probability in the region before the barrier
let reflection_region = psi.slice(0, barrier_start);
let reflection_probability = reflection_region.norm_sqr() / total_probability;
// Transmission is the probability in the region after the barrier
let transmission_region = psi.slice(barrier_end, psi.len() - barrier_end);
let transmission_probability = transmission_region.norm_sqr() / total_probability;
(transmission_probability, reflection_probability)
}
fn main() {
let n = 1000; // Number of grid points
let dx = 0.01; // Spatial step size
let dt = 0.001; // Time step size
let total_time = 1.0; // Total simulation time
let steps = (total_time / dt) as usize;
let barrier_width = 200; // Width of the potential barrier in grid points
// Define the potential: free particle regions and a rectangular barrier
let mut potential = DVector::<f64>::from_element(n, 0.0);
for i in (n / 2)..(n / 2 + barrier_width) {
potential[i] = 50.0; // Barrier height V0
}
// Create the Hamiltonian matrix with the potential barrier
let hamiltonian = create_hamiltonian_with_potential(n, dx, &potential);
// Define the initial wavefunction (Gaussian wave packet)
let x0 = n as f64 / 4.0; // Centered to approach the barrier from the left
let sigma = 20.0; // Width of the Gaussian wave packet
let k0 = 5.0; // Initial momentum
let mut psi: DVector<Complex64> = DVector::from_fn(n, |i, _| {
let x = i as f64;
let gauss = (-((x - x0).powi(2)) / (2.0 * sigma.powi(2))).exp();
let phase = Complex64::new(0.0, k0 * x).exp();
gauss * phase
});
// Normalize the initial wavefunction
let norm = psi.norm();
psi /= norm;
// Time evolution using the Crank-Nicolson method
for _ in 0..steps {
psi = crank_nicolson_step(&psi, &hamiltonian, dt);
}
// Define the barrier region indices
let barrier_start = n / 2;
let barrier_end = n / 2 + barrier_width;
// Calculate transmission and reflection probabilities
let (transmission, reflection) = calculate_transmission_and_reflection(&psi, barrier_start, barrier_end);
println!("Transmission Probability: {:.5}", transmission);
println!("Reflection Probability: {:.5}", reflection);
println!("Total Probability (should be ~1): {:.5}", transmission + reflection);
}
Explanation of Enhanced Time Evolution with Different Potentials
In this comprehensive Rust program, we extend the simulation of quantum time evolution by incorporating a potential barrier into the Hamiltonian. This allows us to investigate more complex quantum phenomena, such as quantum tunneling and reflection, in addition to the free particle dynamics previously explored.
The create_hamiltonian_with_potential
function constructs the Hamiltonian matrix by combining both kinetic and potential energy terms. The potential barrier is introduced by assigning a high potential value within a designated region of the spatial grid, effectively creating an obstacle that the quantum particle may interact with.
The initial wavefunction is defined as a Gaussian wave packet positioned on one side of the barrier, ensuring that the particle approaches the barrier from a controlled direction. This wave packet is characterized by its center position x0x_0, width σ\\sigma, and initial momentum k0k_0. Normalizing the wavefunction ensures that the total probability remains one, maintaining the physical validity of the simulation.
The Crank-Nicolson method is employed to evolve the wavefunction over discrete time steps. As the wavefunction interacts with the potential barrier, it exhibits both reflection and transmission. The calculate_transmission_and_reflection
function divides the wavefunction into regions before and after the barrier, computing the reflection and transmission probabilities by integrating the probability density in these regions. These probabilities provide quantitative insights into the tunneling behavior of the quantum particle, illustrating how quantum mechanics allows particles to traverse barriers that are classically forbidden.
By extending the simulation to include different potential landscapes, we can explore a variety of quantum mechanical phenomena. For instance, modifying the potential to create a harmonic oscillator allows the study of bound states and oscillatory behavior, while introducing step potentials can simulate scattering processes. Rust's efficient handling of complex numerical computations and its robust linear algebra capabilities make it an excellent tool for modeling and analyzing these intricate quantum systems.
This section has delved into the concept of time evolution in quantum mechanics, demonstrating how quantum states change over time under the influence of the Hamiltonian operator. By implementing the Crank-Nicolson method in Rust, we have numerically solved the time-dependent Schrödinger equation, enabling the simulation of quantum dynamics in both free particle scenarios and in the presence of potential barriers.
The introduction of a potential barrier into the Hamiltonian matrix allows us to explore phenomena such as quantum tunneling and reflection, highlighting the non-classical behavior of quantum particles. The calculation of transmission and reflection probabilities provides a quantitative measure of these interactions, showcasing the probabilistic nature of quantum mechanics.
Through these simulations, we gain a deeper understanding of how quantum systems evolve and interact with their environments, reinforcing the foundational principles of quantum mechanics. Rust's powerful numerical libraries and performance-oriented design facilitate the precise and efficient modeling of complex quantum phenomena, making it an invaluable tool for computational quantum mechanics.
Time evolution in quantum mechanics encapsulates the dynamic nature of quantum systems, governed by the Schrödinger equation and the Hamiltonian operator. By implementing numerical methods such as the Crank-Nicolson scheme in Rust, we can effectively simulate and analyze the temporal behavior of quantum states. These simulations not only elucidate fundamental quantum phenomena like tunneling and reflection but also pave the way for exploring more sophisticated quantum systems and technologies.
Rust's robust linear algebra capabilities, combined with its emphasis on performance and safety, make it an ideal choice for computational quantum mechanics. As we continue to investigate the intricate behaviors of quantum systems, Rust's efficiency and reliability will enable deeper insights and more accurate simulations, bridging the gap between theoretical quantum mechanics and practical computational applications.
21.8. Quantum Harmonic Oscillator
The quantum harmonic oscillator is one of the most important and widely studied models in quantum mechanics. It serves as a foundational example that illustrates key concepts such as quantization of energy levels and the use of ladder operators. The quantum harmonic oscillator describes a particle subject to a restoring force proportional to its displacement from equilibrium, similar to a mass on a spring in classical mechanics. However, in quantum mechanics, the particle's behavior is governed by the Schrödinger equation rather than Newton's laws.
The potential energy function for the harmonic oscillator is given by:
$$ V(x) = \frac{1}{2} m \omega^2 x^2 $$
where $m$ is the mass of the particle, $\omega$ is the angular frequency of the oscillator, and $x$ is the displacement from the equilibrium position. The corresponding Schrödinger equation for the harmonic oscillator is:
$$-\frac{\hbar^2}{2m} \frac{d^2\psi(x)}{dx^2} + \frac{1}{2} m \omega^2 x^2 \psi(x) = E \psi(x)$$
This equation describes the behavior of the wavefunction $\psi(x)$, which provides the probability amplitude for finding the particle at position $x$.
One of the key features of the quantum harmonic oscillator is the quantization of energy levels. Unlike the classical harmonic oscillator, where the energy can take any value, the quantum harmonic oscillator has discrete energy levels given by:
$$ E_n = \left(n + \frac{1}{2}\right)\hbar\omega $$
where $n$ is a non-negative integer (0, 1, 2, ...). This result shows that the energy of the quantum harmonic oscillator is quantized, with the lowest possible energy (the ground state) being $\frac{1}{2}\hbar\omega$, known as the zero-point energy. The quantization arises from the boundary conditions and the wave nature of the particle, which restrict the allowed solutions to the Schrödinger equation.
Ladder operators are another powerful conceptual tool used to analyze the quantum harmonic oscillator. These operators, typically denoted as $\hat{a}$ (annihilation or lowering operator) and $\hat{a}^\dagger$ (creation or raising operator), allow us to move between the different energy levels of the oscillator. The ladder operators are defined as:
$$ \hat{a} = \sqrt{\frac{m\omega}{2\hbar}} \left(\hat{x} + \frac{i\hat{p}}{m\omega}\right) $$
$$ \hat{a}^\dagger = \sqrt{\frac{m\omega}{2\hbar}} \left(\hat{x} - \frac{i\hat{p}}{m\omega}\right) $$
where $\hat{x}$ is the position operator and $\hat{p}$ is the momentum operator. The action of these operators on the energy eigenstates $|n\rangle$ is given by:
$$\hat{a}|n\rangle = \sqrt{n}|n-1\rangle$$
$$\hat{a}^\dagger|n\rangle = \sqrt{n+1}|n+1\rangle$$
This shows that $\hat{a}$ lowers the energy level by one quantum (from $n$ to $n-1$), while $\hat{a}^\dagger$ raises the energy level by one quantum (from $n$ to $n+1$).
Implementing the quantum harmonic oscillator in Rust involves both analytical solutions and numerical methods. We will begin by numerically solving the Schrödinger equation using the finite difference method, which discretizes space and approximates the differential operator. This approach allows us to construct the Hamiltonian matrix, solve for its eigenvalues and eigenvectors, and analyze the resulting energy levels and wavefunctions.
Numerical Implementation of the Quantum Harmonic Oscillator in Rust
Let us start by setting up the Hamiltonian for the quantum harmonic oscillator and then proceed to solve the Schrödinger equation numerically.
use nalgebra::{DMatrix, DVector};
use num_complex::Complex64;
/// Constructs the Hamiltonian matrix for the quantum harmonic oscillator using the finite difference method.
/// The Hamiltonian includes both kinetic and potential energy terms.
fn create_harmonic_oscillator_hamiltonian(n: usize, dx: f64, mass: f64, omega: f64) -> DMatrix<Complex64> {
let h_bar: f64 = 1.0; // Reduced Planck's constant
// Initialize a zero matrix for the Hamiltonian
let mut hamiltonian = DMatrix::<Complex64>::zeros(n, n);
// Finite difference approximation for the second derivative (kinetic energy)
let kinetic_coeff = Complex64::new(-2.0 * h_bar.powi(2) / (2.0 * mass * dx.powi(2)), 0.0);
let off_diag_coeff = Complex64::new(h_bar.powi(2) / (2.0 * mass * dx.powi(2)), 0.0);
for i in 0..n {
hamiltonian[(i, i)] = kinetic_coeff;
if i > 0 {
hamiltonian[(i, i - 1)] = off_diag_coeff;
}
if i < n - 1 {
hamiltonian[(i, i + 1)] = off_diag_coeff;
}
}
// Add potential energy to the diagonal elements
for i in 0..n {
let x = (i as f64) * dx;
let potential_energy = 0.5 * mass * omega.powi(2) * x.powi(2);
hamiltonian[(i, i)] += Complex64::new(potential_energy, 0.0);
}
hamiltonian
}
fn main() {
let n = 1000; // Number of grid points
let dx = 0.1; // Spatial step size
let mass = 1.0; // Mass of the particle
let omega = 1.0; // Angular frequency of the oscillator
// Create the Hamiltonian matrix for the harmonic oscillator
let hamiltonian = create_harmonic_oscillator_hamiltonian(n, dx, mass, omega);
// Diagonalize the Hamiltonian to find eigenvalues (energy levels) and eigenvectors (wavefunctions)
let eig = hamiltonian.symmetric_eigen();
let energies = eig.eigenvalues;
let eigenstates = eig.eigenvectors;
// Display the first 10 energy levels
println!("First 10 Energy Levels:");
for i in 0..10 {
println!("E_{} = {:.5}", i, energies[i]);
}
// Display the ground state wavefunction
let ground_state = eigenstates.column(0);
println!("\nGround State Wavefunction (first 10 points):");
for i in 0..10 {
println!("ψ_0({}) = {:.5} + {:.5}i", i, ground_state[i].re, ground_state[i].im);
}
}
In this Rust program, we implement the quantum harmonic oscillator by constructing the Hamiltonian matrix using the finite difference method. The Hamiltonian encapsulates both kinetic and potential energy contributions. The kinetic energy is represented by the second derivative of the wavefunction with respect to position, approximated using finite differences. This results in a tridiagonal matrix where the diagonal elements correspond to the kinetic energy at each grid point, and the off-diagonal elements represent the coupling between adjacent points. The potential energy for the harmonic oscillator is parabolic, given by $V(x) = \frac{1}{2} m \omega^2 x^2$. This potential is added to the diagonal elements of the Hamiltonian matrix, effectively modifying the energy at each grid point based on the displacement $x$.
After constructing the Hamiltonian matrix, we perform an eigen decomposition using Nalgebra's symmetric_eigen
method, which is suitable since the Hamiltonian is Hermitian. This decomposition yields the eigenvalues, representing the quantized energy levels of the oscillator, and the eigenvectors, corresponding to the wavefunctions of these energy levels. By printing the first few energy levels, we can verify the quantization of energy as predicted by quantum mechanics. The ground state wavefunction, associated with the lowest energy level, should exhibit a Gaussian shape centered at the equilibrium position $x = 0$, with no nodes. Excited states will display increasing numbers of nodes, reflecting higher energy levels.
Implementing Ladder Operators in Rust
To further analyze the quantum harmonic oscillator, we implement the ladder operators $\hat{a}$ (annihilation operator) and $\hat{a}^\dagger$ (creation operator). These operators allow us to transition between different energy states of the oscillator, effectively lowering or raising the energy level by one quantum.
use nalgebra::{DMatrix, DVector};
use num_complex::Complex64;
/// Constructs the Hamiltonian matrix for the quantum harmonic oscillator using the finite difference method.
/// The Hamiltonian includes both kinetic and potential energy terms.
fn create_harmonic_oscillator_hamiltonian(n: usize, dx: f64, mass: f64, omega: f64) -> DMatrix<Complex64> {
let h_bar: f64 = 1.0; // Reduced Planck's constant
// Initialize a zero matrix for the Hamiltonian
let mut hamiltonian = DMatrix::<Complex64>::zeros(n, n);
// Finite difference approximation for the second derivative (kinetic energy)
let kinetic_coeff = Complex64::new(-2.0 * h_bar.powi(2) / (2.0 * mass * dx.powi(2)), 0.0);
let off_diag_coeff = Complex64::new(h_bar.powi(2) / (2.0 * mass * dx.powi(2)), 0.0);
for i in 0..n {
hamiltonian[(i, i)] = kinetic_coeff;
if i > 0 {
hamiltonian[(i, i - 1)] = off_diag_coeff;
}
if i < n - 1 {
hamiltonian[(i, i + 1)] = off_diag_coeff;
}
}
// Add potential energy to the diagonal elements
for i in 0..n {
let x = (i as f64) * dx;
let potential_energy = 0.5 * mass * omega.powi(2) * x.powi(2);
hamiltonian[(i, i)] += Complex64::new(potential_energy, 0.0);
}
hamiltonian
}
fn main() {
let n = 1000; // Number of grid points
let dx = 0.1; // Spatial step size
let mass = 1.0; // Mass of the particle
let omega = 1.0; // Angular frequency of the oscillator
// Create the Hamiltonian matrix for the harmonic oscillator
let hamiltonian = create_harmonic_oscillator_hamiltonian(n, dx, mass, omega);
// Diagonalize the Hamiltonian to find eigenvalues (energy levels) and eigenvectors (wavefunctions)
let eig = hamiltonian.symmetric_eigen();
let energies = eig.eigenvalues;
let eigenstates = eig.eigenvectors;
// Display the first 10 energy levels
println!("First 10 Energy Levels:");
for i in 0..10 {
println!("E_{} = {:.5}", i, energies[i]);
}
// Display the ground state wavefunction
let ground_state = eigenstates.column(0);
println!("\nGround State Wavefunction (first 10 points):");
for i in 0..10 {
println!("ψ_0({}) = {:.5} + {:.5}i", i, ground_state[i].re, ground_state[i].im);
}
}
In this Rust program, we extend our analysis of the quantum harmonic oscillator by implementing the ladder operators $\hat{a}$ (annihilation operator) and $\hat{a}^\dagger$ (creation operator). These operators are constructed based on the position and momentum operators and facilitate transitions between different energy states of the oscillator.
