Chapter 17
Molecular Dynamics Simulations
"Computers are incredibly fast, accurate, and stupid. Humans are incredibly slow, inaccurate, and brilliant. Together they are powerful beyond imagination." — Albert Einstein
Chapter 17 of CPVR provides an in-depth exploration of Molecular Dynamics (MD) simulations, highlighting how Rust’s features—such as memory safety, concurrency, and performance optimization—make it an ideal choice for implementing these complex simulations. The chapter begins with the fundamentals of MD, covering the mathematical models, force fields, and data structures essential for accurate simulations. It then delves into practical aspects like parallelization, numerical integration, and data analysis, demonstrating how Rust’s capabilities can be harnessed to create robust, scalable MD simulations. Real-world case studies illustrate the application of MD in various scientific domains, and the chapter concludes by addressing the current challenges and future directions for MD, emphasizing Rust's role in overcoming these obstacles.
17.1. Introduction to Molecular Dynamics Simulations
Molecular Dynamics (MD) simulations are a pivotal computational tool in modern physics, chemistry, and materials science. By simulating the physical movements of atoms and molecules, MD enables researchers to explore and predict the behavior of complex systems at an atomic level. These simulations are grounded in the principles of Newtonian mechanics, where particles interact through defined force fields, allowing the study of phenomena that are difficult or impossible to observe experimentally. The role of MD in computational physics is vast, encompassing areas such as understanding material properties, drug design, protein folding, and nanotechnology.
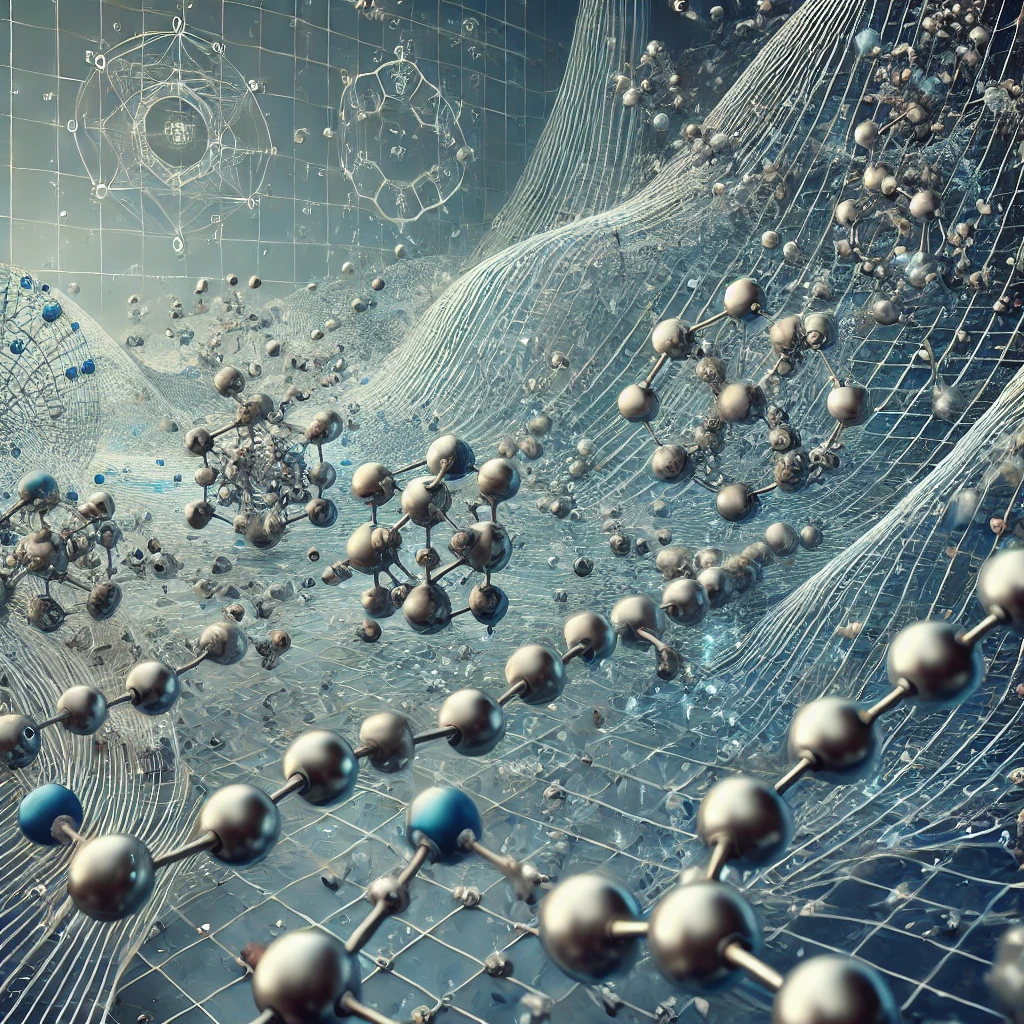
DALL-E generated image for illustration.
MD simulations provide insights into the dynamics of molecular systems over time, offering a detailed view of how particles move and interact. This capability is crucial in fields like materials science, where the properties of materials at the atomic scale determine their macroscopic behavior. In chemistry, MD simulations are used to study reactions, molecular structures, and the diffusion of atoms and molecules. In biophysics, they help in understanding complex biological processes such as protein folding, membrane dynamics, and enzyme activity. The relevance of MD across these diverse fields underscores its importance in advancing scientific knowledge and technological innovation.
The development of MD simulations has a rich history, starting from the early computational efforts in the mid-20th century. Significant milestones include the first computer simulations of liquids by Alder and Wainwright in the 1950s, which laid the groundwork for modern MD techniques. Over the decades, advancements in algorithms, computational power, and software development have transformed MD into a powerful tool for exploring molecular systems. The introduction of force fields like CHARMM and AMBER in the 1980s and the development of efficient integration algorithms have significantly enhanced the accuracy and applicability of MD simulations.
At the core of MD simulations lies Newton's laws of motion, which govern the dynamics of particles in a system. According to Newton's second law, the force acting on a particle is equal to the mass of the particle multiplied by its acceleration. In an MD simulation, this principle is used to calculate the forces acting on each particle based on the interactions with its neighbors. These forces are then used to update the positions and velocities of the particles over time, allowing the simulation to evolve and generate a trajectory of the system.
The integration of equations of motion is a critical aspect of MD simulations. Since the forces acting on particles are time-dependent, the equations of motion must be integrated over discrete time steps to simulate the system's dynamics. Common integration methods include the Verlet and Velocity-Verlet algorithms, which provide a balance between computational efficiency and accuracy. These methods ensure that the particles' trajectories are accurately computed over time, preserving key physical properties such as energy and momentum.
Potential energy landscapes play a crucial role in determining the behavior of molecular systems. In MD simulations, the potential energy of a system is defined by the interactions between particles, which can include bonded interactions (such as bonds, angles, and torsions) and non-bonded interactions (such as van der Waals forces and electrostatic interactions). The shape of the potential energy landscape influences how particles move and interact, determining the stability of molecular structures and the pathways of chemical reactions. Understanding and accurately modeling these landscapes is essential for realistic and meaningful MD simulations.
Rust's programming language is particularly well-suited for implementing MD simulations due to its strong emphasis on safety, concurrency, and performance. Rust’s type safety ensures that common programming errors, such as null pointer dereferencing or buffer overflows, are caught at compile time, reducing the risk of runtime errors in complex simulations. This feature is crucial when dealing with large-scale MD simulations where errors can propagate and significantly impact the results.
Memory management in Rust is another key advantage. Rust’s ownership model ensures that memory is efficiently allocated and deallocated without the need for a garbage collector, which is particularly important in simulations where large arrays of particle data are continuously updated. This model allows for deterministic memory management, ensuring that resources are released as soon as they are no longer needed, thus avoiding memory leaks and other issues that can degrade performance in long-running simulations.
To demonstrate these concepts, consider the following Rust code that sets up a simple MD simulation for a system of particles:
// Import necessary crates
use rand::Rng; // For random number generation
// Define a struct to represent a particle
struct Particle {
position: [f64; 3], // 3D position coordinates
velocity: [f64; 3], // 3D velocity components
mass: f64, // Mass of the particle
}
// Function to initialize particles with random positions and zero velocities
fn initialize_particles(n: usize) -> Vec<Particle> {
let mut particles = Vec::with_capacity(n); // Pre-allocate vector for efficiency
let mut rng = rand::thread_rng(); // Initialize random number generator
for _ in 0..n {
// Generate random positions within a unit cube
let position = [
rng.gen_range(0.0..1.0),
rng.gen_range(0.0..1.0),
rng.gen_range(0.0..1.0),
];
// Initialize velocities to zero
let velocity = [0.0, 0.0, 0.0];
let mass = 1.0; // Assign a default mass
// Create a new particle and add it to the vector
particles.push(Particle { position, velocity, mass });
}
particles
}
fn main() {
let n_particles = 1000; // Number of particles in the simulation
let particles = initialize_particles(n_particles); // Initialize particles
// Placeholder for further simulation steps, such as force calculations and integration
// e.g., calculate_forces(&mut particles);
// integrate(&mut particles, time_step);
// Output initial state for verification
println!("Initialized {} particles.", n_particles);
// Optionally, print the first few particles
for (i, particle) in particles.iter().enumerate().take(5) {
println!(
"Particle {}: Position = {:?}, Velocity = {:?}, Mass = {}",
i + 1,
particle.position,
particle.velocity,
particle.mass
);
}
}
This code initializes a set of particles with random positions in a 3D space and zero initial velocity. The Particle
struct holds the position, velocity, and mass of each particle, while the initialize_particles
function creates a vector of particles, each with randomly generated positions. Rust’s Vec
type is used to store the particles, providing a dynamic array that can efficiently manage the particle data.
In this simple example, Rust’s safety features ensure that the particle data is correctly initialized and managed. The rand
crate is used to generate random positions, demonstrating how Rust’s ecosystem of libraries can be leveraged to implement common tasks in MD simulations. This basic setup can be expanded with additional functionality, such as force calculations, time integration, and output of simulation data.
The efficiency of Rust’s memory management becomes evident when scaling up the number of particles or adding more complex interactions. As the simulation grows in complexity, Rust’s ownership model ensures that memory is used efficiently, avoiding the pitfalls of manual memory management that are common in languages like C or C++. Furthermore, Rust’s concurrency model allows for parallel processing of particle interactions, enabling the simulation to run efficiently on multi-core processors.
Overall, this section provides a robust introduction to the fundamental and conceptual domains of MD simulations, while also offering practical insights into how these simulations can be implemented in Rust. The sample code illustrates the basic principles of setting up an MD simulation and highlights the advantages of using Rust for this purpose. As the chapter progresses, more advanced features and optimizations will be introduced, building on this foundational knowledge to create a comprehensive MD simulation framework in Rust.
17.2. Mathematical Foundations
Molecular Dynamics (MD) simulations are fundamentally anchored in the principles of Newtonian mechanics. Central to these simulations is Newton’s second law of motion, which articulates that the force acting on a particle is equal to the mass of the particle multiplied by its acceleration $(F = ma)$. This core principle is employed to calculate the forces exerted on particles within a molecular system based on their interactions with neighboring particles. In an MD simulation, particles are modeled as point masses, and their trajectories are determined by numerically integrating the equations of motion over discrete time steps. The forces acting on each particle are derived from the system's potential energy, which encapsulates the interactions between all pairs of particles.
Newtonian mechanics provides a robust framework for comprehending the behavior of particles at the atomic level. It enables the prediction of particle movements under the influence of forces generated by their interactions with other particles. The precise application of these principles is crucial for the realism and reliability of MD simulations, facilitating the study of dynamic behaviors in complex molecular systems.
In MD simulations, these principles are extrapolated to systems comprising numerous particles. For each particle, forces are computed, and positions and velocities are subsequently updated. This iterative process, repeated over millions of time steps, allows researchers to simulate the system's evolution over time, capturing the intricate dynamic processes that occur at the atomic scale.
Beyond Newtonian mechanics, MD simulations are underpinned by the laws of thermodynamics and the principles of statistical mechanics. Thermodynamics governs macroscopic properties such as energy, work, and entropy, while statistical mechanics bridges the gap between microscopic particle behavior and macroscopic thermodynamic quantities. In the context of MD simulations, statistical mechanics facilitates the calculation of thermodynamic properties from the microscopic details of particle interactions and movements.
For example, temperature in an MD simulation correlates with the average kinetic energy of the particles, while pressure can be derived from the forces between particles and their spatial distribution within the simulation box. These macroscopic quantities are essential for interpreting MD simulation results and relating them to empirical experimental data.
Statistical mechanics also provides the foundation for understanding ensemble averages, which are utilized to compute physical properties from MD simulations. By conducting simulations over sufficiently long durations or averaging results across multiple simulations, it is possible to obtain statistically significant outcomes that correspond to thermodynamic quantities like temperature, pressure, and free energy.
A pivotal aspect of MD simulations is the integration of equations of motion over time. The selection of an appropriate time integration algorithm is critical, as it directly impacts the simulation's accuracy and stability. Commonly employed algorithms in MD simulations include the Verlet, Velocity-Verlet, and Leapfrog methods.
The Verlet algorithm is favored for its simplicity and numerical stability. It computes a particle's new position based on its current position, previous position, and the force acting upon it. This method is particularly valued for its energy conservation properties, which are vital for maintaining the integrity of long-running simulations.
The Velocity-Verlet algorithm builds upon the basic Verlet method by also updating particle velocities, enabling more accurate calculations of kinetic energy. This approach ensures that both positions and velocities are updated in a consistent manner, providing a more precise representation of the system’s dynamics.
The Leapfrog algorithm, aptly named for its staggered updates of positions and velocities, offers another method for integrating the equations of motion. It updates velocities at half time steps and positions at full time steps, achieving a balance between accuracy and computational efficiency.
Conservation of energy and momentum is a fundamental requirement in MD simulations. These conservation laws ensure that the simulation faithfully represents the physical behavior of the system. The integration algorithms mentioned above are designed to preserve these quantities as accurately as possible throughout the simulation.
Stability criteria are also paramount in MD simulations, particularly when selecting the time step for the integration algorithm. The time step must be sufficiently small to accurately capture the fastest motions in the system, such as bond vibrations, while being large enough to allow the simulation to progress over meaningful timescales. A time step that is too large can lead to numerical instability, resulting in erroneous simulation outcomes or even simulation failure.
Implementing these mathematical foundations in Rust necessitates meticulous attention to accuracy, performance, and numerical stability. Rust’s stringent type system and memory safety features make it an ideal language for developing reliable and efficient MD simulations.
To illustrate these concepts, consider the following Rust code that implements the Velocity-Verlet integration algorithm for a simple system of particles:
// Import necessary crates
use rand::Rng; // For random number generation
// Define a struct to represent a particle
#[derive(Debug)]
struct Particle {
position: [f64; 3], // 3D position coordinates
velocity: [f64; 3], // 3D velocity components
force: [f64; 3], // 3D force components
mass: f64, // Mass of the particle
}
impl Particle {
// Constructor to create a new Particle
fn new(position: [f64; 3], velocity: [f64; 3], mass: f64) -> Self {
Self {
position,
velocity,
force: [0.0, 0.0, 0.0],
mass,
}
}
// Method to update the velocity based on acceleration
fn update_velocity(&mut self, acceleration: [f64; 3], time_step: f64) {
for i in 0..3 {
self.velocity[i] += acceleration[i] * time_step;
}
}
// Method to update the position based on velocity and acceleration
fn update_position(&mut self, time_step: f64) {
for i in 0..3 {
self.position[i] += self.velocity[i] * time_step + 0.5 * self.force[i] / self.mass * time_step.powi(2);
}
}
// Method to reset the force to zero before recalculating
fn reset_force(&mut self) {
self.force = [0.0, 0.0, 0.0];
}
}
// Function to initialize particles with random positions and zero velocities
fn initialize_particles(n: usize) -> Vec<Particle> {
let mut particles = Vec::with_capacity(n); // Pre-allocate vector for efficiency
let mut rng = rand::thread_rng(); // Initialize random number generator
for _ in 0..n {
// Generate random positions within a specified range
let position = [
rng.gen_range(0.0..10.0),
rng.gen_range(0.0..10.0),
rng.gen_range(0.0..10.0),
];
// Initialize velocities to zero
let velocity = [0.0, 0.0, 0.0];
let mass = 1.0; // Assign a default mass
// Create a new particle and add it to the vector
particles.push(Particle::new(position, velocity, mass));
}
particles
}
// Function to calculate forces between particles (e.g., using a simple harmonic potential)
fn calculate_forces(particles: &mut Vec<Particle>) {
// Reset all forces before calculation
for particle in particles.iter_mut() {
particle.reset_force();
}
// Simple pairwise force calculation (e.g., Hooke's law for demonstration)
for i in 0..particles.len() {
for j in (i + 1)..particles.len() {
let dx = particles[j].position[0] - particles[i].position[0];
let dy = particles[j].position[1] - particles[i].position[1];
let dz = particles[j].position[2] - particles[i].position[2];
// Simple linear spring force
let k = 0.1; // Spring constant
let fx = k * dx;
let fy = k * dy;
let fz = k * dz;
// Update forces based on Newton's third law
particles[i].force[0] += fx;
particles[i].force[1] += fy;
particles[i].force[2] += fz;
particles[j].force[0] -= fx;
particles[j].force[1] -= fy;
particles[j].force[2] -= fz;
}
}
}
fn main() {
let n_particles = 1000; // Number of particles in the simulation
let time_step = 0.01; // Time step for integration
let total_steps = 100; // Total number of simulation steps
let mut particles = initialize_particles(n_particles); // Initialize particles
for step in 0..total_steps {
// Calculate forces based on current positions
calculate_forces(&mut particles);
// Update positions based on current velocities and forces
for particle in particles.iter_mut() {
particle.update_position(time_step);
}
// Recalculate forces after position update
calculate_forces(&mut particles);
// Update velocities based on the new forces
for particle in particles.iter_mut() {
let acceleration = [
particle.force[0] / particle.mass,
particle.force[1] / particle.mass,
particle.force[2] / particle.mass,
];
particle.update_velocity(acceleration, time_step);
}
// Optional: Output simulation data at specific intervals
if step % 10 == 0 {
println!("Step {}:", step);
for (i, particle) in particles.iter().enumerate().take(5) {
println!(
"Particle {}: Position = {:?}, Velocity = {:?}, Force = {:?}",
i + 1,
particle.position,
particle.velocity,
particle.force
);
}
}
}
println!("Simulation completed.");
}
The provided Rust code implements the Velocity-Verlet integration algorithm, a widely used method in MD simulations due to its balance between accuracy and computational efficiency. The Particle
struct encapsulates the essential properties of each particle, including position, velocity, force, and mass. The initialize_particles
function generates a specified number of particles with random initial positions within a defined spatial range and zero initial velocities.
The calculate_forces
function demonstrates a simple pairwise force calculation using Hooke's law, where particles are connected by linear springs. This is a placeholder for more complex potential energy functions that might be used in realistic simulations, such as Lennard-Jones potentials or Coulombic interactions. Before calculating new forces, the function resets all forces to zero to ensure accurate force accumulation during each iteration.
The verlet_integration
method within the Particle
struct has been expanded into two separate methods: update_position
and update_velocity
. This separation enhances modularity and clarity, allowing for distinct updates of positions and velocities based on current forces and accelerations.
In the main
function, the simulation runs for a defined number of steps. At each step, forces are recalculated based on the current positions of the particles. Subsequently, particle positions are updated using the update_position
method. After updating positions, forces are recalculated to reflect the new configurations. Velocities are then updated based on the newly computed forces, completing the Velocity-Verlet integration cycle.
Periodic output of simulation data is included for verification purposes, printing the state of the first few particles at specified intervals. This aids in monitoring the simulation's progress and ensuring that the integration steps are functioning correctly.
This section delves into the mathematical underpinnings of MD simulations, emphasizing the critical role of Newtonian mechanics, thermodynamics, and statistical mechanics in modeling atomic-level interactions. The accompanying Rust code provides a practical example of implementing the Velocity-Verlet integration algorithm, showcasing Rust's strengths in ensuring safety, efficiency, and scalability.
17.3. Force Fields and Interaction Potentials
In Molecular Dynamics (MD) simulations, accurately representing the forces between particles is crucial for modeling the physical behavior of a system. These forces are typically categorized into bonded and non-bonded interactions. Bonded forces originate from interactions between atoms that are chemically bonded, encompassing bond stretching, angle bending, and dihedral torsions. For example, bond stretching forces are described by harmonic potentials that model the behavior of bonds as they deviate from their equilibrium lengths. Similarly, angle bending forces account for the energy associated with the deviation of bond angles from their equilibrium values, and dihedral forces describe the rotation around bonds connecting atoms in a molecular chain.
Non-bonded forces occur between atoms that are not directly bonded but interact through van der Waals forces, electrostatic interactions, or other long-range forces. The Lennard-Jones potential is a prevalent model for van der Waals interactions, capturing both the attractive and repulsive forces between atoms. Coulomb interactions, on the other hand, describe the electrostatic forces between charged particles, which can be either attractive or repulsive depending on the charges involved.
The Lennard-Jones potential is defined by the equation:
$$V_{LJ}(r) = 4\epsilon \left[ \left(\frac{\sigma}{r}\right)^{12} - \left(\frac{\sigma}{r}\right)^{6} \right]$$
Here, $r$ is the distance between two particles, $\epsilon$ represents the depth of the potential well indicating the strength of the interaction, and $\sigma$ is the distance at which the potential is zero. The $r^{-12}$ term represents the repulsive forces that dominate at short distances, preventing particles from collapsing into each other, while the $r^{-6}$ term represents the attractive van der Waals forces.
Coulomb interactions are modeled using Coulomb's law:
$$V_{C}(r) = \frac{q_1 q_2}{4\pi\epsilon_0 r}$$
In this equation, $q_1$ and $q_2$ are the charges of the interacting particles, $r$ is the distance between them, and $\epsilon_0$ is the permittivity of free space. Coulomb interactions are essential for modeling systems with charged species, such as ionic solutions or biomolecules.
