Chapter 8
Spectral Methods
"The greatest scientists are artists as well." — Albert Einstein
Chapter 8 of CPVR delves into spectral methods, a powerful numerical technique for solving differential equations with high accuracy. It introduces the fundamental concepts of spectral methods and Fourier transforms, highlighting their advantages over traditional numerical approaches. The chapter explores the application of these methods to partial differential equations, detailing their implementation in Rust. Key practical considerations include leveraging Rust’s advanced features for performance optimization and ensuring numerical stability. Case studies illustrate real-world applications, while advanced topics offer insights into future directions for research and development in spectral methods. The chapter emphasizes the role of Rust in enhancing computational efficiency and accuracy in spectral analysis.
8.1. Introduction to Spectral Methods
Spectral methods are a class of numerical techniques used in computational physics to solve differential equations, particularly partial differential equations (PDEs). These methods leverage the power of spectral (frequency-based) representations, such as Fourier series and transforms, to convert differential equations into algebraic equations that are easier to solve computationally. The key idea behind spectral methods is to represent the solution to a PDE as a sum of basis functions, typically sine and cosine functions in the case of Fourier methods. The coefficients of these basis functions capture the frequency components of the solution, and solving the PDE translates into finding these coefficients.
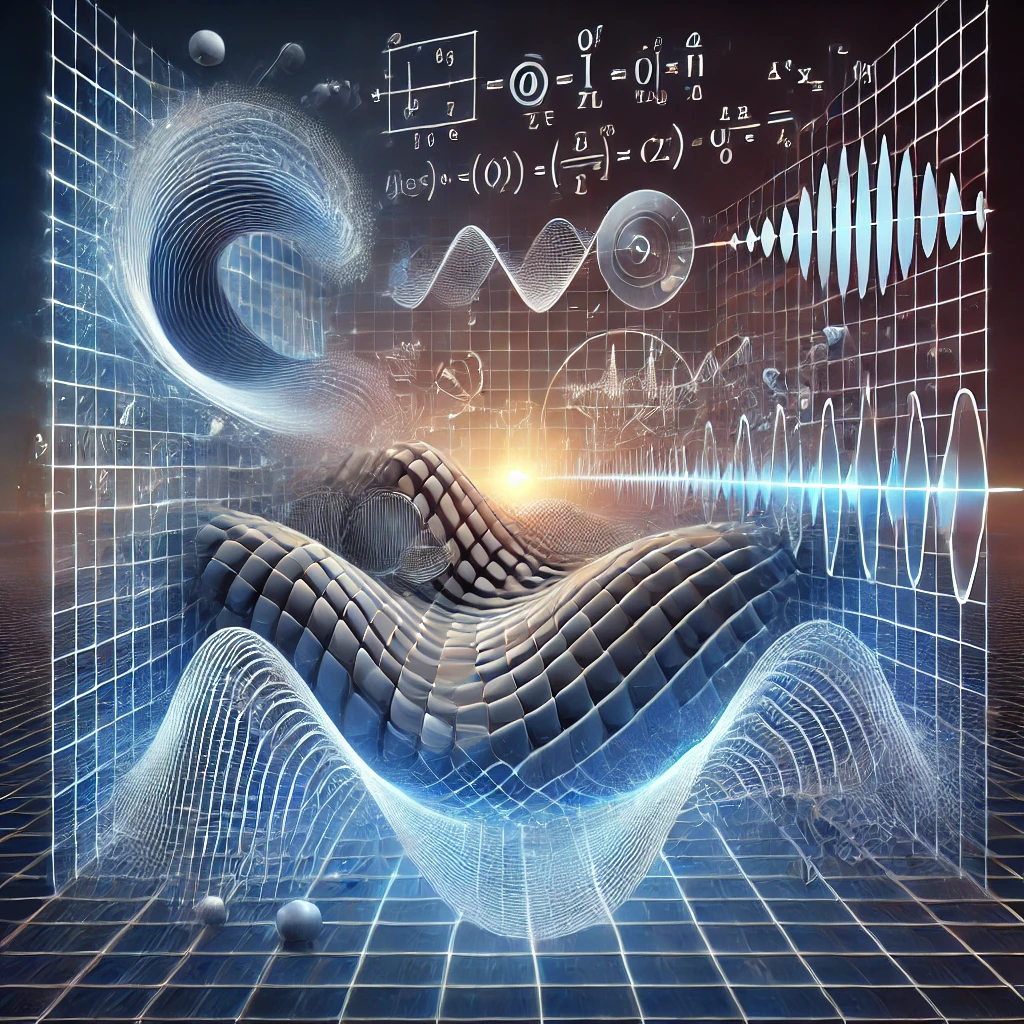
DALL-E generated illustration of spectal method.
Spectral methods are significant in computational physics due to their high accuracy and efficiency, especially for problems with smooth solutions. Unlike finite difference and finite element methods, which approximate the solution locally, spectral methods approximate the solution globally by considering the entire domain at once. This global approach often results in exponential convergence rates for smooth problems, making spectral methods particularly attractive for simulations requiring high precision.
A Fourier series is a way to represent a periodic function as a sum of sine and cosine functions, each with a specific frequency, amplitude, and phase. The Fourier transform extends this idea to non-periodic functions, transforming them from the time (or spatial) domain into the frequency domain. In the frequency domain, differential operators such as differentiation and integration become algebraic operations, which are much simpler to handle computationally. This transformation is the cornerstone of spectral methods, as it allows complex PDEs to be converted into a system of algebraic equations.
The Fourier transform of a function $f(x)$ is given by:
$$\hat{f}(k) = \int_{-\infty}^{\infty} f(x) e^{-2\pi i k x} \, dx$$
where $\hat{f}(k)$ is the Fourier transform of $f(x)$, and $k$ is the frequency variable. The inverse Fourier transform can be used to reconstruct the original function from its frequency components.
The power of spectral methods lies in their ability to transform differential equations into algebraic equations via the Fourier transform. Consider a simple example: the one-dimensional heat equation
$$\frac{\partial u}{\partial t} = \alpha \frac{\partial^2 u}{\partial x^2}$$
where $u(x,t)$ is the temperature distribution, $t$ is time, $x$ is the spatial coordinate, and $\alpha$ is the thermal diffusivity. Applying the Fourier transform to this equation with respect to the spatial variable $x$ transforms the spatial derivative into a multiplication by $k^2$, where $k$ is the wave number (frequency):
$$\frac{\partial \hat{u}}{\partial t} = -\alpha k^2 \hat{u}$$
This is now an ordinary differential equation in the frequency domain, which is significantly easier to solve.
Spectral methods differ from finite difference (FDM) and finite element methods (FEM) in several key ways. FDM approximates derivatives by using local differences between neighboring points, while FEM approximates the solution over small subdomains called elements. In contrast, spectral methods approximate the solution using global basis functions that span the entire domain. This global nature gives spectral methods their distinctive advantage in accuracy and convergence, particularly for problems with smooth solutions.
The accuracy of spectral methods can often be orders of magnitude higher than that of FDM or FEM for the same number of grid points, especially when the solution is smooth. This is because spectral methods can capture the global behavior of the solution more effectively. However, spectral methods can be less effective for problems with discontinuities or sharp gradients, where local methods like FDM and FEM may perform better.
One of the main advantages of spectral methods is their rapid convergence. For smooth problems, spectral methods exhibit exponential convergence, meaning that the error decreases exponentially as the number of basis functions (or grid points) increases. This is in stark contrast to the polynomial convergence of finite difference and finite element methods, where the error decreases as a power law with respect to the number of grid points.
The global nature of spectral methods also allows for a more accurate representation of the solution with fewer degrees of freedom, making them highly efficient for certain types of problems. This efficiency is particularly valuable in high-dimensional problems where the computational cost of traditional methods can become prohibitive.
Spectral methods are widely used in solving PDEs across various fields of physics, including fluid dynamics, quantum mechanics, and electromagnetism. These methods are particularly well-suited for problems involving wave phenomena, such as the Schrödinger equation in quantum mechanics or the Navier-Stokes equations in fluid dynamics.
Implementing spectral methods in Rust involves efficiently handling Fourier transforms and ensuring that the computation is both accurate and fast. Rust’s strong emphasis on performance and safety makes it well-suited for implementing spectral methods, particularly when dealing with large datasets or high-dimensional problems.