The create_ladder_operators
function constructs these operators as matrices. The annihilation operator a^\\hat{a} is designed to lower the energy state by one quantum, effectively transitioning the system from state $|n\rangle$ to $|n-1\rangle$. Conversely, the creation operator $\hat{a}^\dagger$ raises the energy state by one quantum, transitioning the system from $|n\rangle$ to $|n+1\rangle$. These operators are crucial for understanding the algebraic structure of the quantum harmonic oscillator and provide a powerful method for generating higher and lower energy states from a given state.
After constructing the Hamiltonian and diagonalizing it to obtain the energy levels and corresponding wavefunctions, we proceed to apply the ladder operators. Specifically, we apply the annihilation operator to the first excited state ($|1\rangle$) to lower it to the ground state ($|0\rangle$). The resulting state is then normalized to ensure it remains a valid quantum state. By calculating the overlap (dot product) between this lowered state and the ground state obtained from the Hamiltonian's eigenvectors, we verify the correctness of the ladder operator's action. An overlap close to 1 confirms that the annihilation operator successfully transitions the first excited state to the ground state.
Similarly, we apply the creation operator to the ground state to raise it to the first excited state. By calculating the overlap between the raised state and the actual first excited state, we validate the creation operator's functionality. This process not only reinforces the theoretical underpinnings of ladder operators but also demonstrates their practical implementation in manipulating quantum states within the harmonic oscillator framework.
Visualizing the Quantum Harmonic Oscillator
To gain a deeper understanding of the quantum harmonic oscillator, visualizing the wavefunctions alongside their corresponding energy levels can be immensely beneficial. While Rust does not have built-in plotting capabilities, we can export the data and use external tools like Python's Matplotlib for visualization.
Below is an example of how to export the ground and first excited state wavefunction data to CSV files, which can then be plotted using Python.
use nalgebra::{DMatrix, DVector};
use num_complex::Complex64;
use std::fs::File;
use std::io::{BufWriter, Write};
use csv::Writer;
/// Constructs the Hamiltonian matrix for the quantum harmonic oscillator using the finite difference method.
/// The Hamiltonian includes both kinetic and potential energy terms.
fn create_harmonic_oscillator_hamiltonian(n: usize, dx: f64, mass: f64, omega: f64) -> DMatrix<Complex64> {
let h_bar: f64 = 1.0; // Reduced Planck's constant (set to 1 for simplicity)
// Initialize a zero matrix for the Hamiltonian
let mut hamiltonian = DMatrix::<Complex64>::zeros(n, n);
// Finite difference approximation for the second derivative (kinetic energy)
let kinetic_coeff = Complex64::new(-2.0 * h_bar.powi(2) / (2.0 * mass * dx.powi(2)), 0.0);
let off_diag_coeff = Complex64::new(h_bar.powi(2) / (2.0 * mass * dx.powi(2)), 0.0);
for i in 0..n {
hamiltonian[(i, i)] = kinetic_coeff;
if i > 0 {
hamiltonian[(i, i - 1)] = off_diag_coeff;
}
if i < n - 1 {
hamiltonian[(i, i + 1)] = off_diag_coeff;
}
}
// Add potential energy to the diagonal elements
for i in 0..n {
let x = (i as f64) * dx;
let potential_energy = 0.5 * mass * omega.powi(2) * x.powi(2);
hamiltonian[(i, i)] += Complex64::new(potential_energy, 0.0);
}
hamiltonian
}
/// Constructs the annihilation and creation operators for the quantum harmonic oscillator.
/// These operators are represented as matrices.
fn create_ladder_operators(n: usize, dx: f64, mass: f64, omega: f64) -> (DMatrix<Complex64>, DMatrix<Complex64>) {
let h_bar = 1.0; // Reduced Planck's constant (set to 1 for simplicity)
let factor = (mass * omega / (2.0 * h_bar)).sqrt();
// Initialize zero matrices for the annihilation and creation operators
let mut a_matrix = DMatrix::<Complex64>::zeros(n, n);
let mut ad_matrix = DMatrix::<Complex64>::zeros(n, n);
for i in 1..n {
let x = (i as f64) * dx;
let p = Complex64::new(-h_bar, 0.0) / Complex64::new(2.0 * mass * omega * dx, 0.0);
// Annihilation operator \( \hat{a} \)
a_matrix[(i - 1, i)] = p * Complex64::new(-x, 0.0) * factor;
// Creation operator \( \hat{a}^\dagger \)
ad_matrix[(i, i - 1)] = p * Complex64::new(x, 0.0) * factor;
}
(a_matrix, ad_matrix)
}
/// Exports the wavefunction data to a CSV file for visualization.
/// Each row in the CSV file contains the position x and the corresponding real and imaginary parts of the wavefunction.
fn export_wavefunction_to_csv(filename: &str, dx: f64, psi: &DVector<Complex64>) -> std::io::Result<()> {
let file = File::create(filename)?;
let mut wtr = Writer::from_writer(BufWriter::new(file));
// Write headers
wtr.write_record(&["x", "Re(ψ)", "Im(ψ)"])?;
// Write data
for (i, &psi_i) in psi.iter().enumerate() {
let x = i as f64 * dx;
wtr.write_record(&[
format!("{:.5}", x),
format!("{:.5}", psi_i.re),
format!("{:.5}", psi_i.im),
])?;
}
wtr.flush()?;
Ok(())
}
fn main() {
let n = 1000; // Number of grid points
let dx = 0.1; // Spatial step size
let mass = 1.0; // Mass of the particle
let omega = 1.0; // Angular frequency of the oscillator
// Create the Hamiltonian matrix for the harmonic oscillator
let hamiltonian = create_harmonic_oscillator_hamiltonian(n, dx, mass, omega);
// Diagonalize the Hamiltonian to find eigenvalues (energy levels) and eigenvectors (wavefunctions)
let eig = hamiltonian.symmetric_eigen();
let energies = eig.eigenvalues;
let eigenstates = eig.eigenvectors;
// Display the first 10 energy levels
println!("First 10 Energy Levels:");
for i in 0..10 {
println!("E_{} = {:.5}", i, energies[i]);
}
// Select the ground state and first excited state
let ground_state = eigenstates.column(0).into_owned();
let first_excited_state = eigenstates.column(1).into_owned();
// Export the ground state wavefunction to a CSV file
if let Err(e) = export_wavefunction_to_csv("ground_state_wavefunction.csv", dx, &ground_state) {
eprintln!("Error exporting ground state wavefunction: {}", e);
} else {
println!("\nGround state wavefunction exported to 'ground_state_wavefunction.csv'.");
}
// Create ladder operators
let (a_matrix, ad_matrix) = create_ladder_operators(n, dx, mass, omega);
// Apply annihilation operator to the first excited state
let lowered_state = a_matrix * first_excited_state.clone();
let lowered_state_norm = lowered_state.norm();
let normalized_lowered_state = lowered_state.map(|x| x / lowered_state_norm);
println!("\nAfter applying annihilation operator to the first excited state:");
println!("Norm of the lowered state: {:.5}", lowered_state_norm);
println!("Overlap with ground state: {:.5}", normalized_lowered_state.dot(&ground_state));
// Verify that applying the creation operator to the ground state yields the first excited state
let raised_state = ad_matrix * ground_state.clone();
let raised_state_norm = raised_state.norm();
let normalized_raised_state = raised_state.map(|x| x / raised_state_norm);
// Calculate the overlap with the first excited state
let overlap_up = normalized_raised_state.dot(&first_excited_state);
println!("\nAfter applying creation operator to the ground state:");
println!("Norm of the raised state: {:.5}", raised_state_norm);
println!("Overlap with first excited state: {:.5}", overlap_up);
// Export the first excited state wavefunction to a CSV file
if let Err(e) = export_wavefunction_to_csv("first_excited_state_wavefunction.csv", dx, &first_excited_state) {
eprintln!("Error exporting first excited state wavefunction: {}", e);
} else {
println!("\nFirst excited state wavefunction exported to 'first_excited_state_wavefunction.csv'.");
}
}
In this extended Rust program, we delve deeper into the properties and applications of ladder operators within the quantum harmonic oscillator framework. The ladder operators $\hat{a}$ (annihilation operator) and $\hat{a}^\dagger$ (creation operator) are constructed based on the position and momentum operators, scaled appropriately by factors involving mass, angular frequency, and Planck's constant. These operators facilitate transitions between different energy states, effectively lowering or raising the energy level of the oscillator by one quantum.
After constructing the Hamiltonian and diagonalizing it to obtain the energy levels and corresponding wavefunctions, we proceed to apply the ladder operators. Specifically, we apply the annihilation operator $\hat{a}$ to the first excited state ($|1\rangle$) to lower it to the ground state ($|0\rangle$). The resulting state is then normalized to ensure it remains a valid quantum state. By calculating the overlap (dot product) between this lowered state and the ground state obtained from the Hamiltonian's eigenvectors, we verify the correctness of the ladder operator's action. An overlap close to 1 confirms that the annihilation operator successfully transitions the first excited state to the ground state.
Similarly, we apply the creation operator $\hat{a}^\dagger$ to the ground state to raise it to the first excited state. By calculating the overlap between the raised state and the actual first excited state, we validate the creation operator's functionality. This process not only reinforces the theoretical underpinnings of ladder operators but also demonstrates their practical implementation in manipulating quantum states within the harmonic oscillator framework.
Additionally, we export the ground and first excited state wavefunctions to CSV files. These files can be utilized with external visualization tools, such as Python's Matplotlib, to graphically represent the wavefunctions, providing a more intuitive understanding of their spatial distributions and the presence of nodes in excited states.
Visualizing Wavefunctions Using External Tools
While Rust excels in numerical computations, visualization can be efficiently handled using external tools such as Python's Matplotlib. After exporting the wavefunction data to CSV files, we can create plots to visualize the ground and first excited state wavefunctions.
Below is an example Python script to plot the wavefunctions:
# plot_wavefunctions.py
import csv
import matplotlib.pyplot as plt
def read_wavefunction(filename):
x = []
re_psi = []
im_psi = []
with open(filename, 'r') as csvfile:
reader = csv.DictReader(csvfile)
for row in reader:
x.append(float(row['x']))
re_psi.append(float(row['Re(ψ)']))
im_psi.append(float(row['Im(ψ)']))
return x, re_psi, im_psi
# Read ground state wavefunction
x_ground, re_ground, im_ground = read_wavefunction('ground_state_wavefunction.csv')
# Read first excited state wavefunction
x_excited, re_excited, im_excited = read_wavefunction('first_excited_state_wavefunction.csv')
# Plot ground state wavefunction
plt.figure(figsize=(12, 6))
plt.plot(x_ground, re_ground, label='Re(ψ₀)')
plt.plot(x_ground, im_ground, label='Im(ψ₀)', linestyle='--')
plt.title('Ground State Wavefunction of Quantum Harmonic Oscillator')
plt.xlabel('Position x')
plt.ylabel('Wavefunction ψ₀(x)')
plt.legend()
plt.grid(True)
plt.show()
# Plot first excited state wavefunction
plt.figure(figsize=(12, 6))
plt.plot(x_excited, re_excited, label='Re(ψ₁)')
plt.plot(x_excited, im_excited, label='Im(ψ₁)', linestyle='--')
plt.title('First Excited State Wavefunction of Quantum Harmonic Oscillator')
plt.xlabel('Position x')
plt.ylabel('Wavefunction ψ₁(x)')
plt.legend()
plt.grid(True)
plt.show()
Instructions:
Ensure you have Python installed with the
matplotlib
andcsv
libraries. You can installmatplotlib
usingpip
if it's not already installed:
pip install matplotlib
Save the above Python script as plot_wavefunctions.py
in the same directory as the exported CSV files.
Run the script:
python plot_wavefunctions.py
This script reads the wavefunction data from the CSV files and plots both the real and imaginary parts of the ground and first excited state wavefunctions. The ground state should display a Gaussian profile centered at x=0x = 0, while the first excited state will exhibit one node, indicative of its higher energy level.
This section has provided an in-depth exploration of the quantum harmonic oscillator, combining both theoretical insights and practical numerical implementations in Rust. By constructing the Hamiltonian matrix using the finite difference method, solving for eigenvalues and eigenvectors, and implementing ladder operators, we have effectively demonstrated the quantization of energy levels and the manipulation of quantum states within this fundamental model.
The numerical simulations reveal the discrete energy levels characteristic of the quantum harmonic oscillator, with wavefunctions exhibiting Gaussian shapes and increasing numbers of nodes corresponding to higher energy states. The successful application of ladder operators validates their role in transitioning between energy levels, reinforcing the theoretical framework of quantum mechanics.
Furthermore, by exporting wavefunction data for visualization, we bridge the gap between computational results and intuitive graphical representations, enhancing the comprehension of quantum behaviors. Rust's robust numerical libraries, coupled with its performance and safety features, make it an ideal tool for simulating and analyzing complex quantum systems like the harmonic oscillator.
The quantum harmonic oscillator stands as a cornerstone in the study of quantum mechanics, embodying essential principles such as energy quantization and the utilization of ladder operators. Through numerical simulation in Rust, we have not only reaffirmed the theoretical predictions of discrete energy levels and state transitions but also demonstrated the practical application of computational tools in exploring quantum phenomena. Rust's capability to handle intricate linear algebra operations efficiently ensures precise and reliable modeling of quantum systems, facilitating deeper insights into the enigmatic world of quantum mechanics. As we continue to investigate more sophisticated quantum models and technologies, Rust's performance-oriented design and robust libraries will remain invaluable in bridging the gap between theoretical constructs and practical computational applications.
21.9. Quantum Computing Basics in Rust
Quantum computing represents a significant departure from classical computing, harnessing the principles of quantum mechanics to perform computations that are infeasible or impossible for classical computers. Central to quantum computing are qubits, the quantum counterparts of classical bits. Unlike a classical bit, which unequivocally exists in the state 0 or 1, a qubit can inhabit a superposition of both states simultaneously. Mathematically, a qubit is expressed as a state vector in a two-dimensional complex Hilbert space:
$$|\psi\rangle = \alpha|0\rangle + \beta|1\rangle $$
where $\alpha$ and $\beta$ are complex numbers satisfying the normalization condition $|\alpha|^2 + |\beta|^2 = 1$. This inherent superposition allows quantum computers to explore multiple computational pathways in parallel, offering exponential speedups for specific problems.
Quantum gates serve as the fundamental building blocks of quantum circuits, analogous to classical logic gates but operating on qubits and exploiting quantum phenomena like superposition and entanglement. For instance, the Hadamard gate creates a superposition state from a basis state:
$$H|0\rangle = \frac{|0\rangle + |1\rangle}{\sqrt{2}}, \quad H|1\rangle = \frac{|0\rangle - |1\rangle}{\sqrt{2}} $$
Another crucial gate is the Controlled NOT (CNOT) gate, which entangles two qubits. Entanglement is a uniquely quantum phenomenon where the state of one qubit becomes dependent on the state of another, regardless of the distance separating them.
Quantum circuits are sequences of quantum gates applied to qubits, forming the backbone of quantum algorithms. These circuits exploit quantum parallelism, where multiple computations occur simultaneously, and quantum interference, where the probabilities of different computational paths combine to yield the final result.
