Chapter 11
Numerical Solutions to Newtonian Mechanics
"The great advances in physics have often been driven by a shift in our ability to use mathematics to model the natural world." — Richard Feynman
Chapter 11 delves into the numerical solutions of Newtonian mechanics using Rust, offering a thorough exploration of key concepts and methods. It begins with an introduction to the fundamentals of Newtonian mechanics, highlighting how differential equations describe physical systems. The chapter progresses through numerical methods for solving these equations, including Euler’s method and Runge-Kutta methods, and integrates various techniques for numerical integration. It also covers handling systems of differential equations and introduces advanced topics such as adaptive step size methods and symplectic integrators. Each section provides practical Rust implementations and code examples, illustrating how to effectively model and solve mechanical systems computationally.
11.1. Newtonian Mechanics and Numerical Methods
Newtonian mechanics is the foundation of classical physics, describing the motion of objects under the influence of forces. The core principles include Newton's three laws of motion, which govern the relationships between the motion of an object and the forces acting upon it. Additionally, the conservation of momentum and energy are critical concepts that maintain that, in a closed system, the total momentum and energy remain constant unless acted upon by external forces. These principles allow us to model and predict the behavior of mechanical systems.
While these principles provide a strong theoretical framework, solving complex mechanical systems analytically can be challenging or even impossible. Analytical solutions, which involve deriving exact solutions using algebra and calculus, are often limited to simple systems with a small number of degrees of freedom. However, many real-world problems, such as the motion of multiple interacting bodies or systems with non-linear forces, do not yield to such straightforward analytical techniques. This limitation necessitates the use of numerical methods, which approximate the solution of differential equations by discretizing them and solving them iteratively.
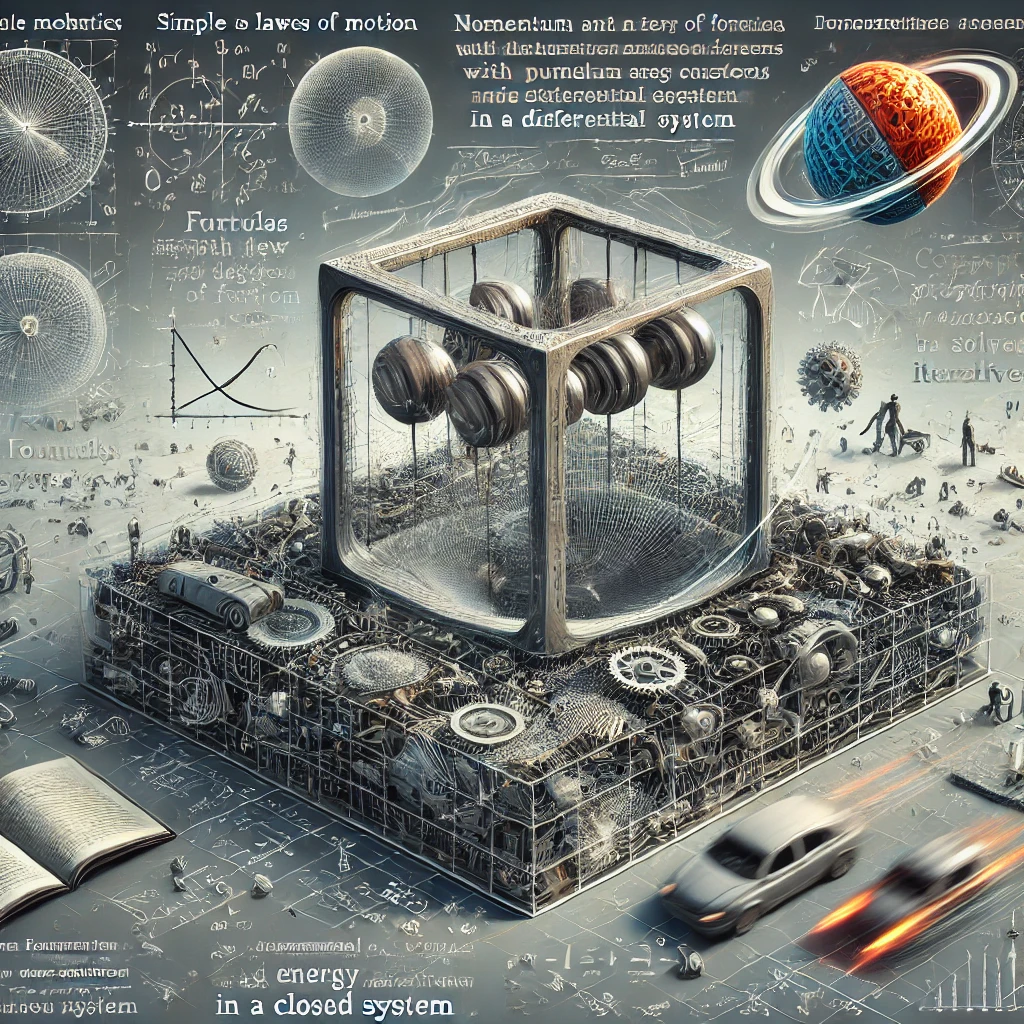
DALL-E generated image for Newtonian mechanics.
Numerical methods bridge the gap between theory and practice by providing a way to simulate the behavior of complex systems over time. Unlike analytical solutions, which produce an exact answer, numerical solutions offer an approximate result that becomes more accurate as the computational power and refinement of the method increase. The trade-off is that numerical methods require careful consideration of stability, precision, and computational cost to ensure that the results are both reliable and efficient.
There are many situations in computational physics where analytical solutions are impractical or impossible to obtain. For instance, systems with many interacting particles, such as the N-body problem in celestial mechanics, are notorious for their complexity. Similarly, systems with non-linear forces or those that involve chaotic behavior cannot be solved analytically and require numerical approaches. In these cases, numerical methods become indispensable tools.
One key concept in numerical methods is the idea of numerical stability. Stability refers to the method's ability to control the propagation of errors during computation. An unstable numerical method can cause small errors to grow exponentially, leading to incorrect results. Precision, on the other hand, relates to the degree of accuracy of the numerical solution. Precision is influenced by factors such as the choice of algorithm, the size of the time step, and the discretization of space. Together, stability and precision determine the reliability of the numerical solution.
Another important consideration is the discretization of time and space. In numerical methods, continuous time and space are divided into discrete intervals. For example, time is often divided into small time steps, and space can be represented as a grid of points. This discretization allows the differential equations governing the system to be approximated by algebraic equations that can be solved iteratively. The choice of time step and spatial resolution is crucial; too large a time step can lead to inaccurate results, while too small a time step can increase computational cost without significantly improving accuracy.
Rust is an ideal language for implementing numerical methods due to its emphasis on safety, concurrency, and performance. It provides powerful libraries and tools that are well-suited for numerical computations in physics.
To start with, let’s consider a simple example: solving the motion of a single particle under a constant force using the Euler method, one of the simplest numerical integration techniques. The Euler method approximates the solution of the differential equation by taking small steps along the solution curve.
The following Rust implementation demonstrates the motion of a single particle under a constant force using the Euler method. In this simulation, a particle with a specified mass experiences a constant force, and its velocity and position are updated at each time step based on basic kinematic equations derived from Newton’s second law (F = ma).
// Define the parameters for the simulation.
const DT: f64 = 0.01; // Time step for simulation updates.
const NUM_STEPS: usize = 1000; // Total number of simulation steps.
const MASS: f64 = 1.0; // Mass of the particle.
const FORCE: f64 = 10.0; // Constant force acting on the particle.
fn main() {
// Initialize the particle's initial position and velocity.
let mut position = 0.0;
let mut velocity = 0.0;
// Perform the simulation over a fixed number of time steps.
for step in 0..NUM_STEPS {
// Update the velocity using the acceleration (Force / Mass).
velocity += (FORCE / MASS) * DT;
// Update the position using the current velocity.
position += velocity * DT;
// Output the current time, position, and velocity.
println!(
"Time: {:.2}, Position: {:.5}, Velocity: {:.5}",
DT * step as f64, position, velocity
);
}
}
In the code above, the particle’s motion is simulated by iteratively updating its velocity and position. The velocity is updated based on the constant acceleration derived from the force acting on the particle, while the position is updated by integrating the velocity over the time step. Each iteration corresponds to a small increment of time (DT), and the loop runs for NUM_STEPS iterations, simulating the particle's motion over the given duration. This straightforward approach, employing the Euler method, demonstrates how numerical integration techniques can model the dynamic evolution of mechanical systems where analytical solutions are difficult or impossible to obtain.
We initialize the particle’s position and velocity to zero. The simulation then runs for NUM_STEPS
iterations. In each iteration, we update the velocity using the formula velocity += (FORCE / MASS) DT
, which is derived from Newton’s second law, $F = ma$. The position is then updated using position += velocity DT
. This approach is a direct application of the Euler method, where we incrementally update the state of the system based on the current rate of change.
Finally, the program prints the current time, position, and velocity at each step. This output can be used to analyze the motion of the particle over time. Although this example is simple, it illustrates the core principles of numerical integration and how they can be implemented in Rust.
This Rust implementation showcases how numerical methods can be used to simulate the motion of a particle under a constant force. By adjusting the parameters, such as the time step or the number of steps, we can explore how these changes affect the accuracy and stability of the simulation. This example serves as a foundation for more complex simulations that involve multiple particles, non-linear forces, and more sophisticated numerical techniques.
11.2. Euler Method
The Euler method is one of the simplest numerical integration techniques used to solve ordinary differential equations (ODEs), making it particularly useful in the context of Newtonian mechanics. At its core, the Euler method is a straightforward iterative procedure that approximates the solution of a differential equation by using the slope at the current point to estimate the value at the next point. This method is derived directly from Newton's second law of motion, which states that the force acting on an object is equal to the mass of the object multiplied by its acceleration (F=maF = maF=ma). Acceleration, being the derivative of velocity, can be integrated to find the velocity, and similarly, velocity can be integrated to find the position.
The Euler method begins with an initial condition—such as the initial position and velocity of a particle—and updates these values incrementally over small time steps. The basic idea is to use the current velocity to estimate the new position and then use the current acceleration to update the velocity. Mathematically, if $v(t)$ is the velocity at time $t$ and $x(t)$ is the position at time $t$, the Euler method updates these quantities as follows:
$$ v(t + \Delta t) = v(t) + a(t) \cdot \Delta t $$
$$x(t + \Delta t) = x(t) + v(t) \cdot \Delta t$$
where $\Delta t$ is the time step and $a(t)$ is the acceleration at time $t$.
This method is particularly useful for solving simple mechanical systems, such as projectile motion, where the equations of motion are straightforward, and the forces involved are constant or vary in a predictable manner. For example, in projectile motion, the only force acting on the object is gravity, which provides a constant acceleration. Similarly, in the case of a simple harmonic oscillator, the restoring force is proportional to the displacement, making it a suitable candidate for the Euler method.