Below is an example of how to implement a basic spectral method using the Fast Fourier Transform (FFT) in Rust, leveraging the rustfft crate. In this example, a sine wave is transformed from the time (or spatial) domain into the frequency domain, and the magnitudes of its frequency components are printed.
use rustfft::{FftPlanner, num_complex::Complex};
use std::f64::consts::PI;
fn main() {
let n = 1024; // Number of sample points
let mut planner = FftPlanner::new();
let fft = planner.plan_fft_forward(n);
// Generate a sample function, here a sine wave, discretized over n points.
let mut input: Vec<Complex<f64>> = (0..n)
.map(|i| {
let x = 2.0 * PI * (i as f64) / (n as f64);
Complex::new(x.sin(), 0.0)
})
.collect();
// Perform the FFT in-place on the input data.
fft.process(&mut input);
// Print the magnitudes of the first 10 frequency components.
for (i, complex) in input.iter().enumerate().take(10) {
println!("Frequency {}: Magnitude = {}", i, complex.norm());
}
}
The code utilizes the FftPlanner
to create an optimized Fast Fourier Transform (FFT) plan tailored to the specific size of the input data. This plan is crucial for efficiently performing the FFT, which converts the input vector from the time (or spatial) domain into the frequency domain. The input vector in this example is initialized with a sine wave, represented as a vector of complex numbers where the real part contains the sine values and the imaginary part is zero. Once the FFT plan is established, the process
method executes the FFT in place on this input data, transforming the sine wave into its frequency components. The output of this process is a series of frequency components, where each component's magnitude indicates the strength of the corresponding frequency in the original sine wave signal. This transformation provides valuable insights into the frequency characteristics of the signal, demonstrating how the FFT can be applied to analyze and understand the frequency content of time-domain data.
This example demonstrates how to implement the Fourier transform, a fundamental operation in spectral methods, in Rust. By efficiently managing memory and leveraging Rust's performance features, the spectral method can be implemented in a way that is both fast and safe.
Spectral methods offer a powerful approach to solving differential equations in computational physics, particularly for problems with smooth solutions. By transforming PDEs into algebraic equations via Fourier transforms, spectral methods provide high accuracy and rapid convergence compared to traditional methods like finite difference and finite element methods. Rust's performance and safety features make it an excellent choice for implementing spectral methods, particularly when dealing with complex, high-dimensional problems. The example provided shows how to leverage Rust's capabilities to perform the Fast Fourier Transform, a key component of spectral methods, efficiently and effectively.
8.2. Fourier Transforms in Rust
Fourier transforms are a cornerstone of spectral methods in computational physics, providing a powerful tool for analyzing signals and solving differential equations. The core idea behind a Fourier transform is that any complex signal or function can be decomposed into a sum of simpler sinusoidal components (sine and cosine waves), each characterized by a specific frequency. This decomposition allows for the analysis of the frequency content of signals, making Fourier transforms essential in fields like signal processing, quantum mechanics, and fluid dynamics.
The Fourier transform maps a function from the time (or spatial) domain to the frequency domain, where the behavior of the system can often be more easily analyzed. For periodic signals, the Fourier series represents the signal as a sum of discrete frequency components, while the Fourier transform generalizes this idea to non-periodic signals.
In computational contexts, we commonly use the Discrete Fourier Transform (DFT) and its more efficient variant, the Fast Fourier Transform (FFT). The DFT provides a way to compute the Fourier transform of discrete data points, converting a sequence of values from the time domain into corresponding values in the frequency domain. However, the DFT is computationally expensive, with a complexity of $O(N^2)$ for $N$ data points. The FFT algorithm reduces this complexity to $O(N \log N)$, making it feasible to perform Fourier analysis on large datasets.
The Discrete Fourier Transform (DFT) is defined for a sequence of NNN complex numbers $x_0, x_1, \dots, x_{N-1}$ as:
$$X_k = \sum_{n=0}^{N-1} x_n \cdot e^{-i \cdot 2\pi \cdot k \cdot n / N}, \quad k = 0, 1, \dots, N-1$$
where $X_k$ represents the frequency component at index $k$, and $i$ is the imaginary unit. The DFT converts the input sequence from the time domain to the frequency domain, where each $X_k$ corresponds to the amplitude and phase of a particular frequency component in the original sequence.
The Fast Fourier Transform (FFT) is an algorithm that efficiently computes the DFT by exploiting symmetries in the computation. The FFT reduces the computational cost by recursively breaking down the DFT into smaller DFTs, which can be solved independently and combined to produce the final result. This reduction in computational complexity is crucial for practical applications, especially when dealing with large datasets or real-time processing.
The FFT algorithm relies on the divide-and-conquer strategy, which breaks down the DFT into smaller sub-problems that can be solved more efficiently. The most common FFT algorithm is the Cooley-Tukey algorithm, which is particularly effective when the length of the input sequence NNN is a power of two. The Cooley-Tukey algorithm works by recursively dividing the input sequence into even and odd-indexed elements, solving the DFTs of these smaller sequences, and combining the results using the "butterfly" operation, which is a key feature of the FFT.
The primary advantage of the FFT over the DFT is its computational efficiency. By reducing the complexity from $O(N^2)$ to $O(N \log N)$, the FFT makes it feasible to perform spectral analysis on large datasets and in real-time applications. This efficiency is particularly valuable in physics simulations, where large-scale data processing is often required.
Another important conceptual idea is the relationship between the time domain and the frequency domain. In the time domain, signals are represented as functions of time or space, while in the frequency domain, the same signals are represented as a sum of sinusoidal components, each with a specific frequency. The FFT allows for the transformation between these domains, enabling the analysis of how different frequency components contribute to the overall behavior of the system.
Rust provides several libraries (crates) that facilitate the implementation of FFTs. One of the most popular crates for this purpose is rustfft
, which offers a robust and efficient implementation of the FFT algorithm. The rustfft
crate is designed to handle both real and complex data, and it supports various FFT operations, including forward and inverse FFTs.
Here’s an example of how to implement and use the FFT in Rust using the rustfft
crate:
use rustfft::{FftPlanner, num_complex::Complex};
use std::f64::consts::PI;
fn main() {
let n = 1024; // Number of sample points for the transform
let mut planner = FftPlanner::new();
// Create an FFT plan for forward transformation of n points
let fft = planner.plan_fft_forward(n);
// Generate a sine wave as sample input.
// Each sample is represented as a complex number (real part contains sine values, imaginary part is 0).
let mut input: Vec<Complex<f64>> = (0..n)
.map(|i| {
let x = 2.0 * PI * (i as f64) / (n as f64);
Complex::new(x.sin(), 0.0)
})
.collect();
// Execute the FFT in place on the input vector.
fft.process(&mut input);
// Output the magnitudes of the first 10 frequency components for inspection.
for (i, complex) in input.iter().enumerate().take(10) {
println!("Frequency {}: Magnitude = {}", i, complex.norm());
}
}
The code demonstrates the use of the FftPlanner
to optimize and execute the Fast Fourier Transform (FFT) on input data. Initially, the FftPlanner
creates an FFT plan tailored to the input size nnn, allowing for efficient reuse of this plan across multiple FFT operations, thus improving overall performance. The input data is generated as a sine wave, represented as a vector of complex numbers where each element corresponds to a specific time or spatial position, with the sine function shaping the signal. The FFT is then applied to this data using the process
method, which transforms the sine wave from the time domain into the frequency domain. The output of this transformation is a set of frequency components, where each component's magnitude reflects the strength of a specific frequency present in the original sine wave. This analysis enables a deeper understanding of the signal's frequency characteristics, illustrating the FFT's capability to decompose complex time-domain signals into their constituent frequencies.
When implementing FFT in Rust, several performance optimization techniques can be employed to ensure that the computation is as efficient as possible:
Use of Efficient Libraries: Utilizing well-optimized libraries like
rustfft
ensures that the underlying FFT computation is as fast as possible. These libraries are often written in highly optimized Rust code or even interface with low-level languages like C or assembly for maximum performance.Memory Management: Rust’s ownership system ensures safe memory management, but it’s still important to minimize unnecessary memory allocations. For example, by preallocating vectors and reusing them across multiple FFT operations, you can avoid the overhead associated with repeated memory allocations and deallocations.