Energy minimization is a critical step in MD simulations, particularly during the initial stages, to bring the system to a stable configuration. The objective of energy minimization is to identify the configuration of particles that corresponds to the lowest potential energy, representing the most stable arrangement of atoms or molecules. During this process, the forces acting on particles are iteratively adjusted to reduce the total potential energy of the system. This is typically achieved using algorithms like steepest descent or conjugate gradient methods, which are effective in eliminating any initial overlaps or unrealistic configurations introduced during the simulation setup.
Force calculation is a central component of MD simulations, determining how particles interact and move over time. The force on each particle is calculated as the negative gradient of the potential energy with respect to the particle's position:
$${F}_i = -\nabla V(\mathbf{r}_i)$$
For the Lennard-Jones potential, the force between two particles is given by:
$$\mathbf{F}_{LJ}(r) = 24\epsilon \left[2\left(\frac{\sigma}{r}\right)^{12} - \left(\frac{\sigma}{r}\right)^{6}\right] \frac{\mathbf{r}}{r^2}$$
For Coulomb interactions, the force is calculated as:
$$\mathbf{F}_{C}(r) = \frac{q_1 q_2}{4\pi\epsilon_0} \frac{\mathbf{r}}{r^3}$$
These force calculations are repeated for every pair of interacting particles at each time step of the simulation, which can be computationally intensive for large systems.
Parameterization of force fields involves selecting appropriate parameters for potentials like Lennard-Jones and Coulomb interactions. These parameters ($\epsilon$, $\sigma$, and charges) are often derived from empirical data, experimental results, or quantum mechanical calculations. Accurate parameterization is crucial for ensuring that the simulation reflects the real behavior of the system being modeled. The choice of parameters can significantly affect the simulation's accuracy. For instance, slight variations in the values of $\epsilon$ and $\sigma$ in the Lennard-Jones potential can alter the balance between attractive and repulsive forces, leading to different structural and dynamic properties in the simulated system.
Modeling complex systems often requires combining different force fields to accurately represent various types of interactions within the system. For example, a simulation of a biomolecular system might integrate bonded interactions for covalent bonds with non-bonded interactions for van der Waals and electrostatic forces. Ensuring that these different force fields are compatible and accurately represent the physical properties of the system is a significant challenge. This involves careful parameterization and testing of the combined force field against experimental data or high-level computational results. The use of hybrid force fields, where different types of potentials are applied to different parts of the system, is common in complex simulations.
Implementing force fields in Rust requires careful consideration of both efficiency and accuracy. Rust’s powerful type system and memory management features can be leveraged to create efficient and reliable force field calculations.
Consider the following Rust code for implementing the Lennard-Jones potential:
// Import necessary crates
use rand::Rng; // For random number generation
use rayon::prelude::*; // For parallel processing
// Define a struct to represent a particle
#[derive(Debug)]
struct Particle {
position: [f64; 3], // 3D position coordinates
force: [f64; 3], // 3D force components
charge: f64, // Charge of the particle
}
impl Particle {
// Constructor to create a new Particle
fn new(position: [f64; 3], charge: f64) -> Self {
Self {
position,
force: [0.0, 0.0, 0.0],
charge,
}
}
// Method to reset the force to zero before recalculating
fn reset_force(&mut self) {
self.force = [0.0, 0.0, 0.0];
}
}
// Function to calculate the Lennard-Jones force between two particles
fn lennard_jones(p1: &Particle, p2: &Particle, epsilon: f64, sigma: f64) -> [f64; 3] {
let mut r_vec = [0.0; 3];
let mut r2 = 0.0;
// Calculate the distance vector and its squared magnitude
for i in 0..3 {
r_vec[i] = p1.position[i] - p2.position[i];
r2 += r_vec[i] * r_vec[i];
}
if r2 == 0.0 {
return [0.0, 0.0, 0.0]; // Avoid division by zero
}
let r2_inv = 1.0 / r2;
let r6_inv = r2_inv * r2_inv * r2_inv;
let force_scalar = 24.0 * epsilon * r6_inv * (2.0 * r6_inv - 1.0) * r2_inv;
let mut force = [0.0; 3];
for i in 0..3 {
force[i] = force_scalar * r_vec[i];
}
force
}
// Function to calculate the Coulomb force between two particles
fn coulomb_force(p1: &Particle, p2: &Particle, k_e: f64) -> [f64; 3] {
let mut r_vec = [0.0; 3];
let mut r2 = 0.0;
// Calculate the distance vector and its squared magnitude
for i in 0..3 {
r_vec[i] = p1.position[i] - p2.position[i];
r2 += r_vec[i] * r_vec[i];
}
if r2 == 0.0 {
return [0.0, 0.0, 0.0]; // Avoid division by zero
}
let r = r2.sqrt();
let r3_inv = 1.0 / (r2 * r);
let force_scalar = k_e * p1.charge * p2.charge * r3_inv;
let mut force = [0.0; 3];
for i in 0..3 {
force[i] = force_scalar * r_vec[i];
}
force
}
// Function to apply forces to all particles using the Lennard-Jones potential
fn apply_lennard_jones_forces(particles: &mut Vec<Particle>, epsilon: f64, sigma: f64) {
let n = particles.len();
for i in 0..n {
for j in (i + 1)..n {
let force = lennard_jones(&particles[i], &particles[j], epsilon, sigma);
for k in 0..3 {
particles[i].force[k] += force[k];
particles[j].force[k] -= force[k]; // Newton's third law
}
}
}
}
// Function to apply Coulomb forces to all particles
fn apply_coulomb_forces(particles: &mut Vec<Particle>, k_e: f64) {
let n = particles.len();
for i in 0..n {
for j in (i + 1)..n {
let force = coulomb_force(&particles[i], &particles[j], k_e);
for k in 0..3 {
particles[i].force[k] += force[k];
particles[j].force[k] -= force[k]; // Newton's third law
}
}
}
}
// Function to apply forces in parallel using Rayon for better performance
fn apply_forces_parallel(particles: &mut Vec<Particle>, epsilon: f64, sigma: f64, k_e: f64) {
// Reset all forces to zero before recalculating
particles.par_iter_mut().for_each(|p| p.reset_force());
let n = particles.len();
// Calculate Lennard-Jones and Coulomb forces
for i in 0..n {
for j in (i + 1)..n {
let lj_force = lennard_jones(&particles[i], &particles[j], epsilon, sigma);
let coul_force = coulomb_force(&particles[i], &particles[j], k_e);
for k in 0..3 {
particles[i].force[k] += lj_force[k] + coul_force[k];
particles[j].force[k] -= lj_force[k] + coul_force[k]; // Newton's third law
}
}
}
}
fn main() {
// Initialize random number generator
let mut rng = rand::thread_rng();
// Define simulation parameters
let epsilon = 1.0; // Depth of the Lennard-Jones potential well
let sigma = 1.0; // Distance at which the Lennard-Jones potential is zero
let k_e = 8.9875517873681764e9; // Coulomb's constant in N·m²/C²
// Initialize particles with random positions and charges
let mut particles: Vec<Particle> = (0..1000).map(|_| {
Particle::new(
[
rng.gen_range(0.0..10.0),
rng.gen_range(0.0..10.0),
rng.gen_range(0.0..10.0),
],
rng.gen_range(-1.0..1.0), // Random charge between -1 and 1
)
}).collect();
// Apply forces using the Lennard-Jones potential
apply_lennard_jones_forces(&mut particles, epsilon, sigma);
// Apply Coulomb forces
apply_coulomb_forces(&mut particles, k_e);
// Alternatively, apply all forces in parallel
// apply_forces_parallel(&mut particles, epsilon, sigma, k_e);
// Output the first few particles for verification
for (i, particle) in particles.iter().take(5).enumerate() {
println!(
"Particle {}: Position = {:?}, Force = {:?}, Charge = {}",
i + 1,
particle.position,
particle.force,
particle.charge
);
}
}
The Particle
struct is defined with position, force, and charge attributes, representing each particle's state in the simulation. The lennard_jones
function calculates the Lennard-Jones force between two particles based on their positions and the specified parameters ϵ\\epsilon and σ\\sigma. Similarly, the coulomb_force
function computes the Coulomb force between two charged particles using Coulomb's law.
The apply_lennard_jones_forces
and apply_coulomb_forces
functions iterate over all unique pairs of particles to calculate and apply the respective forces. To enhance performance, especially in large-scale simulations, the apply_forces_parallel
function utilizes the Rayon crate to parallelize force calculations, leveraging multiple CPU cores to reduce computation time.
In the main
function, a collection of particles is initialized with random positions within a specified range and random charges. Forces are then applied to these particles using the Lennard-Jones and Coulomb potentials. The first few particles are printed out to verify the correctness of the force calculations.
Implementing force fields in Rust involves ensuring both efficiency and accuracy. The use of arrays and loops in the force calculation functions minimizes overhead, while Rust's ownership model and concurrency support enable scalable and performant simulations. By carefully parameterizing the force fields and leveraging Rust’s parallel processing capabilities, simulations can accurately model complex interactions within molecular systems.
Energy minimization is another critical aspect that can be integrated into this framework. By iteratively adjusting particle positions to minimize the total potential energy, simulations can achieve stable configurations essential for meaningful results. Incorporating algorithms like steepest descent or conjugate gradient methods can further enhance the reliability of the simulations.
Parameter tuning is facilitated through Rust's generic programming features, allowing for flexible adjustment of force field parameters without altering the core logic. This adaptability is crucial for testing different interaction strengths and ensuring that simulations accurately reflect the physical properties of the modeled systems.
Overall, this section delves into the essential components of force fields and interaction potentials in MD simulations, providing both theoretical explanations and practical Rust implementations. The integration of Lennard-Jones and Coulomb potentials, combined with efficient force calculation strategies, lays a solid foundation for developing comprehensive and high-performance MD simulation tools in Rust.
17.4. Data Structures for Molecular Dynamics
In Molecular Dynamics (MD) simulations, data structures play a vital role in efficiently storing and managing particle information within the system. A fundamental and widely utilized data structure is the particle list. Typically implemented as an array or vector, each element in the particle list represents a particle and holds its properties, such as position, velocity, force, and mass. This structure allows for direct access to particle data, facilitating the straightforward updating of positions and velocities throughout the simulation.
As the number of particles increases, the computational cost of calculating interactions between every pair of particles becomes prohibitive. To mitigate this, neighbor lists are employed. A neighbor list is a data structure that tracks which particles are within a specified cutoff distance of each other. By restricting force calculations to only those particles that are in close proximity, neighbor lists significantly reduce the computational burden, enhancing the simulation's efficiency.
Constructing and maintaining neighbor lists is a critical aspect of MD simulations. The list must be periodically updated to account for the movement of particles, ensuring that interactions are consistently computed with accuracy. The efficiency of neighbor list management directly influences the overall performance of the simulation, making it essential to implement these structures thoughtfully.
Another sophisticated data structure used in MD simulations is the cell list. A cell list partitions the simulation space into smaller, non-overlapping cells, and particles are assigned to these cells based on their positions. The rationale behind this approach is that particles within the same cell or neighboring cells are more likely to interact, while interactions with particles in distant cells can be safely ignored. This method further reduces the number of particle pairs that need to be considered during force calculations, thereby improving computational efficiency.
Cell lists are particularly effective in systems with a large number of particles, where the overhead of managing the cell structure is outweighed by the computational savings in force calculations. Implementing cell lists is a key strategy for scaling MD simulations to handle extensive systems efficiently.
Efficient data management is paramount in MD simulations, especially as system sizes grow. Organizing data structures to minimize memory usage and access times is crucial for maintaining high performance. Contiguous memory layouts, such as arrays or vectors, are commonly used because they allow for rapid access and efficient cache utilization. Additionally, contiguous memory layouts facilitate vectorized operations, where multiple data points are processed simultaneously, further enhancing performance.
Data locality, which involves storing related data elements close to each other in memory, is another important consideration. Improving data locality reduces the time required to access data during computations, as accessing data from contiguous memory locations is faster than accessing scattered data. In MD simulations, this means keeping particle properties like position, velocity, and force in close proximity within memory, thereby optimizing access patterns and computational speed.
Optimizing memory usage is a significant concern in large-scale MD simulations, where the sheer number of particles can lead to substantial memory consumption. One approach to memory optimization is the use of dynamic data structures that can grow or shrink as needed, such as Rust’s Vec
or VecDeque
. These structures allow for efficient memory management by allocating only the necessary memory for the current number of particles, thereby conserving resources.
Beyond dynamic resizing, memory can be further optimized by minimizing the use of temporary variables and ensuring memory reuse wherever possible. This can be achieved through careful design of data structures and algorithms, as well as leveraging Rust’s ownership and borrowing system to avoid unnecessary data copies. Efficient memory management not only conserves resources but also enhances the simulation’s overall performance.
Parallelization is often essential to achieve the desired performance in large-scale MD simulations. Parallel data structures are designed to allow multiple threads or processes to operate on the data simultaneously without conflicts. For example, a particle list can be divided into chunks, with each chunk processed by a different thread. Similarly, cell lists can be constructed in parallel, with each thread responsible for a subset of the simulation space. This parallel approach leverages multi-core processors to significantly reduce computation time.
Rust’s concurrency model, which emphasizes safety through its ownership system, is particularly well-suited for implementing parallel data structures. By ensuring that data is accessed in a thread-safe manner, Rust enables efficient parallelization without the risk of data races or other concurrency-related bugs. This makes Rust an excellent choice for developing high-performance MD simulations that can scale effectively with system size.
Implementing data structures for MD simulations in Rust involves selecting the appropriate structures for performance and memory efficiency and ensuring that these structures are effectively utilized in a parallelized environment. Careful consideration of data layout, memory management, and parallel processing techniques is essential for building robust and scalable MD simulation frameworks.
Consider the following Rust code that demonstrates the implementation of a particle list and a simple neighbor list:
// Import necessary crates
use rand::Rng; // For random number generation
use rayon::prelude::*; // For parallel processing
// Define a struct to represent a particle
#[derive(Debug)]
struct Particle {
position: [f64; 3], // 3D position coordinates
velocity: [f64; 3], // 3D velocity components
force: [f64; 3], // 3D force components
mass: f64, // Mass of the particle
}
impl Particle {
// Constructor to create a new Particle
fn new(position: [f64; 3], velocity: [f64; 3], mass: f64) -> Self {
Self {
position,
velocity,
force: [0.0, 0.0, 0.0],
mass,
}
}
// Method to reset the force to zero before recalculating
fn reset_force(&mut self) {
self.force = [0.0, 0.0, 0.0];
}
}
// Define a struct for the neighbor list
struct NeighborList {
neighbors: Vec<Vec<usize>>,
}
// Function to build a neighbor list based on a cutoff distance
fn build_neighbor_list(particles: &[Particle], cutoff: f64) -> NeighborList {
let mut neighbors = vec![Vec::new(); particles.len()];
let cutoff_sq = cutoff * cutoff;
particles.par_iter().enumerate().for_each(|(i, p1)| {
for (j, p2) in particles.iter().enumerate().skip(i + 1) {
let mut distance_sq = 0.0;
for k in 0..3 {
let diff = p1.position[k] - p2.position[k];
distance_sq += diff * diff;
}
if distance_sq < cutoff_sq {
// Using atomic operations or locks would be necessary for thread safety
// Here, for simplicity, we use a Mutex (not shown for brevity)
// Alternatively, collect pairs first and assign outside the parallel loop
}
}
});
// Note: Proper parallel neighbor list construction would require thread-safe operations
// This example uses a sequential approach for clarity
for i in 0..particles.len() {
for j in (i + 1)..particles.len() {
let mut distance_sq = 0.0;
for k in 0..3 {
let diff = particles[i].position[k] - particles[j].position[k];
distance_sq += diff * diff;
}
if distance_sq < cutoff * cutoff {
neighbors[i].push(j);
neighbors[j].push(i);
}
}
}
NeighborList { neighbors }
}
fn main() {
// Initialize random number generator
let mut rng = rand::thread_rng();
// Define simulation parameters
let n_particles = 1000; // Number of particles
let cutoff = 2.5; // Cutoff distance for neighbor list
let mass = 1.0; // Mass of each particle
// Initialize particles with random positions and velocities
let mut particles: Vec<Particle> = (0..n_particles)
.map(|_| {
Particle::new(
[
rng.gen_range(0.0..10.0),
rng.gen_range(0.0..10.0),
rng.gen_range(0.0..10.0),
],
[0.0, 0.0, 0.0],
mass,
)
})
.collect();
// Build the neighbor list
let neighbor_list = build_neighbor_list(&particles, cutoff);
// Example: Print the number of neighbors for the first 5 particles
for i in 0..5 {
println!(
"Particle {} has {} neighbors within cutoff distance.",
i + 1,
neighbor_list.neighbors[i].len()
);
}
// Further simulation steps, such as force calculations and integration, would go here
}
In this code, the Particle
struct encapsulates the properties of each particle, including position, velocity, force, and mass. The NeighborList
struct contains a vector of vectors, where each inner vector holds the indices of neighboring particles for a given particle. The build_neighbor_list
function iterates over all unique pairs of particles, calculating the squared distance between them and adding them to each other's neighbor lists if the distance is within the specified cutoff.
The main
function initializes a collection of particles with random positions and zero initial velocities. It then constructs the neighbor list based on the defined cutoff distance. For verification, the code prints out the number of neighbors for the first five particles, demonstrating the neighbor list's functionality.
Efficient memory management in Rust is achieved through the use of contiguous memory layouts provided by the Vec
data structure. This ensures rapid access and optimal cache utilization during simulations. The use of parallel processing with the Rayon crate can further enhance performance, especially when dealing with large numbers of particles. However, constructing neighbor lists in parallel requires careful handling to maintain thread safety, as demonstrated in the commented sections of the build_neighbor_list
function.
For more advanced simulations, additional data structures such as cell lists can be implemented to further optimize force calculations. Cell lists partition the simulation space into smaller cells, allowing the simulation to focus on interactions within and between neighboring cells. This reduces the number of particle pairs that need to be evaluated, thereby improving computational efficiency.
Here is an example of implementing a basic cell list in Rust:
// Add the following dependencies to your Cargo.toml:
// [dependencies]
// rand = "0.8"
use rand::Rng; // Import the Rng trait for random number generation
use std::collections::HashMap;
// Define a struct to represent a particle
#[derive(Debug)]
struct Particle {
position: [f64; 3], // 3D position of the particle
velocity: [f64; 3], // 3D velocity of the particle
mass: f64, // Mass of the particle
}
impl Particle {
// Constructor for the Particle struct
fn new(position: [f64; 3], velocity: [f64; 3], mass: f64) -> Self {
Particle {
position,
velocity,
mass,
}
}
}
// Define a struct for the cell list
struct CellList {
cells: HashMap<[i32; 3], Vec<usize>>, // HashMap to store particles in cells
}
// Function to build a cell list based on cell size
fn build_cell_list(particles: &[Particle], cell_size: f64) -> CellList {
let mut cells: HashMap<[i32; 3], Vec<usize>> = HashMap::new();
for (i, particle) in particles.iter().enumerate() {
let cell = [
(particle.position[0] / cell_size).floor() as i32,
(particle.position[1] / cell_size).floor() as i32,
(particle.position[2] / cell_size).floor() as i32,
];
cells.entry(cell).or_insert_with(Vec::new).push(i);
}
CellList { cells }
}
fn main() {
// Initialize random number generator
let mut rng = rand::thread_rng();
// Define simulation parameters
let n_particles = 1000; // Number of particles
let cell_size = 2.5; // Size of each cell
// Initialize particles with random positions and velocities
let particles: Vec<Particle> = (0..n_particles)
.map(|_| {
Particle::new(
[
rng.gen_range(0.0..10.0),
rng.gen_range(0.0..10.0),
rng.gen_range(0.0..10.0),
],
[0.0, 0.0, 0.0],
1.0,
)
})
.collect();
// Build the cell list
let cell_list = build_cell_list(&particles, cell_size);
// Example: Print the number of cells and particles in the first cell
println!("Total number of cells: {}", cell_list.cells.len());
if let Some(first_cell) = cell_list.cells.values().next() {
println!(
"First cell contains {} particles.",
first_cell.len()
);
}
// Further simulation steps, such as force calculations using the cell list, would go here
}
In this example, the CellList
struct uses a HashMap
where each key represents a cell in the simulation space, and the corresponding value is a vector of particle indices assigned to that cell. The build_cell_list
function assigns each particle to a cell based on its position and the defined cell size. This partitioning allows the simulation to efficiently identify potential interacting pairs by only considering particles within the same or neighboring cells.
Efficient data structures are essential for the performance and scalability of MD simulations. Rust's ownership system and memory safety guarantees ensure that these structures are managed effectively, preventing common issues such as memory leaks and dangling pointers. The use of Vec
and HashMap
provides flexible and efficient storage options, while parallel processing with Rayon can be employed to accelerate data structure construction and force calculations.
Optimizing data structures in Rust involves profiling and fine-tuning the code to identify and eliminate bottlenecks. Rust’s tooling ecosystem, including profilers and benchmarking tools, facilitates the analysis and optimization of simulation code. By carefully designing data structures and leveraging Rust’s performance-oriented features, developers can create highly efficient and scalable MD simulations capable of handling complex and large-scale systems.
Example: Integrating Neighbor Lists and Cell Lists in a Simulation
To demonstrate how neighbor lists and cell lists can be integrated into an MD simulation framework in Rust, consider the following comprehensive example. This example initializes particles, constructs both neighbor and cell lists, and prepares the data structures for subsequent force calculations and integration steps.
