Chapter 15
Continuum Mechanics Simulations
"The physicist is not merely a man of science, but also a man who has the ability to make the invisible visible." — Richard Feynman
Chapter 15 delves into the implementation of continuum mechanics simulations using Rust, providing a comprehensive guide from fundamental concepts to advanced topics. It covers the basics of continuum mechanics, mathematical formulations, and the Finite Element Method (FEM). The chapter includes detailed discussions on stress and strain analysis, dynamic simulations, and material models. Additionally, it addresses practical aspects such as boundary conditions, load applications, post-processing, and validation. By integrating these elements, the chapter equips readers with the skills to develop robust continuum mechanics simulations and tackle complex problems in computational physics using Rust.
15.1. Introduction to Continuum Mechanics
In this section, we delve into the fundamentals of continuum mechanics, an essential area of computational physics that deals with the behavior of continuous materials. The principles governing continuum mechanics are crucial for simulating physical phenomena such as fluid flow, structural deformation, and heat conduction, where materials are treated as continuous media rather than as discrete particles.
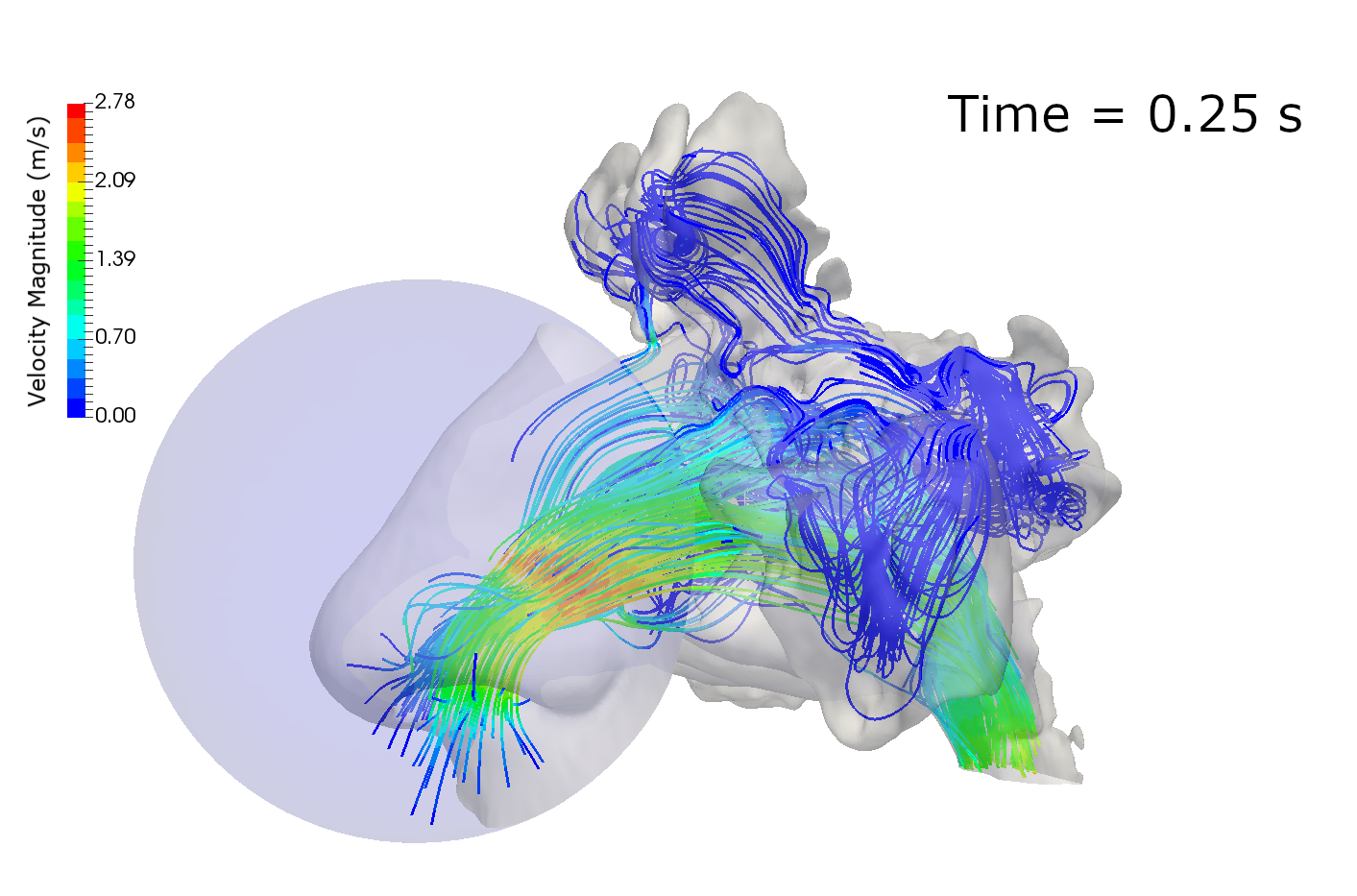
Illustration of computational continuum mechanics.
Unlike discrete models that consider materials as collections of particles, continuum mechanics assumes that materials are continuously distributed, allowing for the application of differential equations to describe physical properties. This assumption simplifies the modeling of complex materials and enables the analysis of phenomena like stress and strain, which are critical for predicting how materials will react to forces and deformations.
Continuum vs. discrete models is another fundamental distinction. In continuum models, the material is assumed to be infinitely divisible, with no gaps or discontinuities. This contrasts with discrete models, where materials are composed of distinct particles or elements. The essential assumptions in continuum mechanics involve treating materials as continuous, homogeneous, and isotropic, although these assumptions can be relaxed in more complex models.
Stress refers to the internal forces within a material, while strain measures the resulting deformation. Tensor notation is used to express these quantities mathematically, providing a compact and powerful way to describe the relationships between different components of stress and strain. Field equations, such as the Cauchy momentum equation and the Navier-Stokes equations, are employed to model the behavior of continuous materials under various conditions.
The role of continuum assumptions is critical in simulations, as they define the scope and applicability of the models used. For instance, in simulating fluid flow or structural deformation, the assumption that the material behaves as a continuum allows the use of differential equations to predict the material's response accurately.
A simple example might involve simulating the deformation of a solid object under stress. This can be done by implementing the fundamental equations governing stress and strain in Rust.
For example, consider a basic Rust code snippet to represent stress and strain in a 2D material:
// Cargo.toml dependencies:
// [dependencies]
// none required for this minimal example
/// Represents the 2D strain tensor components for a linear elastic material.
/// epsilon_xx, epsilon_yy, and epsilon_xy refer to the normal and shear strains in 2D.
struct Strain {
epsilon_xx: f64,
epsilon_yy: f64,
epsilon_xy: f64,
}
/// Represents the 2D stress tensor components for a linear elastic material.
/// sigma_xx, sigma_yy, and sigma_xy store the normal and shear stresses, respectively.
struct Stress {
sigma_xx: f64,
sigma_yy: f64,
sigma_xy: f64,
}
/// Computes the stress tensor from the given strain tensor for a linear elastic material,
/// applying Hooke's law in 2D using Lamé’s constants lambda and mu.
/// 'modulus' is Young's modulus (E), and 'poisson_ratio' is Poisson's ratio (ν).
fn compute_stress(strain: &Strain, modulus: f64, poisson_ratio: f64) -> Stress {
// Compute Lamé's parameters (λ and μ) for a linear isotropic material.
// lambda = (νE) / ((1 + ν)(1 - 2ν)) and mu = E / (2(1 + ν))
let lambda = poisson_ratio * modulus / ((1.0 + poisson_ratio) * (1.0 - 2.0 * poisson_ratio));
let mu = modulus / (2.0 * (1.0 + poisson_ratio));
// Stress–strain relationships in 2D (plane stress or plane strain) can be simplified.
// In this example, we assume a plane stress context for demonstration.
Stress {
sigma_xx: lambda * (strain.epsilon_xx + strain.epsilon_yy) + 2.0 * mu * strain.epsilon_xx,
sigma_yy: lambda * (strain.epsilon_xx + strain.epsilon_yy) + 2.0 * mu * strain.epsilon_yy,
sigma_xy: 2.0 * mu * strain.epsilon_xy,
}
}
fn main() {
// Example usage: Suppose we have a 2D material with a strain state and known material properties.
let strain_example = Strain {
epsilon_xx: 0.001, // 0.1% strain in the x direction
epsilon_yy: -0.0005, // -0.05% strain in the y direction (possibly due to Poisson effect)
epsilon_xy: 0.0002, // 0.02% shear strain
};
// Material properties, for instance, steel-like material:
let youngs_modulus = 210e9; // E in Pascals
let poisson_ratio = 0.3; // Typical for steel
// Compute the resulting 2D stress components based on the linear elastic model.
let stress_result = compute_stress(&strain_example, youngs_modulus, poisson_ratio);
println!("Computed Stress Tensor:");
println!("sigma_xx: {:.6e} Pa", stress_result.sigma_xx);
println!("sigma_yy: {:.6e} Pa", stress_result.sigma_yy);
println!("sigma_xy: {:.6e} Pa", stress_result.sigma_xy);
}
In this code, two simple struct
s, Strain
and Stress
, store the relevant tensor components for a 2D problem. The compute_stress
function uses standard formulas for a linear elastic, isotropic material—Hooke’s law in 2D plane conditions—to compute stress from strain. The parameters $\lambda$ and $\mu$ (Lamé constants) are derived from Young’s modulus EEE and Poisson’s ratio $\nu$. While this example only shows how to convert strain to stress, the same principles apply when constructing larger FEA simulations that discretize domains, assemble stiffness matrices, impose boundary conditions, and solve for nodal displacements. The stress at each location (or each element) is then computed based on the strain derived from these displacements.
Continuum mechanics underlies various computational physics applications. By assuming materials are continuously distributed and sufficiently smooth, we can apply powerful differential equations to model how solids deform or fluids flow under specified loads and boundary conditions. Rust’s performance and memory safety features make it particularly well-suited for implementing these continuum models, as it can handle the often complex data structures needed and ensure safe parallelization for large-scale simulations.
This overview establishes the foundation for deeper explorations into continuum mechanics, including non-linear material behaviors, multi-dimensional simulations, coupling with fluid flow models, and more advanced finite element techniques. Rust’s modern toolchain and extensive ecosystem can significantly aid researchers and engineers in building robust, high-performance codes that accurately reflect continuum mechanics principles.
15.2. Mathematical Formulation and Equations
Continuum mechanics provides the theoretical foundation for modeling materials as continuous media, where properties such as mass, momentum, and energy are conserved. The governing equations of continuum mechanics include Cauchy’s equations, Navier–Cauchy equations, and related formulations such as the Navier–Stokes equations for fluid flow. All of these rely on tensor algebra to describe how different components of stress, strain, and other physical quantities vary throughout a continuous body.
