Chapter 12
Simulating Rigid Body Dynamics
"In physics, as in all science, we are looking for the simplest possible explanations of complex phenomena." — Steven Weinberg
Chapter 12 of CPVR delves into the simulation of rigid body dynamics, providing a comprehensive framework for understanding and implementing these simulations using Rust. The chapter begins by introducing the fundamental principles of rigid body dynamics, covering both translational and rotational motion. It then explores the mathematical tools, including Euler’s equations and quaternion representation, necessary for accurately modeling rigid body behavior. The chapter also addresses various numerical methods for integrating these equations, ensuring stability and accuracy in simulations. Practical implementation in Rust is emphasized, with detailed discussions on data structures, algorithm design, and optimization techniques. Case studies demonstrate the application of these concepts to both simple and complex systems, while advanced topics like constraints, friction, and soft body dynamics are explored to provide a deeper understanding of the field. The chapter concludes with performance considerations and visualization techniques, ensuring that readers are equipped to create efficient, real-time simulations in Rust.
12.1. Introduction to Rigid Body Dynamics
Rigid body dynamics is a crucial area of study in computational physics, particularly in simulations where the deformation of objects can be neglected. A rigid body is defined as an object that does not deform under the influence of forces; this means that the distances between any two points within the body remain constant over time. This simplification allows us to focus on the translational and rotational motion of the body as a whole, rather than considering the internal stresses and strains that would be necessary for deformable bodies.
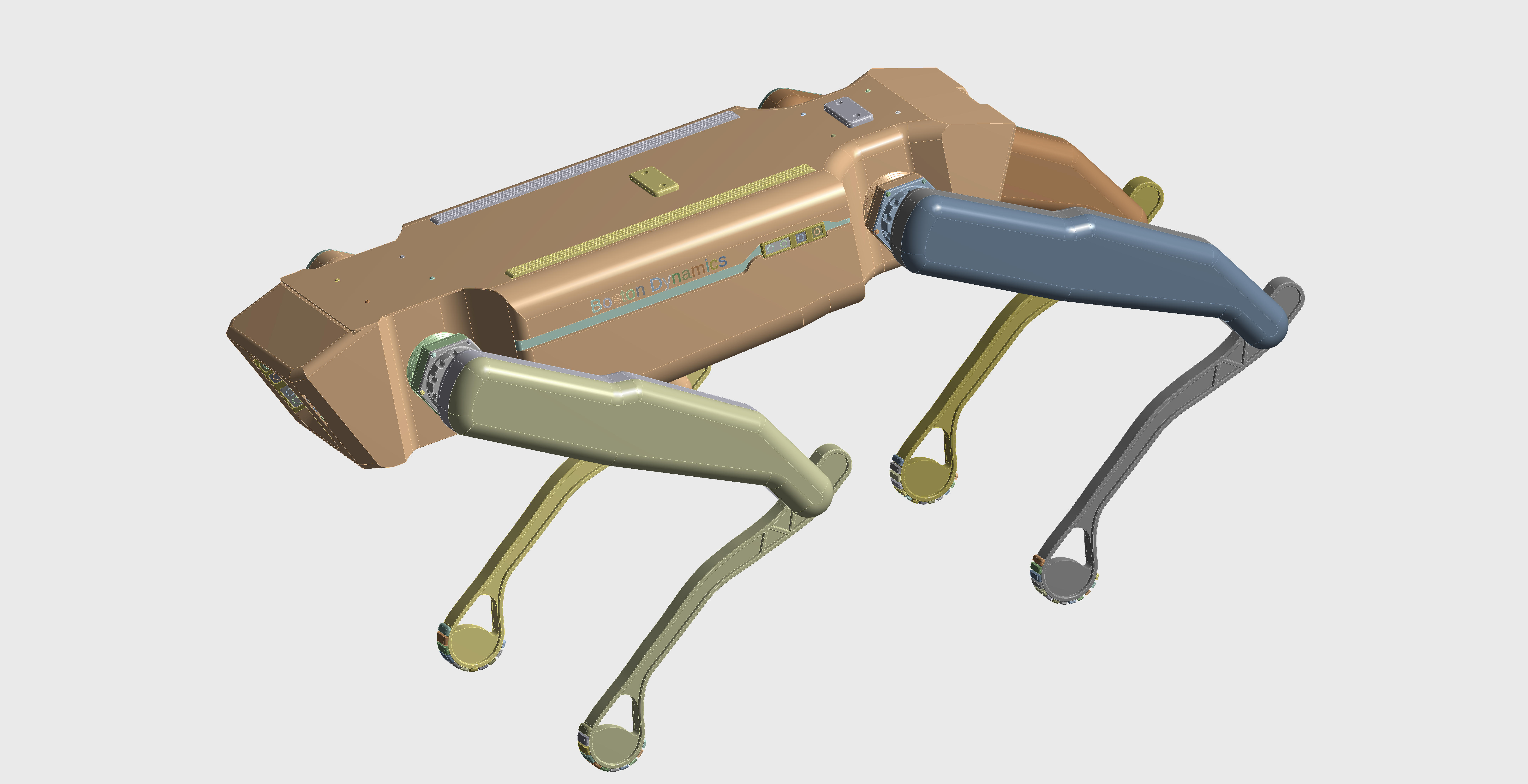
Illustration of rigid body dynamic of animal robot.
A rigid body in three-dimensional space has six degrees of freedom: three for translation along the x, y, and z axes, and three for rotation about these axes. These degrees of freedom are governed by Newton's laws of motion. For rigid bodies, Newton's first law states that a body will remain in a state of uniform motion unless acted upon by an external force or torque. The second law relates the force acting on the body to its acceleration, while the third law asserts that for every action, there is an equal and opposite reaction. These laws are foundational to understanding how forces and torques affect the motion of rigid bodies.
In understanding rigid body dynamics, it is essential to distinguish it from particle dynamics. While particles can only translate (as they have no dimensions), rigid bodies can both translate and rotate. This introduces additional complexity, as one must consider not just the linear motion of the body's center of mass but also its rotational motion about the center of mass.
Key physical quantities in rigid body dynamics include the position and orientation of the body, its linear and angular velocity, its mass, and its moment of inertia. The position and orientation describe where the body is in space and how it is oriented. Linear velocity describes the rate of change of the body's position, while angular velocity describes the rate of change of its orientation. Mass is a measure of the body's inertia to translational motion, while the moment of inertia is a measure of its resistance to rotational motion. The moment of inertia depends on the mass distribution relative to the axis of rotation. The concept of the center of mass is also vital; it is the point at which the entire mass of the body can be considered to be concentrated for the purposes of analyzing translational motion.
Simulating rigid body dynamics involves solving the equations of motion derived from Newton's laws for both translational and rotational motion. These equations can be complex, especially when considering the rotational aspects, but Rust provides robust tools and libraries that can help manage this complexity.
To begin, let's consider the translational motion of a rigid body. The equation governing translational motion is derived from Newton's second law:
$$\mathbf{F} = m \mathbf{a}$$
where $\mathbf{F}$ represents the net force acting on the body, mmm is its mass, and a\\mathbf{a}a is its resulting acceleration. In Rust, we can encapsulate these properties and operations within a RigidBody
struct. The following code demonstrates a basic implementation that accounts for mass, position, velocity, and the forces acting on the body:
use nalgebra::Vector3;
// Define the RigidBody struct to encapsulate basic translational properties.
struct RigidBody {
mass: f32,
position: Vector3<f32>,
velocity: Vector3<f32>,
force: Vector3<f32>,
}
impl RigidBody {
// Constructor for RigidBody initializing mass, position, velocity, and zeroed force.
fn new(mass: f32, position: Vector3<f32>, velocity: Vector3<f32>) -> Self {
RigidBody {
mass,
position,
velocity,
force: Vector3::new(0.0, 0.0, 0.0),
}
}
// Method to apply an external force to the rigid body.
fn apply_force(&mut self, force: Vector3<f32>) {
self.force += force;
}
// Updates the state of the rigid body over a time step `dt`.
fn update(&mut self, dt: f32) {
// Compute the acceleration using Newton's second law.
let acceleration = self.force / self.mass;
// Update velocity based on the computed acceleration.
self.velocity += acceleration * dt;
// Update position using the new velocity.
self.position += self.velocity * dt;
// Reset the applied force for the next update cycle.
self.force = Vector3::new(0.0, 0.0, 0.0);
}
}
fn main() {
// Create a new RigidBody instance.
let mut body = RigidBody::new(
1.0,
Vector3::new(0.0, 0.0, 0.0),
Vector3::new(1.0, 0.0, 0.0),
);
// Apply a force and update the state over time.
body.apply_force(Vector3::new(0.0, 10.0, 0.0));
body.update(0.1);
// Print the updated position and velocity.
println!("Position: {:?}", body.position);
println!("Velocity: {:?}", body.velocity);
}
In this example, the RigidBody
struct has fields for mass, position, velocity, and force. The apply_force
method allows external forces to be applied to the body, while the update
method integrates the equations of motion over a small time step dt
. The acceleration is calculated by dividing the force by the mass, and then this acceleration is used to update the velocity and position of the body.
Rotational motion is more complex, as it involves angular velocity and torque, and requires considering the body's moment of inertia. The moment of inertia tensor III relates the angular velocity $\mathbf{\omega}$ to the angular momentum $\mathbf{L}$:
$$\mathbf{L} = I \mathbf{\omega}$$
The rotational analogue to Newton's second law is:
$$\mathbf{\tau} = I \mathbf{\alpha}$$
where $\mathbf{\tau}$ is the torque and $\mathbf{\alpha}$ is the angular acceleration. Similar to the translational case, we can extend the RigidBody
struct to handle rotational dynamics. This would involve storing the body's orientation (e.g., as a quaternion), its angular velocity, and its moment of inertia tensor.
use nalgebra::Vector3;
// Extended RigidBody struct to include rotational properties.
struct RigidBody {
mass: f32,
position: Vector3<f32>,
velocity: Vector3<f32>,
force: Vector3<f32>,
// Orientation represented as a quaternion for stability and to avoid gimbal lock.
orientation: Quaternion<f32>,
// Angular velocity vector representing rotation rate.
angular_velocity: Vector3<f32>,
// Accumulated torque acting on the body.
torque: Vector3<f32>,
// Inertia tensor matrix representing the distribution of mass.
inertia_tensor: Matrix3<f32>,
}
impl RigidBody {
fn new(mass: f32, position: Vector3<f32>, velocity: Vector3<f32>) -> Self {
RigidBody {
mass,
position,
velocity,
force: Vector3::new(0.0, 0.0, 0.0),
}
}
// Method to apply an external force to the rigid body.
fn apply_force(&mut self, force: Vector3<f32>) {
self.force += force;
}
// Updated method that now integrates both translation and rotation over time dt.
fn update(&mut self, dt: f32) {
// Update translational motion.
let acceleration = self.force / self.mass;
self.velocity += acceleration * dt;
self.position += self.velocity * dt;
// Compute angular acceleration by applying the inverse of the inertia tensor to the torque.
let angular_acceleration = self.inertia_tensor
.inverse()
.expect("Inertia tensor inversion failed") * self.torque;
// Update the angular velocity.
self.angular_velocity += angular_acceleration * dt;
// Update the orientation using quaternion multiplication.
// The rotation angle is the magnitude of angular velocity times the time step.