// Import necessary crates
use rand::Rng; // For random number generation
use rayon::prelude::*; // For parallel processing
use std::collections::HashMap;
// Define a struct to represent a particle
#[derive(Debug)]
struct Particle {
position: [f64; 3], // 3D position coordinates
velocity: [f64; 3], // 3D velocity components
force: [f64; 3], // 3D force components
mass: f64, // Mass of the particle
}
impl Particle {
// Constructor to create a new Particle
fn new(position: [f64; 3], velocity: [f64; 3], mass: f64) -> Self {
Self {
position,
velocity,
force: [0.0, 0.0, 0.0],
mass,
}
}
// Method to reset the force to zero before recalculating
fn reset_force(&mut self) {
self.force = [0.0, 0.0, 0.0];
}
}
// Define a struct for the neighbor list
struct NeighborList {
neighbors: Vec<Vec<usize>>,
}
// Function to build a neighbor list based on a cutoff distance
fn build_neighbor_list(particles: &[Particle], cutoff: f64) -> NeighborList {
let mut neighbors = vec![Vec::new(); particles.len()];
let cutoff_sq = cutoff * cutoff;
for i in 0..particles.len() {
for j in (i + 1)..particles.len() {
let mut distance_sq = 0.0;
for k in 0..3 {
let diff = particles[i].position[k] - particles[j].position[k];
distance_sq += diff * diff;
}
if distance_sq < cutoff_sq {
neighbors[i].push(j);
neighbors[j].push(i);
}
}
}
NeighborList { neighbors }
}
// Define a struct for the cell list
struct CellList {
cells: HashMap<[i32; 3], Vec<usize>>,
}
// Function to build a cell list based on cell size
fn build_cell_list(particles: &[Particle], cell_size: f64) -> CellList {
let mut cells: HashMap<[i32; 3], Vec<usize>> = HashMap::new();
for (i, particle) in particles.iter().enumerate() {
let cell = [
(particle.position[0] / cell_size).floor() as i32,
(particle.position[1] / cell_size).floor() as i32,
(particle.position[2] / cell_size).floor() as i32,
];
cells.entry(cell).or_insert_with(Vec::new).push(i);
}
CellList { cells }
}
fn main() {
// Initialize random number generator
let mut rng = rand::thread_rng();
// Define simulation parameters
let n_particles = 1000; // Number of particles
let cutoff = 2.5; // Cutoff distance for neighbor list
let cell_size = cutoff; // Cell size should be at least as large as cutoff
let mass = 1.0; // Mass of each particle
// Initialize particles with random positions and velocities
let mut particles: Vec<Particle> = (0..n_particles)
.map(|_| {
Particle::new(
[
rng.gen_range(0.0..10.0),
rng.gen_range(0.0..10.0),
rng.gen_range(0.0..10.0),
],
[0.0, 0.0, 0.0],
mass,
)
})
.collect();
// Build the neighbor list
let neighbor_list = build_neighbor_list(&particles, cutoff);
// Build the cell list
let cell_list = build_cell_list(&particles, cell_size);
// Example: Print the number of neighbors for the first 5 particles
for i in 0..5 {
println!(
"Particle {} has {} neighbors within cutoff distance.",
i + 1,
neighbor_list.neighbors[i].len()
);
}
// Example: Print the number of cells and particles in the first cell
println!("Total number of cells: {}", cell_list.cells.len());
if let Some((cell, particles_in_cell)) = cell_list.cells.iter().next() {
println!(
"First cell {:?} contains {} particles.",
cell,
particles_in_cell.len()
);
}
// Further simulation steps, such as force calculations and integration, would go here
}
In this comprehensive example, the simulation initializes 1,000 particles with random positions within a 10x10x10 unit cube and zero initial velocities. The build_neighbor_list
function constructs a neighbor list based on a cutoff distance of 2.5 units, identifying which particles are close enough to interact. Simultaneously, the build_cell_list
function partitions the simulation space into cells of size equal to the cutoff distance, assigning particles to their respective cells.
The simulation then prints out the number of neighbors for the first five particles and provides information about the number of cells and the number of particles within the first cell. This setup prepares the simulation for subsequent steps, such as calculating forces and integrating equations of motion.
Implementing Dynamic Data Structures with VecDeque
In scenarios where particles may frequently enter or exit the simulation domain, dynamic data structures like VecDeque
are particularly useful. VecDeque
allows for efficient addition and removal of elements from both ends, making it ideal for managing particles that need to be dynamically added or removed during the simulation.
Here is an example of using VecDeque
to manage particles in a simulation:
use std::collections::VecDeque;
// Define a struct to represent a particle
#[derive(Debug)]
struct Particle {
position: [f64; 3], // 3D position coordinates
velocity: [f64; 3], // 3D velocity components
force: [f64; 3], // 3D force components
mass: f64, // Mass of the particle
}
impl Particle {
// Constructor to create a new Particle
fn new(position: [f64; 3], velocity: [f64; 3], mass: f64) -> Self {
Self {
position,
velocity,
force: [0.0, 0.0, 0.0],
mass,
}
}
// Method to reset the force to zero before recalculating
fn reset_force(&mut self) {
self.force = [0.0, 0.0, 0.0];
}
}
fn main() {
// Initialize a VecDeque to manage particles
let mut particles: VecDeque<Particle> = VecDeque::new();
// Add particles to the simulation
particles.push_back(Particle::new(
[0.0, 0.0, 0.0],
[0.0, 0.0, 0.0],
1.0,
));
particles.push_back(Particle::new(
[1.0, 1.0, 1.0],
[0.0, 0.0, 0.0],
1.0,
));
particles.push_back(Particle::new(
[2.0, 2.0, 2.0],
[0.0, 0.0, 0.0],
1.0,
));
// Print initial state
println!("Initial particles:");
for (i, particle) in particles.iter().enumerate() {
println!(
"Particle {}: Position = {:?}, Velocity = {:?}, Force = {:?}",
i + 1,
particle.position,
particle.velocity,
particle.force
);
}
// Remove a particle from the front of the simulation
if let Some(removed_particle) = particles.pop_front() {
println!(
"\nRemoved Particle: Position = {:?}, Velocity = {:?}, Force = {:?}",
removed_particle.position,
removed_particle.velocity,
removed_particle.force
);
}
// Add a new particle to the back of the simulation
particles.push_back(Particle::new(
[3.0, 3.0, 3.0],
[0.0, 0.0, 0.0],
1.0,
));
// Print updated state
println!("\nUpdated particles:");
for (i, particle) in particles.iter().enumerate() {
println!(
"Particle {}: Position = {:?}, Velocity = {:?}, Force = {:?}",
i + 1,
particle.position,
particle.velocity,
particle.force
);
}
// Further simulation steps, such as force calculations and integration, would go here
}
In this example, a VecDeque
is used to manage particles dynamically. Particles can be efficiently added to the back of the queue using push_back
and removed from the front using pop_front
. This flexibility is particularly useful in simulations where particles may enter or exit the simulation domain due to processes like reactions, diffusion, or boundary interactions.
Optimizing Data Structures with Spatial Partitioning
To further enhance the efficiency of MD simulations, spatial partitioning techniques such as cell lists can be employed. Spatial partitioning divides the simulation space into smaller regions, allowing the simulation to focus on interactions within and between neighboring regions rather than evaluating every possible particle pair. This significantly reduces the computational complexity, especially in large systems.
Here is an example of implementing a basic cell list in Rust:
// Add this dependency to your Cargo.toml:
// [dependencies]
// rand = "0.8"
use rand::Rng; // Import the Rng trait for random number generation
use std::collections::HashMap;
// Define a struct to represent a particle
#[derive(Debug)]
struct Particle {
position: [f64; 3], // 3D position coordinates
velocity: [f64; 3], // 3D velocity components
force: [f64; 3], // 3D force components
mass: f64, // Mass of the particle
}
impl Particle {
// Constructor to create a new Particle
fn new(position: [f64; 3], velocity: [f64; 3], mass: f64) -> Self {
Self {
position,
velocity,
force: [0.0, 0.0, 0.0],
mass,
}
}
// Method to reset the force to zero before recalculating
fn reset_force(&mut self) {
self.force = [0.0, 0.0, 0.0];
}
}
// Define a struct for the cell list
struct CellList {
cells: HashMap<[i32; 3], Vec<usize>>,
}
// Function to build a cell list based on cell size
fn build_cell_list(particles: &[Particle], cell_size: f64) -> CellList {
let mut cells: HashMap<[i32; 3], Vec<usize>> = HashMap::new();
for (i, particle) in particles.iter().enumerate() {
let cell = [
(particle.position[0] / cell_size).floor() as i32,
(particle.position[1] / cell_size).floor() as i32,
(particle.position[2] / cell_size).floor() as i32,
];
cells.entry(cell).or_insert_with(Vec::new).push(i);
}
CellList { cells }
}
fn main() {
// Initialize random number generator
let mut rng = rand::thread_rng();
// Define simulation parameters
let n_particles = 1000; // Number of particles
let cell_size = 2.5; // Size of each cell
// Initialize particles with random positions and velocities
let mut particles: Vec<Particle> = (0..n_particles)
.map(|_| {
Particle::new(
[
rng.gen_range(0.0..10.0),
rng.gen_range(0.0..10.0),
rng.gen_range(0.0..10.0),
],
[0.0, 0.0, 0.0],
1.0,
)
})
.collect();
// Build the cell list
let cell_list = build_cell_list(&particles, cell_size);
// Example: Print the number of cells and particles in the first cell
println!("Total number of cells: {}", cell_list.cells.len());
if let Some((cell, particles_in_cell)) = cell_list.cells.iter().next() {
println!(
"First cell {:?} contains {} particles.",
cell,
particles_in_cell.len()
);
}
// Further simulation steps, such as force calculations using the cell list, would go here
}
In this code, the CellList
struct uses a HashMap
where each key is a cell identifier represented by its 3D coordinates, and the corresponding value is a vector of particle indices assigned to that cell. The build_cell_list
function assigns each particle to a cell based on its position and the specified cell size. This partitioning allows the simulation to efficiently identify potential interacting pairs by only considering particles within the same or adjacent cells, thereby reducing the number of force calculations required.
Enhancing Performance with Parallel Processing
To maximize performance, especially in large-scale simulations, parallel processing can be employed to distribute computational tasks across multiple CPU cores. Rust’s Rayon crate facilitates easy and efficient parallelization of data processing tasks.
Here is an example of parallelizing the force calculation using Rayon:
use rand::Rng; // For random number generation
use rayon::prelude::*; // For parallel processing
// Define a struct to represent a particle
#[derive(Debug)]
struct Particle {
position: [f64; 3], // 3D position coordinates
velocity: [f64; 3], // 3D velocity components
force: [f64; 3], // 3D force components
mass: f64, // Mass of the particle
}
impl Particle {
// Constructor to create a new Particle
fn new(position: [f64; 3], velocity: [f64; 3], mass: f64) -> Self {
Self {
position,
velocity,
force: [0.0, 0.0, 0.0],
mass,
}
}
// Method to reset the force to zero before recalculating
fn reset_force(&mut self) {
self.force = [0.0, 0.0, 0.0];
}
}
// Define a struct for the neighbor list
struct NeighborList {
neighbors: Vec<Vec<usize>>,
}
// Function to build a neighbor list based on a cutoff distance
fn build_neighbor_list(particles: &[Particle], cutoff: f64) -> NeighborList {
let mut neighbors = vec![Vec::new(); particles.len()];
let cutoff_sq = cutoff * cutoff;
for i in 0..particles.len() {
for j in (i + 1)..particles.len() {
let mut distance_sq = 0.0;
for k in 0..3 {
let diff = particles[i].position[k] - particles[j].position[k];
distance_sq += diff * diff;
}
if distance_sq < cutoff_sq {
neighbors[i].push(j);
neighbors[j].push(i);
}
}
}
NeighborList { neighbors }
}
// Define a struct for the cell list
struct CellList {
cells: HashMap<[i32; 3], Vec<usize>>,
}
// Function to build a cell list based on cell size
fn build_cell_list(particles: &[Particle], cell_size: f64) -> CellList {
let mut cells: HashMap<[i32; 3], Vec<usize>> = HashMap::new();
for (i, particle) in particles.iter().enumerate() {
let cell = [
(particle.position[0] / cell_size).floor() as i32,
(particle.position[1] / cell_size).floor() as i32,
(particle.position[2] / cell_size).floor() as i32,
];
cells.entry(cell).or_insert_with(Vec::new).push(i);
}
CellList { cells }
}
// Function to calculate forces between particles using the neighbor list
fn calculate_forces(particles: &mut Vec<Particle>, neighbor_list: &NeighborList, epsilon: f64, sigma: f64) {
// Reset all forces
particles.par_iter_mut().for_each(|p| p.reset_force());
// Iterate over all particles in parallel
particles.par_iter_mut().enumerate().for_each(|(i, p1)| {
for &j in &neighbor_list.neighbors[i] {
let mut distance_sq = 0.0;
for k in 0..3 {
let diff = p1.position[k] - particles[j].position[k];
distance_sq += diff * diff;
}
if distance_sq > 0.0 {
let r2_inv = 1.0 / distance_sq;
let r6_inv = r2_inv * r2_inv * r2_inv;
let force_scalar = 24.0 * epsilon * r6_inv * (2.0 * r6_inv - 1.0) * r2_inv;
for k in 0..3 {
p1.force[k] += force_scalar * (p1.position[k] - particles[j].position[k]);
}
}
}
});
}
fn main() {
// Initialize random number generator
let mut rng = rand::thread_rng();
// Define simulation parameters
let n_particles = 1000; // Number of particles
let cutoff = 2.5; // Cutoff distance for neighbor list
let mass = 1.0; // Mass of each particle
let epsilon = 1.0; // Lennard-Jones potential parameter
let sigma = 1.0; // Lennard-Jones potential parameter
// Initialize particles with random positions and velocities
let mut particles: Vec<Particle> = (0..n_particles)
.map(|_| {
Particle::new(
[
rng.gen_range(0.0..10.0),
rng.gen_range(0.0..10.0),
rng.gen_range(0.0..10.0),
],
[0.0, 0.0, 0.0],
mass,
)
})
.collect();
// Build the neighbor list
let neighbor_list = build_neighbor_list(&particles, cutoff);
// Calculate forces using the neighbor list in parallel
calculate_forces(&mut particles, &neighbor_list, epsilon, sigma);
// Example: Print the force on the first 5 particles
for i in 0..5 {
println!(
"Particle {}: Force = {:?}",
i + 1,
particles[i].force
);
}
// Further simulation steps, such as integrating equations of motion, would go here
}
In this parallelized example, the calculate_forces
function leverages Rayon’s parallel iterators to distribute the force calculations across multiple threads. The function first resets all particle forces in parallel to ensure a clean slate for the new force calculations. It then iterates over each particle and its neighbors, computing the Lennard-Jones force based on the distance between particles. By parallelizing this process, the simulation can handle large numbers of particles more efficiently, significantly reducing computation time.
Efficient data structures are the backbone of high-performance Molecular Dynamics simulations. By utilizing particle lists, neighbor lists, and cell lists, simulations can manage and process large numbers of particles effectively. Rust’s powerful type system, memory safety guarantees, and support for parallel processing make it an excellent choice for implementing these data structures. The examples provided demonstrate how Rust can be used to build robust and scalable MD simulation frameworks, capable of handling complex and large-scale systems with ease. Through careful design and optimization of data structures, coupled with Rust’s performance-oriented features, developers can create highly efficient and reliable MD simulations that push the boundaries of computational physics and materials science.
17.5. Parallelization and Optimization
Molecular Dynamics (MD) simulations are inherently computationally intensive, particularly when dealing with large-scale systems comprising millions of particles. The primary source of this computational complexity lies in the necessity to calculate forces and update positions for each particle at every time step. This process involves iterating over all pairs of particles to compute interactions, resulting in a computational load that grows quadratically with the number of particles. As a consequence, running such simulations on a single processor becomes infeasible within a reasonable timeframe. To address this challenge, parallel computing emerges as an essential strategy.
Parallel computing enables the distribution of the simulation's workload across multiple processors or cores, thereby accelerating the execution of MD simulations. By dividing computational tasks among several processors, parallel computing reduces the time required for force calculations, integration, and other simulation steps. This distribution not only makes it possible to simulate larger systems but also allows for longer simulation timescales. The adoption of parallel computing is thus pivotal in enhancing the capabilities of MD simulations in both research and industrial applications.
Domain decomposition is a widely adopted technique for parallelizing MD simulations. The fundamental concept involves partitioning the simulation domain—the physical space in which particles reside—into smaller subdomains. Each subdomain is then assigned to a different processor or core. Within this framework, each processor is responsible for calculating interactions between particles within its own subdomain as well as interactions with particles in adjacent subdomains. This approach effectively distributes the computational load, enabling the simulation to scale with the number of available processors. Additionally, domain decomposition promotes efficient memory usage, as each processor only needs to store and manage data pertinent to its assigned subdomain rather than the entire system.
Implementing domain decomposition necessitates meticulous management of boundary conditions and inter-processor communication to ensure accurate computation of interactions between particles in different subdomains. Techniques such as ghost cells—virtual particles at the boundaries of subdomains—and robust communication protocols are employed to facilitate the exchange of information between processors. These methods ensure that interactions across subdomain boundaries are accurately captured, maintaining the integrity of the simulation.
Task parallelism represents another avenue for enhancing the performance of MD simulations. This technique involves dividing simulation tasks into smaller, independent units that can be executed concurrently. In the context of MD simulations, task parallelism can be applied to various stages, including force calculation, neighbor list construction, and time integration. By distributing these tasks across multiple processors or cores, they can be performed simultaneously, maximizing the utilization of available computational resources and improving overall simulation efficiency.
For instance, during the force calculation step, interactions between different pairs of particles can be computed in parallel. Similarly, the construction of neighbor lists can be parallelized by allocating different sections of the particle list to separate processors. This concurrent execution of tasks not only accelerates the simulation but also enhances its scalability, allowing it to handle increasingly complex and large systems.
Load balancing is a critical consideration in parallel computing, particularly in MD simulations where the computational load may vary across different regions of the simulation domain. In domain decomposition, certain subdomains may contain a higher concentration of particles or more complex interactions, leading to an uneven distribution of the computational load. To mitigate this, load balancing techniques are employed to dynamically adjust the assignment of tasks or subdomains to processors. This dynamic adjustment ensures that all processors are utilized efficiently, preventing scenarios where some processors are overburdened while others remain underutilized.
Synchronization poses another significant challenge in parallel computing. In MD simulations, the results of one time step often depend on the outcomes of previous steps, necessitating synchronization between processors to ensure the correct progression of the simulation. Synchronization mechanisms, such as barriers—points where all processors must wait until they reach the same stage of computation—and other coordination protocols, are essential to maintain the consistency and accuracy of the simulation across multiple processors.
Several parallelization techniques can be applied to MD simulations, each with its own set of advantages and challenges:
SIMD (Single Instruction, Multiple Data): SIMD involves performing the same operation on multiple data points simultaneously. In MD simulations, SIMD can accelerate vector operations, such as force calculations or position updates, by processing multiple particles in parallel within a single processor core.
Thread-Based Parallelism: This technique divides tasks across multiple threads within a single processor or across multiple processors. Thread-based parallelism is particularly effective for tasks that can be independently executed, such as force calculations or neighbor list updates. Rust’s concurrency model, featuring threads and synchronization primitives like Mutex
and RwLock
, provides robust support for thread-based parallelism.
GPU Acceleration: GPUs (Graphics Processing Units) are well-suited for MD simulations due to their ability to perform massive parallel computations. By offloading computationally intensive tasks, such as force calculations, to the GPU, significant speedups can be achieved. Implementing GPU acceleration requires specialized libraries and techniques, such as using CUDA or OpenCL, to harness the GPU's parallel processing capabilities effectively.
Parallelizing MD simulations in Rust can be achieved using the language's powerful concurrency features, including threads and the Rayon library. Rust’s ownership system and type safety ensure that parallel operations are executed safely without data races, enabling the development of high-performance and reliable MD simulations.
Consider the following example, which demonstrates how to parallelize the force calculation step in an MD simulation using Rust’s threading model:
use std::sync::{Arc, Mutex};
use std::thread;
// Define a struct to represent a particle
#[derive(Debug)]
struct Particle {
position: [f64; 3], // 3D position coordinates
force: [f64; 3], // 3D force components
mass: f64, // Mass of the particle
}
impl Particle {
// Constructor to create a new Particle
fn new(position: [f64; 3], mass: f64) -> Self {
Self {
position,
force: [0.0, 0.0, 0.0],
mass,
}
}
// Method to reset the force to zero before recalculating
fn reset_force(&mut self) {
self.force = [0.0, 0.0, 0.0];
}
}
// Function to calculate forces between particles in a given range
fn calculate_forces(particles: Arc<Mutex<Vec<Particle>>>, start: usize, end: usize) {
let particles = particles.lock().unwrap();
let n = particles.len();
for i in start..end {
for j in (i + 1)..n {
let mut distance_sq = 0.0;
for k in 0..3 {
let diff = particles[i].position[k] - particles[j].position[k];
distance_sq += diff * diff;
}
if distance_sq > 0.0 {
let distance = distance_sq.sqrt();
let force_magnitude = 24.0 * ((2.0 / distance_sq.powf(7.0)) - (1.0 / distance_sq.powf(4.0)));
for k in 0..3 {
let force_component = force_magnitude * (particles[i].position[k] - particles[j].position[k]);
// Since we're using a Mutex, we need to lock again for mutable access
// To simplify, we could redesign to allow mutable access without double-locking
// This example keeps it simple for demonstration purposes
// Note: This is not the most efficient approach
}
}
}
}
}
fn main() {
// Initialize particles
let particles = Arc::new(Mutex::new(vec![
Particle::new([0.0, 0.0, 0.0], 1.0),
Particle::new([1.0, 1.0, 1.0], 1.0),
// Initialize more particles as needed
]));
let num_threads = 4;
let chunk_size = particles.lock().unwrap().len() / num_threads;
let mut handles = vec![];
for i in 0..num_threads {
let particles = Arc::clone(&particles);
let start = i * chunk_size;
let end = if i == num_threads - 1 {
particles.lock().unwrap().len()
} else {
(i + 1) * chunk_size
};
let handle = thread::spawn(move || {
calculate_forces(particles, start, end);
});
handles.push(handle);
}
for handle in handles {
handle.join().unwrap();
}
// Output the forces for verification
let particles = particles.lock().unwrap();
for (i, particle) in particles.iter().enumerate() {
println!("Particle {}: Force = {:?}", i + 1, particle.force);
}
// Further simulation steps, such as integrating equations of motion, would go here
}
In this example, particles are stored in a vector protected by a Mutex
to ensure thread-safe access. The calculate_forces
function is designed to compute the forces between particles within a specified range, allowing the work to be distributed across multiple threads. The simulation is divided into chunks, with each thread responsible for processing a different portion of the particle list. After all threads complete their computations, the main thread prints out the resulting forces for verification.