Parallelism: Rust’s concurrency model, supported by libraries like
rayon
, can be used to parallelize FFT operations, especially when processing multiple signals or performing multiple FFTs in sequence. This can significantly reduce computation time on multi-core processors.SIMD and Low-Level Optimizations: For even greater performance, Rust’s support for SIMD (Single Instruction, Multiple Data) instructions can be leveraged to process multiple data points simultaneously. Crates like
packed_simd
orstd::simd
can be used to implement these optimizations, though they require a deeper understanding of low-level programming.
Here’s an example of using Rust’s parallelism with rayon
to optimize multiple FFT operations:
use rustfft::{FftPlanner, num_complex::Complex};
use rayon::prelude::*;
use std::f64::consts::PI;
fn main() {
let n = 1024;
let mut planner = FftPlanner::new();
let fft = planner.plan_fft_forward(n);
// Create a vector of sine waves to process in parallel
let signals: Vec<Vec<Complex<f64>>> = (0..8)
.map(|_| {
(0..n)
.map(|i| {
let x = 2.0 * PI * (i as f64) / (n as f64);
Complex::new(x.sin(), 0.0)
})
.collect()
})
.collect();
// Perform FFT on each signal in parallel
signals
.into_par_iter()
.for_each(|mut signal| {
fft.process(&mut signal);
// Output first 10 frequencies for demonstration
for (i, complex) in signal.iter().enumerate().take(10) {
println!("Frequency {}: Magnitude = {}", i, complex.norm());
}
});
}
The code leverages the Rayon crate to achieve parallelism by processing multiple sine wave signals concurrently, effectively utilizing multi-core processors to enhance performance. Each sine wave signal undergoes transformation using the Fast Fourier Transform (FFT), and the processing of these signals is performed in parallel, allowing for simultaneous computations and reducing overall execution time. Additionally, the code is designed with memory optimization in mind, ensuring that the generation and processing of input signals are handled efficiently without unnecessary memory allocations. This careful management of memory resources minimizes overhead and contributes to improved performance, allowing for more efficient handling and analysis of large datasets or multiple signals. By combining parallel processing with optimized memory usage, the code demonstrates an effective approach to high-performance computation and data processing.
Fourier transforms, particularly the FFT, play a vital role in spectral methods and the broader field of computational physics. By transforming signals from the time domain to the frequency domain, these methods enable efficient analysis and solution of complex physical problems. Rust’s ecosystem, with crates like rustfft
and rayon
, provides robust tools for implementing these methods efficiently.
8.3. Spectral Methods for PDEs
Spectral methods are highly effective for solving partial differential equations (PDEs) such as the heat equation and wave equation. These equations are central to many areas of physics, including thermodynamics, fluid dynamics, and quantum mechanics. The heat equation, which describes the distribution of heat (or temperature) in a given region over time, is a classic example of a PDE where spectral methods excel. Similarly, the wave equation, which describes the propagation of waves (such as sound or light) through a medium, is another common application.
The key advantage of spectral methods in solving these PDEs lies in their ability to transform the original differential equations into algebraic systems that are easier to solve computationally. By expanding the solution in terms of basis functions, often sine and cosine functions in the case of Fourier spectral methods, the PDE is converted into a set of algebraic equations for the coefficients of these basis functions. Solving this system yields the solution to the PDE in the form of a series expansion.
To illustrate the transformation process, consider the one-dimensional heat equation:
$$\frac{\partial u(x,t)}{\partial t} = \alpha \frac{\partial^2 u(x,t)}{\partial x^2}$$
where $u(x,t)$ is the temperature distribution, $x$ is the spatial coordinate, ttt is time, and $\alpha$ is the thermal diffusivity. Applying a Fourier transform to the spatial variable $x$ transforms the spatial derivatives into algebraic terms. Specifically, the second derivative with respect to $x$ becomes multiplication by $-k^2$, where $k$ is the wave number.
The heat equation in the frequency domain becomes:
$$\frac{\partial \hat{u}(k,t)}{\partial t} = -\alpha k^2 \hat{u}(k,t)$$
This is now an ordinary differential equation (ODE) in time for each Fourier mode $\hat{u}(k,t)$, which can be solved using standard techniques. The solution in the frequency domain is then transformed back into the spatial domain using the inverse Fourier transform, yielding the solution to the original PDE.
Boundary conditions play a critical role in the application of spectral methods to PDEs. The choice of boundary conditions can significantly affect the accuracy and convergence of the solution. Common boundary conditions include Dirichlet boundary conditions, where the solution is fixed at the boundaries, and Neumann boundary conditions, where the derivative of the solution is specified at the boundaries.
In spectral methods, boundary conditions are often incorporated into the choice of basis functions. For example, if Dirichlet boundary conditions are imposed (e.g., $u(0,t) = u(L,t) = 0$, the sine series is a natural choice for the basis functions, as sine functions inherently satisfy these boundary conditions.
The orthogonality of basis functions is another important concept in spectral methods. Orthogonal basis functions, such as sine and cosine functions in the Fourier series, ensure that the coefficients in the series expansion are independent of one another, simplifying the solution process. Orthogonality also helps in minimizing errors and ensuring the stability of the numerical method.
Implementing spectral methods in Rust involves using Fourier transforms to solve PDEs efficiently. Rust’s performance characteristics make it well-suited for these computational tasks, and crates like rustfft
can be leveraged to handle the Fourier transforms required for spectral methods.
Below is a Rust implementation that solves the one-dimensional heat equation using spectral methods:
use rustfft::{FftPlanner, num_complex::Complex};
use std::f64::consts::PI;
fn main() {
let n = 64; // Number of grid points
let alpha = 0.01; // Thermal diffusivity
let dt = 0.01; // Time step
let t_final = 1.0; // Final time
let mut planner = FftPlanner::new();
// Prepare FFT and inverse FFT plans tailored to the number of sample points.
let fft = planner.plan_fft_forward(n);
let ifft = planner.plan_fft_inverse(n);
// Generate the initial temperature distribution as a Gaussian pulse.
let mut u: Vec<Complex<f64>> = (0..n)
.map(|i| {
let x = 2.0 * PI * (i as f64) / (n as f64);
Complex::new(f64::exp(-100.0 * (x - PI).powi(2)), 0.0)
})
.collect();
// Transform the initial condition to the frequency domain.
fft.process(&mut u);
// Precompute the factors for time-stepping: the wave numbers (frequencies) for each grid point.
let k: Vec<Complex<f64>> = (0..n)
.map(|i| {
let k_i = if i <= n / 2 {
i as f64
} else {
(i as f64) - n as f64
};
// The second derivative in the Fourier space corresponds to multiplication by -k^2.
Complex::new(-k_i.powi(2), 0.0)
})
.collect();
// Determine the number of time steps.
let steps = (t_final / dt) as usize;
// Time-stepping loop: update each Fourier mode based on the heat equation's evolution in the frequency domain.
for _ in 0..steps {
for i in 0..n {
// Multiply each Fourier mode by the exponential factor, which corresponds to advancing the solution in time.
u[i] = u[i] * Complex::new(f64::exp(k[i].re * alpha * dt), 0.0);
}
}
// Transform the solution back to the spatial domain using the inverse FFT.
ifft.process(&mut u);
// Output the final temperature distribution.
for i in 0..n {
println!("x = {}, u(x) = {}", i, u[i].re);
}
}
The code implements a numerical solution to a heat equation problem using spectral methods, specifically leveraging Fourier transforms. Initially, a grid with nnn points is set up, and the initial temperature distribution is defined as a Gaussian pulse centered at $x = \pi$. This spatial distribution is then transformed into the frequency domain using the Fast Fourier Transform (FFT), which converts the spatial representation into Fourier modes. To advance the solution in time, each Fourier mode is modified by multiplying it with an exponential factor that incorporates the thermal diffusivity and the wave number $k$, reflecting the evolution of the temperature field according to the heat equation. After applying the time-stepping updates in the frequency domain, the inverse FFT (IFFT) is used to transform the updated Fourier modes back into the spatial domain, yielding the updated temperature distribution. Finally, the code outputs the temperature distribution at each grid point, providing a snapshot of the solution after the specified time steps. This approach efficiently handles the time evolution of the temperature field by exploiting the properties of Fourier transforms to solve the heat equation in the frequency domain.