// The axis is defined by the normalized angular velocity.
self.orientation = self.orientation
* Quaternion::from_axis_angle(
&self.angular_velocity.normalize(),
self.angular_velocity.magnitude() * dt,
);
// Reset force and torque accumulators for the next update cycle.
self.force = Vector3::new(0.0, 0.0, 0.0);
self.torque = Vector3::new(0.0, 0.0, 0.0);
}
}
In this extended example, the RigidBody
struct now includes fields for orientation, angular velocity, torque, and the inertia tensor. The update
method has been expanded to include the integration of rotational motion. The angular acceleration is computed by inverting the inertia tensor and applying it to the torque. The orientation is updated using the quaternion representation, which is more stable and less prone to gimbal lock compared to Euler angles.
This implementation demonstrates Rust’s capability to handle the complexities of rigid body dynamics while ensuring memory safety and performance. Rust's type system and strong compile-time checks help prevent common errors such as dividing by zero or mismanaging resources, which are particularly important in a computational physics context where simulations can be both numerically intensive and sensitive to small errors.
This approach provides a robust foundation for simulating rigid body dynamics and can be extended further to incorporate more advanced features such as collision detection and response, constraints, and real-time visualization, all within the powerful and safe environment that Rust offers.
12.2. Mathematical Foundations
The mathematical underpinnings of rigid body dynamics are deeply rooted in linear algebra, which provides the essential framework for describing and manipulating the position, orientation, and overall motion of bodies within three-dimensional space. Central to this framework are vectors, matrices, and the various transformations they enable. Vectors, which carry both magnitude and direction, are indispensable for representing physical quantities such as position, velocity, and forces. Matrices, in turn, facilitate linear transformations—including scaling, rotation, and translation—making it possible to modify a rigid body's orientation and position efficiently.
In the realm of rotational dynamics, quaternions play a pivotal role. Unlike Euler angles, quaternions represent rotations without suffering from the limitations of gimbal lock and offer smooth interpolation between rotations (often implemented via spherical linear interpolation or slerp). This makes them particularly useful in simulations where smooth and continuous rotational motion is required. Euler's equations, which describe the rotation of a rigid body about its center of mass by accounting for the angular velocity and the moments of inertia, further solidify our mathematical foundation. These equations are critical for comprehending how torque influences a rigid body's rotational behavior.
Choosing a suitable representation for rotations is crucial for both simulation accuracy and computational efficiency. Although Euler angles are conceptually straightforward, their susceptibility to gimbal lock—where the loss of one degree of freedom occurs—can complicate simulations involving rotations around multiple axes. In contrast, quaternions not only circumvent gimbal lock but also offer a more stable and computationally efficient way to represent 3D rotations. Yet, rotation matrices remain useful in many calculations, such as when applying transformations directly to vector quantities. Therefore, the ability to convert between matrices, quaternions, and Euler angles is essential for creating flexible, robust simulation systems.
Rust provides powerful tools for implementing these mathematical foundations, notably through libraries such as [nalgebra](https://crates.io/crates/nalgebra) and [cgmath](https://crates.io/crates/cgmath). These crates furnish comprehensive support for vectors, matrices, and quaternions, streamlining the necessary computations for simulating rigid body dynamics.
To illustrate these concepts, consider a basic example that demonstrates vector and matrix operations using the nalgebra crate. In this example, we define a function that rotates a vector using a rotation matrix:
// Import necessary types from the nalgebra crate.
use nalgebra::{Vector3, Matrix3};
/// Rotates a given vector `v` by multiplying it with the provided `rotation_matrix`.
fn rotate_vector(v: Vector3<f32>, rotation_matrix: Matrix3<f32>) -> Vector3<f32> {
// Matrix multiplication rotates the vector.
rotation_matrix * v
}
fn main() {
// Define a vector along the x-axis.
let v = Vector3::new(1.0, 0.0, 0.0);
// Define a rotation matrix for a 90-degree rotation around the z-axis.
// This rotates the vector in the xy-plane.
let rotation_matrix = Matrix3::new(
0.0, -1.0, 0.0,
1.0, 0.0, 0.0,
0.0, 0.0, 1.0,
);
// Rotate the vector using the rotation matrix.
let rotated_v = rotate_vector(v, rotation_matrix);
println!("Rotated Vector: {:?}", rotated_v);
}
In this code snippet, the rotate_vector
function takes both a vector and a rotation matrix as inputs, returning the rotated vector as the result of their multiplication. The nalgebra crate simplifies operations on vectors and matrices, enabling a clear and concise implementation of a 90-degree rotation around the z-axis.
Next, we explore quaternion operations, which are especially useful for smoothly interpolating between rotations. Using nalgebra’s UnitQuaternion
type, the following example shows how to apply a quaternion rotation to a vector:
// Import necessary types from nalgebra.
use nalgebra::{Vector3, UnitQuaternion};
/// Applies a rotation to a vector `v` using the provided quaternion `rotation`.
fn rotate_with_quaternion(v: Vector3<f32>, rotation: UnitQuaternion<f32>) -> Vector3<f32> {
// Quaternion multiplication performs the rotation.
rotation * v
}
fn main() {
// Define a vector along the x-axis.
let v = Vector3::new(1.0, 0.0, 0.0);
// Define an axis for rotation: the z-axis in this case.
let axis = Vector3::z_axis();
// Create a quaternion representing a 90-degree rotation about the z-axis.
let rotation = UnitQuaternion::from_axis_angle(&axis, std::f32::consts::FRAC_PI_2);
// Rotate the vector using the quaternion.
let rotated_v = rotate_with_quaternion(v, rotation);
println!("Rotated Vector with Quaternion: {:?}", rotated_v);
}
Here, the rotate_with_quaternion
function demonstrates how a quaternion can be used to perform a rotation on a vector. By defining a quaternion that represents a 90-degree rotation about the z-axis, we avoid the pitfalls of gimbal lock while ensuring smooth rotational behavior.
Finally, we turn to Euler's equations, which govern the rotational motion of a rigid body by relating torque and angular acceleration through the body's inertia tensor. The following code provides an example of how Euler's equations can be implemented in Rust for a simple rigid body:
// Import necessary types from nalgebra.
use nalgebra::{Vector3, Matrix3};
/// Represents a rigid body with only rotational dynamics.
struct RigidBody {
// The current angular velocity vector.
angular_velocity: Vector3<f32>,
// The inertia tensor representing the mass distribution.
inertia_tensor: Matrix3<f32>,
}
impl RigidBody {
/// Constructs a new RigidBody with the given angular velocity and inertia tensor.
fn new(angular_velocity: Vector3<f32>, inertia_tensor: Matrix3<f32>) -> Self {
RigidBody {
angular_velocity,
inertia_tensor,
}
}
/// Updates the rigid body's angular velocity based on an applied torque and time step `dt`.
fn update_rotation(&mut self, torque: Vector3<f32>, dt: f32) {
// Calculate angular acceleration using the inverse of the inertia tensor.
let angular_acceleration = self.inertia_tensor
.try_inverse()
.expect("Failed to invert inertia tensor") * torque;
// Update the angular velocity based on the angular acceleration.
self.angular_velocity += angular_acceleration * dt;
}
}
fn main() {
// Define an initial angular velocity around the y-axis.
let angular_velocity = Vector3::new(0.0, 1.0, 0.0);
// Define the inertia tensor for a uniform sphere.
// For a uniform sphere, the inertia scalar is 2/5 along each principal axis.
let inertia_tensor = Matrix3::new(
2.0 / 5.0, 0.0, 0.0,
0.0, 2.0 / 5.0, 0.0,
0.0, 0.0, 2.0 / 5.0,
);
// Create a rigid body with the initial angular velocity and inertia tensor.
let mut body = RigidBody::new(angular_velocity, inertia_tensor);
// Define a torque vector to apply to the rigid body.
let torque = Vector3::new(0.0, 0.0, 1.0);
// Update the rotational state over a small time step.
body.update_rotation(torque, 0.01);
println!("Updated Angular Velocity: {:?}", body.angular_velocity);
}
In this example, the RigidBody
struct encapsulates the rotational properties of a body using an angular velocity vector and an inertia tensor. The update_rotation
method computes the angular acceleration (by taking the inverse of the inertia tensor and multiplying it by the applied torque) and then updates the angular velocity accordingly. This example demonstrates how Euler's equations are seamlessly integrated into a simulation framework using Rust.
Together, these examples illustrate how Rust—armed with libraries like nalgebra—empowers developers to construct simulations with a robust mathematical foundation. The language’s strong type system, combined with the expressiveness of its mathematical libraries, makes it an excellent choice for implementing complex models of rigid body dynamics while ensuring both efficiency and numerical stability.
12.3. Numerical Integration Techniques
Numerical integration is indispensable in simulating rigid body dynamics because it allows us to solve the equations of motion over time and predict the system’s future state. Essentially, we approximate how bodies evolve by updating their positions and velocities at discrete time steps. The numerical methods commonly employed in these simulations are broadly divided into explicit and implicit techniques.
Explicit methods compute the system’s state at the next time step directly from the current state. Their relative simplicity and lower computational cost make them attractive for many problems. However, these methods can be sensitive to the size of the time step and may suffer from numerical instability, particularly when dealing with stiff systems. The Euler method is the simplest explicit integrator; it updates the state using current values of velocity and acceleration but is known to incur significant numerical errors and instability if the time step is too large.
In contrast, implicit methods require solving equations involving the state at the next time step. This characteristic generally leads to improved stability, particularly in stiff systems, but these methods are computationally more demanding because they often involve iterative solvers. A typical example is the backward Euler method.
Beyond these basic approaches, more sophisticated methods exist. Verlet integration, widely used in molecular dynamics, is an explicit method that delivers better stability and energy conservation compared to the Euler method. It achieves this by leveraging both current and previous positions to compute the new position. Meanwhile, Runge-Kutta methods—most notably the fourth-order Runge-Kutta (RK4) method—strike a balance between accuracy and computational cost. RK4 considers multiple intermediate evaluations of the derivative, resulting in greater accuracy in the state estimation, which can be critical for simulating complex dynamics.
Stability and accuracy are central concerns when choosing a numerical integration technique. Stability refers to the algorithm’s ability to minimize the growth of numerical error over time, while accuracy quantifies how close the numerical solution is to the exact, theoretical solution. Both properties are significantly influenced by the time step used and the inherent characteristics of the integrator. Additionally, error analysis in numerical integration distinguishes between truncation errors (stemming from the discrete nature of the approximation) and round-off errors (resulting from finite numerical precision).
Implementing these integrators in Rust leverages the language’s strong type system and memory safety guarantees, ensuring that the code remains both efficient and robust. Below are detailed implementations of the Euler method, Verlet integration, and the fourth-order Runge-Kutta method, each accompanied by commentary to clarify their operation and trade-offs.
Euler Method Implementation
In this example, a RigidBody
struct encapsulates the position, velocity, and acceleration of a body. The euler_step
method uses the current acceleration to update the velocity and position in an explicit manner:
// Import the nalgebra crate for vector operations.
use nalgebra::Vector3;
/// A simple representation of a rigid body for translational motion.
struct RigidBody {
position: Vector3<f32>,
velocity: Vector3<f32>,
acceleration: Vector3<f32>,
}
impl RigidBody {
/// Constructs a new `RigidBody` with the specified initial state.
fn new(position: Vector3<f32>, velocity: Vector3<f32>, acceleration: Vector3<f32>) -> Self {
RigidBody {
position,
velocity,
acceleration,
}
}
/// Updates the state of the rigid body using the Euler integration method.