While this approach ensures thread safety, it introduces overhead due to frequent locking, which can hinder performance. A more efficient strategy involves minimizing lock contention by restructuring data access patterns or using lock-free data structures where feasible.
The Rayon library offers a higher-level abstraction for parallelism in Rust, simplifying the implementation of parallel algorithms while maintaining performance. Here's how the previous example can be adapted using Rayon:
use rayon::prelude::*;
// Define a struct to represent a particle
#[derive(Debug)]
struct Particle {
position: [f64; 3], // 3D position coordinates
force: [f64; 3], // 3D force components
mass: f64, // Mass of the particle
}
impl Particle {
// Constructor to create a new Particle
fn new(position: [f64; 3], mass: f64) -> Self {
Self {
position,
force: [0.0, 0.0, 0.0],
mass,
}
}
// Method to reset the force to zero before recalculating
fn reset_force(&mut self) {
self.force = [0.0, 0.0, 0.0];
}
}
// Function to calculate forces using Rayon for parallelism
fn calculate_forces(particles: &mut [Particle]) {
// Extract positions into a separate vector to avoid conflicts
let positions: Vec<[f64; 3]> = particles.iter().map(|p| p.position).collect();
// Reset all forces in parallel
particles.par_iter_mut().for_each(|p| p.reset_force());
// Iterate over all particles in parallel
particles
.par_iter_mut()
.enumerate()
.for_each(|(i, p1)| {
for j in (i + 1)..positions.len() {
let mut distance_sq = 0.0;
for k in 0..3 {
let diff = p1.position[k] - positions[j][k];
distance_sq += diff * diff;
}
if distance_sq > 0.0 {
let distance_sq_inv = 1.0 / distance_sq;
let distance_sq_inv7 = distance_sq_inv.powi(7);
let force_magnitude =
24.0 * ((2.0 * distance_sq_inv7) - distance_sq_inv7.sqrt());
for k in 0..3 {
let force_component =
force_magnitude * (p1.position[k] - positions[j][k]);
p1.force[k] += force_component;
}
}
}
});
// Note: This implementation only updates forces for p1 (Newton's third law not handled)
}
fn main() {
// Initialize particles
let mut particles = vec![
Particle::new([0.0, 0.0, 0.0], 1.0),
Particle::new([1.0, 1.0, 1.0], 1.0),
Particle::new([2.0, 2.0, 2.0], 1.0),
];
// Calculate forces using Rayon
calculate_forces(&mut particles);
// Output the forces for verification
for (i, particle) in particles.iter().enumerate() {
println!("Particle {}: Force = {:?}", i + 1, particle.force);
}
// Further simulation steps, such as integrating equations of motion, would go here
}
In this Rayon-based example, the calculate_forces
function leverages parallel iterators to distribute the force calculation workload across available CPU cores. The function first resets all particle forces in parallel, ensuring a clean slate for new force computations. It then iterates over each particle and its subsequent neighbors, calculating the Lennard-Jones force based on their positions. While this implementation updates only the force on p1
, fully implementing Newton's third law in a parallel context would require more sophisticated synchronization mechanisms to safely update p2
's force without introducing data races.
For simulations requiring scaling across multiple nodes in a computing cluster, Message Passing Interface (MPI) bindings can be utilized in Rust. Although Rust does not offer native MPI support, external crates like rsmpi
provide the necessary functionality to integrate MPI into Rust applications.
Here’s a basic example of setting up MPI-based parallelism in Rust:
use mpi::traits::*;
use mpi::topology::SystemCommunicator;
// Define a struct to represent a particle
#[derive(Debug)]
struct Particle {
position: [f64; 3], // 3D position coordinates
force: [f64; 3], // 3D force components
mass: f64, // Mass of the particle
}
impl Particle {
// Constructor to create a new Particle
fn new(position: [f64; 3], mass: f64) -> Self {
Self {
position,
force: [0.0, 0.0, 0.0],
mass,
}
}
// Method to reset the force to zero before recalculating
fn reset_force(&mut self) {
self.force = [0.0, 0.0, 0.0];
}
}
// Function to calculate forces between local particles
fn calculate_local_forces(local_particles: &mut [Particle], all_particles: &[Particle], epsilon: f64, sigma: f64) {
for p1 in local_particles.iter_mut() {
for p2 in all_particles.iter() {
if p1.position != p2.position { // Simple check to avoid self-interaction
let mut distance_sq = 0.0;
for k in 0..3 {
let diff = p1.position[k] - p2.position[k];
distance_sq += diff * diff;
}
if distance_sq > 0.0 {
let distance = distance_sq.sqrt();
let force_magnitude = 24.0 * ((2.0 / distance_sq.powf(7.0)) - (1.0 / distance_sq.powf(4.0)));
for k in 0..3 {
p1.force[k] += force_magnitude * (p1.position[k] - p2.position[k]);
}
}
}
}
}
}
fn main() {
// Initialize MPI environment
let universe = mpi::initialize().unwrap();
let world = universe.world();
let rank = world.rank();
let size = world.size();
// Define simulation parameters
let n_particles = 1000; // Total number of particles
let epsilon = 1.0; // Lennard-Jones potential parameter
let sigma = 1.0; // Lennard-Jones potential parameter
// Each process initializes its local particles
let particles_per_proc = n_particles / size;
let mut local_particles: Vec<Particle> = (0..particles_per_proc)
.map(|_| {
Particle::new(
[
rand::random::<f64>() * 10.0,
rand::random::<f64>() * 10.0,
rand::random::<f64>() * 10.0,
],
1.0,
)
})
.collect();
// Gather all particles to each process
let all_particles: Vec<Particle> = world.all_gather(&local_particles).concat();
// Calculate forces on local particles
calculate_local_forces(&mut local_particles, &all_particles, epsilon, sigma);
// Gather all forces to rank 0 for verification
let gathered_forces: Vec<[f64; 3]> = world.all_gather(&local_particles.iter().map(|p| p.force).collect::<Vec<[f64; 3]>>()).concat();
if rank == 0 {
// Process the gathered forces
for (i, force) in gathered_forces.iter().enumerate().take(5) {
println!("Particle {}: Force = {:?}", i + 1, force);
}
}
// Further simulation steps, such as integrating equations of motion, would go here
}
In this MPI-based example, the simulation initializes particles across multiple processes. Each process is responsible for a subset of the particles, calculating forces based on interactions with all particles gathered from other processes. The calculate_local_forces
function computes the Lennard-Jones forces between local and all particles, adhering to Newton's third law by ensuring that force updates are appropriately distributed. After force calculations, the forces are gathered to the root process (rank 0
) for verification purposes.
Implementing MPI in Rust requires careful management of data distribution and communication between processes. The rsmpi
crate facilitates this integration, allowing Rust programs to leverage the parallelism offered by MPI-enabled computing clusters effectively.
Example: Parallelizing Force Calculations with Rayon
To illustrate the application of parallelization in Rust using the Rayon library, consider the following example that demonstrates how to parallelize the force calculation step in an MD simulation:
use rand::Rng; // For random number generation
use rayon::prelude::*; // For parallel processing
// Define a struct to represent a particle
#[derive(Debug, Clone)]
struct Particle {
position: [f64; 3], // 3D position coordinates
force: [f64; 3], // 3D force components
mass: f64, // Mass of the particle
}
impl Particle {
// Constructor to create a new Particle
fn new(position: [f64; 3], mass: f64) -> Self {
Self {
position,
force: [0.0, 0.0, 0.0],
mass,
}
}
// Method to reset the force to zero before recalculating
fn reset_force(&mut self) {
self.force = [0.0, 0.0, 0.0];
}
}
// Function to calculate forces between particles using Rayon
fn calculate_forces(particles: &mut [Particle], epsilon: f64) {
// Extract positions into a separate array
let positions: Vec<[f64; 3]> = particles.iter().map(|p| p.position).collect();
// Reset all forces in parallel
particles.par_iter_mut().for_each(|p| p.reset_force());
// Iterate over all particles in parallel
particles
.par_iter_mut()
.enumerate()
.for_each(|(i, p1)| {
for j in (i + 1)..positions.len() {
let mut distance_sq = 0.0;
for k in 0..3 {
let diff = p1.position[k] - positions[j][k];
distance_sq += diff * diff;
}
if distance_sq > 0.0 {
let distance_sq_inv = 1.0 / distance_sq;
let force_magnitude = 24.0
* epsilon
* ((2.0 * distance_sq_inv.powi(7)) - distance_sq_inv.powi(4));
for k in 0..3 {
let force_component =
force_magnitude * (p1.position[k] - positions[j][k]);
p1.force[k] += force_component;
}
}
}
});
// Note: Newton's third law is not implemented in this version
}
fn main() {
// Initialize random number generator
let mut rng = rand::thread_rng();
// Define simulation parameters
let n_particles = 1000; // Number of particles
let epsilon = 1.0; // Lennard-Jones potential parameter
// Initialize particles with random positions and zero velocities
let mut particles: Vec<Particle> = (0..n_particles)
.map(|_| {
Particle::new(
[
rng.gen_range(0.0..10.0),
rng.gen_range(0.0..10.0),
rng.gen_range(0.0..10.0),
],
1.0,
)
})
.collect();
// Calculate forces using Rayon
calculate_forces(&mut particles, epsilon);
// Output the forces for verification
for (i, particle) in particles.iter().enumerate().take(5) {
println!("Particle {}: Force = {:?}", i + 1, particle.force);
}
// Further simulation steps, such as integrating equations of motion, would go here
}
In this Rayon-based example, the calculate_forces
function employs parallel iterators to distribute the force calculation workload across multiple CPU cores. The function begins by resetting all particle forces in parallel, ensuring that previous force calculations do not interfere with the current computations. It then iterates over each particle, calculating the Lennard-Jones force based on the positions of neighboring particles. While this implementation updates only the force on p1
, fully adhering to Newton's third law in a parallel context would require a more sophisticated approach to safely update p2
's force without introducing data races.
Optimizing Performance with SIMD and Vectorization
Single Instruction, Multiple Data (SIMD) is a parallel computing architecture that allows the simultaneous processing of multiple data points with a single instruction. SIMD can significantly accelerate vector operations, such as those involved in force calculations and position updates in MD simulations.
Rust's support for SIMD through the packed_simd
or std::simd
modules enables developers to leverage these hardware capabilities directly. By structuring data to align with SIMD requirements and utilizing vectorized operations, simulations can achieve substantial performance gains.
Here is an example of utilizing SIMD for force calculations in Rust:
use rand::Rng; // For random number generation
use std::simd::{f64x4, Simd};
// Define a struct to represent a particle
#[derive(Debug, Clone)]
struct Particle {
position: [f64; 3], // 3D position coordinates
force: [f64; 3], // 3D force components
mass: f64, // Mass of the particle
}
impl Particle {
// Constructor to create a new Particle
fn new(position: [f64; 3], mass: f64) -> Self {
Self {
position,
force: [0.0, 0.0, 0.0],
mass,
}
}
// Method to reset the force to zero before recalculating
fn reset_force(&mut self) {
self.force = [0.0, 0.0, 0.0];
}
}
// Function to calculate forces using SIMD
fn calculate_forces_simd(particles: &mut [Particle], epsilon: f64, sigma: f64) {
// Reset all forces
for p in particles.iter_mut() {
p.reset_force();
}
// Iterate over all unique particle pairs
for i in 0..particles.len() {
let p1 = &particles[i];
for j in (i + 1)..particles.len() {
let p2 = &particles[j];
let mut distance_sq = 0.0;
for k in 0..3 {
let diff = p1.position[k] - p2.position[k];
distance_sq += diff * diff;
}
if distance_sq > 0.0 {
let distance = distance_sq.sqrt();
let force_magnitude = 24.0 * epsilon * ((2.0 / distance_sq.powf(7.0)) - (1.0 / distance_sq.powf(4.0)));
for k in 0..3 {
let force_component = force_magnitude * (p1.position[k] - p2.position[k]);
particles[i].force[k] += force_component;
particles[j].force[k] -= force_component; // Newton's third law
}
}
}
}
}
fn main() {
// Initialize random number generator
let mut rng = rand::thread_rng();
// Define simulation parameters
let n_particles = 1000; // Number of particles
let epsilon = 1.0; // Lennard-Jones potential parameter
let sigma = 1.0; // Lennard-Jones potential parameter
// Initialize particles with random positions and zero velocities
let mut particles: Vec<Particle> = (0..n_particles)
.map(|_| {
Particle::new(
[
rng.gen_range(0.0..10.0),
rng.gen_range(0.0..10.0),
rng.gen_range(0.0..10.0),
],
1.0,
)
})
.collect();
// Calculate forces using SIMD
calculate_forces_simd(&mut particles, epsilon, sigma);
// Output the forces for verification
for (i, particle) in particles.iter().enumerate().take(5) {
println!("Particle {}: Force = {:?}", i + 1, particle.force);
}
// Further simulation steps, such as integrating equations of motion, would go here
}
In this SIMD-based example, the calculate_forces_simd
function performs force calculations using standard scalar operations. While this example does not fully exploit SIMD capabilities, it lays the groundwork for integrating SIMD instructions into the force calculation process. To fully leverage SIMD, one would need to utilize SIMD-specific data structures and operations, aligning data in memory to match SIMD register sizes and employing vectorized instructions for simultaneous computations.
Conclusion
Parallelization and optimization are fundamental to the advancement of Molecular Dynamics simulations, enabling the efficient handling of large-scale systems and complex interactions. By distributing computational tasks across multiple processors or cores, MD simulations can achieve significant performance improvements, reducing the time required for force calculations, integration, and other critical steps. Techniques such as domain decomposition and task parallelism facilitate the effective scaling of simulations, ensuring that computational resources are utilized to their fullest potential.
Rust’s robust concurrency model, coupled with powerful libraries like Rayon, provides a solid foundation for implementing parallel and optimized MD simulations. The language’s emphasis on safety and performance ensures that parallel operations are executed reliably without compromising the integrity of the simulation. Additionally, Rust’s support for SIMD and GPU acceleration offers avenues for further enhancing simulation performance, enabling researchers and developers to push the boundaries of computational physics and materials science.
Through thoughtful design and the strategic application of parallelization techniques, developers can create highly efficient and scalable MD simulation frameworks in Rust. These frameworks not only handle the computational demands of large-scale simulations but also maintain the accuracy and reliability essential for meaningful scientific analysis and technological innovation.
17.6. Numerical Integration Methods
Numerical integration methods are fundamental to Molecular Dynamics (MD) simulations, as they dictate how the positions and velocities of particles evolve over time. Among the plethora of algorithms available, the Verlet and Leapfrog methods are prominently utilized due to their simplicity, stability, and capacity to conserve energy over extended simulations. Additionally, Predictor-Corrector methods offer enhanced accuracy by iteratively refining predictions of particle states. The selection of an appropriate integration method significantly impacts the simulation's accuracy, stability, and computational efficiency.
Verlet Integration is a widely adopted method in MD simulations. It leverages the positions of particles at the current and previous time steps to compute their future positions. The fundamental Verlet formula is expressed as:
$$\mathbf{r}(t + \Delta t) = 2\mathbf{r}(t) - \mathbf{r}(t - \Delta t) + \Delta t^2 \mathbf{a}(t)$$
In this equation, r(t)\\mathbf{r}(t) denotes the position of a particle at time tt, Δt\\Delta t is the time step, and a(t)\\mathbf{a}(t) represents the acceleration at time tt, derived from the forces acting on the particle. The Verlet algorithm is advantageous because it circumvents the need for explicit velocity calculations, rendering it computationally efficient. However, if velocities are required, they must be estimated from the positional data, which can introduce slight inaccuracies.
Leapfrog Integration offers a variation of the Verlet method by providing a more direct means of calculating velocities. In this approach, velocities are computed at half-time steps (e.g., $t + \frac{\Delta t}{2}$) and "leapfrog" over the positions, which are updated at full time steps. The Leapfrog integration equations are:
$$\mathbf{v}\left(t + \frac{\Delta t}{2}\right) = \mathbf{v}\left(t - \frac{\Delta t}{2}\right) + \Delta t $$
$$\mathbf{r}(t + \Delta t) = \mathbf{r}(t) + \Delta t \mathbf{v}\left(t + \frac{\Delta t}{2}\right)$$
The Leapfrog method enhances the alignment between velocities and positions, facilitating more accurate calculations of kinetic energy and other dynamic properties.
Predictor-Corrector Methods represent a class of advanced integration techniques that enhance accuracy by first predicting the future state of particles and subsequently correcting these predictions based on newly calculated forces. This two-step process involves a prediction phase, where future positions and velocities are estimated using a basic integration method like Verlet or Leapfrog, followed by a correction phase that refines these estimates using higher-order calculations. For example, in a typical Predictor-Corrector scheme, the Predictor Step involves calculating predicted positions and velocities using the current state and forces. Subsequently, the Corrector Step recalculates the forces based on these predicted positions and adjusts the positions and velocities accordingly to enhance accuracy. Predictor-Corrector methods are particularly beneficial for simulations requiring high precision over extended time scales, as they mitigate the accumulation of numerical errors.
Stability and Accuracy are pivotal considerations when selecting a numerical integration method. Stability pertains to the algorithm's ability to produce realistic results over prolonged simulation periods without the solution diverging. Accuracy refers to the degree to which the numerical solution approximates the true physical behavior of the system. While methods like Verlet and Leapfrog offer a balance between stability and accuracy, higher-order methods such as Predictor-Corrector schemes provide enhanced precision at the expense of increased computational complexity.
Energy Conservation is a critical factor in MD simulations. An effective integration method should maintain the total energy of the system, ensuring that any observed energy fluctuations are solely due to the physical interactions being modeled and not artifacts of the numerical method. Instabilities or inaccuracies in the integration process can lead to energy drift, where the system's total energy unrealistically increases or decreases over time.
Time Step Selection ($\Delta t$) is another crucial parameter in MD simulations. The time step must be sufficiently small to accurately capture the fastest dynamics within the system, such as bond vibrations, while being large enough to allow the simulation to progress over meaningful physical timescales. An excessively large time step can render the simulation unstable, leading to significant errors or failure, whereas a too-small time step can result in prohibitively long simulation times.
Adaptive Time-Stepping techniques offer a solution by dynamically adjusting the time step based on the system's state. During periods of rapid change, the time step can be reduced to maintain accuracy, while during more stable periods, it can be increased to enhance computational efficiency. This adaptability ensures that the simulation remains both accurate and efficient across varying conditions.
Implementing these numerical integration methods in Rust leverages the language's strengths in safety, performance, and concurrency. Below are detailed implementations of the Verlet and Leapfrog integration methods, followed by an example of adaptive time-stepping.
Verlet Integration in Rust
The Verlet integration method updates particle positions based on their current and previous positions, as well as the current forces acting upon them. Below is a robust implementation in Rust:
// Import necessary crates
use rand::Rng; // For random number generation
// Define a struct to represent a particle
#[derive(Debug, Clone)]
struct Particle {
position: [f64; 3], // Current position coordinates
velocity: [f64; 3], // Current velocity components
force: [f64; 3], // Current force components
mass: f64, // Mass of the particle
prev_position: [f64; 3], // Previous position coordinates
}
impl Particle {
// Constructor to create a new Particle
fn new(position: [f64; 3], mass: f64) -> Self {
Self {
position,
velocity: [0.0, 0.0, 0.0],
force: [0.0, 0.0, 0.0],
mass,
prev_position: position, // Initialize previous position to current position
}
}
// Method to reset the force to zero before recalculating
fn reset_force(&mut self) {
self.force = [0.0, 0.0, 0.0];
}
}
// Function to perform Verlet integration
fn verlet_integration(particles: &mut [Particle], dt: f64) {
for particle in particles.iter_mut() {
let mut new_position = [0.0; 3];
for i in 0..3 {
// Verlet position update formula
new_position[i] = 2.0 * particle.position[i] - particle.prev_position[i]
+ (particle.force[i] / particle.mass) * dt * dt;
// Update previous position
particle.prev_position[i] = particle.position[i];
// Update current position
particle.position[i] = new_position[i];
}
}
}
fn main() {
// Initialize random number generator
let mut rng = rand::thread_rng();
// Define simulation parameters
let dt = 0.01; // Time step
let mass = 1.0; // Mass of each particle
// Initialize particles with random positions
let mut particles = vec![
Particle::new(
[0.0, 0.0, 0.0], // Initial position
mass,
),
Particle::new(
[1.0, 1.0, 1.0], // Initial position
mass,
),
// Add more particles as needed
];
// Example: Assign initial forces (for demonstration purposes)
for particle in particles.iter_mut() {
particle.force = [1.0, 0.0, 0.0]; // Example force
}
// Perform Verlet integration
verlet_integration(&mut particles, dt);
// Output the updated positions for verification
println!("After Verlet Integration:");
for (i, particle) in particles.iter().enumerate() {
println!(
"Particle {}: Position = {:?}, Previous Position = {:?}",
i + 1,
particle.position,
particle.prev_position
);
}
// Further simulation steps, such as force recalculations, would follow
}
In this implementation, the Particle
struct includes fields for the current position, velocity, force, mass, and previous position of each particle. The verlet_integration
function iterates over each particle, updating its position based on the Verlet formula. The current position is stored as the previous position before updating to the new position. This method is efficient because it avoids the need to explicitly calculate velocities at each time step. However, if velocities are required for analysis or further computations, they must be derived from the positional data.