This implementation showcases how spectral methods can be applied to solve the heat equation efficiently in Rust. The FFT and IFFT are used to handle the transformation between the spatial and frequency domains, while the time-stepping loop advances the solution in time.
In practice, different PDEs and physical problems may require the handling of various boundary conditions and grid resolutions. Rust’s type system and ownership model help ensure that these considerations are managed safely and efficiently.
For example, handling Neumann boundary conditions might involve adjusting the Fourier series to include cosine functions, which naturally satisfy derivative-based boundary conditions. Similarly, adjusting grid resolutions involves modifying the number of grid points and ensuring that the FFT and IFFT operations are performed correctly for the new resolution.
Spectral methods offer a powerful approach to solving PDEs in computational physics, particularly for problems with smooth solutions and well-defined boundary conditions. By transforming PDEs into algebraic systems using Fourier transforms, these methods enable efficient and accurate solutions. Rust’s performance and safety features make it an excellent choice for implementing spectral methods, as demonstrated by the provided example of solving the heat equation. The ability to handle different boundary conditions and grid resolutions further enhances the applicability of spectral methods in various physical contexts, making them a valuable tool in the computational physicist’s toolkit.
8.4. Implementation of Spectral Methods in Rust
Spectral methods leverage the representation of a solution to a differential equation as a sum of basis functions—in many cases, sine and cosine functions for Fourier spectral methods—to transform the original differential problem into one that is algebraically tractable. Rust’s language features, including efficient array handling, powerful iterators, a strict type system, and robust concurrency support, make it an excellent platform for implementing spectral methods. In this section, we illustrate how to design data structures and implement spectral computations in Rust, focusing on Fourier transforms and their efficient execution. We will also discuss how Rust’s memory safety and parallelism features contribute to reliable and high-performance implementations.
Data Structures for Spectral Methods
A key component of spectral methods is the representation of Fourier coefficients—the amplitudes corresponding to the various frequency components of the solution. Rust’s ownership system and efficient array types allow us to define custom data structures that encapsulate these coefficients, ensuring both safe access and modification. For instance, the following example defines a FourierCoefficients
struct, which uses the Array1
type from the ndarray crate to store a vector of complex numbers. This design provides getter and setter methods, ensuring that any changes to the coefficients are controlled and type-safe.
use num_complex::Complex;
use ndarray::Array1;
struct FourierCoefficients {
data: Array1<Complex<f64>>,
}
impl FourierCoefficients {
/// Creates a new instance with a specified number of coefficients initialized to zero.
fn new(size: usize) -> Self {
FourierCoefficients {
data: Array1::zeros(size),
}
}
/// Retrieves the Fourier coefficient at the given index.
fn get(&self, index: usize) -> Complex<f64> {
self.data[index]
}
/// Sets the Fourier coefficient at the given index to the specified value.
fn set(&mut self, index: usize, value: Complex<f64>) {
self.data[index] = value;
}
}
fn main() {
let mut coeffs = FourierCoefficients::new(1024);
coeffs.set(0, Complex::new(1.0, 0.0));
println!("Coefficient at index 0: {}", coeffs.get(0));
}
In this code, the FourierCoefficients
struct centralizes the management of Fourier coefficients by encapsulating them in an Array1
. This design not only simplifies the handling of large datasets typical of spectral computations but also integrates seamlessly with Rust’s ecosystem. The use of getter and setter methods enforces data encapsulation and makes it easier to extend the functionality in the future (for instance, incorporating normalization routines or spectral filtering operations).
Parallel Spectral Computations
Rust’s concurrency model, which emphasizes memory safety through ownership and borrowing, makes it feasible to perform computationally intensive spectral methods in parallel. When multiple Fourier transforms are required—such as processing different segments of a signal or handling high-dimensional data—parallelism can dramatically reduce execution time.
For instance, if you have multiple signals to process, you can leverage the Rayon crate to distribute the FFT computations across multiple threads. The following example demonstrates how to perform parallel FFT operations on a collection of signals:
use rustfft::{FftPlanner, num_complex::Complex};
use rayon::prelude::*;
use std::f64::consts::PI;
fn parallel_fft(signals: &mut [Vec<Complex<f64>>]) {
// Create an FFT planner and plan a forward FFT for the signal length.
let mut planner = FftPlanner::new();
let fft = planner.plan_fft_forward(signals[0].len());
// Process each signal in parallel using Rayon.
signals.par_iter_mut().for_each(|signal| {
fft.process(signal);
});
}
fn main() {
let n = 1024;
// Create a vector of 8 sine wave signals.
let mut signals: Vec<Vec<Complex<f64>>> = (0..8)
.map(|_| {
(0..n)
.map(|i| {
let x = 2.0 * PI * (i as f64) / (n as f64);
Complex::new(x.sin(), 0.0)
})
.collect()
})
.collect();
// Process all signals in parallel using FFT.
parallel_fft(&mut signals);
// Print the first 10 frequency components for two sample signals.
for (i, signal) in signals.iter().enumerate().take(2) {
println!("Signal {}: First 10 frequency components:", i);
for (j, complex) in signal.iter().enumerate().take(10) {
println!(" Frequency {}: Magnitude = {}", j, complex.norm());
}
}
}
This code leverages Rayon’s par_iter_mut()
to convert a standard iterator into a parallel iterator, allowing the FFT to be computed concurrently on each signal. By doing so, the workload is distributed across multiple CPU cores, significantly speeding up the overall computation. Rust’s strict ownership and borrowing rules ensure that each thread processes its own data safely without encountering data races.
Managing Numerical Precision
When implementing spectral methods, numerical precision is vital, especially in the context of Fourier transforms, where round-off errors can impact the accuracy of the computed coefficients. Rust supports both f32
and f64
types, with f64
generally preferred for spectral methods due to its higher precision. In scenarios where even f64
is not sufficient—for example, when working with sensitive simulations or systems where small errors can lead to large differences—Rust’s ecosystem offers libraries such as rug
for arbitrary-precision arithmetic. Using rug::Float
enables computations at precision levels beyond standard floating-point types, thereby mitigating the impact of numerical errors.
use rug::Float;
fn high_precision_spectral_computation() -> Float {
// Create high-precision Floats with 100 bits of precision.
let x = Float::with_val(100, 1.0e7) + Float::with_val(100, 1.0);
let y = Float::with_val(100, 1.0e7);
let result = x - y;
result
}
fn main() {
let result = high_precision_spectral_computation();
println!("High-precision result: {}", result);
}
The above example demonstrates how to perform a simple high-precision arithmetic operation using the rug
crate. In the context of spectral methods, such high-precision calculations may be critical to ensuring that small numerical errors do not propagate and compromise the overall accuracy of the simulation.
A Comprehensive Spectral Method Example
To provide a practical perspective on how to implement spectral methods in Rust, consider the following complete example that solves the one-dimensional heat equation using spectral techniques. This approach leverages Fourier transforms to convert the heat equation into the frequency domain, applies time-stepping, and then transforms the solution back into the spatial domain.
use rustfft::{FftPlanner, num_complex::Complex};
use std::f64::consts::PI;
/// Solves the one-dimensional heat equation using spectral methods.
///
/// The heat equation is given by:
/// ∂u/∂t = α ∂²u/∂x²
///
/// # Arguments
/// * `n` - Number of grid points.
/// * `alpha` - Thermal diffusivity.
/// * `dt` - Time step.
/// * `steps` - Number of time-stepping iterations.
fn solve_heat_equation(n: usize, alpha: f64, dt: f64, steps: usize) -> Vec<Complex<f64>> {
let mut planner = FftPlanner::new();
let fft = planner.plan_fft_forward(n);
let ifft = planner.plan_fft_inverse(n);
// Define the initial condition, here a Gaussian pulse centered at x = π.
let mut u: Vec<Complex<f64>> = (0..n)
.map(|i| {
let x = 2.0 * PI * (i as f64) / (n as f64);
Complex::new(f64::exp(-100.0 * (x - PI).powi(2)), 0.0)
})
.collect();
// Transform the initial condition to the frequency domain.
fft.process(&mut u);
// Precompute Fourier space factors: compute the wave number for each component.
let k: Vec<Complex<f64>> = (0..n)
.map(|i| {
let k_i = if i <= n / 2 {
i as f64
} else {
(i as f64) - n as f64
};
// Differentiation in Fourier space is equivalent to multiplication by -k².
