Chapter 7
Monte Carlo Simulations
"As far as I am concerned, the most fundamental aspect of science is the drive to understand nature, and this drive is often stimulated by the quest for better methods and tools." — Roger Penrose
Chapter 7 of CPVR delves into the application of Monte Carlo simulations within the realm of computational physics. It begins with a foundational overview of Monte Carlo methods, highlighting their stochastic nature and significance in solving complex physical systems. The chapter progresses by setting up the Rust environment tailored for such simulations, emphasizing Rust's performance and safety benefits. It explores the implementation of random number generators and Monte Carlo algorithms, including MCMC and importance sampling, and focuses on optimizing computational efficiency for handling large datasets. The chapter also covers techniques for visualizing and analyzing simulation results and provides case studies to illustrate practical applications in physics. Rust’s robust features and libraries, combined with Monte Carlo methodologies, offer a powerful toolkit for advancing computational physics research.
7.1. Introduction to Monte Carlo Simulations
Monte Carlo simulations are a class of computational algorithms that rely on repeated random sampling to obtain numerical results. The name "Monte Carlo" is derived from the Monte Carlo Casino in Monaco, reflecting the stochastic, or random, nature of these methods. Monte Carlo methods are particularly useful in scenarios where deterministic algorithms are infeasible due to the complexity of the system or the high dimensionality of the problem space. In computational physics, Monte Carlo simulations are employed to model systems with many coupled degrees of freedom, such as fluids, disordered materials, and complex interacting particles.
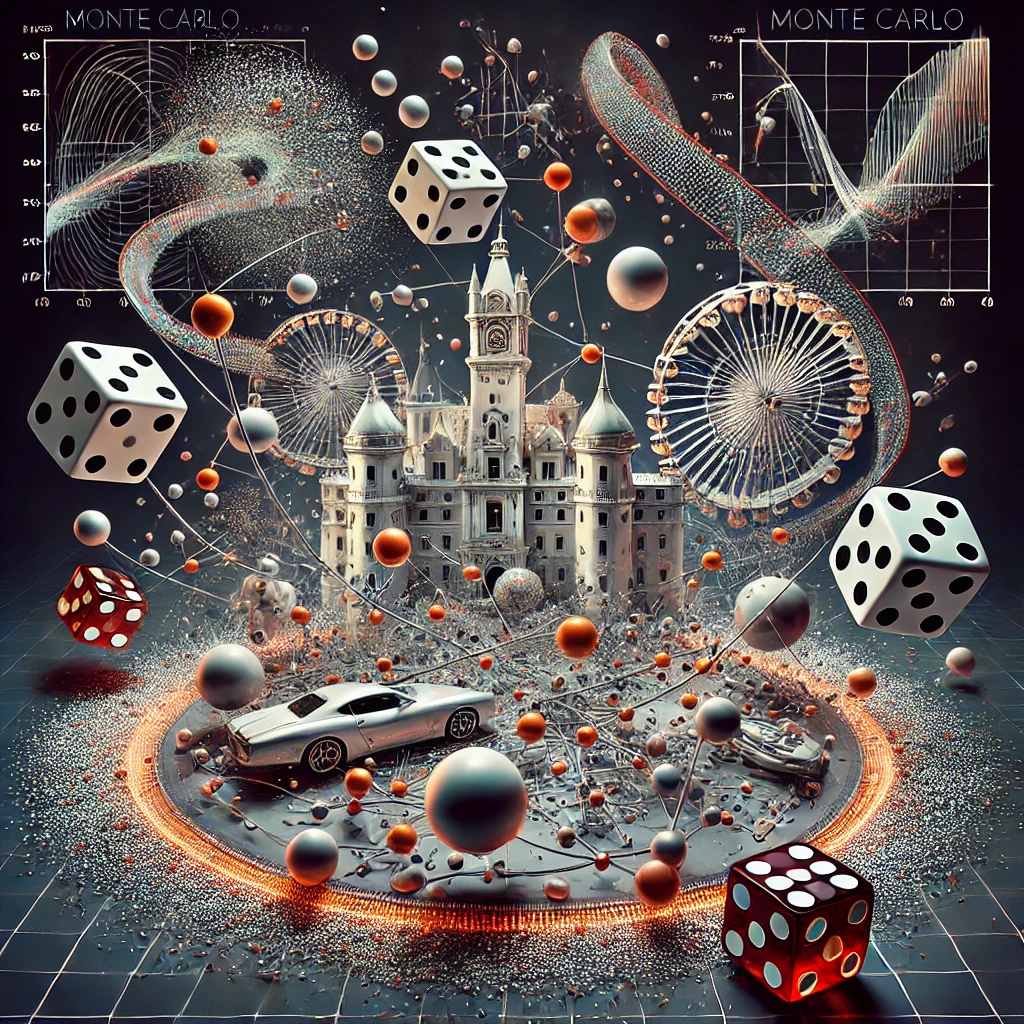
DALL-E generated image for Monte Carlo simulation.
The basic idea behind Monte Carlo methods is to use randomness to solve problems that might be deterministic in principle. For example, to estimate the value of π, one could randomly generate points in a square that encloses a quarter circle and count how many fall within the quarter circle. The ratio of points inside the quarter circle to the total number of points, multiplied by 4, gives an estimate of π. This is a simple example, but the same principle is applied to far more complex systems in physics.
At the heart of Monte Carlo simulations is the concept of random sampling. In this context, random sampling refers to generating a large number of random variables and using these to explore the possible states of a system. This is particularly powerful in systems where the state space is vast or continuous, making exhaustive enumeration impractical.
Monte Carlo methods can be used to solve a variety of problems in physics by simulating the probabilistic behavior of particles or fields. For instance, in statistical mechanics, Monte Carlo simulations are used to estimate the thermodynamic properties of systems at equilibrium by sampling the configurations of particles according to the Boltzmann distribution. In quantum field theory, they are used to evaluate path integrals, which are otherwise intractable due to the infinite-dimensional nature of the field configurations.
The power of Monte Carlo methods lies in their ability to model the probabilistic nature of physical systems. By averaging the results of many random samples, one can obtain a reliable approximation of the expected value of a quantity, even if the underlying process is highly complex and non-linear. The accuracy of the simulation generally improves as the number of samples increases, although this also increases computational cost.
Monte Carlo simulations are employed across a broad spectrum of physical problems. In statistical mechanics, they are used to study phase transitions by simulating the behavior of large ensembles of particles. For example, the Ising model, which describes ferromagnetism in materials, is often studied using Monte Carlo methods to understand how magnetic domains evolve at different temperatures.
In quantum field theory, Monte Carlo methods are used to calculate properties of quantum fields, such as vacuum expectation values and propagators, which are crucial for understanding particle interactions at a fundamental level. The lattice QCD (Quantum Chromodynamics) method, for instance, uses Monte Carlo simulations to study the behavior of quarks and gluons, the fundamental constituents of matter.
In particle physics, Monte Carlo methods are indispensable for simulating particle collisions in large detectors, such as those at the Large Hadron Collider (LHC). These simulations help physicists understand the outcomes of experiments by comparing the simulated data with actual experimental results.