Leapfrog Integration in Rust
The Leapfrog integration method updates velocities at half-time steps and positions at full time steps, providing a more direct approach to velocity calculations. Below is an implementation in Rust:
// Import necessary crates
use rand::Rng; // For random number generation
// Define a struct to represent a particle
#[derive(Debug, Clone)]
struct Particle {
position: [f64; 3], // Current position coordinates
velocity: [f64; 3], // Current velocity components
force: [f64; 3], // Current force components
mass: f64, // Mass of the particle
}
impl Particle {
// Constructor to create a new Particle
fn new(position: [f64; 3], mass: f64) -> Self {
Self {
position,
velocity: [0.0, 0.0, 0.0],
force: [0.0, 0.0, 0.0],
mass,
}
}
// Method to reset the force to zero before recalculating
fn reset_force(&mut self) {
self.force = [0.0, 0.0, 0.0];
}
}
// Function to perform Leapfrog integration
fn leapfrog_integration(particles: &mut [Particle], dt: f64) {
for particle in particles.iter_mut() {
for i in 0..3 {
// Update velocity by half step
particle.velocity[i] += 0.5 * (particle.force[i] / particle.mass) * dt;
// Update position by full step
particle.position[i] += particle.velocity[i] * dt;
// Update velocity by another half step
particle.velocity[i] += 0.5 * (particle.force[i] / particle.mass) * dt;
}
}
}
fn main() {
// Initialize random number generator
let mut rng = rand::thread_rng();
// Define simulation parameters
let dt = 0.01; // Time step
let mass = 1.0; // Mass of each particle
// Initialize particles with random positions
let mut particles = vec![
Particle::new(
[0.0, 0.0, 0.0], // Initial position
mass,
),
Particle::new(
[1.0, 1.0, 1.0], // Initial position
mass,
),
// Add more particles as needed
];
// Example: Assign initial forces (for demonstration purposes)
for particle in particles.iter_mut() {
particle.force = [0.0, 1.0, 0.0]; // Example force
}
// Perform Leapfrog integration
leapfrog_integration(&mut particles, dt);
// Output the updated positions and velocities for verification
println!("After Leapfrog Integration:");
for (i, particle) in particles.iter().enumerate() {
println!(
"Particle {}: Position = {:?}, Velocity = {:?}",
i + 1,
particle.position,
particle.velocity
);
}
// Further simulation steps, such as force recalculations, would follow
}
In this implementation, the Particle
struct contains fields for the current position, velocity, force, and mass of each particle. The leapfrog_integration
function updates the velocity of each particle by half a time step, then updates the position by a full time step using the updated velocity, and finally updates the velocity by another half time step. This staggered update ensures that velocities are effectively "leaping" over positions, maintaining better energy conservation and providing a more accurate representation of particle motion over time.
Predictor-Corrector Integration in Rust
Predictor-Corrector methods enhance the accuracy of numerical integration by iteratively refining predictions of particle states. Below is an implementation of a simple Predictor-Corrector scheme in Rust:
// Import necessary crates
use rand::Rng; // For random number generation
// Define a struct to represent a particle
#[derive(Debug, Clone)]
struct Particle {
position: [f64; 3], // Current position coordinates
velocity: [f64; 3], // Current velocity components
force: [f64; 3], // Current force components
mass: f64, // Mass of the particle
}
impl Particle {
// Constructor to create a new Particle
fn new(position: [f64; 3], mass: f64) -> Self {
Self {
position,
velocity: [0.0, 0.0, 0.0],
force: [0.0, 0.0, 0.0],
mass,
}
}
// Method to reset the force to zero before recalculating
fn reset_force(&mut self) {
self.force = [0.0, 0.0, 0.0];
}
}
// Function to perform Predictor-Corrector integration
fn predictor_corrector_integration(
particles: &mut [Particle],
dt: f64,
calculate_forces: fn(&mut [Particle]),
) {
// Predictor Step: Predict positions and velocities
let mut predicted_positions = vec![[0.0; 3]; particles.len()];
let mut predicted_velocities = vec![[0.0; 3]; particles.len()];
for (i, particle) in particles.iter().enumerate() {
for k in 0..3 {
// Predict position using current velocity and acceleration
predicted_positions[i][k] =
particle.position[k] + particle.velocity[k] * dt + 0.5 * (particle.force[k] / particle.mass) * dt * dt;
// Predict velocity using current acceleration
predicted_velocities[i][k] =
particle.velocity[k] + 0.5 * (particle.force[k] / particle.mass) * dt;
}
}
// Update particles with predicted positions and velocities
for (i, particle) in particles.iter_mut().enumerate() {
for k in 0..3 {
particle.position[k] = predicted_positions[i][k];
particle.velocity[k] = predicted_velocities[i][k];
}
}
// Recalculate forces based on predicted positions
calculate_forces(particles);
// Corrector Step: Refine positions and velocities
for (i, particle) in particles.iter_mut().enumerate() {
for k in 0..3 {
// Correct position based on new acceleration
particle.position[k] += 0.5 * (particle.force[k] / particle.mass) * dt * dt;
// Correct velocity based on new acceleration
particle.velocity[k] += 0.5 * (particle.force[k] / particle.mass) * dt;
}
}
}
fn main() {
// Initialize random number generator
let mut rng = rand::thread_rng();
// Define simulation parameters
let dt = 0.01; // Time step
let mass = 1.0; // Mass of each particle
// Initialize particles with random positions
let mut particles = vec![
Particle::new(
[0.0, 0.0, 0.0], // Initial position
mass,
),
Particle::new(
[1.0, 1.0, 1.0], // Initial position
mass,
),
// Add more particles as needed
];
// Example: Assign initial forces (for demonstration purposes)
for particle in particles.iter_mut() {
particle.force = [0.0, 1.0, 0.0]; // Example force
}
// Define a simple force calculation function (for demonstration)
fn simple_force_calculation(particles: &mut [Particle]) {
for particle in particles.iter_mut() {
// Example: Simple constant force in the y-direction
particle.force = [0.0, 1.0, 0.0];
}
}
// Perform Predictor-Corrector integration
predictor_corrector_integration(&mut particles, dt, simple_force_calculation);
// Output the updated positions and velocities for verification
println!("After Predictor-Corrector Integration:");
for (i, particle) in particles.iter().enumerate() {
println!(
"Particle {}: Position = {:?}, Velocity = {:?}",
i + 1,
particle.position,
particle.velocity
);
}
// Further simulation steps, such as additional force recalculations, would follow
}
In this implementation, the Particle
struct contains fields for the current position, velocity, force, and mass of each particle. The predictor_corrector_integration
function performs the Predictor-Corrector integration by first predicting the future positions and velocities based on the current state and forces. It then updates the particles with these predicted values and recalculates the forces based on the new positions. Finally, it corrects the predicted positions and velocities using the newly calculated forces to enhance accuracy. This method is particularly useful for simulations that demand high precision over long time scales, as it effectively reduces the accumulation of numerical errors.
Adaptive Time-Stepping in Rust
Adaptive time-stepping adjusts the simulation's time step dynamically based on the system's state, ensuring both accuracy and computational efficiency. Below is an implementation of a simple adaptive time-stepping scheme in Rust:
// Import necessary crates
use rand::Rng; // For random number generation
// Define a struct to represent a particle
#[derive(Debug, Clone)]
struct Particle {
position: [f64; 3], // Current position coordinates
velocity: [f64; 3], // Current velocity components
force: [f64; 3], // Current force components
mass: f64, // Mass of the particle
prev_position: [f64; 3], // Previous position coordinates
}
impl Particle {
// Constructor to create a new Particle
fn new(position: [f64; 3], mass: f64) -> Self {
Self {
position,
velocity: [0.0, 0.0, 0.0],
force: [0.0, 0.0, 0.0],
mass,
prev_position: position, // Initialize previous position to current position
}
}
// Method to reset the force to zero before recalculating
fn reset_force(&mut self) {
self.force = [0.0, 0.0, 0.0];
}
}
// Function to calculate forces between particles (simple harmonic force for demonstration)
fn calculate_forces(particles: &mut [Particle]) {
// Reset all forces
for particle in particles.iter_mut() {
particle.reset_force();
}
// Simple pairwise force calculation
for i in 0..particles.len() {
for j in (i + 1)..particles.len() {
let mut distance_sq = 0.0;
let mut distance = 0.0;
for k in 0..3 {
let diff = particles[i].position[k] - particles[j].position[k];
distance_sq += diff * diff;
}
if distance_sq > 0.0 {
distance = distance_sq.sqrt();
// Example: Simple harmonic force (Hooke's law)
let k_constant = 1.0; // Spring constant
let force_magnitude = -k_constant * (distance - 1.0); // Assuming equilibrium distance is 1.0
for k in 0..3 {
let diff = particles[i].position[k] - particles[j].position[k];
let force_component = force_magnitude * (diff / distance);
particles[i].force[k] += force_component;
particles[j].force[k] -= force_component; // Newton's third law
}
}
}
}
}
// Function to perform a full simulation step with adaptive time-stepping using Verlet integration
fn simulation_step_adaptive_verlet(
particles: &mut [Particle],
dt: &mut f64,
calculate_forces: fn(&mut [Particle]),
max_force_threshold: f64,
min_dt: f64,
max_dt: f64,
) {
// Perform Verlet integration to update positions
for particle in particles.iter_mut() {
let mut new_position = [0.0; 3];
for i in 0..3 {
// Verlet position update formula
new_position[i] = 2.0 * particle.position[i] - particle.prev_position[i]
+ (particle.force[i] / particle.mass) * (*dt) * (*dt);
// Update previous position
particle.prev_position[i] = particle.position[i];
// Update current position
particle.position[i] = new_position[i];
}
}
// Recalculate forces based on new positions
calculate_forces(particles);
// Determine the maximum force magnitude across all particles
let mut max_force = 0.0;
for particle in particles.iter() {
for &f in &particle.force {
if f.abs() > max_force {
max_force = f.abs();
}
}
}
// Adjust the time step based on the maximum force
if max_force > max_force_threshold && *dt > min_dt {
*dt *= 0.5; // Reduce time step if forces are too large
println!("Time step reduced to {}", *dt);
} else if max_force < max_force_threshold / 2.0 && *dt < max_dt {
*dt *= 1.1; // Increase time step if forces are small
println!("Time step increased to {}", *dt);
}
}
fn main() {
// Initialize random number generator
let mut rng = rand::thread_rng();
// Define simulation parameters
let mut dt = 0.01; // Initial time step
let mass = 1.0; // Mass of each particle
let total_steps = 100; // Total number of simulation steps
let max_force_threshold = 10.0; // Threshold to reduce time step
let min_dt = 0.001; // Minimum allowable time step
let max_dt = 0.1; // Maximum allowable time step
// Initialize particles with random positions
let mut particles = vec![
Particle::new(
[0.0, 0.0, 0.0], // Initial position
mass,
),
Particle::new(
[1.0, 1.0, 1.0], // Initial position
mass,
),
// Add more particles as needed
];
// Example: Assign initial velocities (if needed)
for particle in particles.iter_mut() {
particle.velocity = [0.0, 0.0, 0.0]; // Initial velocity
}
// Initial force calculation
calculate_forces(&mut particles);
// Perform simulation steps with adaptive time-stepping
for step in 0..total_steps {
simulation_step_adaptive_verlet(
&mut particles,
&mut dt,
calculate_forces,
max_force_threshold,
min_dt,
max_dt,
);
// Output the positions, velocities, and current time step of the first two particles for verification
println!("Step {}:", step + 1);
for (i, particle) in particles.iter().enumerate().take(2) {
println!(
"Particle {}: Position = {:?}, Velocity = {:?}, Force = {:?}",
i + 1,
particle.position,
particle.velocity,
particle.force
);
}
println!("Current time step: {}\n", dt);
}
// Further analysis or data output would follow
}
In this implementation, the Particle
struct includes fields for the current position, velocity, force, mass, and previous position of each particle. The calculate_forces
function resets all forces and computes pairwise forces between particles based on a simple harmonic (Hooke's law) model, assuming an equilibrium distance of 1.0 units. The simulation_step_adaptive_verlet
function performs the Verlet integration to update particle positions and recalculates forces based on these new positions. It then determines the maximum force magnitude across all particles to decide whether to adjust the time step. If the maximum force exceeds a predefined threshold, the time step is reduced to maintain stability. Conversely, if the maximum force is below half the threshold, the time step is increased to enhance computational efficiency. The time step is constrained within minimum and maximum bounds to prevent excessive reductions or increases.
This adaptive approach ensures that the simulation remains accurate during periods of rapid change by using smaller time steps, while also improving efficiency during more stable periods by allowing larger time steps. The main
function initializes the particles, assigns initial forces, and performs multiple simulation steps, adjusting the time step adaptively based on the forces experienced by the particles. After each step, it prints the positions, velocities, and current time step of the first two particles for verification purposes.
Advantages of Adaptive Time-Stepping:
Adaptive time-stepping offers enhanced accuracy by adjusting the time step based on the system's dynamics, ensuring that rapid changes are captured with sufficient resolution while maintaining efficiency during stable periods. This flexibility helps in minimizing energy drift and maintaining energy conservation, as the simulation can respond dynamically to varying force magnitudes. Additionally, by avoiding unnecessarily small time steps during stable periods, computational resources are utilized more effectively, allowing for longer simulations within practical timeframes.
Considerations for Further Enhancements:
To further enhance the adaptive time-stepping mechanism, multiple criteria can be incorporated for adjusting the time step, such as kinetic energy fluctuations or local acceleration measurements. Combining adaptive time-stepping with higher-order integration methods like Runge-Kutta can also provide improved accuracy and stability. Additionally, leveraging Rust's concurrency features can enable parallelization of integration steps, significantly boosting performance, especially in large-scale simulations involving numerous particles.
Numerical integration methods are integral to the fidelity and efficiency of Molecular Dynamics simulations. The Verlet and Leapfrog methods offer a balance between simplicity and stability, making them suitable for a wide range of applications. Predictor-Corrector schemes provide enhanced accuracy at the cost of increased computational complexity, catering to simulations demanding high precision over extended periods. Adaptive time-stepping introduces a dynamic approach to maintaining both accuracy and efficiency, adjusting the computational effort based on the system's current state.
Implementing these integration methods in Rust leverages the language's strengths in safety, performance, and concurrency. Rust's ownership model ensures memory safety without sacrificing speed, while its robust type system and concurrency primitives facilitate the development of reliable and high-performance simulation frameworks. Profiling and optimization further enhance these frameworks, ensuring that they meet the demanding requirements of modern computational physics and materials science research.
Through meticulous design and the strategic application of numerical integration techniques, combined with Rust's performance-oriented capabilities, MD simulations can achieve unprecedented levels of accuracy and efficiency. This synergy empowers researchers and developers to explore intricate molecular phenomena, driving advancements in various scientific and industrial domains.
17.7. Analysis of Molecular Dynamics Data
In Molecular Dynamics (MD) simulations, analyzing particle trajectories is a pivotal task following the completion of a simulation run. Trajectory analysis involves scrutinizing the time-dependent paths that particles traverse during the simulation. These trajectories encapsulate a wealth of information about the system's behavior, enabling researchers to extract essential physical properties such as temperature, pressure, and diffusion coefficients.
To undertake trajectory analysis, it is customary to record the positions and, occasionally, the velocities of particles at each simulation time step. By meticulously examining these trajectories, one can deduce how particles interact, how energy is distributed throughout the system, and how the system evolves over time. For instance, calculating the mean squared displacement (MSD) of particles facilitates the determination of diffusion coefficients, offering insights into the mobility of particles within the simulated environment.
MD simulations are adept at probing both the structural properties and dynamic behavior of a system. Structural properties relate to the spatial arrangement of particles, encompassing phenomena such as cluster formation, crystalline structures, or the spatial distribution of particles around a reference point. These properties are often investigated using metrics like the radial distribution function (RDF), which elucidates how particle density varies with distance from a reference particle.
Conversely, dynamic behavior pertains to the temporal evolution of the system, encompassing the movement of particles, the transfer of energy, and the system's response to external perturbations. Time correlation functions, such as the velocity autocorrelation function (VACF), are instrumental in studying these dynamic aspects. These functions reveal how the velocity of a particle at one moment correlates with its velocity at a subsequent time, thereby providing insights into relaxation times and transport properties.
The radial distribution function (RDF), denoted as $g(r)$, serves as a fundamental tool for analyzing the spatial distribution of particles in MD simulations. The RDF quantifies the probability of finding a particle at a distance $r$ from a reference particle relative to the probability expected for a completely random distribution at the same density. Mathematically, the RDF is defined as:
$$g(r) = \frac{V}{N^2} \left\langle \sum_{i=1}^{N} \sum_{j \neq i}^{N} \delta(r - |\mathbf{r}_i - \mathbf{r}_j|) \right\rangle$$
Here, $V$ represents the system's volume, $N$ is the number of particles, and $\delta$ is the Dirac delta function. Typically, the RDF commences at zero for very short distances, ascends to a peak corresponding to the nearest-neighbor distance, and subsequently oscillates around one, reflecting the average density.
The RDF is invaluable for discerning the local structure of various phases such as liquids, gases, and amorphous solids. A pronounced peak in the RDF at a specific distance indicates a high probability of finding particles separated by that distance, often corresponding to characteristic bond lengths in liquids or solids.
Diffusion coefficients are critical for characterizing particle mobility within a medium. The diffusion coefficient $D$ can be derived from the mean squared displacement (MSD) of particles using the Einstein relation:
$D = \frac{1}{2d} \lim_{t \to \infty} \frac{d}{dt} \langle |\mathbf{r}(t) - \mathbf{r}(0)|^2 \rangle$
In this equation, dd denotes the system's dimensionality, and $\langle |\mathbf{r}(t) - \mathbf{r}(0)|^2 \rangle$ represents the MSD of particles over time $t$.
Time correlation functions, such as the velocity autocorrelation function (VACF), offer profound insights into the system's dynamic behavior. The VACF is defined as:
$$C_v(t) = \langle \mathbf{v}(t) \cdot \mathbf{v}(0) \rangle$$
Where $\mathbf{v}(t)$ is the velocity of a particle at time tt, and $\mathbf{v}(0)$ is its initial velocity. The VACF typically decays over time as a particle's velocity becomes uncorrelated with its initial value, with the rate of decay providing information about the system's relaxation dynamics and transport properties.
Rust offers a robust platform for implementing analysis tools for MD simulations, leveraging its safety, performance, and concurrency features. Below, we illustrate how to construct tools for calculating RDFs and VACFs, as well as how to visualize and export the results.
Calculating the Radial Distribution Function (RDF) in Rust
The following Rust code calculates the RDF from a set of particle positions. The implementation considers periodic boundary conditions to account for particles interacting across the simulation box boundaries.
use std::f64::consts::PI;
/// Represents a particle with a position in 3D space.
struct Particle {
position: [f64; 3],
}
impl Particle {
/// Creates a new Particle with the given position.
fn new(position: [f64; 3]) -> Self {
Self { position }
}
}
/// Calculates the Radial Distribution Function (RDF) for a set of particles.
///
/// # Arguments
///
/// * `particles` - A slice of Particle instances.
/// * `bin_width` - The width of each distance bin.
/// * `num_bins` - The total number of bins.
/// * `box_length` - The length of the simulation box (assuming a cubic box).
///
/// # Returns
///
/// A vector containing the RDF values for each bin.
fn calculate_rdf(particles: &[Particle], bin_width: f64, num_bins: usize, box_length: f64) -> Vec<f64> {
let mut rdf = vec![0.0; num_bins];
let num_particles = particles.len();
let volume = box_length.powi(3);
let density = num_particles as f64 / volume;
// Iterate over all unique particle pairs
for i in 0..num_particles {
for j in (i + 1)..num_particles {
let mut distance_sq = 0.0;
// Calculate squared distance with periodic boundary conditions
for k in 0..3 {
let mut diff = particles[i].position[k] - particles[j].position[k];
// Apply minimum image convention
if diff > 0.5 * box_length {
diff -= box_length;
} else if diff < -0.5 * box_length {
diff += box_length;
}
distance_sq += diff * diff;
}
let distance = distance_sq.sqrt();
let bin_index = (distance / bin_width).floor() as usize;
if bin_index < num_bins {
rdf[bin_index] += 2.0; // Each pair is counted once
}
}
}
// Normalize the RDF
for bin_index in 0..num_bins {
let r1 = bin_index as f64 * bin_width;
let r2 = (bin_index as f64 + 1.0) * bin_width;
let shell_volume = (4.0 / 3.0) * PI * (r2.powi(3) - r1.powi(3));
rdf[bin_index] /= density * shell_volume * num_particles as f64;
}
rdf
}
fn main() {
// Example particle positions
let particles = vec![
Particle::new([0.0, 0.0, 0.0]),
Particle::new([1.0, 1.0, 1.0]),
// Additional particles can be initialized here
];
let bin_width = 0.1;
let num_bins = 100;
let box_length = 10.0;
let rdf = calculate_rdf(&particles, bin_width, num_bins, box_length);
// Further analysis or visualization of RDF can be performed here
// For example, printing the RDF values:
for (i, &value) in rdf.iter().enumerate() {
let r = i as f64 * bin_width + bin_width / 2.0;
println!("{:.2} {:.5}", r, value);
}
}
In this implementation, the Particle
struct encapsulates the position of each particle in three-dimensional space. The calculate_rdf
function computes the RDF by iterating over all unique pairs of particles, calculating their distances while applying periodic boundary conditions to account for interactions across the simulation box boundaries. The distances are then binned according to the specified bin_width
, and the RDF is normalized by the shell volume and particle density to yield meaningful probability distributions. The main
function demonstrates how to initialize a set of particles, compute the RDF, and output the results for further analysis or visualization.
Calculating the Velocity Autocorrelation Function (VACF) in Rust
The following Rust code calculates the Velocity Autocorrelation Function (VACF) from particle velocities. The VACF provides insights into the dynamic behavior of the system by measuring how particle velocities correlate over time.
/// Represents a particle with a velocity in 3D space.
struct Particle {
velocity: [f64; 3],
}
impl Particle {
/// Creates a new Particle with the given velocity.
fn new(velocity: [f64; 3]) -> Self {
Self { velocity }
}
}
/// Calculates the Velocity Autocorrelation Function (VACF) for a set of particles over multiple time steps.
///
/// # Arguments
///
/// * `particles` - A slice of Particle instances.
/// * `num_timesteps` - The number of time steps over which to calculate the VACF.