Complex::new(-k_i.powi(2), 0.0)
})
.collect();
// Time-stepping loop: advance the solution in the frequency domain.
for _ in 0..steps {
for i in 0..n {
// Multiply each Fourier mode by an exponential factor corresponding to the time evolution.
u[i] *= Complex::new(f64::exp(k[i].re * alpha * dt), 0.0);
}
}
// Transform the solution back to the spatial domain using the inverse FFT.
ifft.process(&mut u);
u
}
fn main() {
let n = 64;
let alpha = 0.01;
let dt = 0.01;
let steps = 1000;
let final_u = solve_heat_equation(n, alpha, dt, steps);
// Output the final temperature distribution in the spatial domain.
for i in 0..n {
println!("x = {}, u(x) = {}", i, final_u[i].re);
}
}
In this comprehensive example:
Initialization:\
A one-dimensional grid of nn points is created, and an initial condition is defined as a Gaussian pulse centered at x=πx = \\pi. Each grid point’s value is stored as a Complex
, where the imaginary parts start as zero.
Forward FFT:\
The initial temperature distribution u(x,0)u(x, 0) is transformed into the frequency domain using the FFT. This process converts the spatial differential equation into a system of ordinary differential equations (ODEs) for each Fourier mode.
Precomputation of Frequency Factors:\
The wave number kk for each grid point is computed. In Fourier space, the second spatial derivative becomes a multiplication by −k2-k^2.
Time-Stepping:\
The time evolution of the system is applied in the frequency domain by updating each Fourier mode with an exponential factor. This factor, which involves the thermal diffusivity α\\alpha and time step dtdt, represents the solution to the ODE governing each mode.
Inverse FFT:\
After advancing the solution in time, the inverse FFT transforms the updated frequency domain data back into the spatial domain, yielding the temperature distribution u(x,t)u(x,t) at the final time.
Output:\
The final solution is printed, showing the temperature at each grid point.
Optimization and Safety Considerations
Rust’s emphasis on memory safety and performance is particularly beneficial for spectral methods, which often involve intensive computations on large datasets. By using efficient data structures (like vectors of complex numbers) and leveraging libraries such as rustfft, you can achieve both high performance and robust error handling. Additionally, the inherent safety guarantees of Rust help ensure that operations such as FFTs are performed without common pitfalls such as out-of-bounds memory access or data races, even when parallelism is introduced.
For scenarios that require even more precision than provided by f64, or where numerical errors need to be mitigated, Rust’s ecosystem offers libraries like rug for arbitrary-precision arithmetic. Similarly, for problems involving high-dimensional spectral methods or requiring the application of spectral methods in parallel, Rust’s support for concurrency (using crates like rayon) can be combined with spectral methods to efficiently handle large-scale simulations.
Implementing spectral methods in Rust involves careful design of data structures to store Fourier coefficients, efficient use of libraries to perform FFTs, and mindful application of time-stepping schemes in the frequency domain. Rust’s powerful type system, ownership model, and concurrency features enable both high performance and safety when managing the large datasets typical in spectral methods. The example presented here—solving the one-dimensional heat equation—demonstrates how differential equations can be transformed into algebraic ones in the frequency domain, solved efficiently, and then transformed back into the spatial domain for analysis. This approach not only exemplifies the power of spectral methods for problems with smooth solutions but also highlights Rust’s capability to manage the computational complexity and precision required in modern scientific computing applications.
8.5. Case Studies and Applications
Spectral methods have proven to be highly effective in solving complex problems across various domains of physics, including fluid dynamics, quantum mechanics, and electromagnetic theory. Their ability to provide accurate solutions with fewer computational resources compared to traditional numerical methods like finite difference or finite element methods makes them particularly valuable in scenarios where high precision is required.
In fluid dynamics, for example, spectral methods are used to simulate turbulent flows, which are characterized by a wide range of interacting scales. These methods are also employed in quantum mechanics to solve the Schrödinger equation for systems with periodic boundary conditions, enabling the accurate computation of wavefunctions and energy levels.
The effectiveness of spectral methods in these applications stems from their ability to exploit the smoothness of the underlying physical fields. By representing these fields in terms of global basis functions, spectral methods can achieve exponential convergence, meaning that the error decreases rapidly as the number of basis functions increases. This property is particularly advantageous in simulations where capturing fine details is crucial.
Interpreting the results of spectral methods requires an understanding of the relationship between the frequency domain and the physical phenomena being modeled. For example, in fluid dynamics, the Fourier coefficients obtained from a spectral simulation can be directly related to the energy distribution among different scales of motion. High-frequency components correspond to small-scale structures, while low-frequency components represent large-scale motions.
In quantum mechanics, the spectral decomposition of the wavefunction provides insights into the contributions of different momentum states to the overall quantum state. The smoothness of the wavefunction, as captured by the spectral method, can indicate the presence of certain physical features, such as bound states or resonance structures.
Comparison with results obtained from other numerical methods, such as finite difference or finite element methods, often highlights the superior accuracy and efficiency of spectral methods, particularly for problems with smooth solutions. However, in cases where the solution exhibits discontinuities or sharp gradients, traditional methods may be more appropriate, as spectral methods can suffer from Gibbs phenomena, where oscillations occur near discontinuities.
To illustrate the practical implementation of spectral methods in Rust, consider the following case study: solving the two-dimensional incompressible Navier-Stokes equations, which describe the motion of fluid in two dimensions. The spectral method is particularly well-suited for this problem due to its ability to accurately capture the smooth variations in the velocity field.
Case Study: Solving the 2D Incompressible Navier-Stokes Equations
The 2D incompressible Navier-Stokes equations can be written as:
$$\frac{\partial \mathbf{u}}{\partial t} + \mathbf{u} \cdot \nabla \mathbf{u} = -\nabla p + \nu \nabla^2 \mathbf{u}$$
where $\mathbf{u} = (u, v)$ is the velocity field, $p$ is the pressure, and ν\\nuν is the kinematic viscosity. The spectral method solves these equations by transforming them into the Fourier domain, where the differential operators become algebraic.
Here is an example of implementing this in Rust:
use rustfft::{FftPlanner, num_complex::Complex};
use ndarray::{Array2, Zip};
use std::f64::consts::PI;
/// Solves the 2D incompressible Navier-Stokes equations using spectral methods.
/// The function transforms the velocity field into the Fourier domain, applies time-stepping,
/// and then converts the result back into the spatial domain.
///
/// # Arguments
/// * `n` - Number of grid points in the x-direction.
/// * `m` - Number of grid points in the y-direction.
/// * `dt` - Time step for the simulation.
/// * `steps` - Number of time-stepping iterations.
/// * `nu` - Kinematic viscosity.
fn solve_navier_stokes_2d(
n: usize,
m: usize,
dt: f64,
steps: usize,
nu: f64,
) -> Array2<Complex<f64>> {
let mut planner: FftPlanner<f64> = FftPlanner::new();
let fft = planner.plan_fft_forward(n);
let _ifft = planner.plan_fft_inverse(n); // Placeholder for inverse FFT if needed.
// Initialize the velocity field in Fourier space.
let mut u_hat = Array2::zeros((n, m));
for _ in 0..steps {
// Compute the nonlinear term in Fourier space.
let non_linear_term = compute_nonlinear_term(&u_hat, n, m);
// Update the Fourier coefficients using an explicit Euler scheme.
Zip::from(&mut u_hat).and(&non_linear_term).for_each(|u, &nl| {
let k_x = 2.0 * PI / (n as f64);
let k_y = 2.0 * PI / (m as f64);
*u += Complex::new(-dt * (k_x.powi(2) + k_y.powi(2)) * nu, 0.0) * nl;
});
// Optionally, you may transform back to physical space here to monitor the solution.
// For brevity, we leave it in Fourier space.
}
u_hat
}
/// Placeholder function to compute the nonlinear term in Fourier space.
