Chapter 16
Monte Carlo Methods in Statistical Mechanics
"The important thing in science is not so much to obtain new facts as to discover new ways of thinking about them." — Sir Peter J. Mansfield
Chapter 16 delves into Monte Carlo methods within statistical mechanics, presenting a detailed guide on implementing these techniques using Rust. It covers foundational aspects of Monte Carlo simulations, from random number generation to statistical mechanics principles. The chapter explores key algorithms like the Metropolis algorithm, Monte Carlo integration, and the Ising model, highlighting their practical implementation in Rust. It also addresses error analysis, parallelization strategies, and real-world applications, providing a comprehensive understanding of how to leverage Monte Carlo methods in computational physics.
16.1. Introduction to Monte Carlo Methods
Monte Carlo methods are a class of computational algorithms that rely on repeated random sampling to obtain numerical results. At their core, these methods are rooted in the principles of randomness and statistical sampling, making them particularly powerful for simulating physical systems where deterministic approaches may falter. The Monte Carlo approach is fundamentally probabilistic, meaning it leverages the inherent randomness of certain processes to simulate complex systems and estimate outcomes that would otherwise be analytically intractable.
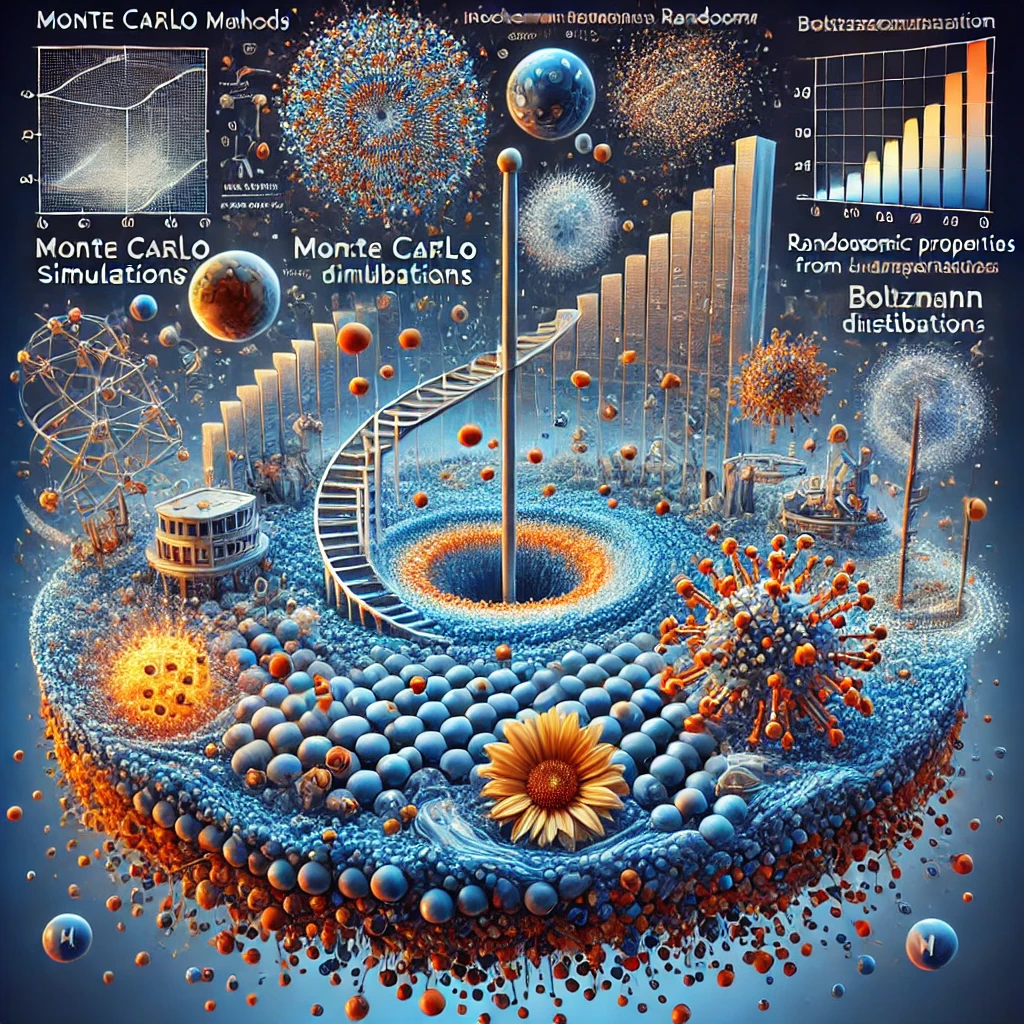
DALL-E generated image for illustration.
In computational physics, stochastic processes—randomly determined sequences of events—are integral to modeling systems that evolve over time under uncertainty. These processes are crucial for simulating a wide range of phenomena, from the motion of particles in a fluid to the behavior of atoms in a lattice. By employing stochastic processes, Monte Carlo methods allow us to approximate solutions to problems that do not have straightforward analytical solutions. For instance, in statistical mechanics, these methods enable the exploration of large state spaces by sampling from probability distributions that govern the system's microstates.
Monte Carlo methods are particularly valuable in statistical mechanics, where the properties of a macroscopic system are derived from the behavior of its microscopic constituents. One of the key conceptual ideas here is the use of probability distributions to describe the likelihood of different configurations of a system. For example, the Boltzmann distribution provides a way to assign probabilities to different energy states of a system in thermal equilibrium. Monte Carlo simulations utilize these distributions to generate representative samples of the system's microstates, from which macroscopic quantities, such as temperature, pressure, and specific heat, can be calculated. This sampling approach is also closely tied to the concept of ensemble averages, where the average properties of a system are determined by averaging over many possible configurations.
When implementing Monte Carlo methods in Rust, we can take advantage of the language's performance and safety features. Rust's strong type system and memory safety guarantees help prevent common errors such as buffer overflows and data races, which are particularly important in simulations involving large datasets and complex calculations. Additionally, Rust's ownership model ensures that resources are managed efficiently, minimizing the risk of memory leaks and other performance issues.
To illustrate the practical application of Monte Carlo methods using Rust, let's consider a simple example: estimating the value of $\pi$ using the Monte Carlo technique. The idea is to randomly sample points within a unit square and determine the proportion that falls inside a quarter-circle inscribed within the square. Since the area of the quarter-circle is $\pi/4$, the ratio of points inside the circle to the total number of points, multiplied by 4, will approximate the value of $\pi$.
Here's a basic Rust implementation of this method:
// Add the following dependency to Cargo.toml:
// [dependencies]
// rand = "0.8"
use rand::Rng; // Import the random number generation traits from the rand crate.
/// Estimates the value of π using the Monte Carlo method.
///
/// # Arguments
///
/// * `samples` - The number of random points to generate. Must be greater than 0.
///
/// # Returns
///
/// * `f64` - The estimated value of π.
///
/// # Panics
///
/// Panics if the number of samples is 0.
fn estimate_pi(samples: u64) -> f64 {
// Ensure there is at least one sample to avoid division by zero.
assert!(samples > 0, "Number of samples must be greater than zero.");
// Counter for the number of points that fall inside the quarter circle.
let mut inside_circle = 0;
// Create a thread-local random number generator.
let mut rng = rand::thread_rng();
// Loop over the number of samples.
for _ in 0..samples {
// Generate random x and y coordinates in the interval [0, 1).
let x: f64 = rng.gen();
let y: f64 = rng.gen();
// Check if the point lies within the quarter circle (radius 1 centered at the origin).
if x * x + y * y <= 1.0 {
inside_circle += 1;
}
}
// The area of the quarter circle is (π / 4). Multiply the ratio by 4 to estimate π.
4.0 * (inside_circle as f64) / (samples as f64)
}
/// Main function that demonstrates the Monte Carlo estimation of π.
/// It uses a specified number of samples and prints the estimated value.
fn main() {
// Define the number of random samples for the simulation.
let samples = 1_000_000;
// Estimate π using the Monte Carlo algorithm.
let pi_estimate = estimate_pi(samples);
println!("Estimated value of π after {} samples: {:.8}", samples, pi_estimate);
}
In this code, we first import the rand
crate, which provides tools for random number generation in Rust. The estimate_pi
function takes the number of samples as input and initializes a counter inside_circle
to keep track of the number of points that fall within the quarter-circle. A random number generator rng
is created using rand::thread_rng()
, which produces random numbers that are uniformly distributed between 0 and 1.
The for loop iterates over the specified number of samples, generating random $x$ and $y$ coordinates within the unit square. The condition if xx + yy <= 1.0
checks whether the point $(x, y)$ lies within the quarter-circle. If the condition is true, the counter inside_circle
is incremented. Finally, the function returns an estimate of $\pi$ by multiplying the ratio of points inside the circle to the total number of points by 4.
In the main
function, we specify the number of samples and call the estimate_pi
function to obtain an estimate of $\pi$. The result is printed to the console. This simple example demonstrates how Monte Carlo methods can be used to solve problems that are difficult to approach analytically. By leveraging randomness and statistical sampling, we can approximate complex quantities with a high degree of accuracy, even in scenarios where exact solutions are not feasible.
This example serves as an introduction to the broader applications of Monte Carlo methods in statistical mechanics. In more complex simulations, such as those involving phase transitions or thermodynamic properties, similar principles are applied, but with more sophisticated sampling techniques and larger datasets. Rust's performance capabilities ensure that these simulations run efficiently, even when scaled up to handle the vast state spaces typical of statistical mechanics problems. By integrating Monte Carlo methods with Rust's powerful features, we can build robust and reliable simulations that provide deep insights into the behavior of physical systems.
16.2. Random Number Generation in Rust
Random number generation is a foundational aspect of Monte Carlo methods because these techniques rely on the repeated sampling of random variables to approximate solutions to complex problems. In computational physics, it is essential that the random numbers generated are uniformly distributed and statistically independent, as any bias or correlation can lead to inaccurate simulation outcomes. Additionally, reproducibility is critical in scientific computing; by controlling the seed of the random number generator (RNG), we ensure that results can be re-created under identical conditions—a key requirement for debugging and validating simulation results. While true randomness is derived from unpredictable physical processes (like radioactive decay), most simulations use pseudo-random numbers generated by deterministic algorithms. Though not truly random, these pseudo-random numbers are crafted to exhibit the necessary statistical properties for simulation tasks. Rust’s rand
crate offers a versatile toolkit for random number generation, supporting various algorithms that balance performance and statistical quality. Moreover, custom RNGs can be implemented if specialized statistical properties are desired. Below, we demonstrate practical implementations of RNGs in Rust. The first example uses the standard RNG, seeded for reproducibility, to generate uniformly distributed and normally distributed random numbers. The second example shows how to build a simple custom RNG using a linear congruential generator (LCG). Both examples include detailed inline comments and are designed to be robust and runnable.