Rust’s strong emphasis on safety and concurrency makes it an excellent choice for implementing Monte Carlo simulations. Below is a basic example of a Monte Carlo simulation in Rust, demonstrating how to estimate the value of π by randomly sampling points in a unit square.
use rand::Rng;
/// Estimates the value of π using Monte Carlo simulation with a given number of samples.
fn monte_carlo_pi(samples: usize) -> f64 {
let mut inside_circle = 0;
let mut rng = rand::thread_rng();
for _ in 0..samples {
let x: f64 = rng.gen(); // Generates a random float in [0, 1)
let y: f64 = rng.gen();
// Check if the point lies inside the unit circle.
if x * x + y * y <= 1.0 {
inside_circle += 1;
}
}
// The ratio of points inside the circle to the total number of points,
// multiplied by 4, approximates π.
4.0 * (inside_circle as f64) / (samples as f64)
}
fn main() {
let samples = 1_000_000;
let pi_estimate = monte_carlo_pi(samples);
println!("Estimated value of π with {} samples: {}", samples, pi_estimate);
}
The monte_carlo_pi
function exemplifies a Monte Carlo method by generating random $(x, y)$ points within a unit square using the rand::Rng
trait, which ensures these numbers are uniformly distributed between 0 and 1. The function checks each point to determine if it lies inside the unit circle by evaluating the condition $x^2 + y^2 \leq 1$; if true, it increments the inside_circle
counter. This process is repeated for a large number of points, and $π$ is then estimated by multiplying the ratio of points inside the circle to the total number of points by 4, reflecting the relationship between the area of the quarter-circle and $π/4$. Furthermore, Rust’s robust concurrency model allows this simulation to be optimized for multi-core systems by parallelizing the loop with the rayon
crate, distributing the computation across multiple threads to achieve faster and more efficient performance.
This basic example showcases how Monte Carlo simulations can be implemented in Rust. For more complex systems, one would typically extend this approach by using more sophisticated probabilistic models and possibly introducing parallel computation to handle the large number of samples required for accurate results.
7.2. Setting Up the Rust Environment for Monte Carlo Simulations
Setting up the Rust environment for Monte Carlo simulations involves configuring your development environment with the necessary tools, libraries, and dependencies to facilitate efficient and reliable computational physics code. Rust's performance, memory safety, and concurrency features make it an excellent choice for Monte Carlo methods, which typically require generating large amounts of random data and handling complex numerical computations. By leveraging Rust’s ecosystem, you can build simulations that are both fast and robust.
At the heart of a Monte Carlo simulation in Rust are crates such as rand for random number generation and ndarray for numerical operations. The rand crate offers a wide variety of random number generators and distributions, which are essential for simulating stochastic processes, while ndarray provides powerful tools for working with arrays and matrices—key components when dealing with large datasets and multidimensional numerical computations. Rust’s strong type system, zero-cost abstractions, and safe concurrency model ensure that your simulations are free from common bugs like data races and memory errors, even under heavy computational loads.
To set up your Rust environment for Monte Carlo simulations, you first need to install Rust (using [rustup](https://rustup.rs/)). Once installed, create a new project using Cargo, Rust’s build system and package manager. Next, add the necessary dependencies by modifying your Cargo.toml file to include the rand and ndarray crates:
[dependencies]
rand = "0.8"
ndarray = "0.15"
With these dependencies installed, you are ready to write Monte Carlo simulations in Rust. Consider the following example that demonstrates how to estimate the value of π using random sampling. In this example, the rand crate is used to generate random numbers uniformly in the interval \[0, 1), and a simple Monte Carlo method is applied to count the number of randomly generated points that fall within the unit circle. The result is used to estimate π:
use rand::Rng;
use ndarray::Array1;
/// Estimates the value of π using Monte Carlo simulation.
///
/// # Arguments
///
/// * `samples` - The number of random points to generate.
fn monte_carlo_pi(samples: usize) -> f64 {
let mut rng = rand::thread_rng();
let mut inside_circle = 0;
for _ in 0..samples {
let x: f64 = rng.gen();
let y: f64 = rng.gen();
// Check if the point (x, y) is inside the unit circle.
if x * x + y * y <= 1.0 {
inside_circle += 1;
}
}
// The ratio of points inside the circle to total samples, multiplied by 4,
// approximates the value of π.
4.0 * (inside_circle as f64) / (samples as f64)
}
fn main() {
let samples = 1_000_000;
let pi_estimate = monte_carlo_pi(samples);
println!("Estimated value of π with {} samples: {}", samples, pi_estimate);
// Example using ndarray for array operations
let arr = Array1::from_vec(vec![1.0, 2.0, 3.0]);
println!("Array example: {:?}", arr);
}
In this code, the rand::thread_rng() function initializes a random number generator that is safe for use in concurrent contexts. The simulation loops over a large number of samples, generating random (x, y) coordinates, and then checking whether those points lie inside the unit circle. The ratio of such points to the total number of samples is used to provide an estimate of π. Additionally, the code includes a simple demonstration of the ndarray crate by creating and printing a one-dimensional array.
Once your code is written, you can compile and run it using Cargo with the command:
cargo run
This command compiles the project and executes the main function, running both the Monte Carlo simulation and the example ndarray usage.
Overall, setting up your Rust environment for Monte Carlo simulations involves installing Rust, creating a new Cargo project, and adding the appropriate dependencies like rand and ndarray. Rust’s inherent performance and memory safety features, combined with its robust ecosystem, provide an ideal platform for scientific computing. By following these steps, you ensure that your simulations are developed in a high-performance, safe, and reliable manner—key qualities for complex computational physics tasks.
7.3. Implementing Random Number Generators in Rust
Random number generation is a cornerstone of Monte Carlo simulations and many other computational physics applications. In Rust, pseudo‐random number generators (PRNGs) produce sequences of numbers that mimic the properties of truly random sequences, even though they are deterministic. High-quality randomness is crucial in simulations because any bias or correlation in the generated numbers can lead to inaccurate or skewed results. Equally important is reproducibility; by seeding a PRNG with a fixed value, you can ensure that the same sequence of random numbers is generated across different runs, which is essential for debugging and validating scientific computations.
Two common types of PRNGs are the Mersenne Twister and Linear Congruential Generators (LCGs). The Mersenne Twister, known for its very long period (approximately 219937−12^{19937}-1) and excellent statistical properties, is widely used in simulations, while LCGs provide simplicity and speed at the cost of shorter periods and lesser randomness quality. In Rust, the rand crate offers a rich set of PRNGs, including implementations that follow these algorithms, as well as an array of utilities for generating numbers from various distributions.
Below is an example that demonstrates the basic usage of the rand crate to generate random numbers of different types:
use rand::Rng;
fn main() {
// Create a thread-local random number generator.
let mut rng = rand::thread_rng();
// Generate a random floating-point number between 0 and 1.
let random_f64: f64 = rng.gen();
println!("Random f64: {}", random_f64);
// Generate a random unsigned 32-bit integer.
let random_u32: u32 = rng.gen();
println!("Random u32: {}", random_u32);
// Generate a random integer in the range 1 to 100.
let random_range: i32 = rng.gen_range(1..101);
println!("Random i32 in range 1-100: {}", random_range);
}
In this example, rand::thread_rng()
creates a fast, thread-local PRNG (which, by default, is based on a high-quality algorithm like the Mersenne Twister), and the gen()
and gen_range()
methods produce random numbers of specified types and ranges.