While the Euler method is easy to understand and implement, it has several limitations, particularly in terms of accuracy and stability. The method is only first-order accurate, meaning the error in the solution is proportional to the time step $\Delta t$. As a result, smaller time steps are required to achieve higher accuracy, but this comes at the cost of increased computational effort. Additionally, the Euler method is prone to error propagation. In each step, a small error introduced due to the approximation can grow over time, especially in long simulations, leading to significant deviations from the true solution.
One critical aspect of using the Euler method is the selection of the time step $\Delta t$. A time step that is too large can result in significant inaccuracies, as the method assumes that the velocity and acceleration remain constant over each interval, which is often not the case in real-world systems. Conversely, a very small time step can improve accuracy but will require more iterations, increasing the computational load. Balancing these factors is essential for obtaining reliable results.
When compared to other numerical methods, such as the Runge-Kutta methods, the Euler method is computationally efficient because of its simplicity. However, this efficiency comes at the expense of accuracy and stability. For problems where high precision is not required, or where computational resources are limited, the Euler method might be sufficient. However, for more complex or sensitive systems, more advanced methods are usually preferred.
Implementing the Euler method in Rust provides an excellent opportunity to explore efficient and safe code practices in a systems programming language. Rust’s emphasis on memory safety and concurrency makes it well-suited for performing numerical simulations.
The following Rust implementation demonstrates the Euler method by simulating the motion of a projectile under the influence of gravity. In this example, the simulation begins with an initial position and velocity, and the Euler method is used to update these values over a series of time steps.
// Constants for the simulation
const DT: f64 = 0.01; // Time step (in seconds)
const NUM_STEPS: usize = 1000; // Number of time steps in the simulation
const GRAVITY: f64 = -9.81; // Acceleration due to gravity (m/s^2)
const INITIAL_VELOCITY: f64 = 50.0; // Initial velocity (m/s)
const LAUNCH_ANGLE: f64 = 45.0; // Launch angle (degrees)
fn main() {
// Convert launch angle from degrees to radians
let launch_angle_rad = LAUNCH_ANGLE.to_radians();
// Initialize the projectile's initial position (x, y) and its velocity components (vx, vy)
let mut x = 0.0;
let mut y = 0.0;
// Compute horizontal and vertical velocity components using the cosine and sine of the launch angle
let mut vx = INITIAL_VELOCITY * launch_angle_rad.cos();
let mut vy = INITIAL_VELOCITY * launch_angle_rad.sin();
// Simulation loop: iteratively update the projectile's state over time using the Euler method
for step in 0..NUM_STEPS {
// Update the position based on the current velocity
x += vx * DT;
y += vy * DT;
// Update the vertical velocity considering the constant acceleration due to gravity
vy += GRAVITY * DT;
// Output the current time, position, and velocity components
println!("Time: {:.2}, X: {:.2}, Y: {:.2}, VX: {:.2}, VY: {:.2}",
step as f64 * DT, x, y, vx, vy);
// Break the loop if the projectile has hit the ground (y < 0)
if y < 0.0 {
break;
}
}
}
In this code, we start by defining the constants for the simulation: the time step $\Delta t$, the number of steps to simulate, the acceleration due to gravity, the initial velocity of the projectile, and the launch angle. The launch angle is converted from degrees to radians, as trigonometric functions in Rust operate on radians.
The initial position of the projectile is set to $(x, y) = (0, 0)$, and the initial velocity components $v_x$ and $v_y$ are calculated using the cosine and sine of the launch angle, respectively. The simulation loop then iteratively updates the position and velocity of the projectile using the Euler method.
At each time step, the position is updated based on the current velocity, and the velocity in the y-direction is updated by adding the product of gravity and the time step. The x-direction velocity remains constant because no horizontal forces are acting on the projectile. After updating the position and velocity, the program prints the current time, position, and velocity components. The loop breaks if the projectile hits the ground (i.e., when $y < 0$).
This simple simulation demonstrates the Euler method in action. The accuracy of the results can be adjusted by changing the time step $\Delta t$. Smaller time steps will yield more accurate results at the cost of additional computations, while larger time steps will make the simulation run faster but with less precision.
In a real-world application, this method could be extended to simulate more complex systems, such as multiple projectiles, air resistance, or varying gravitational fields. Rust’s strong type system, ownership model, and error handling make it a powerful tool for developing robust and safe numerical simulations, ensuring that resources are managed correctly and that potential errors are caught at compile time.
This implementation of the Euler method in Rust is not only efficient but also highlights the importance of understanding the limitations and appropriate use cases for this numerical technique. By experimenting with different time steps and initial conditions, one can gain a deeper insight into the dynamics of the system being simulated and the trade-offs involved in numerical computation.
11.3. Improved Euler and Heun’s Methods
The Improved Euler method, also known as the midpoint method or the second-order Runge-Kutta method, and Heun’s method, are enhancements of the basic Euler method. These methods are designed to provide greater accuracy and stability while still maintaining a relatively simple computational approach. The key idea behind these methods is to correct the simple forward Euler step by incorporating additional information about the system’s behavior within each time step.
In the basic Euler method, the next value of the solution is estimated by taking a step based on the derivative (slope) at the current point. This can lead to significant errors, especially when the function’s behavior changes rapidly. The Improved Euler method addresses this by taking an initial estimate of the slope at the beginning of the interval (as in the basic Euler method), then calculating the slope at the midpoint of the interval using the initial estimate to get a better prediction of the slope. The final estimate is obtained by averaging these two slopes.
First, calculate the intermediate values using the initial slope:
$$v_{\text{mid}} = v(t) + \frac{\Delta t}{2} \cdot a(t)$$
$$x_{\text{mid}} = x(t) + \frac{\Delta t}{2} \cdot v(t)$$
Then, use these intermediate values to compute the next step:
$$v(t + \Delta t) = v(t) + \Delta t \cdot a(t_{\text{mid}})$$
$$x(t + \Delta t) = x(t) + \Delta t \cdot v_{\text{mid}}$$
Heun’s method takes a slightly different approach. It is often considered a predictor-corrector method where an initial prediction (using the Euler method) is corrected by taking the average of the initial slope and the slope at the end of the interval. This leads to an equation similar to the Improved Euler method but with a different way of combining the slope estimates.
The application of these methods is particularly advantageous in more complex mechanical systems, where the dynamics are not well-captured by the basic Euler method. For example, systems with non-linear forces or those where higher accuracy is needed without a significant increase in computational cost benefit greatly from these methods.
One of the key advantages of the Improved Euler and Heun’s methods over the basic Euler method is the reduction of local truncation error. In the Euler method, the error per step is proportional to the square of the time step $\Delta t$, leading to cumulative errors that can significantly affect the accuracy of the solution over time. The Improved Euler and Heun’s methods, being second-order methods, have local truncation errors proportional to $\Delta t^3$, which means that the cumulative error grows more slowly as the simulation progresses.
This improvement in accuracy comes with a trade-off in computational complexity. Both methods require additional function evaluations per step (calculating the slope at an intermediate point or using a predictor-corrector step), which increases the computational cost. However, the gain in accuracy often justifies this cost, particularly in systems where precision is crucial.
The conditions under which these methods outperform the basic Euler method generally involve scenarios where the system’s dynamics change rapidly within each time step or where long-term accuracy is important. For instance, in simulations of oscillatory systems like simple harmonic oscillators or in scenarios involving non-linear forces, these methods provide a more accurate and stable solution compared to the basic Euler method.
Implementing the Improved Euler and Heun’s methods in Rust involves handling the additional computation steps while ensuring that the code remains efficient and safe. Let’s consider the same projectile motion problem but now using the Improved Euler method to see how it enhances accuracy.
// Constants for the simulation
const DT: f64 = 0.01; // Time step (in seconds)
const NUM_STEPS: usize = 1000; // Number of time steps
const GRAVITY: f64 = -9.81; // Acceleration due to gravity (m/s^2)
const INITIAL_VELOCITY: f64 = 50.0; // Initial velocity (m/s)
const LAUNCH_ANGLE: f64 = 45.0; // Launch angle (degrees)
fn main() {
// Convert launch angle to radians since trigonometric functions in Rust use radians
let launch_angle_rad = LAUNCH_ANGLE.to_radians();
// Initialize the projectile's position and velocity components
let mut x = 0.0;
let mut y = 0.0;
let mut vx = INITIAL_VELOCITY * launch_angle_rad.cos();
let mut vy = INITIAL_VELOCITY * launch_angle_rad.sin();
// Simulation loop using the Improved Euler method
for step in 0..NUM_STEPS {
// Calculate intermediate (midpoint) values using the initial slope
let vx_mid = vx; // For constant horizontal velocity, the value remains the same.
let vy_mid = vy + GRAVITY * DT / 2.0; // Midpoint vertical velocity
let x_mid = x + vx * DT / 2.0; // Midpoint position in x-direction
let y_mid = y + vy * DT / 2.0; // Midpoint position in y-direction
// Update velocity: only the vertical component is affected by gravity
vy += GRAVITY * DT;
// Update position using the midpoint values
x += vx_mid * DT;
y += vy_mid * DT;
// Output the current state of the projectile
println!(
"Time: {:.2}, X: {:.2}, Y: {:.2}, VX: {:.2}, VY: {:.2}",
step as f64 * DT, x, y, vx, vy
);
// Break if the projectile hits the ground (y becomes negative)
if y < 0.0 {
break;
}
}
}
In this implementation, we start similarly to the basic Euler method by defining the constants and initial conditions for the projectile. The main difference comes in the simulation loop, where we first calculate the intermediate values for velocity and position at the midpoint of the time step. This is done by taking the initial velocity and updating it halfway through the time step to get $v_{\text{mid}}$, and similarly for the position $x_{\text{mid}}$ and $y_{\text{mid}}$.
Once the midpoint values are computed, they are used to update the final position and velocity. By using the midpoint values rather than the initial ones, the Improved Euler method provides a better estimate of the system's state at the next time step, resulting in a more accurate simulation.
This code demonstrates how the Improved Euler method reduces error compared to the basic Euler method. By incorporating the additional information from the midpoint, the method effectively balances accuracy with computational efficiency.
For Heun’s method, the implementation would involve predicting the next state using the basic Euler method and then correcting this prediction by averaging the slope at the beginning and end of the interval. The structure of the code would be similar, with adjustments to how the final position and velocity are calculated.
// Constants for the simulation
const DT: f64 = 0.01; // Time step (in seconds)
const NUM_STEPS: usize = 1000; // Number of time steps
const GRAVITY: f64 = -9.81; // Acceleration due to gravity (m/s^2)
const INITIAL_VELOCITY: f64 = 50.0; // Initial velocity (m/s)
const LAUNCH_ANGLE: f64 = 45.0; // Launch angle (degrees)
fn main() {
// Convert launch angle from degrees to radians.
let launch_angle_rad = LAUNCH_ANGLE.to_radians();
// Initialize the projectile's starting position and velocity components.
let mut x = 0.0;
let mut y = 0.0;
let mut vx = INITIAL_VELOCITY * launch_angle_rad.cos();
let mut vy = INITIAL_VELOCITY * launch_angle_rad.sin();
// Simulation loop using Heun's method
for step in 0..NUM_STEPS {
// Predictor step: estimate next position and velocity using Euler's method.
let x_euler = x + vx * DT;
let y_euler = y + vy * DT;
let vy_euler = vy + GRAVITY * DT;
// Corrector step: update position and velocity by averaging the slopes.