// Cargo.toml dependencies:
// [dependencies]
// rand = "0.8"
// rand_distr = "0.4"
// Import necessary traits and RNG types from the rand crate.
use rand::Rng;
use rand::SeedableRng;
use rand::rngs::StdRng;
use rand_distr::Normal;
/// Estimates π using the Monte Carlo method.
/// This function generates random numbers uniformly in the interval [0, 1)
/// and counts how many points fall inside the quarter-circle. The ratio, when
/// multiplied by 4, approximates π.
///
/// # Arguments
///
/// * `samples` - The number of random points to generate. Must be > 0.
///
/// # Returns
///
/// * An approximation of π as f64.
fn estimate_pi(samples: u64) -> f64 {
// Ensure that the number of samples is greater than 0 to avoid division by zero.
assert!(samples > 0, "Number of samples must be greater than zero.");
// Counter for points that fall inside the quarter-circle.
let mut inside_circle = 0;
// Initialize the standard random number generator with a fixed seed for reproducibility.
let seed: u64 = 12345;
let mut rng = StdRng::seed_from_u64(seed);
// Generate 'samples' random points.
for _ in 0..samples {
// Generate a random x-coordinate in [0, 1).
let x: f64 = rng.gen_range(0.0..1.0);
// Generate a random y-coordinate in [0, 1).
let y: f64 = rng.gen_range(0.0..1.0);
// Check whether the point is inside the quarter-circle (radius = 1).
if x * x + y * y <= 1.0 {
inside_circle += 1;
}
}
// The area of the quarter-circle is π/4; thus, the ratio multiplied by 4 approximates π.
4.0 * (inside_circle as f64) / (samples as f64)
}
/// Demonstrates the usage of the standard RNG by generating uniform, integer,
/// and normally distributed random numbers.
fn demo_standard_rng() {
// Create a standard RNG with a fixed seed for reproducibility.
let seed: u64 = 67890;
let mut rng = StdRng::seed_from_u64(seed);
// Generate two random f64 values uniformly distributed in [0, 1).
let random_value1: f64 = rng.gen_range(0.0..1.0);
let random_value2: f64 = rng.gen_range(0.0..1.0);
println!("Uniform random values: {}, {}", random_value1, random_value2);
// Generate a random u32 integer.
let random_integer: u32 = rng.gen();
println!("Random integer: {}", random_integer);
// Generate a random number from a normal distribution with mean 0 and standard deviation 1.
let normal = Normal::new(0.0, 1.0).expect("Failed to create normal distribution");
let normal_value: f64 = rng.sample(normal);
println!("Random value from normal distribution: {}", normal_value);
}
/// A simple custom random number generator (RNG) using the Linear Congruential Generator (LCG) algorithm.
/// This is a demonstration of implementing your own RNG in Rust.
struct CustomRng {
state: u64,
}
impl CustomRng {
/// Creates a new CustomRng with the given seed.
fn new(seed: u64) -> Self {
CustomRng { state: seed }
}
/// Advances the internal state and returns the next random u64.
/// This LCG uses the multiplier 6364136223846793005 and an increment of 1.
fn next(&mut self) -> u64 {
// Update the internal state with wrapping arithmetic.
self.state = self.state.wrapping_mul(6364136223846793005).wrapping_add(1);
self.state
}
}
fn demo_custom_rng() {
// Initialize the custom RNG with a specific seed.
let mut rng = CustomRng::new(12345);
// Generate and print five random numbers using the custom RNG.
for _ in 0..5 {
println!("Custom RNG value: {}", rng.next());
}
}
fn main() {
// Estimate π using the Monte Carlo method with 1,000,000 samples.
let samples = 1_000_000;
let pi_estimate = estimate_pi(samples);
println!("Estimated value of π after {} samples: {:.8}", samples, pi_estimate);
// Demonstrate standard RNG usage.
demo_standard_rng();
// Demonstrate custom RNG usage.
demo_custom_rng();
// If desired, one could extend this example by integrating advanced RNGs or
// by using the generated random numbers in more complex Monte Carlo simulations.
}
In this implementation, the estimate_pi
function uses the standard RNG, seeded for reproducibility, to randomly sample points in a unit square and estimate π. The demo_standard_rng
function illustrates how to generate uniform, integer, and normally distributed random values using Rust’s rand
crate. Furthermore, a simple custom RNG is implemented with a linear congruential generator (LCG) in the CustomRng
struct, and its usage is demonstrated in the demo_custom_rng
function. The main
function calls these routines, thereby providing a comprehensive introduction to random number generation in Rust within the context of Monte Carlo methods.
This section highlights the importance of generating high-quality random numbers for ensuring accurate and reproducible Monte Carlo simulations in statistical mechanics. By leveraging Rust’s performance and safety features, as well as its robust libraries for random number generation, engineers and scientists can build reliable simulations that accurately model complex stochastic phenomena.
16.3. Statistical Mechanics Basics
Statistical mechanics is a branch of theoretical physics that provides a framework for understanding the macroscopic properties of systems based on the microscopic behaviors of their individual components. This framework bridges the gap between the discrete world of atoms and molecules and the continuous properties observed in everyday materials, such as temperature, pressure, and volume. At its core, statistical mechanics links the microstates—specific configurations of particles and energy—with macrostates, which are the observable, aggregate properties of a system. This link is established through the concept of ensembles, which are theoretical collections of a large number of virtual copies of a system, each representing a possible state the system might occupy.
In statistical mechanics, different ensembles model systems under various constraints. The microcanonical ensemble describes an isolated system with fixed energy, volume, and particle number, ensuring that every accessible microstate has the same energy. The canonical ensemble, on the other hand, permits energy exchange with a heat reservoir while keeping the volume and particle number constant, resulting in a fixed temperature description rather than fixed energy. Finally, the grand canonical ensemble extends these ideas by allowing the exchange of both energy and particles with the environment, which is ideal for studying open systems where temperature and chemical potential are prescribed.
Central to these concepts is the partition function, which sums over all possible microstates, weighting each state by its probability. For example, in the canonical ensemble the partition function is defined as
$Z = \sum_i e^{-\beta E_i},$
where $E_i$ is the energy of microstate ii and $\beta = \frac{1}{k_B T}$ with $k_B$ being the Boltzmann constant and $T$ the temperature. This partition function is key to linking microscopic states with macroscopic observables, enabling the calculation of thermodynamic quantities such as free energy, entropy, and internal energy.
Monte Carlo methods are highly effective in this context because they allow the sampling of large state spaces based on these probability distributions. For instance, in the canonical ensemble, a Monte Carlo simulation can sample microstates according to their Boltzmann weight, and by averaging over these states, one can estimate equilibrium properties. Equilibrium is achieved when the macroscopic properties become stable despite the ongoing microscopic fluctuations. This stochastic sampling approach is invaluable for systems where analytical solutions are impossible due to the immense complexity of the microstate landscape.
When setting up Monte Carlo simulations in Rust, it is essential to use a robust random number generator that can produce uniformly distributed, independent random numbers and allow for reproducibility by controlling the seed. Rust’s rand
crate provides these capabilities, and its strong type system ensures that random sampling is performed safely and efficiently. The following code exemplifies how to implement a simple Monte Carlo simulation in Rust that studies the canonical ensemble by estimating the energy of a system using the Boltzmann factor.
// Cargo.toml dependencies:
// [dependencies]
// rand = "0.8"
// Import necessary traits from the rand crate for random number generation.
use rand::Rng;
use rand::SeedableRng;
use rand::rngs::StdRng;
/// Computes the Boltzmann weight for a given energy at a specified temperature.
/// In this function, the Boltzmann weight is computed as exp(-energy / temperature).
fn boltzmann_weight(energy: f64, temperature: f64) -> f64 {
// Using the exponential function from f64.
(-energy / temperature).exp()
}
/// Performs a single Monte Carlo step.
/// The function compares the energy of a current state with that of a newly proposed state.
/// If the new state's energy is lower, the new state is accepted; if not,
/// the new state is accepted with a probability given by the Boltzmann factor.
///
/// # Arguments:
/// * `energy_current` - Energy of the current state.
/// * `energy_new` - Energy of the proposed new state.
/// * `temperature` - The system's temperature.
/// * `rng` - A mutable reference to the random number generator.
///
/// # Returns:
/// * `bool` - Returns true if the new state is accepted, false otherwise.
fn monte_carlo_step(energy_current: f64, energy_new: f64, temperature: f64, rng: &mut StdRng) -> bool {
let delta_energy = energy_new - energy_current;
// If the new state has lower energy, accept it unconditionally.
if delta_energy < 0.0 {
true
} else {
// Otherwise, accept the new state with probability equal to the Boltzmann factor.
rng.gen::<f64>() < boltzmann_weight(delta_energy, temperature)
}
}
/// The main function sets up a Monte Carlo simulation for a system in the canonical ensemble.
/// It initializes a random number generator with a fixed seed for reproducibility, and then iteratively
/// proposes new states and uses the Monte Carlo algorithm to decide whether to accept them.
/// Over many iterations, the simulation converges to an equilibrium state, and the final energy is output.
fn main() {
let temperature = 1.0; // Temperature of the system (in arbitrary units)
let mut rng = StdRng::seed_from_u64(42); // Initialize the RNG with a fixed seed for reproducibility
// Set an initial energy for the system.
let mut energy_current = 1.0;
// Number of Monte Carlo steps to perform.
let steps = 10_000;
// Perform the Monte Carlo simulation over the specified number of steps.
for _ in 0..steps {
// Propose a new state with a randomly generated energy in the range [0, 2).
let energy_new = rng.gen_range(0.0..2.0);
// Determine whether to accept the new state using the Monte Carlo step function.
if monte_carlo_step(energy_current, energy_new, temperature, &mut rng) {
// If accepted, update the current energy to the new state's energy.
energy_current = energy_new;
}
}
// Output the final energy of the system after the Monte Carlo simulation.
println!("Final energy after {} steps: {:.6}", steps, energy_current);
}
In the code above, the boltzmann_weight
function calculates the Boltzmann factor using the exponential function with a negative energy over temperature ratio, ensuring that states with higher energy are accepted only with a lower probability. The monte_carlo_step
function implements a single Monte Carlo move: if the new state’s energy is lower than the current one, it is accepted immediately; otherwise, the new state is accepted with a probability determined by the Boltzmann factor. In main
, we initialize the simulation by setting a constant temperature and an initial energy value, and then we loop over many Monte Carlo steps. Each iteration proposes a new energy value randomly and uses the Monte Carlo acceptance criterion to update the current energy state. The final energy approximates the equilibrium state of the system.