At the heart of continuum mechanics is the concept of a constitutive model, which describes how materials respond to external loads. Linear elastic models (e.g., Hooke’s law) assume a direct, proportional relationship between stress and strain, suitable for small deformations. More advanced models consider material and geometric nonlinearities, capturing effects such as plastic deformation or large strains. The distinction between isotropy (same properties in all directions) and anisotropy (direction-dependent properties) further refines these models, leading to more accurate simulations.
Understanding how the equations of continuum mechanics are derived from fundamental physical laws (e.g., conservation of mass, momentum, and energy) reinforces the assumptions that underlie the models. For instance, deriving the Cauchy momentum equation from Newton’s second law in a continuous medium underscores the continuum assumption and clarifies the role of the stress tensor in governing how forces are transmitted within a material.
Below is a code snippet that illustrates a common constitutive model in continuum mechanics: the linear elasticity model for a 3D material. This Rust example defines stress and strain tensors and then uses Hooke’s law with Lamé’s parameters ($\lambda$ and $\mu$) to compute stress from strain.
use std::fmt;
/// A 3D strain tensor for a linear elastic material, storing normal (xx, yy, zz) and shear (xy, xz, yz) components.
#[derive(Debug)]
struct StrainTensor {
epsilon_xx: f64,
epsilon_yy: f64,
epsilon_zz: f64,
epsilon_xy: f64,
epsilon_xz: f64,
epsilon_yz: f64,
}
/// A 3D stress tensor, mirroring the structure of the strain tensor.
#[derive(Debug)]
struct StressTensor {
sigma_xx: f64,
sigma_yy: f64,
sigma_zz: f64,
sigma_xy: f64,
sigma_xz: f64,
sigma_yz: f64,
}
/// Implements a 3D linear elastic constitutive model (Hooke's law).
/// Takes a strain tensor along with material properties (Young's modulus, Poisson's ratio) and
/// calculates the corresponding stress tensor.
/// The Lamé parameters λ and μ are derived as follows:
/// λ = (νE) / [(1 + ν)(1 - 2ν)]
/// μ = E / [2(1 + ν)]
fn compute_stress_linear(strain: &StrainTensor, young_modulus: f64, poisson_ratio: f64) -> StressTensor {
let lambda = poisson_ratio * young_modulus
/ ((1.0 + poisson_ratio) * (1.0 - 2.0 * poisson_ratio));
let mu = young_modulus / (2.0 * (1.0 + poisson_ratio));
let trace = strain.epsilon_xx + strain.epsilon_yy + strain.epsilon_zz;
StressTensor {
sigma_xx: lambda * trace + 2.0 * mu * strain.epsilon_xx,
sigma_yy: lambda * trace + 2.0 * mu * strain.epsilon_yy,
sigma_zz: lambda * trace + 2.0 * mu * strain.epsilon_zz,
sigma_xy: mu * strain.epsilon_xy,
sigma_xz: mu * strain.epsilon_xz,
sigma_yz: mu * strain.epsilon_yz,
}
}
fn main() {
// Example usage of the linear elasticity model:
// Suppose a small 3D deformation occurs in a material like steel (E ~ 210 GPa, ν ~ 0.3).
let strain_example = StrainTensor {
epsilon_xx: 0.0005,
epsilon_yy: -0.0001,
epsilon_zz: 0.0002,
epsilon_xy: 0.0003,
epsilon_xz: -0.0002,
epsilon_yz: 0.0001,
};
let youngs_modulus = 210e9; // E in Pascals
let poisson_ratio = 0.3; // Typical for steel
let stress_result = compute_stress_linear(&strain_example, youngs_modulus, poisson_ratio);
println!("Computed Stress Tensor:\n{:?}", stress_result);
}
In this example, the code defines a simple 3D strain model that captures six independent components $(\epsilon_{xx}, \epsilon_{yy}, \epsilon_{zz}, \epsilon_{xy}, \epsilon_{xz}, \epsilon_{yz})$ for a linear elastic material. The compute_stress_linear
function calculates the associated stress components by using standard formulas from continuum mechanics with Hooke’s law for isotropic, linear elasticity. Here, $\lambda$ and $\mu$ (Lamé constants) are derived from Young’s modulus (EE) and Poisson’s ratio ($\nu$).
Beyond stress–strain relationships, continuum mechanics also involves solving partial differential equations (PDEs) that express how these stresses and strains evolve in a continuous body under load. Numerically solving such PDEs often involves discretization methods like the finite element method (FEM) or finite difference method (FDM). Below is an example illustrating a 1D heat equation solver using a simple finite difference scheme. While this code targets a thermal problem, it demonstrates the general approach of discretizing PDEs in Rust:
/// Solves the 1D heat equation:
/// ∂T/∂t = α ∂²T/∂x²
/// using an explicit finite difference method.
/// - `initial_temp` is the initial temperature distribution.
/// - `alpha` is the thermal diffusivity.
/// - `dx` and `dt` are the spatial and temporal steps, respectively.
/// - `time_steps` is the number of time iterations to perform.
fn solve_heat_equation_1d(
initial_temp: Vec<f64>,
alpha: f64,
dx: f64,
dt: f64,
time_steps: usize,
) -> Vec<f64> {
let mut temp = initial_temp.clone();
let mut new_temp = initial_temp.clone();
let n = temp.len();
for _ in 0..time_steps {
for i in 1..(n - 1) {
new_temp[i] = temp[i] + alpha * dt / (dx * dx) *
(temp[i + 1] - 2.0 * temp[i] + temp[i - 1]);
}
// Update the temperature distribution for the next iteration.
temp.copy_from_slice(&new_temp);
}
temp
}
fn main() {
// Example initial condition: a rod of length 1 m, discretized into 11 points.
let n = 11;
let dx = 1.0 / (n as f64 - 1.0);
let alpha = 1e-5; // Thermal diffusivity
let dt = 0.5 * dx * dx / alpha; // Time step for stability
let time_steps = 50;
let initial_temp = vec![300.0; n]; // Uniform initial temperature (300 K)
// Solve for the temperature distribution over 'time_steps'.
let final_temp = solve_heat_equation_1d(initial_temp, alpha, dx, dt, time_steps);
println!("Final temperature distribution after {} steps:", time_steps);
for (i, &t) in final_temp.iter().enumerate() {
println!("Node {}: {:.2} K", i, t);
}
}
In this heat equation solver, each iteration updates the temperature at interior points based on the finite difference approximation of the second spatial derivative $\frac{\partial^2 T}{\partial x^2}$. Boundary conditions (e.g., fixed temperatures at the ends of the rod) can be enforced by holding the end nodes constant, ensuring the PDE solution matches the physical scenario.
The examples shown illustrate how to implement continuum mechanics concepts in Rust: from basic stress–strain relationships to solving PDEs via discretization. Such code typically appears in larger FEA frameworks where domain discretization, matrix assembly, boundary condition enforcement, and solver routines all come together. Rust’s strengths in performance, safety, and parallel programming align well with the computational demands of large-scale continuum mechanics simulations, making it a promising platform for advanced research and industrial applications alike.
15.3. Finite Element Method (FEM)
The Finite Element Method (FEM) is one of the most powerful and versatile numerical techniques used in continuum mechanics. Its fundamental approach involves dividing a continuous domain into smaller, simpler elements, approximating the behavior of physical fields—such as displacement, temperature, or pressure—using interpolation (shape) functions. Through this discretization, complex differential equations are transformed into a system of algebraic equations that can be solved using computational methods. FEM’s flexibility in handling complex geometries and boundary conditions makes it widely applicable across engineering disciplines, from structural analysis to heat transfer and fluid flow.
FEM computations often proceed through several core steps:
Mesh Generation, where the geometry is discretized into elements (e.g., triangles in 2D, tetrahedra in 3D).
Shape Function Definition, where polynomials or other basis functions approximate field variables within each element.
Element Matrix Assembly, where local stiffness (or analogous) matrices are computed for each element and aggregated into a global matrix.
Application of Boundary Conditions, imposing constraints or loads at nodes.
Solution of the Resulting System, typically a large sparse system of equations.
Post-Processing, which includes computing stresses, strains, or other derived quantities and visualizing results.
Below is a Rust code example that demonstrates how one might represent and assemble a simple triangular element in 2D, define linear shape functions, and compute a (highly simplified) local stiffness matrix. We then show how these local matrices are incorporated into a global stiffness matrix for the domain. While the snippet is intentionally minimal, it lays out the core structures and function outlines in a clear, logical manner.
// Cargo.toml dependencies:
// [dependencies]
// none required for minimal example
/// A 2D node in the finite element mesh, storing its coordinates (x, y).
#[derive(Debug, Clone)]
struct Node {
x: f64,
y: f64,
}
/// A triangular element defined by three nodes.
/// In a real FEA code, one typically stores references/indices to global node data.
#[derive(Debug, Clone)]
struct Element {
nodes: [Node; 3],
}
impl Element {
/// Computes linear shape functions for a triangular element at a given point (xi, eta)
/// in the element's local coordinate system. The shape functions for a linear triangle (L1, L2, L3) are:
/// N1 = 1 - xi - eta
/// N2 = xi
/// N3 = eta
/// This example uses a simple local (ξ, η) coordinate system for demonstration.
fn shape_functions(&self, xi: f64, eta: f64) -> [f64; 3] {
[
1.0 - xi - eta, // N1
xi, // N2
eta, // N3
]
}
/// Calculates the determinant of the Jacobian (2D) for a linear triangular element.
/// This is effectively twice the area of the triangle and is needed when integrating
/// local matrices or transforming derivatives between local and global coordinates.
fn jacobian(&self) -> f64 {
let (x1, y1) = (self.nodes[0].x, self.nodes[0].y);
let (x2, y2) = (self.nodes[1].x, self.nodes[1].y);
let (x3, y3) = (self.nodes[2].x, self.nodes[2].y);
// Determinant of [[x2 - x1, x3 - x1], [y2 - y1, y3 - y1]]
(x2 - x1) * (y3 - y1) - (x3 - x1) * (y2 - y1)
}
}
/// Computes a local stiffness matrix for a linear triangular element in 2D.
/// This is a placeholder example, illustrating how one might incorporate shape function
/// derivatives, the material properties, and the Jacobian to form a local matrix.
fn compute_local_stiffness(element: &Element, young_modulus: f64, poisson_ratio: f64) -> [[f64; 3]; 3] {
// For demonstration, we compute a trivial matrix or do a placeholder calculation.