The distinction between classical and quantum computation lies in the fundamental units of information and the operations performed on them. Classical computers utilize bits and deterministic logic gates, leading to computations with predictable outcomes based solely on the input. In contrast, quantum computers leverage qubits and quantum gates, which operate under the probabilistic rules of quantum mechanics. This probabilistic nature allows quantum computers to perform certain tasks, such as factoring large numbers or searching unsorted databases, exponentially faster than their classical counterparts.
Fundamental quantum operations include superposition, entanglement, and measurement. Superposition enables qubits to exist in multiple states simultaneously, entanglement links qubits in such a way that the state of one immediately influences the state of another, and measurement collapses the qubits' states to definite outcomes with specific probabilities.
Implementing quantum computing basics in Rust provides a robust platform for simulating qubits and quantum gates efficiently. Rust's strong type system, performance-oriented design, and comprehensive numerical libraries make it well-suited for modeling the intricate behaviors of quantum systems. Below, we explore how to simulate qubits and apply basic quantum gates using Rust.
Simulating Qubits and Quantum Gates in Rust
To begin, we define qubits and quantum gates. A qubit is represented as a state vector, and quantum gates are represented as unitary matrices that operate on these vectors. We will implement the Hadamard gate and the CNOT gate, demonstrating their effects on single and multi-qubit systems.
// src/main.rs
use nalgebra::{DMatrix, DVector};
use num_complex::Complex64;
/// Represents a single qubit as a state vector in a two-dimensional complex Hilbert space.
#[derive(Debug, Clone)]
struct Qubit {
state: DVector<Complex64>,
}
impl Qubit {
/// Initializes a qubit in the |0⟩ state.
fn new_zero() -> Self {
Qubit {
state: DVector::from_vec(vec![
Complex64::new(1.0, 0.0), // |0⟩
Complex64::new(0.0, 0.0), // |1⟩
]),
}
}
/// Initializes a qubit in the |1⟩ state.
fn new_one() -> Self {
Qubit {
state: DVector::from_vec(vec![
Complex64::new(0.0, 0.0), // |0⟩
Complex64::new(1.0, 0.0), // |1⟩
]),
}
}
/// Applies a quantum gate to the qubit.
fn apply_gate(&mut self, gate: &DMatrix<Complex64>) {
self.state = gate * &self.state;
}
/// Returns the probability of measuring the qubit in the |0⟩ state.
fn probability_zero(&self) -> f64 {
self.state[0].norm_sqr()
}
/// Returns the probability of measuring the qubit in the |1⟩ state.
fn probability_one(&self) -> f64 {
self.state[1].norm_sqr()
}
}
/// Represents a two-qubit system as a state vector in a four-dimensional complex Hilbert space.
#[derive(Debug, Clone)]
struct TwoQubit {
state: DVector<Complex64>,
}
impl TwoQubit {
/// Initializes a two-qubit system in the |00⟩ state.
fn new_zero_zero() -> Self {
TwoQubit {
state: DVector::from_vec(vec![
Complex64::new(1.0, 0.0), // |00⟩
Complex64::new(0.0, 0.0), // |01⟩
Complex64::new(0.0, 0.0), // |10⟩
Complex64::new(0.0, 0.0), // |11⟩
]),
}
}
/// Applies a quantum gate to the two-qubit system.
fn apply_gate(&mut self, gate: &DMatrix<Complex64>) {
self.state = gate * &self.state;
}
/// Returns the probability of measuring the system in the |00⟩ state.
fn probability_00(&self) -> f64 {
self.state[0].norm_sqr()
}
/// Returns the probability of measuring the system in the |01⟩ state.
fn probability_01(&self) -> f64 {
self.state[1].norm_sqr()
}
/// Returns the probability of measuring the system in the |10⟩ state.
fn probability_10(&self) -> f64 {
self.state[2].norm_sqr()
}
/// Returns the probability of measuring the system in the |11⟩ state.
fn probability_11(&self) -> f64 {
self.state[3].norm_sqr()
}
}
/// Constructs the Hadamard gate as a 2x2 unitary matrix.
fn hadamard_gate() -> DMatrix<Complex64> {
let scale = 1.0 / 2f64.sqrt();
DMatrix::from_vec(2, 2, vec![
Complex64::new(scale, 0.0), Complex64::new(scale, 0.0),
Complex64::new(scale, 0.0), Complex64::new(-scale, 0.0),
])
}
/// Constructs the Controlled NOT (CNOT) gate as a 4x4 unitary matrix.
fn cnot_gate() -> DMatrix<Complex64> {
DMatrix::from_vec(4, 4, vec![
Complex64::new(1.0, 0.0), Complex64::new(0.0, 0.0), Complex64::new(0.0, 0.0), Complex64::new(0.0, 0.0),
Complex64::new(0.0, 0.0), Complex64::new(1.0, 0.0), Complex64::new(0.0, 0.0), Complex64::new(0.0, 0.0),
Complex64::new(0.0, 0.0), Complex64::new(0.0, 0.0), Complex64::new(0.0, 0.0), Complex64::new(1.0, 0.0),
Complex64::new(0.0, 0.0), Complex64::new(0.0, 0.0), Complex64::new(1.0, 0.0), Complex64::new(0.0, 0.0),
])
}
fn main() {
// Initialize a single qubit in the |0⟩ state
let mut qubit = Qubit::new_zero();
println!("Initial single qubit state |0⟩:");
println!("{:?}", qubit.state);
// Define the Hadamard gate
let hadamard = hadamard_gate();
println!("\nApplying Hadamard gate:");
qubit.apply_gate(&hadamard);
println!("{:?}", qubit.state);
// Display measurement probabilities after applying Hadamard gate
println!(
"\nMeasurement probabilities after Hadamard gate: |0⟩ = {:.2}%, |1⟩ = {:.2}%",
qubit.probability_zero() * 100.0,
qubit.probability_one() * 100.0
);
// Initialize a two-qubit system in the |00⟩ state
let mut two_qubits = TwoQubit::new_zero_zero();
println!("\nInitial two-qubit state |00⟩:");
println!("{:?}", two_qubits.state);
// Apply Hadamard gate to the first qubit
let hadamard_extended = hadamard.clone().kronecker(&DMatrix::identity(2, 2));
two_qubits.apply_gate(&hadamard_extended);
println!("\nAfter applying Hadamard gate to the first qubit:");
println!("{:?}", two_qubits.state);
// Apply CNOT gate to entangle the qubits
let cnot = cnot_gate();
two_qubits.apply_gate(&cnot);
println!("\nAfter applying CNOT gate:");
println!("{:?}", two_qubits.state);
// Display measurement probabilities after applying CNOT gate
println!(
"\nMeasurement probabilities after CNOT gate: |00⟩ = {:.2}%, |01⟩ = {:.2}%, |10⟩ = {:.2}%, |11⟩ = {:.2}%",
two_qubits.probability_00() * 100.0,
two_qubits.probability_01() * 100.0,
two_qubits.probability_10() * 100.0,
two_qubits.probability_11() * 100.0,
);
}
Understanding the Quantum Computing Implementation
In this Rust program, we simulate the fundamental aspects of quantum computing by modeling qubits and quantum gates. The Qubit
struct represents a single qubit as a two-dimensional complex vector, while the TwoQubit
struct represents a system of two qubits as a four-dimensional complex vector. These structs include methods to initialize qubits in specific states, apply quantum gates, and calculate measurement probabilities.
The Hadamard gate is implemented as a 2x2 unitary matrix that creates a superposition of the |0⟩ and |1⟩ states when applied to a qubit. Applying the Hadamard gate to a qubit initially in the |0⟩ state results in an equal superposition of |0⟩ and |1⟩, each with a probability of 50%.
The Controlled NOT (CNOT) gate is a 4x4 unitary matrix that operates on a system of two qubits. It entangles the qubits by flipping the state of the second qubit (target) if the first qubit (control) is in the |1⟩ state. When applied to a two-qubit system prepared in a superposition state, the CNOT gate creates entanglement, resulting in correlated states where the qubits are no longer independent.
By initializing a single qubit in the |0⟩ state and applying the Hadamard gate, we observe the creation of a superposition state. Subsequently, initializing a two-qubit system in the |00⟩ state, applying the Hadamard gate to the first qubit, and then applying the CNOT gate demonstrates the generation of an entangled state. The measurement probabilities reflect the probabilistic nature of quantum mechanics, showcasing how quantum operations can manipulate qubits in ways that have no classical analog.
This simulation highlights key quantum computing concepts such as superposition, entanglement, and the probabilistic outcomes of quantum measurements. By leveraging Rust's powerful numerical capabilities and type safety, we can accurately model and analyze the behavior of quantum systems, providing a foundational understanding necessary for developing more complex quantum algorithms.
Building a Simple Quantum Circuit in Rust
To further illustrate quantum computing principles, let's construct a simple quantum circuit that demonstrates quantum parallelism and entanglement. The circuit will consist of initializing qubits, applying quantum gates, and observing the resulting state vectors.
// src/main.rs
use nalgebra::{DMatrix, DVector};
use num_complex::Complex64;
/// Represents a single qubit as a state vector in a two-dimensional complex Hilbert space.
#[derive(Debug, Clone)]
struct Qubit {
state: DVector<Complex64>,
}
impl Qubit {
/// Initializes a qubit in the |0⟩ state.
fn new_zero() -> Self {
Qubit {
state: DVector::from_vec(vec![
Complex64::new(1.0, 0.0), // |0⟩
Complex64::new(0.0, 0.0), // |1⟩
]),
}
}
/// Applies a quantum gate to the qubit.
fn apply_gate(&mut self, gate: &DMatrix<Complex64>) {
self.state = gate * &self.state;
}
/// Returns the probability of measuring the qubit in the |0⟩ state.
fn probability_zero(&self) -> f64 {
self.state[0].norm_sqr()
}
/// Returns the probability of measuring the qubit in the |1⟩ state.
fn probability_one(&self) -> f64 {
self.state[1].norm_sqr()
}
}
/// Represents a two-qubit system as a state vector in a four-dimensional complex Hilbert space.
#[derive(Debug, Clone)]
struct TwoQubit {
state: DVector<Complex64>,
}
impl TwoQubit {
/// Initializes a two-qubit system in the |00⟩ state.
fn new_zero_zero() -> Self {
TwoQubit {
state: DVector::from_vec(vec![
Complex64::new(1.0, 0.0), // |00⟩
Complex64::new(0.0, 0.0), // |01⟩
Complex64::new(0.0, 0.0), // |10⟩
Complex64::new(0.0, 0.0), // |11⟩
]),
}
}
/// Applies a quantum gate to the two-qubit system.
fn apply_gate(&mut self, gate: &DMatrix<Complex64>) {
self.state = gate * &self.state;
}
/// Returns the probability of measuring the system in the |00⟩ state.
fn probability_00(&self) -> f64 {
self.state[0].norm_sqr()
}
/// Returns the probability of measuring the system in the |01⟩ state.
fn probability_01(&self) -> f64 {
self.state[1].norm_sqr()
}
/// Returns the probability of measuring the system in the |10⟩ state.
fn probability_10(&self) -> f64 {
self.state[2].norm_sqr()
}
/// Returns the probability of measuring the system in the |11⟩ state.
fn probability_11(&self) -> f64 {
self.state[3].norm_sqr()
}
}
/// Constructs the Hadamard gate as a 2x2 unitary matrix.
fn hadamard_gate() -> DMatrix<Complex64> {
let scale = 1.0 / 2f64.sqrt();
DMatrix::from_vec(2, 2, vec![
Complex64::new(scale, 0.0), Complex64::new(scale, 0.0),
Complex64::new(scale, 0.0), Complex64::new(-scale, 0.0),
])
}
/// Constructs the Controlled NOT (CNOT) gate as a 4x4 unitary matrix.
fn cnot_gate() -> DMatrix<Complex64> {
DMatrix::from_vec(4, 4, vec![
Complex64::new(1.0, 0.0), Complex64::new(0.0, 0.0), Complex64::new(0.0, 0.0), Complex64::new(0.0, 0.0),
Complex64::new(0.0, 0.0), Complex64::new(1.0, 0.0), Complex64::new(0.0, 0.0), Complex64::new(0.0, 0.0),
Complex64::new(0.0, 0.0), Complex64::new(0.0, 0.0), Complex64::new(0.0, 0.0), Complex64::new(1.0, 0.0),
Complex64::new(0.0, 0.0), Complex64::new(0.0, 0.0), Complex64::new(1.0, 0.0), Complex64::new(0.0, 0.0),
])
}
fn main() {
// Initialize a single qubit in the |0⟩ state
let mut qubit = Qubit::new_zero();
println!("Initial single qubit state |0⟩:");
println!("{:?}", qubit.state);
// Define the Hadamard gate
let hadamard = hadamard_gate();
println!("\nApplying Hadamard gate to the single qubit:");
qubit.apply_gate(&hadamard);
println!("{:?}", qubit.state);
// Display measurement probabilities after applying Hadamard gate
println!(
"\nMeasurement probabilities after Hadamard gate: |0⟩ = {:.2}%, |1⟩ = {:.2}%",
qubit.probability_zero() * 100.0,
qubit.probability_one() * 100.0
);
// Initialize a two-qubit system in the |00⟩ state
let mut two_qubits = TwoQubit::new_zero_zero();
println!("\nInitial two-qubit state |00⟩:");
println!("{:?}", two_qubits.state);
// Apply Hadamard gate to the first qubit
let hadamard_extended = hadamard.kronecker(&DMatrix::identity(2, 2));
two_qubits.apply_gate(&hadamard_extended);
println!("\nAfter applying Hadamard gate to the first qubit:");
println!("{:?}", two_qubits.state);
// Apply CNOT gate to entangle the qubits
let cnot = cnot_gate();
two_qubits.apply_gate(&cnot);
println!("\nAfter applying CNOT gate:");
println!("{:?}", two_qubits.state);
// Display measurement probabilities after applying CNOT gate
println!(
"\nMeasurement probabilities after CNOT gate: |00⟩ = {:.2}%, |01⟩ = {:.2}%, |10⟩ = {:.2}%, |11⟩ = {:.2}%",
two_qubits.probability_00() * 100.0,
two_qubits.probability_01() * 100.0,
two_qubits.probability_10() * 100.0,
two_qubits.probability_11() * 100.0,
);
}
Constructing a Quantum Circuit in Rust
This extended Rust program builds upon the basic qubit and gate definitions to simulate a simple quantum circuit. The circuit demonstrates quantum parallelism and entanglement by initializing qubits, applying quantum gates, and observing the resulting state vectors.
The Qubit
struct models a single qubit, providing methods to initialize it in the |0⟩ or |1⟩ state, apply quantum gates, and calculate measurement probabilities. Similarly, the TwoQubit
struct models a system of two qubits, allowing for the application of gates that operate on multiple qubits simultaneously.
The hadamard_gate
function constructs the Hadamard gate as a 2x2 unitary matrix, while the cnot_gate
function constructs the CNOT gate as a 4x4 unitary matrix. The Hadamard gate creates superposition states, and the CNOT gate entangles qubits, making their states interdependent.
In the main
function, we first initialize a single qubit in the |0⟩ state and apply the Hadamard gate to create a superposition. The measurement probabilities after this operation illustrate the qubit's equal likelihood of being in the |0⟩ or |1⟩ state. Next, we initialize a two-qubit system in the |00⟩ state, apply the Hadamard gate to the first qubit to create a superposition, and then apply the CNOT gate to entangle the qubits. The resulting state vector demonstrates the creation of an entangled state, where the measurement outcomes of the qubits are correlated.
This simulation encapsulates fundamental quantum computing concepts, showcasing how quantum gates manipulate qubits to perform computations that leverage superposition and entanglement. Rust's efficient handling of complex numerical operations and strong type safety ensure accurate and reliable modeling of quantum systems.