// For the x-direction, the slope remains constant.
x += (vx + vx) * DT / 2.0;
// For the y-direction, average the current slope and the predicted slope.
y += (vy + vy_euler) * DT / 2.0;
// Update the vertical velocity by averaging the effect of gravity.
vy += (GRAVITY + GRAVITY) * DT / 2.0;
// Output the current state of the simulation.
println!(
"Time: {:.2}, X: {:.2}, Y: {:.2}, VX: {:.2}, VY: {:.2}",
step as f64 * DT, x, y, vx, vy
);
// Terminate if the projectile hits the ground.
if y < 0.0 {
break;
}
}
}
In Heun’s method, the code first predicts the position and velocity at the next time step using the Euler method. Then, these predicted values are used to correct the initial estimate by averaging the slopes at the current and predicted points, providing a more accurate result.
These implementations illustrate the key advantages of the Improved Euler and Heun’s methods. By refining the slope calculation within each time step, these methods offer significant improvements in accuracy over the basic Euler method, particularly in systems where precision is critical. Furthermore, Rust’s strong typing and ownership model ensure that these simulations are both efficient and safe, making it easier to handle larger and more complex systems without sacrificing performance.
11.4. Verlet Integration and Leapfrog Method
Verlet integration is a powerful numerical method used in the simulation of Newtonian mechanics, particularly in systems where long-term stability and conservation of energy and momentum are critical. This method is especially valued in computational physics for its ability to maintain these physical properties over time, making it ideal for simulating systems such as orbital mechanics or molecular dynamics.
The basic idea behind Verlet integration is to calculate positions and velocities at staggered time points. This method provides a more accurate approximation of the trajectory of a system by using both the current and previous positions to determine the next position. There are several variants of the Verlet method, including the standard Verlet, velocity Verlet, and leapfrog methods, each offering different advantages depending on the system being simulated.
The leapfrog method, a variant of Verlet integration, is named for the way it "leaps" over the velocity at each time step, updating positions and velocities in a staggered fashion. This staggered update is key to the method's ability to preserve the symplectic nature of the equations of motion, which is crucial for maintaining the system's energy and momentum over long simulations.
In orbital mechanics, for instance, where planets orbit a star over long periods, preserving energy and angular momentum is vital for accuracy. The leapfrog method excels in such scenarios because it minimizes the accumulation of numerical errors that can otherwise lead to significant deviations from the true orbit over time.
The symplectic nature of Verlet integration and its variants refers to the method's ability to preserve the geometric properties of the Hamiltonian systems, which describe the evolution of a mechanical system in phase space. A symplectic integrator, like Verlet, ensures that the phase space volume is conserved, leading to better long-term stability and accuracy in simulations of conservative systems, where total energy remains constant.
This contrasts with non-symplectic methods, such as the Euler or Runge-Kutta methods, which can lead to gradual drifts in energy and momentum, especially in long-term simulations. The stability of Verlet integration makes it particularly suitable for systems where maintaining the correct energy distribution is essential, such as in molecular dynamics simulations, where atoms or molecules interact over long periods.
Verlet integration's error characteristics are also noteworthy. While the method has a local error of $O(\Delta t^2)$, its global error is $O(\Delta t^2)$, meaning that the accuracy improves significantly with smaller time steps. More importantly, the method’s long-term behavior is superior to many other methods, as it does not suffer from energy drift, making it ideal for simulating systems over extended periods.
To illustrate the practical application of Verlet integration and the leapfrog method, let's implement these methods in Rust to simulate the orbit of a planet around a star, a classic problem in orbital mechanics.
First, we will implement the standard Verlet method. In this example, we simulate a two-dimensional orbit where a planet moves under the gravitational pull of a star.
use std::f64::consts::PI;
// Constants for the simulation
const DT: f64 = 0.01; // Time step (in years)
const G: f64 = 4.0 * PI * PI; // Gravitational constant (AU^3 / year^2 / solar mass)
const NUM_STEPS: usize = 10000; // Number of simulation steps
fn main() {
// Initial conditions for a circular orbit:
// position (x, y) in astronomical units (AU) and velocity (vx, vy) in AU/year.
let mut x = 1.0;
let mut y = 0.0;
let mut vx = 0.0;
let mut vy = 2.0 * PI; // Velocity for a circular orbit
// For Verlet integration, we need the previous position.
// We estimate the previous position using a backward Euler step.
let mut prev_x = x - vx * DT;
let mut prev_y = y - vy * DT;
// Simulation loop using the Verlet method
for _ in 0..NUM_STEPS {
// Compute the distance from the origin (the star's position).
let r = (x * x + y * y).sqrt();
// Calculate acceleration components using Newton's law of gravitation.
let ax = -G * x / (r * r * r);
let ay = -G * y / (r * r * r);
// Compute new positions using the Verlet integration formula.
let new_x = 2.0 * x - prev_x + ax * DT * DT;
let new_y = 2.0 * y - prev_y + ay * DT * DT;
// Update previous positions for the next step.
prev_x = x;
prev_y = y;
// Update current position with the newly computed values.
x = new_x;
y = new_y;
// Output the current state for analysis.
println!("X: {:.5}, Y: {:.5}", x, y);
}
}
In this implementation, we start by defining constants such as the time step $\Delta t$, the gravitational constant $G$ (in astronomical units, years, and solar masses), and the number of simulation steps. The initial position and velocity of the planet are set to create a simple circular orbit.
The Verlet method calculates the new position of the planet using the current and previous positions. The acceleration components $ax$ and $ay$ are determined based on Newton’s law of gravitation, where the force is proportional to the inverse square of the distance rrr between the planet and the star. The new position is then calculated using the formula:
$$x_{\text{new}} = 2x - x_{\text{prev}} + a_x \Delta t^2$$
$$y_{\text{new}} = 2y - y_{\text{prev}} + a_y \Delta t^2$$
This method inherently conserves momentum and energy, making it well-suited for long-term simulations of orbital mechanics.
Next, let’s implement the leapfrog method, which improves upon the Verlet method by providing a more stable way to update velocities and positions.
use std::f64::consts::PI;
// Constants for the simulation
const DT: f64 = 0.01; // Time step (in years)
const G: f64 = 4.0 * PI * PI; // Gravitational constant (AU^3 / year^2 / solar mass)
const NUM_STEPS: usize = 10000; // Number of simulation steps
fn main() {
// Initial conditions for the orbit: position (x, y) and velocity (vx, vy).
let mut x = 1.0;
let mut y = 0.0;
let mut vx = 0.0;
let mut vy = 2.0 * PI; // Velocity to maintain a circular orbit
// Update velocity by half a time step to initialize the leapfrog method.
let r = (x * x + y * y).sqrt();
let ax = -G * x / (r * r * r);
let ay = -G * y / (r * r * r);
vx += ax * DT / 2.0;
vy += ay * DT / 2.0;
// Simulation loop using the leapfrog method
for _ in 0..NUM_STEPS {
// Update position using the half-step velocity.
x += vx * DT;
y += vy * DT;
// Recalculate the acceleration at the new position.
let r = (x * x + y * y).sqrt();
let ax = -G * x / (r * r * r);
let ay = -G * y / (r * r * r);
// Update the velocity by a full time step using the new acceleration.
vx += ax * DT;
vy += ay * DT;
// Output the updated position for analysis.
println!("X: {:.5}, Y: {:.5}", x, y);
}
}
In the leapfrog method, we begin by updating the velocity components $v_x$ and $v_y$ by half a time step to synchronize the velocity with the position update. This is crucial for maintaining the method’s stability and energy conservation properties.
The position is then updated using the half-step velocity, followed by a full update of the velocity using the new position. The leapfrog method effectively "leaps" over the position to update the velocity, then uses this new velocity to update the position in the next iteration. This staggered update process is what gives the leapfrog method its name and its symplectic properties.
The leapfrog method is particularly advantageous in large-scale simulations, such as simulating the orbits of multiple planets or particles in molecular dynamics. Its ability to maintain the system’s total energy over time makes it ideal for long-term simulations, where small numerical errors could otherwise accumulate and destabilize the system.
When implementing these methods in Rust, there are several techniques you can use to optimize the code for large-scale simulations. One approach is to use Rust’s ownership and borrowing system to avoid unnecessary memory allocations, which can be crucial when dealing with large numbers of particles or planets.
For example, instead of recalculating the distance $r$ in every iteration, you can optimize the code by storing and reusing intermediate values where possible. Additionally, leveraging Rust’s concurrency features, such as threads or async, can help distribute the computation across multiple CPU cores, significantly speeding up the simulation.
By using Rust’s strong type system and memory safety guarantees, you can also ensure that your simulation remains free from common errors such as data races or memory leaks, which are particularly important in simulations that run over long periods or involve complex interactions between many objects.
These optimizations, combined with the inherent stability of Verlet and leapfrog methods, make Rust an excellent choice for implementing large-scale simulations in computational physics, allowing you to accurately model complex systems while maintaining high performance and reliability.
11.5. Runge-Kutta Methods
The Runge-Kutta methods are a family of iterative techniques used to solve ordinary differential equations (ODEs). Among these, the fourth-order Runge-Kutta method, commonly referred to as RK4, is particularly popular due to its balance between accuracy and computational efficiency. RK4 is widely used in various fields, including computational physics, due to its ability to handle complex systems with nonlinear dynamics effectively.
The RK4 method is derived from the Taylor series expansion, aiming to approximate the solution of ODEs with higher accuracy than simpler methods like Euler’s. While the Euler method uses only the slope at the beginning of the time step to estimate the next value, RK4 considers multiple evaluations of the slope at different points within the time step. This results in a more accurate approximation of the solution, especially for systems with rapidly changing dynamics.
Mathematically, the RK4 method can be expressed as follows:
Calculate the slopes $(k1, k2, k3, k4)$ at different points within the time step:
$$k_1 = f(t_n, y_n)$$
$$k_2 = f\left(t_n + \frac{\Delta t}{2}, y_n + \frac{k_1 \Delta t}{2}\right)$$
$$k_3 = f\left(t_n + \frac{\Delta t}{2}, y_n + \frac{k_2 \Delta t}{2}\right)$$
$$k_4 = f(t_n + \Delta t, y_n + k_3 \Delta t)$$
-Combine these slopes to calculate the next value of the solution:
$$y_{n+1} = y_n + \frac{\Delta t}{6} (k_1 + 2k_2 + 2k_3 + k_4)$$
This method effectively averages the slopes at different points, giving a more accurate prediction of the system’s state at the next time step. The RK4 method is particularly well-suited for complex mechanical systems, such as those with nonlinear forces or where interactions between multiple components lead to chaotic behavior.