///
/// This method performs a simple update using the current velocity and acceleration:
/// - Velocity is updated using: velocity += acceleration * dt
/// - Position is then updated using the new velocity: position += velocity * dt
fn euler_step(&mut self, dt: f32) {
self.velocity += self.acceleration * dt;
self.position += self.velocity * dt;
}
}
fn main() {
// Initialize a rigid body at the origin with an initial velocity in the x-direction
// and acceleration representing gravity in the negative y-direction.
let mut body = RigidBody::new(
Vector3::new(0.0, 0.0, 0.0), // Initial position at the origin.
Vector3::new(1.0, 0.0, 0.0), // Initial velocity along the x-axis.
Vector3::new(0.0, -9.81, 0.0) // Acceleration simulating gravity.
);
let dt = 0.01; // Define the time step.
// Simulate for 100 time steps.
for _ in 0..100 {
body.euler_step(dt);
println!("Position (Euler): {:?}", body.position);
}
}
This implementation demonstrates how the Euler method is used to update the velocity and position explicitly. Its simplicity comes at the expense of potential instability if the time step is not sufficiently small.
Verlet Integration Implementation
Verlet integration generally provides greater stability and improved conservation of energy, particularly in systems where long-term accuracy in position updates is critical. By keeping track of the previous position, it calculates the next position using both the current and previous positions, alongside the acceleration:
// Import the nalgebra crate for vector operations.
use nalgebra::Vector3;
/// A representation of a rigid body using Verlet integration,
/// which maintains both the current and previous positions.
struct RigidBody {
position: Vector3<f32>,
prev_position: Vector3<f32>,
acceleration: Vector3<f32>,
}
impl RigidBody {
/// Constructs a new `RigidBody` given an initial position and acceleration.
/// Initially, the previous position is set equal to the starting position.
fn new(position: Vector3<f32>, acceleration: Vector3<f32>) -> Self {
RigidBody {
position,
prev_position: position,
acceleration,
}
}
/// Updates the rigid body's position using the Verlet integration method.
///
/// This approach computes the new position using the formula:
/// new_position = 2 * position - prev_position + acceleration * dt^2
fn verlet_step(&mut self, dt: f32) {
let new_position = 2.0 * self.position - self.prev_position + self.acceleration * dt * dt;
self.prev_position = self.position;
self.position = new_position;
}
}
fn main() {
// Initialize a rigid body at the origin with an acceleration representing gravity.
let mut body = RigidBody::new(
Vector3::new(0.0, 0.0, 0.0),
Vector3::new(0.0, -9.81, 0.0)
);
let dt = 0.01;
// Set an initial previous position to represent an initial velocity along the x-axis.
body.prev_position = body.position - Vector3::new(1.0 * dt, 0.0, 0.0);
// Simulate for 100 time steps.
for _ in 0..100 {
body.verlet_step(dt);
println!("Position (Verlet): {:?}", body.position);
}
}
This Verlet implementation effectively leverages both the current and prior positions to obtain a more stable and energy-conserving update. It is particularly useful when simulating systems where maintaining physical fidelity over long time spans is crucial.
Fourth-Order Runge-Kutta (RK4) Implementation
The RK4 method is one of the most popular higher-order integration techniques due to its balance between computational cost and accuracy. It evaluates intermediate slopes (or derivatives) at several points within the time step to provide a weighted average that more accurately approximates the state at the next time step:
// Import the nalgebra crate for vector operations.
use nalgebra::Vector3;
/// A representation of a rigid body for which we simulate translational motion using RK4.
struct RigidBody {
position: Vector3<f32>,
velocity: Vector3<f32>,
acceleration: Vector3<f32>,
}
impl RigidBody {
/// Constructs a new `RigidBody` with the given position, velocity, and acceleration.
fn new(position: Vector3<f32>, velocity: Vector3<f32>, acceleration: Vector3<f32>) -> Self {
RigidBody {
position,
velocity,
acceleration,
}
}
/// Updates the state of the rigid body using the fourth-order Runge-Kutta method.
///
/// RK4 calculates several intermediate "slopes" to more accurately approximate the change.
fn rk4_step(&mut self, dt: f32) {
// k1 values represent initial slopes based on the current state.
let k1_v = self.acceleration;
let k1_p = self.velocity;
// k2 values use an estimate mid-way through the interval using k1.
let k2_v = self.acceleration;
let k2_p = self.velocity + 0.5 * k1_v * dt;
// k3 values refine the mid-way estimate further using k2.
let k3_v = self.acceleration;
let k3_p = self.velocity + 0.5 * k2_v * dt;
// k4 values are computed using the estimate at the end of the interval.
let k4_v = self.acceleration;
let k4_p = self.velocity + k3_v * dt;
// Update velocity and position using a weighted average of the slopes.
self.velocity += (k1_v + 2.0 * k2_v + 2.0 * k3_v + k4_v) * (dt / 6.0);
self.position += (k1_p + 2.0 * k2_p + 2.0 * k3_p + k4_p) * (dt / 6.0);
}
}
fn main() {
// Initialize a rigid body at the origin with an initial velocity and acceleration due to gravity.
let mut body = RigidBody::new(
Vector3::new(0.0, 0.0, 0.0),
Vector3::new(1.0, 0.0, 0.0),
Vector3::new(0.0, -9.81, 0.0)
);
let dt = 0.01;
// Simulate the system for 100 time steps using RK4.
for _ in 0..100 {
body.rk4_step(dt);
println!("Position (RK4): {:?}", body.position);
}
}
In this RK4 implementation, the calculation of intermediate slopes (denoted by k1, k2, k3, and k4) effectively captures the state’s evolution within the time step. The resulting updates to velocity and position are more accurate compared to simpler methods like Euler, though they require additional computations.
Each of these examples demonstrates a different numerical integration technique with its own trade-offs in terms of accuracy, stability, and computational cost. By carefully selecting the appropriate method and managing time step sizes, one can accurately simulate the complex dynamics of rigid bodies in a computationally efficient manner. Rust’s robust type system and safety guarantees further ensure that these simulations remain reliable and free from common programming errors.
12.4. Collision Detection and Response
Collision detection and response are central to simulating rigid body dynamics, particularly in scenarios where objects frequently interact—such as within physics engines for games or high-fidelity simulations. Collision detection involves determining when and where two or more bodies intersect or come into contact. This process relies on several key concepts including bounding volumes, contact points, and collision normals.
Bounding volumes are simplified geometric representations (e.g., spheres, boxes, or capsules) used to approximate the shape of more complex objects. They serve as an efficient first step—often called broad-phase collision detection—to quickly eliminate pairs of objects that are unlikely to collide. Once a potential collision is flagged, narrow-phase collision detection algorithms are applied to calculate exact contact points and determine the collision normals—the directions perpendicular to the contact surfaces at the point of impact.
Once a collision is detected, the response mechanism defines how the interacting bodies adjust their motion. There are two primary approaches to collision response: impulse-based methods and force-based methods. Impulse-based methods apply an instantaneous change in velocity to the colliding objects, which is calculated from the collision impulse and relies on the conservation of momentum (and sometimes energy) principles. This approach is popular in real-time simulations due to its computational speed. In contrast, force-based methods apply continuous forces over time to achieve a more gradual response; although these methods can be more physically accurate, they typically incur a higher computational cost.
Collision detection techniques themselves can be classified as either discrete or continuous. Discrete collision detection checks for overlaps at fixed time intervals. However, if objects move too quickly relative to the chosen time step, the simulation may miss collisions—a problem known as "tunneling." Continuous collision detection helps to overcome this issue by predicting object paths between time steps, ensuring that fast-moving objects still register collisions, albeit with increased computational demands.
An essential consideration when handling collisions is the conservation of momentum and energy. In an ideal elastic collision, both momentum and kinetic energy are conserved so that the total state (pre- and post-collision) remains unchanged. In real-world scenarios, inelastic collisions occur where kinetic energy is transformed into other forms (e.g., heat or deformation) while momentum continues to be conserved.
Rust’s robust ecosystem, including libraries such as [nalgebra](https://crates.io/crates/nalgebra) and [parry3d](https://crates.io/crates/parry3d), provides powerful tools for implementing collision detection and response. Below are refined examples demonstrating basic collision detection using bounding spheres and implementing an impulse-based collision response, followed by a brief look at using the parry3d crate for more advanced collision detection.
Bounding Spheres for Collision Detection
This first example demonstrates a simple collision detection algorithm using bounding spheres. The idea is to check whether the distance between the centers of two spheres is less than the sum of their radii, which would indicate an overlap.
// Import the nalgebra crate for vector operations.
use nalgebra::Vector3;
/// A simple bounding sphere that approximates the shape of an object.
struct Sphere {
center: Vector3<f32>,
radius: f32,
}
impl Sphere {
/// Constructs a new Sphere given a center point and a radius.
fn new(center: Vector3<f32>, radius: f32) -> Self {
Sphere { center, radius }
}
/// Determines if this sphere is colliding with another sphere.
///
/// The method computes the Euclidean distance between the two centers and
/// compares it to the sum of the radii. If the distance is less, a collision
/// is detected.
fn is_colliding(&self, other: &Sphere) -> bool {
let distance = (self.center - other.center).norm();
distance < (self.radius + other.radius)
}
}
fn main() {
// Create two spheres with given centers and radii.
let sphere1 = Sphere::new(Vector3::new(0.0, 0.0, 0.0), 1.0);
let sphere2 = Sphere::new(Vector3::new(1.5, 0.0, 0.0), 1.0);
// Check for collision between the two spheres.
if sphere1.is_colliding(&sphere2) {
println!("Spheres are colliding!");
} else {
println!("Spheres are not colliding.");
}
}
In this code, the is_colliding
method computes the distance between the centers using the norm of their difference. If this distance is less than the sum of the radii, then the spheres are colliding—a simple yet effective broad-phase collision test.
Impulse-Based Collision Response
Following collision detection, impulse-based methods adjust the velocities of colliding bodies to simulate a realistic separation while conserving momentum. In the example below, a RigidBody
struct represents a body with position, velocity, and mass. An impulse calculated from the relative velocities and the collision normal is then applied to update the velocities of the colliding bodies.
// Import the nalgebra crate for vector operations.
use nalgebra::Vector3;
/// A simplified rigid body representation for collision response.
struct RigidBody {
position: Vector3<f32>,
velocity: Vector3<f32>,
mass: f32,
}
impl RigidBody {
/// Constructs a new RigidBody with the given position, velocity, and mass.
fn new(position: Vector3<f32>, velocity: Vector3<f32>, mass: f32) -> Self {
RigidBody {
position,
velocity,
mass,
}
}
/// Applies an instantaneous impulse to the rigid body, updating its velocity.
fn apply_impulse(&mut self, impulse: Vector3<f32>) {
// The change in velocity is the impulse divided by the object's mass.
self.velocity += impulse / self.mass;
}
}
/// Calculates the impulse to apply during a collision between two rigid bodies.