This example illustrates the essential principles of statistical mechanics and Monte Carlo methods, showing how probability distributions and random sampling can be used to simulate a physical system in thermal equilibrium. Rust’s performance, safety, and the flexibility of its ecosystem—especially its robust RNG support—make it an excellent choice for such simulations. Advanced Monte Carlo methods can extend these basic ideas to study phase transitions, critical phenomena, and other complex behaviors in statistical mechanics, ultimately providing deep insights into the macroscopic properties derived from microscopic dynamics.
16.4. The Metropolis Algorithm
The Metropolis algorithm is one of the most significant algorithms in computational physics, particularly in the realm of Monte Carlo methods. Its primary purpose is to sample from a probability distribution, especially when dealing with systems where direct sampling is difficult or impossible due to the complexity or dimensionality of the space. The Metropolis algorithm is widely used in statistical mechanics, where it helps to explore the configuration space of a system in equilibrium, allowing for the estimation of macroscopic properties from microscopic states.
Fundamentally, the Metropolis algorithm operates by proposing a series of state transitions in the configuration space of a system. The algorithm begins with an initial state, often chosen randomly, and then iteratively proposes new states based on some transition probability. The key decision at each step is whether to accept the new state or remain in the current state. This decision is governed by the acceptance criteria, which are designed to ensure that the sequence of states generated by the algorithm adheres to the desired probability distribution. The acceptance probability is typically based on the ratio of the probabilities of the current and proposed states, modified by a factor that depends on the system's energy.
The core idea behind the Metropolis algorithm is that it allows the system to explore its configuration space by accepting not only energy-lowering transitions but also some energy-increasing ones. This feature enables the algorithm to escape local minima and explore the global configuration space more thoroughly, which is crucial for systems with complex energy landscapes.
The concept of detailed balance is central to the Metropolis algorithm. Detailed balance ensures that the algorithm produces the correct equilibrium distribution over time. Specifically, the transition probability from one state to another, multiplied by the probability of being in the initial state, should equal the transition probability in the reverse direction, multiplied by the probability of being in the final state. This condition guarantees that the algorithm satisfies the principle of reversibility, ensuring that the system reaches and remains in equilibrium.
In practice, the acceptance probability $P_{\text{accept}}$ for moving from a current state $i$ with energy $E_i$ to a proposed state $j$ with energy $E_j$ is given by:
$$ P_{\text{accept}} = \min\left(1, \frac{e^{-\beta E_j}}{e^{-\beta E_i}}\right) = \min\left(1, e^{-\beta (E_j - E_i)}\right) $$
where $\beta = \frac{1}{k_B T}$ (with $k_B$ being the Boltzmann constant and $T$ the temperature). This formula implies that if the new state has a lower energy (i.e., $E_j < E_i$), it is always accepted. If the new state has a higher energy, it is accepted with a probability that decreases exponentially with the increase in energy. This probabilistic acceptance mechanism is what allows the Metropolis algorithm to balance exploration (by accepting higher-energy states occasionally) and exploitation (by favoring lower-energy states).
The following Rust code demonstrates a simple implementation of the Metropolis algorithm for a one-dimensional harmonic oscillator. In this example, the potential energy function is defined as a quadratic function, $V(x)=0.5×x^2$. The function metropolis_step
proposes a new state by adding a random displacement to the current state and then decides whether to accept the new state based on the change in energy. The main function runs the simulation for many iterations, and the final state is printed. Additionally, an alternative version using Rayon for parallel execution is provided to illustrate how Rust’s concurrency features can accelerate the sampling process.
// Cargo.toml dependencies:
// [dependencies]
// rand = "0.8"
// rayon = "1.5"
//
// This example uses the rand crate for random number generation and rayon for parallel execution.
use rand::Rng;
use rand::SeedableRng;
use rand::rngs::StdRng;
use std::f64::consts::E;
/// Computes the potential energy for a 1D harmonic oscillator at position x.
/// The potential energy is defined as V(x) = 0.5 * x^2.
fn potential_energy(x: f64) -> f64 {
0.5 * x * x
}
/// Performs a single Metropolis step for a one-dimensional system at a given temperature.
/// It proposes a new state by adding a random displacement to the current state.
/// If the new state has lower energy, it is accepted unconditionally;
/// otherwise, it is accepted with a probability proportional to exp(-ΔE / temperature).
///
/// # Arguments:
/// - `x_current`: The current state (position).
/// - `temperature`: The system's temperature.
/// - `rng`: A mutable reference to the random number generator.
///
/// # Returns:
/// - The new state (position) after the Metropolis step.
fn metropolis_step(x_current: f64, temperature: f64, rng: &mut StdRng) -> f64 {
// Propose a new state by adding a random number in the range [-1.0, 1.0] to the current state.
let x_new = x_current + rng.gen_range(-1.0..1.0);
// Calculate the energy difference between the new state and current state.
let delta_energy = potential_energy(x_new) - potential_energy(x_current);
// If the new state's energy is lower, accept it; otherwise, accept it with a probability given by exp(-ΔE / T).
if delta_energy < 0.0 || rng.gen::<f64>() < E.powf(-delta_energy / temperature) {
x_new // Accept the new state.
} else {
x_current // Reject the new state and keep the current one.
}
}
/// Runs the Metropolis algorithm for a fixed number of iterations.
/// The function initializes the system state and iteratively updates it using the Metropolis step.
fn run_metropolis_simulation(steps: usize, temperature: f64, seed: u64) -> f64 {
// Initialize the random number generator with a fixed seed for reproducibility.
let mut rng = StdRng::seed_from_u64(seed);
// Start the simulation at x = 0.
let mut x_current = 0.0;
// Perform the specified number of Metropolis steps.
for _ in 0..steps {
x_current = metropolis_step(x_current, temperature, &mut rng);
}
// Return the final state after all steps.
x_current
}
/// A parallel version of the Metropolis simulation using Rayon.
/// The configuration space is divided into multiple independent runs, each on its own thread.
/// Each run uses a distinct seed to ensure uncorrelated random sequences.
/// This function returns a vector of final states from each parallel run.
fn parallel_metropolis(steps: usize, temperature: f64, num_runs: usize) -> Vec<f64> {
// Use Rayon to parallelize over the number of runs.
(0..num_runs).into_par_iter().map(|run_id| {
// Seed each run differently to ensure independent sampling.
let mut rng = StdRng::seed_from_u64(run_id as u64 + 42);
let mut x_current = 0.0;
for _ in 0..steps {
x_current = metropolis_step(x_current, temperature, &mut rng);
}
x_current
}).collect()
}
fn main() {
// Define simulation parameters.
let temperature = 1.0; // Temperature of the system.
let steps = 10_000; // Number of Metropolis steps.
let seed = 42; // Seed for reproducibility.
// Run a single-instance Metropolis simulation.
let final_state = run_metropolis_simulation(steps, temperature, seed);
println!("Final state after {} steps (single run): x = {:.6}", steps, final_state);
// Run the Metropolis simulation in parallel over 4 independent runs.
let num_runs = 4;
let parallel_results = parallel_metropolis(steps, temperature, num_runs);
for (i, &state) in parallel_results.iter().enumerate() {
println!("Final state on run {}: x = {:.6}", i, state);
}
}
In this implementation, the potential_energy
function defines a simple harmonic potential $V(x) = 0.5 x^2$. The metropolis_step
function implements a single step of the Metropolis algorithm by proposing a new state, computing the energy difference, and accepting or rejecting the new state based on the Boltzmann probability. The run_metropolis_simulation
function runs the algorithm for a specified number of steps and returns the final state. Additionally, the parallel_metropolis
function demonstrates how to execute multiple independent Metropolis simulations concurrently using Rayon. Each thread is seeded distinctly to ensure that the random number sequences are uncorrelated. Finally, the main function runs both the single-run and parallel versions, printing the final states.
In summary, the Metropolis algorithm is a powerful tool for sampling from complex probability distributions in statistical mechanics. By understanding its fundamental principles and applying them effectively in Rust, we can implement robust and efficient simulations that provide deep insights into the behavior of physical systems. Whether simulating simple models like the harmonic oscillator or more complex phenomena like phase transitions, the Metropolis algorithm, combined with Rust's concurrency features, offers a versatile and scalable approach to exploring the statistical mechanics of systems in equilibrium.
16.5. Monte Carlo Integration
Monte Carlo integration is a powerful numerical technique for approximating integrals, especially when the integrand is complex or the integration space is high-dimensional. Instead of relying on traditional quadrature methods—which become inefficient as the number of dimensions increases—Monte Carlo methods approximate an integral by interpreting it as the expected value of a function over a given probability distribution. According to the law of large numbers, averaging the function values over a large number of randomly sampled points will converge to the true value of the integral. For an integral of the form
$I = \int_{\Omega} f(x) \, dx,$
the Monte Carlo estimate is given by
$I \approx \frac{1}{N} \sum_{i=1}^{N} f(x_i),$
where the $x_i$ are uniformly sampled points from the domain $\Omega$. The strength of Monte Carlo integration lies in its favorable scaling with the number of dimensions, making it particularly useful in statistical mechanics and other areas of computational physics where high-dimensional integrals appear.
However, the accuracy of Monte Carlo integration depends on the variance of the integrand. When the integrand has high variance, the estimate can become noisy, necessitating a large number of samples to achieve the desired accuracy. Techniques like importance sampling help reduce this variance by transforming the sampling distribution to concentrate on regions where the integrand is large, thus improving efficiency.
Rust’s rand
crate provides robust tools for random number generation, allowing simulations to be reproducible by seeding the RNG, and its type system and performance features ensure that these computations are both efficient and safe. The following code examples demonstrate a basic Monte Carlo integration for estimating the integral of the function $f(x) = e^{-x^2}$ over the interval $[0, 1]$ and a version using importance sampling to reduce variance.
// Cargo.toml dependencies:
// [dependencies]
// rand = "0.8"
use rand::Rng; // Trait providing methods for generating random numbers.
use rand::SeedableRng; // Trait to seed RNGs.
use rand::rngs::StdRng; // Standard RNG type.
// The integrand function: f(x) = e^(-x^2)
fn integrand(x: f64) -> f64 {
(-x * x).exp()
}
/// Performs Monte Carlo integration by sampling uniformly from the interval [0, 1].