One of the primary advantages of the RK4 method is its balance between accuracy and computational cost. While higher-order methods, like RK4, require more function evaluations per step compared to simpler methods like Euler’s, they achieve significantly better accuracy for a given step size. This makes RK4 an efficient choice for many problems in computational physics, where accuracy is critical but computational resources are limited.
Another key aspect of RK4 is its ability to handle stiff systems more effectively than lower-order methods. A stiff system is one where certain components evolve much faster than others, leading to numerical difficulties if not handled properly. RK4 can mitigate some of these difficulties by using a more sophisticated approach to estimate the system’s evolution over each time step. However, in very stiff systems, even RK4 might struggle, and adaptive step-size control becomes important. Adaptive step-size control dynamically adjusts the time step based on the system's behavior, ensuring stability and accuracy without unnecessarily small time steps.
When compared to lower-order methods, RK4 offers a significant improvement in accuracy, particularly for systems with nonlinear dynamics. While the Euler method or even the Improved Euler method might be sufficient for simple or linear systems, RK4 shines in more complex scenarios. It provides a robust and reliable way to simulate the behavior of mechanical systems where small errors can quickly accumulate, leading to incorrect results.
Implementing the RK4 method in Rust involves several steps, including defining the system's state, calculating derivatives, and updating the state using the RK4 algorithm. Let’s consider an example of a nonlinear system: the simulation of a simple pendulum, which exhibits nonlinear dynamics due to the sine function in its governing equations.
The equation of motion for a simple pendulum is given by:
$$\frac{d^2\theta}{dt^2} = -\frac{g}{L} \sin(\theta)$$
Where: $\theta$ is the angular displacement, $g$ is the acceleration due to gravity, $L$ is the length of the pendulum.
This second-order differential equation can be broken down into two first-order equations:
$$\frac{d\theta}{dt} = \omega$$
$$\frac{d\omega}{dt} = -\frac{g}{L} \sin(\theta)$$
Here’s how you can implement the RK4 method for this system in Rust:
use std::f64::consts::PI;
const G: f64 = 9.81; // Acceleration due to gravity (m/s^2)
const L: f64 = 1.0; // Length of the pendulum (m)
const DT: f64 = 0.01; // Time step (s)
const NUM_STEPS: usize = 1000; // Number of steps
struct Pendulum {
theta: f64, // Angular displacement (rad)
omega: f64, // Angular velocity (rad/s)
}
impl Pendulum {
fn new(theta: f64, omega: f64) -> Self {
Pendulum { theta, omega }
}
/// Computes the derivatives dθ/dt and dω/dt at the current state.
fn derivatives(&self) -> (f64, f64) {
(self.omega, -G / L * self.theta.sin())
}
/// Updates the pendulum state using the RK4 method.
fn update(&mut self, dt: f64) {
// Calculate first slope (k1)
let (k1_theta, k1_omega) = self.derivatives();
// Estimate state at midpoint using k1
let mid_state1 = Pendulum {
theta: self.theta + 0.5 * k1_theta * dt,
omega: self.omega + 0.5 * k1_omega * dt,
};
let (k2_theta, k2_omega) = mid_state1.derivatives();
// Calculate second midpoint slope using k2
let mid_state2 = Pendulum {
theta: self.theta + 0.5 * k2_theta * dt,
omega: self.omega + 0.5 * k2_omega * dt,
};
let (k3_theta, k3_omega) = mid_state2.derivatives();
// Estimate state at the end of the interval using k3
let end_state = Pendulum {
theta: self.theta + k3_theta * dt,
omega: self.omega + k3_omega * dt,
};
let (k4_theta, k4_omega) = end_state.derivatives();
// Combine all slopes to update the state.
self.theta += dt / 6.0 * (k1_theta + 2.0 * k2_theta + 2.0 * k3_theta + k4_theta);
self.omega += dt / 6.0 * (k1_omega + 2.0 * k2_omega + 2.0 * k3_omega + k4_omega);
}
}
fn main() {
// Initialize the pendulum with a 45° (π/4 rad) displacement and zero initial velocity.
let mut pendulum = Pendulum::new(PI / 4.0, 0.0);
// Run the simulation and update the pendulum state using the RK4 method.
for step in 0..NUM_STEPS {
pendulum.update(DT);
println!("Time: {:.2}, Theta: {:.5}, Omega: {:.5}", step as f64 * DT, pendulum.theta, pendulum.omega);
}
}
In this implementation, the Pendulum
struct represents the state of the pendulum, with fields for the angular displacement $\theta$ and angular velocity $\omega$. The derivatives
method computes the derivatives $\frac{d\theta}{dt}$ and $\frac{d\omega}{dt}$, which correspond to the current angular velocity and the angular acceleration, respectively.
The update
method implements the RK4 algorithm. It calculates the four slopes $(k_1, k_2, k_3, k_4)$ at different points within the time step. These slopes are then combined to update the state of the pendulum at the next time step. This method provides a robust and accurate way to simulate the pendulum’s motion, even in the presence of nonlinear dynamics.
The main function initializes the pendulum with a 45-degree angle and no initial velocity. It then runs the simulation for a specified number of steps, printing the pendulum’s state at each step.
When implementing the RK4 method in Rust, several optimizations can be applied to enhance performance and numerical stability. For example, using Rust’s f64
type ensures high precision in floating-point calculations, which is essential for maintaining accuracy in simulations involving small time steps.
To further optimize the code, you can leverage Rust’s ownership model to minimize memory allocations and avoid unnecessary copies of data. The derivatives
and update
methods can be designed to borrow rather than copy the pendulum state, reducing overhead and improving efficiency.
For large-scale simulations, such as solving chaotic systems or coupled oscillators, Rust’s concurrency features can be employed to distribute the computational load across multiple CPU cores. Using libraries like Rayon, you can parallelize the RK4 computations, significantly speeding up the simulation while maintaining the accuracy and robustness of the RK4 method.
As a case study, consider simulating a system of coupled oscillators using the RK4 method. Each oscillator’s motion is influenced by its neighbors, leading to complex, often chaotic, behavior. Implementing this in Rust with RK4 allows you to accurately capture the system’s dynamics while taking advantage of Rust’s performance and safety features.
In summary, the RK4 method is a powerful tool in computational physics for solving complex mechanical systems with nonlinear dynamics. Its implementation in Rust not only ensures accuracy and stability but also leverages Rust’s strengths in performance and safety, making it an excellent choice for large-scale and long-term simulations in various domains of physics.
11.6. Adaptive Step-Size Control
Adaptive step-size control is a crucial technique in numerical integration, particularly when dealing with complex systems where dynamics can vary significantly over time. Unlike fixed step-size methods—where the time step Δt\\Delta t remains constant—the adaptive approach dynamically adjusts $\Delta t$ based on the system's behavior. This adaptive strategy enables the numerical solver to take larger steps when the solution is smooth and reduce the step size in regions of rapid change, thereby enhancing both accuracy and efficiency.
One of the most well-known adaptive algorithms is the Dormand-Prince method, a variant of the Runge-Kutta method. This technique not only provides an estimate of the solution at each step but also computes an estimate of the local truncation error. Using these error estimates, the method adjusts the time step: if the error is too large, the step is reduced; if the error is small, the step can be increased. This error control mechanism is vital in ensuring that the numerical solution remains within a specified tolerance while minimizing computational effort.
Accurate error estimation is central to adaptive methods. A reliable error estimate allows the algorithm to adjust the step size intelligently, ensuring that the solution is both accurate and computationally efficient. In practice, adaptive methods often require fewer function evaluations compared to fixed step-size methods in regions where the solution behaves smoothly, making them especially effective for stiff systems or problems with multiple time scales.
Implementing adaptive step-size control in Rust involves several key components: defining the system's state, calculating derivatives, applying the Dormand-Prince method to estimate both the solution and its error, and dynamically adjusting the time step based on this error estimate. Consider the following example, which uses the Dormand-Prince method to simulate a stiff system modeled by an autocatalytic chemical reaction:
// Tolerance for error control
const TOL: f64 = 1e-6;
// Maximum and minimum allowed time steps
const MAX_STEP: f64 = 0.1;
const MIN_STEP: f64 = 1e-6;
// Initial step size
const INITIAL_STEP: f64 = 0.01;
// Reaction rate constant for the autocatalytic reaction
const K1: f64 = 0.5;
/// The right-hand side (RHS) of the differential equation:
/// dy/dt = k1 * y * (1 - y)
fn rhs(y: f64) -> f64 {
K1 * y * (1.0 - y)
}
/// Implements the Dormand-Prince method for a single time step.
/// Returns a tuple containing the 5th-order solution estimate and an error estimate.
fn dormand_prince(y: f64, dt: f64) -> (f64, f64) {
// Calculate the slopes at different points within the time step.
let k1 = dt * rhs(y);
let k2 = dt * rhs(y + k1 / 5.0);
let k3 = dt * rhs(y + 3.0 / 40.0 * k1 + 9.0 / 40.0 * k2);
let k4 = dt * rhs(y + 44.0 / 45.0 * k1 - 56.0 / 15.0 * k2 + 32.0 / 9.0 * k3);
let k5 = dt * rhs(y + 19372.0 / 6561.0 * k1 - 25360.0 / 2187.0 * k2 + 64448.0 / 6561.0 * k3 - 212.0 / 729.0 * k4);
let k6 = dt * rhs(y + 9017.0 / 3168.0 * k1 - 355.0 / 33.0 * k2 + 46732.0 / 5247.0 * k3 + 49.0 / 176.0 * k4 - 5103.0 / 18656.0 * k5);
// 4th-order estimate.
let y4 = y + 35.0 / 384.0 * k1 + 500.0 / 1113.0 * k3 + 125.0 / 192.0 * k4
- 2187.0 / 6784.0 * k5 + 11.0 / 84.0 * k6;
// 5th-order estimate.
let k7 = dt * rhs(y4);
let y5 = y + 5179.0 / 57600.0 * k1 + 7571.0 / 16695.0 * k3 + 393.0 / 640.0 * k4
- 92097.0 / 339200.0 * k5 + 187.0 / 2100.0 * k6 + 1.0 / 40.0 * k7;
// Calculate the local error as the absolute difference between the 4th and 5th order estimates.
let error = (y5 - y4).abs();
(y5, error)
}
/// Attempts an adaptive step, adjusting the time step until the error is within tolerance.
/// Returns the next value of y.
fn adaptive_step(y: f64, dt: &mut f64) -> f64 {
loop {
let (y_next, error) = dormand_prince(y, *dt);
// Accept the step if the error is below the tolerance.
if error < TOL {
return y_next;
} else {
// Otherwise, reduce the step size.
*dt *= 0.5;
if *dt < MIN_STEP {
*dt = MIN_STEP;
return y_next;
}
}
}
}
fn main() {
let mut y = 0.01; // Initial concentration of the reactant.
let mut t = 0.0; // Start time.
let mut dt = INITIAL_STEP; // Start with the initial time step.