For simulations where reproducibility is important—allowing you to generate the same sequence of random numbers for debugging or experimental verification—you can seed the PRNG manually. The SeedableRng trait in Rust allows you to create a PRNG with a defined starting seed, ensuring deterministic behavior:
use rand::{Rng, SeedableRng};
use rand::rngs::StdRng;
fn main() {
let seed: u64 = 12345;
// Create a seeded random number generator.
let mut rng: StdRng = SeedableRng::seed_from_u64(seed);
let random_f64: f64 = rng.gen();
println!("Random f64 with seed {}: {}", seed, random_f64);
let random_u32: u32 = rng.gen();
println!("Random u32 with seed {}: {}", seed, random_u32);
let random_range: i32 = rng.gen_range(1..101);
println!("Random i32 in range 1-100 with seed {}: {}", seed, random_range);
}
By initializing the PRNG with a fixed seed, you guarantee that each time the program is run with that seed, the same sequence of random numbers is produced. This reproducibility is vital when comparing simulation results or debugging numerical methods.
In many Monte Carlo simulations, it is also necessary to generate random numbers that follow specific probability distributions. For example, a simulation might require normally distributed values to model measurement noise or thermal fluctuations. The rand_distr crate, an extension to the rand ecosystem, provides a comprehensive set of distributions. The following code demonstrates generating random numbers from a normal distribution:
use rand::Rng;
use rand_distr::{Distribution, Normal};
fn main() {
let mut rng = rand::thread_rng();
// Create a standard normal distribution (mean = 0, standard deviation = 1).
let normal_dist = Normal::new(0.0, 1.0).unwrap();
// Sample a single random number from the normal distribution.
let random_normal: f64 = normal_dist.sample(&mut rng);
println!("Random number from normal distribution: {}", random_normal);
// Generate 10 random numbers following the standard normal distribution.
let random_normals: Vec<f64> = (0..10).map(|_| normal_dist.sample(&mut rng)).collect();
println!("10 random numbers from normal distribution: {:?}", random_normals);
}
In this example, Normal::new(0.0, 1.0)
constructs a normal distribution with mean 0 and standard deviation 1. By invoking sample(&mut rng)
, you generate random numbers that obey this distribution. This functionality is fundamental in modeling systems where the outcomes are influenced by stochastic processes.
Together, the rand and rand_distr crates provide robust, flexible, and reproducible methods for random number generation in Rust. These tools are essential for implementing Monte Carlo simulations, where the quality of randomness directly affects the validity of the simulation outcomes. By effectively leveraging these libraries, you can ensure that your computational physics applications produce accurate and reliable results while maintaining high performance and safety.
7.4. Designing Monte Carlo Simulation Algorithms
Monte Carlo simulations are powerful tools in computational physics for solving problems that are deterministic in principle but too complex to solve directly. The design of Monte Carlo algorithms revolves around the idea of random sampling to approximate solutions to these complex problems. Among the most important Monte Carlo methods are the Markov Chain Monte Carlo (MCMC) technique, the Metropolis-Hastings algorithm, and importance sampling. Each of these methods plays a crucial role in tackling different types of problems, from estimating integrals in high-dimensional spaces to sampling from probability distributions that are difficult to describe analytically.
Markov Chain Monte Carlo (MCMC) is a class of algorithms that generates samples from a probability distribution by constructing a Markov chain that has the desired distribution as its equilibrium distribution. The chain is then sampled at different points to estimate properties of the distribution. MCMC is particularly useful in scenarios where the dimensionality of the problem is high, making direct sampling infeasible.
The Metropolis-Hastings algorithm is one of the most widely used MCMC methods. It builds a Markov chain by proposing new states based on a simple, proposal distribution and then accepting or rejecting these states based on an acceptance criterion that ensures the correct equilibrium distribution. The power of the Metropolis-Hastings algorithm lies in its ability to sample from complex distributions by accepting or rejecting proposed states, making it ideal for simulations in fields like statistical mechanics and Bayesian inference.
Importance sampling is a technique used to reduce variance in Monte Carlo estimates by sampling from a distribution that is more representative of the regions of interest in the problem. This is especially useful when the probability distribution has areas with low probability that contribute significantly to the expected value. By reweighting samples according to their likelihood, importance sampling improves the efficiency of Monte Carlo simulations, making it possible to obtain accurate results with fewer samples.
The efficiency and convergence of Monte Carlo algorithms are critical considerations when designing simulations. Efficiency refers to the ability of an algorithm to produce accurate results with a minimal number of samples. Convergence, on the other hand, refers to the behavior of the algorithm as the number of iterations increases—specifically, whether the algorithm approaches the true distribution or value that is being estimated.
In the context of MCMC methods like the Metropolis-Hastings algorithm, efficiency can be influenced by the choice of the proposal distribution. If the proposal distribution is too narrow, the algorithm may take a long time to explore the state space, leading to slow convergence. Conversely, if the proposal distribution is too broad, the algorithm may frequently reject proposed states, also leading to inefficiency.
Convergence diagnostics are tools used to assess whether a Monte Carlo algorithm has converged. Techniques like trace plots, autocorrelation analysis, and the Gelman-Rubin statistic help determine whether the Markov chain has reached equilibrium and is properly sampling from the target distribution. Ensuring convergence is essential because premature termination of the algorithm can lead to biased results.
Implementing Monte Carlo algorithms in Rust involves taking advantage of the language’s performance features, such as zero-cost abstractions and strong type safety, while also utilizing available crates for random number generation and numerical operations. Below are implementation examples for the Metropolis-Hastings algorithm and importance sampling, followed by a discussion of optimization techniques.
Below is a basic implementation of the Metropolis-Hastings algorithm in Rust, which samples from a one-dimensional Gaussian target distribution. The code uses the rand and rand_distr crates for random number generation and handling distributions:
use rand::Rng;
use rand_distr::{Normal, Distribution};
/// Implements the Metropolis-Hastings algorithm to generate samples from a Gaussian distribution.
///
/// # Arguments
/// * `samples` - The number of samples to generate.