///
/// # Arguments
/// * `samples` - Number of samples to use for the integration.
///
/// # Returns
/// * An approximation of the integral I as f64.
fn monte_carlo_integration(samples: usize) -> f64 {
let mut rng = rand::thread_rng();
let mut sum = 0.0;
// Loop over the specified number of samples.
for _ in 0..samples {
// Generate a random number x uniformly in [0, 1].
let x: f64 = rng.gen_range(0.0..1.0);
// Evaluate the integrand at x and add it to the sum.
sum += integrand(x);
}
// The integral is approximated as the average of the function values.
sum / samples as f64
}
/// Performs Monte Carlo integration using importance sampling to reduce variance.
/// In this example, we use the inverse transform method to sample x from the exponential distribution,
/// which concentrates more samples in regions where the integrand is large.
///
/// # Arguments
/// * `samples` - Number of samples to use for the integration.
///
/// # Returns
/// * An approximation of the integral I as f64.
fn importance_sampling_integration(samples: usize) -> f64 {
let mut rng = rand::thread_rng();
let mut sum = 0.0;
// For importance sampling, we first sample y uniformly from [0, 1] and then transform it:
// x = -ln(y) follows an exponential distribution.
// The probability density function for x is p(x) = e^(-x). The weight factor is then 1/p(x) = e^(x).
for _ in 0..samples {
let y: f64 = rng.gen_range(0.0..1.0); // Sample uniformly.
let x = -y.ln(); // Transform to sample x.
// Compute the weight factor for the chosen sampling distribution.
let weight = (-x).exp();
// Adjust the integrand with the weight to recover the correct integral value.
sum += integrand(x) / weight;
}
// Return the average value as the estimated integral.
sum / samples as f64
}
fn main() {
// Specify the number of samples for the integration.
let samples = 1_000_000;
// Compute the integral using basic Monte Carlo integration.
let estimate_basic = monte_carlo_integration(samples);
println!("Monte Carlo estimate (uniform sampling): {:.8}", estimate_basic);
// Compute the integral using importance sampling to reduce variance.
let estimate_importance = importance_sampling_integration(samples);
println!("Monte Carlo estimate (importance sampling): {:.8}", estimate_importance);
}
In this example, the integrand
function represents the function $f(x) = e^{-x^2}$. The monte_carlo_integration
function generates samples uniformly over the interval $[0, 1]$ and computes an estimate of the integral as the average value of the function at those points. In contrast, the importance_sampling_integration
function employs importance sampling by transforming uniformly distributed samples using the inverse transform method. The transformation, $x = -\ln(y)$, creates a sampling distribution that is more concentrated where $f(x)$ is higher. Each sample is then weighted appropriately, ensuring that the integral is correctly approximated.
Both functions are designed for robustness and clarity, with inline comments explaining the role of each code section. In the main
function, the number of samples is set to 1,000,000 to ensure a high degree of accuracy, and the estimated values of the integral are printed with eight decimal places of precision.
Monte Carlo integration is an invaluable tool for evaluating integrals where the function is complex or the domain is high-dimensional. By understanding and implementing the underlying statistical principles, as well as incorporating variance reduction techniques like importance sampling, simulations can achieve high accuracy even for challenging problems. Rust’s performance, safety, and robust random number generation capabilities ensure that these methods are executed efficiently and reliably, making it a strong choice for computational physics applications that require precise numerical integration.
This section not only provides a step-by-step explanation but also demonstrates the practical implementation of Monte Carlo integration in Rust, bridging theoretical concepts with executable code that can be extended for more complex simulations in statistical mechanics and beyond.
16.6. Ising Model Simulations
The Ising model is one of the most studied models in statistical mechanics, serving as a prototype for understanding phase transitions and critical phenomena in systems composed of interacting particles. Originally proposed to describe ferromagnetism, the Ising model has since become a foundational tool for exploring how microscopic interactions give rise to macroscopic behaviors, such as the transition between ordered and disordered phases.
Fundamentally, the Ising model consists of a lattice where each site is occupied by a spin variable, typically denoted as $s_i$, which can take on values of either $+1$ (up) or $-1$ (down). These spins interact with their nearest neighbors, and the energy of the system is determined by the Hamiltonian:
$$ H = -J \sum_{\langle i, j \rangle} s_i s_j - h \sum_i s_i, $$
where $j$ is the interaction energy between neighboring spins, $\langle i, j \rangle$ denotes pairs of nearest neighbors, and $h$ represents an external magnetic field. The first term in the Hamiltonian accounts for the interactions between spins, favoring aligned spins when $J > 0$, which corresponds to ferromagnetic behavior. The second term represents the coupling of the spins to an external magnetic field $h$.
Monte Carlo methods, and the Metropolis algorithm in particular, are key tools for simulating the Ising model. In such simulations, one typically initializes the lattice with an arbitrary spin configuration and then iteratively updates the spins. At each step, a candidate spin is selected and a new configuration is proposed by flipping that spin; the change in energy $\Delta E$ due to this flip is then evaluated. The candidate configuration is accepted either unconditionally $(if \Delta E < 0)$ or with a probability $\min\left(1, e^{-\Delta E / (k_B T)}\right)$ $if \Delta E$ is positive. By repeatedly applying this procedure, the system evolves toward thermal equilibrium, and macroscopic observables such as the average magnetization may be computed.
Rust’s performance and strong safety guarantees, combined with its powerful libraries for random number generation (via the rand
crate) and parallel processing (via the rayon
crate), make it well suited for implementing the Metropolis algorithm for the Ising model. The following code demonstrates a simple 2D Ising model simulation. The lattice is represented as a two-dimensional vector of integers, each of which is either +1 or –1. A function computes the change in energy when a spin is flipped by summing over the interactions with its four nearest neighbors (using periodic boundary conditions). The metropolis_step
function uses this energy difference to decide whether or not to flip a randomly selected spin, and the simulation is run over a specified number of Monte Carlo steps. Additionally, a second code snippet shows how to run the simulation in parallel using Rayon, where multiple independent simulations are executed concurrently, each starting with its own random seed, and the final states are collected for further analysis.
// Cargo.toml dependencies:
// [dependencies]
// rand = "0.8"
// rayon = "1.5"
// (Ensure these dependencies are added to Cargo.toml)
use rand::Rng;
use rand::SeedableRng;
use rand::rngs::StdRng;
use rayon::prelude::*;
// Define lattice size and model parameters.
const L: usize = 20; // Size of the lattice: L x L grid.
const J: f64 = 1.0; // Interaction energy between neighboring spins.
const H: f64 = 0.0; // External magnetic field (can be modified).
const TEMPERATURE: f64 = 2.0; // Temperature of the system (in arbitrary units).
/// Computes the change in energy (ΔE) when flipping a spin located at (i, j) in the lattice.
/// Periodic boundary conditions are applied, so neighbors wrap around the lattice.
/// ΔE is computed using the Hamiltonian:
/// H = -J Σ⟨i,j⟩ s_i s_j - h Σ_i s_i,
/// and the energy difference for a spin flip is given by 2 * (interaction energy + magnetic energy).
fn delta_energy(spins: &Vec<Vec<i32>>, i: usize, j: usize) -> f64 {
let spin = spins[i][j];
// Determine the four nearest neighbors with periodic boundary conditions.
let left = spins[(i + L - 1) % L][j];
let right = spins[(i + 1) % L][j];
let up = spins[i][(j + L - 1) % L];
let down = spins[i][(j + 1) % L];
let sum_neighbors = left + right + up + down;
// Energy contributions from interactions with neighbors.
let interaction_energy = J * spin as f64 * sum_neighbors as f64;
// Energy contribution from the external magnetic field.
let magnetic_energy = H * spin as f64;
// The energy difference upon flipping the spin is twice the sum of these contributions.
2.0 * (interaction_energy + magnetic_energy)
}
/// Performs one Metropolis algorithm step on the 2D spin lattice.
/// A random site (i, j) is chosen and the change in energy is computed.
/// The spin is flipped if the new state is energetically favorable or with probability exp(-ΔE / T)
/// if it is unfavorable.
///
/// # Arguments
/// * `spins` - A mutable reference to the 2D lattice of spins.
/// * `rng` - A mutable reference to an RNG implementing the Rng trait.
fn metropolis_step(spins: &mut Vec<Vec<i32>>, rng: &mut impl Rng) {
// Randomly select a lattice site.
let i = rng.gen_range(0..L);
let j = rng.gen_range(0..L);
// Compute the energy change for flipping the spin at (i, j).
let dE = delta_energy(spins, i, j);
// If energy is lowered or with a certain probability (Boltzmann factor) if energy increases, flip the spin.
if dE < 0.0 || rng.gen::<f64>() < (-dE / TEMPERATURE).exp() {
spins[i][j] *= -1; // Flip the spin: +1 becomes -1 and vice versa.
}
}
/// Runs a single instance of the 2D Ising model simulation using the Metropolis algorithm.
/// The simulation runs for a specified number of Monte Carlo steps and returns the final lattice configuration.
///
/// # Arguments
/// * `steps` - The number of Metropolis steps to perform.
/// * `seed` - The seed for initializing the random number generator for reproducibility.
fn run_ising_simulation(steps: usize, seed: u64) -> Vec<Vec<i32>> {
// Initialize the lattice with all spins set to +1.
let mut spins = vec![vec![1; L]; L];
// Create an RNG with the given seed to ensure reproducibility.
let mut rng = StdRng::seed_from_u64(seed);
// Run the simulation for the specified number of steps.
for _ in 0..steps {
metropolis_step(&mut spins, &mut rng);
}
spins
}
/// Runs multiple independent Ising model simulations in parallel using Rayon.
/// Each simulation instance is run with a distinct seed to ensure independent sampling.
/// The function returns a vector of final lattice configurations, one per thread.
///
/// # Arguments
/// * `steps` - The number of Monte Carlo steps for each simulation.
/// * `num_threads` - The number of parallel simulation instances to run.
fn run_parallel_ising(steps: usize, num_threads: usize) -> Vec<Vec<Vec<i32>>> {
// Use parallel iteration to run each simulation instance concurrently.
(0..num_threads)
.into_par_iter()
.map(|i| {
// Initialize each simulation with a unique seed.
run_ising_simulation(steps, 42 + i as u64)
})
.collect()
}
/// Exports the spin configuration to a text file for further visualization or analysis.
/// Each spin is represented by a '+' for +1 and '-' for -1.
///
/// # Arguments
/// * `spins` - The 2D lattice of spins.