// In real FEA, one would compute the B-matrix, D-matrix (material properties),
// and integrate over the element domain using shape function derivatives.
let mut local_stiff = [[0.0; 3]; 3];
let area_times_factor = element.jacobian().abs() * (young_modulus / (1.0 - poisson_ratio * poisson_ratio)) / 1000.0;
for i in 0..3 {
for j in 0..3 {
local_stiff[i][j] = area_times_factor * if i == j { 2.0 } else { -1.0 };
}
}
local_stiff
}
/// Assembles the global stiffness matrix for a 2D problem with a specified set of elements and material properties.
/// 'num_nodes' is the total number of nodes in the mesh.
fn assemble_global_stiffness(elements: &[Element], num_nodes: usize, young_modulus: f64, poisson_ratio: f64) -> Vec<Vec<f64>> {
// 2D: Each node typically has 2 degrees of freedom (e.g., x and y displacements).
let dof = 2 * num_nodes;
let mut global_stiffness = vec![vec![0.0; dof]; dof];
// For each element, compute the local stiffness matrix and add its contributions
// to the global matrix at the appropriate indices.
for element in elements {
let local_mat = compute_local_stiffness(element, young_modulus, poisson_ratio);
// Here, assume each element is connected to 3 distinct nodes, each node having 2 DOF.
// For demonstration, we sum the local stiffness matrix into the global one.
for (local_i, node_i) in element.nodes.iter().enumerate() {
// In a real code, one would look up the global node ID from the mesh,
// then map node_i's x and y DOF to the correct global matrix indices.
// This snippet shows a simplified version, ignoring node indexing and mapping complexities.
let global_i_x = 2 * local_i;
let global_i_y = 2 * local_i + 1;
for (local_j, node_j) in element.nodes.iter().enumerate() {
let global_j_x = 2 * local_j;
let global_j_y = 2 * local_j + 1;
// Insert the local stiffness values into the global matrix.
// Real implementations must handle x-y cross terms too.
global_stiffness[global_i_x][global_j_x] += local_mat[local_i][local_j];
global_stiffness[global_i_y][global_j_y] += local_mat[local_i][local_j];
}
}
}
global_stiffness
}
fn main() {
// Example usage: build a small mesh of two triangular elements.
// In practice, you'd have a more sophisticated mesh generator and indexing approach.
let node0 = Node { x: 0.0, y: 0.0 };
let node1 = Node { x: 1.0, y: 0.0 };
let node2 = Node { x: 0.0, y: 1.0 };
let node3 = Node { x: 1.0, y: 1.0 };
// Two triangles: (node0, node1, node2) and (node2, node1, node3).
let elem1 = Element { nodes: [node0.clone(), node1.clone(), node2.clone()] };
let elem2 = Element { nodes: [node2.clone(), node1.clone(), node3.clone()] };
let elements = vec![elem1, elem2];
let num_nodes = 4; // We have 4 distinct nodes total.
// Material properties for a simple linear elastic material.
let young_modulus = 210e9; // Pa, e.g. steel
let poisson_ratio = 0.3; // Typical for metals
// Assemble the global stiffness matrix for the entire mesh.
let global_k = assemble_global_stiffness(&elements, num_nodes, young_modulus, poisson_ratio);
println!("Global stiffness matrix (somewhat simplified):");
for row in global_k.iter() {
println!("{:?}", row);
}
}
In this simplified code:
Mesh Representation\
The Node
and Element
structs define the mesh for a 2D triangular problem. In practice, a more advanced data structure would track which node belongs to which element and handle indexing more rigorously.
Shape Functions and Jacobian\
The shape_functions
method shows linear shape functions for a triangle, while jacobian
calculates the determinant needed for integrals. Real implementations would compute shape function derivatives and evaluate integrals via numerical quadrature to build local stiffness matrices.
Local Matrix Computation\
The compute_local_stiffness
function is intentionally simplified—real FEA codes compute a B-matrix (relating strains to nodal displacements), a D-matrix (representing material properties), and perform integral evaluations over the element domain.
Global Matrix Assembly\
The function assemble_global_stiffness
accumulates each element’s local matrix into the global stiffness matrix, reflecting how local behaviors combine to model the entire domain. Proper indexing ensures each element’s contributions map to the correct rows/columns in the global matrix, corresponding to each node’s degrees of freedom.
Boundary Conditions and Solving\
Typically, boundary conditions would be applied by modifying rows/columns in the global stiffness matrix and the force vector. A solver (e.g., direct LU decomposition or iterative Conjugate Gradient) would then produce displacements. For brevity, these aspects are left out in the snippet but are essential in practice.
This overview highlights FEM’s core concepts—mesh discretization, shape function interpolation, local matrix assembly, and forming the global system. In real engineering applications, one also handles boundary conditions, advanced material models, nonlinearities, dynamic effects, and more. Rust’s robustness, performance, and concurrency primitives make it particularly suitable for large-scale finite element simulations, where efficient memory usage and safe parallel execution are critical.
15.4. Stress and Strain Analysis
Stress and strain analysis is a cornerstone of continuum mechanics, providing insights into how materials respond to external forces. This section delves into the fundamental concepts of stress and strain tensors, principal stresses and strains, and Mohr’s circle, followed by conceptual ideas around stress-strain relationships, failure criteria, and the importance of accurate stress analysis. The section concludes with practical implementation of stress and strain calculations in Rust, along with visualization and validation techniques.
Fundamental Concepts begin with the definitions and computations of stress and strain tensors. Stress is a measure of internal forces within a material, expressed as a tensor that relates force per unit area across different planes within the material. The stress tensor is typically represented in a matrix form, with components corresponding to normal and shear stresses on various planes. Strain, on the other hand, quantifies the deformation of the material, representing changes in length relative to the original dimensions. The strain tensor, similarly to the stress tensor, captures these deformations across different directions.
Principal stresses and strains are critical points within the stress and strain tensors where the shear stress is zero, and the normal stress reaches its maximum or minimum values. These principal values are particularly important because they provide the clearest indication of the material’s response under load, helping engineers predict potential failure points. The principal stresses are the eigenvalues of the stress tensor, and the corresponding eigenvectors indicate the directions of these stresses.
Mohr’s circle is a graphical tool used to visualize the state of stress at a point, particularly useful for understanding the relationships between normal and shear stresses on different planes. By plotting Mohr’s circle, one can easily identify the principal stresses and the maximum shear stress, as well as visualize how the state of stress changes as the material is rotated.
Conceptual Ideas focus on stress-strain relationships, which form the foundation for understanding how materials behave under various loading conditions. These relationships are typically defined through constitutive models, which link the stress tensor to the strain tensor. The simplest and most widely used model is Hooke’s law, applicable to linear elastic materials where stress is directly proportional to strain. More complex models, such as those for plasticity or viscoelasticity, account for non-linear behavior where the material does not return to its original shape after the load is removed.
Failure criteria are essential for determining the conditions under which materials will fail. Common criteria include the von Mises stress criterion for ductile materials and the maximum principal stress criterion for brittle materials. These criteria are used to predict whether the material will yield or fracture under a given load, making them crucial for design and safety assessments.
The importance of accurate stress analysis cannot be overstated. Predicting material behavior under load is vital for ensuring the safety and reliability of structures and components. Accurate stress analysis allows engineers to design structures that can withstand the expected loads without failing, ensuring both performance and safety.
In terms of practical implementation in Rust, stress and strain calculations can be encoded efficiently. Consider a Rust implementation that computes the stress tensor from a given strain tensor using Hooke’s law for an isotropic material:
// Cargo.toml dependencies:
// [dependencies]
// none required for minimal example of stress/strain
// optionally add plotters for Mohr’s circle visualization
/// A 3D strain tensor storing normal (xx, yy, zz) and shear (xy, xz, yz) components.
#[derive(Debug)]
struct StrainTensor {
epsilon_xx: f64,
epsilon_yy: f64,
epsilon_zz: f64,
epsilon_xy: f64,
epsilon_xz: f64,
epsilon_yz: f64,
}
/// A 3D stress tensor that mirrors the structure of the strain tensor.
#[derive(Debug)]
struct StressTensor {
sigma_xx: f64,
sigma_yy: f64,
sigma_zz: f64,
sigma_xy: f64,
sigma_xz: f64,
sigma_yz: f64,
}
/// Computes the 3D stress from a strain state for an isotropic, linear elastic material,
/// relying on Hooke’s law. The Lamé parameters λ and μ are derived from the Young’s modulus E
/// and Poisson’s ratio ν:
/// λ = (νE) / [(1+ν)(1−2ν)] and μ = E / [2(1+ν)].
fn compute_stress_linear(strain: &StrainTensor, young_modulus: f64, poisson_ratio: f64) -> StressTensor {
let lambda = poisson_ratio * young_modulus
/ ((1.0 + poisson_ratio) * (1.0 - 2.0 * poisson_ratio));
let mu = young_modulus / (2.0 * (1.0 + poisson_ratio));
let trace = strain.epsilon_xx + strain.epsilon_yy + strain.epsilon_zz;
StressTensor {
sigma_xx: lambda * trace + 2.0 * mu * strain.epsilon_xx,
sigma_yy: lambda * trace + 2.0 * mu * strain.epsilon_yy,
sigma_zz: lambda * trace + 2.0 * mu * strain.epsilon_zz,
sigma_xy: mu * strain.epsilon_xy,
sigma_xz: mu * strain.epsilon_xz,
sigma_yz: mu * strain.epsilon_yz,
}
}
fn main() {
// Suppose we have a strain state in a small region of a steel component:
let example_strain = StrainTensor {
epsilon_xx: 0.0005,
epsilon_yy: -0.0001,
epsilon_zz: 0.0002,
epsilon_xy: 0.0003,
epsilon_xz: 0.0,
epsilon_yz: -0.0002,
};
let young_modulus = 210e9; // Typical for steel
let poisson_ratio = 0.3;
let stress_result = compute_stress_linear(&example_strain, young_modulus, poisson_ratio);
println!("Computed Stress Tensor:\n{:#?}", stress_result);
}
In this example, the StressTensor
and StrainTensor
structs represent the stress and strain components in three dimensions. The compute_stress
function uses Hooke’s law to calculate the stress tensor from the strain tensor, incorporating material properties such as Young’s modulus and Poisson’s ratio. The Lamé parameters, lambda
and mu
, are derived from these material properties and play a crucial role in defining the relationship between stress and strain in isotropic materials.