/// * `sigma` - The standard deviation of the proposal distribution.
fn metropolis_hastings(samples: usize, sigma: f64) -> Vec<f64> {
let mut rng = rand::thread_rng();
// Define the proposal distribution (a Gaussian centered at 0 with standard deviation sigma)
let normal = Normal::new(0.0, sigma).unwrap();
let mut x = 0.0; // Initial guess
let mut result = Vec::with_capacity(samples);
for _ in 0..samples {
// Propose a new state by adding a random perturbation.
let x_new = x + normal.sample(&mut rng);
// For the standard normal target distribution, the acceptance ratio simplifies:
// ratio = exp(-0.5 * (x_new^2 - x^2))
let acceptance_ratio = (-0.5 * (x_new.powi(2) - x.powi(2))).exp();
if rng.gen::<f64>() < acceptance_ratio {
x = x_new; // Accept the new state
}
result.push(x);
}
result
}
fn main() {
let samples = 10_000;
let sigma = 1.0; // Standard deviation for the proposal distribution.
let generated_samples = metropolis_hastings(samples, sigma);
println!("First 10 samples: {:?}", &generated_samples[..10]);
}
The metropolis_hastings
function implements the Metropolis-Hastings algorithm, a Markov Chain Monte Carlo (MCMC) method used to generate samples from a target distribution. In this function, a Gaussian distribution with a mean of 0 and a standard deviation of sigma
is used as the proposal distribution, centered around the current state x
. This ensures that new candidate states are proposed in the vicinity of the current state, allowing for a controlled exploration of the sample space. The acceptance ratio, which is crucial to the algorithm, is computed based on the target distribution—here, the standard normal distribution. This ratio determines the probability of accepting the proposed state: if a state has a higher probability under the target distribution, it is more likely to be accepted; otherwise, it may be rejected, in which case the current state is retained. The algorithm iteratively generates samples in this manner, gradually building a sequence of states that approximate the target distribution. The function ultimately returns a vector containing the samples generated by this process. In the main function, the algorithm is used to generate 10,000 samples, with the first 10 samples printed to demonstrate the output, showcasing how the Metropolis-Hastings algorithm efficiently explores the target distribution's landscape.
Another important Monte Carlo technique is importance sampling, which is used to reduce the variance of estimators by sampling from a proposal distribution that better captures the regions that contribute most to the integral or expected value. With importance sampling, each sample is assigned a weight to correct for the difference between the target and proposal distributions. The following example demonstrates how to perform importance sampling in Rust:
use rand::Rng;
use rand_distr::{Normal, Distribution};
/// Estimates the expected value of a function under a target distribution by importance sampling.
///
/// # Arguments
/// * `samples` - The number of samples to generate.
/// * `target` - A function that computes the target function value for a given input.
/// * `proposal_sigma` - The standard deviation of the proposal Gaussian distribution.
fn importance_sampling<F>(samples: usize, target: F, proposal_sigma: f64) -> f64
where
F: Fn(f64) -> f64,
{
let mut rng = rand::thread_rng();
let proposal = Normal::new(0.0, proposal_sigma).unwrap();
let mut total_weighted_value = 0.0;
let mut total_weight = 0.0;
for _ in 0..samples {
let x = proposal.sample(&mut rng);
// The weight is determined by the ratio of the target density to the proposal density.
// For a standard normal target and a standard normal proposal, the weight simplifies to exp(-0.5*x^2).
let weight = (-0.5 * x.powi(2)).exp();
total_weighted_value += target(x) * weight;
total_weight += weight;
}
total_weighted_value / total_weight
}
fn main() {
let samples = 10_000;
let proposal_sigma = 1.0;
// For example, estimate the expected value of exp(x) under the standard normal distribution.
let estimated_value = importance_sampling(samples, |x| x.exp(), proposal_sigma);
println!("Estimated expected value: {}", estimated_value);
}
The importance_sampling
function illustrates the process of estimating the expected value of a function under a target distribution by sampling from a different, more easily sampled distribution—known as the proposal distribution. In this code, the proposal distribution is a Gaussian distribution with a standard deviation of proposal_sigma
, chosen for its simplicity in generating samples. Since these samples are not drawn directly from the target distribution, each sample is assigned a weight, calculated as the likelihood ratio between the target distribution (here, a standard normal distribution) and the proposal distribution. This weighting adjusts for the discrepancy between the distributions, ensuring that the final estimate accurately reflects the target distribution. The expected value of a given function, such as the exponential function exp(x)
, is then calculated by taking the weighted sum of the function's values across all samples and dividing it by the sum of the weights. This method provides an efficient way to approximate expectations when direct sampling from the target distribution is difficult. In the main function, the code demonstrates the application of importance sampling by estimating the expected value of exp(x)
under a normal distribution, showcasing the practicality and effectiveness of this technique in scenarios where straightforward sampling is infeasible.
To optimize Monte Carlo simulations in Rust, consider the following techniques:
Parallelism: Rust’s
rayon
crate can be used to parallelize the sampling process, distributing the work across multiple CPU cores. This can significantly reduce computation time for large-scale simulations.Efficient Data Structures: Use Rust’s powerful type system to define efficient data structures that minimize memory usage and maximize performance. For example, use fixed-size arrays when possible to avoid the overhead associated with dynamic memory allocation.
Seeding for Reproducibility: Ensure reproducibility by carefully controlling the seeding of random number generators. This is critical for debugging and verifying the correctness of simulations.
Avoiding Numerical Instabilities: Pay close attention to potential sources of numerical instability, especially in algorithms that involve divisions or exponentiations. Use Rust’s
f64::EPSILON
or other techniques to handle small numbers safely.
Designing Monte Carlo simulation algorithms in Rust involves understanding fundamental techniques like MCMC, the Metropolis-Hastings algorithm, and importance sampling. The efficiency and convergence of these algorithms are critical for their success in computational physics. By leveraging Rust’s performance features and safety guarantees, you can implement robust and efficient Monte Carlo algorithms. The provided code examples demonstrate how these algorithms can be implemented in Rust, highlighting the importance of careful algorithm design and optimization in achieving accurate and efficient simulations.
7.5. Handling Large Datasets and Computational Efficiency
In computational physics, particularly when running Monte Carlo simulations, handling large datasets and ensuring computational efficiency are paramount. These simulations often involve processing extensive collections of particle states, large matrices representing discretized systems, or millions of random samples used to approximate physical quantities. Effective data management—which includes efficient storage, retrieval, and manipulation of data—is essential for maintaining both the speed and the accuracy of the computations.
One of the primary challenges in large-scale simulations is memory consumption. Large datasets can quickly exhaust available memory or force the system to perform excessive memory reallocations, which slow down the simulation. Rust’s ownership system helps manage memory in a predictable way, and its standard collections like Vec
are highly optimized. When the size of the dataset is known in advance, preallocating memory using methods like Vec::with_capacity()
can improve performance by minimizing the need for incremental memory growth.
For example, the following code preallocates a vector to store a large dataset of random floating-point numbers:
use rand::Rng;
fn generate_large_dataset(size: usize) -> Vec<f64> {
let mut dataset = Vec::with_capacity(size);
let mut rng = rand::thread_rng();
for _ in 0..size {
dataset.push(rng.gen());
}
dataset
}
fn main() {
let size = 10_000_000;
let dataset = generate_large_dataset(size);
println!("Generated a dataset with {} elements.", dataset.len());
}
In this snippet, preallocating the vector with with_capacity(size)
prevents repeated reallocations as new elements are added, which reduces overhead and improves performance when dealing with millions of entries.
Efficient data structures are also crucial. For example, the ndarray
crate provides multi-dimensional arrays optimized for numerical operations, making it ideal for scientific computing. Consider the following example, which initializes and populates a large matrix using ndarray
:
use ndarray::Array2;
fn main() {
let n = 1000;
// Create a 2D array (matrix) with dimensions n x n, all elements initialized to 0.0.
let mut matrix = Array2::<f64>::zeros((n, n));
// Populate the matrix with data, here simply using the sum of indices.
for i in 0..n {
for j in 0..n {
matrix[[i, j]] = (i + j) as f64;
}
}
println!("Matrix element [500, 500]: {}", matrix[[500, 500]]);
}
The Array2::zeros((n, n))
function efficiently allocates a two-dimensional array. The ndarray crate’s ability to perform operations on large arrays, combined with Rust’s safety guarantees, makes it well-suited for handling the data-intensive tasks that are common in Monte Carlo and other numerical simulations.