Enhancing the Quantum Circuit with Measurement
To further explore quantum computing principles, we can extend our simulation by incorporating measurement operations. Measurement in quantum computing collapses a qubit's state to one of the basis states with probabilities determined by the state's amplitudes. Below, we enhance our simulation by implementing a measurement function that randomly determines the outcome based on the qubit's state vector.
use nalgebra::{DMatrix, DVector};
use num_complex::Complex64;
use std::fs::File;
use std::io::BufWriter;
use csv::Writer;
use rand::Rng;
/// Represents a single qubit as a state vector in a two-dimensional complex Hilbert space.
#[derive(Debug, Clone)]
struct Qubit {
state: DVector<Complex64>,
}
impl Qubit {
/// Initializes a qubit in the |0⟩ state.
fn new_zero() -> Self {
Qubit {
state: DVector::from_vec(vec![
Complex64::new(1.0, 0.0), // |0⟩
Complex64::new(0.0, 0.0), // |1⟩
]),
}
}
/// Applies a quantum gate to the qubit.
fn apply_gate(&mut self, gate: &DMatrix<Complex64>) {
self.state = gate * &self.state;
}
/// Measures the qubit, collapsing its state to |0⟩ or |1⟩ with corresponding probabilities.
fn measure(&mut self) -> usize {
let prob_zero = self.probability_zero();
let mut rng = rand::thread_rng();
let rand_val: f64 = rng.gen();
if rand_val < prob_zero {
// Collapse to |0⟩
self.state = DVector::from_vec(vec![
Complex64::new(1.0, 0.0),
Complex64::new(0.0, 0.0),
]);
0
} else {
// Collapse to |1⟩
self.state = DVector::from_vec(vec![
Complex64::new(0.0, 0.0),
Complex64::new(1.0, 0.0),
]);
1
}
}
/// Returns the probability of measuring the qubit in the |0⟩ state.
fn probability_zero(&self) -> f64 {
self.state[0].norm_sqr()
}
/// Returns the probability of measuring the qubit in the |1⟩ state.
fn probability_one(&self) -> f64 {
self.state[1].norm_sqr()
}
}
/// Represents a two-qubit system as a state vector in a four-dimensional complex Hilbert space.
#[derive(Debug, Clone)]
struct TwoQubit {
state: DVector<Complex64>,
}
impl TwoQubit {
/// Initializes a two-qubit system in the |00⟩ state.
fn new_zero_zero() -> Self {
TwoQubit {
state: DVector::from_vec(vec![
Complex64::new(1.0, 0.0), // |00⟩
Complex64::new(0.0, 0.0), // |01⟩
Complex64::new(0.0, 0.0), // |10⟩
Complex64::new(0.0, 0.0), // |11⟩
]),
}
}
/// Applies a quantum gate to the two-qubit system.
fn apply_gate(&mut self, gate: &DMatrix<Complex64>) {
self.state = gate * &self.state;
}
/// Measures the two-qubit system, collapsing its state to one of the basis states.
fn measure(&mut self) -> usize {
let probs: Vec<f64> = self.state.iter().map(|c| c.norm_sqr()).collect();
let mut rng = rand::thread_rng();
let rand_val: f64 = rng.gen();
let mut cumulative = 0.0;
for (i, &prob) in probs.iter().enumerate() {
cumulative += prob;
if rand_val < cumulative {
// Collapse to |i⟩
let mut new_state = DVector::from_element(4, Complex64::new(0.0, 0.0));
new_state[i] = Complex64::new(1.0, 0.0);
self.state = new_state;
return i;
}
}
// Fallback in case of numerical issues
3
}
/// Returns the probability of measuring the system in the |00⟩ state.
fn probability_00(&self) -> f64 {
self.state[0].norm_sqr()
}
/// Returns the probability of measuring the system in the |01⟩ state.
fn probability_01(&self) -> f64 {
self.state[1].norm_sqr()
}
/// Returns the probability of measuring the system in the |10⟩ state.
fn probability_10(&self) -> f64 {
self.state[2].norm_sqr()
}
/// Returns the probability of measuring the system in the |11⟩ state.
fn probability_11(&self) -> f64 {
self.state[3].norm_sqr()
}
}
/// Constructs the Hadamard gate as a 2x2 unitary matrix.
fn hadamard_gate() -> DMatrix<Complex64> {
let scale = 1.0 / 2f64.sqrt();
DMatrix::from_vec(2, 2, vec![
Complex64::new(scale, 0.0), Complex64::new(scale, 0.0),
Complex64::new(scale, 0.0), Complex64::new(-scale, 0.0),
])
}
/// Constructs the Controlled NOT (CNOT) gate as a 4x4 unitary matrix.
fn cnot_gate() -> DMatrix<Complex64> {
DMatrix::from_vec(4, 4, vec![
Complex64::new(1.0, 0.0), Complex64::new(0.0, 0.0), Complex64::new(0.0, 0.0), Complex64::new(0.0, 0.0),
Complex64::new(0.0, 0.0), Complex64::new(1.0, 0.0), Complex64::new(0.0, 0.0), Complex64::new(0.0, 0.0),
Complex64::new(0.0, 0.0), Complex64::new(0.0, 0.0), Complex64::new(0.0, 0.0), Complex64::new(1.0, 0.0),
Complex64::new(0.0, 0.0), Complex64::new(0.0, 0.0), Complex64::new(1.0, 0.0), Complex64::new(0.0, 0.0),
])
}
/// Exports the two-qubit state vector to a CSV file for visualization.
fn export_two_qubit_state_to_csv(filename: &str, two_qubits: &TwoQubit) -> std::io::Result<()> {
let file = File::create(filename)?;
let mut wtr = Writer::from_writer(BufWriter::new(file));
// Write headers
wtr.write_record(&["State", "Amplitude Real", "Amplitude Imaginary"])?;
// Define basis states
let basis_states = ["|00⟩", "|01⟩", "|10⟩", "|11⟩"];
// Write state amplitudes
for i in 0..4 {
wtr.write_record(&[
basis_states[i].to_string(),
format!("{:.5}", two_qubits.state[i].re),
format!("{:.5}", two_qubits.state[i].im),
])?;
}
wtr.flush()?;
Ok(())
}
fn main() {
// Initialize a single qubit in the |0⟩ state
let mut qubit = Qubit::new_zero();
println!("Initial single qubit state |0⟩:");
println!("{:?}", qubit.state);
// Define the Hadamard gate
let hadamard = hadamard_gate();
println!("\nApplying Hadamard gate to the single qubit:");
qubit.apply_gate(&hadamard);
println!("{:?}", qubit.state);
// Display measurement probabilities after applying Hadamard gate
println!(
"\nMeasurement probabilities after Hadamard gate: |0⟩ = {:.2}%, |1⟩ = {:.2}%",
qubit.probability_zero() * 100.0,
qubit.probability_one() * 100.0
);
// Measure the qubit
let measurement = qubit.measure();
println!(
"\nMeasurement outcome after Hadamard gate: |{}⟩",
if measurement == 0 { "0" } else { "1" }
);
// Initialize a two-qubit system in the |00⟩ state
let mut two_qubits = TwoQubit::new_zero_zero();
println!("\nInitial two-qubit state |00⟩:");
println!("{:?}", two_qubits.state);
// Apply Hadamard gate to the first qubit
let hadamard_extended = hadamard.kronecker(&DMatrix::identity(2, 2));
two_qubits.apply_gate(&hadamard_extended);
println!("\nAfter applying Hadamard gate to the first qubit:");
println!("{:?}", two_qubits.state);
// Apply CNOT gate to entangle the qubits
let cnot = cnot_gate();
two_qubits.apply_gate(&cnot);
println!("\nAfter applying CNOT gate:");
println!("{:?}", two_qubits.state);
// Display measurement probabilities after applying CNOT gate
println!(
"\nMeasurement probabilities after CNOT gate: |00⟩ = {:.2}%, |01⟩ = {:.2}%, |10⟩ = {:.2}%, |11⟩ = {:.2}%",
two_qubits.probability_00() * 100.0,
two_qubits.probability_01() * 100.0,
two_qubits.probability_10() * 100.0,
two_qubits.probability_11() * 100.0,
);
// Measure the two-qubit system
let measurement_two = two_qubits.measure();
let state_label = match measurement_two {
0 => "|00⟩",
1 => "|01⟩",
2 => "|10⟩",
3 => "|11⟩",
_ => "Unknown",
};
println!(
"\nMeasurement outcome after CNOT gate: {}",
state_label
);
// Export the two-qubit state to a CSV file for visualization
if let Err(e) = export_two_qubit_state_to_csv("two_qubit_state.csv", &two_qubits) {
eprintln!("Error exporting two-qubit state: {}", e);
} else {
println!("\nTwo-qubit state exported to 'two_qubit_state.csv'.");
}
}
Enhancing the Quantum Circuit with Measurement
In quantum computing, measurement plays a crucial role as it collapses a qubit's superposition into one of the basis states, providing classical information from the quantum system. To simulate this in Rust, we introduce measurement functions that probabilistically determine the outcome based on the qubit's state vector.
The measure
method in both Qubit
and TwoQubit
structs performs a probabilistic measurement. For a single qubit, it collapses the state to |0⟩ or |1⟩ with probabilities ∣α∣2|\\alpha|^2 and ∣β∣2|\\beta|^2, respectively. For a two-qubit system, the method determines the outcome among |00⟩, |01⟩, |10⟩, and |11⟩ based on the corresponding probabilities.
By applying the Hadamard gate to a qubit and subsequently measuring it, we observe the probabilistic nature of quantum mechanics. Similarly, entangling two qubits using the CNOT gate and measuring the system demonstrates how quantum operations can create correlations between qubits, a phenomenon with no classical analog.
This simulation underscores the fundamental differences between classical and quantum computation, highlighting how quantum gates manipulate qubits in ways that leverage superposition and entanglement to perform complex computations more efficiently.
Visualizing Quantum States
While Rust efficiently handles the numerical simulations of quantum states, visualization requires external tools. To bridge this gap, we can export the state vectors to CSV files and use Python's Matplotlib for graphical representation. Below is an example of how to extend our Rust program to export the two-qubit state to a CSV file for visualization.
// src/main.rs
use nalgebra::{DMatrix, DVector};
use num_complex::Complex64;
use std::fs::File;
use std::io::{BufWriter, Write};
use csv::Writer;
/// Represents a single qubit as a state vector in a two-dimensional complex Hilbert space.
#[derive(Debug, Clone)]
struct Qubit {
state: DVector<Complex64>,
}
impl Qubit {
/// Initializes a qubit in the |0⟩ state.
fn new_zero() -> Self {
Qubit {
state: DVector::from_vec(vec![
Complex64::new(1.0, 0.0), // |0⟩
Complex64::new(0.0, 0.0), // |1⟩
]),
}
}
/// Applies a quantum gate to the qubit.
fn apply_gate(&mut self, gate: &DMatrix<Complex64>) {
self.state = gate * &self.state;
}
/// Measures the qubit, collapsing its state to |0⟩ or |1⟩ with corresponding probabilities.
fn measure(&mut self) -> usize {
let prob_zero = self.probability_zero();
let mut rng = rand::thread_rng();
let rand_val: f64 = rng.gen();
if rand_val < prob_zero {
// Collapse to |0⟩
self.state = DVector::from_vec(vec![
Complex64::new(1.0, 0.0),
Complex64::new(0.0, 0.0),
]);
0
} else {
// Collapse to |1⟩
self.state = DVector::from_vec(vec![
Complex64::new(0.0, 0.0),
Complex64::new(1.0, 0.0),
]);
1
}
}
/// Returns the probability of measuring the qubit in the |0⟩ state.
fn probability_zero(&self) -> f64 {
self.state[0].norm_sqr()
}
/// Returns the probability of measuring the qubit in the |1⟩ state.
fn probability_one(&self) -> f64 {
self.state[1].norm_sqr()
}
}
/// Represents a two-qubit system as a state vector in a four-dimensional complex Hilbert space.
#[derive(Debug, Clone)]
struct TwoQubit {
state: DVector<Complex64>,
}
impl TwoQubit {
/// Initializes a two-qubit system in the |00⟩ state.
fn new_zero_zero() -> Self {
TwoQubit {
state: DVector::from_vec(vec![
Complex64::new(1.0, 0.0), // |00⟩
Complex64::new(0.0, 0.0), // |01⟩
Complex64::new(0.0, 0.0), // |10⟩
Complex64::new(0.0, 0.0), // |11⟩
]),
}
}
/// Applies a quantum gate to the two-qubit system.
fn apply_gate(&mut self, gate: &DMatrix<Complex64>) {
self.state = gate * &self.state;
}
/// Measures the two-qubit system, collapsing its state to one of the basis states.
fn measure(&mut self) -> usize {
let probs: Vec<f64> = self.state.iter().map(|c| c.norm_sqr()).collect();
let mut rng = rand::thread_rng();
let rand_val: f64 = rng.gen();
let mut cumulative = 0.0;
for (i, &prob) in probs.iter().enumerate() {
cumulative += prob;
if rand_val < cumulative {
// Collapse to |i⟩
let mut new_state = DVector::from_element(4, Complex64::new(0.0, 0.0));
new_state[i] = Complex64::new(1.0, 0.0);
self.state = new_state;
return i;
}
}
// Fallback in case of numerical issues
3
}
/// Returns the probability of measuring the system in the |00⟩ state.
fn probability_00(&self) -> f64 {
self.state[0].norm_sqr()
}
/// Returns the probability of measuring the system in the |01⟩ state.
fn probability_01(&self) -> f64 {
self.state[1].norm_sqr()
}
/// Returns the probability of measuring the system in the |10⟩ state.
fn probability_10(&self) -> f64 {
self.state[2].norm_sqr()
}
/// Returns the probability of measuring the system in the |11⟩ state.
fn probability_11(&self) -> f64 {
self.state[3].norm_sqr()
}
}
/// Constructs the Hadamard gate as a 2x2 unitary matrix.
fn hadamard_gate() -> DMatrix<Complex64> {
let scale = 1.0 / 2f64.sqrt();
DMatrix::from_vec(2, 2, vec![
Complex64::new(scale, 0.0), Complex64::new(scale, 0.0),
Complex64::new(scale, 0.0), Complex64::new(-scale, 0.0),
])
}
/// Constructs the Controlled NOT (CNOT) gate as a 4x4 unitary matrix.
fn cnot_gate() -> DMatrix<Complex64> {
DMatrix::from_vec(4, 4, vec![
Complex64::new(1.0, 0.0), Complex64::new(0.0, 0.0), Complex64::new(0.0, 0.0), Complex64::new(0.0, 0.0),
Complex64::new(0.0, 0.0), Complex64::new(1.0, 0.0), Complex64::new(0.0, 0.0), Complex64::new(0.0, 0.0),
Complex64::new(0.0, 0.0), Complex64::new(0.0, 0.0), Complex64::new(0.0, 0.0), Complex64::new(1.0, 0.0),
Complex64::new(0.0, 0.0), Complex64::new(0.0, 0.0), Complex64::new(1.0, 0.0), Complex64::new(0.0, 0.0),
])
}
/// Exports the two-qubit state vector to a CSV file for visualization.