///
/// This function calculates the impulse based on the component of the relative
/// velocity along the collision normal, the masses of the bodies, and a restitution
/// coefficient, which represents the elasticity of the collision.
fn calculate_impulse(rb1: &RigidBody, rb2: &RigidBody, normal: Vector3<f32>) -> Vector3<f32> {
// Compute the relative velocity between the bodies.
let relative_velocity = rb1.velocity - rb2.velocity;
// Project the relative velocity onto the collision normal.
let velocity_along_normal = relative_velocity.dot(&normal);
// If the bodies are already separating, no impulse is needed.
if velocity_along_normal > 0.0 {
return Vector3::new(0.0, 0.0, 0.0);
}
// Define the restitution coefficient (elasticity): 1.0 is perfectly elastic.
let restitution = 0.8;
// Calculate the impulse magnitude using the conservation of momentum.
let impulse_magnitude = -(1.0 + restitution) * velocity_along_normal /
(1.0 / rb1.mass + 1.0 / rb2.mass);
// Return the impulse vector by scaling the normal.
impulse_magnitude * normal
}
fn main() {
// Create two rigid bodies moving towards each other.
let mut body1 = RigidBody::new(
Vector3::new(0.0, 0.0, 0.0),
Vector3::new(1.0, 0.0, 0.0),
2.0,
);
let mut body2 = RigidBody::new(
Vector3::new(2.0, 0.0, 0.0),
Vector3::new(-1.0, 0.0, 0.0),
2.0,
);
// The collision normal is defined along the line of impact.
let collision_normal = Vector3::new(1.0, 0.0, 0.0).normalize();
// Calculate the collision impulse.
let impulse = calculate_impulse(&body1, &body2, collision_normal);
// Apply the impulse to both bodies (one receives the negative of the impulse).
body1.apply_impulse(impulse);
body2.apply_impulse(-impulse);
println!("Body 1 Velocity after collision: {:?}", body1.velocity);
println!("Body 2 Velocity after collision: {:?}", body2.velocity);
}
In this example, the relative velocity between the two bodies is first decomposed along the collision normal. An impulse is then calculated using a restitution factor and the masses of the bodies, after which the impulse is applied to adjust their velocities. This instantaneous change helps simulate the separation expected after a collision.
Advanced Collision Detection with parry3d
For more complex shapes and continuous collision detection, the [parry3d](https://crates.io/crates/parry3d) crate provides advanced functionality. The following snippet demonstrates how to detect collisions between two Axis-Aligned Bounding Boxes (AABBs) using parry3d’s utilities:
// Import necessary types from the parry3d crate.
use nalgebra::Vector3;
use parry3d::bounding_volume::AABB;
use parry3d::query::{contact, Contact};
fn main() {
// Define two AABBs by their minimum and maximum points.
let aabb1 = Aabb::new(Point3::new(-1.0, -1.0, -1.0), Point3::new(1.0, 1.0, 1.0));
let aabb2 = Aabb::new(Point3::new(0.5, 0.5, 0.5), Point3::new(1.5, 1.5, 1.5));
// Check if the AABBs intersect.
if aabb1.intersects(&aabb2) {
println!("AABBs are colliding!");
} else {
println!("AABBs are not colliding.");
}
}
Here, the parry3d crate’s intersect
function is used to check for intersections between two AABBs. This technique is part of a narrow-phase collision detection strategy, vital for handling more complex geometrical interactions.
These examples illustrate a progression from simple bounding volume collision detection to an impulse-based collision response mechanism, and finally to advanced collision detection using dedicated libraries. By leveraging Rust’s powerful libraries and its stringent safety guarantees, it is possible to implement robust, high-performance collision detection and response systems that are well-suited for realistic rigid body dynamics in diverse applications.
12.5. Rigid Body Simulation Engine in Rust
Building a rigid body simulation engine requires a clear understanding of its architecture and the role of its core components. At its heart, a simulation engine consists of several interacting subsystems:
Physics World: This represents the entire environment where physical entities—rigid bodies, forces, and constraints—coexist. It is responsible for maintaining and updating the state of all objects, driving the simulation loop.
Rigid Body: Each physical entity is represented as a rigid body with properties such as mass, position, velocity, orientation, and applied forces.
Integrator: This component updates the state of the physics world by integrating the equations of motion using numerical methods (e.g., Euler, Verlet, or Runge-Kutta).
Collision Detection and Response: This module identifies when rigid bodies interact (collide) and determines how to resolve these collisions to conserve momentum and, if applicable, energy.
Time-Stepping Mechanism: The simulation’s progression over time can either use fixed time steps (providing consistent updates) or variable time steps (adapting to real-time constraints). Fixed steps are often easier to handle, though they may require interpolation for smooth real-time rendering.
A modular design is key to creating a flexible and maintainable simulation engine. For instance, the collision detection subsystem should operate independently from the rigid body definitions or the integrator, allowing each component to be developed and optimized separately. Moreover, efficient data structures and algorithms are crucial for managing the computational complexity—especially in real-time applications where many bodies interact simultaneously. Rust’s ownership model and concurrency features, such as those provided by the Rayon crate, aid in ensuring that the simulation remains safe, efficient, and scalable.
Below is a progressively refined example that builds a basic simulation engine, beginning with defining the core types (RigidBody and PhysicsWorld) and then demonstrating how to integrate parallel processing and visualization.
Basic Simulation Engine Components
In this initial example, we define a simple RigidBody
structure that holds position, velocity, mass, and accumulated force. An update
method is provided to compute the next state based on a fixed time step. The PhysicsWorld
then collects a list of rigid bodies and steps through the simulation by updating each body.
// Import the nalgebra crate for vector operations.
use nalgebra::Vector3;
/// A basic representation of a rigid body for translational dynamics.
struct RigidBody {
position: Vector3<f32>,
velocity: Vector3<f32>,
mass: f32,
force: Vector3<f32>,
}
impl RigidBody {
/// Constructs a new RigidBody with the given initial position, velocity, and mass.
/// The force is initialized to zero.
fn new(position: Vector3<f32>, velocity: Vector3<f32>, mass: f32) -> Self {
RigidBody {
position,
velocity,
mass,
force: Vector3::new(0.0, 0.0, 0.0),
}
}
/// Applies an external force to the rigid body.
fn apply_force(&mut self, force: Vector3<f32>) {
self.force += force;
}
/// Updates the state of the rigid body by integrating the equations of motion.
/// This uses a simple explicit Euler integration.
fn update(&mut self, dt: f32) {
// Compute acceleration from force and mass.
let acceleration = self.force / self.mass;
// Update velocity using the acceleration.
self.velocity += acceleration * dt;
// Update position using the new velocity.
self.position += self.velocity * dt;
// Reset the force accumulator for the next update.
self.force = Vector3::new(0.0, 0.0, 0.0);
}
}
/// Represents the simulation environment containing all the rigid bodies and the time step.
struct PhysicsWorld {
bodies: Vec<RigidBody>,
time_step: f32,
}
impl PhysicsWorld {
/// Creates a new PhysicsWorld with the specified fixed time step.
fn new(time_step: f32) -> Self {
PhysicsWorld {
bodies: Vec::new(),
time_step,
}
}
/// Adds a rigid body to the simulation.
fn add_body(&mut self, body: RigidBody) {
self.bodies.push(body);
}
/// Advances the simulation by one time step, updating all bodies.
fn step(&mut self) {
for body in &mut self.bodies {
body.update(self.time_step);
}
}
}
fn main() {
// Create a PhysicsWorld with a fixed time step.
let mut world = PhysicsWorld::new(0.01);
// Create two rigid bodies with initial positions and velocities.
let body1 = RigidBody::new(Vector3::new(0.0, 0.0, 0.0), Vector3::new(1.0, 0.0, 0.0), 2.0);
let body2 = RigidBody::new(Vector3::new(0.0, 0.0, 0.0), Vector3::new(0.0, 1.0, 0.0), 2.0);
// Add the bodies to the physics world.
world.add_body(body1);
world.add_body(body2);
// Run the simulation for 100 time steps.
for _ in 0..100 {
world.step();
}
}
In this basic engine, each rigid body is updated by computing its acceleration from the applied force, updating its velocity, and then its position. The physics world contains all bodies and advances the simulation state uniformly at every step.
Parallelizing the Simulation with Rayon
For real-time applications with many bodies, performance improvements can be achieved by updating the bodies concurrently. With Rust’s Rayon library, we can easily parallelize the simulation loop.
// Add the Rayon crate to Cargo.toml to enable parallel iteration:
// [dependencies]
// rayon = "1.5"
// nalgebra = "0.30"
use rayon::prelude::*;
use nalgebra::Vector3;
struct RigidBody {
position: Vector3<f32>,
velocity: Vector3<f32>,
mass: f32,
force: Vector3<f32>,
}
impl RigidBody {
fn new(position: Vector3<f32>, velocity: Vector3<f32>, mass: f32) -> Self {
RigidBody {
position,
velocity,
mass,
force: Vector3::new(0.0, 0.0, 0.0),
}
}
fn apply_force(&mut self, force: Vector3<f32>) {
self.force += force;
}
fn update(&mut self, dt: f32) {
let acceleration = self.force / self.mass;
self.velocity += acceleration * dt;
self.position += self.velocity * dt;
self.force = Vector3::new(0.0, 0.0, 0.0);
}
}
struct PhysicsWorld {
bodies: Vec<RigidBody>,
time_step: f32,
}
impl PhysicsWorld {
fn new(time_step: f32) -> Self {
PhysicsWorld {
bodies: Vec::new(),
time_step,
}
}
fn add_body(&mut self, body: RigidBody) {
self.bodies.push(body);
}
/// Advances the simulation by updating all bodies in parallel using Rayon.
fn step(&mut self) {
// par_iter_mut() safely iterates over bodies concurrently.
self.bodies.par_iter_mut().for_each(|body| {
body.update(self.time_step);
});
}
}
fn main() {
let mut world = PhysicsWorld::new(0.01);
let body1 = RigidBody::new(Vector3::new(0.0, 0.0, 0.0), Vector3::new(1.0, 0.0, 0.0), 2.0);
let body2 = RigidBody::new(Vector3::new(0.0, 0.0, 0.0), Vector3::new(0.0, 1.0, 0.0), 2.0);
world.add_body(body1);
world.add_body(body2);
for _ in 0..100 {
world.step();
}
}
Here, the step
method leverages Rayon’s par_iter_mut()
to update each rigid body concurrently. This approach allows the simulation engine to scale efficiently on multi-core processors.
Integrating with Real-Time Visualization
For interactive applications or educational demonstrations, it is often useful to integrate the simulation engine with a visualization tool. Rust offers several game and rendering frameworks such as bevy or ggez. The example below demonstrates a simple integration with bevy—a popular Rust game engine—where physics results drive the visual updates.
// Add bevy to Cargo.toml:
// [dependencies]
// bevy = "0.9"
// nalgebra = "0.30"
use bevy::prelude::*;
use nalgebra::Vector3;
/// A component that holds physics-related properties.
struct PhysicsBody {
velocity: Vector3<f32>,
mass: f32,
}
/// The system that updates the transform of each entity based on its velocity.
fn physics_system(mut query: Query<(&mut Transform, &PhysicsBody)>, time: Res<Time>) {
// Update each entity based on its PhysicsBody component.
for (mut transform, physics_body) in query.iter_mut() {
let dt = time.delta_seconds();
// Convert nalgebra::Vector3 to bevy::Vec3 for transform updates.
transform.translation += Vec3::new(
physics_body.velocity.x * dt,
physics_body.velocity.y * dt,
physics_body.velocity.z * dt,
);
}
}
/// Initializes the scene by spawning entities with PhysicsBody and Transform components.
fn setup(mut commands: Commands) {
commands.spawn_bundle((
PhysicsBody {
velocity: Vector3::new(1.0, 0.0, 0.0),
mass: 1.0,
},
Transform::default(),
GlobalTransform::default(),
));
}
fn main() {
App::build()
// Add default plugins, which include windowing, rendering, etc.