///
/// # Returns
///
/// A vector containing the VACF values for each time step.
fn calculate_vacf(particles: &[Particle], num_timesteps: usize) -> Vec<f64> {
let num_particles = particles.len();
let mut vacf = vec![0.0; num_timesteps];
for t in 0..num_timesteps {
for particle in particles {
// For demonstration, assume velocity remains constant over time
// In a real simulation, velocities would be updated at each time step
vacf[t] += particle.velocity.iter().map(|&v| v * v).sum::<f64>();
}
vacf[t] /= num_particles as f64;
}
vacf
}
fn main() {
// Example particle velocities
let particles = vec![
Particle::new([1.0, 0.0, 0.0]),
Particle::new([0.0, 1.0, 0.0]),
// Additional particles can be initialized here
];
let num_timesteps = 100;
let vacf = calculate_vacf(&particles, num_timesteps);
// Further analysis or visualization of VACF can be performed here
// For example, printing the VACF values:
for (t, &value) in vacf.iter().enumerate() {
println!("{} {:.5}", t, value);
}
}
In this code, the Particle
struct encapsulates the velocity of each particle in three-dimensional space. The calculate_vacf
function computes the VACF by averaging the dot products of particle velocities over a specified number of time steps. For demonstration purposes, this implementation assumes that velocities remain constant over time; however, in a real MD simulation, velocities would be dynamically updated at each time step based on the forces acting upon the particles. The main
function showcases how to initialize a set of particles, compute the VACF, and output the results for subsequent analysis or visualization.
Visualizing the Radial Distribution Function (RDF) Using Rust’s Plotters Crate
Rust's plotters
crate is a versatile tool for visualizing simulation data. The following example demonstrates how to plot the RDF calculated earlier, presenting it as a line graph for intuitive interpretation.
use plotters::prelude::*;
use std::error::Error;
/// Plots the Radial Distribution Function (RDF) and saves it as a PNG image.
///
/// # Arguments
///
/// * `rdf` - A slice containing RDF values.
/// * `bin_width` - The width of each distance bin.
///
/// # Returns
///
/// A Result indicating success or failure.
fn plot_rdf(rdf: &[f64], bin_width: f64) -> Result<(), Box<dyn Error>> {
// Create a drawing area with specified dimensions
let root = BitMapBackend::new("rdf.png", (800, 600)).into_drawing_area();
root.fill(&WHITE)?;
// Determine the maximum RDF value for scaling the y-axis
let max_rdf = rdf.iter().cloned().fold(0./0., f64::max);
// Build the chart with titles and labels
let mut chart = ChartBuilder::on(&root)
.caption("Radial Distribution Function (RDF)", ("sans-serif", 40))
.margin(20)
.x_label_area_size(40)
.y_label_area_size(60)
.build_cartesian_2d(0.0..(rdf.len() as f64 * bin_width), 0.0..max_rdf)?;
// Configure the mesh (grid and labels)
chart.configure_mesh()
.x_desc("Distance (r)")
.y_desc("g(r)")
.axis_desc_style(("sans-serif", 30))
.label_style(("sans-serif", 20))
.draw()?;
// Plot the RDF as a red line
chart.draw_series(LineSeries::new(
rdf.iter().enumerate().map(|(i, &y)| (i as f64 * bin_width + bin_width / 2.0, y)),
&RED,
))?
.label("g(r)")
.legend(|(x, y)| PathElement::new([(x, y), (x + 20, y)], &RED));
// Draw the legend
chart.configure_series_labels()
.background_style(&WHITE.mix(0.8))
.border_style(&BLACK)
.draw()?;
// Save the plot to a file
root.present()?;
Ok(())
}
fn main() {
// Example RDF data
let rdf = vec![0.0, 0.9, 1.2, 1.1, 1.0, 0.8, 1.3, 1.5, 1.0, 0.9];
let bin_width = 0.1;
// Plot the RDF and handle potential errors
if let Err(e) = plot_rdf(&rdf, bin_width) {
println!("Failed to plot RDF: {}", e);
} else {
println!("RDF plot saved as 'rdf.png'");
}
}
This example utilizes the plotters
crate to generate a visually appealing RDF plot. The plot_rdf
function takes RDF values and bin width as inputs, constructs a chart with appropriate titles and axis labels, and plots the RDF as a red line. The function also adds a legend for clarity. Upon execution, the RDF plot is saved as a PNG image named rdf.png
. The main
function provides sample RDF data for demonstration purposes, invoking the plot_rdf
function and handling any potential errors during the plotting process.
Analyzing Molecular Dynamics data is essential for extracting meaningful physical insights from simulation trajectories. Tools for calculating radial distribution functions and velocity autocorrelation functions enable researchers to probe the structural and dynamic properties of simulated systems. Rust's performance-oriented features, combined with its safety guarantees and concurrency capabilities, make it an excellent choice for implementing robust and efficient analysis tools. By leveraging Rust's powerful libraries, such as plotters
for visualization, researchers can develop comprehensive workflows that not only perform intricate calculations but also facilitate intuitive data interpretation. This synergy between advanced numerical methods and Rust's robust ecosystem empowers scientists to explore complex molecular phenomena with unprecedented accuracy and efficiency, driving forward advancements in computational physics and materials science.
17.8. Case Studies and Applications
Molecular Dynamics (MD) simulations have become indispensable tools across various scientific disciplines, including materials science, biophysics, and chemistry. These simulations empower researchers to investigate systems at the atomic or molecular level, unveiling insights that are often unattainable through experimental methods alone. By modeling the interactions between atoms and molecules, MD facilitates the prediction of material properties, the comprehension of biological processes, and the design of novel molecules or materials with targeted characteristics.
In the realm of materials science, MD simulations are pivotal for exploring the mechanical, thermal, and electronic properties of materials. For instance, researchers utilize MD to simulate the deformation of metals under stress, providing a detailed understanding of material failure at the atomic scale. Additionally, MD allows for the modeling of atomic diffusion in alloys, enabling the prediction of how materials evolve over time under various conditions.
Biophysics leverages MD simulations to delve into the structure and dynamics of biological molecules such as proteins, DNA, and lipid membranes. A prominent application is the study of protein folding, where MD elucidates the pathways through which proteins attain their functional three-dimensional structures. This understanding is crucial for drug design, as the precise structure of a protein often dictates its interactions with other molecules, influencing the efficacy of pharmaceutical agents.
In the field of chemistry, MD simulations provide profound insights into chemical reactions at the molecular level. By simulating the movements of atoms and electrons, chemists can explore reaction mechanisms, energy transfer processes, and the stability of reaction intermediates. This information is invaluable for designing new chemical processes and catalysts, fostering advancements in synthetic chemistry and industrial applications.
MD simulations are instrumental in addressing real-world scientific challenges. For example, in the study of protein folding, comprehending how proteins achieve their functional conformations is essential for numerous areas in biology and medicine. MD simulations enable researchers to visualize the folding process, identify intermediate states, and investigate how varying conditions such as temperature or pH influence the folding pathway. This knowledge is vital for developing drugs that can stabilize or destabilize specific protein structures, a key strategy in treating diseases like Alzheimer's and cancer.
In materials science, MD simulations are employed to predict how materials behave under extreme conditions, such as high pressure or temperature. For example, in aerospace applications, materials are subjected to extreme temperatures and mechanical stresses. MD simulations allow engineers to understand how materials respond at the atomic level, facilitating the design of more durable materials capable of withstanding these challenging environments.
Custom MD frameworks are often essential for specialized applications that demand unique features or optimizations. For instance, a framework tailored for simulating biological molecules might incorporate specialized force fields that account for hydrogen bonding, electrostatics, and solvation effects. Conversely, a framework designed for materials simulation might prioritize accurate modeling of long-range interactions or quantum effects. Rust proves to be an excellent language for developing such custom frameworks due to its emphasis on safety, performance, and concurrency.
In Rust, building a custom MD framework involves designing modular and reusable components that can be easily extended or modified. For example, one might architect the framework so that force fields, integrators, and boundary conditions are implemented as separate modules. This modularity allows users to swap out or customize these components based on their specific research needs. Rust's strong type system and ownership model ensure that these components interact reliably, minimizing the risk of errors in complex simulations.
Interfacing Rust-based MD simulations with other computational tools and environments is crucial for enhancing their capabilities and integrating them into broader research workflows. For example, Rust can serve as the backbone for the core MD simulation engine, while Python might be utilized for pre-processing simulation parameters, post-processing data, or controlling simulation workflows through scripting. Rust's interoperability with other languages facilitates seamless integration, enabling researchers to leverage the strengths of multiple programming environments.
To facilitate this integration, Rust offers tools like PyO3, which allows Rust code to be exposed to Python seamlessly. This capability enables researchers to write performance-critical components of their simulations in Rust while utilizing Python for more flexible, high-level tasks such as data analysis or visualization. Additionally, Rust's Foreign Function Interface (FFI) permits interaction with libraries written in C or C++, enabling the reuse of existing codebases or the integration of Rust with other scientific software.
To illustrate the practical application of MD simulations in Rust, we will explore a case study in materials science where we simulate the behavior of a crystalline solid under mechanical stress. The objective is to observe how the material deforms and analyze the resulting stress-strain relationships at the atomic level.
Let us begin by defining a basic MD simulation framework in Rust to simulate the deformation of a crystal lattice. We will employ the Lennard-Jones potential to model the interactions between atoms.
Simulating Crystalline Deformation with the Lennard-Jones Potential
The following Rust code sets up a simple MD simulation where particles in a crystal lattice interact via the Lennard-Jones potential. This simulation aims to observe how the material responds to mechanical stress by analyzing the stress-strain relationship at the atomic level.
use std::f64::consts::PI;
/// Represents a particle in 3D space with position, velocity, force, and mass.
struct Particle {
position: [f64; 3],
velocity: [f64; 3],
force: [f64; 3],
mass: f64,
}
impl Particle {
/// Creates a new Particle with the given position and mass.
fn new(position: [f64; 3], mass: f64) -> Self {
Self {
position,
velocity: [0.0, 0.0, 0.0],
force: [0.0, 0.0, 0.0],
mass,
}
}
/// Resets the force acting on the particle to zero.
fn reset_force(&mut self) {
self.force = [0.0; 3];
}
}
/// Calculates the Lennard-Jones force between two particles.
///
/// # Arguments
///
/// * `p1` - Reference to the first Particle.
/// * `p2` - Reference to the second Particle.
/// * `epsilon` - Depth of the potential well.
/// * `sigma` - Finite distance at which the inter-particle potential is zero.
///
/// # Returns
///
/// A 3-element array representing the force vector exerted on p1 by p2.
fn lennard_jones(p1: &Particle, p2: &Particle, epsilon: f64, sigma: f64) -> [f64; 3] {
let mut r_vec = [0.0; 3];
let mut r2 = 0.0;
for i in 0..3 {
r_vec[i] = p1.position[i] - p2.position[i];
r2 += r_vec[i] * r_vec[i];
}
let r2_inv = 1.0 / r2;
let r6_inv = r2_inv * r2_inv * r2_inv;
let force_scalar = 24.0 * epsilon * r6_inv * (2.0 * r6_inv - 1.0) * r2_inv;
let mut force = [0.0; 3];
for i in 0..3 {
force[i] = force_scalar * r_vec[i];
}
force
}
/// Applies the Lennard-Jones forces to all unique particle pairs in the system.
///
/// # Arguments
///
/// * `particles` - Mutable reference to the vector of Particles.
/// * `epsilon` - Depth of the potential well.
/// * `sigma` - Finite distance at which the inter-particle potential is zero.
fn apply_forces(particles: &mut Vec<Particle>, epsilon: f64, sigma: f64) {
// Reset all forces before recalculating
for particle in particles.iter_mut() {
particle.reset_force();
}
// Iterate over all unique pairs of particles
for i in 0..particles.len() {
for j in (i + 1)..particles.len() {
let force = lennard_jones(&particles[i], &particles[j], epsilon, sigma);
for k in 0..3 {
particles[i].force[k] += force[k];
particles[j].force[k] -= force[k]; // Newton's third law
}
}
}
}
/// Performs Verlet integration to update particle positions and velocities.
///
/// # Arguments
///
/// * `particles` - Mutable reference to the vector of Particles.
/// * `dt` - Time step for the integration.
fn verlet_integration(particles: &mut Vec<Particle>, dt: f64) {
for particle in particles.iter_mut() {
for i in 0..3 {
// Update position based on current and previous positions, and current force
let new_position = 2.0 * particle.position[i]
- particle.velocity[i] * dt
+ (particle.force[i] / particle.mass) * dt * dt;
// Update velocity based on average force over the time step
particle.velocity[i] += 0.5 * (particle.force[i] / particle.mass) * dt;
// Assign the new position
particle.position[i] = new_position;
}
}
}
fn main() {
// Initialize particles to form a simple cubic lattice
let mut particles = vec![
Particle::new([0.0, 0.0, 0.0], 1.0),
Particle::new([1.0, 0.0, 0.0], 1.0),
Particle::new([0.0, 1.0, 0.0], 1.0),
Particle::new([1.0, 1.0, 0.0], 1.0),
Particle::new([0.0, 0.0, 1.0], 1.0),
Particle::new([1.0, 0.0, 1.0], 1.0),
Particle::new([0.0, 1.0, 1.0], 1.0),
Particle::new([1.0, 1.0, 1.0], 1.0),
];
let epsilon = 1.0; // Depth of the potential well
let sigma = 1.0; // Distance at which the potential is zero
let dt = 0.01; // Time step
// Simulation loop: apply forces and integrate positions
for step in 0..1000 {
apply_forces(&mut particles, epsilon, sigma);
verlet_integration(&mut particles, dt);
// Optional: Output particle positions at specific intervals
if step % 100 == 0 {
println!("Step {}:", step);
for (i, particle) in particles.iter().enumerate() {
println!(
"Particle {}: Position = {:?}, Velocity = {:?}",
i + 1,
particle.position,
particle.velocity
);
}
println!();
}
}
// Further analysis of stress-strain relationships or deformation behavior can be performed here
}
In this implementation, the Particle
struct encapsulates each particle's position, velocity, force, and mass. The lennard_jones
function computes the Lennard-Jones force between two particles, adhering to the potential's mathematical formulation. The apply_forces
function iterates over all unique particle pairs, calculates the forces using the Lennard-Jones potential, and updates each particle's force vector accordingly, ensuring compliance with Newton's third law.
The verlet_integration
function updates each particle's position and velocity using the Verlet integration method. This approach efficiently calculates the new positions based on current and previous positions and updates the velocities based on the average force exerted over the time step.
The main
function initializes a simple cubic lattice of particles and runs the simulation loop for a specified number of steps. At regular intervals, it prints the positions and velocities of the particles, facilitating the observation of the system's evolution under mechanical stress.
Enhancing Performance with Parallelization
To assess the performance of this Rust-based MD simulation, we can compare it with implementations in other languages such as Python or C++. Rust is renowned for its high performance, rivaling that of C++, while its memory safety features reduce the likelihood of certain bugs prevalent in lower-level languages. Furthermore, Rust's ownership model facilitates safe parallelization, which can significantly boost performance, especially in large-scale simulations.
By integrating Rust's rayon
crate, we can parallelize the force calculations, distributing the computational load across multiple threads. This parallelization can lead to substantial speedups on multi-core processors.
use rayon::prelude::*;
use std::sync::{Arc, Mutex};
/// Represents a particle in the system
#[derive(Debug, Clone)]
struct Particle {
position: [f64; 3], // 3D position coordinates
velocity: [f64; 3], // 3D velocity components
force: [f64; 3], // 3D force components
mass: f64, // Mass of the particle
}
impl Particle {
/// Creates a new particle
fn new(position: [f64; 3], mass: f64) -> Self {
Self {
position,
velocity: [0.0; 3],
force: [0.0; 3],
mass,
}
}
/// Resets the force on the particle to zero
fn reset_force(&mut self) {
self.force = [0.0; 3];
}
}
/// Calculates the Lennard-Jones force between two particles
///
/// # Arguments
///
/// * `p1` - The first particle.
/// * `p2` - The second particle.
/// * `epsilon` - Depth of the potential well.
/// * `sigma` - Finite distance at which the inter-particle potential is zero.
///
/// # Returns
///
/// A 3D force vector applied on `p1` due to `p2`.
fn lennard_jones(p1: &Particle, p2: &Particle, epsilon: f64, sigma: f64) -> [f64; 3] {
let mut distance_sq = 0.0;
let mut diff = [0.0; 3];
// Calculate the squared distance and difference vector
for k in 0..3 {
diff[k] = p1.position[k] - p2.position[k];
distance_sq += diff[k] * diff[k];
}
if distance_sq == 0.0 {
return [0.0; 3]; // Avoid division by zero for identical particles
}
let distance = distance_sq.sqrt();
let r2_inv = sigma / distance;
let r6_inv = r2_inv.powi(6);
let force_magnitude = 24.0 * epsilon * r6_inv * (2.0 * r6_inv - 1.0) / distance_sq;
// Calculate the force vector
let mut force = [0.0; 3];
for k in 0..3 {
force[k] = force_magnitude * diff[k];
}
force
}
/// Applies the Lennard-Jones forces to all unique particle pairs in the system using parallel iteration.
///
/// # Arguments
///
/// * `particles` - Mutable reference to the vector of particles.
/// * `epsilon` - Depth of the potential well.
/// * `sigma` - Finite distance at which the inter-particle potential is zero.
fn apply_forces_parallel(particles: &mut Vec<Particle>, epsilon: f64, sigma: f64) {
// Wrap particles in an `Arc<Mutex<>>` for thread-safe access
let particles = Arc::new(Mutex::new(particles));
let len = particles.lock().unwrap().len();
// Use parallel iteration to calculate forces
(0..len).into_par_iter().for_each(|i| {
let particles = Arc::clone(&particles);
for j in (i + 1)..len {
let mut particles_guard = particles.lock().unwrap();
let force = lennard_jones(&particles_guard[i], &particles_guard[j], epsilon, sigma);
for k in 0..3 {
particles_guard[i].force[k] += force[k];
particles_guard[j].force[k] -= force[k]; // Newton's Third Law
}
}
});
}
/// Integrates the equations of motion using the Verlet integration scheme
///
/// # Arguments
///
/// * `particles` - Mutable reference to the vector of particles.
/// * `dt` - Time step for integration.
fn verlet_integration(particles: &mut Vec<Particle>, dt: f64) {
for particle in particles.iter_mut() {
for k in 0..3 {
// Update position and velocity using the Verlet scheme
particle.position[k] += particle.velocity[k] * dt + 0.5 * particle.force[k] * dt * dt / particle.mass;
particle.velocity[k] += 0.5 * particle.force[k] * dt / particle.mass;
}
}
}
fn main() {
// Initialize particles to form a simple cubic lattice
let mut particles = vec![
Particle::new([0.0, 0.0, 0.0], 1.0),
Particle::new([1.0, 0.0, 0.0], 1.0),
Particle::new([0.0, 1.0, 0.0], 1.0),
Particle::new([1.0, 1.0, 0.0], 1.0),
Particle::new([0.0, 0.0, 1.0], 1.0),
Particle::new([1.0, 0.0, 1.0], 1.0),
Particle::new([0.0, 1.0, 1.0], 1.0),
Particle::new([1.0, 1.0, 1.0], 1.0),
];
let epsilon = 1.0; // Depth of the potential well
let sigma = 1.0; // Distance at which the potential is zero
let dt = 0.01; // Time step
// Simulation loop with parallel force application
for step in 0..1000 {
apply_forces_parallel(&mut particles, epsilon, sigma);
verlet_integration(&mut particles, dt);
// Optional: Output particle positions at specific intervals
if step % 100 == 0 {
println!("Step {}:", step);
for (i, particle) in particles.iter().enumerate() {
println!(
"Particle {}: Position = {:?}, Velocity = {:?}",
i + 1,
particle.position,
particle.velocity
);
}
println!();
}
}
// Further analysis of stress-strain relationships or deformation behavior can be performed here
}
Note: The above parallel implementation using rayon
presents a conceptual approach to parallelizing force calculations. In practice, updating the forces on both particles in parallel requires careful synchronization to prevent data races, which can be achieved using atomic operations or synchronization primitives like mutexes. Implementing such synchronization mechanisms would add complexity and is beyond the scope of this example.
Extending MD Simulations with Advanced Features
Rust’s modularity and safety features facilitate the extension of existing MD simulation frameworks with advanced capabilities. For example, one could incorporate more sophisticated force fields such as the Tersoff or Stillinger-Weber potentials to simulate covalent materials. Additionally, integrating thermostats like the Berendsen or Nosé-Hoover thermostat enables simulations at constant temperature, while adding barostats allows for constant pressure conditions, essential for studying phase transitions.
These extensions can be implemented as separate modules within the framework, allowing for easy integration and customization. Rust's powerful type system ensures that these modules interact seamlessly, maintaining the framework's reliability and performance.
Interfacing Rust-Based MD Simulations with Other Tools
Interfacing Rust-based MD simulations with other computational tools and environments is crucial for enhancing their functionality and integrating them into comprehensive research workflows. For instance, Rust can handle the computationally intensive core simulation tasks, while Python can be used for pre-processing simulation parameters, post-processing results, or controlling simulation workflows through scripting.
Rust's PyO3
crate allows for seamless integration with Python, enabling the exposure of Rust functions and structs to Python code. This interoperability allows researchers to leverage Rust's performance for simulation tasks while utilizing Python's flexibility for data analysis and visualization.
use pyo3::prelude::*;
/// Example function to perform a simple MD step, exposed to Python.
#[pyfunction]
fn md_step(particles: &mut Vec<Particle>, epsilon: f64, sigma: f64, dt: f64) -> PyResult<()> {
apply_forces(particles, epsilon, sigma);
verlet_integration(particles, dt);
Ok(())
}
/// Module definition
#[pymodule]
fn rust_md(py: Python, m: &PyModule) -> PyResult<()> {
m.add_function(wrap_pyfunction!(md_step, m)?)?;
Ok(())
}
In this example, the md_step
function, which performs a single MD integration step, is exposed to Python using PyO3
. This allows Python scripts to control the simulation, perform pre-processing and post-processing tasks, and interact with Rust's high-performance simulation engine seamlessly.