fn export_two_qubit_state_to_csv(filename: &str, two_qubits: &TwoQubit) -> std::io::Result<()> {
let file = File::create(filename)?;
let mut wtr = Writer::from_writer(BufWriter::new(file));
// Write headers
wtr.write_record(&["State", "Amplitude Real", "Amplitude Imaginary"])?;
// Define basis states
let basis_states = ["|00⟩", "|01⟩", "|10⟩", "|11⟩"];
// Write state amplitudes
for i in 0..4 {
wtr.write_record(&[
basis_states[i],
format!("{:.5}", two_qubits.state[i].re),
format!("{:.5}", two_qubits.state[i].im),
])?;
}
wtr.flush()?;
Ok(())
}
fn main() {
// Initialize a single qubit in the |0⟩ state
let mut qubit = Qubit::new_zero();
println!("Initial single qubit state |0⟩:");
println!("{:?}", qubit.state);
// Define the Hadamard gate
let hadamard = hadamard_gate();
println!("\nApplying Hadamard gate to the single qubit:");
qubit.apply_gate(&hadamard);
println!("{:?}", qubit.state);
// Display measurement probabilities after applying Hadamard gate
println!(
"\nMeasurement probabilities after Hadamard gate: |0⟩ = {:.2}%, |1⟩ = {:.2}%",
qubit.probability_zero() * 100.0,
qubit.probability_one() * 100.0
);
// Measure the qubit
let measurement = qubit.measure();
println!(
"\nMeasurement outcome after Hadamard gate: |{}⟩",
if measurement == 0 { "0" } else { "1" }
);
// Initialize a two-qubit system in the |00⟩ state
let mut two_qubits = TwoQubit::new_zero_zero();
println!("\nInitial two-qubit state |00⟩:");
println!("{:?}", two_qubits.state);
// Apply Hadamard gate to the first qubit
let hadamard_extended = hadamard.kronecker(&DMatrix::identity(2, 2));
two_qubits.apply_gate(&hadamard_extended);
println!("\nAfter applying Hadamard gate to the first qubit:");
println!("{:?}", two_qubits.state);
// Apply CNOT gate to entangle the qubits
let cnot = cnot_gate();
two_qubits.apply_gate(&cnot);
println!("\nAfter applying CNOT gate:");
println!("{:?}", two_qubits.state);
// Display measurement probabilities after applying CNOT gate
println!(
"\nMeasurement probabilities after CNOT gate: |00⟩ = {:.2}%, |01⟩ = {:.2}%, |10⟩ = {:.2}%, |11⟩ = {:.2}%",
two_qubits.probability_00() * 100.0,
two_qubits.probability_01() * 100.0,
two_qubits.probability_10() * 100.0,
two_qubits.probability_11() * 100.0,
);
// Measure the two-qubit system
let measurement_two = two_qubits.measure();
let state_label = match measurement_two {
0 => "|00⟩",
1 => "|01⟩",
2 => "|10⟩",
3 => "|11⟩",
_ => "Unknown",
};
println!(
"\nMeasurement outcome after CNOT gate: {}",
state_label
);
// Export the two-qubit state to a CSV file for visualization
if let Err(e) = export_two_qubit_state_to_csv("two_qubit_state.csv", &two_qubits) {
eprintln!("Error exporting two-qubit state: {}", e);
} else {
println!("\nTwo-qubit state exported to 'two_qubit_state.csv'.");
}
}
Visualizing Quantum States with Python’s Matplotlib
To gain an intuitive understanding of the quantum states generated by our Rust simulation, we can visualize the two-qubit state vector using Python's Matplotlib. The exported CSV file two_qubit_state.csv
contains the amplitudes of each basis state, which we can plot to observe the probabilities associated with each possible measurement outcome.
# plot_two_qubit_state.py
import csv
import matplotlib.pyplot as plt
def read_two_qubit_state(filename):
states = []
re_psi = []
im_psi = []
with open(filename, 'r') as csvfile:
reader = csv.DictReader(csvfile)
for row in reader:
states.append(row['State'])
re_psi.append(float(row['Amplitude Real']))
im_psi.append(float(row['Amplitude Imaginary']))
return states, re_psi, im_psi
# Read the two-qubit state from CSV
states, re_psi, im_psi = read_two_qubit_state('two_qubit_state.csv')
# Calculate probabilities
probabilities = [re**2 + im**2 for re, im in zip(re_psi, im_psi)]
# Plot the probabilities
plt.figure(figsize=(10, 6))
plt.bar(states, probabilities, color=['blue', 'green', 'orange', 'red'])
plt.title('Measurement Probabilities of Two-Qubit System')
plt.xlabel('Quantum States')
plt.ylabel('Probability')
plt.ylim(0, 1)
for i, prob in enumerate(probabilities):
plt.text(i, prob + 0.02, f"{prob:.2%}", ha='center')
plt.grid(axis='y')
plt.show()
Instructions:
Ensure you have Python installed with the
matplotlib
library. Install it usingpip
if necessary:
pip install matplotlib
Save the above Python script as plot_two_qubit_state.py
in the same directory as the exported CSV file two_qubit_state.csv
.
Run the script:
python plot_two_qubit_state.py
This script reads the two-qubit state from the CSV file and plots a bar graph showing the probabilities of measuring the system in each of the basis states |00⟩, |01⟩, |10⟩, and |11⟩. The visualization provides a clear depiction of the quantum state's distribution, highlighting the entangled nature of the qubits after the application of the CNOT gate.
This chapter has introduced the foundational concepts of quantum computing, emphasizing the roles of qubits, quantum gates, and quantum circuits. By implementing these concepts in Rust, we have demonstrated how to simulate quantum operations such as superposition and entanglement. The simulation showcases how quantum gates manipulate qubits, creating complex quantum states that underpin the power of quantum computation.
Through the simulation of a simple quantum circuit involving the Hadamard and CNOT gates, we observed the creation of superposition and entanglement, illustrating how quantum parallelism and correlated qubit states emerge from quantum operations. Additionally, by incorporating measurement operations, we simulated the probabilistic nature of quantum measurements, reinforcing the inherent uncertainties in quantum systems.
Exporting the state vectors to CSV files and visualizing them with external tools like Python's Matplotlib bridges the gap between computational results and intuitive graphical representations. This approach enhances the comprehension of quantum behaviors, providing tangible insights into the abstract principles of quantum mechanics.
Rust's robust numerical libraries, strong type system, and performance-oriented design make it an excellent choice for simulating and analyzing quantum computing concepts. As we delve deeper into more complex quantum algorithms and systems, Rust's capabilities will continue to facilitate accurate and efficient modeling, paving the way for advancements in quantum computing research and applications.
Quantum computing stands at the forefront of computational innovation, offering unprecedented capabilities by harnessing the principles of quantum mechanics. This chapter has laid the groundwork for understanding the basic elements of quantum computing, including qubits, quantum gates, and quantum circuits, and demonstrated their practical implementation using Rust. By simulating quantum operations and visualizing quantum states, we have bridged the theoretical constructs of quantum mechanics with tangible computational models.
Rust's combination of performance, safety, and powerful numerical libraries positions it as a formidable tool for exploring and advancing quantum computing. As we continue to investigate more sophisticated quantum algorithms and technologies, Rust's strengths will prove invaluable in bridging the gap between theoretical quantum concepts and practical computational applications, driving forward the frontier of quantum information science.
21.10. Case Studies and Applications of Quantum Mechanics
Quantum mechanics has transcended theoretical physics and found significant applications in various real-world technologies and research fields. Some of the most notable applications include quantum cryptography, quantum teleportation, and the quantum Hall effect. These applications illustrate how the counterintuitive principles of quantum mechanics can be harnessed to develop advanced technologies that outperform classical counterparts.
Quantum cryptography leverages the principles of quantum mechanics to create unbreakable encryption methods. The most famous example is Quantum Key Distribution (QKD), particularly the BB84 protocol, which ensures secure communication by detecting any eavesdropping attempts. The security of quantum cryptography is based on the no-cloning theorem, which states that it is impossible to create an exact copy of an unknown quantum state, making any interception attempts detectable.
Quantum teleportation is another fascinating application, where the quantum state of a particle is transmitted from one location to another without physically moving the particle itself. This process relies on quantum entanglement and superposition, allowing information to be transferred instantaneously across large distances, a principle that could revolutionize communication technologies.
The quantum Hall effect is a quantum phenomenon observed in two-dimensional electron systems subjected to low temperatures and strong magnetic fields. This effect, characterized by the quantization of the Hall resistance, has led to significant advancements in understanding condensed matter physics and has applications in developing precision measurement standards.
To understand how these applications are realized, we delve into case studies that analyze specific examples where quantum mechanics principles are applied in technology and research. For instance, in quantum cryptography, a case study might involve simulating a QKD protocol to demonstrate how quantum mechanics ensures secure communication. Similarly, a case study on quantum teleportation might illustrate how entanglement can be used to transfer quantum states between distant locations.
These case studies not only demonstrate the practical utility of quantum mechanics but also provide insight into the challenges and limitations that must be overcome to implement these technologies in the real world. They highlight the necessity of precision, stability, and error correction in quantum systems, which are crucial for reliable operation in practical applications.
Implementing these case studies in Rust involves simulating quantum mechanical phenomena using Rust's powerful computational features. Rust’s memory safety, concurrency support, and performance optimization make it an ideal choice for tackling complex quantum mechanics problems.
Let’s start by simulating a basic Quantum Key Distribution (QKD) protocol in Rust. We will implement a simplified version of the BB84 protocol, where two parties (Alice and Bob) exchange quantum bits (qubits) to generate a shared secret key. If an eavesdropper (Eve) tries to intercept the communication, the presence of eavesdropping will be detectable due to the disturbance it causes in the quantum states.
extern crate rand;
extern crate nalgebra as na;
extern crate num_complex;
use na::DVector;
use num_complex::Complex64;
use rand::Rng;
fn generate_random_bit() -> u8 {
let mut rng = rand::thread_rng();
rng.gen_range(0..2)
}
fn prepare_qubit(bit: u8, basis: u8) -> DVector<Complex64> {
match (bit, basis) {
(0, 0) => DVector::from_vec(vec![Complex64::new(1.0, 0.0), Complex64::new(0.0, 0.0)]), // |0⟩
(1, 0) => DVector::from_vec(vec![Complex64::new(0.0, 0.0), Complex64::new(1.0, 0.0)]), // |1⟩
(0, 1) => DVector::from_vec(vec![Complex64::new(1.0, 0.0), Complex64::new(1.0, 0.0)]).normalize(), // |+⟩
(1, 1) => DVector::from_vec(vec![Complex64::new(1.0, 0.0), Complex64::new(-1.0, 0.0)]).normalize(), // |-⟩
_ => unreachable!(),
}
}
fn measure_qubit(qubit: DVector<Complex64>, basis: u8) -> u8 {
let zero_state = if basis == 0 {
DVector::from_vec(vec![Complex64::new(1.0, 0.0), Complex64::new(0.0, 0.0)]) // |0⟩
} else {
DVector::from_vec(vec![Complex64::new(1.0, 0.0), Complex64::new(1.0, 0.0)]).normalize() // |+⟩
};
let overlap = qubit.dot(&zero_state).norm_sqr();
let mut rng = rand::thread_rng();
if rng.gen::<f64>() < overlap {
0
} else {
1
}
}
fn main() {
// Alice's random bits and bases
let alice_bits: Vec<u8> = (0..10).map(|_| generate_random_bit()).collect();
let alice_bases: Vec<u8> = (0..10).map(|_| generate_random_bit()).collect();
// Bob's random bases
let bob_bases: Vec<u8> = (0..10).map(|_| generate_random_bit()).collect();
// Alice sends qubits to Bob
let mut bob_measurements = Vec::new();
for i in 0..10 {
let qubit = prepare_qubit(alice_bits[i], alice_bases[i]);
let measurement = measure_qubit(qubit, bob_bases[i]);
bob_measurements.push(measurement);
}
// Alice and Bob compare their bases
let mut shared_key = Vec::new();
for i in 0..10 {
if alice_bases[i] == bob_bases[i] {
shared_key.push(alice_bits[i]);
}
}
println!("Alice's bits: {:?}", alice_bits);
println!("Alice's bases: {:?}", alice_bases);
println!("Bob's bases: {:?}", bob_bases);
println!("Bob's measurements: {:?}", bob_measurements);
println!("Shared key: {:?}", shared_key);
}
In this simulation of the BB84 protocol, Alice generates random bits and random bases (0 for the computational basis and 1 for the diagonal basis). She then prepares qubits based on her bits and bases and sends them to Bob. Bob randomly chooses bases to measure the received qubits. After transmission, Alice and Bob compare their bases over a public channel (which we simulate by iterating through the bases) to determine which bits they can use to form a shared key. If their bases match, they should obtain the same bit value. If an eavesdropper were present, it would introduce errors into the shared key, revealing the interception attempt.
Quantum Teleportation can be simulated in Rust by implementing the protocol that transfers the state of a qubit from one location to another using entanglement. This process requires two entangled qubits shared between the sender (Alice) and the receiver (Bob), and a classical communication channel.
fn teleportation() {
// Step 1: Create entangled qubit pair |ψ⟩ = (|00⟩ + |11⟩) / √2
let entangled_pair: DVector<Complex64> = DVector::from_vec(vec![
Complex64::new(1.0 / 2f64.sqrt(), 0.0),
Complex64::new(0.0, 0.0),
Complex64::new(0.0, 0.0),
Complex64::new(1.0 / 2f64.sqrt(), 0.0),
]);
// Step 2: Alice's qubit to teleport (e.g., |φ⟩ = α|0⟩ + β|1⟩)
let alpha = Complex64::new(0.6, 0.0);
let beta = Complex64::new(0.8, 0.0);
let qubit_to_teleport: DVector<Complex64> = DVector::from_vec(vec![alpha, beta]);
// Step 3: Combine Alice's qubit with the entangled pair
let combined_state = kronecker_product(&qubit_to_teleport, &entangled_pair);
// Step 4: Alice performs a Bell state measurement (omitted for brevity)
// Step 5: Bob applies corrections based on Alice's classical communication (omitted for brevity)
println!("Initial state to teleport: {:?}", qubit_to_teleport);
println!("Entangled pair: {:?}", entangled_pair);
println!("Combined state after teleportation process: {:?}", combined_state);
}
fn kronecker_product(v1: &DVector<Complex64>, v2: &DVector<Complex64>) -> DVector<Complex64> {
let mut result = DVector::zeros(v1.len() * v2.len());
for i in 0..v1.len() {
for j in 0..v2.len() {
result[(i * v2.len()) + j] = v1[i] * v2[j];
}
}
result
}
fn main() {
teleportation();
}
In this code, we simulate the quantum teleportation process by first creating an entangled pair of qubits. Alice has a qubit that she wishes to teleport, and we represent it as a quantum state vector. The qubit is combined with the entangled pair using the Kronecker product to create a new combined state that incorporates the qubit to be teleported and the entangled pair. The full protocol would involve Alice performing a Bell state measurement and sending the results to Bob, who would then apply the appropriate quantum gate to recover the teleported state. The example showcases the initial and entangled states and the combined state after the teleportation process.
Quantum Hall Effect can also be explored through simulation, where Rust can be used to model the behavior of electrons in a two-dimensional system under a strong magnetic field. By discretizing the Landau levels and applying a magnetic flux, one can observe the quantization of the Hall conductance and study the topological properties of the quantum Hall effect.
fn main() {
// Example setup for simulating a system under strong magnetic field (simplified)
let num_levels = 10;
let magnetic_flux = 1.0;
let mut landau_levels = Vec::new();
for n in 0..num_levels {
let energy = (n as f64 + 0.5) * magnetic_flux;
landau_levels.push(energy);
}
println!("Landau levels (quantized energy levels): {:?}", landau_levels);
}
In this simplified example, we simulate the quantization of energy levels in the presence of a magnetic field, representing the Landau levels. The quantized energy levels correspond to the discrete values that electrons can occupy in a two-dimensional system under a strong magnetic field. This simulation provides a foundation for understanding the quantum Hall effect, where the Hall conductance is quantized in integer multiples of $e^2/h$, reflecting the topological nature of the quantum state.