// Run the simulation until the total time reaches 10 units.
while t < 10.0 {
y = adaptive_step(y, &mut dt);
t += dt;
// If the step size is below MAX_STEP, allow increasing it gradually.
if dt < MAX_STEP {
dt = (dt * 1.2).min(MAX_STEP);
}
println!("Time: {:.4}, Concentration: {:.5}, Step size: {:.5}", t, y, dt);
}
}
In this implementation, the rhs
function defines the differential equation for an autocatalytic chemical reaction, where the rate of change of the concentration y is proportional to $y⋅(1−y)$. The dormand_prince
function implements the Dormand-Prince method, calculating intermediate slopes ($k_1$ through $k_7$) to generate two solution estimates—a fourth-order and a fifth-order approximation—and then computes the local error as the absolute difference between these estimates.
The adaptive_step
function repeatedly attempts to take a step using the current step size $Δt$ and checks if the estimated error is within the predefined tolerance TOL
. If the error exceeds the tolerance, it reduces the time step until an acceptable estimate is achieved, ensuring that the simulation maintains the desired accuracy without unnecessary computational effort.
The main
function initializes the system with an initial reactant concentration of 0.01 at time 0.0 and runs the simulation until the total time reaches 10 units. After each step, the function prints out the current time, concentration, and time step. Additionally, if the current step size is below the maximum allowed, the step size is gradually increased to improve efficiency where possible.
This adaptive step-size control mechanism optimizes the balance between accuracy and computational cost by dynamically adjusting the step size based on local error estimates. In systems with complex or stiff dynamics, such an approach ensures that the solution remains accurate without the inefficiencies associated with a uniformly small time step across the entire simulation. Rust’s strong type system, effective memory management, and low-overhead abstractions enable the implementation of such advanced numerical methods in a way that is both robust and performant.
11.7. Higher-Order Methods and Multi-Step Methods
Higher-order integration methods and multi-step methods are advanced techniques used in numerical solutions to differential equations, particularly when solving Newtonian mechanics problems. These methods extend the basic concepts of single-step methods, like the Euler or Runge-Kutta methods, by leveraging information from multiple previous steps to achieve greater accuracy and efficiency.
One prominent class of multi-step methods includes the Adams-Bashforth and Adams-Moulton methods. The Adams-Bashforth method is an explicit multi-step method, meaning it calculates the next step based purely on known information from previous steps. In contrast, the Adams-Moulton method is an implicit multi-step method, requiring the solution of an equation to predict the next step, which generally makes it more stable but also more computationally intensive.
The derivation of these methods involves using polynomial interpolation to approximate the function being integrated. In the case of the Adams-Bashforth method, the interpolation is based on the derivatives (slopes) at previous time steps, while the Adams-Moulton method uses both the current and previous slopes. These methods are particularly advantageous for solving problems with smooth dynamics, where the function and its derivatives do not change rapidly.
Higher-order accuracy refers to the ability of a numerical method to achieve a more accurate solution with fewer steps or a larger time step. However, this increased accuracy comes at the cost of higher computational complexity. For example, while a fourth-order method like Runge-Kutta requires more function evaluations per step than a first-order method like Euler's, it typically produces more accurate results for the same step size, or allows for larger step sizes without sacrificing accuracy.
In multi-step methods, the trade-offs between accuracy and computational cost are nuanced. Explicit methods like Adams-Bashforth are computationally cheaper per step since they do not require solving equations, but they can be less stable for stiff systems. Implicit methods like Adams-Moulton, on the other hand, are more stable and can handle stiff systems better, but they require solving nonlinear equations at each step, which increases computational cost.
Predictor-corrector schemes combine the advantages of explicit and implicit methods. In these schemes, an explicit method like Adams-Bashforth is used to predict the solution at the next step, and then an implicit method like Adams-Moulton corrects this prediction. This approach balances computational efficiency with stability, making it suitable for a wide range of problems, especially when dealing with smooth dynamics where stability is a concern.
Evaluating the stability regions of higher-order methods is crucial for understanding when and how to apply them effectively. Stability regions define the range of step sizes and problem parameters where the method will produce stable solutions. For example, higher-order methods can have narrower stability regions, meaning they require careful selection of step sizes to avoid numerical instability.
To implement higher-order and multi-step methods in Rust, let’s consider a practical example: simulating the propagation of a wave in a medium, governed by the one-dimensional wave equation. This equation can be discretized and solved using a combination of Adams-Bashforth and Adams-Moulton methods.
The wave equation in one dimension is given by:
$$\frac{\partial^2 u}{\partial t^2} = c^2 \frac{\partial^2 u}{\partial x^2}$$
Where: $u(x, t)$ represents the wave amplitude at position $x$ and time $t$, $c$ is the speed of the wave in the medium.
This second-order equation can be split into two first-order equations, which are more suitable for numerical integration:
$$\frac{\partial u}{\partial t} = v$$
$$\frac{\partial v}{\partial t} = c^2 \frac{\partial^2 u}{\partial x^2}$$
Here’s how you can implement a simple multi-step method in Rust, combining Adams-Bashforth and Adams-Moulton:
use std::f64::consts::PI;
const DX: f64 = 0.01; // Spatial step size
const DT: f64 = 0.001; // Time step size
const C: f64 = 1.0; // Wave speed
const L: usize = 100; // Number of spatial points
const STEPS: usize = 1000; // Number of time steps
fn main() {
// Initialize the wave amplitude (u) and wave velocity (v) at time t.
let mut u = vec![0.0; L];
let mut v = vec![0.0; L];
// Set the initial condition: A Gaussian pulse centered in the medium.
for i in 0..L {
let x = i as f64 * DX;
u[i] = (-(x - 0.5).powi(2) / 0.01).exp();
}
// Clone the initial state to store previous step values.
let mut u_prev = u.clone();
let mut v_prev = v.clone();
// Simulation loop using a predictor-corrector scheme.
for _ in 0..STEPS {
// Predictor step using the explicit Adams-Bashforth method.
let mut u_pred = vec![0.0; L];
let mut v_pred = vec![0.0; L];
for i in 1..L-1 {
// Predict the wave amplitude using the current velocity.
u_pred[i] = u[i] + DT * v[i];
// Predict the wave velocity using finite differences for the spatial second derivative.
v_pred[i] = v[i] + DT * C * C * (u[i+1] - 2.0*u[i] + u[i-1]) / DX.powi(2);
}
// Corrector step using the implicit Adams-Moulton method.
for i in 1..L-1 {
// Update the wave amplitude by averaging the current and predicted slopes.
u[i] = 0.5 * (u[i] + u_pred[i] + DT * v_pred[i]);
// Update the wave velocity by averaging the current and predicted values.
v[i] = 0.5 * (v[i] + v_pred[i] + DT * C * C * (u_pred[i+1] - 2.0*u_pred[i] + u_pred[i-1]) / DX.powi(2));
}
// Update stored previous values for the next time step.
u_prev.copy_from_slice(&u);
v_prev.copy_from_slice(&v);
}
// Output the final wave amplitude for each spatial point.
for i in 0..L {
println!("x = {:.2}, u = {:.5}", i as f64 * DX, u[i]);
}
}
In this implementation, we simulate the propagation of a wave along a one-dimensional medium using a combination of the Adams-Bashforth and Adams-Moulton methods. The wave is initialized with a Gaussian pulse centered in the medium. The wave equation is discretized in both space and time, with $u$ representing the wave amplitude and $v$ its velocity.
The Adams-Bashforth method is used as a predictor to estimate the wave amplitude and velocity at the next time step. This step is explicit, meaning it uses information from previous time steps to make the prediction. The Adams-Moulton method is then used as a corrector, adjusting the prediction by taking into account the new information from the predictor step. This implicit step enhances the stability of the simulation.
The arrays u_prev
and v_prev
store the previous values of the wave amplitude and velocity, which are necessary for the multi-step method. The predictor step calculates the new u_pred
and v_pred
values using the current values of u
and v
. The corrector step then refines these predictions to obtain the final values for u
and v
at the next time step.
This combination of Adams-Bashforth and Adams-Moulton methods provides a robust and stable solution to the wave equation, capturing the smooth dynamics of wave propagation with high accuracy. The use of a predictor-corrector scheme ensures that the method remains efficient while maintaining the desired stability, even for large-scale simulations.
When implementing higher-order and multi-step methods in Rust for large-scale simulations, several optimization techniques can be applied to manage the complexity and improve performance. One key technique is to minimize memory usage by reusing arrays or using in-place updates, which can reduce the overhead associated with managing large data sets.
Another optimization strategy involves leveraging Rust's concurrency model to parallelize the computation of the predictor and corrector steps. Since these steps can be computed independently for different spatial points, using Rust's multi-threading capabilities can significantly speed up the simulation, especially when dealing with large grids or multiple dimensions.
In addition, when dealing with more complex systems, such as fluid dynamics simulations or wave propagation in three dimensions, it is crucial to carefully select the step sizes and evaluate the stability regions of the chosen method. For instance, while higher-order methods provide better accuracy, they may also have narrower stability regions, requiring careful tuning of the step size to avoid numerical instability.
In summary, higher-order and multi-step methods offer powerful tools for solving smooth dynamic systems in computational physics. Their implementation in Rust, coupled with optimization techniques, enables efficient and accurate simulations of large-scale problems, making them invaluable for studying complex physical phenomena in fields such as fluid dynamics, wave mechanics, and beyond.
11.8. Handling Constraints and External Forces
In many physical systems, constraints and external forces play a crucial role in determining the system's behavior. Constraints can be thought of as conditions that restrict the motion of a system, such as a particle confined to move along a fixed path or a pendulum that can only swing within a certain range. External forces, such as damping or driving forces, add complexity to the system by introducing non-conservative elements that can alter energy and momentum over time.
Handling constraints in numerical simulations often requires special techniques to ensure that the system adheres to the imposed restrictions throughout the simulation. For instance, in systems with fixed or moving boundaries, like a particle trapped in a box or a bead constrained to move along a wire, constraints must be enforced explicitly. One common approach is the use of Lagrange multipliers, a mathematical technique that allows for the inclusion of constraints directly into the equations of motion.
External forces, such as damping (which opposes motion and reduces energy over time) or driving forces (which add energy to the system), also need to be carefully incorporated into numerical simulations. These forces can significantly impact the stability of the system and the conservation of energy, making it essential to choose appropriate numerical methods and step sizes to maintain accuracy.
Applications of constrained systems and external forces are abundant in physics, with examples including the simulation of pendulums (which are constrained to swing along an arc), springs with damping (where the force depends on velocity), and systems under external fields (like charged particles in an electric field).
Lagrange multipliers are a powerful technique used to enforce constraints in simulations. The basic idea is to introduce additional variables (the Lagrange multipliers) that enforce the constraints by adding terms to the equations of motion. This approach allows the system to evolve while strictly adhering to the constraints, ensuring that the simulated behavior remains physically accurate.