Parallel processing further enhances computational efficiency. Monte Carlo simulations are inherently parallel since each random sample is independent. Rust’s Rayon crate provides an easy way to parallelize computations using parallel iterators. Below is an example that demonstrates how to parallelize a Monte Carlo simulation to estimate the value of π:
use rand::Rng;
use rayon::prelude::*;
/// Estimates the value of π using Monte Carlo simulation in parallel.
fn monte_carlo_pi_parallel(samples: usize) -> f64 {
let inside_circle: usize = (0..samples)
.into_par_iter() // Convert the range into a parallel iterator.
.map(|_| {
let mut rng = rand::thread_rng();
let x: f64 = rng.gen();
let y: f64 = rng.gen();
if x * x + y * y <= 1.0 { 1 } else { 0 }
})
.sum();
4.0 * (inside_circle as f64) / (samples as f64)
}
fn main() {
let samples = 10_000_000;
let pi_estimate = monte_carlo_pi_parallel(samples);
println!("Estimated value of π with {} samples: {}", samples, pi_estimate);
}
This implementation leverages Rayon’s into_par_iter()
to distribute the workload of generating random points and checking them across multiple threads. By parallelizing the loop, the simulation can process a significantly larger number of samples in less time compared to a sequential approach, which is critical for reducing statistical error in Monte Carlo estimates.
In summary, handling large datasets and optimizing computational efficiency are central to robust Monte Carlo simulations. Using techniques such as preallocating memory with Vec::with_capacity()
, leveraging efficient data structures from crates like ndarray
, and parallelizing computations with Rayon can dramatically improve performance. Rust’s strong memory safety model and zero-cost abstractions ensure that these optimizations do not compromise the safety or clarity of your code, making it an excellent choice for high-performance scientific computing.
7.6. Visualizing and Analyzing Results
In Monte Carlo simulations, the ability to visualize and analyze the results is crucial for interpreting the outcomes and drawing meaningful conclusions. Data visualization serves as a powerful tool to present complex data in an intuitive and accessible way, enabling researchers to identify patterns, trends, and anomalies that might not be immediately apparent from raw data alone. Visualization techniques commonly used in the analysis of Monte Carlo simulations include histograms, scatter plots, line graphs, and heatmaps. These techniques help in understanding the distribution of sampled data, the convergence of simulation results, and the overall behavior of the system being studied.
Effective data visualization in computational physics also requires understanding the underlying statistical properties of the data. This includes calculating and visualizing measures of central tendency (e.g., mean, median), dispersion (e.g., variance, standard deviation), and distribution (e.g., probability density functions). These statistical tools help in estimating errors, assessing the accuracy of the simulation, and validating the results against theoretical expectations or experimental data.
Interpreting the results of Monte Carlo simulations involves understanding the statistical nature of the data generated by the simulation. Since Monte Carlo methods rely on random sampling, the results are inherently probabilistic, and their accuracy depends on the number of samples taken. The more samples that are generated, the closer the simulation results are likely to be to the true value.
Statistical analysis of Monte Carlo results often involves estimating the mean and variance of the quantities of interest, which can be used to compute confidence intervals and assess the reliability of the simulation. For example, the standard error of the mean provides an estimate of how much the sample mean is expected to vary from the true mean, and confidence intervals can be constructed to give a range within which the true value is likely to lie with a certain probability.
Error estimation is another key aspect of analyzing Monte Carlo simulation results. In practice, the errors in Monte Carlo simulations can arise from both statistical fluctuations (due to finite sampling) and systematic biases (due to the simulation model or numerical methods used). By performing a thorough error analysis, researchers can quantify the uncertainties in their results and ensure that they are making robust conclusions.
While Rust excels in performance and safety for computational tasks, data visualization is typically performed using specialized tools and libraries that may not be native to Rust. However, Rust can be easily integrated with powerful visualization tools like Python’s Matplotlib or data analysis frameworks like Jupyter Notebooks. This can be done by exporting data from Rust and then using external tools to generate the visualizations.
For visualizing Monte Carlo simulation results directly in Rust, you can use libraries like plotters
, which provides functionality for creating various types of plots and charts.
Here’s how you can create a histogram to visualize the distribution of results from a Monte Carlo simulation using the plotters
crate:
use plotters::prelude::*;
use rand::Rng;
/// Runs a Monte Carlo simulation to estimate π using a given number of samples.
fn monte_carlo_pi(samples: usize) -> f64 {
let mut rng = rand::thread_rng();
let mut inside_circle = 0;
for _ in 0..samples {
let x: f64 = rng.gen();
let y: f64 = rng.gen();
if x * x + y * y <= 1.0 {
inside_circle += 1;
}
}
4.0 * (inside_circle as f64) / (samples as f64)
}
fn main() -> Result<(), Box<dyn std::error::Error>> {
let simulation_runs = 10_000;
let bin_count = 20; // Number of bins for the histogram
// Perform multiple simulation runs to obtain a distribution of π estimates.
let pi_estimates: Vec<f64> = (0..simulation_runs)
.map(|_| monte_carlo_pi(1_000))
.collect();
// Create bins for the histogram.
let min_pi = 3.0;
let max_pi = 4.0;
let bin_width = (max_pi - min_pi) / bin_count as f64;
let mut bins = vec![0; bin_count];
for &pi in &pi_estimates {
let bin = ((pi - min_pi) / bin_width).floor() as usize;
if bin < bin_count {
bins[bin] += 1;
}
}
// Set up the drawing area for the histogram using the plotters crate.
let root = BitMapBackend::new("histogram.png", (640, 480)).into_drawing_area();
root.fill(&WHITE)?;
let max_count = *bins.iter().max().unwrap();
let mut chart = ChartBuilder::on(&root)
.caption("Histogram of π Estimates", ("sans-serif", 40))
.margin(10)
.x_label_area_size(40)
.y_label_area_size(40)
.build_cartesian_2d(min_pi..max_pi, 0..max_count)?;
chart.configure_mesh().draw()?;
// Draw the histogram as a series of rectangles.
chart.draw_series(
bins.iter().enumerate().map(|(i, &count)| {
let bin_start = min_pi + i as f64 * bin_width;
let bin_end = bin_start + bin_width;
Rectangle::new(
[(bin_start, 0), (bin_end, count)],
BLUE.filled(),
)
}),
)?;
println!("Histogram saved to 'histogram.png'.");
Ok(())
}
The code demonstrates the process of estimating the value of π using a Monte Carlo simulation and visualizing the results with a histogram. The monte_carlo_pi
function runs the simulation with a specified number of samples to approximate π, and the main function executes this simulation multiple times to obtain a distribution of π estimates. To visualize these estimates, the plotters
crate is employed to create a histogram. The BitMapBackend::new("histogram.png", (640, 480))
function initializes an image backend for plotting, setting the output to a PNG file with a resolution of 640x480 pixels and a white background. The ChartBuilder::on(&root)
function configures the chart by defining margins, labels, and axes, while the caption
method adds a title to the histogram, and build_cartesian_2d(3.0..4.0, 0..200)?
sets up the Cartesian coordinate system with defined ranges for the x and y axes. Finally, the draw_series
method creates and plots the vertical histogram using the π estimates, generating a visual representation of the simulation results which is saved as a PNG file. This approach provides a clear graphical depiction of how the estimates of π vary across different runs of the simulation.