Visualizing the results of stress and strain calculations is an important part of analysis. Rust can be paired with visualization libraries such as plotters
or gnuplot
to create graphical representations like Mohr’s circle or stress distribution plots. For example, using plotters
, one might visualize the principal stresses by plotting Mohr’s circle:
use plotters::prelude::*;
use std::error::Error;
fn plot_mohrs_circle(center: f64, radius: f64) -> Result<(), Box<dyn Error>> {
let root = BitMapBackend::new("mohrs_circle.png", (640, 480)).into_drawing_area();
root.fill(&WHITE)?;
let x_range = (center - 1.5 * radius)..(center + 1.5 * radius);
let y_range = (-1.5 * radius)..(1.5 * radius);
let mut chart = ChartBuilder::on(&root)
.caption("Mohr's Circle", ("sans-serif", 40))
.margin(20)
.build_cartesian_2d(x_range, y_range)?;
chart.configure_mesh().draw()?;
// Parameterize the circle in angles from 0 to 360 degrees.
let steps = 360;
let circle_points = (0..=steps).map(|deg| {
let theta = deg as f64 * std::f64::consts::PI / 180.0;
let x = center + radius * theta.cos();
let y = radius * theta.sin();
(x, y)
});
chart.draw_series(LineSeries::new(circle_points, &RED))?;
Ok(())
}
fn main() -> Result<(), Box<dyn std::error::Error>> {
let sigma_avg = 50e6; // Suppose 50 MPa average normal stress
let circle_radius = 25e6; // 25 MPa half-difference between principal stresses
plot_mohrs_circle(sigma_avg, circle_radius)?;
println!("Mohr's circle plot saved as mohrs_circle.png.");
Ok(())
}
This code uses the plotters
crate to generate a visualization of Mohr’s circle. The plot_mohrs_circle
function takes the center and radius of Mohr’s circle and plots it on a Cartesian coordinate system. This visualization helps engineers and scientists interpret the stress state in a material, making it easier to identify critical points like maximum shear stress and principal stresses.
Validation with benchmark problems is essential to ensure that the simulations produce accurate and reliable results. This often involves comparing the results from the Rust implementation with known solutions or experimental data. For instance, a simple cantilever beam under a point load at its end can serve as a benchmark problem. The stress distribution along the beam can be calculated analytically and compared with the results from the Rust simulation to verify its accuracy.
15.5. Dynamic Simulations and Time Integration
Dynamic simulations are essential for understanding how materials or structures respond when loads vary with time. Unlike static analyses—where loads and conditions remain constant—dynamic simulations capture the evolution of a system under time-dependent forces. Examples include analyzing the vibration of a bridge as vehicles pass over it, modeling seismic responses in buildings, or predicting stress wave propagation in solids subject to impact. The main challenge in dynamic analysis is to accurately account for temporal changes while maintaining numerical stability and convergence. This is achieved by employing time integration methods that evolve the solution over discrete time steps.
Time integration methods fall broadly into explicit and implicit categories. Explicit methods, such as the forward (or central) Euler method, update the system state at the next time step directly from the current state. They are relatively simple to implement and computationally efficient for problems with mild stiffness; however, they require small time steps to preserve stability. In contrast, implicit methods, like backward Euler or the Newmark-beta method, involve solving a set of equations at each time step, making them more robust for stiff problems but also more computationally demanding. In practice, the choice of method is dictated by the problem’s stiffness, the desired accuracy, and the available computational resources.
In addition to time integration methods, it is important to handle dynamic boundary conditions appropriately. For example, if a structure is subject to time-varying loads, boundary conditions such as prescribed displacements or forces must be updated at each time step. Visualizing the transient response—such as the propagation of stress waves or the deformation of a vibrating beam—provides further insight into the dynamic behavior of the system.
The code below demonstrates a simple explicit time integration scheme for the one-dimensional wave equation, which models the propagation of waves in a medium. In this example, the function explicit_time_step
computes the next time step based on the current and previous displacement profiles, the wave speed, and the chosen time and spatial steps. In addition, a simple animation function using the plotters crate is provided to visualize the evolution of the wave over time.
// Cargo.toml dependencies:
// [dependencies]
// plotters = "0.3"
/// Advances the solution of the 1D wave equation using an explicit time-stepping scheme.
/// The wave equation is given by:
/// ∂²u/∂t² = c² ∂²u/∂x²
/// where u(x, t) is the displacement, c is the wave speed.
/// This function computes the next time step u_next based on the previous state (u_prev)
/// and the current state (u_curr). The parameters dt and dx represent the temporal and spatial
/// discretization steps, respectively.
fn explicit_time_step(u_prev: &[f64], u_curr: &[f64], c: f64, dt: f64, dx: f64) -> Vec<f64> {
let n = u_curr.len();
let mut u_next = vec![0.0; n];
// Apply the finite difference scheme to the interior points.
// Here, we use the central difference approximation in time and space.
for i in 1..(n - 1) {
u_next[i] = 2.0 * u_curr[i] - u_prev[i]
+ (c * c * dt * dt / (dx * dx)) * (u_curr[i + 1] - 2.0 * u_curr[i] + u_curr[i - 1]);
}
// Boundary conditions: here we assume fixed boundaries (u = 0 at the ends).
u_next[0] = 0.0;
u_next[n - 1] = 0.0;
u_next
}
/// Uses the plotters crate to create an animation of the wave propagation.
/// The function takes a vector of states, where each state is a time step's displacement vector,
/// and generates an animated GIF displaying the evolution of the wave along the 1D domain.
use plotters::prelude::*;
use std::error::Error;
fn animate_wave(wave_states: &[Vec<f64>], output_file: &str) -> Result<(), Box<dyn Error>> {
// Create a GIF backend with specified dimensions.
let root = BitMapBackend::gif(output_file, (640, 480), 100)?
.into_drawing_area();
root.fill(&WHITE)?;
// Determine the x-axis (spatial grid) based on the length of a state vector.
let n = wave_states[0].len();
let x_range = 0..n;
// Using plotters’ animated drawing area to create a series of frames.
for (step, state) in wave_states.iter().enumerate() {
let mut chart = ChartBuilder::on(&root)
.caption(format!("Wave Propagation, Step {}", step), ("sans-serif", 30))
.margin(20)
.x_label_area_size(30)
.y_label_area_size(30)
.build_cartesian_2d(x_range.clone(), -1.0f64..1.0f64)?;
chart.configure_mesh().draw()?;
chart.draw_series(LineSeries::new(
(0..n).map(|i| (i, state[i])),
&RED,
))?;
// Each frame is flushed automatically to the GIF.
}
root.present()?;
Ok(())
}
fn main() -> Result<(), Box<dyn Error>> {
// Parameters for the wave equation
let n = 101; // Number of spatial grid points
let dx = 1.0 / (n - 1) as f64; // Spatial step size
let c = 1.0; // Wave speed
// Stability criterion for explicit method: dt <= dx / c.
// Using a conservative value for dt.
let dt = 0.005;
// Number of time steps to simulate.
let time_steps = 200;
// Initial conditions: a single pulse in the middle of the domain.
let mut u_prev = vec![0.0; n];
let mut u_curr = vec![0.0; n];
// Apply an initial displacement pulse at the center.
u_curr[n / 2] = 1.0;
// Vector to hold all time step states for animation.
let mut wave_states: Vec<Vec<f64>> = Vec::with_capacity(time_steps);
wave_states.push(u_curr.clone());
// Time-stepping loop to simulate wave propagation.
for _ in 0..time_steps {
let u_next = explicit_time_step(&u_prev, &u_curr, c, dt, dx);
// Update the states: shift current to previous and u_next becomes the current.
u_prev = u_curr;
u_curr = u_next;
wave_states.push(u_curr.clone());
}
// Animate the wave propagation and save the result as a GIF.
animate_wave(&wave_states, "wave_animation.gif")?;
println!("Wave animation generated and saved as 'wave_animation.gif'.");
Ok(())
}
In this final example, the function explicit_time_step
advances the solution of the 1D wave equation using an explicit finite difference scheme. It computes the displacement at the next time step by considering the current and previous displacements, using a central difference approximation in both time and space. Boundary conditions are enforced by setting the endpoints to zero (representing fixed ends).
The function animate_wave
uses the plotters crate to generate an animated GIF. It loops through a series of wave states (the displacement vectors over time) and plots each state as a frame. The Cartesian coordinate system is defined based on the number of spatial grid points and a fixed range for displacements, while each frame's caption is updated to indicate the current time step.
The main
function sets up the initial conditions for the wave, simulates the wave propagation over several time steps, collects the states for visualization, and finally calls the animation function to generate the GIF. This complete example demonstrates both the dynamic simulation of a wave and the subsequent time integration, providing insight into how dynamic systems evolve over time and how those results can be visualized using Rust.
This section highlights that dynamic simulations and time integration are central to understanding transient behavior in continuum mechanics. Rust's performance and safety, combined with its modern concurrency and visualization tools, make it an ideal choice for developing efficient, robust dynamic simulation codes in computational physics.
15.6. Material Models and Constitutive Laws
Material models and constitutive laws form the heart of continuum mechanics simulations by establishing the mathematical relationships that describe how materials deform under load. These models encapsulate a material’s response to external forces, capturing behaviors ranging from linear elastic responses—where stress is directly proportional to strain—to more complex nonlinear effects such as plasticity, viscoelasticity, and hyperelasticity. In linear elasticity, small deformations are considered, and the stress–strain relationship is expressed via Hooke’s law using material constants such as Young’s modulus and Poisson’s ratio. This simple model serves as a cornerstone for many structural analyses, as it assumes the material behaves the same regardless of the magnitude of the applied load (up to the yield point). In contrast, plasticity models account for permanent deformation when the load exceeds a material’s yield strength, and viscoelastic or hyperelastic models capture time-dependent or large-strain behavior, respectively. The choice of material model significantly affects simulation accuracy and must be matched to the material behavior being studied. Robust implementation of these constitutive laws in a computational code ensures that the simulated response closely mirrors experimental reality and thus is critical for design and safety assessments. With Rust’s emphasis on performance, memory safety, and strong type systems, these models can be implemented with reliability and efficiency. The code examples below illustrate a basic linear elastic constitutive model and a simplified plasticity model. In the linear elastic example, stress is computed directly from the strain tensor using Hooke’s law in three dimensions, while the plasticity example introduces a yield criterion and a hardening modulus to account for permanent deformations, tracking the evolution of plastic strain.
// Define a 3D strain tensor containing normal and shear components.
#[derive(Debug)]
struct StrainTensor {
epsilon_xx: f64,
epsilon_yy: f64,
epsilon_zz: f64,
epsilon_xy: f64,
epsilon_xz: f64,
epsilon_yz: f64,
}
// Define a 3D stress tensor to capture the resulting stress components.
#[derive(Debug, Default)]
struct StressTensor {
sigma_xx: f64,
sigma_yy: f64,
sigma_zz: f64,
sigma_xy: f64,
sigma_xz: f64,
sigma_yz: f64,
}
// A simple material model for linear elasticity.