/// In a complete implementation, this function would compute the convolution
/// corresponding to the nonlinear term \(\mathbf{u} \cdot \nabla \mathbf{u}\)
/// in the Fourier domain.
fn compute_nonlinear_term(
_u_hat: &Array2<Complex<f64>>,
n: usize,
m: usize,
) -> Array2<Complex<f64>> {
Array2::zeros((n, m))
}
fn main() {
let n = 64;
let m = 64;
let dt = 0.01;
let steps = 1000;
let nu = 0.01;
let final_u_hat = solve_navier_stokes_2d(n, m, dt, steps, nu);
println!("Final Fourier coefficients: {:?}", final_u_hat);
}
The code snippet initializes the velocity field for solving the Navier-Stokes equations, starting with zero velocity in the Fourier domain. This approach simplifies the initial condition setup, although more complex velocity distributions can be used in practical applications. The nonlinear term, $\mathbf{u} \cdot \nabla \mathbf{u}$, is computed in the Fourier domain to leverage the efficiency of Fourier space calculations. This typically involves either directly performing convolution in Fourier space or using a pseudospectral method where the convolution is first computed in physical space before transforming back to Fourier space. For time-stepping, the code uses an explicit Euler scheme to update the velocity field, which, while straightforward, may be replaced with more sophisticated methods to enhance stability and accuracy. Finally, the inverse FFT is applied to convert the updated velocity field from Fourier space back to physical space, making it possible to analyze or visualize the results in a more interpretable format.
Once the spectral method has been applied and the solution has been computed, the results must be analyzed and visualized to gain insights into the physical phenomena being modeled. In Rust, libraries such as ndarray
for data manipulation and plotters
or plotlib
for visualization can be used to handle these tasks.
Here’s how you might visualize the final velocity field obtained from the Navier-Stokes simulation:
use rustfft::{FftPlanner, num_complex::Complex};
use ndarray::{Array2, Zip};
use std::f64::consts::PI;
/// Solves the 2D incompressible Navier-Stokes equations using spectral methods.
/// The function transforms the velocity field into the Fourier domain, applies time-stepping,
/// and then converts the result back into the spatial domain.
///
/// # Arguments
/// * `n` - Number of grid points in the x-direction.
/// * `m` - Number of grid points in the y-direction.
/// * `dt` - Time step for the simulation.
/// * `steps` - Number of time-stepping iterations.
/// * `nu` - Kinematic viscosity.
fn solve_navier_stokes_2d(
n: usize,
m: usize,
dt: f64,
steps: usize,
nu: f64,
) -> Array2<Complex<f64>> {
let mut planner: FftPlanner<f64> = FftPlanner::new();
let fft = planner.plan_fft_forward(n);
let _ifft = planner.plan_fft_inverse(n); // Placeholder for inverse FFT if needed.
// Initialize the velocity field in Fourier space.
let mut u_hat = Array2::zeros((n, m));
for _ in 0..steps {
// Compute the nonlinear term in Fourier space.
let non_linear_term = compute_nonlinear_term(&u_hat, n, m);
// Update the Fourier coefficients using an explicit Euler scheme.
Zip::from(&mut u_hat).and(&non_linear_term).for_each(|u, &nl| {
let k_x = 2.0 * PI / (n as f64);
let k_y = 2.0 * PI / (m as f64);
*u += Complex::new(-dt * (k_x.powi(2) + k_y.powi(2)) * nu, 0.0) * nl;
});
// Optionally, you may transform back to physical space here to monitor the solution.
// For brevity, we leave it in Fourier space.
}
u_hat
}
/// Placeholder function to compute the nonlinear term in Fourier space.
/// In a complete implementation, this function would compute the convolution
/// corresponding to the nonlinear term \(\mathbf{u} \cdot \nabla \mathbf{u}\)
/// in the Fourier domain.
fn compute_nonlinear_term(
_u_hat: &Array2<Complex<f64>>,
n: usize,
m: usize,
) -> Array2<Complex<f64>> {
Array2::zeros((n, m))
}
fn main() {
let n = 64;
let m = 64;
let dt = 0.01;
let steps = 1000;
let nu = 0.01;
let final_u_hat = solve_navier_stokes_2d(n, m, dt, steps, nu);
println!("Final Fourier coefficients: {:?}", final_u_hat);
}
The visualize_velocity_field
function utilizes the plotters
crate to create a 2D visualization of the velocity field derived from the Fourier coefficients. Initially, the Fourier coefficients are transformed back to the physical domain using an inverse Fourier transform, which reconstructs the spatial representation of the velocity field. This spatial data is then plotted on a Cartesian plane, where each grid point is represented as a red circle. This graphical representation provides a clear and intuitive view of the fluid's flow patterns and structures, making it easier to analyze and interpret the behavior of the velocity field within the simulated fluid dynamics.
The case studies presented in this section demonstrate the practical application of spectral methods to real-world problems in computational physics. By leveraging Rust’s performance and safety features, these methods can be implemented efficiently, providing accurate solutions to complex PDEs such as the Navier-Stokes equations. The provided Rust code examples illustrate the core concepts of spectral methods, from transforming PDEs into the Fourier domain to visualizing the results. The combination of spectral methods with Rust’s powerful ecosystem makes this approach highly effective for a wide range of applications in computational physics.
8.6. Advanced Topics and Future Directions
Advanced spectral methods have evolved significantly over the years, and contemporary techniques such as spectral element methods (SEMs) and high-order spectral methods are now at the forefront of solving complex physical problems. SEMs seamlessly merge the geometric flexibility of finite element methods with the rapid convergence and high accuracy of spectral methods. In these methods, the computational domain is partitioned into discrete elements, and within each element, a spectral method is applied to approximate the solution. This hybrid approach allows for exceptional accuracy in resolving complex geometries and irregular boundaries while still benefiting from the global convergence properties of spectral methods.
High-order spectral methods further enhance solution accuracy by employing basis functions of higher degree, typically involving high-order polynomials. These methods can achieve exponential convergence for smooth problems and are particularly advantageous in fields such as fluid dynamics, where capturing fine details and subtle phenomena is critical. The implementation of high-order methods often results in significant improvements in accuracy without a proportional increase in computational cost, especially when the underlying physical solution is smooth.
The continued advancement and integration of these techniques are expanding the application of spectral methods into more challenging and diverse physical systems, such as weather prediction, climate modeling, and astrophysical simulations. One emerging trend is the integration of spectral methods with machine learning (ML). Machine learning algorithms can be employed to accelerate spectral computations by learning from previous simulation data, predicting the evolution of spectral coefficients, or optimizing the selection of basis functions. This hybrid approach holds the promise of dramatically reducing computational cost while preserving or even enhancing accuracy, particularly in scenarios where conventional spectral methods are computationally expensive.
Another promising direction is the increased utilization of modern hardware architectures, including GPUs and other specialized processors. As these hardware technologies advance, spectral methods can leverage their parallel computing capabilities to execute large-scale simulations more efficiently. For instance, by offloading intensive Fourier transform calculations to a GPU, one can significantly reduce runtime, making real-time simulations and large-scale multi-dimensional problems more tractable.
Rust is well-positioned to support these advancements due to its focus on performance, memory safety, and concurrency. The language's powerful concurrency model, built on the principles of ownership and borrowing, helps prevent common pitfalls such as data races during parallel execution. This, combined with Rust’s zero-cost abstractions and the ability to interface with low-level hardware, ensures that Rust-based implementations of spectral methods are both robust and efficient. The development of dedicated Rust crates and libraries tailored to advanced spectral computations will further drive progress in this area.
In addition to hardware acceleration, the refinement of existing libraries—such as rustfft for fast Fourier transforms and ndarray for multi-dimensional array operations—will improve the support for spectral methods in Rust. Enhancements such as more efficient handling of complex data types, support for non-uniform grids, and integration with GPU acceleration libraries like wgpu or CUDA will expand the practical applications of spectral methods.
The future also holds exciting possibilities for merging spectral methods with data-driven approaches. For example, employing machine learning to predict spectral coefficients or to dynamically adjust the basis functions in response to localized features in the simulation could lead to adaptive spectral methods that further optimize computational effort. Such methods would allow the simulation grid to be refined selectively in areas of sharp gradients or high complexity while maintaining coarser resolution in smoother regions, thereby balancing computational cost with accuracy.