This example demonstrates how to integrate Rust with the plotters
crate for direct visualization of Monte Carlo simulation results. The histogram created in this example shows the distribution of the estimated values of π, allowing you to visually assess the accuracy and precision of the simulation.
For more complex or interactive visualizations, it is often practical to export the data from Rust to be visualized using external tools like Python's Matplotlib or Jupyter Notebooks. Data can be exported in common formats like CSV or JSON.
use std::fs::File;
use std::io::Write;
fn main() -> std::io::Result<()> {
let samples = 10_000;
let pi_estimates: Vec<f64> = (0..samples)
.map(|_| monte_carlo_pi(1_000))
.collect();
let mut file = File::create("pi_estimates.csv")?;
for estimate in pi_estimates {
writeln!(file, "{}", estimate)?;
}
Ok(())
}
The code snippet illustrates how to export $π$ estimates generated from a Monte Carlo simulation to a CSV file, enabling seamless integration with various data analysis and visualization tools. By writing the simulation results to a CSV file, the data can be easily imported into tools like Python or R, which offer advanced libraries and functionalities for detailed analysis and graphical representation. This approach leverages Rust’s high performance and efficiency during the simulation process, while capitalizing on the extensive capabilities of external tools for further data processing. Exporting data to CSV is a strategic way to combine Rust's computational speed with the analytical power and visualization options provided by other languages, facilitating comprehensive exploration and presentation of the results. This interoperability enhances the overall workflow, making it possible to perform complex analyses and generate insightful visualizations without sacrificing the efficiency of the simulation phase.
Visualizing and analyzing the results of Monte Carlo simulations is a crucial step in understanding the behavior of the system under study and ensuring the accuracy of the simulation. Rust, while primarily a systems programming language, offers robust tools like the plotters
crate for generating visualizations directly. For more complex visualizations, Rust can seamlessly integrate with external tools by exporting data in standard formats like CSV. By combining Rust’s computational efficiency with powerful visualization tools, researchers can effectively interpret the results of their simulations, perform statistical analyses, and estimate errors, leading to more accurate and reliable scientific conclusions.
7.7. Case Studies and Applications in Computational Physics
Monte Carlo simulations have become indispensable in computational physics, providing a flexible framework to tackle problems that are analytically intractable or prohibitively expensive with deterministic methods. Their versatility allows researchers to study a wide variety of systems—from the microscopic interactions of particles to macroscopic phenomena—and to validate theoretical models against experimental data. Below, we explore several case studies that demonstrate the practical applications of Monte Carlo simulations in physics, using Rust to implement efficient, high-performance algorithms.
Case Study 1: The Ising Model in Statistical Mechanics
The Ising model is a classic tool for understanding ferromagnetism and phase transitions in statistical mechanics. It represents a lattice of spins, where each spin can be in one of two states (up or down), and the interactions between neighboring spins determine the system’s total energy. Monte Carlo simulations using the Metropolis-Hastings algorithm can sample spin configurations according to the Boltzmann distribution, thereby modeling how the system evolves towards equilibrium.
Below is an example implementation in Rust that uses a 2D lattice of spins and applies the Metropolis-Hastings algorithm to simulate the Ising model:
use rand::Rng;
/// Simulates the 2D Ising model using the Metropolis-Hastings algorithm.
///
/// # Arguments
/// * `n` - The size of the lattice (n x n).
/// * `temperature` - The temperature of the system.
/// * `iterations` - The number of iterations to perform.
fn ising_model_metropolis(n: usize, temperature: f64, iterations: usize) -> Vec<i32> {
let mut rng = rand::thread_rng();
// Initialize the lattice with all spins set to +1.
let mut spins = vec![1; n * n];
for _ in 0..iterations {
for _ in 0..n * n {
let i = rng.gen_range(0..n);
let j = rng.gen_range(0..n);
let index = |i, j| ((i % n) * n) + (j % n);
// Compute the local energy change directly here
let delta_e = 2
* spins[index(i, j)]
* (spins[index(i + 1, j)] + spins[index(i.wrapping_sub(1), j)]
+ spins[index(i, j + 1)] + spins[index(i, j.wrapping_sub(1))]);
if delta_e <= 0 || rng.gen::<f64>() < (-delta_e as f64 / temperature).exp() {
spins[index(i, j)] *= -1; // Flip the spin
}
}
}
spins
}
fn main() {
let n = 20;
let temperature = 2.0;
let iterations = 1_000_000;
let final_spins = ising_model_metropolis(n, temperature, iterations);
// Display the first row of the lattice as a demonstration.
println!("Final spin configuration (first row): {:?}", &final_spins[..n]);
}
In this simulation, each spin on a 20×20 lattice is updated based on its interactions with its neighbors. The algorithm computes the change in energy resulting from a possible spin flip and accepts the new configuration according to the Metropolis criterion. Analyzing the final spin configuration can help determine quantities like the magnetization, which are key to understanding the model's phase transitions.
Case Study 2: Neutron Transport in Nuclear Physics
Monte Carlo methods are widely used in nuclear physics to model neutron transport. In these simulations, neutrons are tracked as they traverse a medium, with random interactions such as scattering, absorption, and fission modeled probabilistically. The resulting data can be used to calculate important quantities like neutron flux and reaction rates, which are critical for reactor design and radiation shielding.
Consider the following Rust example that simulates neutron transport by tracking the distance that neutrons travel before they are absorbed:
use rand::Rng;
/// Simulates neutron transport by tracking the distance each neutron travels until absorption.
///
/// # Arguments
/// * `neutrons` - The number of neutrons to simulate.
/// * `steps` - The maximum number of steps a neutron can take.
fn neutron_transport_simulation(neutrons: usize, steps: usize) -> Vec<f64> {
let mut rng = rand::thread_rng();
let mut distances = Vec::with_capacity(neutrons);
for _ in 0..neutrons {
let mut distance = 0.0;
for _ in 0..steps {
// Generate a random step length.
let step_length: f64 = rng.gen();
// Randomly decide whether the neutron is absorbed (10% chance).
let interaction: f64 = rng.gen();
distance += step_length;
if interaction < 0.1 {
break;
}
}
distances.push(distance);
}
distances
}
fn main() {
let neutrons = 10_000;
let steps = 100;
let distances = neutron_transport_simulation(neutrons, steps);
let average_distance: f64 = distances.iter().sum::<f64>() / distances.len() as f64;
println!("Average distance traveled by neutrons: {}", average_distance);
}
Here, the simulation tracks 10,000 neutrons, each taking up to 100 steps. In each step, a random distance is added and a random number determines whether the neutron is absorbed. The average distance traveled by the neutrons provides insight into the material's neutron transport properties.