#[derive(Debug)]
struct Material {
young_modulus: f64, // Young's modulus, E
poisson_ratio: f64, // Poisson's ratio, ν
}
/// Computes the stress tensor using a linear elastic constitutive model (Hooke’s law) for an isotropic material.
/// The Lamé parameters λ and μ are computed from the material properties.
/// λ = (νE) / [(1+ν)(1−2ν)] and μ = E / [2(1+ν)].
/// The stress components are then calculated as:
/// σ_xx = λ(ε_xx+ε_yy+ε_zz) + 2μ ε_xx, etc.
fn compute_linear_elastic_stress(strain: &StrainTensor, material: &Material) -> StressTensor {
let lambda = material.poisson_ratio * material.young_modulus
/ ((1.0 + material.poisson_ratio) * (1.0 - 2.0 * material.poisson_ratio));
let mu = material.young_modulus / (2.0 * (1.0 + material.poisson_ratio));
let trace = strain.epsilon_xx + strain.epsilon_yy + strain.epsilon_zz;
StressTensor {
sigma_xx: lambda * trace + 2.0 * mu * strain.epsilon_xx,
sigma_yy: lambda * trace + 2.0 * mu * strain.epsilon_yy,
sigma_zz: lambda * trace + 2.0 * mu * strain.epsilon_zz,
sigma_xy: mu * strain.epsilon_xy,
sigma_xz: mu * strain.epsilon_xz,
sigma_yz: mu * strain.epsilon_yz,
}
}
// A simple material model for plasticity, capturing a one-dimensional case.
// This model tracks the accumulated plastic strain and uses a yield criterion and hardening modulus.
#[derive(Debug)]
struct PlasticMaterial {
young_modulus: f64,
yield_stress: f64, // Stress beyond which plastic deformation occurs.
hardening_modulus: f64, // Modulus describing how the material hardens after yielding.
plastic_strain: f64, // Accumulated plastic strain.
}
impl PlasticMaterial {
fn new(young_modulus: f64, yield_stress: f64, hardening_modulus: f64) -> Self {
PlasticMaterial {
young_modulus,
yield_stress,
hardening_modulus,
plastic_strain: 0.0,
}
}
}
/// Computes the one-dimensional stress using a simple plasticity model.
/// For demonstration, this function considers only the axial strain (epsilon_xx).
/// It computes a trial stress based on the elastic strain (total strain minus plastic strain)
/// and, if this exceeds the yield stress, adjusts the plastic strain accordingly.
fn compute_plastic_stress(strain: &StrainTensor, material: &mut PlasticMaterial) -> f64 {
// Consider axial strain only for simplicity.
let elastic_strain = strain.epsilon_xx - material.plastic_strain;
let trial_stress = material.young_modulus * elastic_strain;
if trial_stress.abs() > material.yield_stress {
// Calculate additional plastic strain required.
let plastic_increment = (trial_stress.abs() - material.yield_stress)
/ (material.young_modulus + material.hardening_modulus);
// Update the accumulated plastic strain, considering the sign of the trial stress.
material.plastic_strain += plastic_increment * trial_stress.signum();
// Compute the stress with hardening: σ = σ_yield + H * (plastic_increment)
trial_stress.signum() * (material.yield_stress + material.hardening_modulus * plastic_increment)
} else {
// If within elastic limits, return the trial stress.
trial_stress
}
}
fn main() {
// Example: Linear elastic behavior in 3D for a small strain state.
let strain_elastic = StrainTensor {
epsilon_xx: 0.0005,
epsilon_yy: -0.0002,
epsilon_zz: 0.0001,
epsilon_xy: 0.0003,
epsilon_xz: 0.0,
epsilon_yz: -0.0001,
};
let material = Material {
young_modulus: 210e9, // Young's modulus in Pascals (e.g., steel)
poisson_ratio: 0.3, // Typical for steel
};
let stress_elastic = compute_linear_elastic_stress(&strain_elastic, &material);
println!("Linear Elastic Stress Tensor:\n{:#?}", stress_elastic);
// Example: Plasticity in a 1D axial problem.
// For simplicity, we only consider the axial strain (epsilon_xx).
let strain_plastic = StrainTensor {
epsilon_xx: 0.002, // High strain to trigger yielding
epsilon_yy: 0.0,
epsilon_zz: 0.0,
epsilon_xy: 0.0,
epsilon_xz: 0.0,
epsilon_yz: 0.0,
};
// Define a plastic material with given parameters.
let mut plastic_material = PlasticMaterial::new(210e9, 250e6, 10e9);
let plastic_stress = compute_plastic_stress(&strain_plastic, &mut plastic_material);
println!("Computed Plastic Stress (1D axial): {:.6e} Pa", plastic_stress);
}
In this refined example, the code begins by defining the StrainTensor
and StressTensor
structs to hold 3D stress–strain components. The function compute_linear_elastic_stress
then implements Hooke’s law by computing Lamé’s parameters from the input Young’s modulus and Poisson’s ratio to determine the stress tensor for a linear elastic material. Next, a simple plasticity model is introduced through the PlasticMaterial
struct, which tracks yield stress, hardening, and accumulated plastic strain. The compute_plastic_stress
function computes a trial elastic stress, checks if it exceeds a yield criterion, and, if necessary, updates the plastic strain while computing the final stress value considering strain hardening. The main
function demonstrates the usage of both the linear elastic and plasticity models by computing and printing the resultant stress for given strain states.
This implementation shows how material models and constitutive laws from continuum mechanics can be robustly coded in Rust. With these fundamental building blocks, engineers and researchers are well-equipped to simulate the complex behavior of real-world materials and validate their models against experimental data, leveraging Rust's performance and safety for high-fidelity continuum mechanics simulations.
15.7. Boundary Conditions and Load Application
Boundary conditions and load application are essential aspects of continuum mechanics simulations because they define how a material interacts with its environment and how external forces are imparted to the system. The proper application of boundary conditions ensures that the simulation accurately reflects the intended physical constraints, while the correct application of loads is necessary to capture the material's response under realistic operating conditions. For example, in structural simulations, Dirichlet boundary conditions may be used to fix the displacement at certain nodes (i.e., clamping parts of a structure), whereas Neumann boundary conditions are used to impose forces or fluxes at the boundaries. In addition, distributed loads—such as those from wind pressure or traffic loads on a bridge—must be appropriately apportioned over the relevant nodes or elements to accurately simulate the overall effect on the structure.
Implementing these conditions in Rust involves writing functions that directly modify the displacement and force vectors of the simulation. For instance, applying a Dirichlet boundary condition can be as simple as iterating over a list of boundary node indices and setting their corresponding displacements to a prescribed value. Similarly, a distributed load can be applied by dividing the total load evenly among the nodes on which the load is to act. To validate these implementations, engineers often compare the simulation output to analytical benchmarks; for instance, in a cantilever beam analysis, ensuring that the predicted displacements and reaction forces match the expected theoretical results is critical.
Below are the Rust code examples that implement Dirichlet boundary conditions and distributed load applications, along with a simple demonstration in a main function.
// Importing nalgebra is not strictly necessary for these vector-based examples,
// but is commonly used in FEA simulations.
use nalgebra::{DVector};
/// Applies a Dirichlet boundary condition by setting the displacement of all specified boundary nodes
/// to a prescribed value. This function modifies the `displacement` vector in-place.
///
/// # Arguments
///
/// * `displacement` - A mutable vector of nodal displacements.
/// * `boundary_nodes` - A vector containing the indices of the nodes where the displacement is prescribed.
/// * `value` - The displacement value to set for all boundary nodes.
fn apply_dirichlet_boundary(displacement: &mut Vec<f64>, boundary_nodes: &Vec<usize>, value: f64) {
// Iterate over the provided node indices and set their displacements.
for &node in boundary_nodes.iter() {
displacement[node] = value;
}
}
/// Applies a distributed load by evenly distributing a total load across the specified nodes.
/// This function adds the load contribution to each node in the `force_vector`.
///
/// # Arguments
///
/// * `force_vector` - A mutable vector of nodal forces.
/// * `load_nodes` - A vector containing the indices of nodes where the load should be applied.
/// * `total_load` - The total load to be distributed among the specified nodes.
fn apply_distributed_load(force_vector: &mut Vec<f64>, load_nodes: &Vec<usize>, total_load: f64) {
// Calculate the load contribution per node.
let load_per_node = total_load / load_nodes.len() as f64;
// Add the load to each specified node in the force vector.
for &node in load_nodes.iter() {
force_vector[node] += load_per_node;
}
}
fn main() {
// Assume a simple simulation with 6 nodes. Typically, displacements and forces
// are managed for each degree of freedom (DOF), but for simplicity, we consider a single DOF per node.
let num_nodes = 6;
// Initialize a displacement vector representing the nodal displacements (in meters).
// Initially, all nodes have zero displacement.
let mut displacements = vec![0.0; num_nodes];
// Initialize a force vector representing the nodal forces (in Newtons).
// Initially, all nodes have zero applied force.
let mut force_vector = vec![0.0; num_nodes];
// Define boundary nodes where the displacement is fixed (e.g., nodes 0 and 5 are clamped).
let boundary_nodes = vec![0, 5];
// Apply Dirichlet boundary conditions: fix the displacement at nodes 0 and 5 to 0.
apply_dirichlet_boundary(&mut displacements, &boundary_nodes, 0.0);
// Assume a distributed load is applied along the span at nodes 2, 3, and 4.
let load_nodes = vec![2, 3, 4];
let total_load = 3000.0; // Total load in Newtons.
// Apply the distributed load across the specified nodes.
apply_distributed_load(&mut force_vector, &load_nodes, total_load);
// For demonstration, print the updated displacement and force vectors.
println!("Displacement vector after applying Dirichlet boundary conditions: {:?}", displacements);
println!("Force vector after applying distributed load: {:?}", force_vector);
// In a full FEA simulation, the displacement and force vectors are used to form and solve
// the system of equations representing the structure. The results would then be validated and visualized.
}
In this implementation, the apply_dirichlet_boundary
function ensures that the displacements at the designated boundary nodes are fixed to the specified value (here, zero). The apply_distributed_load
function takes a total load, divides it equally among the provided nodes, and adds it to the corresponding entries in the force vector. These functions serve as the fundamental building blocks for applying boundary conditions and loads in continuum mechanics simulations.
After setting up the vectors, the main function demonstrates their usage by initializing a simple model with six nodes, applying fixed displacement at nodes 0 and 5, and distributing a load across nodes 2, 3, and 4. The updated vectors are printed, offering a basic validation of the implementation. In an actual FEA simulation, these steps would be integrated into the solution procedure for the governing equations, and additional validation would be performed by comparing computed displacements and reaction forces with theoretical or experimental benchmarks.