.add_plugins(DefaultPlugins)
// Run the setup system once at startup.
.add_startup_system(setup.system())
// Add the physics system to update each frame.
.add_system(physics_system.system())
.run();
}
In the bevy example, entities are spawned with a PhysicsBody
component that stores the velocity and mass, and a Transform
component that determines the position in 3D space. The physics_system
then updates these transforms every frame based on the elapsed time, effectively visualizing the motion of the simulated bodies. This tight integration between the physics simulation and real-time rendering creates an interactive simulation environment.
Building a rigid body simulation engine in Rust involves constructing a modular system composed of a physics world, rigid bodies, numerical integrators, collision detection/response modules, and a time-stepping mechanism. Rust’s powerful type system, concurrency capabilities, and rich ecosystem of libraries (such as nalgebra, rayon, and bevy) allow you to create safe and efficient simulations that scale to real-world applications. This engine can serve as the foundation for further extensions such as more sophisticated collision handling or integration with advanced visualization tools, making it a valuable resource for both educational purposes and high-performance computational physics applications.
12.6. Optimizing Performance and Accuracy
Optimizing both performance and accuracy is critical in a rigid body simulation, especially when the simulation is running in real time where both speed and precision must be maintained. The first step is to profile your simulation to identify any bottlenecks—those parts of the code that consume the most computational resources. Profiling can reveal inefficiencies such as slow mathematical operations, excessive memory allocations, or suboptimal cache utilization.
A common challenge in physics simulations is the limitation of floating-point precision. Because floating-point numbers have finite precision, small rounding errors can accumulate over time, eventually leading to significant inaccuracies. To mitigate these issues, you can:
Use higher precision data types (e.g.,
f64
instead off32
) where necessary.Structure calculations to minimize error propagation.
Employ techniques such as Kahan summation to reduce numerical errors during accumulation.
Balancing accuracy and performance is fundamentally a trade-off. High accuracy often demands more complex algorithms and smaller time steps—which can increase computational cost—whereas prioritizing performance might entail simplifying calculations or increasing time steps at the expense of accuracy. The goal is to find the right balance where the simulation runs quickly enough in real time while maintaining sufficient accuracy for reliable results.
Modern processors also offer parallel computing techniques such as SIMD (Single Instruction, Multiple Data) and multi-threading. SIMD allows you to perform the same operation on multiple data points simultaneously, which is especially beneficial for operations on vectors and matrices. Multi-threading, through libraries like Rayon, enables you to distribute the computational load across multiple CPU cores.
Rust’s ecosystem provides several tools to optimize performance and accuracy. For example, you can use cargo-flamegraph to generate flamegraphs that visually represent where the execution time is spent:
cargo install flamegraph
cargo flamegraph --bin my_simulation
This tool helps identify bottlenecks—such as a heavy matrix multiplication routine—that might be candidates for inlining or SIMD optimization.
Inlining small, frequently called functions can also reduce function call overhead. In Rust, you can suggest inlining with the #[inline(always)]
attribute:
#[inline(always)]
fn vector_add(a: Vector3<f32>, b: Vector3<f32>) -> Vector3<f32> {
Vector3::new(a.x + b.x, a.y + b.y, a.z + b.z)
}
However, caution is required since overuse of inlining can increase binary size.
Another optimization strategy involves improving memory layout. Using a structure-of-arrays (SoA) layout rather than an array-of-structures (AoS) can greatly improve cache efficiency, especially when dealing with a large number of rigid bodies. Here’s an example that reorganizes rigid body data for better cache performance:
// Import nalgebra for vector operations.
use nalgebra::Vector3;
/// A structure-of-arrays (SoA) representation for many rigid bodies.
/// Each property is stored in a separate vector to improve spatial locality.
struct RigidBodySoA {
positions: Vec<Vector3<f32>>,
velocities: Vec<Vector3<f32>>,
masses: Vec<f32>,
}
impl RigidBodySoA {
/// Constructs a new RigidBodySoA with a specified capacity.
fn new(capacity: usize) -> Self {
RigidBodySoA {
positions: Vec::with_capacity(capacity),
velocities: Vec::with_capacity(capacity),
masses: Vec::with_capacity(capacity),
}
}
/// Adds a new rigid body by pushing its properties onto the respective vectors.
fn add_body(&mut self, position: Vector3<f32>, velocity: Vector3<f32>, mass: f32) {
self.positions.push(position);
self.velocities.push(velocity);
self.masses.push(mass);
}
/// Updates the state of all bodies with a simple Euler step.
/// This loop benefits from improved cache performance thanks to SoA layout.
fn update(&mut self, dt: f32) {
for i in 0..self.positions.len() {
// Apply gravity for this example.
self.velocities[i] += Vector3::new(0.0, -9.81, 0.0) * dt;
self.positions[i] += self.velocities[i] * dt;
}
}
}
SIMD operations can further optimize vector and matrix arithmetic. For example, the following code uses SIMD via the standard library’s experimental std::simd
module (or you can use the packed_simd
crate on stable Rust) to perform vector addition:
// Note: Requires nightly Rust or the packed_simd crate on stable Rust.
use std::simd::{f32x4, Simd};
/// Adds two slices of f32 values using SIMD to process four elements simultaneously.
/// Any remaining elements are processed sequentially.
fn simd_add(a: &mut [f32], b: &[f32]) {
let len = a.len();
let simd_chunks = len / 4;
for i in 0..simd_chunks {
let idx = i * 4;
// Load 4 elements into SIMD registers.
let va = f32x4::from_slice(&a[idx..(idx + 4)]);
let vb = f32x4::from_slice(&b[idx..(idx + 4)]);
// Perform SIMD addition.
let result = va + vb;
// Write the result back to the slice.
result.write_to_slice(&mut a[idx..(idx + 4)]);
}
// Process any remaining elements that don't fit into a SIMD register.
for i in simd_chunks * 4..len {
a[i] += b[i];
}
}
By operating on multiple data points in parallel, SIMD operations can significantly speed up array arithmetic—a common operation in physics simulations.
A real-world case study might involve optimizing a Rust-based rigid body simulation by:
Profiling the code with cargo-flamegraph and identifying that vector arithmetic dominates the computational cost.
Replacing critical vector operations with SIMD-accelerated code.
Switching from an AoS to an SoA data layout to improve cache efficiency.
Upgrading from a simple Euler integrator to a higher-order method such as RK4, which, while slightly more computationally expensive per step, allows for larger time steps or greater accuracy.
Parallelizing the physics update loop using the Rayon crate, as demonstrated here:
use rayon::prelude::*;
use nalgebra::Vector3;
/// Parallel update function that distributes updates across multiple CPU cores.
fn parallel_update(positions: &mut [Vector3<f32>], velocities: &mut [Vector3<f32>], dt: f32) {
positions.par_iter_mut().enumerate().for_each(|(i, pos)| {
// Apply gravity to update the velocity.
velocities[i] += Vector3::new(0.0, -9.81, 0.0) * dt;
// Update position based on new velocity.
*pos += velocities[i] * dt;
});
}
By distributing the workload across available CPU cores, the simulation leverages parallelism to achieve significant performance gains, which are essential when handling many rigid bodies in real time.
Optimizing performance and accuracy in a rigid body simulation involves a multi-faceted approach: profiling to identify bottlenecks, mitigating floating-point precision issues, and refining the data layout for better cache utilization. Additionally, leveraging advanced techniques such as SIMD and multi-threading further boosts performance. Rust's powerful toolchain—including cargo-flamegraph for profiling, Rayon for parallelism, and libraries supporting SIMD—empowers developers to build simulations that are not only fast but also maintain high levels of accuracy. By carefully balancing these optimizations, you can create efficient, real-time simulations even in demanding applications.
12.7. Advanced Topics in Rigid Body Dynamics
Below is the refined version of Chapter 12, Part 7, "Advanced Topics in Rigid Body Dynamics." This version maintains the narrative style of your original text while enhancing clarity, detail, and code robustness. The discussion now more clearly distinguishes between rigid body dynamics and related simulation topics, and the code examples include detailed comments to help explain their function and structure.
In advanced simulations, rigid body dynamics frequently intersect with other domains of computational physics—such as soft body dynamics, constraints, and joint systems. Understanding these interactions is critical when building simulations that model complex, real-world behavior.
Soft body dynamics concerns objects that deform under applied forces, unlike rigid bodies, whose shapes remain constant. Soft bodies require more elaborate simulations because the internal structure and deformation introduce extra degrees of freedom. In rigid body simulations, however, the primary focus is on translational and rotational motion without internal deformation.
When simulating systems composed of multiple interconnected rigid bodies—for instance, a robotic arm or a series of mechanical linkages—constraints and joints become essential. Constraints limit the relative motion between bodies and enable the modeling of physical connections such as hinges, sliders, and ball-and-socket joints. Accurately handling these constraints is vital for achieving realistic motion, especially in articulated systems where several bodies move in a coordinated fashion.
Advanced rigid body simulations may also incorporate additional phenomena such as soft constraints, articulated bodies, and fluid-structure interactions. Soft constraints allow connections that exhibit limited flexibility, thereby providing more realistic movement. Articulated bodies are composed of rigid bodies connected by joints, requiring careful constraint management to simulate natural motion. Fluid-structure interaction, on the other hand, addresses the complex interplay between fluid flow and solid bodies—for example, simulating a ship's movement through water or the behavior of a parachute in the air.
Implementing these advanced topics in Rust means leveraging and extending existing libraries to manage the added complexity. Below, we start by implementing a basic hinge joint in a rigid body system, followed by an example using the powerful rapier crate for more comprehensive simulations.
Basic Hinge Joint Implementation
A hinge joint allows two rigid bodies to rotate relative to each other about a single fixed axis—much like the way a door swings on its hinges. The following code defines a simple rigid body structure with properties for position, orientation (using quaternions to avoid gimbal lock), velocity, angular velocity, mass, and inertia. A corresponding HingeJoint
struct stores the indices of the connected bodies, the hinge axis, and an anchor point. Its apply_constraint
method calculates and applies an angular correction based on the difference in orientation between the two bodies.
use nalgebra::{Vector3, UnitQuaternion};
/// A basic rigid body structure with translational and rotational properties.
struct RigidBody {
position: Vector3<f32>,
orientation: UnitQuaternion<f32>,
velocity: Vector3<f32>,
angular_velocity: Vector3<f32>,
mass: f32,
inertia: Vector3<f32>, // Simplified representation for demonstration purposes.