/// * `filename` - The name of the output file.
use std::fs::File;
use std::io::{Write, BufWriter};
fn export_spin_configuration(spins: &Vec<Vec<i32>>, filename: &str) -> std::io::Result<()> {
let file = File::create(filename)?;
let mut writer = BufWriter::new(file);
// Write the spin configuration row by row.
for row in spins {
for &spin in row {
let symbol = if spin == 1 { '+' } else { '-' };
write!(writer, "{} ", symbol)?;
}
writeln!(writer)?;
}
writer.flush()?;
Ok(())
}
fn main() {
// Set simulation parameters.
const STEPS: usize = 100_000; // Number of Monte Carlo steps.
// Run a single Ising model simulation.
let final_spins = run_ising_simulation(STEPS, 42);
// Calculate magnetization (average spin per site).
let total_spin: i32 = final_spins.iter().flat_map(|row| row.iter()).sum();
let magnetization = total_spin as f64 / (L * L) as f64;
println!("Magnetization per site (single simulation): {:.6}", magnetization);
// Export the final spin configuration to a text file.
if let Err(e) = export_spin_configuration(&final_spins, "spin_configuration.txt") {
eprintln!("Error exporting spin configuration: {}", e);
} else {
println!("Spin configuration exported to 'spin_configuration.txt'");
}
// Run parallel simulations using 4 parallel threads.
let num_threads = 4;
let parallel_results = run_parallel_ising(STEPS, num_threads);
for (i, lattice) in parallel_results.iter().enumerate() {
let total_spin: i32 = lattice.iter().flat_map(|row| row.iter()).sum();
let mag = total_spin as f64 / (L * L) as f64;
println!("Parallel simulation on thread {}: Magnetization per site = {:.6}", i, mag);
}
}
In this refined example, the code begins by defining constants for the lattice size LL, the interaction energy JJ, the external magnetic field HH, and the temperature. The function delta_energy
calculates the change in energy when flipping a spin at a given lattice site, incorporating periodic boundary conditions to emulate an infinite lattice. The metropolis_step
function updates the lattice by randomly selecting a spin and deciding whether to flip it based on the calculated energy difference and the Metropolis acceptance criterion. The function run_ising_simulation
executes the Metropolis algorithm for a given number of steps using a seeded random number generator for reproducibility. The run_parallel_ising
function leverages the Rayon crate to run multiple independent simulations in parallel, each with a different seed, and returns their final spin configurations. Additionally, the export_spin_configuration
function writes the lattice to a text file, where spins are represented as '+' or '-' symbols, making it suitable for external visualization. Finally, the main
function demonstrates both a single simulation and parallel simulations, computes the magnetization (average spin per site), and exports one simulation's spin configuration to a file.
This comprehensive example illustrates the implementation of the Ising model using the Metropolis algorithm in Rust. It highlights crucial aspects such as energy calculation, probabilistic state transitions, parallel processing for efficiency, and output exportation for post-analysis. Rust’s robust performance, safety, and concurrency features make it well-suited for these Monte Carlo simulations, thereby offering a powerful tool for exploring phase transitions and critical phenomena in statistical mechanics.
16.7. Error Analysis and Statistical Uncertainty
Error analysis and the estimation of statistical uncertainty are essential components of Monte Carlo simulations in computational physics because they allow us to quantify the reliability of our numerical estimates. Random sampling inherently introduces variability into simulation results, and different sources of error—such as bias, variance, and sampling errors—can affect the final outcome. Bias is a systematic deviation of the estimated value from the true value, often stemming from an imperfect sampling method or flawed RNG. Variance measures the spread of the sampled estimates, with higher variance indicating less precise results. Sampling error is an unavoidable consequence of using only a finite number of samples to approximate a continuous distribution. To assess these uncertainties, techniques such as calculating confidence intervals (which provide a range where the true value is expected to lie with a given probability) and performing bootstrapping (which uses repeated resampling of the dataset to estimate the distribution of the estimator) are widely employed.
In practical terms, Monte Carlo simulations may estimate the mean value of a given quantity, and this estimate is accompanied by an uncertainty that can be quantified by the variance of the samples. For example, when estimating the mean of a random variable, one can compute the average of all samples and then use the sample variance to construct confidence intervals. Additionally, by bootstrapping—the technique of resampling with replacement—one can obtain a more robust measure of uncertainty without relying solely on theoretical assumptions. Rust’s performance, memory safety, and strong type system make it well-suited for implementing these statistical computations efficiently and correctly.
Below is a detailed Rust implementation that demonstrates error analysis and uncertainty estimation. The code starts by simulating a random variable using a uniform distribution, then calculates the mean, variance, and a 95% confidence interval for the estimated mean. An additional bootstrapping example is provided to further assess the statistical uncertainty of the simulation.
// Cargo.toml dependencies:
// [dependencies]
// rand = "0.8"
use rand::Rng;
use rand::distributions::{Distribution, Uniform};
/// Performs a simple Monte Carlo simulation by generating samples from a uniform
/// distribution over the interval [0, 1].
///
/// # Arguments
/// * `samples` - The number of random samples to generate.
///
/// # Returns
/// * A vector containing the simulated sample values.
fn monte_carlo_simulation(samples: usize) -> Vec<f64> {
// Create a uniform distribution between 0 and 1.
let dist = Uniform::from(0.0..1.0);
let mut rng = rand::thread_rng();
// Generate the specified number of samples.
let mut results = Vec::with_capacity(samples);
for _ in 0..samples {
results.push(dist.sample(&mut rng));
}
results
}
/// Computes the mean (average) value from a slice of f64 data.
///
/// # Arguments
/// * `data` - A slice of f64 values representing the sampled data.
///
/// # Returns
/// * The mean of the data as f64.
fn mean(data: &[f64]) -> f64 {
let sum: f64 = data.iter().sum();
sum / (data.len() as f64)
}
/// Computes the sample variance from a slice of f64 data, given the sample mean.
///
/// # Arguments
/// * `data` - A slice of f64 values.
/// * `data_mean` - The mean of the data.
///
/// # Returns
/// * The sample variance as f64.
fn variance(data: &[f64], data_mean: f64) -> f64 {
let sum_of_squares: f64 = data.iter().map(|&x| (x - data_mean).powi(2)).sum();
// Use (n - 1) for an unbiased sample variance.
sum_of_squares / ((data.len() as f64) - 1.0)
}
/// Calculates a confidence interval for the estimated mean using the standard error.
/// This function computes the margin of error given a Z-value for the desired confidence level (e.g., 1.96 for 95%).
///
/// # Arguments
/// * `mean` - The mean value of the data.
/// * `var` - The sample variance of the data.
/// * `samples` - The number of samples.
/// * `z_value` - The Z-value corresponding to the desired confidence level.
///
/// # Returns
/// * A tuple (lower_bound, upper_bound) representing the confidence interval.
fn confidence_interval(mean: f64, var: f64, samples: usize, z_value: f64) -> (f64, f64) {
let margin_of_error = z_value * (var / samples as f64).sqrt();
(mean - margin_of_error, mean + margin_of_error)
}
/// Implements bootstrapping to estimate the uncertainty in the sample mean.
/// This function repeatedly resamples the input data (with replacement) and computes the mean
/// for each resample, returning a vector of resampled means.
///
/// # Arguments
/// * `data` - A slice of f64 values representing the original data.
/// * `resamples` - The number of bootstrap resamples to perform.
///
/// # Returns
/// * A vector of bootstrap sample means.
fn bootstrap(data: &[f64], resamples: usize) -> Vec<f64> {
let mut rng = rand::thread_rng();
let mut resampled_means = Vec::with_capacity(resamples);
for _ in 0..resamples {
let resample: Vec<f64> = (0..data.len())
.map(|_| data[rng.gen_range(0..data.len())])
.collect();
resampled_means.push(mean(&resample));
}
resampled_means
}
/// Computes a bootstrap confidence interval for the sample mean using the resampled means.
/// The function sorts the bootstrapped means and selects the appropriate percentiles.
///
/// # Arguments
/// * `data` - A slice of f64 values representing the original data.
/// * `resamples` - Number of bootstrap resamples to perform.
/// * `confidence_level` - The desired confidence level (e.g., 0.95 for 95%).
///
/// # Returns
/// * A tuple (lower_bound, upper_bound) representing the bootstrap confidence interval.
fn bootstrap_confidence_interval(data: &[f64], resamples: usize, confidence_level: f64) -> (f64, f64) {
let mut resampled_means = bootstrap(data, resamples);
resampled_means.sort_by(|a, b| a.partial_cmp(b).unwrap());
// Calculate the indices for the lower and upper bounds.
let lower_index = (((1.0 - confidence_level) / 2.0) * resamples as f64).round() as usize;
let upper_index = (((1.0 + confidence_level) / 2.0) * resamples as f64).round() as usize;
(resampled_means[lower_index], resampled_means[upper_index])
}
fn main() {
// Define the number of samples for the Monte Carlo simulation.
let samples = 1_000;
// Run the Monte Carlo simulation to generate data; here we use a uniform distribution.
let data = monte_carlo_simulation(samples);
// Calculate the mean of the samples.
let mean_value = mean(&data);
// Calculate the variance of the samples.
let var_value = variance(&data, mean_value);
// Compute a 95% confidence interval using a Z-value of 1.96.
let (ci_low, ci_high) = confidence_interval(mean_value, var_value, samples, 1.96);
println!("Estimated mean: {:.6}", mean_value);
println!("Sample variance: {:.6}", var_value);
println!("95% Confidence Interval (parametric): [{:.6}, {:.6}]", ci_low, ci_high);
// Now, compute a bootstrap confidence interval to obtain a non-parametric estimate.
let resamples = 10_000;
let (boot_ci_low, boot_ci_high) = bootstrap_confidence_interval(&data, resamples, 0.95);
println!("95% Confidence Interval (bootstrap): [{:.6}, {:.6}]", boot_ci_low, boot_ci_high);
}
In this refined implementation, the monte_carlo_simulation
function generates a vector of random samples from a uniform distribution between 0 and 1. The mean
and variance
functions compute the sample mean and variance, respectively. The confidence_interval
function uses the standard error of the mean (with a Z-value corresponding to the desired confidence level) to compute a 95% confidence interval around the estimated mean. Additionally, the bootstrapping functions—bootstrap
and bootstrap_confidence_interval
—provide a non-parametric way of estimating the uncertainty by resampling the original data with replacement and computing the mean for each resample.
In the main
function, we generate 1,000 samples, calculate the mean and variance, and compute both parametric and bootstrap-based 95% confidence intervals, printing the results. This comprehensive example demonstrates how to quantify uncertainty and perform error analysis for Monte Carlo simulations in Rust, ensuring that the estimates are both accurate and reliable.