This approach highlights Rust's capability to handle complex boundary conditions and load applications safely and efficiently while ensuring that the simulation accurately reflects the physical scenario under consideration.
15.8. Post-Processing and Visualization
Post-processing and visualization are critical components of continuum mechanics simulations, allowing us to extract, interpret, and present the results in a meaningful way. This section explores the fundamental concepts of extracting results, computing derived quantities, and the importance of visual representation. The conceptual ideas cover data interpretation, graphical representation, and comparison with theoretical solutions. Finally, the practical implementation focuses on using Rust libraries for visualization, creating plots and graphs, and validating results, with sample codes provided to illustrate these techniques.
Fundamental Concepts begin with the process of extracting results from simulations. After running a simulation, the raw data typically consists of field variables like displacement, stress, or strain at various points in the domain. Extracting these results involves pulling the relevant data from the simulation output in a format that can be further analyzed or visualized. This step is crucial because it allows us to focus on specific quantities of interest and perform additional calculations if needed.
Computing derived quantities is often necessary to gain deeper insights into the material behavior. For instance, in stress analysis, von Mises stress is a derived quantity that provides a scalar measure of stress, which is useful for assessing the potential for yielding in materials. The calculation of von Mises stress from the stress tensor components involves the following formula:
$$ \sigma_{vM} = \sqrt{\frac{1}{2} \left[ (\sigma_{xx} - \sigma_{yy})^2 + (\sigma_{yy} - \sigma_{zz})^2 + (\sigma_{zz} - \sigma_{xx})^2 + 6(\sigma_{xy}^2 + \sigma_{yz}^2 + \sigma_{zx}^2) \right]} $$
This equation takes the components of the stress tensor and combines them to produce a single value that is easier to interpret in the context of material failure.
Visual representation is the final step in post-processing, where the extracted and derived data is presented in a clear and accurate manner. Visualization techniques like contour plots, vector fields, and 3D surface plots are essential for interpreting the results of complex simulations. Effective visual representation not only aids in understanding the simulation outcomes but also helps in communicating these results to others.
Conceptual Ideas focus on data interpretation, which involves understanding what the simulation results mean in a physical context. For example, a high von Mises stress value in a particular region of a component might indicate a risk of yielding, while a displacement field might reveal how a structure deforms under load. Interpreting these results correctly is essential for making informed decisions based on the simulation.
Graphical representation of data is another crucial aspect, as it allows the results to be communicated effectively. Using plots, graphs, and other visual tools, we can highlight key aspects of the simulation, such as stress concentrations, deformation patterns, or the effects of different loading conditions. These visualizations make complex data more accessible and easier to analyze.
Comparison with theoretical solutions is an important part of validating simulation results. By comparing the outputs of the simulation with known analytical solutions or experimental data, we can assess the accuracy of the simulation and identify any discrepancies. This step is essential for ensuring that the simulation is reliable and that the results can be trusted.
The following Rust code demonstrates a practical implementation of stress and strain computations for a linear elastic material in 3D. It defines data structures for stress and strain tensors, implements a function to compute the stress tensor using Hooke’s law for an isotropic material, and includes a function to calculate von Mises stress. Additionally, a simple plotting function using the plotters crate is presented to visualize a hypothetical distribution of von Mises stress across a structure.
// Cargo.toml dependencies for the following code:
// [dependencies]
// nalgebra = "0.30"
// plotters = "0.3"
/// A 3D strain tensor representing normal strains (ε_xx, ε_yy, ε_zz) and shear strains (ε_xy, ε_xz, ε_yz).
#[derive(Debug)]
struct StrainTensor {
epsilon_xx: f64,
epsilon_yy: f64,
epsilon_zz: f64,
epsilon_xy: f64,
epsilon_xz: f64,
epsilon_yz: f64,
}
/// A 3D stress tensor representing normal stresses (σ_xx, σ_yy, σ_zz) and shear stresses (σ_xy, σ_xz, σ_yz).
#[derive(Debug, Default)]
struct StressTensor {
sigma_xx: f64,
sigma_yy: f64,
sigma_zz: f64,
sigma_xy: f64,
sigma_xz: f64,
sigma_yz: f64,
}
/// Computes the 3D stress tensor from a given strain tensor for an isotropic, linear elastic material
/// using Hooke’s law. Material properties are defined by Young's modulus and Poisson's ratio. The Lamé parameters
/// are computed as follows:
/// λ = (νE)/[(1+ν)(1-2ν)]
/// μ = E/[2(1+ν)]
/// The stress components are then calculated by incorporating the trace of the strain tensor.
fn compute_linear_elastic_stress(strain: &StrainTensor, young_modulus: f64, poisson_ratio: f64) -> StressTensor {
let lambda = poisson_ratio * young_modulus / ((1.0 + poisson_ratio) * (1.0 - 2.0 * poisson_ratio));
let mu = young_modulus / (2.0 * (1.0 + poisson_ratio));
// Calculate the trace of the strain tensor.
let trace = strain.epsilon_xx + strain.epsilon_yy + strain.epsilon_zz;
StressTensor {
sigma_xx: lambda * trace + 2.0 * mu * strain.epsilon_xx,
sigma_yy: lambda * trace + 2.0 * mu * strain.epsilon_yy,
sigma_zz: lambda * trace + 2.0 * mu * strain.epsilon_zz,
sigma_xy: mu * strain.epsilon_xy,
sigma_xz: mu * strain.epsilon_xz,
sigma_yz: mu * strain.epsilon_yz,
}
}
/// Computes the von Mises stress from a given 3D stress tensor. This scalar value is commonly used
/// to assess the yield criterion in ductile materials, consolidating the normal and shear stress components.
fn compute_von_mises(stress: &StressTensor) -> f64 {
// Extract stress tensor components.
let sigma_xx = stress.sigma_xx;
let sigma_yy = stress.sigma_yy;
let sigma_zz = stress.sigma_zz;
let sigma_xy = stress.sigma_xy;
let sigma_xz = stress.sigma_xz;
let sigma_yz = stress.sigma_yz;
// Compute the von Mises stress using the formula:
// σ_vM = sqrt(0.5 * [ (σ_xx - σ_yy)^2 + (σ_yy - σ_zz)^2 + (σ_zz - σ_xx)^2 + 6(σ_xy^2 + σ_yz^2 + σ_zx^2) ])
((sigma_xx - sigma_yy).powi(2)
+ (sigma_yy - sigma_zz).powi(2)
+ (sigma_zz - sigma_xx).powi(2)
+ 6.0 * (sigma_xy.powi(2) + sigma_yz.powi(2) + sigma_xz.powi(2)))
.sqrt() / (2.0_f64).sqrt()
}
use plotters::prelude::*;
use std::error::Error;
/// Plots a simple line graph of a vector of von Mises stress values using the plotters crate.
/// This function creates a PNG file showing the distribution of von Mises stress along a structure.
fn plot_von_mises_distribution(von_mises_values: &Vec<f64>, output_file: &str) -> Result<(), Box<dyn Error>> {
// Create a drawing area with specified size.
let root = BitMapBackend::new(output_file, (800, 600)).into_drawing_area();
root.fill(&WHITE)?;
let x_range = 0..von_mises_values.len();
let y_max = von_mises_values.iter().cloned().fold(0./0., f64::max); // Maximum von Mises value for y-axis limit
let mut chart = ChartBuilder::on(&root)
.caption("Von Mises Stress Distribution", ("sans-serif", 40).into_font())
.margin(20)
.x_label_area_size(40)
.y_label_area_size(40)
.build_cartesian_2d(x_range, 0.0..y_max)?;
chart.configure_mesh().draw()?;
// Plot the von Mises stress values as a connected line series.
chart.draw_series(LineSeries::new(
(0..von_mises_values.len()).map(|i| (i, von_mises_values[i])),
&RED,
))?;
root.present()?;
Ok(())
}
fn main() -> Result<(), Box<dyn Error>> {
// Example: Compute stress from a given strain state.
let strain_example = StrainTensor {
epsilon_xx: 0.0005,
epsilon_yy: -0.0002,
epsilon_zz: 0.0001,
epsilon_xy: 0.0003,
epsilon_xz: 0.0,
epsilon_yz: -0.0001,
};
let young_modulus = 210e9; // Example: steel, in Pascals.
let poisson_ratio = 0.3; // Typical value for steel.
// Compute the stress tensor from the strain state.
let stress = compute_linear_elastic_stress(&strain_example, young_modulus, poisson_ratio);
println!("Computed Stress Tensor:\n{:#?}", stress);
// Compute the von Mises stress from the stress tensor.
let von_mises = compute_von_mises(&stress);
println!("Von Mises Stress: {:.6e} Pa", von_mises);
// Example: Create a sample vector of von Mises stress values (e.g., over several elements or nodes).
let von_mises_values = vec![100e6, 110e6, 120e6, 115e6, 130e6, 125e6, 140e6];
// Plot the von Mises stress distribution and save it as a PNG image.
plot_von_mises_distribution(&von_mises_values, "von_mises_distribution.png")?;
println!("The von Mises stress distribution has been saved to 'von_mises_distribution.png'.");
Ok(())
}
In this refined example, the code begins by defining data structures for the 3D strain and stress tensors. The compute_linear_elastic_stress
function computes the stress tensor using Hooke’s law for an isotropic material, with the Lamé parameters calculated from Young’s modulus and Poisson’s ratio. The compute_von_mises
function then processes the stress tensor components to yield a scalar von Mises stress value.
The second part of the code demonstrates how to visualize the distribution of von Mises stress using the plotters crate. The plot_von_mises_distribution
function constructs a Cartesian plot from the vector of von Mises values and saves the resulting chart as a PNG image. Finally, the main
function ties these pieces together by computing stress from a sample strain state, calculating the von Mises stress, and plotting a sample distribution.
Validation of results is essential to ensure the accuracy and reliability of the simulation. This can involve comparing the computed von Mises stress or other derived quantities against known theoretical solutions or experimental data. For example, if simulating a simple tensile test, the von Mises stress distribution should match the expected linear distribution along the length of the specimen. If discrepancies are found, it may indicate an issue with the simulation setup, boundary conditions, or material model.
15.9. Validation and Verification of Simulations
Validation and verification (V&V) are critical processes in computational simulations that ensure numerical results are accurate, reliable, and physically meaningful. In continuum mechanics simulations, these processes involve comparing simulation outputs with analytical solutions, experimental data, or benchmark problems, and performing convergence studies to assess how refinements in spatial or temporal discretization lead to more accurate results. Validation confirms that the simulation model is a true representation of the real-world system, while verification tests the correctness of the numerical implementation. Error analysis, including assessing discretization and round-off errors, and benchmarking against standard problems, provides insight into the accuracy and performance of the simulation. For instance, in a simulation of the one-dimensional heat equation, comparing the numerical solution to an analytical solution over a range of grid resolutions can help determine if the numerical method is converging. Similarly, when evaluating stress distributions in structural analysis, comparing the computed stress with the theoretical distribution for a simple case, such as a cantilever beam under load, verifies the model's correctness.