Another research frontier involves exploring new basis functions—such as wavelets or other localized functions—that might better capture localized phenomena while preserving the exponential convergence characteristics of spectral methods. These developments could broaden the scope of spectral methods, making them applicable to a wider range of problems that include both smooth and rapidly varying solutions.
To illustrate one potential future implementation, consider an adaptive spectral method in Rust. The following conceptual code snippet outlines a basic approach where the grid resolution is adjusted dynamically based on a refinement criterion:
use rustfft::{FftPlanner, num_complex::Complex};
use ndarray::{Array2, Zip};
use std::f64::consts::PI;
fn adaptive_spectral_method(
n: usize,
m: usize,
dt: f64,
steps: usize,
nu: f64,
) -> Array2<Complex<f64>> {
let mut planner = FftPlanner::new();
let fft = planner.plan_fft_forward(n);
let ifft = planner.plan_fft_inverse(n);
// Initialize the velocity field in Fourier space.
let mut u_hat = Array2::zeros((n, m));
for step in 0..steps {
// Compute the nonlinear term in Fourier space.
let non_linear_term = compute_nonlinear_term(&u_hat, n, m);
// Update the Fourier coefficients using an explicit Euler scheme.
Zip::from(&mut u_hat).and(&non_linear_term).for_each(|u, &nl| {
let k_x = 2.0 * PI / (n as f64);
let k_y = 2.0 * PI / (m as f64);
*u += Complex::new(-dt * (k_x.powi(2) + k_y.powi(2)) * nu, 0.0) * nl;
});
// Periodically adapt the grid resolution based on the estimated error or complexity.
if step % 100 == 0 {
adapt_grid_resolution(&mut u_hat);
}
// Optionally, transform back to physical space to monitor the solution.
let mut u_hat_flat: Vec<_> = u_hat.iter().cloned().collect();
ifft.process(&mut u_hat_flat);
// Transform `u_hat_flat` back into a 2D array if needed for further processing.
// (This step is skipped here for simplicity.)
}
u_hat
}
fn adapt_grid_resolution(_u_hat: &mut Array2<Complex<f64>>) {
// Placeholder function for grid adaptation.
// Analyze the current solution and adjust the number of grid points to refine or coarsen the mesh.
}
fn compute_nonlinear_term(
_u_hat: &Array2<Complex<f64>>,
_n: usize,
_m: usize,
) -> Array2<Complex<f64>> {
// Placeholder for the nonlinear term calculation.
Array2::zeros((_n, _m))
}
fn main() {
let n = 64;
let m = 64;
let dt = 0.01;
let steps = 1000;
let nu = 0.01;
let final_u_hat = adaptive_spectral_method(n, m, dt, steps, nu);
println!("Final adaptive Fourier coefficients: {:?}", final_u_hat);
}
This conceptual implementation outlines an adaptive spectral method in which the grid resolution is periodically adjusted based on a refinement criterion. Although the functions compute_nonlinear_term
and adapt_grid_resolution
are placeholders, the structure illustrates how one might integrate adaptive techniques with spectral methods to improve efficiency and accuracy.
As computational needs and hardware capabilities continue to evolve, the future of spectral methods will likely involve deeper integration with machine learning, parallel processing, and adaptive techniques. By combining spectral methods with data-driven models, researchers could predict spectral coefficients more efficiently or optimize basis functions to best capture the nuances of complex phenomena. Furthermore, as GPUs and other specialized processors become more prevalent, harnessing their power through safe, concurrent programming in Rust will open new frontiers in real-time and large-scale simulations.
In conclusion, advanced spectral methods—encompassing spectral elements, high-order techniques, and adaptive strategies—offer a promising path forward for solving ever-more complex PDEs in computational physics. The integration of these methods with machine learning and the acceleration capabilities of modern hardware represents a dynamic and exciting area of research. Rust’s emphasis on safety, performance, and concurrency, together with its growing ecosystem of specialized libraries, positions it as an ideal platform for exploring and advancing these cutting-edge computational techniques. The ideas and code examples presented here provide a glimpse into the future directions of spectral methods and underscore the potential for further innovation in both algorithm development and practical applications.
8.7. Conclusion
In conclusion, Chapter 8 underscores the importance of spectral methods in computational physics and the power of Rust in implementing these techniques. By combining theoretical insights with practical Rust implementations, the chapter equips readers with the tools to solve complex differential equations efficiently and accurately, paving the way for future advancements in computational science.
8.7.1. Further Learning with GenAI
These prompts will help delve into the nuances of spectral methods, the intricacies of Fourier transforms, and the specifics of implementing these techniques efficiently in Rust. Each prompt aims to provide a robust and detailed answer, covering fundamental principles, advanced topics, and real-world applications.
What are spectral methods, and how do they fundamentally differ from finite difference and finite element methods in terms of mathematical formulation and computational efficiency? Explore the underlying mathematical concepts behind spectral methods, focusing on global approximations using basis functions versus the local approximations in finite difference and finite element methods. Discuss how these methods differ in terms of accuracy, convergence rates, and computational complexity, and explain the practical implications of these differences when applied to solving partial differential equations (PDEs) in computational physics.
Can you provide a detailed explanation of the mathematical principles behind spectral methods, including the role of orthogonal functions and basis expansions? Delve into the importance of orthogonality and the choice of basis functions, such as sine, cosine, or Chebyshev polynomials, in spectral methods. Explain how basis expansions allow for global approximations of solutions to PDEs, and analyze how these principles lead to the high accuracy and exponential convergence properties of spectral methods for problems with smooth solutions.
How do Fourier transforms work at a mathematical level, including the derivation and interpretation of the Discrete Fourier Transform (DFT) and Fast Fourier Transform (FFT)? Provide a step-by-step derivation of the DFT and explain how it transforms time-domain data into the frequency domain. Discuss the mathematical significance of Fourier coefficients and frequency components in the DFT. Analyze how the FFT algorithm optimizes the DFT, reducing computational complexity from O(n^2) to O(n log n), and explain the practical benefits of this efficiency in large-scale numerical simulations.
Discuss the computational complexity of FFT algorithms in detail, including time complexity and space complexity. Provide a thorough analysis of the time complexity (O(n log n)) and space complexity considerations of FFT. Discuss how this computational efficiency enables the scaling of FFT-based simulations to large problem sizes. Include specific examples of performance impacts in applications such as signal processing, image analysis, and solving PDEs in computational physics, emphasizing the trade-offs between performance, memory use, and precision.
How can Fourier transforms be applied to analyze and solve partial differential equations (PDEs) in computational physics? Provide a comprehensive example of using Fourier transforms to solve a PDE, such as the heat equation or Poisson's equation. Detail the steps involved, including transforming the PDE into the frequency domain, solving the algebraic equations, and transforming the solution back into the time or spatial domain. Discuss the advantages of this approach in terms of simplifying the problem and improving computational efficiency compared to traditional time-stepping methods.
What are some common pitfalls and challenges associated with implementing FFT in numerical simulations? Identify issues such as numerical instability, aliasing, and boundary condition mismatches. Discuss how improper grid resolution or handling of periodicity can lead to inaccurate results. Provide strategies for mitigating these challenges, such as padding the data to prevent aliasing, using windowing functions, or applying appropriate boundary treatments.
How are spectral methods specifically applied to solve the heat equation and the wave equation? Provide a detailed, step-by-step explanation of how spectral methods transform these PDEs into algebraic systems. Discuss the process of selecting appropriate basis functions (e.g., sine and cosine for periodic boundary conditions), computing the spectral coefficients, and using these coefficients to approximate the solution. Highlight the advantages of spectral methods, such as higher accuracy and faster convergence compared to finite difference approaches.
What is the significance of basis functions and orthogonality in spectral methods? Explain how basis functions are used to represent the solution of a PDE, and why orthogonal basis functions, such as trigonometric or Chebyshev polynomials, are essential for simplifying the computation of spectral coefficients. Discuss how different choices of basis functions affect the accuracy, convergence rate, and numerical stability of spectral methods, and provide examples of scenarios where specific basis functions are advantageous.