}
/// A hinge joint that connects two rigid bodies, allowing rotation around a single axis.
struct HingeJoint {
body_a: usize,
body_b: usize,
hinge_axis: Vector3<f32>,
anchor_point: Vector3<f32>,
}
impl HingeJoint {
/// Constructs a new hinge joint connecting two bodies.
fn new(body_a: usize, body_b: usize, hinge_axis: Vector3<f32>, anchor_point: Vector3<f32>) -> Self {
HingeJoint {
body_a,
body_b,
hinge_axis,
anchor_point,
}
}
/// Applies the hinge constraint by adjusting the angular velocities of the connected bodies.
fn apply_constraint(&self, bodies: &mut [RigidBody]) {
// Split the mutable slice to avoid borrowing the same element twice.
let (left, right) = bodies.split_at_mut(self.body_b.max(self.body_a));
let (body_a, body_b) = if self.body_a < self.body_b {
(&mut left[self.body_a], &mut right[0])
} else {
(&mut right[0], &mut left[self.body_b])
};
// Compute the relative orientation: how body B is rotated relative to body A.
let relative_orientation = body_b.orientation * body_a.orientation.conjugate();
// Extract the axis and angle from the relative orientation.
if let Some((axis, angle)) = relative_orientation.axis_angle() {
// Compute the angular correction using the hinge axis.
let angular_correction = axis.into_inner().cross(&self.hinge_axis) * angle;
// Apply a proportional correction based on body masses.
body_a.angular_velocity += angular_correction / body_a.mass;
body_b.angular_velocity -= angular_correction / body_b.mass;
}
}
}
fn main() {
// Initialize two rigid bodies with default orientations and zero initial velocities.
let mut bodies = vec![
RigidBody {
position: Vector3::new(0.0, 0.0, 0.0),
orientation: UnitQuaternion::identity(),
velocity: Vector3::new(0.0, 0.0, 0.0),
angular_velocity: Vector3::new(0.0, 0.0, 0.0),
mass: 1.0,
inertia: Vector3::new(1.0, 1.0, 1.0),
},
RigidBody {
position: Vector3::new(1.0, 0.0, 0.0),
orientation: UnitQuaternion::identity(),
velocity: Vector3::new(0.0, 0.0, 0.0),
angular_velocity: Vector3::new(0.0, 0.0, 0.0),
mass: 1.0,
inertia: Vector3::new(1.0, 1.0, 1.0),
},
];
// Create a hinge joint connecting the two bodies.
let hinge = HingeJoint::new(0, 1, Vector3::new(0.0, 0.0, 1.0), Vector3::new(0.5, 0.0, 0.0));
// Apply the hinge constraint to adjust angular velocities.
hinge.apply_constraint(&mut bodies);
println!("Body A Angular Velocity: {:?}", bodies[0].angular_velocity);
println!("Body B Angular Velocity: {:?}", bodies[1].angular_velocity);
}
This example demonstrates a basic strategy for enforcing a hinge constraint by computing a correction based on the deviation from the desired hinge axis. In practice, a full constraint solver would involve additional considerations like impulse accumulation, stabilization, and integration with the broader simulation.
Leveraging Rapier for Advanced Simulations
For more advanced physical simulations that involve constraints, joints, and other interactions, Rust’s [rapier](https://rapier.rs/) crate provides a comprehensive physics engine with support for 2D and 3D dynamics. Rapier simplifies tasks such as creating rigid bodies, handling collisions, and managing a variety of joints. The following example sets up a basic simulation with two dynamic rigid bodies connected by a hinge-like joint using rapier.
// Add the following dependencies to Cargo.toml:
// rapier3d = "0.12"
// nalgebra = "0.30"
use rapier3d::prelude::*;
fn main() {
// Create the physics pipeline and supporting structures.
let mut physics_pipeline = PhysicsPipeline::new();
let gravity = vector![0.0, -9.81, 0.0];
let integration_parameters = IntegrationParameters::default();
let mut islands = IslandManager::new();
let mut broad_phase = BroadPhase::new();
let mut narrow_phase = NarrowPhase::new();
let mut bodies = RigidBodySet::new();
let mut colliders = ColliderSet::new();
let mut joints = JointSet::new();
// Create the first dynamic rigid body.
let rigid_body1 = RigidBodyBuilder::new_dynamic()
.translation(vector![0.0, 2.0, 0.0])
.build();
let rigid_body1_handle = bodies.insert(rigid_body1);
// Create the second dynamic rigid body.
let rigid_body2 = RigidBodyBuilder::new_dynamic()
.translation(vector![0.0, 1.0, 0.0])
.build();
let rigid_body2_handle = bodies.insert(rigid_body2);
// Define a hinge joint by specifying local anchors and axes.
// This joint connects the two bodies and permits rotation around the specified axis.
let axis = vector![0.0, 0.0, 1.0];
let hinge = JointBuilder::new_fixed() // A fixed joint here is used as a placeholder.
.local_anchor1(point![0.0, -0.5, 0.0])
.local_anchor2(point![0.0, 0.5, 0.0])
.local_axis1(axis)
.local_axis2(axis)
.build();
joints.insert(rigid_body1_handle, rigid_body2_handle, hinge);
// Run the simulation loop for 100 time steps.
for _ in 0..100 {
physics_pipeline.step(
&gravity,
&integration_parameters,
&mut islands,
&mut broad_phase,
&mut narrow_phase,
&mut bodies,
&mut colliders,
&mut joints,
None,
&(),
);
}
let body1 = bodies.get(rigid_body1_handle).unwrap();
let body2 = bodies.get(rigid_body2_handle).unwrap();
println!("Body 1 Position: {:?}", body1.position());
println!("Body 2 Position: {:?}", body2.position());
}
In this rapier example, we set up a physics world with gravity and create two dynamic rigid bodies. We then connect them with a joint built via JointBuilder
. This example highlights rapier’s capacity to handle complex constraints and collisions, enabling simulations that combine multiple advanced phenomena with reliability and performance.
Compound Objects and Articulated Systems
Advanced simulations often involve compound objects—assemblages of rigid bodies fixed together—and articulated systems that feature multiple connected bodies capable of independent motion (such as robotic arms or skeletal models). The following example shows how to create a compound object in rapier by connecting a base body and a child body with a fixed joint:
use rapier3d::prelude::*;
fn main() {
// Initialize sets to manage rigid bodies and colliders.
let mut bodies = RigidBodySet::new();
let mut colliders = ColliderSet::new();
let mut impulse_joints = ImpulseJointSet::new();
let mut multibody_joints = MultibodyJointSet::new();
let mut ccd_solver = CCDSolver::new();
// Create a dynamic base body.
let base_body = RigidBodyBuilder::dynamic()
.translation(vector![0.0, 0.0, 0.0])
.build();
let base_handle = bodies.insert(base_body);
// Create a dynamic child body positioned relative to the base.
let child_body = RigidBodyBuilder::dynamic()
.translation(vector![1.0, 0.0, 0.0])
.build();
let child_handle = bodies.insert(child_body);
// Connect the child body to the base body using a fixed joint.
let fixed_joint = FixedJointBuilder::new()
.local_anchor1(point![1.0, 0.0, 0.0])
.local_anchor2(point![0.0, 0.0, 0.0])
.build();
impulse_joints.insert(base_handle, child_handle, fixed_joint, true);
// Set up basic simulation parameters.
let gravity = vector![0.0, -9.81, 0.0];
let mut physics_pipeline = PhysicsPipeline::new();
let integration_parameters = IntegrationParameters::default();
let mut islands = IslandManager::new();
// Instantiate a concrete BroadPhase object (using BroadPhaseMultiSap) and NarrowPhase.
let mut broad_phase = BroadPhaseMultiSap::new();
let mut narrow_phase = NarrowPhase::new();
// Run a simple simulation loop.
for _ in 0..100 {
physics_pipeline.step(
&gravity,
&integration_parameters,
&mut islands,
&mut broad_phase, // Pass the concrete broad phase.
&mut narrow_phase, // Then the narrow phase.
&mut bodies,
&mut colliders,
&mut impulse_joints,
&mut multibody_joints,
&mut ccd_solver,
None, // Optional QueryPipeline (not used here).
&(), // Default PhysicsHooks (no hooks used here).
&() // Default EventHandler (no events handled here).
);
}
let base = bodies.get(base_handle).unwrap();
let child = bodies.get(child_handle).unwrap();
println!("Base Position: {:?}", base.position());
println!("Child Position: {:?}", child.position());
}
In this simulation, the base and child bodies are connected into a compound object via a fixed joint, causing them to move as a unit. Articulated systems (where the joint allows some degree of movement) can be similarly built by selecting an appropriate joint type (e.g., hinge or slider) to match the desired constraints.
Advanced topics in rigid body dynamics—such as integrating soft body behavior, constraints, joints, and even fluid-structure interactions—expand the scope of simulations from simple rigid movements to rich, multi-physical systems. Whether you implement a basic hinge joint manually or harness the capabilities of advanced crates like rapier, understanding these interactions enables the creation of sophisticated, realistic simulations. Rust’s performance-oriented design, combined with its strong type safety and extensive ecosystem, makes it an excellent choice for pushing the boundaries of what’s possible in real-time physics simulation.
12.8. Case Study: Building a Complete Simulation
Building a complete rigid body simulation involves integrating all the techniques discussed in the previous sections. It begins with constructing basic data structures to model rigid bodies and a physics world, continues through collision detection and numerical integration, and extends into optimization, testing, and eventual expansion with additional features. The overarching goal is to create a system that accurately simulates real-world physical scenarios while remaining performant and extensible.
Testing and validation are critical at every step. By comparing simulation results with analytical solutions or experimental data, we ensure that the simulation behaves as expected—for example, checking whether trajectories match theoretical predictions or verifying that momentum conservation holds during collisions. A well-designed simulation engine must also be modular, so that different components (rigid body dynamics, collision detection, numerical integration, etc.) can be developed, tested, and optimized independently.
Below, we present a step-by-step case study: a simple simulation of a stack of blocks falling under gravity, colliding with the ground, and interacting with one another.
Step 1: Define the Basic Structures
We begin by defining the fundamental structures for rigid bodies and the physics world. The RigidBody
struct models an object with position, velocity, orientation (using quaternions), angular velocity, mass, inertia, and accumulators for force and torque. The integrate
method updates the state based on accumulated forces and torques, and the PhysicsWorld
struct manages a collection of bodies while applying gravity and stepping the simulation forward.
use nalgebra::{Vector3, UnitQuaternion};
/// A rigid body represents a physical object with translational and rotational properties.
struct RigidBody {
position: Vector3<f32>,
velocity: Vector3<f32>,
orientation: UnitQuaternion<f32>,
angular_velocity: Vector3<f32>,
mass: f32,
inertia: Vector3<f32>,
force: Vector3<f32>,
torque: Vector3<f32>,
}
impl RigidBody {
/// Creates a new rigid body with a given position and mass.
/// Other properties are initialized to zero or identity.
fn new(position: Vector3<f32>, mass: f32) -> Self {
RigidBody {
position,
velocity: Vector3::zeros(),
orientation: UnitQuaternion::identity(),
angular_velocity: Vector3::zeros(),
mass,
inertia: Vector3::new(1.0, 1.0, 1.0),
force: Vector3::zeros(),
torque: Vector3::zeros(),
}
}
/// Applies an external force at a specified point (relative to the body's center).
fn apply_force(&mut self, force: Vector3<f32>, point: Vector3<f32>) {
self.force += force;
self.torque += point.cross(&force);
}
/// Applies a simple friction model to reduce horizontal velocity.
fn apply_friction(&mut self, friction_coefficient: f32) {
self.velocity.x *= 1.0 - friction_coefficient;
self.velocity.z *= 1.0 - friction_coefficient;
}
/// Integrates the equations of motion using a simple explicit Euler method.
/// This updates both translational and rotational states.
fn integrate(&mut self, dt: f32) {
self.apply_friction(0.01); // Apply friction for more realistic behavior.