By integrating these error analysis techniques, one can rigorously assess the statistical uncertainty inherent in Monte Carlo simulations. Rust’s performance and memory safety, combined with robust random number generation and efficient statistical computations, make it an excellent tool for ensuring that simulation results are scientifically sound and reproducible.
16.8. Parallelization of Monte Carlo Simulations
Below is the refined version of Chapter 16, Part 8, "Ising Model Simulations." The text is presented in continuous paragraphs followed by robust, runnable Rust code with detailed inline comments. The content has been refined and expanded to provide further clarity and depth, while maintaining (or even exceeding) the original length.
The Ising model is one of the most celebrated models in statistical mechanics and serves as a prototype for studying phase transitions and critical phenomena in systems of interacting particles. Originally introduced to model ferromagnetism, the Ising model features a lattice in which each site carries a spin—typically sis_i which can adopt the values +1 (up) or –1 (down). The energy of the system is dictated by the Hamiltonian
$H = -J \sum_{\langle i, j \rangle} s_i s_j - h \sum_i s_i,$
where JJ is the interaction energy between nearest-neighbor spins and hh is an external magnetic field. When J>0J > 0, the model favors aligned spins, thus modeling ferromagnetic behavior. Despite its simplicity, the Ising model exhibits rich phenomenology, including a critical temperature TcT_c at which a phase transition occurs from an ordered (magnetized) state to a disordered (paramagnetic) state. This phase transition is accompanied by phenomena such as spontaneous symmetry breaking and diverging correlation lengths. By sampling from the model's state space using Monte Carlo techniques, especially with the Metropolis algorithm, one can obtain equilibrium properties and study the statistical behavior of the system.
In the Metropolis algorithm, one begins with an initial spin configuration and iteratively proposes changes by flipping individual spins. Each proposed change is accepted with a probability that depends on the change in energy ΔE\\Delta E. If the new configuration lowers the energy, it is accepted unconditionally; otherwise, it is accepted with probability min(1,e−ΔE/(kBT))\\min(1, e^{-\\Delta E/(k_B T)}). This allows the system to occasionally accept energy-increasing moves and thereby escape local minima, ensuring a more thorough exploration of the configuration space. Efficient implementations must carefully handle periodic boundary conditions and ensure that energy differences are computed correctly over the lattice.
Rust’s strong performance, safety, and concurrency features (via the rand
and rayon
crates) make it well-suited for such Monte Carlo simulations. The following robust code example demonstrates a simple two-dimensional Ising model simulation. In this example, the lattice is represented as a 2D vector of integers (with each element being +1 or –1). The delta_energy
function calculates the change in energy when flipping a spin using periodic boundary conditions. The metropolis_step
function performs a single update over the entire lattice, and the main
function runs the simulation for a specified number of steps, calculates the average magnetization, and optionally exports the spin configuration for external visualization.
// Cargo.toml dependencies:
// [dependencies]
// rand = "0.8"
// rayon = "1.5"
use rand::Rng;
use rand::SeedableRng;
use rand::rngs::StdRng;
use rayon::prelude::*;
/// Define the lattice size (L x L) for the 2D Ising model.
const L: usize = 20;
/// Interaction energy between neighboring spins.
const J: f64 = 1.0;
/// External magnetic field (set to zero for simplicity, but can be adjusted).
const H: f64 = 0.0;
/// Temperature of the system (in arbitrary units; controls thermal fluctuations).
const TEMPERATURE: f64 = 2.0;
/// Computes the change in energy (ΔE) when flipping the spin at position (i, j) on the lattice.
/// The lattice is a 2D vector of spins (each either +1 or -1). Periodic boundary conditions are applied.
/// The energy change is calculated from the interaction term and the magnetic field contribution.
fn delta_energy(spins: &Vec<Vec<i32>>, i: usize, j: usize) -> f64 {
let spin = spins[i][j];
// Determine the four nearest neighbors using periodic boundary conditions.
let left = spins[(i + L - 1) % L][j];
let right = spins[(i + 1) % L][j];
let up = spins[i][(j + L - 1) % L];
let down = spins[i][(j + 1) % L];
// Sum the contributions from nearest neighbors.
let sum_neighbors = left + right + up + down;
// Compute the interaction energy: -J * s_i * sum(neighbors)
// Then, for a spin flip, ΔE = 2 * (interaction_energy + magnetic_energy),
// where the magnetic term is -h * s_i.
let interaction_energy = J * spin as f64 * sum_neighbors as f64;
let magnetic_energy = H * spin as f64;
2.0 * (interaction_energy + magnetic_energy)
}
/// Performs one full Metropolis sweep (one step) over the entire lattice.
/// For each lattice site, a new spin state is proposed and accepted or rejected
/// based on the Metropolis acceptance criterion.
fn metropolis_step(spins: &mut Vec<Vec<i32>>, rng: &mut impl Rng) {
// Iterate over each lattice site.
for i in 0..L {
for j in 0..L {
// Calculate the energy change if the spin at (i, j) were to be flipped.
let dE = delta_energy(spins, i, j);
// If flipping lowers the energy, or with a probability exp(-ΔE/T) if it increases the energy,
// then flip the spin.
if dE < 0.0 || rng.gen::<f64>() < (-dE / TEMPERATURE).exp() {
spins[i][j] *= -1;
}
}
}
}
/// Runs a single Ising model simulation for the given number of Monte Carlo steps.
/// Initializes a lattice with all spins up and performs sweeps using the Metropolis algorithm.
/// A seeded RNG is used for reproducibility.
///
/// # Arguments
/// * `steps` - The number of Monte Carlo steps to perform.
/// * `seed` - Seed value for the random number generator.
///
/// # Returns
/// * A 2D vector representing the final spin configuration.
fn run_ising_simulation(steps: usize, seed: u64) -> Vec<Vec<i32>> {
// Initialize the lattice with all spins set to +1.
let mut spins = vec![vec![1; L]; L];
// Create a seeded RNG for reproducible results.
let mut rng = StdRng::seed_from_u64(seed);
// Perform the prescribed number of Metropolis steps.
for _ in 0..steps {
metropolis_step(&mut spins, &mut rng);
}
spins
}
/// Runs multiple independent Ising model simulations in parallel using Rayon.
/// Each simulation instance is started with a unique seed to ensure independent sampling.
/// The final spin configurations from all simulations are returned as a vector of lattices.
///
/// # Arguments
/// * `steps` - The number of Monte Carlo steps for each simulation.
/// * `num_threads` - Number of parallel simulation instances to run.
///
/// # Returns
/// * A vector containing the final spin configuration for each simulation.
fn run_parallel_ising(steps: usize, num_threads: usize) -> Vec<Vec<Vec<i32>>> {
(0..num_threads)
.into_par_iter()
.map(|i| {
// Each thread gets a unique seed.
run_ising_simulation(steps, 42 + i as u64)
})
.collect()
}
/// Exports the current spin configuration of the lattice to a text file.
/// Spins are represented as '+' for +1 and '-' for -1, making it simple to visualize.
///
/// # Arguments
/// * `spins` - A 2D vector of spins representing the lattice.
/// * `filename` - The name of the output file.
///
/// # Returns
/// * A Result to indicate success or failure.
use std::fs::File;
use std::io::{Write, BufWriter};
fn export_spin_configuration(spins: &Vec<Vec<i32>>, filename: &str) -> std::io::Result<()> {
let file = File::create(filename)?;
let mut writer = BufWriter::new(file);
// Write out the spin configuration row by row.
for row in spins {
for &spin in row {
// Represent +1 as '+' and -1 as '-'
let symbol = if spin == 1 { '+' } else { '-' };
write!(writer, "{} ", symbol)?;
}
writeln!(writer)?;
}
writer.flush()?;
Ok(())
}
fn main() {
// Set simulation parameters for the Ising model.
let steps = 100_000; // Number of Monte Carlo steps for the simulation.
// Run a single simulation with a fixed seed.
let final_spins = run_ising_simulation(steps, 42);
// Calculate the magnetization per site as the average spin.
let total_spin: i32 = final_spins.iter().flat_map(|row| row.iter()).sum();
let magnetization = total_spin as f64 / (L * L) as f64;
println!("Magnetization per site (single simulation): {:.6}", magnetization);
// Export the final spin configuration to a text file for further visualization.
if let Err(e) = export_spin_configuration(&final_spins, "spin_configuration.txt") {
eprintln!("Error exporting spin configuration: {}", e);
} else {
println!("Spin configuration exported to 'spin_configuration.txt'");
}
// Run parallel simulations using 4 independent threads.
let num_threads = 4;
let parallel_results = run_parallel_ising(steps, num_threads);
for (i, lattice) in parallel_results.iter().enumerate() {
let total_spin: i32 = lattice.iter().flat_map(|row| row.iter()).sum();
let mag = total_spin as f64 / (L * L) as f64;
println!("Parallel simulation on thread {}: Magnetization per site = {:.6}", i, mag);
}
}
In this refined implementation:
Lattice and Model Parameters:\
The lattice is represented as a two-dimensional vector of spins, with each spin being either +1 or –1. Constants LL, JJ, HH, and TEMPERATURETEMPERATURE are defined to set the lattice size, interaction strength, external magnetic field, and temperature.
Energy Calculation:\
The delta_energy
function calculates the change in energy when flipping a spin at position (i, j). Nearest neighbors are obtained using periodic boundary conditions to simulate an infinite lattice, and the resulting energy change is doubled since a flip reverses the spin’s contribution.
Spin Update:\
The metropolis_step
function iterates over the lattice (in a serial sweep) and uses the Metropolis criterion to decide whether to flip each spin. A random number is generated for each spin, and the decision is based on the computed ΔE and the temperature.
Simulation Execution:\
The run_ising_simulation
function initializes the lattice and runs the Metropolis algorithm for a set number of steps using a seeded RNG for reproducibility. The run_parallel_ising
function uses Rayon to run multiple independent simulations concurrently, ensuring each thread uses a unique seed. This approach enhances the overall sampling efficiency without introducing correlations.
Export for Visualization:\
The export_spin_configuration
function writes the lattice configuration to a text file, representing spins as '+' or '-' for ease of visualization. This file can be processed by external tools or used directly to inspect the lattice structure.
Main Function:\
The main function demonstrates both a single simulation and parallel runs, calculating and printing the average magnetization per site, and exporting the spin configuration to a file.