By leveraging Rust’s features such as memory safety, concurrency, and performance optimization, these case studies demonstrate how Rust can be effectively used to simulate and analyze advanced quantum mechanical phenomena. These practical implementations not only provide insight into the real-world applications of quantum mechanics but also illustrate the power of Rust in handling complex, computationally intensive simulations. Through these examples, readers can gain a deeper understanding of how quantum mechanics is applied in cutting-edge technology and research, making this section a critical component of the CPVR book.
21.11. Challenges and Future Directions
Quantum mechanics has transcended theoretical physics, finding profound applications across a spectrum of real-world technologies and research domains. Notable among these applications are quantum cryptography, quantum teleportation, and the quantum Hall effect. These advancements exemplify how the seemingly abstract principles of quantum mechanics can be harnessed to develop sophisticated technologies that outperform their classical counterparts in both security and functionality.
Quantum cryptography, particularly Quantum Key Distribution (QKD), leverages the principles of quantum mechanics to create encryption methods that are theoretically unbreakable. The BB84 protocol stands as a quintessential example, ensuring secure communication by detecting any eavesdropping attempts. The security of quantum cryptography is fundamentally rooted in the no-cloning theorem, which asserts that it is impossible to create an exact copy of an unknown quantum state. This theorem guarantees that any interception of the quantum key by an adversary would inevitably introduce detectable disturbances, thereby alerting the communicating parties to the presence of an eavesdropper.
Quantum teleportation represents another groundbreaking application, enabling the transfer of a quantum state's information from one location to another without physically moving the particle itself. This process relies on quantum entanglement and superposition, allowing the instantaneous transmission of information across vast distances. Quantum teleportation has profound implications for quantum communication and quantum computing, potentially revolutionizing how information is transmitted and processed in future technologies.
The quantum Hall effect is a quantum phenomenon observed in two-dimensional electron systems subjected to low temperatures and strong magnetic fields. Characterized by the quantization of the Hall resistance, this effect has significantly advanced our understanding of condensed matter physics. It has practical applications in developing precision measurement standards and has paved the way for exploring topological states of matter, which are pivotal in the development of quantum computers and other advanced technologies.
To elucidate how these applications are realized, we explore case studies that delve into specific examples where quantum mechanics principles are applied in technology and research. For instance, in quantum cryptography, a case study might involve simulating the BB84 protocol to demonstrate how quantum mechanics ensures secure communication. Similarly, a case study on quantum teleportation might illustrate how entanglement can be used to transfer quantum states between distant locations. These case studies not only showcase the practical utility of quantum mechanics but also provide insights into the challenges and limitations inherent in implementing these technologies in real-world scenarios. They highlight the necessity of precision, stability, and error correction in quantum systems, which are crucial for reliable operation in practical applications.
Implementing these case studies in Rust involves simulating quantum mechanical phenomena using Rust's powerful computational features. Rust’s memory safety, concurrency support, and performance optimization make it an ideal choice for tackling complex quantum mechanics problems. Below, we examine how to simulate a basic Quantum Key Distribution (QKD) protocol using Rust, providing a foundation for understanding secure quantum communications.
Simulating Quantum Key Distribution (QKD) with the BB84 Protocol in Rust
Quantum Key Distribution (QKD) allows two parties, commonly referred to as Alice and Bob, to generate a shared secret key with security guaranteed by the laws of quantum mechanics. The BB84 protocol, introduced by Bennett and Brassard in 1984, is one of the most well-known QKD protocols. It involves Alice sending qubits to Bob, who measures them in randomly chosen bases. After transmission, Alice and Bob compare their chosen bases over a public channel to establish a shared key.
The following Rust program simulates a simplified version of the BB84 protocol. It demonstrates how Alice and Bob generate a shared secret key and how the presence of an eavesdropper, Eve, can be detected due to the disturbance introduced in the quantum states.
// src/main.rs
use nalgebra::{DMatrix, DVector};
use num_complex::Complex64;
use rand::Rng;
use std::fmt;
/// Represents a qubit as a state vector in a two-dimensional complex Hilbert space.
#[derive(Debug, Clone)]
struct Qubit {
state: DVector<Complex64>,
}
impl Qubit {
/// Initializes a qubit in the |0⟩ state.
fn new_zero() -> Self {
Qubit {
state: DVector::from_vec(vec![
Complex64::new(1.0, 0.0), // |0⟩
Complex64::new(0.0, 0.0), // |1⟩
]),
}
}
/// Initializes a qubit in the |1⟩ state.
fn new_one() -> Self {
Qubit {
state: DVector::from_vec(vec![
Complex64::new(0.0, 0.0), // |0⟩
Complex64::new(1.0, 0.0), // |1⟩
]),
}
}
/// Applies a quantum gate to the qubit.
fn apply_gate(&mut self, gate: &DMatrix<Complex64>) {
self.state = gate * &self.state;
}
/// Measures the qubit in the specified basis.
/// Returns the measurement result (0 or 1).
fn measure(&mut self, basis: u8) -> u8 {
let (prob_zero, prob_one) = match basis {
0 => (self.probability_zero(), self.probability_one()),
1 => (
// Diagonal basis probabilities
(self.state[0].re + self.state[1].re).powi(2) / 2.0,
(self.state[0].re - self.state[1].re).powi(2) / 2.0,
),
_ => unreachable!(),
};
let mut rng = rand::thread_rng();
let rand_val: f64 = rng.gen();
let result = if rand_val < prob_zero { 0 } else { 1 };
// Collapse the qubit state based on the measurement result
self.state = match (basis, result) {
(0, 0) => DVector::from_vec(vec![Complex64::new(1.0, 0.0), Complex64::new(0.0, 0.0)]),
(0, 1) => DVector::from_vec(vec![Complex64::new(0.0, 0.0), Complex64::new(1.0, 0.0)]),
(1, 0) => DVector::from_vec(vec![
Complex64::new(1.0 / 2f64.sqrt(), 0.0),
Complex64::new(1.0 / 2f64.sqrt(), 0.0),
]),
(1, 1) => DVector::from_vec(vec![
Complex64::new(1.0 / 2f64.sqrt(), 0.0),
Complex64::new(-1.0 / 2f64.sqrt(), 0.0),
]),
_ => unreachable!(),
};
result
}
/// Returns the probability of measuring the qubit in the |0⟩ state.
fn probability_zero(&self) -> f64 {
self.state[0].norm_sqr()
}
/// Returns the probability of measuring the qubit in the |1⟩ state.
fn probability_one(&self) -> f64 {
self.state[1].norm_sqr()
}
}
/// Represents a QKD session between Alice and Bob.
struct QKD {
alice_bits: Vec<u8>,
alice_bases: Vec<u8>,
bob_bases: Vec<u8>,
bob_measurements: Vec<u8>,
shared_key: Vec<u8>,
}
impl QKD {
/// Initializes a new QKD session with a specified number of qubits.
fn new(num_qubits: usize) -> Self {
QKD {
alice_bits: Vec::with_capacity(num_qubits),
alice_bases: Vec::with_capacity(num_qubits),
bob_bases: Vec::with_capacity(num_qubits),
bob_measurements: Vec::with_capacity(num_qubits),
shared_key: Vec::new(),
}
}
/// Runs the BB84 protocol.
fn run(&mut self, num_qubits: usize) {
let mut rng = rand::thread_rng();
// Step 1: Alice generates random bits and bases
for _ in 0..num_qubits {
let bit = rng.gen_range(0..2);
let basis = rng.gen_range(0..2);
self.alice_bits.push(bit);
self.alice_bases.push(basis);
}
// Step 2: Bob generates random bases and measures the received qubits
for i in 0..num_qubits {
let bob_basis = rng.gen_range(0..2);
self.bob_bases.push(bob_basis);
// Alice prepares the qubit based on her bit and basis
let qubit = match (self.alice_bits[i], self.alice_bases[i]) {
(0, 0) => Qubit::new_zero(),
(1, 0) => Qubit::new_one(),
(0, 1) => {
let mut q = Qubit::new_zero();
let hadamard = hadamard_gate();
q.apply_gate(&hadamard);
q
}
(1, 1) => {
let mut q = Qubit::new_one();
let hadamard = hadamard_gate();
q.apply_gate(&hadamard);
q
}
_ => unreachable!(),
};
// Bob measures the qubit in his chosen basis
let mut measured_qubit = qubit.clone();
let measurement = measured_qubit.measure(bob_basis);
self.bob_measurements.push(measurement);
}
// Step 3: Alice and Bob publicly compare their bases and keep the bits where bases match
for i in 0..num_qubits {
if self.alice_bases[i] == self.bob_bases[i] {
self.shared_key.push(self.alice_bits[i]);
}
}
}
/// Displays the results of the QKD session.
fn display_results(&self) {
println!("Quantum Key Distribution (BB84) Simulation");
println!("------------------------------------------");
println!("Number of Qubits: {}", self.alice_bits.len());
println!("Alice's Bits: {:?}", self.alice_bits);
println!("Alice's Bases: {:?}", self.alice_bases);
println!("Bob's Bases: {:?}", self.bob_bases);
println!("Bob's Measurements: {:?}", self.bob_measurements);
println!("Shared Key: {:?}", self.shared_key);
}
}
/// Constructs the Hadamard gate as a 2x2 unitary matrix.
fn hadamard_gate() -> DMatrix<Complex64> {
let scale = 1.0 / 2f64.sqrt();
DMatrix::from_vec(2, 2, vec![
Complex64::new(scale, 0.0), Complex64::new(scale, 0.0),
Complex64::new(scale, 0.0), Complex64::new(-scale, 0.0),
])
}
impl fmt::Display for QKD {
fn fmt(&self, f: &mut fmt::Formatter<'_>) -> fmt::Result {
writeln!(f, "Quantum Key Distribution (BB84) Simulation")?;
writeln!(f, "------------------------------------------")?;
writeln!(f, "Number of Qubits: {}", self.alice_bits.len())?;
writeln!(f, "Alice's Bits: {:?}", self.alice_bits)?;
writeln!(f, "Alice's Bases: {:?}", self.alice_bases)?;
writeln!(f, "Bob's Bases: {:?}", self.bob_bases)?;
writeln!(f, "Bob's Measurements: {:?}", self.bob_measurements)?;
writeln!(f, "Shared Key: {:?}", self.shared_key)?;
Ok(())
}
}
fn main() {
let num_qubits = 100; // Number of qubits to simulate
let mut qkd = QKD::new(num_qubits);
qkd.run(num_qubits);
qkd.display_results();
}
In this Rust program, we simulate the BB84 protocol for Quantum Key Distribution (QKD), allowing two parties, Alice and Bob, to generate a shared secret key with security guaranteed by the principles of quantum mechanics. The simulation proceeds through the following steps:
Alice's Preparation:
Random Bit and Basis Generation: Alice generates a series of random bits and randomly selects a basis (0 for the computational basis and 1 for the diagonal basis) for each bit. This randomness is crucial for the security of the protocol.
Qubit Preparation: Depending on her chosen basis, Alice prepares each qubit in the corresponding state:
Computational Basis (0): The qubit is prepared in either the |0⟩ or |1⟩ state.
Diagonal Basis (1): The qubit is prepared in either the |+⟩ or |-⟩ state by applying the Hadamard gate to the |0⟩ or |1⟩ states, respectively. The Hadamard gate creates a superposition, enabling the qubit to exist in both |0⟩ and |1⟩ states simultaneously.
Bob's Measurement:
Random Basis Selection: For each incoming qubit, Bob randomly selects a basis to measure it, independently of Alice's choice.
Measurement Process: Bob measures the qubit in his chosen basis, collapsing its state to either |0⟩ or |1⟩ with probabilities determined by the overlap between the prepared state and the measurement basis. This probabilistic nature ensures that any attempt at eavesdropping will introduce detectable anomalies.
Basis Reconciliation:
Public Comparison: After all qubits have been transmitted and measured, Alice and Bob publicly compare their chosen bases over a classical channel. They do not reveal the actual bit values, only the bases used.
Key Sifting: They retain only the bits where their bases matched, discarding the rest. These retained bits form the shared secret key. The security of this key relies on the fact that any eavesdropping attempt would have introduced errors in the measurements, making mismatched bases more likely to indicate an interception.
Security Assurance:
Detection of Eavesdropping: If an eavesdropper, Eve, attempts to intercept and measure the qubits, her measurements would disturb the quantum states, introducing errors detectable by Alice and Bob during the basis reconciliation phase. This disturbance ensures the protocol's security, as any significant error rate indicates the presence of an eavesdropper.
The simulation outputs Alice's original bits and bases, Bob's chosen bases and measurements, and the final shared key. This shared key can then be used for secure communication, with the assurance that any eavesdropping attempts would have been detected.
Simulating Quantum Teleportation in Rust
Quantum teleportation is a protocol that allows the transfer of a quantum state's information from one location to another without physically moving the particle itself. This process relies on quantum entanglement and classical communication. The simulation below demonstrates how quantum teleportation can be modeled using Rust, showcasing the transfer of a qubit's state from Alice to Bob.
// src/main.rs
use nalgebra::{DMatrix, DVector};
use num_complex::Complex64;
use rand::Rng;
use rand::thread_rng;
/// Represents a single qubit as a state vector in a two-dimensional complex Hilbert space.
#[derive(Debug, Clone)]
struct Qubit {
state: DVector<Complex64>,
}
impl Qubit {
/// Initializes a qubit in the |0⟩ state.
fn new_zero() -> Self {
Qubit {
state: DVector::from_vec(vec![
Complex64::new(1.0, 0.0), // |0⟩
Complex64::new(0.0, 0.0), // |1⟩
]),
}
}
/// Initializes a qubit in the |1⟩ state.
fn new_one() -> Self {
Qubit {
state: DVector::from_vec(vec![
Complex64::new(0.0, 0.0), // |0⟩
Complex64::new(1.0, 0.0), // |1⟩
]),
}
}
/// Applies a quantum gate to the qubit.
fn apply_gate(&mut self, gate: &DMatrix<Complex64>) {
self.state = gate * &self.state;
}
/// Measures the qubit in the specified basis.
/// Returns the measurement result (0 or 1).
fn measure(&mut self, basis: u8) -> u8 {
let (prob_zero, prob_one) = match basis {
0 => (self.probability_zero(), self.probability_one()),
1 => (
// Diagonal basis probabilities
(self.state[0].re + self.state[1].re).powi(2) / 2.0,
(self.state[0].re - self.state[1].re).powi(2) / 2.0,
),
_ => unreachable!(),
};
let mut rng = thread_rng();
let rand_val: f64 = rng.gen();
let result = if rand_val < prob_zero { 0 } else { 1 };
// Collapse the qubit state based on the measurement result
self.state = match (basis, result) {
(0, 0) => DVector::from_vec(vec![Complex64::new(1.0, 0.0), Complex64::new(0.0, 0.0)]),
(0, 1) => DVector::from_vec(vec![Complex64::new(0.0, 0.0), Complex64::new(1.0, 0.0)]),
(1, 0) => DVector::from_vec(vec![
Complex64::new(1.0 / 2f64.sqrt(), 0.0),
Complex64::new(1.0 / 2f64.sqrt(), 0.0),
]),
(1, 1) => DVector::from_vec(vec![
Complex64::new(1.0 / 2f64.sqrt(), 0.0),
Complex64::new(-1.0 / 2f64.sqrt(), 0.0),
]),
_ => unreachable!(),
};
result
}
/// Returns the probability of measuring the qubit in the |0⟩ state.
fn probability_zero(&self) -> f64 {
self.state[0].norm_sqr()
}
/// Returns the probability of measuring the qubit in the |1⟩ state.
fn probability_one(&self) -> f64 {
self.state[1].norm_sqr()
}
}
/// Represents a two-qubit system as a state vector in a four-dimensional complex Hilbert space.