// Update linear motion.
let acceleration = self.force / self.mass;
self.velocity += acceleration * dt;
self.position += self.velocity * dt;
// Update rotational motion.
let angular_acceleration = self.inertia.component_mul(&self.torque);
self.angular_velocity += angular_acceleration * dt;
self.orientation = self.orientation *
UnitQuaternion::from_scaled_axis(self.angular_velocity * dt);
// Reset force and torque for the next step.
self.force = Vector3::zeros();
self.torque = Vector3::zeros();
}
}
/// The physics world manages all rigid bodies and applies global forces such as gravity.
struct PhysicsWorld {
bodies: Vec<RigidBody>,
gravity: Vector3<f32>,
}
impl PhysicsWorld {
/// Creates a new physics world with specified gravity.
fn new(gravity: Vector3<f32>) -> Self {
PhysicsWorld {
bodies: Vec::new(),
gravity,
}
}
/// Adds a rigid body to the simulation.
fn add_body(&mut self, body: RigidBody) {
self.bodies.push(body);
}
/// Steps the simulation forward by a time increment dt.
/// Applies gravity and then integrates each body's state.
fn step(&mut self, dt: f32) {
// Apply gravity and integrate motion for each body.
for body in &mut self.bodies {
body.apply_force(self.gravity * body.mass, Vector3::zeros());
body.integrate(dt);
// Check collision with the ground after integration.
check_collision_with_ground(body);
}
// Check and resolve collisions between bodies.
for i in 0..self.bodies.len() {
for j in i + 1..self.bodies.len() {
// Using split_at_mut ensures we get two mutable references safely.
let (left, right) = self.bodies.split_at_mut(j);
check_collision_between_bodies(&mut left[i], &mut right[0]);
}
}
}
}
use nalgebra::{Vector3, UnitQuaternion};
/// A rigid body represents a physical object with translational and rotational properties.
struct RigidBody {
position: Vector3<f32>,
velocity: Vector3<f32>,
orientation: UnitQuaternion<f32>,
angular_velocity: Vector3<f32>,
mass: f32,
inertia: Vector3<f32>,
force: Vector3<f32>,
torque: Vector3<f32>,
}
impl RigidBody {
/// Creates a new rigid body with a given position and mass.
/// Other properties are initialized to zero or identity.
fn new(position: Vector3<f32>, mass: f32) -> Self {
RigidBody {
position,
velocity: Vector3::zeros(),
orientation: UnitQuaternion::identity(),
angular_velocity: Vector3::zeros(),
mass,
inertia: Vector3::new(1.0, 1.0, 1.0),
force: Vector3::zeros(),
torque: Vector3::zeros(),
}
}
/// Applies an external force at a specified point (relative to the body's center).
fn apply_force(&mut self, force: Vector3<f32>, point: Vector3<f32>) {
self.force += force;
self.torque += point.cross(&force);
}
/// Applies a simple friction model to reduce horizontal velocity.
fn apply_friction(&mut self, friction_coefficient: f32) {
self.velocity.x *= 1.0 - friction_coefficient;
self.velocity.z *= 1.0 - friction_coefficient;
}
/// Integrates the equations of motion using a simple explicit Euler method.
/// This updates both translational and rotational states.
fn integrate(&mut self, dt: f32) {
self.apply_friction(0.01); // Apply friction for more realistic behavior.
// Update linear motion.
let acceleration = self.force / self.mass;
self.velocity += acceleration * dt;
self.position += self.velocity * dt;
// Update rotational motion.
let angular_acceleration = self.inertia.component_mul(&self.torque);
self.angular_velocity += angular_acceleration * dt;
self.orientation = self.orientation *
UnitQuaternion::from_scaled_axis(self.angular_velocity * dt);
// Reset force and torque for the next step.
self.force = Vector3::zeros();
self.torque = Vector3::zeros();
}
}
/// The physics world manages all rigid bodies and applies global forces such as gravity.
struct PhysicsWorld {
bodies: Vec<RigidBody>,
gravity: Vector3<f32>,
}
impl PhysicsWorld {
/// Creates a new physics world with specified gravity.
fn new(gravity: Vector3<f32>) -> Self {
PhysicsWorld {
bodies: Vec::new(),
gravity,
}
}
/// Adds a rigid body to the simulation.
fn add_body(&mut self, body: RigidBody) {
self.bodies.push(body);
}
/// Steps the simulation forward by a time increment dt.
/// Applies gravity and then integrates each body's state.
fn step(&mut self, dt: f32) {
// Apply gravity and integrate motion for each body.
for body in &mut self.bodies {
body.apply_force(self.gravity * body.mass, Vector3::zeros());
body.integrate(dt);
// Check collision with the ground after integration.
check_collision_with_ground(body);
}
// Check and resolve collisions between bodies.
for i in 0..self.bodies.len() {
for j in i + 1..self.bodies.len() {
// Using split_at_mut ensures we get two mutable references safely.
let (left, right) = self.bodies.split_at_mut(j);
check_collision_between_bodies(&mut left[i], &mut right[0]);
}
}
}
}
Step 2: Implement Collision Detection and Response
Next, we implement simple collision detection functions. The function check_collision_with_ground
ensures that if a body penetrates the ground (assumed to be at y = 0), its vertical position is clamped and its vertical velocity reversed, simulating a bounce with some restitution. The function check_collision_between_bodies
handles collisions between pairs of bodies using a basic impulse method based on their masses and relative velocities.
/// Detects collision between a rigid body and the ground (assumed at y = 0)
/// and applies a simple restitution response.
fn check_collision_with_ground(body: &mut RigidBody) {
if body.position.y < 0.0 {
body.position.y = 0.0;
// Reverse vertical velocity and apply a restitution coefficient.
body.velocity.y = -body.velocity.y * 0.8;
}
}
/// Detects and resolves collisions between two bodies assuming they are unit-sized blocks.
fn check_collision_between_bodies(body1: &mut RigidBody, body2: &mut RigidBody) {
let distance = (body1.position - body2.position).norm();
let min_distance = 1.0; // Assume each block has a unit size.
if distance < min_distance {
// Calculate the collision normal from body1 to body2.
let normal = (body2.position - body1.position).normalize();
let relative_velocity = body2.velocity - body1.velocity;
let separating_velocity = relative_velocity.dot(&normal);
// Only resolve if the bodies are moving toward each other.
if separating_velocity < 0.0 {
let restitution = 0.8;
let impulse = -(1.0 + restitution) * separating_velocity /
(1.0 / body1.mass + 1.0 / body2.mass);
let impulse_vector = impulse * normal;
// Adjust velocities based on the calculated impulse.
body1.velocity -= impulse_vector / body1.mass;
body2.velocity += impulse_vector / body2.mass;
}
}
}
/// Detects collision between a rigid body and the ground (assumed at y = 0)
/// and applies a simple restitution response.
fn check_collision_with_ground(body: &mut RigidBody) {
if body.position.y < 0.0 {
body.position.y = 0.0;
// Reverse vertical velocity and apply a restitution coefficient.
body.velocity.y = -body.velocity.y * 0.8;
}
}
/// Detects and resolves collisions between two bodies assuming they are unit-sized blocks.
fn check_collision_between_bodies(body1: &mut RigidBody, body2: &mut RigidBody) {
let distance = (body1.position - body2.position).norm();
let min_distance = 1.0; // Assume each block has a unit size.
if distance < min_distance {
// Calculate the collision normal from body1 to body2.
let normal = (body2.position - body1.position).normalize();
let relative_velocity = body2.velocity - body1.velocity;
let separating_velocity = relative_velocity.dot(&normal);
// Only resolve if the bodies are moving toward each other.
if separating_velocity < 0.0 {
let restitution = 0.8;
let impulse = -(1.0 + restitution) * separating_velocity /
(1.0 / body1.mass + 1.0 / body2.mass);
let impulse_vector = impulse * normal;
// Adjust velocities based on the calculated impulse.
body1.velocity -= impulse_vector / body1.mass;
body2.velocity += impulse_vector / body2.mass;
}
}
}
Step 3: Develop a Specific Scenario
We then construct a specific simulation scenario—a stack of blocks that falls under gravity and interacts with both the ground and each other. In this example, five blocks are stacked vertically with slight spacing. The simulation runs for a number of discrete time steps, and finally, the positions of the blocks are printed.
fn main() {
// Create a physics world with Earth's gravity.
let mut world = PhysicsWorld::new(Vector3::new(0.0, -9.81, 0.0));
// Create a stack of 5 blocks.
for i in 0..5 {
// Each block is positioned such that they form a stack.
let block = RigidBody::new(Vector3::new(0.0, i as f32 + 0.5, 0.0), 1.0);
world.add_body(block);
}
// Define the time step and number of simulation steps.
let dt = 0.01;
for _ in 0..500 {
world.step(dt);
}
// Output the final positions of all blocks.
for (i, body) in world.bodies.iter().enumerate() {
println!("Block {}: Position = {:?}", i, body.position);
}
}
fn main() {
// Create a physics world with Earth's gravity.
let mut world = PhysicsWorld::new(Vector3::new(0.0, -9.81, 0.0));
// Create a stack of 5 blocks.
for i in 0..5 {
// Each block is positioned such that they form a stack.
let block = RigidBody::new(Vector3::new(0.0, i as f32 + 0.5, 0.0), 1.0);
world.add_body(block);
}
// Define the time step and number of simulation steps.
let dt = 0.01;
for _ in 0..500 {
world.step(dt);
}
// Output the final positions of all blocks.
for (i, body) in world.bodies.iter().enumerate() {
println!("Block {}: Position = {:?}", i, body.position);
}
}
Step 4: Extend the Simulation with Additional Features
To add realism, you can extend the simulation with features such as friction. In this example, the apply_friction
method reduces the horizontal velocities of bodies on each integration step, simulating frictional forces that slow the motion along the ground. This extension helps ensure that the behavior of the blocks—such as their sliding and eventual settling—is more realistic.
The friction has been integrated directly into the integrate
method in our RigidBody
implementation above.
Step 5: Testing and Validation
Robust simulation requires testing and validation. One useful test is to verify conservation of momentum. For a closed system (with no external forces other than gravity, which affects all bodies equally), the total momentum should be conserved within numerical limits. For example, you might calculate the total momentum before and after the simulation to confirm that it does not change dramatically.
/// Computes the total momentum of the system by summing the momenta of all bodies.
fn total_momentum(world: &PhysicsWorld) -> Vector3<f32> {
world.bodies.iter().map(|b| b.velocity * b.mass).sum()
}
fn main() {
let mut world = PhysicsWorld::new(Vector3::new(0.0, -9.81, 0.0));
// Create a stack of blocks as before.
for i in 0..5 {
let block = RigidBody::new(Vector3::new(0.0, i as f32 + 0.5, 0.0), 1.0);
world.add_body(block);
}
let initial_momentum = total_momentum(&world);
println!("Initial total momentum: {:?}", initial_momentum);
let dt = 0.01;
for _ in 0..500 {
world.step(dt);
}
let final_momentum = total_momentum(&world);
println!("Final total momentum: {:?}", final_momentum);
}
This test prints the total momentum before and after the simulation, providing insight into whether momentum is approximately conserved, as expected.
12.8.1. Summary and Further Exploration
In this case study, we have integrated core techniques—rigid body dynamics, collision detection and response, numerical integration, and optimization—into a complete simulation of a stack of falling blocks. The simulation has been validated through simple tests such as momentum conservation and can be further extended by adding friction, damping, or more complex interactions.