For example, consider a particle constrained to move on a circular path. Without constraints, the particle would move freely according to the forces acting on it. However, to keep the particle on the circle, a constraint must be imposed, which can be done using Lagrange multipliers. The resulting equations of motion will include terms that prevent the particle from deviating from the circular path.
External forces like damping and driving forces influence the system's stability and energy conservation. Damping forces, which typically oppose velocity, reduce the system's energy over time, leading to steady-state behavior in systems like damped harmonic oscillators. Driving forces, on the other hand, can inject energy into the system, leading to periodic or chaotic behavior depending on the nature of the force and the system.
The presence of constraints and external forces necessitates careful consideration of the numerical methods and step sizes used in simulations. For instance, constraints can introduce stiffness into the system, requiring smaller time steps or more advanced integration methods like implicit solvers to maintain stability. Similarly, external forces may require adaptive step-size control to accurately capture the system's behavior without sacrificing computational efficiency.
To implement constrained systems and handle external forces in Rust, we’ll consider a practical example: simulating a damped harmonic oscillator, which is a mass attached to a spring with a damping force proportional to the velocity. This example combines both constraints (the spring force that restricts the mass’s motion) and an external damping force.
The equation of motion for a damped harmonic oscillator is given by:
$$m \frac{d^2x}{dt^2} + c \frac{dx}{dt} + kx = 0$$
Where: $m$ is the mass, $c$ is the damping coefficient, $k$ is the spring constant, and $x(t)$ is the displacement from equilibrium. This second-order differential equation can be rewritten as two first-order equations:
$$\frac{dx}{dt} = v$$
$$m \frac{dv}{dt} = -cv - kx$$
Here’s how you can implement the simulation of this system in Rust:
struct DampedHarmonicOscillator {
mass: f64,
damping: f64,
spring_const: f64,
position: f64,
velocity: f64,
}
impl DampedHarmonicOscillator {
fn new(mass: f64, damping: f64, spring_const: f64, position: f64, velocity: f64) -> Self {
DampedHarmonicOscillator {
mass,
damping,
spring_const,
position,
velocity,
}
}
fn derivatives(&self) -> (f64, f64) {
let acceleration = (-self.damping * self.velocity - self.spring_const * self.position) / self.mass;
(self.velocity, acceleration)
}
fn step(&mut self, dt: f64) {
let (k1_x, k1_v) = self.derivatives();
let mid_state = DampedHarmonicOscillator {
mass: self.mass,
damping: self.damping,
spring_const: self.spring_const,
position: self.position + 0.5 * k1_x * dt,
velocity: self.velocity + 0.5 * k1_v * dt,
};
let (k2_x, k2_v) = mid_state.derivatives();
self.position += k2_x * dt;
self.velocity += k2_v * dt;
}
}
fn main() {
let mut oscillator = DampedHarmonicOscillator::new(1.0, 0.1, 10.0, 1.0, 0.0); // Initialize system
let dt = 0.01; // Time step
for step in 0..1000 {
oscillator.step(dt);
println!("Time: {:.2}, Position: {:.5}, Velocity: {:.5}",
step as f64 * dt, oscillator.position, oscillator.velocity);
}
}
In this implementation, the DampedHarmonicOscillator
struct represents the system’s state, including the mass, damping coefficient, spring constant, position, and velocity. The derivatives
method computes the rate of change of the position and velocity, which are then used to update the state in the step
method.
The step
method implements a simple second-order Runge-Kutta (midpoint) method, which is used to integrate the system’s equations of motion. This method provides a balance between accuracy and computational cost, making it suitable for simulating the damped harmonic oscillator.
In the main
function, we initialize the oscillator with a mass of 1.0 kg, a damping coefficient of 0.1 Ns/m, and a spring constant of 10 N/m. The initial position is set to 1.0 m, and the initial velocity is 0.0 m/s. The simulation then runs for 1000 time steps, with the position and velocity printed at each step.
This example demonstrates how constraints (the spring force) and external forces (the damping force) can be handled in a numerical simulation. The spring force acts as a constraint that pulls the mass back toward the equilibrium position, while the damping force opposes the motion, reducing the system’s energy over time.
When dealing with more complex systems, such as those involving multiple constraints or external forces, it’s important to optimize the code to handle the added complexity efficiently. Rust’s ownership model and memory management features can help ensure that the simulation remains performant, even when dealing with large or stiff systems.
For example, when simulating a constrained particle system, where multiple particles are subject to constraints such as fixed distances or angles between them, it’s important to structure the code to minimize memory allocations and maximize reuse of data structures. Using Rust’s Vec
collections for storing particle positions and velocities allows for dynamic memory management, but careful attention must be paid to avoid unnecessary copies or reallocations.
Additionally, parallel processing can be employed to speed up simulations that involve a large number of particles or complex interactions. Rust’s concurrency model, with its emphasis on safety and thread management, provides powerful tools for distributing the computational load across multiple CPU cores.
Another technique is to use Rust’s algebraic data types and traits to abstract over different types of forces and constraints, making the code more modular and easier to extend. For example, you could define a Force
trait that encapsulates the behavior of different forces, allowing the same integration code to handle various types of forces without modification.
In conclusion, handling constraints and external forces in numerical simulations requires a careful approach to both the conceptual design and the practical implementation. By leveraging Rust’s powerful features, it’s possible to build robust and efficient simulations that accurately capture the behavior of constrained systems under external influences. This approach is essential for solving a wide range of problems in computational physics, from simple oscillators to complex multi-particle systems.
11.9. Visualization and Analysis
Visualization is a crucial aspect of understanding and analyzing the behavior of mechanical systems in computational physics. Numerical solutions often produce large datasets that describe the evolution of a system over time or space. Visualizing these solutions helps researchers and engineers gain insights into the underlying dynamics, validate the correctness of simulations, and identify patterns or anomalies that may not be immediately apparent from raw data alone.
Effective visualization allows one to see how physical quantities like position, velocity, and energy change over time, revealing behaviors such as oscillations, steady states, or chaotic dynamics. For example, plotting the trajectory of a pendulum or the energy of a system over time can provide a clear picture of how the system evolves and whether it conserves energy, as expected from the theoretical model.
Rust, known for its performance and safety, also supports data visualization through various libraries that make it possible to generate plots and diagrams directly from simulation data. Libraries like plotters
and egui
are commonly used in Rust for creating visualizations ranging from simple line plots to complex interactive diagrams. These tools enable users to render high-quality graphics that can be used for analysis, debugging, and presentation purposes.
Visualization plays a significant role in debugging and validating numerical simulations. By graphically representing the output of a simulation, one can quickly identify discrepancies between the expected and actual behavior of the system. For example, if a simulation of a harmonic oscillator does not produce the expected sinusoidal motion in a plot, this could indicate a bug in the numerical integration code or incorrect initial conditions.
Data representation techniques such as phase space plots and energy diagrams are particularly useful in visualizing mechanical systems. A phase space plot, which shows the trajectory of a system in a space defined by its position and momentum, can reveal important properties such as stability and periodicity. Energy diagrams, which plot kinetic, potential, and total energy over time, are essential for verifying energy conservation in conservative systems.
However, visualizing high-dimensional data presents challenges. As the number of variables in a system increases, it becomes more difficult to represent all the relevant information in a single plot. Techniques like dimensionality reduction (e.g., Principal Component Analysis) or using multiple linked views (e.g., plotting different aspects of the data side by side) can help address these challenges, but they also introduce complexity in the interpretation of the results.
To implement data visualization in Rust, let’s consider an example where we simulate the motion of a simple harmonic oscillator and visualize the results using the plotters
crate. The plotters
crate is a powerful and flexible library that supports drawing various types of plots, including line plots, histograms, and scatter plots.
The harmonic oscillator can be described by the differential equation:
$$\frac{d^2x}{dt^2} = -\omega^2 x$$
Where: $x(t)$ is the position of the oscillator at time $t$ and $\omega$ is the angular frequency. This second-order equation can be broken down into two first-order equations:
$$\frac{dx}{dt} = v$$
$$\frac{dv}{dt} = -\omega^2 x$$
Here’s how you can simulate the system and visualize the results in Rust:
use plotters::prelude::*;
// Constants for the simulation
const DT: f64 = 0.01; // Time step (seconds)
const OMEGA: f64 = 1.0; // Angular frequency (rad/s)
const STEPS: usize = 1000; // Number of time steps
fn main() -> Result<(), Box<dyn std::error::Error>> {
// Initial conditions for the harmonic oscillator
let mut x = 1.0; // Initial position (meters)
let mut v = 0.0; // Initial velocity (meters/second)
// Vectors to store simulation results for plotting
let mut positions = Vec::with_capacity(STEPS);
let mut velocities = Vec::with_capacity(STEPS);
let mut energies = Vec::with_capacity(STEPS);
// Simulation loop using Euler's method for simplicity
for _ in 0..STEPS {
// Update velocity and position
v += -OMEGA.powi(2) * x * DT; // acceleration = -omega^2 * x
x += v * DT;
// Compute total energy: sum of kinetic and potential energy
let energy = 0.5 * (v.powi(2) + OMEGA.powi(2) * x.powi(2));
// Record the state at each time step
positions.push(x);
velocities.push(v);
energies.push(energy);
}
// Create a drawing area to plot the oscillator's position over time
let root_area = BitMapBackend::new("oscillator_plot.png", (800, 600)).into_drawing_area();
root_area.fill(&WHITE)?;
// Set up the chart with axis labels and title
let mut chart = ChartBuilder::on(&root_area)
.caption("Simple Harmonic Oscillator", ("sans-serif", 50))
.margin(10)
.x_label_area_size(30)
.y_label_area_size(30)
.build_cartesian_2d(0.0..(STEPS as f64) * DT, -1.5..1.5)?;
// Draw the grid and axis labels
chart.configure_mesh().draw()?;
// Plot the position x(t) as a blue line
chart
.draw_series(LineSeries::new(
positions.iter().enumerate().map(|(i, &x)| (i as f64 * DT, x)),
&BLUE,
))?
.label("x(t)")
.legend(|(x, y)| PathElement::new([(x, y), (x + 20, y)], &BLUE));
// Configure and draw the legend
chart.configure_series_labels()
.background_style(&WHITE.mix(0.8))
.border_style(&BLACK)
.draw()?;
// Save the chart to a PNG file
root_area.present()?;
println!("Plot saved to 'oscillator_plot.png'");
Ok(())
}
In this example, the system is simulated using the Euler method, which updates the position $x$ and velocity $v$ at each time step. The results are stored in vectors for positions, velocities, and energies. These vectors are then used to create a plot that visualizes the position of the oscillator over time.
The plotters
crate is used to generate the plot. A BitMapBackend
is created to draw the chart, specifying the output file (oscillator_plot.png
) and the dimensions of the plot. The ChartBuilder
is used to set up the chart, including the axes, labels, and margin. The LineSeries
is then plotted, representing the position $x(t)$ as a function of time.
The final plot is saved as a PNG file, allowing for further analysis and sharing. The use of plotters
provides a high level of customization for the chart, enabling the creation of clear and accurate visualizations that effectively convey the behavior of the simulated system.