Case Study 3: Monte Carlo Integration in Electromagnetism
Monte Carlo integration is a versatile method used to approximate the value of an integral, particularly useful for high-dimensional integrals that are challenging to solve analytically. In electromagnetism, Monte Carlo integration can be employed to estimate potentials or fields in complex geometries. The method involves randomly sampling points in the integration domain, evaluating the integrand at these points, and averaging the results scaled by the domain's volume.
The following Rust example estimates the integral of the sine function over the interval \[0,π\]\[0, \\pi\], which can be related to problems in electromagnetism:
use rand::Rng;
/// Estimates the integral of a function `f` over the interval [a, b] using Monte Carlo integration.
///
/// # Arguments
/// * `f` - The function to integrate.
/// * `samples` - The number of samples to use.
/// * `a` - The lower bound of the integration interval.
/// * `b` - The upper bound of the integration interval.
fn monte_carlo_integration<F>(f: F, samples: usize, a: f64, b: f64) -> f64
where
F: Fn(f64) -> f64,
{
let mut rng = rand::thread_rng();
let mut sum = 0.0;
for _ in 0..samples {
let x = rng.gen_range(a..b);
sum += f(x);
}
(b - a) * sum / samples as f64
}
fn main() {
let samples = 1_000_000;
let integral = monte_carlo_integration(|x| x.sin(), samples, 0.0, std::f64::consts::PI);
println!("Estimated value of the integral: {}", integral);
}
In this example, the Monte Carlo method approximates the integral of sin(x)\\sin(x) over \[0,π\]\[0, \\pi\] by generating one million random samples. The function values are averaged and then scaled by the length of the interval. Comparing the numerical result with the known analytical solution helps validate the accuracy of the technique.
Integrating Monte Carlo Simulations in Real-World Applications
The case studies detailed above illustrate how Monte Carlo simulations can be tailored to different areas of computational physics:
The Ising Model uses random sampling to simulate spin configurations and study phase transitions in magnetic systems.
Neutron Transport models the probabilistic interactions of neutrons within a medium to assess critical parameters like neutron flux.
Monte Carlo Integration provides a flexible method for approximating high-dimensional integrals, which are common in electromagnetism and other fields.
In each case, Monte Carlo simulations not only provide valuable insights into physical processes but also help validate theoretical models against experimental data. Rust’s strong safety guarantees, performance optimizations, and robust ecosystem—including libraries for random number generation, matrix operations, and data visualization—make it an excellent platform for implementing these complex simulations.
By effectively designing and deploying Monte Carlo simulations in Rust, researchers can harness the method’s power to address challenging problems in computational physics, explore novel phenomena, and generate insights that drive scientific progress.
7.8. Conclusion
Chapter 7 demonstrates how Monte Carlo simulations, when implemented using Rust, can significantly advance the field of computational physics. By leveraging Rust's strengths in performance and safety, this chapter equips readers with the knowledge to effectively apply Monte Carlo methods to complex physical systems, optimize simulations, and analyze results with precision.
7.8.1. Further Learning with GenAI
These prompts are designed to elicit comprehensive and technically detailed responses, enabling a robust grasp of Monte Carlo methods, Rust programming features, and their integration in computational physics.
Explain the mathematical foundations and theoretical principles of Monte Carlo simulations, providing a detailed exploration of their stochastic nature, probability theory, and how they model systems using random variables. Analyze key concepts such as probability distributions, random sampling techniques (e.g., uniform, Gaussian), and discuss how these affect the accuracy, variance, and convergence of Monte Carlo methods in computational physics. Include examples of typical physical systems where Monte Carlo methods outperform deterministic algorithms.
Describe the process of setting up a Rust environment specifically tailored for Monte Carlo simulations, detailing the installation of essential crates like
rand
for random number generation andndarray
for efficient data handling. Explain how Rust’s safety features (e.g., ownership, memory safety) and performance optimizations (e.g., zero-cost abstractions) make it well-suited for high-performance scientific computing. Provide configuration examples for managing dependencies and project setup inCargo.toml
.Provide a detailed examination of various random number generation algorithms, including the Mersenne Twister, linear congruential generators (LCG), and cryptographically secure generators. Discuss their implementation in Rust using the
rand
crate, assess the statistical quality, periodicity, and performance of each algorithm, and explore best practices for ensuring reproducibility in Monte Carlo simulations. Address potential pitfalls in random number generation that can affect simulation accuracy.Outline the design and implementation of different Monte Carlo simulation algorithms, with an emphasis on Markov Chain Monte Carlo (MCMC) methods such as the Metropolis-Hastings algorithm, and techniques like importance sampling. Analyze their convergence properties, discuss how to assess and ensure mixing and convergence in practice, and explore the trade-offs in efficiency and computational cost. Provide practical coding examples in Rust, including detailed explanations of how to structure and optimize the algorithms.
Analyze strategies for handling and processing large datasets in Monte Carlo simulations, addressing efficient storage, retrieval, and memory management techniques. Discuss how Rust’s ownership model, borrowing, and concurrency features, such as multithreading or async/await, can be leveraged to process large datasets efficiently. Provide examples of parallel data processing, in-memory data structures like
ndarray
, and considerations for optimizing I/O operations in high-performance simulations.Explore methods for visualizing Monte Carlo simulation results using Rust, detailing how Rust can integrate with visualization libraries (e.g., Plotters, Gnuplot) or external tools (e.g., Python’s Matplotlib). Discuss techniques for visualizing large datasets, Monte Carlo sampling distributions, and statistical analysis of results. Provide practical examples of producing effective visualizations for simulation outcomes, including histograms, scatter plots, and convergence diagnostics.
Present detailed case studies where Monte Carlo simulations are applied to complex physical systems, such as lattice QCD, phase transitions in the Ising model, or financial risk modeling. For each case study, outline the specific challenges encountered (e.g., high-dimensional integrals, complex boundary conditions), the algorithms employed, and how Rust’s performance features were used to optimize the simulations. Highlight the lessons learned and insights gained from the application of Monte Carlo methods in these contexts.
Examine advanced Monte Carlo techniques, including nested sampling and simulated annealing, providing detailed algorithmic descriptions and their implementations in Rust. Explore how these techniques are used to address high-dimensional spaces, optimization problems, and systems with multiple local minima. Discuss practical considerations in applying these methods to real-world problems in physics and engineering, including convergence behavior and computational cost.
Describe methods for error estimation and uncertainty quantification in Monte Carlo simulations, discussing variance reduction techniques (e.g., control variates, importance sampling), confidence intervals, and statistical error estimation methods. Explain how Rust’s type system, error handling (e.g.,
Result
andOption
types), and functional programming features contribute to more reliable and maintainable code. Provide examples of error estimation routines in Monte Carlo simulations, ensuring robust uncertainty quantification.Discuss approaches to parallelizing Monte Carlo simulations in Rust, including the use of threads, asynchronous programming, and Rust’s concurrency models (e.g., channels,
Rayon
). Provide practical examples of parallelizing Monte Carlo algorithms such as MCMC or particle simulations, analyzing how these techniques improve performance and scalability. Explore challenges in maintaining memory safety and preventing data races in parallel simulations.Analyze performance optimization strategies for Monte Carlo simulations in Rust, discussing algorithmic improvements (e.g., reducing variance, optimizing sampling methods), hardware utilization (e.g., SIMD, multi-core processors), and the use of profiling tools (e.g.,
perf
,cargo-profiler
) to identify bottlenecks. Explain how to optimize memory access patterns and use Rust’s low-level control over data structures to maximize computational efficiency in large-scale Monte Carlo simulations.Explain how to integrate Rust with external libraries and tools for advanced Monte Carlo simulation tasks, such as interfacing with high-performance libraries like BLAS, LAPACK, or GSL, or connecting with data analysis tools (e.g., NumPy in Python). Provide examples of how to set up Foreign Function Interface (FFI) in Rust and discuss the benefits and challenges of integrating Rust with these external tools for enhancing Monte Carlo simulation capabilities.