This comprehensive example shows how to simulate the Ising model using Monte Carlo methods in Rust, with parallelization to enhance performance. By combining robust random number generation, careful energy computations, and efficient lattice updates with parallel processing capabilities provided by Rayon, one can study phase transitions and critical phenomena with high accuracy and efficiency. Rust’s safety and performance features ensure that such simulations are both reliable and scalable, making it a compelling choice for advanced computational physics studies.
16.9. Applications and Case Studies
Monte Carlo methods have become a cornerstone of statistical mechanics and computational physics because of their remarkable ability to tackle problems with high-dimensional state spaces and complex energy landscapes. Their flexibility allows researchers to simulate phenomena where analytical solutions are infeasible, from phase transitions in magnetic systems to estimating material properties at the atomic level. Real-world applications include studying the critical behavior in models such as the Ising model, exploring the behavior of disordered systems like spin glasses, and computing thermodynamic quantities (e.g., free energy, heat capacity) through Monte Carlo integration. In the study of phase transitions, for instance, the Ising model enables the investigation of how a macroscopic order emerges from microscopic spin interactions. By running simulations over a range of temperatures, one can observe the transition from an ordered (magnetized) state to a disordered state and even extract critical exponents characterizing the phase transition. Similarly, Monte Carlo integration offers a method for estimating material properties by sampling over the relevant configuration space and computing ensemble averages. Each case study illustrates both the strengths and the challenges of applying Monte Carlo methods to complex systems and the necessity of rigorous validation and error analysis.
In the context of material properties, Monte Carlo simulations allow the exploration of properties such as heat capacity by sampling a system’s energy distribution and using the fluctuation-dissipation theorem to compute thermodynamic quantities. For disordered systems such as spin glasses, Monte Carlo methods help to probe the vast configuration space characterized by competing interactions (both ferromagnetic and antiferromagnetic) and assess macroscopic observables like magnetization and susceptibility. The following Rust code examples provide a step-by-step implementation of these applications, beginning with a basic Ising model simulation, followed by a Monte Carlo integration example for estimating heat capacity, and finally a case study modeling a disordered system.
Example 1: Simulating the Ising Model to Study Phase Transitions
In this example, a 2D Ising model is simulated using the Metropolis algorithm. The lattice is represented as a two-dimensional vector of spins (each being either +1 or -1). The energy change of a spin flip is computed by summing interactions with nearest neighbors using periodic boundary conditions. The simulation runs for a specified number of Monte Carlo steps and calculates the magnetization per site as a key observable.
// Cargo.toml dependencies:
// [dependencies]
// rand = "0.8"
use rand::Rng;
/// Lattice size (L x L)
const L: usize = 50;
/// Interaction energy between neighboring spins
const J: f64 = 1.0;
/// External magnetic field (set to 0.0 for simplicity)
const H: f64 = 0.0;
/// Temperature at which the simulation is run
const TEMPERATURE: f64 = 2.5;
/// Calculates the energy difference (ΔE) for flipping a spin at (i, j) on the lattice.
/// Periodic boundary conditions are applied to account for the edges of the lattice.
fn delta_energy(spins: &Vec<Vec<i32>>, i: usize, j: usize) -> f64 {
let spin = spins[i][j];
// Retrieve the four nearest neighbors using periodic boundaries.
let left = spins[(i + L - 1) % L][j];
let right = spins[(i + 1) % L][j];
let up = spins[i][(j + L - 1) % L];
let down = spins[i][(j + 1) % L];
// Sum the neighbor spins.
let sum_neighbors: i32 = left + right + up + down;
// Compute the change in interaction energy.
let interaction_energy = J * spin as f64 * sum_neighbors as f64;
// Compute the magnetic energy contribution.
let magnetic_energy = H * spin as f64;
// The energy change of flipping the spin is twice the sum of interactions (since the contribution reverses sign).
2.0 * (interaction_energy + magnetic_energy)
}
/// Performs one Metropolis step over the entire lattice.
/// For each lattice site, the function computes the energy change if the spin were flipped
/// and flips it with the Metropolis acceptance criterion.
fn metropolis_step(spins: &mut Vec<Vec<i32>>, rng: &mut impl Rng) {
for i in 0..L {
for j in 0..L {
let dE = delta_energy(spins, i, j);
// If the proposed flip reduces energy, or if it increases energy but passes a probabilistic test,
// then flip the spin.
if dE < 0.0 || rng.gen::<f64>() < (-dE / TEMPERATURE).exp() {
spins[i][j] *= -1;
}
}
}
}
fn main() {
// Initialize the lattice: all spins are set to +1.
let mut spins = vec![vec![1; L]; L];
let mut rng = rand::thread_rng();
let steps = 10_000; // Number of Monte Carlo steps
// Run the simulation.
for _ in 0..steps {
metropolis_step(&mut spins, &mut rng);
}
// Calculate the total magnetization.
let total_spin: f64 = spins.iter().flat_map(|row| row.iter()).map(|&s| s as f64).sum();
let magnetization = total_spin / (L * L) as f64;
println!("Magnetization per site after simulation: {:.6}", magnetization);
}
Example 2: Estimating Material Properties with Monte Carlo Integration
This example demonstrates how Monte Carlo integration can be used to estimate thermodynamic properties such as heat capacity. By sampling the energy distribution of a system over a wide range and averaging the energy and its square, one can compute the heat capacity via the fluctuation–dissipation theorem. In this simplified example, we assume a quadratic energy function as a model for the potential energy, and the Boltzmann factor weights each sample appropriately.
use rand::Rng;
/// Defines the potential energy function for a harmonic oscillator: V(x) = 0.5 * x^2.
fn energy(x: f64) -> f64 {
0.5 * x * x
}
/// Uses Monte Carlo integration to estimate the heat capacity Cv of a system at a given temperature.
/// The function samples the energy over the range [-10, 10], computes the weighted average energy and its square,
/// and then estimates Cv via the relation: Cv = (⟨E²⟩ - ⟨E⟩²) / T².
fn monte_carlo_integration(samples: usize, temperature: f64) -> f64 {
let mut rng = rand::thread_rng();
let mut energy_sum = 0.0;
let mut energy_squared_sum = 0.0;
for _ in 0..samples {
// Sample a position x uniformly from the interval [-10, 10].
let x: f64 = rng.gen_range(-10.0..10.0);
let e = energy(x);
let boltzmann_factor = (-e / temperature).exp();
energy_sum += e * boltzmann_factor;
energy_squared_sum += e * e * boltzmann_factor;
}
let avg_energy = energy_sum / samples as f64;
let avg_energy_squared = energy_squared_sum / samples as f64;
(avg_energy_squared - avg_energy * avg_energy) / (temperature * temperature)
}
fn main() {
let samples = 1_000_000;
let temperature = 2.5;
let heat_capacity = monte_carlo_integration(samples, temperature);
println!("Estimated heat capacity: {:.6}", heat_capacity);
}
Example 3: Case Study—Modeling Disordered Systems (Spin Glass)
Monte Carlo methods are also used to study disordered systems. For instance, a spin glass is characterized by random interactions between spins (some ferromagnetic and some antiferromagnetic). In this example, the interaction energies are randomly assigned for each lattice site, and the Monte Carlo simulation is run similarly to the standard Ising model. This allows exploration of how disorder affects macroscopic properties like magnetization.
use rand::Rng;
/// Lattice size for the disordered system.
const L_DISORDER: usize = 10;
/// For disordered systems, we randomly choose interactions of either ferromagnetic (+1) or antiferromagnetic (-1).
const J_VALUES: [f64; 2] = [1.0, -1.0];
/// Temperature for the simulation.
const TEMP_DISORDER: f64 = 1.0;
/// Computes the energy change for flipping a spin in a disordered lattice.
/// Each site’s interactions are provided by the `interactions` matrix, which specifies the coupling
/// constant for each site (assuming the same value for all neighbors in a simplified model).
fn delta_energy_disordered(spins: &Vec<Vec<i32>>, interactions: &Vec<Vec<f64>>, i: usize, j: usize) -> f64 {
let spin = spins[i][j];
// Use periodic boundary conditions to determine neighboring sites.
let neighbors = [
(if i == 0 { L_DISORDER - 1 } else { i - 1 }, j),
((i + 1) % L_DISORDER, j),
(i, if j == 0 { L_DISORDER - 1 } else { j - 1 }),
(i, (j + 1) % L_DISORDER),
];
let mut interaction_energy = 0.0;
for &(ni, nj) in &neighbors {
// Use the provided interaction strength at site (i, j) with neighbor (ni, nj).
interaction_energy += interactions[i][j] * spin as f64 * spins[ni][nj] as f64;
}
2.0 * interaction_energy
}
/// Performs a Metropolis sweep for a disordered Ising model simulation.
/// The lattice spins are updated based on the energy change computed using randomly assigned interactions.
fn metropolis_step_disordered(spins: &mut Vec<Vec<i32>>, interactions: &Vec<Vec<f64>>, rng: &mut impl Rng) {
for i in 0..L_DISORDER {
for j in 0..L_DISORDER {
let dE = delta_energy_disordered(spins, interactions, i, j);
if dE < 0.0 || rng.gen::<f64>() < (-dE / TEMP_DISORDER).exp() {
spins[i][j] *= -1;
}
}
}
}
fn main() {
// Initialize a disordered lattice with all spins set to +1.
let mut spins = vec![vec![1; L_DISORDER]; L_DISORDER];
let mut rng = rand::thread_rng();
// Generate a random interactions matrix with values chosen from J_VALUES.
let interactions: Vec<Vec<f64>> = (0..L_DISORDER).map(|_| {
(0..L_DISORDER).map(|_| {
J_VALUES[rng.gen_range(0..J_VALUES.len())]
}).collect()
}).collect();
let steps = 10_000;
for _ in 0..steps {
metropolis_step_disordered(&mut spins, &interactions, &mut rng);
}
// Compute the magnetization per site for the disordered system.
let total_spin: i32 = spins.iter().flat_map(|row| row.iter()).sum();
let magnetization = total_spin as f64 / (L_DISORDER * L_DISORDER) as f64;
println!("Magnetization per site in disordered system (spin glass): {:.6}", magnetization);
}
In the disordered system example, a lattice of size LDISORDER×LDISORDERL\_{\\text{DISORDER}} \\times L\_{\\text{DISORDER}} is initialized with all spins set to +1. The interactions matrix is randomly generated with each interaction chosen as either +1 or –1, simulating a spin glass. The delta_energy_disordered
function computes the energy change for flipping a spin by considering the interactions with its nearest neighbors under periodic boundary conditions. The Monte Carlo simulation is performed by iterating for a specified number of steps and updating the spins accordingly. Finally, the average magnetization per site is computed and printed, providing an indicator of the system's macroscopic behavior under disorder.