This chapter has provided a comprehensive example to serve as a foundation for more advanced simulations. Rust’s performance, safety, and concurrency features make it an excellent tool for building robust simulations. Although we have demonstrated basic functionality, many areas remain ripe for exploration—such as multi-body dynamics, fluid-structure interactions, and real-time visualization using libraries like bevy.
By continuously refining and extending these techniques, you can build ever more sophisticated simulations that accurately model complex real-world systems. The journey into advanced rigid body dynamics is ongoing, and the foundation laid here will serve as a robust starting point for further exploration and innovation.
This refined case study integrates the concepts and code examples presented throughout the chapter into a single, comprehensive simulation project, setting the stage for further experimentation and advanced applications in computational physics using Rust.
12.9. Conclusion
Chapter 12 of CPVR equips readers with the theoretical knowledge and practical skills needed to simulate rigid body dynamics using Rust. By combining a deep understanding of the underlying physics with robust computational methods, readers can accurately model and analyze a wide range of physical systems, from simple mechanical structures to complex multi-body environments. The chapter lays the groundwork for advanced simulation techniques, enabling the development of high-performance applications in engineering, gaming, and virtual reality.
12.9.1. Further Learning with GenAI
Each prompt is crafted to be robust and comprehensive, encouraging exploration of fundamental concepts, mathematical frameworks, numerical methods, implementation strategies, and advanced topics.
Explain the fundamental principles of rigid body dynamics, focusing on both translational and rotational motion. How do these principles form the basis for simulating physical systems in computational physics, and what challenges arise in accurately modeling these dynamics in Rust? Discuss the mathematical foundations and the considerations needed when implementing these principles in a Rust-based simulation engine.
Derive Euler’s equations for rotational motion from first principles, detailing the role of torque, angular momentum, and the inertia tensor. How do these equations govern the motion of rigid bodies, and what specific considerations must be made when implementing these equations in Rust for stability and accuracy? Explore how numerical errors can affect the results and how Rust's type safety features can help mitigate these issues.
Discuss the advantages, challenges, and mathematical foundations of using quaternions versus Euler angles for representing rotations in rigid body dynamics. How does Rust handle quaternion operations in terms of precision, efficiency, and preventing issues like gimbal lock? Compare the implementation of quaternion and Euler angle rotations in Rust, and evaluate their performance and accuracy in complex simulations.
Analyze the relationships between angular velocity, angular acceleration, and their linear counterparts in rigid body dynamics. How can these relationships be represented mathematically, and what are the best practices for implementing these calculations in Rust, especially when considering real-time applications? Provide examples of how these calculations are used in a Rust-based simulation engine, highlighting any challenges that arise.
Evaluate various time-stepping methods, such as explicit Euler, semi-implicit Euler, and Runge-Kutta, for integrating the equations of motion in rigid body dynamics. Compare their stability, accuracy, and computational cost when implemented in Rust, and discuss how to choose the appropriate method for a given simulation scenario. Consider the trade-offs between simplicity and accuracy, and how these methods can be optimized in Rust for large-scale simulations.
Outline and critically assess the algorithms used for collision detection between rigid bodies, such as the Gilbert-Johnson-Keerthi (GJK) algorithm and the Separating Axis Theorem (SAT). How can these algorithms be efficiently implemented in Rust, and what are the trade-offs in terms of computational complexity and accuracy? Discuss the challenges of implementing these algorithms in real-time simulations, and how Rust's concurrency features can help optimize performance.
Explore the role of the inertia tensor in rigid body dynamics, detailing how it is derived, computed, and used in simulations. Discuss the challenges of dynamically updating the inertia tensor in Rust when the shape of the rigid body changes, and the implications for accurate physical simulation. Provide examples of how the inertia tensor is integrated into a Rust-based physics engine, and how it affects the overall simulation accuracy.
Develop a detailed Rust implementation of a basic rigid body simulation, focusing on the design and optimization of data structures like vectors, matrices, and quaternions. How can you ensure that these structures are both memory-efficient and capable of handling the numerical precision required for complex simulations? Discuss the challenges of balancing performance with accuracy, and how Rust's features can be leveraged to address these challenges.
Compare and contrast different algorithms for updating the state of a rigid body over time, such as Verlet integration, the leapfrog method, and the symplectic integrator. What are the specific considerations for implementing these algorithms in Rust, particularly in terms of preserving energy and momentum in the simulation? Analyze the trade-offs between these methods and provide examples of their implementation in Rust.
Discuss techniques for optimizing the performance of rigid body simulations in Rust, including parallel processing, SIMD (Single Instruction, Multiple Data) optimizations, and memory management strategies. How can these techniques be applied to handle large-scale simulations involving numerous interacting rigid bodies? Provide examples of how these optimizations can be implemented in Rust, and analyze their impact on simulation performance.
Implement a simple mechanical system, such as a pendulum or rotating disc, using Rust, and provide a detailed analysis of the simulation results. How can you validate your simulation against analytical solutions or experimental data, and what debugging techniques can be employed to ensure the accuracy of your implementation? Discuss the importance of validation in physics simulations and the role of Rust's features in achieving accurate results.
Explore the complexities of simulating multi-body systems, such as robotic arms, articulated mechanisms, or spacecraft with multiple thrusters. What additional considerations must be taken into account in Rust, and how can you efficiently handle the interactions and constraints between different bodies in the system? Provide examples of how to model and simulate these systems in Rust, and discuss the challenges of ensuring numerical stability and performance.
Discuss the implementation of constraints and joints in rigid body simulations, such as revolute, prismatic, and fixed joints. How can these constraints be modeled in Rust to ensure physically accurate behavior, and what are the challenges in maintaining numerical stability and preventing constraint drift? Provide examples of constraint implementation in Rust, and analyze the impact of these constraints on the overall simulation.
Analyze the impact of friction and contact forces in rigid body dynamics, focusing on the models used to simulate these forces and their implementation in Rust. How can you accurately simulate the effects of static and kinetic friction, and what numerical challenges arise when modeling contact forces in dynamic simulations? Discuss the importance of friction in realistic simulations and how to address the associated computational challenges in Rust.
Introduce the concept of soft-body dynamics and discuss how it differs from rigid body dynamics in terms of simulation techniques and computational complexity. How can Rust be used to implement a hybrid simulation that combines rigid and soft-body dynamics, and what are the potential applications of such simulations? Provide examples of hybrid simulations in Rust, and analyze the challenges of integrating soft-body and rigid body dynamics.
Explore the concurrency and parallelism features in Rust, such as threads, channels, and the Rayon crate, and discuss how these can be leveraged to parallelize large-scale rigid body simulations. What are the best practices for ensuring thread safety and minimizing contention in a parallelized simulation? Provide examples of parallelized simulations in Rust, and analyze the performance gains and challenges of implementing concurrency.
Develop a real-time rigid body simulation in Rust, focusing on the techniques necessary to ensure the simulation runs efficiently and accurately in an interactive application. Discuss the challenges of balancing computational load with real-time constraints and how to manage these in a Rust-based system. Provide examples of real-time simulations in Rust, and analyze the trade-offs between performance and accuracy in an interactive environment.
Investigate the tools and methods available in Rust for profiling, debugging, and optimizing rigid body simulations. How can you use these tools to identify performance bottlenecks, memory leaks, and numerical instability, and what strategies can be employed to address these issues? Provide examples of how to use Rust's profiling and debugging tools in a physics simulation, and discuss the impact of these tools on improving simulation performance and accuracy.
Discuss the techniques for visualizing rigid body dynamics in 3D using Rust, including the use of graphics libraries such as wgpu or gfx-rs. How can these techniques be integrated with physical simulations to create realistic and interactive visualizations, and what are the challenges in achieving real-time rendering? Provide examples of integrating 3D visualization with a Rust-based physics engine, and analyze the challenges of maintaining performance while rendering complex scenes.
Analyze the process of creating interactive simulations that allow users to manipulate rigid bodies in real-time. What are the key challenges in providing accurate real-time feedback, and how can Rust's performance features be leveraged to ensure responsiveness and realism in the simulation? Provide examples of interactive simulations in Rust, and discuss the techniques used to achieve real-time interactivity and accurate physical behavior.
By engaging with these prompts, you're not just learning a programming language—you're equipping yourself with the tools to model, simulate, and understand the complexities of the physical universe. Let each exercise be a step toward mastering this intricate dance between physics and computation, and take pride in the knowledge that you are building the future of scientific exploration, one line of Rust code at a time.
12.9.2. Assignments for Practice
Each exercise encourages the use of a Generative AI (GenAI) assistant to explore, implement, and refine the concepts.
Exercise 12.1: Implementing Euler’s Equations in Rust
Objective: Write a Rust program that implements Euler’s equations for rotational motion of a rigid body. Use the inertia tensor and angular momentum in your calculations.
Task: Start by deriving Euler’s equations and then translate them into Rust code. Use the GenAI assistant to refine your implementation, ensuring numerical stability and accuracy. Test your program with various initial conditions and compare the results to analytical solutions.
Challenge: Modify your implementation to handle real-time updates to the inertia tensor when the shape of the rigid body changes.
Exercise 12.2: Quaternion vs. Euler Angles for Rotation
Objective: Explore the differences between quaternions and Euler angles for representing rotations in a Rust simulation.
Task: Implement both representations in Rust and create a simulation that demonstrates their differences in handling rotations. Use the GenAI assistant to explore the mathematical foundations of each approach, particularly focusing on avoiding gimbal lock with quaternions.
Challenge: Extend your program to allow interactive rotation manipulation and observe the behavior of both methods in real-time.
Exercise 12.3: Collision Detection and Resolution
Objective: Implement a basic collision detection system using the Gilbert-Johnson-Keerthi (GJK) algorithm in Rust, followed by collision resolution.
Task: Write a Rust implementation of the GJK algorithm for detecting collisions between convex shapes. Use the GenAI assistant to help understand the intricacies of the algorithm and ensure efficient implementation. After detection, implement a simple collision resolution system that adjusts the positions and velocities of the colliding bodies.
Challenge: Optimize your collision detection for performance and extend the resolution system to handle simultaneous multiple collisions.
Exercise 12.4: Simulating Multi-Body Systems
Objective: Create a Rust simulation of a multi-body system, such as a robotic arm or articulated mechanism.
Task: Design and implement the simulation using Rust, focusing on handling the interactions and constraints between the different bodies in the system. Use the GenAI assistant to explore the mathematical models and ensure accurate constraint implementation.
Challenge: Introduce realistic forces such as gravity and friction, and ensure that the system remains stable over time, even under complex interactions.
Exercise 12.5: Real-Time Rigid Body Simulation with Parallel Processing
Objective: Develop a real-time simulation of rigid bodies in Rust, utilizing Rust’s parallel processing capabilities.
Task: Implement the simulation using Rust's concurrency features, such as threads and the
Rayon
crate, to parallelize the computation of rigid body dynamics. Use the GenAI assistant to guide you in optimizing the performance of your simulation.Challenge: Ensure that your simulation runs efficiently and accurately in real-time, and visualize the results using a graphics library like
wgpu
orgfx-rs
.
These exercises are designed to deepen your understanding of rigid body dynamics and Rust's capabilities. As you progress, experiment with different approaches and optimizations, and use GenAI to refine your solutions, ensuring that your skills grow both in technical depth and practical application.
Comments