Molecular Dynamics simulations are powerful tools that enable scientists to explore and understand the behavior of materials, biological molecules, and chemical systems at an atomic or molecular level. Through case studies in materials science, biophysics, and chemistry, it is evident that MD simulations provide invaluable insights that complement experimental approaches. Rust emerges as an excellent language for developing custom MD frameworks due to its emphasis on safety, performance, and concurrency. By leveraging Rust's robust features and its interoperability with other languages and tools, researchers can build efficient, reliable, and extensible simulation frameworks. These frameworks not only facilitate the simulation of complex systems but also integrate seamlessly into broader research workflows, enhancing the capacity to solve real-world scientific problems with precision and efficiency.
17.9. Challenges and Future Directions
Molecular Dynamics (MD) simulations have made remarkable strides over the past few decades, becoming indispensable tools across a multitude of scientific disciplines, including materials science, biophysics, and chemistry. These simulations empower researchers to probe systems at the atomic or molecular level, unveiling insights that are often unattainable through experimental methods alone. By modeling the intricate interactions between atoms and molecules, MD facilitates the prediction of material properties, the elucidation of biological processes, and the design of novel molecules or materials with tailored characteristics.
Despite their widespread utility, MD simulations encounter several persistent challenges that impede their scalability, accuracy, and computational efficiency. Scalability remains a significant concern as MD simulations typically involve a vast number of particles interacting through complex potentials. The computational cost of these simulations often scales with the square of the number of particles (O(N2)O(N^2)) due to the necessity of calculating pairwise interactions, rendering large-scale simulations computationally prohibitive. Addressing this scalability issue is crucial for enabling simulations of larger systems or longer timescales, which are essential for capturing emergent phenomena in complex materials or biological systems.
Accuracy is another critical challenge in MD simulations. While classical MD provides detailed insights into molecular behavior, its precision is inherently limited by the quality of the force fields employed. Traditional force fields are often derived from empirical data and incorporate various approximations, which may fail to accurately capture intricate interactions or quantum mechanical effects. This limitation can lead to discrepancies between simulation results and experimental observations, particularly in systems where electronic structure plays a pivotal role, such as in chemical reactions or electronic materials.
The computational cost associated with MD simulations continues to pose a formidable barrier. High-performance computing resources are frequently required to perform extensive simulations, and even then, simulations can span weeks or months to achieve meaningful results. This challenge is compounded by the necessity of using fine time steps to accurately model fast dynamics, such as bond vibrations, which exponentially increases the number of calculations needed and, consequently, the overall computational burden.
In recent years, machine learning (ML) techniques have been increasingly integrated into MD simulations to enhance both their accuracy and efficiency. A promising avenue is the development of neural network-based force fields. Unlike traditional force fields that rely on fixed functional forms and parameters, ML models can learn complex, non-linear relationships from extensive datasets, potentially offering more accurate and generalizable representations of interatomic interactions. For instance, ML models trained on quantum mechanical calculations can predict potential energy surfaces with high fidelity, enabling the simulation of systems where quantum effects are significant without the prohibitive computational cost of full quantum simulations.
Another compelling application of ML in MD is accelerated sampling. ML algorithms can analyze previous simulation data to identify and focus computational resources on the most critical regions of the conformational space, thereby reducing the number of required time steps and expediting the simulation process. This is particularly advantageous for studying rare events, such as protein folding or chemical reactions, which may occur infrequently and thus demand extensive simulation time to capture accurately.
The integration of quantum mechanics with classical MD, known as hybrid quantum-classical methods, represents a burgeoning trend aimed at bridging the gap between the accuracy of quantum mechanical calculations and the efficiency of classical MD simulations. In these hybrid approaches, the most chemically or physically significant parts of the system, such as reactive sites undergoing bond formation or breaking, are treated using quantum mechanics, while the remainder of the system is modeled using classical MD. This strategy allows for the accurate modeling of processes involving electron transfer or bond dynamics without imposing the computationally intensive requirements of quantum mechanics on the entire system.
To address scalability challenges, several strategies are employed. Parallel computing stands out as one of the most effective methods for scaling MD simulations. By distributing the computational workload across multiple processors or cores, simulations can handle larger systems or extend simulation timescales without a corresponding linear increase in computational cost. Domain decomposition, a common parallelization technique, involves partitioning the simulation space into subdomains, each managed by a different processor. This approach not only reduces the computational burden on individual processors but also facilitates scalability with the number of available processors.
GPU acceleration represents another powerful strategy for enhancing scalability. Graphics Processing Units (GPUs) are adept at performing numerous calculations in parallel, making them well-suited for the repetitive arithmetic operations inherent in MD simulations. By offloading computationally intensive tasks to GPUs, significant speedups can be achieved, enabling the simulation of larger systems or the extension of simulation timescales without a proportional increase in computational resources.
Enhancing the accuracy of MD simulations remains an ongoing endeavor that necessitates the development of more sophisticated force fields and algorithms. Machine learning-based force fields offer a promising direction by providing more accurate representations of molecular interactions compared to traditional empirical force fields. Additionally, incorporating quantum effects directly into force fields or through hybrid methods can substantially improve the accuracy of simulations in systems where quantum mechanics plays a critical role, such as in materials science or biochemistry.
Advanced integration techniques also contribute to improved accuracy by better preserving the physical properties of the system, such as energy conservation, over extended simulation times. Methods like symplectic integrators or higher-order integration schemes can mitigate the accumulation of numerical errors, ensuring that the simulation remains faithful to the underlying physical laws governing the system.
Looking ahead, several trends are poised to shape the future landscape of MD simulations. The continued advancement of GPU technology is expected to drive increased utilization of GPUs in MD simulations, further enhancing performance and scalability. The integration of quantum simulations with classical MD through hybrid quantum-classical methods is likely to gain prominence, enabling more accurate simulations of complex systems without incurring prohibitive computational costs. Machine learning is anticipated to play an increasingly pivotal role in MD, from the development of more accurate force fields to the acceleration of simulations through intelligent sampling techniques.
Rust’s evolving ecosystem is exceptionally well-suited to address these challenges and drive future innovations in MD simulations. Its emphasis on safety, performance, and concurrency makes it an ideal language for developing robust and efficient simulation frameworks. Below, we explore how Rust can be harnessed to implement hybrid quantum-classical methods and integrate machine learning into MD simulations.
Implementing Hybrid Quantum-Classical MD Simulations in Rust
Implementing hybrid quantum-classical MD simulations in Rust involves combining Rust's strengths in performance and safety with external quantum mechanics libraries. The Foreign Function Interface (FFI) in Rust allows seamless integration with libraries written in other languages, such as C or C++, enabling the utilization of existing quantum mechanics codes within Rust-based MD frameworks.
extern crate libc;
use libc::c_double;
/// Represents a particle in 3D space with position, velocity, force, and mass.
struct Particle {
position: [f64; 3],
velocity: [f64; 3],
force: [f64; 3],
mass: f64,
}
impl Particle {
/// Creates a new Particle with the given position and mass.
fn new(position: [f64; 3], mass: f64) -> Self {
Self {
position,
velocity: [0.0, 0.0, 0.0],
force: [0.0, 0.0, 0.0],
mass,
}
}
/// Resets the force acting on the particle to zero.
fn reset_force(&mut self) {
self.force = [0.0; 3];
}
}
/// External quantum mechanics function assumed to be provided by a C library.
/// This function calculates a quantum mechanical energy correction based on particle positions.
extern "C" {
fn quantum_energy(particle_positions: *const c_double, num_particles: usize) -> c_double;
}
/// Applies classical MD forces (e.g., Lennard-Jones) to all particles.
/// Placeholder for actual force calculation implementation.
fn apply_classical_forces(particles: &mut Vec<Particle>) {
// Implement classical force calculations here (e.g., Lennard-Jones potential)
}
/// Performs a hybrid MD simulation step combining classical and quantum forces.
fn hybrid_md_step(particles: &mut Vec<Particle>, dt: f64) {
// Apply classical forces to particles
apply_classical_forces(particles);
// Prepare particle positions for quantum energy calculation
let positions: Vec<c_double> = particles.iter().flat_map(|p| p.position.iter()).copied().collect();
// Calculate quantum energy correction using the external quantum mechanics library
let quantum_correction = unsafe { quantum_energy(positions.as_ptr(), particles.len()) };
// Update forces with quantum correction and perform Verlet integration
for particle in particles.iter_mut() {
for i in 0..3 {
// Apply quantum correction to the force
particle.force[i] += quantum_correction;
// Update velocity based on the average force
particle.velocity[i] += 0.5 * (particle.force[i] / particle.mass) * dt;
// Update position using Verlet integration
let new_position = 2.0 * particle.position[i]
- particle.velocity[i] * dt
+ (particle.force[i] / particle.mass) * dt * dt;
particle.position[i] = new_position;
}
}
}
fn main() {
// Initialize particles to form a simple cubic lattice
let mut particles = vec![
Particle::new([0.0, 0.0, 0.0], 1.0),
Particle::new([1.0, 0.0, 0.0], 1.0),
Particle::new([0.0, 1.0, 0.0], 1.0),
Particle::new([1.0, 1.0, 0.0], 1.0),
Particle::new([0.0, 0.0, 1.0], 1.0),
Particle::new([1.0, 0.0, 1.0], 1.0),
Particle::new([0.0, 1.0, 1.0], 1.0),
Particle::new([1.0, 1.0, 1.0], 1.0),
];
let dt = 0.01; // Time step
// Simulation loop: perform hybrid MD steps
for step in 0..1000 {
hybrid_md_step(&mut particles, dt);
// Optional: Output particle positions and velocities at specific intervals
if step % 100 == 0 {
println!("Step {}:", step);
for (i, particle) in particles.iter().enumerate() {
println!(
"Particle {}: Position = {:?}, Velocity = {:?}",
i + 1,
particle.position,
particle.velocity
);
}
println!();
}
}
// Further analysis of stress-strain relationships or deformation behavior can be performed here
}
In this implementation, the Particle
struct encapsulates each particle's position, velocity, force, and mass. The quantum_energy
function is assumed to be part of an external quantum mechanics library written in C and is linked to Rust via FFI. The apply_classical_forces
function is a placeholder where classical MD force calculations (such as the Lennard-Jones potential) would be implemented. The hybrid_md_step
function combines classical force application with a quantum mechanical energy correction, subsequently updating the particle positions and velocities using the Verlet integration method. The main
function initializes a simple cubic lattice of particles and runs the simulation loop, periodically outputting the state of the system for observation.
Integrating Machine Learning into MD Simulations with Rust
Rust's robust type system and ownership model make it an ideal language for incorporating machine learning (ML) techniques into MD simulations. For example, one could implement a neural network-based force field trained on quantum mechanical data, enhancing the accuracy of force predictions beyond traditional empirical models.
/// Represents a simple neural network for predicting forces based on particle positions.
struct NeuralNetwork {
weights: Vec<f64>,
biases: Vec<f64>,
}
impl NeuralNetwork {
/// Creates a new NeuralNetwork with given weights and biases.
fn new(weights: Vec<f64>, biases: Vec<f64>) -> Self {
Self { weights, biases }
}
/// Predicts the force for a particle based on its position.
///
/// # Arguments
///
/// * `input` - A slice containing the particle's position coordinates.
///
/// # Returns
///
/// The predicted force magnitude.
fn predict(&self, input: &[f64]) -> f64 {
// Simple feedforward neural network with one hidden layer
let mut hidden = 0.0;
for (w, &x) in self.weights.iter().zip(input.iter()) {
hidden += w * x;
}
hidden += self.biases[0];
hidden = hidden.max(0.0); // ReLU activation
let mut output = 0.0;
output += self.weights[3] * hidden;
output += self.biases[1];
output
}
}
/// Applies machine learning-based forces to all particles.
fn apply_ml_forces(particles: &mut Vec<Particle>, nn: &NeuralNetwork) {
for particle in particles.iter_mut() {
let input = particle.position.to_vec();
let predicted_force = nn.predict(&input);
for i in 0..3 {
particle.force[i] += predicted_force;
}
}
}
fn main() {
// Initialize particles to form a simple cubic lattice
let mut particles = vec![
Particle::new([0.0, 0.0, 0.0], 1.0),
Particle::new([1.0, 0.0, 0.0], 1.0),
Particle::new([0.0, 1.0, 0.0], 1.0),
Particle::new([1.0, 1.0, 0.0], 1.0),
Particle::new([0.0, 0.0, 1.0], 1.0),
Particle::new([1.0, 0.0, 1.0], 1.0),
Particle::new([0.0, 1.0, 1.0], 1.0),
Particle::new([1.0, 1.0, 1.0], 1.0),
];
// Define a simple neural network with predefined weights and biases
let nn = NeuralNetwork {
weights: vec![0.5, -0.2, 0.3, 0.7], // Example weights
biases: vec![0.1, -0.1], // Example biases
};
let dt = 0.01; // Time step
// Simulation loop: apply ML-based forces and perform Verlet integration
for step in 0..1000 {
apply_ml_forces(&mut particles, &nn);
verlet_integration(&mut particles, dt);
// Optional: Output particle positions and velocities at specific intervals
if step % 100 == 0 {
println!("Step {}:", step);
for (i, particle) in particles.iter().enumerate() {
println!(
"Particle {}: Position = {:?}, Velocity = {:?}",
i + 1,
particle.position,
particle.velocity
);
}
println!();
}
}
// Further analysis or simulation steps can be performed here
}
In this example, the NeuralNetwork
struct represents a simple feedforward neural network with one hidden layer, consisting of predefined weights and biases. The predict
method calculates the force based on the particle's position using a ReLU activation function in the hidden layer. The apply_ml_forces
function iterates over all particles, using the neural network to predict and apply additional forces based on their positions. The main
function initializes a simple cubic lattice of particles, sets up the neural network with example weights and biases, and runs the simulation loop, periodically outputting the state of the system for observation.
This approach can be extended to more complex neural network architectures and integrated with training pipelines to develop force fields that accurately capture intricate molecular interactions derived from quantum mechanical data.
Overcoming Scalability Challenges with Parallel Computing and GPU Acceleration
Scalability remains a paramount challenge in MD simulations, especially as the number of particles and the complexity of interactions increase. To mitigate this, leveraging parallel computing and GPU acceleration can substantially enhance performance, enabling simulations of larger systems or longer timescales without a corresponding linear increase in computational resources.
use rayon::prelude::*;
use std::sync::{Arc, Mutex};
/// Represents a particle in the system
#[derive(Debug, Clone)]
struct Particle {
position: [f64; 3], // 3D position coordinates
velocity: [f64; 3], // 3D velocity components
force: [f64; 3], // 3D force components
mass: f64, // Mass of the particle
}
impl Particle {
/// Creates a new particle
fn new(position: [f64; 3], mass: f64) -> Self {
Self {
position,
velocity: [0.0; 3],
force: [0.0; 3],
mass,
}
}
/// Resets the force on the particle to zero
fn reset_force(&mut self) {
self.force = [0.0; 3];
}
}
/// Calculates the Lennard-Jones force between two particles
///
/// # Arguments
///
/// * `p1` - The first particle.
/// * `p2` - The second particle.
/// * `epsilon` - Depth of the potential well.
/// * `sigma` - Finite distance at which the inter-particle potential is zero.
///
/// # Returns
///
/// A 3D force vector applied on `p1` due to `p2`.
fn lennard_jones(p1: &Particle, p2: &Particle, epsilon: f64, sigma: f64) -> [f64; 3] {
let mut distance_sq = 0.0;
let mut diff = [0.0; 3];
// Calculate the squared distance and difference vector
for k in 0..3 {
diff[k] = p1.position[k] - p2.position[k];
distance_sq += diff[k] * diff[k];
}
if distance_sq == 0.0 {
return [0.0; 3]; // Avoid division by zero for identical particles
}
let distance = distance_sq.sqrt();
let r2_inv = sigma / distance;
let r6_inv = r2_inv.powi(6);
let force_magnitude = 24.0 * epsilon * r6_inv * (2.0 * r6_inv - 1.0) / distance_sq;
// Calculate the force vector
let mut force = [0.0; 3];
for k in 0..3 {
force[k] = force_magnitude * diff[k];
}
force
}
/// Applies the Lennard-Jones forces to all unique particle pairs in parallel using Rayon.
///
/// # Arguments
///
/// * `particles` - Mutable reference to the vector of Particles.
/// * `epsilon` - Depth of the potential well.
/// * `sigma` - Finite distance at which the inter-particle potential is zero.
fn apply_forces_parallel(particles: &mut Vec<Particle>, epsilon: f64, sigma: f64) {
// Wrap particles in an Arc and Mutex to allow safe concurrent access
let particles = Arc::new(Mutex::new(particles));
// Iterate over all unique pairs in parallel
(0..particles.lock().unwrap().len()).into_par_iter().for_each(|i| {
for j in (i + 1)..particles.lock().unwrap().len() {
let force = lennard_jones(&particles.lock().unwrap()[i], &particles.lock().unwrap()[j], epsilon, sigma);
{
let mut particles = particles.lock().unwrap();
for k in 0..3 {
particles[i].force[k] += force[k];
particles[j].force[k] -= force[k]; // Newton's third law
}
}
}
});
}
/// Integrates the equations of motion using the Verlet integration scheme
///
/// # Arguments
///
/// * `particles` - Mutable reference to the vector of particles.
/// * `dt` - Time step for integration.
fn verlet_integration(particles: &mut Vec<Particle>, dt: f64) {
for particle in particles.iter_mut() {
for k in 0..3 {
// Update position and velocity using the Verlet scheme
particle.position[k] += particle.velocity[k] * dt + 0.5 * particle.force[k] * dt * dt / particle.mass;
particle.velocity[k] += 0.5 * particle.force[k] * dt / particle.mass;
}
}
}
fn main() {
// Initialize particles to form a simple cubic lattice
let mut particles = vec![
Particle::new([0.0, 0.0, 0.0], 1.0),
Particle::new([1.0, 0.0, 0.0], 1.0),
Particle::new([0.0, 1.0, 0.0], 1.0),
Particle::new([1.0, 1.0, 0.0], 1.0),
Particle::new([0.0, 0.0, 1.0], 1.0),
Particle::new([1.0, 0.0, 1.0], 1.0),
Particle::new([0.0, 1.0, 1.0], 1.0),
Particle::new([1.0, 1.0, 1.0], 1.0),
];
let epsilon = 1.0; // Depth of the potential well
let sigma = 1.0; // Distance at which the potential is zero
let dt = 0.01; // Time step
// Simulation loop: apply forces in parallel and perform Verlet integration
for step in 0..1000 {
apply_forces_parallel(&mut particles, epsilon, sigma);
verlet_integration(&mut particles, dt);
// Optional: Output particle positions and velocities at specific intervals
if step % 100 == 0 {
println!("Step {}:", step);
for (i, particle) in particles.iter().enumerate() {
println!(
"Particle {}: Position = {:?}, Velocity = {:?}",
i + 1,
particle.position,
particle.velocity
);
}
println!();
}
}
// Further analysis of stress-strain relationships or deformation behavior can be performed here
}
In this enhanced implementation, the apply_forces_parallel
function leverages Rust's rayon
crate to parallelize the computation of forces across multiple threads, significantly reducing the computational time required for large systems. To safely share and mutate the particles
vector across threads, it is wrapped in an Arc
(Atomic Reference Counted) pointer and a Mutex
, ensuring thread-safe access and modifications. This parallelization approach allows the simulation to scale effectively with the number of available CPU cores, enabling the handling of larger systems or the extension of simulation timescales without a proportional increase in computational resources.
Harnessing GPU Acceleration for Enhanced Performance
Beyond CPU parallelization, GPU acceleration offers another avenue for dramatically improving the performance of MD simulations. GPUs are optimized for parallel processing, making them ideal for handling the repetitive arithmetic operations characteristic of MD force calculations.
// Note: GPU acceleration in Rust can be achieved using crates like `accel`, `rust-cuda`, or `wgpu`.
// The following example uses the `rust-cuda` crate to offload force calculations to the GPU.
use rust_cuda::prelude::*;
use std::error::Error;
/// Represents a particle in 3D space with position, velocity, force, and mass.
struct Particle {
position: [f64; 3],
velocity: [f64; 3],
force: [f64; 3],
mass: f64,
}
impl Particle {
/// Creates a new Particle with the given position and mass.
fn new(position: [f64; 3], mass: f64) -> Self {
Self {
position,
velocity: [0.0, 0.0, 0.0],
force: [0.0, 0.0, 0.0],
mass,
}
}
/// Resets the force acting on the particle to zero.
fn reset_force(&mut self) {
self.force = [0.0; 3];
}
}
/// CUDA kernel for calculating Lennard-Jones forces.
/// Placeholder for actual CUDA implementation.
#[cuda_bind(src = "force_calculation.ptx")]
extern "C" {
fn calculate_forces_cuda(particle_positions: *const f64, num_particles: usize, epsilon: f64, sigma: f64, particle_forces: *mut f64);
}
/// Applies Lennard-Jones forces using GPU acceleration.
fn apply_forces_gpu(particles: &mut Vec<Particle>, epsilon: f64, sigma: f64) -> Result<(), Box<dyn Error>> {
// Reset all forces
for particle in particles.iter_mut() {
particle.reset_force();
}
let num_particles = particles.len();
let mut positions: Vec<f64> = Vec::with_capacity(num_particles * 3);
for particle in particles.iter() {
positions.extend_from_slice(&particle.position);
}
// Allocate memory for forces on the GPU
let mut forces_gpu = vec![0.0f64; num_particles * 3];
let positions_ptr = positions.as_ptr();
let forces_ptr = forces_gpu.as_mut_ptr();
// Launch CUDA kernel to calculate forces
unsafe {
calculate_forces_cuda(positions_ptr, num_particles, epsilon, sigma, forces_ptr);
}
// Retrieve forces from GPU and update particles
for i in 0..num_particles {
for k in 0..3 {
particles[i].force[k] += forces_gpu[i * 3 + k];
}
}
Ok(())
}
fn main() -> Result<(), Box<dyn Error>> {
// Initialize particles to form a simple cubic lattice
let mut particles = vec![
Particle::new([0.0, 0.0, 0.0], 1.0),
Particle::new([1.0, 0.0, 0.0], 1.0),
Particle::new([0.0, 1.0, 0.0], 1.0),
Particle::new([1.0, 1.0, 0.0], 1.0),
Particle::new([0.0, 0.0, 1.0], 1.0),
Particle::new([1.0, 0.0, 1.0], 1.0),
Particle::new([0.0, 1.0, 1.0], 1.0),
Particle::new([1.0, 1.0, 1.0], 1.0),
];
let epsilon = 1.0; // Depth of the potential well
let sigma = 1.0; // Distance at which the potential is zero
let dt = 0.01; // Time step
// Simulation loop: apply GPU-accelerated forces and perform Verlet integration
for step in 0..1000 {
apply_forces_gpu(&mut particles, epsilon, sigma)?;
verlet_integration(&mut particles, dt);
// Optional: Output particle positions and velocities at specific intervals
if step % 100 == 0 {
println!("Step {}:", step);
for (i, particle) in particles.iter().enumerate() {
println!(
"Particle {}: Position = {:?}, Velocity = {:?}",
i + 1,
particle.position,
particle.velocity
);
}
println!();
}
}
// Further analysis of stress-strain relationships or deformation behavior can be performed here
Ok(())
}
Note: Implementing GPU acceleration in Rust requires interfacing with CUDA or other GPU computing frameworks. The above example uses the rust-cuda
crate to offload force calculations to the GPU. The calculate_forces_cuda
function represents a CUDA kernel that performs the actual force computations. Implementing such kernels involves writing CUDA code, compiling it to PTX (Parallel Thread Execution) format, and ensuring proper memory management and synchronization between the CPU and GPU. This example serves as a conceptual demonstration; a complete implementation would require detailed CUDA programming and integration steps.