How can Rust’s core data structures and features (e.g., arrays, slices, iterators) be effectively utilized to implement spectral methods? Provide practical examples of how to leverage Rust’s core language features, such as arrays and iterators, for efficient computation of spectral coefficients, matrix operations, and transformations in spectral methods. Discuss the advantages of Rust’s type system, ownership model, and memory safety in ensuring performance and correctness in numerical simulations.
What are the best practices for ensuring numerical stability and accuracy when implementing spectral methods in Rust? Discuss techniques for managing floating-point precision, error propagation, and round-off errors when performing spectral computations. Provide best practices for validating the correctness of numerical results, such as comparing against analytical solutions or using higher-order precision types. Explain how Rust’s strict compile-time checks and functional programming paradigms help minimize common numerical errors.
How can Rust’s concurrency model and parallelism features be leveraged to optimize the performance of spectral methods computations? Explore how Rust’s multithreading capabilities, async programming, and parallelism libraries (e.g., Rayon) can be applied to distribute computational loads across multiple cores. Provide practical examples of parallelizing key operations in spectral methods, such as FFT computations or matrix factorizations, and demonstrate the performance gains achieved through concurrency.
What methods can be used to benchmark and profile Rust implementations of spectral methods? Discuss tools such as Criterion for benchmarking and
cargo-profiler
orperf
for profiling Rust code. Provide detailed strategies for identifying performance bottlenecks in Rust implementations of spectral methods, such as inefficient memory access patterns or computational hotspots, and outline steps for optimizing these areas.Provide detailed case studies of real-world problems where spectral methods have been successfully applied. Explore specific applications in fluid dynamics (e.g., Navier-Stokes equations), quantum mechanics (e.g., solving the Schrödinger equation), or meteorology (e.g., climate modeling). Discuss how spectral methods were used to model these systems, the challenges encountered, and the results obtained. Highlight the computational techniques and tools (e.g., FFT, basis expansions) used to achieve high accuracy and efficiency.
How do the results from spectral methods compare with those obtained using other numerical methods (e.g., finite difference, finite element) in various applications? Provide a comparative analysis of the strengths and weaknesses of spectral methods relative to finite difference and finite element methods. Discuss in which scenarios spectral methods offer superior accuracy or computational efficiency, and when other methods may be more appropriate due to geometric complexity or boundary conditions. Include real-world examples to illustrate the trade-offs.
What are spectral elements, and how do they enhance traditional spectral methods to handle more complex geometries and boundary conditions? Explain the concept of spectral elements, which combine the high accuracy of spectral methods with the geometric flexibility of finite element methods. Discuss the mathematical formulation of spectral elements, how they enable efficient handling of irregular domains, and provide examples of their application to problems with complex boundaries.
How do high-order spectral methods improve the accuracy and convergence of numerical solutions for differential equations? Discuss the advantages of using high-order basis functions in spectral methods, including exponential convergence for smooth problems. Analyze the trade-offs involved in using high-order methods, such as increased computational cost and potential numerical instability, and provide examples of problems where high-order spectral methods significantly outperform lower-order alternatives.
What emerging trends in computational physics are likely to influence the development and application of spectral methods in the coming years? Explore advancements in algorithms, such as adaptive spectral methods, hybrid methods combining machine learning with spectral techniques, or new hardware architectures like quantum computing. Discuss how these developments could enhance the capabilities and applications of spectral methods in solving complex physical problems.
How can machine learning techniques be integrated with spectral methods to enhance their capabilities and applications? Discuss how neural networks or deep learning techniques can be combined with spectral methods to improve the accuracy, efficiency, or scalability of simulations. Provide examples of research where machine learning is used to approximate spectral coefficients or accelerate convergence in high-dimensional problems.
What are some prominent Rust libraries and crates that facilitate the implementation of spectral methods, and how do they compare in terms of functionality, performance, and ease of use? Provide a detailed review of Rust libraries such as
ndarray
,nalgebra
, orfftw
for numerical computations, matrix operations, and FFTs. Compare these crates with similar tools in other languages (e.g., Python’s NumPy) and discuss their strengths and limitations for implementing spectral methods.How can Rust’s ecosystem be expanded to better support advanced spectral methods and address existing limitations? Identify areas where the Rust ecosystem could benefit from improved support for spectral methods, such as more comprehensive numerical libraries, better visualization tools, or integration with GPU computing. Propose strategies for growing community involvement, contributing to existing crates, and building new tools.
Embrace the learning process with curiosity and determination, knowing that each step you take enhances your skills and contributes to the broader scientific community. Your dedication to understanding these sophisticated techniques will not only advance your knowledge but also pave the way for innovations that could shape the future of computational science. Keep pushing the boundaries of your understanding, and let your passion for problem-solving drive you towards excellence.
8.7.2. Assignments for Practice
These tasks will help you build a solid foundation in computational physics using Rust, enhance your problem-solving skills, and prepare you for tackling complex numerical challenges.
Exercise 8.1: Fourier Transform Implementation in Rust
Develop a Rust program that implements the Fast Fourier Transform (FFT) algorithm. Use a Rust library or crate of your choice, such as rustfft
or ndarray
, to perform the FFT on a set of sample data. Implement the following:
Data Preparation: Generate a signal with a known frequency composition (e.g., a sum of sine waves).
FFT Implementation: Apply the FFT to the signal and obtain the frequency-domain representation.
Validation: Compare the results with the expected frequency components and visualize both the time-domain and frequency-domain data using a plotting library.
Ask GenAI to help you debug issues encountered during the implementation, such as errors in FFT computation or performance bottlenecks. You can also request explanations of specific Rust code snippets used in your implementation.
Exercise 8.2: Spectral Method for Heat Equation
Implement a spectral method to solve the 1D heat equation using Rust. Your implementation should:
Discretization: Transform the continuous heat equation into a discrete system using Fourier series.
Solution: Compute the solution over time for given initial conditions and boundary conditions.
Visualization: Plot the temperature distribution over time to visualize the heat diffusion process.
Request GenAI’s guidance on translating the mathematical formulation of the heat equation into code. Seek help with Rust-specific implementations, such as handling arrays and slices for numerical computations, and ask for advice on visualizing the results effectively.
Exercise 8.3: Benchmarking and Profiling
Create a Rust application that benchmarks and profiles the performance of your spectral methods implementation. Include the following:
Benchmarking: Measure the execution time of key operations, such as FFT and PDE solvers.
Profiling: Use profiling tools (e.g.,
perf
,flamegraph
) to identify performance bottlenecks.Optimization: Implement optimizations based on profiling results and compare performance before and after improvements.
Seek help on setting up benchmarking and profiling tools in Rust. Ask for advice on interpreting profiling data and implementing performance optimizations based on the findings.
Exercise 8.4: Case Study Analysis
Select a real-world case study where spectral methods have been applied (e.g., fluid dynamics simulation). Analyze the following aspects:
Problem Description: Summarize the physical problem being solved and why spectral methods were chosen.
Implementation Details: Describe the implementation approach used, including discretization, boundary conditions, and computational strategies.
Results and Comparisons: Compare the results obtained using spectral methods with those from other numerical methods, and discuss the advantages and limitations.
Ask GenAI for guidance on structuring your case study analysis and request detailed explanations of implementation strategies and comparisons with other methods. Use GenAI to clarify complex concepts and provide insights into the strengths and weaknesses of different approaches.
Exercise 8.5: Advanced Spectral Methods Exploration
Explore advanced spectral methods, such as spectral elements or high-order spectral methods, and implement one of these techniques in Rust. Your task should include:
Mathematical Formulation: Understand and describe the mathematical principles behind the chosen advanced spectral method.
Implementation: Write Rust code to implement the method, including handling complex geometries or high-order approximations.
Comparison: Evaluate the performance and accuracy of the advanced method compared to traditional spectral methods.
Request detailed explanations of the mathematical formulation and implementation steps for advanced spectral methods. Seek advice on handling specific challenges associated with high-order approximations or complex geometries and ask for help in comparing the new method with traditional approaches.
Completing these exercises will not only solidify your grasp of spectral methods but also demonstrate the power and versatility of Rust in computational physics. As you navigate through these tasks, remember that each step brings you closer to mastering the art of numerical analysis and programming excellence. Stay curious, be persistent, and let your achievements inspire further exploration and growth in the exciting field of computational physics.
Comments