Provide techniques for applying Monte Carlo simulations to complex physical systems, such as high-dimensional integrals, multi-scale problems, or systems with many interacting particles (e.g., molecular dynamics or plasma simulations). Discuss specific challenges like slow convergence, high computational cost, and dimensionality, and propose solutions such as adaptive sampling, importance sampling, or hybrid algorithms. Provide implementation examples in Rust.
Discuss debugging techniques for identifying and resolving issues in Monte Carlo simulations coded in Rust, highlighting common pitfalls such as random number generator issues, sampling biases, and numerical instability. Provide best practices for testing, including unit tests, property-based testing, and validation against known solutions. Offer practical examples of troubleshooting techniques and how to ensure the robustness of Monte Carlo simulation code.
Offer guidance on developing custom Monte Carlo algorithms tailored to specific research needs, such as rare-event simulations or domain-specific optimizations (e.g., sampling in quantum mechanics or particle physics). Discuss design considerations, implementation strategies in Rust, and trade-offs between complexity, performance, and flexibility. Include examples of bespoke Monte Carlo algorithms and how they compare to standard methods in terms of efficiency and accuracy.
Explain the statistical mechanics principles underlying Monte Carlo methods, including concepts such as the Boltzmann distribution, phase transitions, and partition functions. Discuss how Monte Carlo methods simulate equilibrium properties and sample phase spaces, and explore how Rust’s numerical capabilities, such as efficient matrix and vector operations, can be leveraged to solve statistical mechanics problems. Provide examples of Monte Carlo applications in thermodynamics and condensed matter physics.
Discuss how Rust’s concurrency features, such as async/await and channels, can be applied to enhance Monte Carlo simulations, focusing on their role in improving parallelism, load balancing, and performance. Provide practical examples of how concurrent simulations can be structured to take advantage of modern multi-core architectures, and analyze the benefits and challenges of implementing these features in large-scale simulations.
Compare Monte Carlo simulations with other numerical methods used in computational physics, such as finite element analysis, molecular dynamics, and lattice Boltzmann methods. Discuss the strengths, limitations, and appropriate use cases for each method, particularly in terms of handling stochasticity, boundary conditions, and high-dimensional problems. Provide practical examples of when Monte Carlo methods are preferable and how they can complement other numerical techniques.
Analyze how Rust’s ownership and borrowing system impacts memory management in Monte Carlo simulations, focusing on strategies for optimizing memory usage in large-scale simulations. Discuss techniques for ensuring data integrity, minimizing memory fragmentation, and efficiently managing large datasets without incurring performance penalties. Provide examples of memory management strategies in Rust that enhance the performance of Monte Carlo simulations.
Investigate emerging trends and innovations in Monte Carlo simulations, such as the use of quantum computing, machine learning-enhanced Monte Carlo methods, and advancements in hardware accelerators (e.g., GPUs, TPUs). Discuss how Rust can be applied to these cutting-edge developments, and explore the potential for Rust-based Monte Carlo simulations to contribute to breakthrough research in these areas.
Explore the educational and practical applications of Monte Carlo simulations in various scientific and engineering fields, such as teaching stochastic processes, simulating financial markets, or modeling physical systems in climate science. Discuss how Monte Carlo methods can be used for both teaching and real-world problem-solving, and examine the role of Rust in providing an efficient and safe platform for implementing these applications.
Remember, as you dive into the intricate details and advanced techniques, you are not just learning a new technology but contributing to the forefront of computational physics. Let your curiosity and dedication drive you to excel, and you’ll find yourself making meaningful strides in solving complex problems and advancing knowledge in the field.
7.8.2. Assignments for Practice
These exercises are designed to challenge you and deepen your understanding of Monte Carlo simulations and Rust programming.
Exercise 7.1: Deep Dive into Random Number Generators
Objective: Gain a thorough understanding of random number generation algorithms, their implementation, and their effects on simulation results.
Ask GenAI to provide a comprehensive comparison of various random number generation algorithms such as the Mersenne Twister, linear congruential generators, and other advanced methods. Request detailed explanations of their mathematical foundations, implementation in Rust using the
rand
crate, and their impact on Monte Carlo simulation accuracy. Additionally, ask for practical examples of how to evaluate the quality and reproducibility of random numbers in different simulation scenarios.
Exercise 7.2: Advanced Monte Carlo Algorithms Implementation
Objective: Develop proficiency in implementing and understanding advanced Monte Carlo algorithms and their applications in Rust.
Engage GenAI in a detailed discussion on advanced Monte Carlo algorithms such as nested sampling and simulated annealing. Request a step-by-step guide for implementing these algorithms in Rust, including code examples and explanations of their theoretical underpinnings. Inquire about their specific use cases in computational physics, their strengths and weaknesses, and how they address complex problem spaces.
Exercise 7.3: Handling and Processing Large Datasets
Objective: Learn advanced techniques for data management and optimization in Monte Carlo simulations using Rust.
Use GenAI to explore advanced strategies for managing and processing large datasets in Monte Carlo simulations. Ask for a detailed explanation of data management techniques, including efficient storage solutions and retrieval methods. Request examples of how Rust’s ownership system and concurrency features can be used to optimize computational efficiency for large-scale simulations. Additionally, seek guidance on using Rust’s
ndarray
crate for handling multidimensional data arrays.
Exercise 7.4: Visualization and Analysis of Simulation Results
Objective: Master the skills of visualizing and analyzing Monte Carlo simulation results using Rust and relevant tools.
Request GenAI’s guidance on integrating Rust with various visualization libraries and tools for analyzing Monte Carlo simulation results. Ask for detailed examples of how to use these libraries to create effective visual representations of data, including plots, histograms, and other statistical analyses. Discuss best practices for interpreting simulation outcomes and how to present findings clearly and meaningfully.
Exercise 7.5: Debugging Complex Monte Carlo Simulations
Objective: Enhance debugging skills and learn how to develop and refine custom Monte Carlo algorithms effectively in Rust.
Engage GenAI in a detailed discussion on debugging Monte Carlo simulations in Rust. Request a comprehensive guide on common issues that arise in simulations, along with practical examples and solutions. Ask for advice on best practices for testing, validating, and troubleshooting simulations to ensure their correctness and reliability. Additionally, seek insights into developing custom Monte Carlo algorithms tailored to specific research needs, including design considerations and implementation challenges.
Approach each exercise with curiosity and determination, and let the knowledge you acquire propel you toward innovative solutions and breakthroughs. Embrace the journey of learning and practice, and allow your expertise to make a significant impact in the field of computational physics.
Comments