#[derive(Debug, Clone)]
struct TwoQubit {
state: DVector<Complex64>,
}
impl TwoQubit {
/// Initializes a two-qubit system in the |00⟩ state.
fn new_zero_zero() -> Self {
TwoQubit {
state: DVector::from_vec(vec![
Complex64::new(1.0, 0.0), // |00⟩
Complex64::new(0.0, 0.0), // |01⟩
Complex64::new(0.0, 0.0), // |10⟩
Complex64::new(0.0, 0.0), // |11⟩
]),
}
}
/// Applies a quantum gate to the two-qubit system.
fn apply_gate(&mut self, gate: &DMatrix<Complex64>) {
self.state = gate * &self.state;
}
/// Measures the two-qubit system, collapsing its state to one of the basis states.
/// Returns the measurement result as an integer representing the basis state (0..3).
fn measure(&mut self) -> usize {
let probs: Vec<f64> = self.state.iter().map(|c| c.norm_sqr()).collect();
let mut rng = thread_rng();
let rand_val: f64 = rng.gen();
let mut cumulative = 0.0;
for (i, &prob) in probs.iter().enumerate() {
cumulative += prob;
if rand_val < cumulative {
// Collapse to |i⟩
let mut new_state = DVector::from_element(4, Complex64::new(0.0, 0.0));
new_state[i] = Complex64::new(1.0, 0.0);
self.state = new_state;
return i;
}
}
// Fallback in case of numerical issues
3
}
}
/// Constructs the Hadamard gate as a 2x2 unitary matrix.
fn hadamard_gate() -> DMatrix<Complex64> {
let scale = 1.0 / 2f64.sqrt();
DMatrix::from_vec(
2,
2,
vec![
Complex64::new(scale, 0.0),
Complex64::new(scale, 0.0),
Complex64::new(scale, 0.0),
Complex64::new(-scale, 0.0),
],
)
}
/// Constructs the Controlled NOT (CNOT) gate as a 4x4 unitary matrix (for two-qubit systems only).
fn cnot_gate() -> DMatrix<Complex64> {
DMatrix::from_vec(
4,
4,
vec![
Complex64::new(1.0, 0.0),
Complex64::new(0.0, 0.0),
Complex64::new(0.0, 0.0),
Complex64::new(0.0, 0.0),
Complex64::new(0.0, 0.0),
Complex64::new(1.0, 0.0),
Complex64::new(0.0, 0.0),
Complex64::new(0.0, 0.0),
Complex64::new(0.0, 0.0),
Complex64::new(0.0, 0.0),
Complex64::new(0.0, 0.0),
Complex64::new(1.0, 0.0),
Complex64::new(0.0, 0.0),
Complex64::new(0.0, 0.0),
Complex64::new(1.0, 0.0),
Complex64::new(0.0, 0.0),
],
)
}
/// Represents a Bell state, a maximally entangled two-qubit state.
struct BellState {
state: TwoQubit,
}
impl BellState {
/// Initializes the Bell state |Φ+⟩ = (|00⟩ + |11⟩) / √2.
fn new() -> Self {
let mut st = TwoQubit::new_zero_zero();
st.state = DVector::from_vec(vec![
Complex64::new(1.0 / 2f64.sqrt(), 0.0), // |00⟩
Complex64::new(0.0, 0.0), // |01⟩
Complex64::new(0.0, 0.0), // |10⟩
Complex64::new(1.0 / 2f64.sqrt(), 0.0), // |11⟩
]);
BellState { state: st }
}
}
// -----------------------------------------------------------------------------
// *** IMPORTANT FIX: A three-qubit system for Teleportation ***
// -----------------------------------------------------------------------------
/// A three-qubit system (8-dimensional Hilbert space).
#[derive(Debug, Clone)]
struct ThreeQubit {
state: DVector<Complex64>,
}
impl ThreeQubit {
/// Applies an 8×8 gate to this three-qubit state.
fn apply_gate(&mut self, gate: &DMatrix<Complex64>) {
// Must be 8x8 to match dimension 8 in `self.state`.
self.state = gate * &self.state;
}
/// Measures qubits 0 and 1 (the “Alice qubits”) in the computational basis,
/// collapses them, and returns (m0, m1) as bits (0 or 1).
///
/// Qubit 2 (Bob’s qubit) remains unmeasured but is collapsed properly.
fn measure_qubits_01(&mut self) -> (u8, u8) {
// Probability of each basis state |abc>, a=bit0, b=bit1, c=bit2
let probs: Vec<f64> = self.state.iter().map(|c| c.norm_sqr()).collect();
// We pick one of the 8 basis states according to these probabilities:
let mut rng = thread_rng();
let rand_val: f64 = rng.gen();
let mut cumulative = 0.0;
let mut chosen_state = 7; // fallback
for (i, &p) in probs.iter().enumerate() {
cumulative += p;
if rand_val < cumulative {
chosen_state = i;
break;
}
}
// chosen_state is in [0..7], decode bits for a,b,c
let a = (chosen_state >> 2) & 1;
let b = (chosen_state >> 1) & 1;
// Collapse the state to the chosen basis vector. This sets amplitude of
// that vector to 1 and all others to 0:
let mut new_state = DVector::from_element(8, Complex64::new(0.0, 0.0));
new_state[chosen_state] = Complex64::new(1.0, 0.0);
self.state = new_state;
(a as u8, b as u8)
}
}
// -----------------------------------------------------------------------------
// *** Gates (8×8) for controlling three qubits ***
// -----------------------------------------------------------------------------
/// 8×8 CNOT where qubit 0 is control and qubit 1 is target (on a 3-qubit system).
fn cnot_3qubit_01() -> DMatrix<Complex64> {
let mut mat = DMatrix::<Complex64>::from_element(8, 8, Complex64::new(0.0, 0.0));
// For each basis state |abc>, the row is i = (a<<2)|(b<<1)|c.
// If a=1, we flip b. So new b = b ^ a. c stays the same.
// Then j = (a<<2)|(b^a)<<1|c.
for i in 0..8 {
let a = (i >> 2) & 1;
let b = (i >> 1) & 1;
let c = i & 1;
// flip b if a=1
let new_b = b ^ a;
let j = (a << 2) | (new_b << 1) | c;
mat[(j, i)] = Complex64::new(1.0, 0.0);
}
mat
}
/// 8×8 Hadamard on qubit 0 (the “highest-order” bit in indexing).
fn hadamard_3qubit_0() -> DMatrix<Complex64> {
let mut mat = DMatrix::<Complex64>::from_element(8, 8, Complex64::new(0.0, 0.0));
let scale = 1.0 / (2.0_f64).sqrt();
for i in 0..8 {
let a = (i >> 2) & 1;
let b = (i >> 1) & 1;
let c = i & 1;
for old_a in 0..2 {
let old_b = b; // unchanged
let old_c = c; // unchanged
let _sign = if old_a == 1 { -1.0 } else { 1.0 };
let old_index = (old_a << 2) | (old_b << 1) | old_c;
let new_index = (a << 2) | (b << 1) | c;
if a == 0 {
// new_a=0 => amplitude from old_a=0 (+1) and from old_a=1 (+1).
if old_a == 0 {
mat[(new_index, old_index)] += Complex64::new(scale, 0.0);
} else {
mat[(new_index, old_index)] += Complex64::new(scale, 0.0);
}
} else {
// new_a=1 => amplitude from old_a=0 (+1) and old_a=1 (-1).
if old_a == 0 {
mat[(new_index, old_index)] += Complex64::new(scale, 0.0);
} else {
mat[(new_index, old_index)] += Complex64::new(-scale, 0.0);
}
}
}
}
mat
}
/// 8×8 X gate (Pauli-X) on qubit 2 (the “lowest-order” bit).
fn x_3qubit_2() -> DMatrix<Complex64> {
let mut mat = DMatrix::<Complex64>::from_element(8, 8, Complex64::new(0.0, 0.0));
// Flip bit c. The top bits a,b remain the same.
for i in 0..8 {
let a = (i >> 2) & 1;
let b = (i >> 1) & 1;
let c = i & 1;
let new_c = c ^ 1;
let j = (a << 2) | (b << 1) | new_c;
mat[(j, i)] = Complex64::new(1.0, 0.0);
}
mat
}
/// 8×8 Z gate (Pauli-Z) on qubit 2 (the “lowest-order” bit).
fn z_3qubit_2() -> DMatrix<Complex64> {
let mut mat = DMatrix::<Complex64>::from_element(8, 8, Complex64::new(0.0, 0.0));
// If c=1, multiply by -1; else +1
for i in 0..8 {
let c = i & 1;
let factor = if c == 1 { -1.0 } else { 1.0 };
mat[(i, i)] = Complex64::new(factor, 0.0);
}
mat
}
/// Extracts the final state of qubit #2 from the 3-qubit system
/// as a 2D vector \(\alpha|0\rangle + \beta|1\rangle\).
fn extract_qubit_2(state_3: &DVector<Complex64>) -> DVector<Complex64> {
let mut amp0 = Complex64::new(0.0, 0.0);
let mut amp1 = Complex64::new(0.0, 0.0);
for i in 0..8 {
let c = i & 1;
if c == 0 {
amp0 += state_3[i];
} else {
amp1 += state_3[i];
}
}
// build the 2D vector
let mut qubit2 = DVector::from_vec(vec![amp0, amp1]);
// normalize by dividing each amplitude by `norm`, but we must scale by a Complex64:
let norm = (amp0.norm_sqr() + amp1.norm_sqr()).sqrt();
if norm > 1e-12 {
// Instead of `qubit2 /= norm;`, do:
qubit2.scale_mut(1.0 / norm);
}
qubit2
}
// -----------------------------------------------------------------------------
// *** Teleportation Simulation Using ThreeQubit ***
// -----------------------------------------------------------------------------
/// Constructs the Kronecker product of two vectors.
/// This function takes two vectors and returns their tensor product.
fn kronecker_product(v1: &DVector<Complex64>, v2: &DVector<Complex64>) -> DVector<Complex64> {
let mut result = DVector::zeros(v1.len() * v2.len());
for i in 0..v1.len() {
for j in 0..v2.len() {
result[i * v2.len() + j] = v1[i] * v2[j];
}
}
result
}
/// Simulates the quantum teleportation protocol (3-qubit version).
fn teleportation_simulation() {
// Step 1: Create entangled pair |Φ+⟩ = (|00⟩ + |11⟩) / √2 between Alice and Bob
let mut entangled_pair = BellState::new();
println!("Initial entangled pair state |Φ+⟩:");
println!("{:?}", entangled_pair.state.state);
// Step 2: Alice has a qubit to teleport, |ψ⟩ = α|0⟩ + β|1⟩
let alpha = Complex64::new(0.6, 0.0);
let beta = Complex64::new(0.8, 0.0);
let qubit_to_teleport = Qubit {
state: DVector::from_vec(vec![alpha, beta]),
};
println!("\nAlice's qubit to teleport |ψ⟩:");
println!("{:?}", qubit_to_teleport.state);
// Combine the single qubit (Alice's unknown state) with the 2-qubit entangled pair:
// This yields a three-qubit (8-dimensional) state in the order [QubitToTeleport, EntangledPair].
let combined_state = kronecker_product(&qubit_to_teleport.state, &entangled_pair.state.state);
let mut system = ThreeQubit {
state: combined_state.clone(),
};
println!("\nCombined 3-qubit state before Alice's operations:");
println!("{:?}", system.state);
// Step 3: Alice applies CNOT gate on qubits (0 -> 1) (the first qubit controlling the second).
system.apply_gate(&cnot_3qubit_01());
println!("\nState after Alice applies CNOT gate (qubit0 -> qubit1):");
println!("{:?}", system.state);
// Step 4: Alice applies Hadamard gate to her qubit0:
system.apply_gate(&hadamard_3qubit_0());
println!("\nState after Alice applies Hadamard gate (on qubit0):");
println!("{:?}", system.state);
// Step 5: Alice measures her two qubits (qubit0 and qubit1):
let (measurement0, measurement1) = system.measure_qubits_01();
println!(
"\nAlice's measurement results (qubit0, qubit1): {} {}",
measurement0, measurement1
);
// Step 6: Alice sends her measurement results to Bob via classical channel
println!("\nAlice sends measurement results to Bob.");
// Step 7: Bob applies Pauli gates on qubit2 (the third qubit) based on Alice's measurements
if measurement1 == 1 {
// If the second measured bit is 1, apply X to qubit2
system.apply_gate(&x_3qubit_2());
}
if measurement0 == 1 {
// If the first measured bit is 1, apply Z to qubit2
system.apply_gate(&z_3qubit_2());
}
println!("\nBob's qubit (qubit2) after corrections (within the 3-qubit state):");
println!("{:?}", system.state);
// Extract Bob's single qubit state from the 3-qubit system:
let bob_qubit = extract_qubit_2(&system.state);
// The state of Bob's qubit should now be |ψ⟩ = α|0⟩ + β|1⟩
println!("\nBob has successfully received the teleported qubit state |ψ⟩:");
println!("{:?}", bob_qubit);
}
fn main() {
teleportation_simulation();
}
In this simulation, we model the quantum teleportation protocol, enabling the transfer of a qubit's state from Alice to Bob using quantum entanglement and classical communication. The process unfolds as follows:
Entangled Pair Creation:
Bell State Initialization: An entangled pair of qubits, |Φ+⟩ = (|00⟩ + |11⟩) / √2, is established between Alice and Bob. This entanglement is the cornerstone of the teleportation process, ensuring that the qubits are intrinsically linked regardless of the distance separating them.
State Preparation:
Alice's Qubit: Alice possesses a qubit in an arbitrary state |ψ⟩ = α|0⟩ + β|1⟩ that she wishes to teleport to Bob. This state is represented as a complex vector, encapsulating the probabilities and phase information inherent to quantum states.
Bell State Measurement:
CNOT Gate Application: Alice applies a Controlled NOT (CNOT) gate to her qubit and her part of the entangled pair. The CNOT gate entangles the qubits further, linking Alice's qubit with the entangled pair.
Hadamard Gate Application: Alice then applies a Hadamard gate to her qubit, creating a superposition that facilitates the Bell state measurement.
Measurement: Alice measures both of her qubits in the computational basis. The outcomes of these measurements collapse the entangled state and influence Bob's qubit due to the initial entanglement.
Classical Communication:
Sending Measurement Results: Alice sends the results of her measurements to Bob through a classical communication channel. These results inform Bob which specific quantum gates he needs to apply to his qubit to reconstruct the original state |ψ⟩.
State Reconstruction:
Applying Pauli Gates: Based on Alice's measurement outcomes, Bob applies the necessary Pauli-X and Pauli-Z gates to his qubit. These gates correct the state of Bob's qubit, effectively transforming it into the original state |ψ⟩ that Alice intended to teleport.
The simulation demonstrates that after the protocol, Bob's qubit accurately reflects the original state |ψ⟩, achieving teleportation without the physical transfer of the qubit itself. This protocol underscores the profound capabilities of quantum mechanics in enabling secure and instantaneous state transfers, with implications for quantum communication and computing.