In Rust, these processes are implemented by coding functions that compute analytical solutions as references, calculate error metrics, and compare results against numerical outputs. The code below first defines an analytical solution for the 1D heat equation and computes an average error between the numerical and analytical solutions. Next, it provides a function for comparing stress distributions by computing the maximum difference between numerical and analytical stress vectors. Finally, a function to refine simulation resolution is shown as a demonstration of how one might adjust simulation parameters to improve accuracy.
// Cargo.toml dependencies:
// [dependencies]
// nalgebra = "0.30" // For vector and matrix operations if needed.
/// Computes the analytical solution for the 1D heat equation
/// given a spatial location x, time t, and thermal diffusivity alpha.
/// The solution used here is: u(x,t) = exp(-alpha * π² * t) * sin(π * x),
/// which is a known solution for specific initial and boundary conditions.
fn analytical_solution_heat_eq(x: f64, t: f64, alpha: f64) -> f64 {
// Use the standard library's exponential and sine functions.
(-(alpha * std::f64::consts::PI.powi(2) * t)).exp() * (std::f64::consts::PI * x).sin()
}
/// Compares a numerical solution to the analytical solution for the heat equation.
/// The function computes the average absolute error over the domain, which indicates
/// the degree of accuracy of the numerical method.
fn verify_heat_equation_solution(numerical_solution: &Vec<f64>, alpha: f64, t: f64, dx: f64) -> f64 {
let mut total_error = 0.0;
for (i, &u_num) in numerical_solution.iter().enumerate() {
let x = i as f64 * dx;
let u_analytical = analytical_solution_heat_eq(x, t, alpha);
total_error += (u_num - u_analytical).abs();
}
// Return the average error across the domain.
total_error / numerical_solution.len() as f64
}
/// Compares numerical and analytical stress distributions by computing the maximum absolute difference
/// between corresponding stress values. This metric serves as an indicator of the accuracy of the simulation.
fn compare_stress_distribution(numerical_stress: &Vec<f64>, analytical_stress: &Vec<f64>) -> f64 {
let mut max_diff = 0.0;
for (&num, &anal) in numerical_stress.iter().zip(analytical_stress.iter()) {
let diff = (num - anal).abs();
if diff > max_diff {
max_diff = diff;
}
}
max_diff
}
/// Represents a function that refines simulation resolution by reducing the time step and spatial resolution.
/// In practice, this function would re-run the simulation with refined parameters.
/// Here, it demonstrates the concept by printing the refined parameters.
fn refine_simulation_resolution(time_step: f64, spatial_resolution: f64) {
// Halve the time step and spatial resolution for improved accuracy.
let new_time_step = time_step / 2.0;
let new_spatial_resolution = spatial_resolution / 2.0;
println!("Refined time step: {}", new_time_step);
println!("Refined spatial resolution: {}", new_spatial_resolution);
// In a full application, the simulation would be re-run using these refined parameters.
}
fn main() {
// Example 1: Verify the 1D heat equation solution.
// Setup simulation parameters.
let alpha = 0.01; // Thermal diffusivity (m^2/s)
let t = 0.1; // Time at which to evaluate (seconds)
let dx = 0.01; // Spatial step size (m)
// Generate a hypothetical numerical solution for the heat equation.
// For demonstration purposes, we simulate a numerical solution by computing the analytical solution.
// In a real simulation, this vector would be the result of a numerical method.
let n_points = 101;
let mut numerical_solution: Vec<f64> = Vec::with_capacity(n_points);
for i in 0..n_points {
let x = i as f64 * dx;
numerical_solution.push(analytical_solution_heat_eq(x, t, alpha));
}
// Verify the numerical solution; expect a near-zero error since we're using the analytical solution.
let avg_error = verify_heat_equation_solution(&numerical_solution, alpha, t, dx);
println!("Average error in heat equation solution: {:.6e}", avg_error);
// Example 2: Compare stress distributions.
// Assume we have numerical and analytical stress distributions as vectors.
let numerical_stress = vec![150.0, 155.0, 160.0, 158.0, 162.0]; // Example numerical results (in MPa)
let analytical_stress = vec![150.0, 156.0, 159.0, 157.0, 163.0]; // Example analytical results (in MPa)
let max_stress_diff = compare_stress_distribution(&numerical_stress, &analytical_stress);
println!("Maximum difference in stress distribution: {:.6e} MPa", max_stress_diff);
// Example 3: Refine simulation resolution.
let time_step = 0.005; // Original time step (seconds)
let spatial_resolution = 0.02; // Original spatial resolution (meters)
refine_simulation_resolution(time_step, spatial_resolution);
// In a full simulation, one would re-run the simulation with the new parameters and then perform additional verification.
}
In the code above, the analytical_solution_heat_eq
function provides an analytical solution to the 1D heat equation for given spatial and temporal inputs, while verify_heat_equation_solution
computes the average absolute error between the numerical and analytical solutions. The compare_stress_distribution
function determines the maximum absolute difference between numerical and analytical stress values. Finally, refine_simulation_resolution
demonstrates how simulation parameters may be refined to improve accuracy, printing the refined values. The main function ties these processes together to illustrate a complete workflow for validation and verification in continuum mechanics simulations.
This section underscores the importance of validation and verification. By comparing numerical results with analytical solutions, performing convergence studies through refinement, and benchmarking against known standards, simulation accuracy can be ensured. Rust’s performance and safety features, along with its support for robust numerical computations and parallel processing, make it an excellent choice for developing high-fidelity continuum mechanics simulations.
15.10. Advanced Topics and Future Directions
Advanced continuum mechanics simulations push the boundaries of what can be modeled by addressing phenomena that span multiple length and time scales, coupling different physical behaviors, and employing cutting-edge numerical algorithms. Multi-scale modeling, for instance, integrates coarse-scale models that capture the overall behavior of a structure with fine-scale models that resolve detailed local phenomena. Such an approach is essential when microstructural features or localized defects significantly affect the global response of a material. In addition to multi-scale modeling, coupling continuum mechanics with other physics—such as fluid-structure interaction—enables the simulation of complex systems where different domains interact. Advanced algorithms like adaptive mesh refinement (AMR) dynamically adjust spatial resolution in regions of interest and machine learning methods are increasingly applied to predict complex material behavior and optimize simulation parameters. These approaches are essential for enhancing accuracy while managing computational cost. Rust’s zero-cost abstractions, strong memory safety, and excellent concurrency support make it especially suitable for implementing these advanced techniques. For example, one can implement a multi-scale simulation framework in Rust by defining a coarse model to describe global system behavior and a fine model to capture local details, with mechanisms for passing information between the two scales. Moreover, Rust’s interoperability with languages like Python—using crates such as pyo3—further extends its utility by combining the performance of Rust with the rich pre- and post-processing ecosystems available in other languages.
Below is a Rust code example that illustrates an elementary multi-scale simulation framework. In this example, the CoarseModel
structure represents the overall behavior of a material, while the FineModel
structure models detailed behavior in a specific region. The code simulates the updating of the coarse model based on global loads and then passes relevant information to the fine model for local refinement. Afterwards, the refined local information is passed back to update the global model. This basic framework can be expanded for more complex multi-scale and coupled simulations.
// No external dependencies are needed for this minimal multi-scale example.
use std::fmt;
/// The CoarseModel struct represents the large-scale properties of a material,
/// such as global stress and global strain.
#[derive(Debug)]
struct CoarseModel {
global_stress: f64,
global_strain: f64,
}
impl CoarseModel {
/// Updates the coarse model with new load or boundary condition information.
/// For demonstration purposes, the global stress and strain are simply incremented.
fn update(&mut self) {
// Update global parameters based on external conditions.
self.global_stress += 0.01;
self.global_strain += 0.005;
}
/// Passes information from the coarse model to create a fine-scale model,
/// amplifying the relevant variables for localized analysis.
fn pass_to_fine(&self) -> FineModel {
FineModel {
local_stress: self.global_stress * 1.5,
local_strain: self.global_strain * 1.2,
region_of_interest: (10, 20),
}
}
}
/// The FineModel struct represents the detailed, local behavior in a region of interest.
/// It contains refined measurements of stress and strain and specifies the region where the detail applies.
#[derive(Debug)]
struct FineModel {
local_stress: f64,
local_strain: f64,
region_of_interest: (usize, usize), // Defines the element range or node indices of the region.
}
impl FineModel {
/// Refines the local model by adjusting stress and strain values.
/// This might represent additional local dynamics or effects not captured in the coarse model.
fn refine(&mut self) {
// Increase values to simulate refined analysis.
self.local_stress *= 1.1;
self.local_strain *= 1.05;
}
/// Passes information from the fine model back to update the coarse model.
/// Typically, this step would involve averaging or otherwise reconciling detailed local behavior with the global model.
fn pass_to_coarse(&self, coarse_model: &mut CoarseModel) {
// Update coarse values using refined local results.
coarse_model.global_stress = self.local_stress * 0.9;
coarse_model.global_strain = self.local_strain * 0.95;
}
}
/// Runs a simple multi-scale simulation where the coarse model is updated,
/// then passes details to a fine model that refines the result,
/// and finally updates the coarse model with the refined data.
fn run_multiscale_simulation() {
let mut coarse_model = CoarseModel {
global_stress: 100.0,
global_strain: 0.02,
};
// Update the coarse model based on external loads or conditions.
coarse_model.update();
// Pass the current state to a fine-scale model for a specific region.
let mut fine_model = coarse_model.pass_to_fine();
// Refine the local model with higher resolution or more detailed physics.
fine_model.refine();
// Update the coarse model with the refined local data.
fine_model.pass_to_coarse(&mut coarse_model);
println!("Updated Coarse Model: {:#?}", coarse_model);
}
fn main() {
// Run the multi-scale simulation example.
run_multiscale_simulation();
// Example of integrating with another language using pyo3 for Python interoperability.
// This allows using Rust for intensive computations while leveraging Python for data handling and visualization.
// The following code creates a simple Python module from Rust using the pyo3 crate.
//
// To compile this module, add pyo3 as a dependency in Cargo.toml and follow the pyo3 setup instructions.
//
// [dependencies]
// pyo3 = { version = "0.18", features = ["extension-module"] }
//
// The code below is commented out for standalone Rust builds but can be enabled for Python integration.
/*
use pyo3::prelude::*;
/// This function doubles each element in the input vector.
/// It serves as a simple simulation routine that can be called from Python.