Exporting simulation data for further analysis or publication is another critical aspect of numerical simulations. In Rust, data can be easily exported to various formats, such as CSV or JSON, using standard libraries like csv
or serde_json
. This allows the simulation results to be imported into other tools, such as Python’s Matplotlib for further visualization or R for statistical analysis.
Here’s an example of exporting the simulation data to a CSV file:
use std::fs::File;
use std::io::Write;
fn export_to_csv(positions: &[f64], velocities: &[f64], energies: &[f64], dt: f64) -> std::io::Result<()> {
// Create a new file to store the simulation data in CSV format.
let mut file = File::create("oscillator_data.csv")?;
// Write the header line.
writeln!(file, "Time,Position,Velocity,Energy")?;
// Iterate through the data vectors and write each record.
for i in 0..positions.len() {
writeln!(
file,
"{:.4},{:.5},{:.5},{:.5}",
i as f64 * dt,
positions[i],
velocities[i],
energies[i]
)?;
}
Ok(())
}
fn main() -> std::io::Result<()> {
// Run the same simulation as before.
let mut x = 1.0;
let mut v = 0.0;
let mut positions = Vec::with_capacity(STEPS);
let mut velocities = Vec::with_capacity(STEPS);
let mut energies = Vec::with_capacity(STEPS);
for _ in 0..STEPS {
v += -OMEGA.powi(2) * x * DT;
x += v * DT;
let energy = 0.5 * (v.powi(2) + OMEGA.powi(2) * x.powi(2));
positions.push(x);
velocities.push(v);
energies.push(energy);
}
// Export the simulation data to a CSV file.
export_to_csv(&positions, &velocities, &energies, DT)?;
println!("Data exported to 'oscillator_data.csv'");
Ok(())
}
This code extends the previous example by adding a function to export the simulation data to a CSV file. The export_to_csv
function writes the time, position, velocity, and energy data to a CSV file, which can then be opened in a spreadsheet program, or imported into another analysis tool.
Visualizing high-dimensional data remains a challenging task. For example, in systems with multiple degrees of freedom, representing the full state of the system in a single plot can be difficult. Techniques such as phase space plots can help visualize relationships between different variables, but they may not capture all aspects of the system’s behavior.
In such cases, it may be necessary to use multiple linked plots or interactive visualizations that allow the user to explore different dimensions of the data dynamically. Tools like egui
, which provides a graphical user interface (GUI) framework in Rust, can be used to create interactive dashboards that display multiple plots simultaneously, allowing for a more comprehensive analysis of complex systems.
In conclusion, visualization and analysis are essential components of numerical simulations in computational physics. Rust, with its robust libraries and efficient performance, provides powerful tools for creating high-quality visualizations that aid in understanding, debugging, and presenting the results of simulations. By implementing these techniques, researchers can gain deeper insights into the behavior of mechanical systems, ensuring that their numerical solutions are both accurate and meaningful.
11.10. Case Studies and Applications
Case studies play a crucial role in demonstrating the practical application of numerical methods in solving real-world problems in Newtonian mechanics. By examining specific problems, such as multi-body dynamics or collision simulations, these case studies provide concrete examples of how theoretical concepts and numerical techniques are applied to complex systems in physics and engineering. They illustrate the challenges involved in selecting appropriate methods, implementing them efficiently, and ensuring the accuracy and robustness of the solutions.
These case studies are not just academic exercises; they are directly relevant to real-world problems. For instance, simulating the motion of planets in a multi-body system can help in understanding gravitational interactions in astrophysics. Similarly, collision simulations are essential in fields ranging from materials science to automotive safety engineering, where understanding the impact dynamics can lead to better designs and safety measures.
By studying these cases, readers can gain insights into how numerical methods can be adapted to tackle a wide range of problems, extending beyond the specific examples presented. This generalizability is crucial for applying the lessons learned to other domains within computational physics, such as fluid dynamics, electromagnetism, or quantum mechanics.
The case studies discussed in this section highlight several key lessons about method selection and implementation challenges. One of the primary considerations is choosing the right numerical method for the problem at hand. For example, while the Euler method might be sufficient for simple, low-precision simulations, more complex problems like multi-body dynamics often require higher-order methods such as Runge-Kutta or symplectic integrators to maintain accuracy and stability over long periods.
Another critical aspect is the implementation challenges that arise in real-world applications. These challenges can include handling large datasets, ensuring numerical stability, optimizing performance, and dealing with edge cases that might not be covered by standard algorithms. The case studies provide examples of how these challenges can be addressed, offering practical insights that can be applied to similar problems.
Moreover, the accuracy, efficiency, and robustness of the implemented methods are key factors that determine the success of a simulation. Accuracy ensures that the results closely match the real-world behavior of the system, efficiency ensures that the simulation runs within a reasonable time frame, and robustness ensures that the method can handle a wide range of conditions without failing. Each case study reflects on these aspects, providing a critical evaluation of the methods used and their outcomes.
To illustrate these concepts, let's consider a detailed walkthrough of a case study involving a multi-body gravitational simulation, such as simulating the motion of planets in a solar system. This problem is a classic example of Newtonian mechanics, where each body in the system exerts a gravitational force on every other body, leading to complex, dynamic interactions.
The equations governing the motion of each body are derived from Newton’s law of gravitation:
$$\mathbf{F}_{ij} = G \frac{m_i m_j}{|\mathbf{r}_i - \mathbf{r}_j|^2} \hat{\mathbf{r}}_{ij}$$
Where: $\mathbf{F}_{ij}$ is the force exerted by body $j$ on body $i$, $G$ is the gravitational constant, $m_i$ and $m_j$ are the masses of the bodies, $\mathbf{r}_i$ and $\mathbf{r}_j$ are the positions of the bodies, $\hat{\mathbf{r}}_{ij}$ is the unit vector pointing from $j$ to $i$.
The acceleration of each body can be found using Newton's second law:
$$\mathbf{a}_i = \sum_{j \neq i} \frac{\mathbf{F}_{ij}}{m_i}$$
The positions and velocities are updated using a numerical integration method, such as the Velocity Verlet method, which is well-suited for this type of problem due to its stability and energy conservation properties.
Here’s how you can implement this in Rust:
use std::f64::consts::PI;
// Define a struct representing a celestial body
#[derive(Clone)]
struct Body {
mass: f64, // Mass in kilograms
position: [f64; 3], // Position in 3D space (meters)
velocity: [f64; 3], // Velocity in 3D space (meters/second)
acceleration: [f64; 3], // Acceleration in 3D space (meters/second^2)
}
impl Body {
// Constructor for creating a new Body instance.
fn new(mass: f64, position: [f64; 3], velocity: [f64; 3]) -> Self {
Body {
mass,
position,
velocity,
acceleration: [0.0; 3],
}
}
// Update the position using the Velocity Verlet algorithm.
// Positions are updated using current velocities and accelerations.
fn update_position(&mut self, dt: f64) {
for i in 0..3 {
self.position[i] += self.velocity[i] * dt + 0.5 * self.acceleration[i] * dt * dt;
}
}
// Update the velocity using the average of the old and new accelerations.
fn update_velocity(&mut self, dt: f64, new_acceleration: [f64; 3]) {
for i in 0..3 {
self.velocity[i] += 0.5 * (self.acceleration[i] + new_acceleration[i]) * dt;
}
self.acceleration = new_acceleration;
}
}
// Compute gravitational acceleration acting on body i due to all other bodies.
fn compute_gravitational_force(bodies: &[Body], i: usize) -> [f64; 3] {
const G: f64 = 6.67430e-11; // Gravitational constant (m^3 kg^-1 s^-2)
let mut force = [0.0; 3];
let body_i = &bodies[i];
for (j, body_j) in bodies.iter().enumerate() {
if i != j {
let mut r = [0.0; 3];
let mut distance_squared = 0.0;
// Compute the distance vector and its squared magnitude.
for k in 0..3 {
r[k] = body_j.position[k] - body_i.position[k];
distance_squared += r[k] * r[k];
}
let distance = distance_squared.sqrt();
// Calculate the force magnitude using Newton's law of gravitation.
let magnitude = G * body_i.mass * body_j.mass / distance_squared;
// Compute force components and accumulate.
for k in 0..3 {
force[k] += magnitude * r[k] / distance;
}
}
}
// Return acceleration by dividing force by the mass of body i.
[force[0] / body_i.mass, force[1] / body_i.mass, force[2] / body_i.mass]
}
fn main() {
// Time step: 1 hour, converted to seconds.
let dt = 60.0 * 60.0;
// Initialize a vector of bodies representing the Sun and the Earth.
let mut bodies = vec![
Body::new(1.989e30, [0.0, 0.0, 0.0], [0.0, 0.0, 0.0]), // Sun
Body::new(5.972e24, [1.496e11, 0.0, 0.0], [0.0, 29780.0, 0.0]), // Earth
];
// Run the simulation for a fixed number of steps.
for step in 0..1000 {
// First, update the positions for all bodies.
for i in 0..bodies.len() {
bodies[i].update_position(dt);
}
// Compute new accelerations for each body based on gravitational forces.
let mut new_accelerations = Vec::with_capacity(bodies.len());
for i in 0..bodies.len() {
new_accelerations.push(compute_gravitational_force(&bodies, i));
}
// Update velocities using the new accelerations.
for i in 0..bodies.len() {
bodies[i].update_velocity(dt, new_accelerations[i]);
}
// Output the Earth's position at each step for analysis.
println!(
"Step {}: Earth Position -> x: {:.3e}, y: {:.3e}, z: {:.3e}",
step, bodies[1].position[0], bodies[1].position[1], bodies[1].position[2]
);
}
}
In this implementation, the Body
struct represents each celestial body in the simulation, storing its mass, position, velocity, and acceleration. The update_position
and update_velocity
methods implement the Velocity Verlet algorithm, which updates the positions and velocities based on the computed accelerations.
The compute_gravitational_force
function calculates the gravitational force acting on each body due to all other bodies in the system, using Newton’s law of gravitation. The acceleration for each body is then updated accordingly.
The main loop iterates over a series of time steps, updating the positions and velocities of all bodies. In this example, we simulate the motion of the Earth around the Sun, printing the Earth's position at each time step.
When scaling these solutions to more complex or large-scale systems, such as simulating entire solar systems or galactic dynamics, several optimization techniques can be applied to improve performance. One approach is to use more efficient data structures, such as spatial partitioning algorithms (e.g., octrees) that reduce the number of force calculations needed by taking advantage of the spatial locality of bodies.
Parallel computing can also be employed to distribute the computation of forces across multiple processors, significantly speeding up the simulation. Rust’s concurrency model, with its emphasis on safe, efficient parallelism, is particularly well-suited for implementing such optimizations.
Troubleshooting in complex simulations often involves debugging issues related to numerical stability, conservation of energy, and performance bottlenecks. Ensuring that the chosen time step is appropriate for the scale of the simulation is crucial; too large a time step can lead to inaccurate results or instability, while too small a time step can result in unnecessarily long computation times.