These examples underscore the broad applicability of Monte Carlo methods in statistical mechanics—from studying phase transitions using the Ising model to estimating thermodynamic properties with integration techniques and exploring disordered systems. Rust’s strong performance, strict compile-time guarantees, and powerful libraries for randomness and concurrency make it a compelling choice for building reliable and scalable simulation tools. By combining robust error analysis with parallelization and advanced sampling techniques, researchers can gain deep insights into complex physical systems using Monte Carlo methods.
16.10. Conclusion
Chapter 16 provides a thorough exploration of Monte Carlo methods in statistical mechanics, emphasizing their implementation using Rust. By covering essential algorithms, practical applications, and advanced techniques, this chapter equips readers with the tools to effectively apply Monte Carlo simulations in their computational physics endeavors. Embrace these methods and leverage Rust's capabilities to advance your understanding of statistical mechanics and beyond.
16.10.1. Further Learning with GenAI
The following prompts focus on theoretical underpinnings, complex algorithms, optimization strategies, and advanced applications. Each prompt aims to delve deeply into the nuances of Monte Carlo simulations, ensuring a thorough understanding and application of these methods in a Rust programming context.
Elaborate on the theoretical foundations of Monte Carlo methods within the context of statistical mechanics, focusing on the roles of Markov chains and stochastic processes. How are these theoretical concepts meticulously implemented in Rust to ensure the accuracy and efficiency of simulations in complex physical systems?
Provide an in-depth analysis of the implementation of various random number generators (RNGs) in Rust, including Mersenne Twister and Linear Congruential Generators. Discuss the trade-offs associated with each RNG in terms of performance, statistical robustness, and suitability for Monte Carlo simulations, particularly in the context of high-stakes scientific computing.
Offer a comprehensive explanation of the Metropolis-Hastings algorithm, including a detailed derivation of the acceptance probability and the underlying detailed balance conditions. How can this algorithm be optimized for performance and accuracy, and what are the best practices for implementing it efficiently in Rust for large-scale simulations?
Analyze the challenges and techniques involved in Monte Carlo integration within high-dimensional spaces. How do issues like dimensionality and convergence rates impact the accuracy of these simulations? Provide a Rust implementation that demonstrates advanced methods for handling high-dimensional integration with a focus on computational efficiency.
Explain the Ising model in the broader context of lattice-based systems and statistical mechanics. How can Monte Carlo methods, such as Metropolis sampling, be effectively utilized to investigate phase transitions and critical phenomena in the Ising model using Rust? Include a detailed implementation example.
Discuss advanced error analysis techniques in Monte Carlo simulations, including variance reduction methods and bootstrapping. How can these techniques be rigorously integrated into Rust-based simulations to enhance both the accuracy and reliability of results? Provide examples of implementation strategies.
Examine the challenges associated with parallelizing Monte Carlo simulations, particularly concerning load balancing and synchronization issues. How can Rust’s concurrency features, including the
rayon
crate andasync/await
mechanisms, be strategically leveraged to overcome these challenges and optimize simulation performance?Detail the implementation of different statistical ensembles (canonical, grand canonical, microcanonical) in Monte Carlo simulations. How can Rust be utilized to model and simulate these ensembles with a high degree of accuracy and computational efficiency? Provide a step-by-step guide for each ensemble type.
Explore various optimization techniques for enhancing Monte Carlo simulations in Rust, including algorithmic improvements, data structures, and memory management strategies. How can these optimizations be applied to significantly boost the performance and scalability of simulations, particularly for large-scale and high-complexity problems?
Provide detailed case studies where Monte Carlo methods have been applied to solve real-world problems, such as in financial modeling or materials science. How can these applications be effectively modeled and simulated using Rust, and what insights can be drawn from these simulations in terms of practical outcomes and computational challenges?
Compare and contrast true random number generators (TRNGs) and pseudo-random number generators (PRNGs) concerning their applicability in Monte Carlo simulations. How does the choice between TRNGs and PRNGs influence the outcomes of simulations, and what are the best practices for selecting and implementing RNGs in Rust for different types of Monte Carlo applications?
Investigate the concept of phase transitions in the Ising model and similar systems. How can Monte Carlo methods be specifically adapted to study these transitions, and what are the implications for simulation accuracy and computational efficiency? Provide a detailed Rust implementation example that illustrates these concepts.
Detail the methodologies for calculating and interpreting error bars and confidence intervals in Monte Carlo simulations. How can Rust be utilized to implement these calculations robustly, and what techniques can be employed to assess and improve the reliability of simulation results in scientific research?
Explore advanced sampling methods aimed at improving the convergence rate of Monte Carlo simulations, such as importance sampling and Hamiltonian Monte Carlo. How can these methods be effectively implemented in Rust to enhance sampling efficiency, particularly in high-dimensional and complex systems?
Explain the Metropolis-Hastings algorithm’s variants, including Parallel Tempering and Replica Exchange Monte Carlo. How do these methods address specific sampling challenges in complex systems, and what are the best practices for implementing these advanced algorithms in Rust?
Discuss strategies for tackling high-dimensional integration problems using Monte Carlo methods, including dimensional reduction techniques and adaptive algorithms. Provide a detailed Rust-based approach for efficiently solving these problems, highlighting the trade-offs and benefits of each technique.
Analyze the impact of parallel computing on Monte Carlo simulations, with a focus on the use of multi-threading and distributed computing. How can Rust’s concurrency and parallelism features be harnessed to maximize simulation performance, and what are the best practices for ensuring scalability and accuracy in large-scale simulations?
Identify common pitfalls and challenges in Monte Carlo simulations, such as convergence issues, autocorrelation, and sampling biases. How can these challenges be effectively mitigated in Rust-based simulations through improved algorithms, debugging strategies, and advanced computational techniques?
Examine the application of Monte Carlo methods to non-equilibrium statistical mechanics. How can Rust be utilized to model and simulate non-equilibrium systems, and what unique challenges and solutions are associated with these simulations, particularly in the context of accurate and efficient computation?
Explore the integration of Monte Carlo methods with other computational techniques, such as machine learning or optimization algorithms. How can Rust facilitate this interdisciplinary approach, and what are the potential benefits and challenges of combining Monte Carlo simulations with advanced computational methodologies?
Discuss the future trends and advancements in Monte Carlo methods and their wide-ranging applications across various scientific fields. How can Rust be strategically positioned to take advantage of these advancements, and what emerging areas of research and development are likely to benefit from Rust’s unique capabilities?
By delving into these advanced topics and mastering their implementation in Rust, you are not only enhancing your computational skills but also pushing the boundaries of what is possible in scientific simulation. The depth of your understanding and the quality of your implementations will shape the future of computational physics.
16.10.2. Assignments for Practice
These exercises cover advanced topics such as high-dimensional integration, algorithm optimization, phase transition analysis, parallelization, and error analysis.
Exercise 16.1: Advanced Monte Carlo Integration
Task: Implement a Monte Carlo integration algorithm in Rust to estimate the value of a high-dimensional integral. Use advanced variance reduction techniques such as importance sampling or control variates to improve accuracy and efficiency. Compare the performance of your implementation with a standard Monte Carlo approach, and analyze the convergence rates and error margins.
Steps:
Define a complex, high-dimensional integral to estimate.
Implement the standard Monte Carlo integration method.
Apply an advanced variance reduction technique to the integration process.
Evaluate and compare the accuracy and performance of both methods.
Provide a detailed analysis of the results and discuss how variance reduction affects the convergence and efficiency of the simulation.
Exercise 16.2: Metropolis-Hastings Algorithm Optimization
Task: Develop a Rust implementation of the Metropolis-Hastings algorithm for sampling from a probability distribution. Optimize the algorithm by tuning parameters such as the proposal distribution and acceptance criteria. Extend your implementation to handle multiple chains and perform parallel sampling using Rust’s concurrency features.
Steps:
Implement the Metropolis-Hastings algorithm in Rust for a chosen probability distribution.
Experiment with different proposal distributions and acceptance criteria to optimize the sampling process.
Extend the implementation to support multiple chains and parallel sampling.
Measure and compare the performance of serial versus parallel sampling.
Discuss the impact of parameter tuning and parallelism on the efficiency and accuracy of the sampling.
Exercise 16.3: Ising Model Simulation with Phase Transition Analysis
Task: Simulate the Ising model on a 2D lattice using the Metropolis algorithm in Rust. Investigate the phase transition by varying parameters such as temperature and lattice size. Analyze the results to identify critical temperatures and compare the simulation outcomes with theoretical predictions.
Steps:
Implement the Metropolis algorithm for the 2D Ising model in Rust.
Perform simulations for various temperatures and lattice sizes.
Analyze the simulation data to identify critical temperatures and phase transitions.
Compare the results with theoretical predictions and discuss any discrepancies.
Provide a comprehensive report on how different parameters affect the phase transition behavior in the Ising model.
Exercise 16.4: Parallelization of Monte Carlo Simulations
Task: Create a parallelized Monte Carlo simulation for a complex problem, such as high-dimensional integration or the Ising model. Utilize Rust’s concurrency features, including threads and async/await, to distribute the computation across multiple cores. Measure the impact of parallelism on the simulation performance and analyze the trade-offs involved.
Steps:
Implement a Monte Carlo simulation for a chosen problem in Rust.
Parallelize the simulation using Rust’s concurrency features (e.g., threads, async/await).
Measure and analyze the performance improvements achieved through parallelism.
Evaluate the trade-offs in terms of complexity and resource usage.
Discuss how parallelism affects the accuracy and efficiency of the simulation and provide insights into best practices for parallel Monte Carlo simulations.
Exercise 16.5: Error Analysis and Statistical Validation
Task: Conduct a detailed error analysis of a Monte Carlo simulation, including estimating statistical errors and validating the simulation results. Implement techniques such as bootstrapping and error propagation in Rust. Provide a comprehensive analysis of the error margins, confidence intervals, and the overall reliability of the simulation outcomes.
Steps:
Implement a Monte Carlo simulation for a specific problem in Rust.
Apply error analysis techniques such as bootstrapping and error propagation to estimate statistical errors.
Calculate and interpret error margins and confidence intervals for the simulation results.
Validate the results using known analytical solutions or benchmark data.
Write a detailed report on the error analysis, discussing the reliability of the simulation outcomes and the effectiveness of the error estimation techniques used.
Approach these exercises with determination and curiosity, as they will not only enhance your technical abilities but also broaden your understanding of computational physics. The skills you develop through these exercises will empower you to tackle sophisticated problems and contribute to innovative solutions in the field.