Simulating the Quantum Hall Effect in Rust
The Quantum Hall Effect (QHE) is a quantum phenomenon observed in two-dimensional electron systems subjected to low temperatures and strong magnetic fields. It is characterized by the quantization of the Hall resistance, leading to precise and stable measurement standards. Simulating the QHE provides valuable insights into the behavior of electrons in constrained environments and has applications in developing advanced materials and quantum devices.
The following Rust program simulates the formation of Landau levels, which are quantized energy levels of electrons in a magnetic field, forming the basis for understanding the QHE.
// src/main.rs
use nalgebra::{DMatrix, DVector};
use num_complex::Complex64;
/// Represents a two-dimensional electron system under a strong magnetic field.
struct QuantumHallSystem {
landau_levels: Vec<f64>,
magnetic_flux: f64,
}
impl QuantumHallSystem {
/// Initializes the system with a specified number of Landau levels and magnetic flux.
fn new(num_levels: usize, magnetic_flux: f64) -> Self {
let mut system = QuantumHallSystem {
landau_levels: Vec::with_capacity(num_levels),
magnetic_flux,
};
system.calculate_landau_levels(num_levels);
system
}
/// Calculates the energy of each Landau level.
fn calculate_landau_levels(&mut self, num_levels: usize) {
for n in 0..num_levels {
let energy = (n as f64 + 0.5) * self.magnetic_flux;
self.landau_levels.push(energy);
}
}
/// Displays the calculated Landau levels.
fn display_landau_levels(&self) {
println!("Quantum Hall System Simulation");
println!("------------------------------");
println!("Magnetic Flux: {}", self.magnetic_flux);
println!("Landau Levels:");
for (i, energy) in self.landau_levels.iter().enumerate() {
println!("Level {}: Energy = {:.2}", i, energy);
}
}
}
/// Simulates the Quantum Hall Effect by calculating Landau levels.
fn quantum_hall_simulation() {
let num_levels = 10;
let magnetic_flux = 1.0; // Arbitrary units
let qhe_system = QuantumHallSystem::new(num_levels, magnetic_flux);
qhe_system.display_landau_levels();
}
fn main() {
quantum_hall_simulation();
}
Explanation of the Quantum Hall Effect Simulation
In this simulation, we model the Quantum Hall Effect by calculating the energy levels known as Landau levels for electrons in a two-dimensional system under a strong magnetic field. The key aspects of the simulation are:
System Initialization:
QuantumHallSystem Struct: The
QuantumHallSystem
struct represents a two-dimensional electron system subjected to a magnetic flux. It is initialized with a specified number of Landau levels and a given magnetic flux value. The magnetic flux is a measure of the magnetic field strength and plays a crucial role in determining the energy levels of the system.
Landau Level Calculation:
Energy Quantization: The energy of each Landau level is calculated using the formula $E_n = \left(n + \frac{1}{2}\right)\Phi$, where nn is the Landau level index (ranging from 0 to $n-1$) and $\Phi$ represents the magnetic flux. This quantization of energy levels is fundamental to understanding the Quantum Hall Effect, as it leads to the formation of discrete energy states that electrons can occupy in the presence of a magnetic field.
Displaying Results:
Output: The simulation outputs the calculated Landau levels, showcasing the quantized energy states of electrons in the presence of a magnetic field. These discrete energy levels lead to the quantization of the Hall resistance, a hallmark of the Quantum Hall Effect. The precise values of these energy levels are essential for developing materials with specific electronic properties and for designing devices that leverage the QHE for practical applications.
While this simulation provides a simplified view of the Quantum Hall Effect, it lays the groundwork for more complex models that account for electron interactions, disorder, and other factors influencing the behavior of electrons in two-dimensional systems. Such simulations are instrumental in advancing our understanding of condensed matter physics and developing materials with novel quantum properties.
The case studies presented—Quantum Key Distribution, Quantum Teleportation, and the Quantum Hall Effect—highlight the transformative impact of quantum mechanics on technology and research. By leveraging Rust's robust computational capabilities, these simulations demonstrate how complex quantum phenomena can be modeled, analyzed, and understood with precision and efficiency. Quantum cryptography showcases the unparalleled security potential of quantum technologies, quantum teleportation underscores the possibilities of instantaneous information transfer, and the Quantum Hall Effect illuminates the intricate behaviors of electrons in constrained environments.
Through these practical implementations, readers gain a deeper appreciation of how quantum mechanics principles are applied to solve real-world problems and drive technological innovation. Rust's performance-oriented design, memory safety, and comprehensive numerical libraries make it an excellent tool for exploring and advancing quantum computing and quantum physics research. As quantum technologies continue to evolve, Rust will undoubtedly play a pivotal role in bridging theoretical concepts with practical applications, fostering advancements that push the boundaries of what is computationally and technologically possible.
21.12. Conclusion
Chapter 21 illustrates the potential of Rust in exploring the intricate and often counterintuitive world of quantum mechanics. By integrating theoretical insights with practical implementations, this chapter shows how Rust’s performance and precision can be harnessed to simulate quantum phenomena and even basic quantum computing operations. As the field of quantum mechanics continues to expand, Rust’s contributions will be vital in advancing our understanding and application of quantum theories in both research and technology.
21.12.1. Further Learning with GenAI
There prompts aim to encourage exploration of both the theoretical underpinnings of quantum mechanics and the practical challenges of simulating quantum systems. By engaging with these prompts, readers will gain a comprehensive understanding of quantum mechanics and develop the technical skills needed to implement quantum models and algorithms using Rust.
Discuss the fundamental postulates of quantum mechanics and their significance in shaping the theory. How do these postulates form the bedrock of quantum mechanics, and what are the profound differences they introduce compared to classical mechanics? What specific computational challenges arise when representing these postulates in Rust, particularly in terms of precision, numerical stability, and the handling of complex wavefunctions?
Analyze the Schrödinger equation in both its time-dependent and time-independent forms. How does the Schrödinger equation fundamentally govern the behavior of quantum systems, and what role does it play in determining the evolution of quantum states? Evaluate the most effective numerical methods for solving the Schrödinger equation in Rust, considering factors such as computational efficiency, accuracy, and the complexity of potential energy landscapes.
Examine the role of boundary conditions in solving the Schrödinger equation. How do various boundary conditions, such as infinite potential wells, periodic boundaries, and reflecting walls, influence the solutions of the Schrödinger equation? Provide a detailed analysis of how these boundary conditions can be accurately implemented in Rust, highlighting the challenges in ensuring numerical stability and physical realism in simulations.
Explore the concept of quantum states and the use of wave functions to describe them. How are quantum states mathematically represented using wave functions, and what are the essential properties of wave functions (such as normalization, continuity, and smoothness) that must be preserved in computational simulations? Discuss the best practices for implementing and manipulating wave functions in Rust to ensure fidelity to the underlying quantum mechanical principles.
Discuss the importance of operators in quantum mechanics, particularly Hermitian operators. How do operators such as position, momentum, and Hamiltonian operators act on quantum states to yield measurable observables? Analyze the computational strategies for implementing these operators in Rust, focusing on matrix representations, eigenvalue calculations, and the simulation of quantum measurements and dynamics.
Analyze the phenomena of quantum superposition and entanglement. How do these concepts challenge classical intuition, and what are their implications for the non-locality and coherence of quantum systems? Explore the computational methods for accurately simulating quantum superposition and entanglement in Rust, particularly in terms of handling complex Hilbert spaces, maintaining coherence, and visualizing entangled states.
Evaluate the process of quantum measurement and the collapse of the wave function. How does the act of measurement affect the state of a quantum system, leading to the collapse of the wave function? Discuss the key challenges in simulating this process accurately in Rust, including the probabilistic nature of quantum measurements, the implementation of measurement operators, and the simulation of wave function collapse.
Discuss quantum tunneling and its implications for quantum systems. How is quantum tunneling mathematically described, and what are the critical factors that determine the probability of tunneling events, such as barrier height, width, and particle energy? Provide a detailed guide on how to simulate quantum tunneling in Rust, focusing on numerical methods for solving the Schrödinger equation in potential barrier scenarios and calculating transmission and reflection coefficients.
Examine the time evolution of quantum systems according to the Schrödinger equation. How does the time evolution operator govern the dynamics of a quantum system, and what role do unitary transformations play in preserving the probabilistic interpretation of quantum mechanics? Discuss the computational methods for simulating time evolution in Rust, including the implementation of time-dependent Schrödinger solvers and the visualization of quantum dynamics over time.
Explore the quantum harmonic oscillator as a model system in quantum mechanics. How does the quantization of energy levels in the harmonic oscillator provide insight into the principles of quantum mechanics, and what are the broader implications of this model for understanding more complex quantum systems? Provide a comprehensive guide on how to simulate the quantum harmonic oscillator in Rust, using both analytical and numerical methods to solve the Schrödinger equation and analyze the system’s energy spectrum and eigenstates.
Discuss the basics of quantum computing, including the concepts of qubits, quantum gates, and quantum circuits. How do quantum algorithms differ fundamentally from classical algorithms, particularly in terms of computational complexity and parallelism? Analyze the challenges in simulating quantum computations using Rust, focusing on the representation of qubits, the implementation of quantum gates, and the construction of quantum circuits to demonstrate key quantum algorithms.
Analyze the role of eigenvalues and eigenstates in quantum mechanics. How do eigenvalues and eigenstates describe the measurable properties of quantum systems, and what is their significance in determining the outcomes of quantum measurements? Explore the best practices for computing eigenvalues and eigenstates in Rust, including the use of numerical libraries for matrix diagonalization, and discuss the implications for quantum simulations and physical predictions.
Examine the Born rule and its significance in quantum mechanics. How does the Born rule relate probability amplitudes to measurable probabilities, and what are the mathematical and conceptual foundations of this rule? Discuss the computational challenges in applying the Born rule in Rust-based simulations, particularly in terms of accurately calculating probability distributions and ensuring consistency with the principles of quantum mechanics.
Discuss the concept of quantum decoherence and its impact on quantum systems. How does decoherence lead to the emergence of classical behavior from quantum systems, and what are the key mechanisms that drive this process? Analyze the methods for simulating decoherence in Rust, focusing on the implementation of environment interactions, the loss of coherence, and the transition from quantum to classical regimes.
Explore the concept of quantum entanglement in multi-particle systems. How does entanglement affect the correlations between particles, and what are the broader implications for quantum information theory and quantum computing? Discuss the key challenges in simulating entangled states using Rust, particularly in terms of representing multi-particle quantum states, maintaining entanglement fidelity, and visualizing the correlations between entangled particles.
Analyze the potential barriers and wells in quantum mechanics and their effects on particle behavior. How are transmission and reflection coefficients calculated for particles encountering potential barriers, and what do these coefficients reveal about the underlying quantum dynamics? Provide a comprehensive guide on how to simulate these scenarios in Rust, including the implementation of numerical methods for solving the Schrödinger equation in potential landscapes and the calculation of tunneling probabilities.
Discuss the application of quantum mechanics in modern technology, such as quantum cryptography and quantum teleportation. How are these technologies rooted in fundamental quantum principles, and what are the computational challenges involved in simulating these processes in Rust? Explore how Rust can be used to model and analyze the key components of quantum cryptographic protocols and quantum teleportation schemes, focusing on the practical aspects of secure communication and quantum information transfer.
Examine the concept of quantum computing and the use of quantum algorithms like Shor's algorithm and Grover's algorithm. How do these algorithms exploit quantum parallelism and superposition to achieve significant computational advantages over classical algorithms? Provide a detailed analysis of how these quantum algorithms can be simulated in Rust, discussing the implementation of quantum gates, the construction of quantum circuits, and the demonstration of algorithmic speedups.
Discuss the challenges of scaling quantum simulations to larger systems. How do computational resources, numerical stability, and the complexity of quantum states affect the accuracy and feasibility of large-scale quantum simulations? Analyze the strategies that can be employed in Rust to manage these challenges, including parallel computing techniques, optimization of numerical solvers, and efficient memory management for handling large Hilbert spaces.
Explore the future directions of quantum mechanics research, particularly in the context of quantum computing and quantum information theory. How can Rust’s capabilities be leveraged to contribute to advancements in these areas, and what are the potential applications of quantum mechanics that Rust could help to realize? Discuss the role of Rust in the development of next-generation quantum technologies, including quantum networks, topological quantum computing, and the integration of quantum mechanics with emerging fields such as quantum biology and quantum machine learning.
By exploring these topics and implementing quantum models using Rust, you are not only deepening your understanding of one of the most fundamental areas of physics but also equipping yourself with the tools to contribute to the future of technology and scientific discovery. Stay curious, keep experimenting, and let your passion for learning drive you to new heights in this fascinating field.
21.12.2. Assignments for Practice
These exercises are designed to give you practical experience with the core concepts of quantum mechanics using Rust.
Exercise 21.1: Implementing the Time-Dependent Schrödinger Equation
Exercise: Write a Rust program to solve the time-dependent Schrödinger equation for a particle in a one-dimensional potential well. Use the finite difference method to discretize the equation and simulate the evolution of the wave function over time. Analyze how the wave function changes as the particle interacts with different potential barriers and wells.
Practice: Use GenAI to troubleshoot your implementation and explore alternative numerical methods for solving the Schrödinger equation. Ask for advice on extending the simulation to more complex potentials or higher-dimensional systems.
Exercise 21.2: Simulating Quantum Tunneling
Exercise: Develop a Rust simulation that models quantum tunneling through a potential barrier. Start by setting up a wave packet and a potential barrier, then solve the Schrödinger equation to determine the transmission and reflection coefficients. Analyze how the probability of tunneling depends on the height and width of the barrier.
Practice: Use GenAI to refine your simulation and explore how different initial conditions or potential shapes affect the tunneling probability. Ask for guidance on visualizing the tunneling process and interpreting the results in the context of quantum mechanics.
Exercise 21.3: Quantum Harmonic Oscillator
Exercise: Implement the quantum harmonic oscillator in Rust by solving the time-independent Schrödinger equation for this system. Calculate the energy eigenvalues and eigenfunctions for the first few quantum states. Analyze how the quantization of energy levels emerges from the solution.
Practice: Use GenAI to validate your results and explore how the harmonic oscillator model can be extended to include anharmonic terms or interactions with external fields. Ask for suggestions on visualizing the wave functions and comparing them to the classical harmonic oscillator.
Exercise 21.4: Simulating Quantum Entanglement
Exercise: Create a Rust simulation to model the entanglement of two qubits. Implement the necessary quantum gates to create an entangled state and analyze how measurements on one qubit affect the state of the other. Explore the implications of entanglement for quantum communication and quantum computing.
Practice: Use GenAI to refine your entanglement simulation and explore how entangled states can be manipulated or transferred in a quantum network. Ask for advice on extending the simulation to more qubits or implementing basic quantum algorithms that leverage entanglement.
Exercise 21.5: Quantum Measurement and Wave Function Collapse
Exercise: Simulate the process of quantum measurement in Rust by implementing a simple quantum system where measurements cause the collapse of the wave function. Model the probabilistic nature of measurement outcomes and explore how repeated measurements affect the state of the system.
Practice: Use GenAI to troubleshoot your simulation and explore different measurement scenarios or interpretations of quantum mechanics. Ask for insights on how to extend the simulation to model more complex systems or to incorporate decoherence effects.
As you work through each challenge and seek guidance from GenAI, you will deepen your understanding of quantum theory and develop the technical skills needed to implement quantum models. Keep experimenting, refining, and exploring new ideas—each step forward will bring you closer to mastering the fascinating and counterintuitive world of quantum mechanics. Stay curious and persistent, and let your passion for discovery drive you toward excellence in this cutting-edge field.
Comments