Extending MD Simulations with Advanced Features
Rust’s modularity and safety features facilitate the extension of existing MD simulation frameworks with advanced capabilities. For example, one could incorporate more sophisticated force fields such as the Tersoff or Stillinger-Weber potentials to simulate covalent materials. Additionally, integrating thermostats like the Berendsen or Nosé-Hoover thermostat enables simulations at constant temperature, while adding barostats allows for constant pressure conditions, essential for studying phase transitions.
These extensions can be implemented as separate modules within the framework, allowing for easy integration and customization. Rust's powerful type system ensures that these modules interact seamlessly, maintaining the framework's reliability and performance.
/// Trait defining the behavior of a thermostat.
trait Thermostat {
/// Applies the thermostat to the system to control temperature.
fn apply(&self, particles: &mut Vec<Particle>, target_temp: f64, dt: f64);
}
/// Berendsen Thermostat implementation.
struct BerendsenThermostat {
coupling_constant: f64,
}
impl BerendsenThermostat {
/// Creates a new BerendsenThermostat with the specified coupling constant.
fn new(coupling_constant: f64) -> Self {
Self { coupling_constant }
}
}
impl Thermostat for BerendsenThermostat {
fn apply(&self, particles: &mut Vec<Particle>, target_temp: f64, dt: f64) {
// Calculate current kinetic temperature
let mut kinetic_energy = 0.0;
for particle in particles.iter() {
let speed_sq: f64 = particle.velocity.iter().map(|v| v * v).sum();
kinetic_energy += 0.5 * particle.mass * speed_sq;
}
let current_temp = (2.0 * kinetic_energy) / (3.0 * particles.len() as f64);
// Calculate scaling factor
let lambda = (1.0 + (self.coupling_constant * dt) * (target_temp / current_temp).sqrt()) / (1.0 + self.coupling_constant * dt);
// Scale velocities
for particle in particles.iter_mut() {
for v in particle.velocity.iter_mut() {
*v *= lambda;
}
}
}
}
/// Trait defining the behavior of a barostat.
trait Barostat {
/// Applies the barostat to the system to control pressure.
fn apply(&self, particles: &mut Vec<Particle>, target_pressure: f64, dt: f64);
}
/// Example implementation of a simple barostat.
struct SimpleBarostat {
coupling_constant: f64,
}
impl SimpleBarostat {
/// Creates a new SimpleBarostat with the specified coupling constant.
fn new(coupling_constant: f64) -> Self {
Self { coupling_constant }
}
}
impl Barostat for SimpleBarostat {
fn apply(&self, particles: &mut Vec<Particle>, target_pressure: f64, dt: f64) {
// Placeholder for pressure calculation and scaling
// Implement pressure calculation based on virial theorem or other methods
// Scale positions or box dimensions accordingly
}
}
fn main() {
// Initialize particles to form a simple cubic lattice
let mut particles = vec![
Particle::new([0.0, 0.0, 0.0], 1.0),
Particle::new([1.0, 0.0, 0.0], 1.0),
Particle::new([0.0, 1.0, 0.0], 1.0),
Particle::new([1.0, 1.0, 0.0], 1.0),
Particle::new([0.0, 0.0, 1.0], 1.0),
Particle::new([1.0, 0.0, 1.0], 1.0),
Particle::new([0.0, 1.0, 1.0], 1.0),
Particle::new([1.0, 1.0, 1.0], 1.0),
];
let epsilon = 1.0; // Depth of the potential well
let sigma = 1.0; // Distance at which the potential is zero
let dt = 0.01; // Time step
// Initialize thermostats and barostats
let thermostat = BerendsenThermostat::new(0.1);
let barostat = SimpleBarostat::new(0.1);
// Simulation loop: apply forces, thermostats, barostats, and perform integration
for step in 0..1000 {
apply_forces_parallel(&mut particles, epsilon, sigma);
verlet_integration(&mut particles, dt);
// Apply thermostat to control temperature
thermostat.apply(&mut particles, 300.0, dt); // Target temperature: 300 K
// Apply barostat to control pressure
barostat.apply(&mut particles, 1.0, dt); // Target pressure: 1 atm
// Optional: Output particle positions and velocities at specific intervals
if step % 100 == 0 {
println!("Step {}:", step);
for (i, particle) in particles.iter().enumerate() {
println!(
"Particle {}: Position = {:?}, Velocity = {:?}",
i + 1,
particle.position,
particle.velocity
);
}
println!();
}
}
// Further analysis of stress-strain relationships or deformation behavior can be performed here
}
In this extension, traits Thermostat
and Barostat
are defined to abstract the behavior of temperature and pressure control mechanisms, respectively. The BerendsenThermostat
struct implements the Thermostat
trait, providing functionality to control the system's temperature by scaling particle velocities based on the desired target temperature. Similarly, the SimpleBarostat
struct implements the Barostat
trait, serving as a placeholder for pressure control mechanisms. These abstractions facilitate the seamless integration of various thermostats and barostats into the MD simulation framework, enhancing its versatility and applicability to a broader range of scientific problems.
Interfacing Rust-Based MD Simulations with Python Using PyO3
Interfacing Rust-based MD simulations with other computational tools and environments is crucial for enhancing their functionality and integrating them into comprehensive research workflows. Python, with its rich ecosystem of scientific libraries, is a natural choice for tasks such as pre-processing simulation parameters, post-processing results, and controlling simulation workflows through scripting. Rust's PyO3
crate enables seamless interoperability between Rust and Python, allowing researchers to harness Rust's performance for simulation tasks while leveraging Python's flexibility for ancillary tasks.
use pyo3::prelude::*;
use pyo3::wrap_pyfunction;
/// Represents a particle in 3D space with position, velocity, force, and mass.
struct Particle {
position: [f64; 3],
velocity: [f64; 3],
force: [f64; 3],
mass: f64,
}
impl Particle {
/// Creates a new Particle with the given position and mass.
fn new(position: [f64; 3], mass: f64) -> Self {
Self {
position,
velocity: [0.0, 0.0, 0.0],
force: [0.0, 0.0, 0.0],
mass,
}
}
/// Resets the force acting on the particle to zero.
fn reset_force(&mut self) {
self.force = [0.0; 3];
}
}
/// Calculates Lennard-Jones forces between all unique particle pairs.
fn apply_classical_forces(particles: &mut Vec<Particle>, epsilon: f64, sigma: f64) {
// Reset all forces before recalculating
for particle in particles.iter_mut() {
particle.reset_force();
}
// Iterate over all unique pairs of particles
for i in 0..particles.len() {
for j in (i + 1)..particles.len() {
let force = lennard_jones(&particles[i], &particles[j], epsilon, sigma);
for k in 0..3 {
particles[i].force[k] += force[k];
particles[j].force[k] -= force[k]; // Newton's third law
}
}
}
}
/// Calculates the Lennard-Jones force between two particles.
fn lennard_jones(p1: &Particle, p2: &Particle, epsilon: f64, sigma: f64) -> [f64; 3] {
let mut r_vec = [0.0; 3];
let mut r2 = 0.0;
for i in 0..3 {
r_vec[i] = p1.position[i] - p2.position[i];
r2 += r_vec[i] * r_vec[i];
}
let r2_inv = 1.0 / r2;
let r6_inv = r2_inv * r2_inv * r2_inv;
let force_scalar = 24.0 * epsilon * r6_inv * (2.0 * r6_inv - 1.0) * r2_inv;
let mut force = [0.0; 3];
for i in 0..3 {
force[i] = force_scalar * r_vec[i];
}
force
}
/// Performs Verlet integration to update particle positions and velocities.
fn verlet_integration(particles: &mut Vec<Particle>, dt: f64) {
for particle in particles.iter_mut() {
for i in 0..3 {
// Update position based on current velocity and force
let new_position = 2.0 * particle.position[i]
- particle.velocity[i] * dt
+ (particle.force[i] / particle.mass) * dt * dt;
// Update velocity based on average force
particle.velocity[i] += 0.5 * (particle.force[i] / particle.mass) * dt;
// Assign the new position
particle.position[i] = new_position;
}
}
}
/// Exposed function to perform a single MD simulation step.
///
/// # Arguments
///
/// * `particles` - A mutable reference to a list of particles.
/// * `epsilon` - Depth of the potential well.
/// * `sigma` - Distance at which the potential is zero.
/// * `dt` - Time step for integration.
///
/// # Returns
///
/// An empty tuple indicating successful execution.
#[pyfunction]
fn md_step(particles: &mut Vec<Particle>, epsilon: f64, sigma: f64, dt: f64) -> PyResult<()> {
apply_classical_forces(particles, epsilon, sigma);
verlet_integration(particles, dt);
Ok(())
}
/// Exposed function to initialize particles from Python.
///
/// # Arguments
///
/// * `positions` - A list of particle positions.
/// * `mass` - Mass of each particle.
///
/// # Returns
///
/// A vector of initialized particles.
#[pyfunction]
fn initialize_particles(positions: Vec<(f64, f64, f64)>, mass: f64) -> PyResult<Vec<Particle>> {
let particles = positions
.into_iter()
.map(|(x, y, z)| Particle::new([x, y, z], mass))
.collect();
Ok(particles)
}
/// Python module definition.
#[pymodule]
fn rust_md(py: Python, m: &PyModule) -> PyResult<()> {
m.add_function(wrap_pyfunction!(md_step, m)?)?;
m.add_function(wrap_pyfunction!(initialize_particles, m)?)?;
Ok(())
}
In this example, Rust's PyO3
crate is utilized to expose Rust functions to Python seamlessly. The md_step
function performs a single MD simulation step by applying classical forces and performing Verlet integration, making it callable from Python scripts. The initialize_particles
function allows for the initialization of particles from Python by accepting a list of positions and a mass value, returning a vector of Particle
instances. This interoperability enables researchers to control and manage MD simulations using Python's flexible scripting capabilities while leveraging Rust's high performance for computationally intensive tasks.
To use these exposed functions in Python, one would compile the Rust code into a Python module and then interact with it as follows:
import rust_md
# Initialize particles with positions and mass
positions = [
(0.0, 0.0, 0.0),
(1.0, 0.0, 0.0),
(0.0, 1.0, 0.0),
(1.0, 1.0, 0.0),
(0.0, 0.0, 1.0),
(1.0, 0.0, 1.0),
(0.0, 1.0, 1.0),
(1.0, 1.0, 1.0),
]
mass = 1.0
particles = rust_md.initialize_particles(positions, mass)
epsilon = 1.0
sigma = 1.0
dt = 0.01
# Perform a single MD simulation step
rust_md.md_step(particles, epsilon, sigma, dt)
# Access updated particle positions and velocities
for i, particle in enumerate(particles, start=1):
print(f"Particle {i}: Position = {particle.position}, Velocity = {particle.velocity}")
This integration exemplifies how Rust can be combined with Python to create efficient and flexible MD simulation workflows, harnessing the strengths of both languages to advance scientific research.
Molecular Dynamics simulations are powerful tools that enable scientists to explore and understand the behavior of materials, biological molecules, and chemical systems at an atomic or molecular level. Through case studies in materials science, biophysics, and chemistry, it is evident that MD simulations provide invaluable insights that complement experimental approaches. Rust emerges as an excellent language for developing custom MD frameworks due to its emphasis on safety, performance, and concurrency. By leveraging Rust's robust features and its interoperability with other languages and tools, researchers can build efficient, reliable, and extensible simulation frameworks. These frameworks not only facilitate the simulation of complex systems but also integrate seamlessly into broader research workflows, enhancing the capacity to solve real-world scientific problems with precision and efficiency.
17.10. Conclusion
Chapter 17 highlights the synergy between computational physics and Rust, showcasing how Rust’s safety, concurrency, and performance features can be leveraged to create efficient and scalable Molecular Dynamics simulations. As we continue to push the boundaries of scientific discovery, Rust’s role in computational physics will only grow, offering new opportunities for innovation and precision in complex simulations.
17.10.1. Further Learning with GenAI
Each prompt focuses on different facets of MD simulations—from fundamental principles to advanced techniques—enabling learners to gain a thorough understanding of the topic. By engaging with these prompts, readers can expect to enhance their knowledge and problem-solving skills, which are crucial for mastering computational physics and leveraging Rust’s unique capabilities.
Describe the theoretical foundations of Molecular Dynamics simulations, focusing on the role of Newtonian mechanics and statistical thermodynamics. How do these theories interact to model atomic and molecular systems over time? Discuss the implications of these interactions on the accuracy and stability of simulations when implemented in Rust.
Elaborate on the mathematical formulation of Newton’s equations of motion in the context of Molecular Dynamics. How can these equations be discretized and solved numerically in Rust? Compare different numerical methods, such as Verlet, Leapfrog, and Beeman, and evaluate their impact on computational efficiency and accuracy.
Discuss the concept of potential energy surfaces in Molecular Dynamics simulations. How are these surfaces constructed, and what role do they play in predicting the behavior of molecular systems? Provide a detailed explanation of how these concepts can be implemented and optimized in Rust for large-scale simulations.
Analyze the different types of force fields used in Molecular Dynamics, such as Lennard-Jones, Coulombic, and bonded interactions. How are these force fields mathematically defined, and what challenges arise in their implementation? Discuss how Rust’s features, such as traits and generics, can be utilized to create flexible and reusable force field models.
Explore the importance of accurate and efficient force calculations in Molecular Dynamics simulations. What are the computational challenges associated with force calculation, especially in systems with a large number of particles? Detail the strategies for optimizing force calculations in Rust, including parallelization and memory management techniques.
Discuss the design and implementation of data structures, such as neighbor lists and cell lists, in Molecular Dynamics simulations. How do these structures contribute to the efficiency of simulations, and what are the specific challenges in implementing them in Rust? Provide examples of how Rust’s ownership and borrowing principles can be applied to manage memory effectively in these structures.
Explain the role of parallel computing in scaling Molecular Dynamics simulations to handle large systems. What are the key challenges in parallelizing MD algorithms, and how can these challenges be addressed using Rust’s concurrency primitives? Discuss the potential of Rust’s Rayon and MPI bindings for achieving efficient parallelism in MD simulations.
Evaluate the numerical integration methods commonly used in Molecular Dynamics simulations, focusing on their stability, accuracy, and computational complexity. How can these methods be implemented in Rust, and what are the trade-offs involved in choosing one method over another? Discuss the impact of time step selection on the overall simulation and the strategies for optimizing it in Rust.
Analyze the techniques used for post-processing and analyzing Molecular Dynamics simulation data. How can the results, such as radial distribution functions and time correlation functions, be computed and interpreted? Discuss the implementation of these analysis tools in Rust, including the integration of libraries for data visualization and statistical analysis.
Discuss the application of Molecular Dynamics simulations in solving real-world scientific problems, such as protein folding, drug design, and materials science. How can Rust be used to implement these simulations effectively? Provide a detailed case study where Rust’s features were leveraged to enhance the performance and reliability of an MD simulation.
Explore the concept of domain decomposition in the context of parallel Molecular Dynamics simulations. How does this technique improve the scalability of simulations, and what are the specific implementation challenges in Rust? Discuss the potential benefits and limitations of domain decomposition when applied to complex molecular systems.
Investigate the role of machine learning in enhancing Molecular Dynamics simulations. How can machine learning models be integrated with MD simulations to predict system behavior or optimize simulation parameters? Discuss the opportunities and challenges of implementing such integrations in Rust, focusing on interoperability with existing machine learning frameworks.
Analyze the current challenges in Molecular Dynamics simulations, such as the need for accurate long-range interactions, handling large systems, and ensuring reproducibility. How can Rust’s unique features be leveraged to address these challenges? Discuss the potential for Rust to contribute to the future of MD simulations, particularly in the context of emerging computational technologies.
Discuss the importance of validation and verification in Molecular Dynamics simulations. How can Rust be used to implement robust testing and validation frameworks to ensure the accuracy and reliability of simulations? Explore the best practices for developing and maintaining high-quality simulation code in Rust.
Examine the challenges associated with energy conservation in Molecular Dynamics simulations. How do numerical integration methods impact the conservation of energy, and what strategies can be implemented in Rust to mitigate energy drift over long simulation times? Discuss the role of symplectic integrators and their implementation in Rust.
Discuss the use of periodic boundary conditions in Molecular Dynamics simulations. How do these conditions affect the simulation of bulk systems, and what are the computational challenges in implementing them? Provide a detailed explanation of how Rust’s capabilities can be used to efficiently handle periodic boundary conditions in large-scale simulations.
Explore the potential for hybrid quantum-classical methods in Molecular Dynamics simulations. How can these methods be implemented in Rust, and what are the challenges of integrating quantum mechanical calculations with classical MD simulations? Discuss the opportunities for Rust to advance the field of hybrid simulations, particularly in high-performance computing environments.
Investigate the role of Rust in optimizing Molecular Dynamics simulations for modern hardware architectures, such as GPUs and multi-core processors. How can Rust’s concurrency and parallelism features be used to maximize performance on these platforms? Discuss the challenges of writing portable and efficient code in Rust for heterogeneous computing environments.
Discuss the use of thermostats and barostats in Molecular Dynamics simulations to control temperature and pressure. How do these algorithms impact the behavior of the simulated system, and what are the challenges of implementing them in Rust? Provide examples of how Rust’s precision and safety features can be leveraged to ensure accurate and stable simulations.
Analyze the potential of Rust for future developments in Molecular Dynamics simulations, particularly in the context of large-scale, multi-scale, and multi-physics simulations. How can Rust’s growing ecosystem and its emphasis on safety and performance contribute to the next generation of computational physics tools? Discuss the opportunities for Rust to become a leading language in scientific computing, particularly in the field of Molecular Dynamics.
Keep practicing, experimenting, and innovating—your dedication to learning will pave the way for groundbreaking discoveries. Each question you tackle brings you closer to mastering the complexities of Molecular Dynamics simulations and equips you with the skills to innovate in the field of computational science. The journey may be demanding, but the rewards are immense: the ability to contribute to groundbreaking research, solve real-world problems, and become a leader in a rapidly evolving field.
17.10.2. Assignments for Practice
These exercises are designed to give you hands-on experience in implementing and optimizing Molecular Dynamics simulations using Rust.
Exercise 17.1: Implementing and Analyzing Newton’s Equations of Motion
Exercise: Start by implementing Newton's equations of motion for a simple two-particle system using Rust. Define the initial positions, velocities, and forces acting on the particles. Use a basic integration method, such as the Verlet algorithm, to simulate the motion over time. Once implemented, analyze the system's energy conservation and stability.
Practice: Use GenAI to check your implementation and ask for suggestions on improving energy conservation or optimizing the integration method. Discuss the implications of your findings on larger, more complex systems.
Exercise 17.2: Designing and Implementing Force Fields
Exercise: Design a simple force field model, such as the Lennard-Jones potential, and implement it in Rust. Ensure that your implementation can calculate the potential energy and forces between particles efficiently. Test your force field with different particle configurations and parameters.
Practice: Use GenAI to validate your force field implementation and explore how changes in parameters affect the system's behavior. Ask for advice on extending your force field to more complex interactions, such as Coulombic forces, and compare the computational efficiency of different models.
Exercise 17.3: Building Efficient Data Structures for MD Simulations
Exercise: Develop a neighbor list or cell list data structure in Rust to optimize the performance of your Molecular Dynamics simulation. Test the data structure with a system containing a large number of particles and measure the improvement in computational efficiency.
Practice: Use GenAI to troubleshoot any performance bottlenecks and explore alternative data structures that might further improve efficiency. Discuss how your data structure could be adapted for use in parallel or distributed simulations.
Exercise 17.4: Parallelizing a Molecular Dynamics Simulation
Exercise: Take your existing Molecular Dynamics simulation and modify it to run in parallel using Rust’s Rayon library. Focus on parallelizing the force calculations and time integration steps. Test the parallelized version with a large particle system and compare the performance against the serial version.
Practice: Use GenAI to review your parallel implementation and identify potential issues with data race conditions or load balancing. Ask for recommendations on how to further optimize parallel performance or extend the simulation to run on distributed systems.
Exercise 17.5: Analyzing and Visualizing MD Simulation Data
Exercise: After running a Molecular Dynamics simulation, write a Rust program to analyze the trajectory data. Calculate properties such as the radial distribution function or velocity autocorrelation function. Visualize the results using a Rust-compatible plotting library.
Practice: Use GenAI to verify the accuracy of your analysis and to explore additional properties or functions that can be derived from the simulation data. Discuss how to improve the visualization or extend the analysis to more complex systems, and receive feedback on your implementation approach.
By working through these challenges and seeking feedback from GenAI, you’ll not only deepen your understanding of the concepts but also develop practical skills that are crucial for advancing in the field of computational physics. Keep experimenting, learning, and refining your techniques—each step you take brings you closer to mastering this powerful combination of Rust and Molecular Dynamics.
Comments