#[pyfunction]
fn simulate_rust(input_data: Vec<f64>) -> Vec<f64> {
input_data.iter().map(|x| x * 2.0).collect()
}
/// Define a Python module named `rust_simulation` containing the `simulate_rust` function.
#[pymodule]
fn rust_simulation(py: Python, m: &PyModule) -> PyResult<()> {
m.add_function(wrap_pyfunction!(simulate_rust, m)?)?;
Ok(())
}
*/
}
In this final example, the code begins by defining two models: a CoarseModel that captures large-scale material behavior and a FineModel for detailed local behavior. The coarse model is updated with global values, and then information is passed to the fine model, which refines these values. The refined results are then fed back into the coarse model, simulating a multi-scale exchange. Inline comments explain each step for clarity.
Additionally, an optional integration example using the pyo3 crate is provided. This integration enables the Rust simulation code to be called from Python, combining Rust’s performance with Python’s extensive data processing and visualization capabilities. The pyo3 integration code is commented out, as it requires additional build configuration.
This section demonstrates advanced topics in continuum mechanics simulations by presenting a flexible multi-scale framework and potential strategies for interfacing with other computational tools. Rust’s strengths in performance, safety, and concurrency make it a promising language for these high-fidelity, advanced simulations, ensuring the ability to tackle complex engineering problems while remaining reliable and scalable.
15.11. Conclusion
Chapter 15 provides a robust foundation for implementing continuum mechanics simulations in Rust, encompassing a wide range of fundamental and advanced topics. By engaging with the detailed exercises and concepts presented, readers will develop a deep understanding of continuum mechanics and acquire practical skills for solving real-world problems in computational physics.
15.11.1. Further Learning with GenAI
These prompts are designed to provide a deep, technical understanding of Continuum Mechanics Simulations using Rust. They focus on complex aspects of the subject, requiring detailed exploration of theoretical concepts, mathematical formulations, and practical implementations.
Discuss the derivation of the governing equations in continuum mechanics, including the Cauchy equations and Navier-Cauchy equations. How do these fundamental equations of motion, derived from conservation principles, translate into discretized forms for Finite Element Method (FEM) applications? Discuss the assumptions (e.g., small deformations, linear elasticity) and the key considerations in implementing these equations in Rust, including performance, accuracy, and computational cost.
Explain the process of transforming a continuum mechanics problem into a finite element problem. This involves selecting appropriate interpolation functions and shape functions for the discretization of the domain. How are shape functions used to approximate field variables (e.g., displacement, stress) within each element? Discuss how you would implement this transformation in Rust and how choices such as the element type (e.g., linear, quadratic) and mesh density impact performance and accuracy.
Provide a detailed explanation of the formulation and numerical stability of the global stiffness matrix in FEM. Describe the process of deriving the element stiffness matrices and assembling them into the global matrix. How are large-scale problems handled in Rust, particularly in terms of memory management and computational efficiency? Discuss strategies for optimizing the matrix assembly process and ensuring numerical stability.
Elaborate on the methods for applying and implementing various boundary conditions in FEM simulations. How are Dirichlet, Neumann, and mixed boundary conditions implemented in Rust, and what are the key challenges in handling them? Discuss how boundary conditions influence the numerical solution, including strategies for managing complex and dynamic boundary conditions in Rust.
Compare and contrast linear and nonlinear constitutive models in continuum mechanics. How do models like hyperelasticity and plasticity differ in their formulation, and what are the challenges in implementing these nonlinear models in Rust? Discuss methods for handling nonlinearities (e.g., iterative solvers) and convergence issues, including advanced material models that account for large deformations and complex material behavior.
Analyze the methods for time integration in dynamic simulations, including explicit and implicit schemes. Discuss the trade-offs between explicit methods (e.g., time-step size constraints) and implicit methods (e.g., computational cost) in terms of stability and accuracy. Provide a detailed explanation of how these methods can be implemented in Rust, with performance considerations for complex dynamic problems.
Discuss the process of calculating and interpreting stress and strain tensors in continuum mechanics. How are these tensors derived from the displacement field, and how are they used to assess material behavior? Provide a detailed implementation in Rust, focusing on tensor operations, numerical stability, and computational efficiency for large-scale problems.
Explain the challenges of implementing advanced material models in Rust, such as viscoelasticity, damage mechanics, and rate-dependent plasticity. What are the specific computational difficulties (e.g., handling time-dependent behavior or damage accumulation), and how can these challenges be addressed in Rust? Discuss strategies for ensuring simulation stability and accuracy.
Describe the process of generating and refining meshes in FEM simulations. How do you handle complex geometries and adaptive meshing techniques in Rust? Discuss tools and libraries (e.g., Tetgen, mesh generation tools) that facilitate these tasks and their integration with Rust for efficient and scalable simulations.
Discuss the strategies for solving large systems of linear equations arising from FEM simulations, such as using direct solvers (e.g., LU decomposition) and iterative solvers (e.g., Conjugate Gradient, GMRES). How do you implement and optimize these solvers in Rust, and what performance considerations (e.g., sparse matrix handling, parallelization) are critical for large-scale simulations?
Elaborate on the techniques for validating and verifying FEM simulations, including error estimation, mesh refinement studies, and benchmarking against analytical solutions. How do you implement these techniques in Rust, and what tools are used to compare numerical results with known solutions or experimental data?
Analyze the implementation of various load types in FEM simulations, such as point loads, distributed loads, and varying boundary conditions. How do you apply these loads in Rust, and what are the key considerations for handling dynamic and complex load cases efficiently in terms of both code structure and computational performance?
Discuss advanced post-processing techniques in FEM simulations, such as extracting simulation results for visualization and analysis. What tools or libraries are available in Rust to visualize stress distributions, deformed shapes, and other simulation outputs? Discuss strategies for integrating Rust with external visualization software like ParaView or VTK.
Provide an in-depth analysis of handling nonlinearities and large deformations in continuum mechanics simulations. How do you implement techniques like Newton-Raphson solvers in Rust to handle non-linearities? Discuss the challenges involved in ensuring convergence and stability when dealing with large deformations and complex material behavior.
Explain the principles and implementation of multi-scale modeling in continuum mechanics. How do you integrate different scales (e.g., micro, meso, and macro) within a unified simulation framework? What are the computational and conceptual challenges associated with multi-scale models, and how can Rust’s performance features be leveraged to handle them?
Describe the role and implementation of tensor algebra in continuum mechanics simulations. How do you efficiently perform tensor operations in Rust, particularly for large-scale problems involving stress and strain calculations? Discuss libraries or custom implementations that support these operations and ensure both accuracy and performance.
Discuss advanced algorithms and techniques used in continuum mechanics simulations, such as adaptive meshing, parallel computing, and optimization algorithms. How can these techniques be implemented in Rust, and what are the trade-offs between computational cost, complexity, and performance? Provide examples where these techniques improve simulation accuracy or efficiency.
Explain how to handle complex boundary conditions and geometries in FEM problems. What strategies and tools are available in Rust to manage intricate boundary conditions and geometric complexities, such as curved surfaces or contact problems? How do these complexities impact the simulation results, and what best practices should be followed?
Analyze the integration of continuum mechanics simulations with external computational tools or libraries. How does Rust interface with established libraries like PETSc or Eigen to enhance simulation capabilities? Discuss the best practices for achieving seamless integration between Rust-based simulations and external numerical libraries.
Discuss emerging trends and future directions in continuum mechanics simulations, including advancements in algorithms, materials modeling, and computational techniques. How can Rust contribute to these developments, and what research areas (e.g., real-time simulations, machine learning-assisted FEM) show the most promise for Rust-based implementations?
By exploring these complex topics, you'll develop a robust understanding of both theoretical and practical aspects of continuum mechanics, positioning yourself at the forefront of computational physics. Embrace the challenges, push the boundaries of your knowledge, and leverage your skills to solve intricate problems and drive innovation.
15.11.2. Assignments for Practice
These advanced exercises are designed to push the boundaries of your skills in continuum mechanics simulations using Rust. They involve tackling complex problems, from nonlinear FEM implementations to sophisticated time integration methods and advanced mesh generation techniques.
Exercise 15.1: Comprehensive FEM Equation Derivation and Implementation
Task: Derive the complete set of governing equations for a nonlinear continuum mechanics problem, such as large deformation elasticity or viscoelasticity. Discretize these equations using the Finite Element Method (FEM) and implement them in Rust. Include the derivation of nonlinear element stiffness matrices and the assembly of a global stiffness matrix. Address nonlinear solver techniques, such as Newton-Raphson iterations, and document the performance and stability of your implementation.
Exercise 15.2: Complex Boundary Conditions with Adaptive Strategies
Task: Implement a finite element simulation in Rust that handles complex boundary conditions, including mixed Dirichlet-Neumann conditions and time-varying boundary conditions. Develop an adaptive boundary condition strategy for a dynamic problem with evolving constraints. Analyze the impact of these boundary conditions on the simulation accuracy and convergence. Compare the results with standard boundary condition implementations and discuss the advantages and limitations of your adaptive approach.
Exercise 15.3: Advanced Dynamic Time Integration and Stability Analysis
Task: Implement and compare several advanced time-stepping methods for dynamic simulations in Rust, including implicit Newmark-beta methods, explicit central-difference methods, and symplectic integrators. Evaluate their stability and accuracy for complex dynamic problems, such as nonlinear oscillators or multi-body systems. Perform a detailed stability analysis and error estimation for each method. Document your findings and suggest best practices for choosing appropriate time integration schemes for different problem types.
Exercise 15.4: Stress and Strain Computation with Complex Material Models
Task: Implement algorithms for calculating stress and strain tensors for complex material models such as hyperelasticity (e.g., Neo-Hookean or Ogden models) and plasticity (e.g., Von Mises yield criterion with hardening). Use Rust to create test problems with known solutions to validate your implementations. Compare the performance and accuracy of different material models in various loading scenarios. Discuss the numerical challenges associated with these models and propose solutions to improve accuracy and efficiency.
Exercise 15.5: Advanced Mesh Generation, Refinement, and Quality Control
Task: Develop a Rust-based tool for automated mesh generation and refinement for complex 3D geometries, incorporating advanced techniques such as mesh smoothing, element quality control, and adaptive refinement based on error estimates. Apply this tool to a challenging continuum mechanics problem with complex boundaries and varying material properties. Evaluate the impact of mesh quality and refinement on simulation accuracy and computational performance. Discuss strategies for optimizing mesh generation and refinement processes and their influence on overall simulation results.
Each challenge is an opportunity to advance your skills and contribute to cutting-edge research and practical applications in computational physics. Embrace the difficulty and push through the complexities—your perseverance and expertise will pave the way for innovative solutions and excellence in the field.
Comments