Additionally, verifying the conservation of energy and momentum throughout the simulation can help identify errors in the implementation. In a well-implemented gravitational simulation, for instance, the total energy (kinetic + potential) should remain nearly constant, aside from numerical errors. Monitoring these quantities can provide valuable feedback during the development process.
The methods demonstrated in these case studies are not limited to Newtonian mechanics; they can be generalized to other domains within computational physics. For example, the techniques used to simulate gravitational interactions can be adapted to solve problems in electrostatics, where the forces between charged particles follow a similar inverse-square law.
In fluid dynamics, multi-body simulations can be used to model the interaction of fluid particles, capturing phenomena such as turbulence or wave propagation. Similarly, in electromagnetism, the methods can be adapted to simulate the motion of charged particles in electric and magnetic fields.
By understanding the principles behind these numerical methods and their implementation challenges, readers can extend the approaches presented in these case studies to a wide range of problems in computational physics, thereby broadening their applicability and impact.
In conclusion, the case studies in this section provide valuable insights into the practical application of numerical methods in Newtonian mechanics. By following the detailed walkthroughs and understanding the underlying concepts, readers can gain a deeper appreciation of how these methods are used to solve real-world problems, as well as how they can be adapted to address challenges in other areas of computational physics. The practical examples and code provided in Rust serve as a foundation for building more complex simulations, ensuring that the solutions are both efficient and accurate.
11.11. Conclusion
In conclusion, Chapter 11 provides a comprehensive guide to applying numerical methods for solving Newtonian mechanics problems using Rust. By covering fundamental concepts, numerical techniques, and advanced topics, the chapter equips readers with the tools to model and analyze physical systems accurately. The practical Rust implementations demonstrate the power and precision of computational physics, encouraging readers to apply these methods to solve complex mechanical problems.
11.11.1. Further Learning with GenAI
These prompts aim to enhance your understanding of how to model and solve physical systems computationally, focusing on practical coding techniques, theoretical insights, and performance considerations.
Explain the significance of numerical methods in solving complex Newtonian mechanics problems, focusing on their necessity when analytical solutions are either impossible or impractical. Provide examples of mechanical systems, such as chaotic systems or multi-body simulations, where numerical approaches are essential, and discuss the specific advantages and limitations of numerical methods compared to analytical approaches.
Discuss the Euler method as a basic numerical integration technique for solving Newtonian mechanics problems. Analyze its accuracy, stability, and error propagation characteristics, especially in long-term simulations, and compare it with more advanced methods like Runge-Kutta. Provide a detailed step-by-step implementation example in Rust, highlighting how the algorithm works in practice for simulating basic motion.
What are the primary limitations of the Euler method in solving Newtonian mechanics problems? Explore how issues like large step size, numerical instability, and cumulative error impact simulation accuracy, particularly in non-linear or chaotic systems. Provide examples of mechanical systems, such as pendulums or planetary orbits, where these limitations become critical.
Compare the Improved Euler method and Heun’s method in terms of their derivation, accuracy, and computational complexity. Discuss how these methods reduce local truncation errors compared to the basic Euler method. Provide insights into when each method is most effective, especially for systems with non-linear dynamics, and illustrate their performance in practical examples.
Explain the Verlet integration method and its significance in simulating systems with conservative forces, such as orbital mechanics or molecular dynamics. Analyze how Verlet preserves energy and momentum over long simulations, making it ideal for simulating long-term behavior. Provide an example implementation in Rust that demonstrates the method's advantages.
How does the leapfrog method, a variant of Verlet integration, differ in solving Newtonian mechanics problems? Analyze its approach, focusing on its stability and accuracy in simulations where long-term behavior is critical, such as planetary orbits. Compare its performance and energy conservation properties with other integration methods.
Discuss the advantages of the fourth-order Runge-Kutta (RK4) method in solving nonlinear dynamics in Newtonian mechanics. Provide a detailed comparison with lower-order methods (Euler, Verlet), focusing on accuracy, computational cost, and the method's applicability to stiff systems or chaotic dynamics. Provide practical examples and Rust code for illustration.
Explore the concept of adaptive step-size control in numerical integration methods, such as Dormand-Prince, which automatically adjusts step sizes to optimize computational efficiency while maintaining accuracy. Provide a Rust implementation and discuss how adaptive methods compare to fixed-step methods, particularly in terms of their performance in complex systems.
Analyze the role of error estimation in adaptive numerical methods. Discuss how error control influences step size choices, and examine the trade-offs between accuracy and computational cost. Provide examples of mechanical systems where adaptive step-size methods are particularly beneficial, such as chaotic systems or stiff equations.
Compare higher-order integration methods like Adams-Bashforth and Adams-Moulton multi-step techniques in the context of Newtonian mechanics. Discuss the derivation, advantages, and types of mechanical systems where these methods excel. Provide examples demonstrating how multi-step methods improve efficiency in long-term simulations.
Explain the predictor-corrector scheme used in multi-step methods for solving Newtonian mechanics problems. Analyze how this approach enhances accuracy and stability, particularly in systems with smooth dynamics. Provide examples of mechanical systems where predictor-corrector methods outperform other techniques.
Discuss methods for handling constraints in mechanical systems, such as fixed or moving boundaries, in numerical simulations. Explore techniques like Lagrange multipliers for enforcing constraints, and discuss the challenges in implementing them using Rust. Provide practical examples, such as pendulum systems with boundary constraints.
Explore the impact of external forces, such as damping or driving forces, on the stability and accuracy of numerical simulations in Newtonian mechanics. Provide examples of how to model these forces in Rust, and discuss their effects on system behavior and numerical stability, particularly in resonant or driven systems.
Analyze how different numerical methods preserve or fail to preserve energy and momentum in mechanical systems. Discuss the implications for long-term simulations, particularly in conservative systems like celestial mechanics, and provide examples of methods (e.g., Verlet or symplectic integrators) that are designed to preserve these quantities.
What are the best practices for visualizing numerical solutions to Newtonian mechanics problems? Discuss tools and libraries available in Rust for creating phase space plots, energy diagrams, and other visualizations that help understand system behavior. Provide examples of how visualization aids in the analysis of complex dynamics.
Examine the challenges involved in visualizing high-dimensional data generated from numerical simulations of Newtonian mechanics. Discuss how data representation techniques in Rust can be used to effectively interpret and analyze complex multi-dimensional simulation results, focusing on practical solutions for data reduction and clarity.
Discuss key strategies for optimizing Rust code to handle large-scale numerical simulations in Newtonian mechanics. Focus on memory management, parallel processing using Rust’s concurrency features, and performance tuning techniques, including examples of optimizing Rust code for large-scale simulations.
Provide a detailed case study on simulating multi-body dynamics using Rust. Discuss the choice of numerical methods (e.g., Runge-Kutta, Verlet), implementation challenges (e.g., collision detection), and code optimization techniques (e.g., parallelization) to ensure accurate and efficient simulation of complex mechanical systems.
Explore the application of numerical methods to collision simulations in Newtonian mechanics. Discuss how methods like Verlet integration and adaptive step-size control handle the complexities of collision events, such as elastic or inelastic collisions, and provide best practices for implementing these simulations in Rust.
Discuss the challenges and solutions for scaling numerical simulations of large systems in Newtonian mechanics. How can Rust’s features, such as concurrency, memory safety, and performance optimization, be leveraged to efficiently simulate large-scale mechanical systems? Provide examples of scalable implementations using multi-threading or distributed.
Exploring the depths of numerical solutions to Newtonian mechanics through Rust offers an exciting opportunity to merge theoretical knowledge with practical implementation. Each prompt challenges you to delve into the intricacies of numerical methods, enhance your coding skills, and gain a profound understanding of physical simulations.
11.11.2. Assignments for Practice
These exercises are designed to provide hands-on experience with the concepts and methods discussed in Chapter 11.
Exercise 11.1: Implementing and Comparing Numerical Methods
Objective: Gain practical experience in coding different numerical methods and analyzing their performance.
Instructions:
Implement Euler’s Method: Write a Rust program to implement Euler’s method for solving a simple differential equation, such as a projectile in free fall. Ensure the code includes detailed comments explaining each step.
Implement Runge-Kutta Method: Write a separate Rust program to implement the fourth-order Runge-Kutta method for the same problem.
Comparison: Run both implementations and compare their accuracy and stability. Use performance metrics and visualizations to analyze the differences. Discuss the results and improvements you would make to each method.
Exercise 11.2: Adaptive Step Size Algorithm
Objective: Develop and test an adaptive step size algorithm to improve numerical integration.
Instructions:
Implement Adaptive Step Size Method: Create a Rust program that incorporates an adaptive step size algorithm for solving a differential equation. Ensure that your implementation adjusts the step size based on the error estimate.
Test and Benchmark: Test the algorithm on various problems, including ones with rapidly changing solutions. Benchmark the performance and accuracy of the adaptive method compared to a fixed step size method.
Analysis: Analyze how well the adaptive step size improves accuracy and efficiency. Document the results and any challenges encountered during implementation.
Exercise 11.3: System of Differential Equations
Objective: Solve and analyze a system of differential equations using Rust.
Instructions:
Implement Solution: Write a Rust program to solve a system of differential equations, such as coupled oscillators. Use a suitable numerical method, like Runge-Kutta, and include proper initialization of variables and parameters.
Visualization: Implement visualization techniques to display the results, such as plotting the trajectories of the oscillators in a phase space diagram.
Interpret Results: Analyze the behavior of the system based on your visualizations. Discuss the impact of different parameters on the system’s dynamics and the accuracy of your solution.
Exercise 11.4: Advanced Numerical Methods for Constrained Systems
Objective: Implement numerical methods for constrained mechanical systems and handle constraints in simulations.
Instructions:
Implement Constrained System Solver: Write a Rust program to solve a constrained mechanical system, such as a pendulum with a fixed length. Implement numerical techniques to handle constraints effectively.
Error Analysis: Conduct an error analysis to evaluate the impact of constraints on solution accuracy. Compare results with and without considering constraints.
Documentation: Document your implementation process, including code snippets, error analysis, and any issues faced. Discuss potential improvements and how constraints affect the accuracy of simulations.
Exercise 10.5: Parallel Computing for Numerical Simulations
Objective: Explore parallel computing techniques to enhance the performance of numerical simulations.
Instructions:
Parallelize Simulations: Modify an existing Rust program for solving differential equations or systems of equations to utilize parallel computing techniques. Use Rust’s concurrency features, such as threads or the Rayon library, to speed up the simulations.
Benchmark Performance: Benchmark the performance of the parallelized program against a sequential version. Measure the speedup achieved and analyze any trade-offs in terms of accuracy or resource usage.
Optimization: Identify and implement optimizations to improve the performance further. Discuss the impact of these optimizations and document the results.
By completing them, you will gain practical skills in implementing and analyzing numerical solutions to Newtonian mechanics problems using Rust.
Comments