Chapter 10
Parallel and Distributed Computing in Rust
"To invent is to strive to reach the ideal, and the only way to achieve it is to improve and develop continuously." — Albert Einstein
Chapter 10 provides a comprehensive exploration of parallel and distributed computing using Rust, essential for tackling complex computational physics problems. It begins with the fundamentals of parallel computing and Rust’s concurrency model, emphasizing safety and efficiency. The chapter then delves into asynchronous programming, explaining its relevance and implementation in Rust. It covers distributed computing concepts, detailing the intricacies of implementing distributed systems. The chapter also discusses parallel algorithms, providing practical examples and performance evaluations. Real-world case studies illustrate the application of these techniques in computational physics, highlighting Rust’s capabilities in handling large-scale, concurrent computations.
10.1. Introduction to Parallel and Distributed Computing
Parallel and distributed computing have become indispensable tools in the realm of computational physics, offering the ability to tackle complex, large-scale problems that would otherwise be intractable on a single machine. At its core, parallel computing involves the simultaneous execution of multiple computational tasks, which can significantly reduce the time required to perform large-scale simulations or data processing tasks. This is achieved by leveraging the capabilities of multi-core processors or multiple processors within a single machine. On the other hand, distributed computing extends this concept by distributing tasks across multiple machines or nodes, enabling the system to scale out and handle problems of a much larger magnitude. This distinction is crucial in computational physics, where the complexity and size of the problems often exceed the capabilities of a single machine.
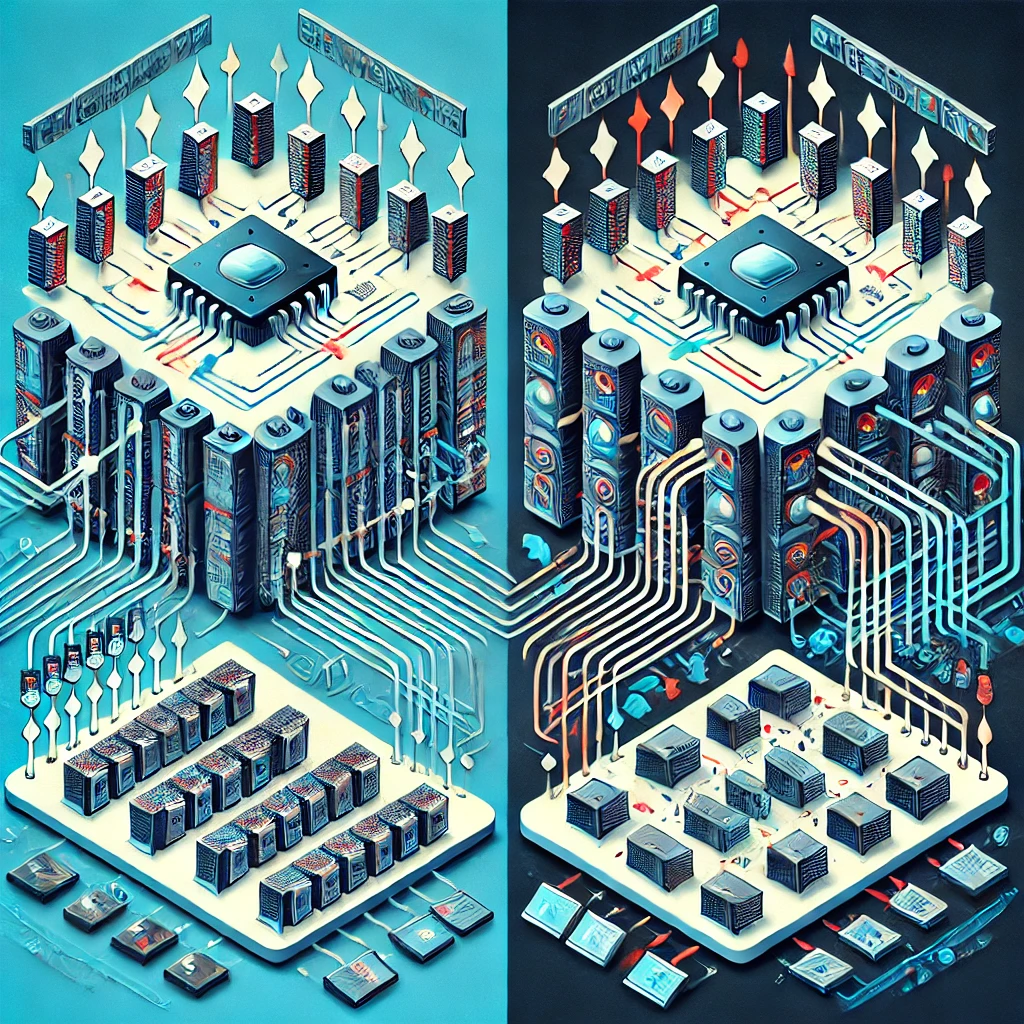
DALL-E illustration for parallel and distributed computing.
In the context of computational physics, the distinction between parallelism and concurrency is particularly important. Parallelism refers to the execution of multiple computations simultaneously, typically across multiple processors or cores. This is often used to speed up the processing of large datasets or to perform multiple independent simulations concurrently. Concurrency, however, is concerned with the structuring of a program to handle multiple tasks that can run independently, but not necessarily at the same time. Concurrency involves designing systems that can manage multiple tasks efficiently, ensuring that resources are used effectively and that the system remains responsive. In Rust, the distinction between parallelism and concurrency is clearly defined, and the language provides robust support for both, making it an ideal choice for developing high-performance applications in computational physics.
Distributed computing, on the other hand, is the process of coordinating tasks across multiple machines. This approach is essential when the computational resources of a single machine are insufficient to handle the problem at hand. Distributed computing systems must be carefully designed to manage communication between nodes, ensure data consistency, and provide fault tolerance. These challenges are particularly pronounced in computational physics, where distributed computing enables the simulation of large systems, such as weather models or large-scale particle simulations, by dividing the problem into smaller parts that can be processed concurrently on different machines. The ability to scale out across multiple machines is a key advantage of distributed computing, allowing computational physicists to tackle problems that would be impossible to solve on a single machine.
In the realm of parallel computing, two primary paradigms emerge: data parallelism and task parallelism. Data parallelism involves dividing a dataset into smaller chunks and processing each chunk in parallel. This approach is particularly useful in simulations where different sections of a grid or different elements of a dataset can be processed independently. For example, in a fluid dynamics simulation, different regions of the fluid can be simulated simultaneously, with each processor handling a specific region of the grid. This can significantly reduce the time required to perform the simulation, as each processor works on a different part of the problem concurrently.
Task parallelism, on the other hand, involves executing different tasks or functions in parallel, which may or may not operate on the same data. In computational physics, this could mean running different parts of a simulation concurrently, such as performing force calculations and updating particle positions in a molecular dynamics simulation. Task parallelism is particularly useful when different parts of the problem can be executed independently, allowing the system to make efficient use of available resources.
However, both parallel and distributed systems come with their own set of challenges. Synchronization, or ensuring that all parts of the system work together correctly, is one of the most significant challenges in parallel computing. Without proper synchronization, issues such as deadlocks—where two or more processes wait indefinitely for each other—or race conditions—where the outcome of a computation depends on the unpredictable timing of events—can occur. These issues can lead to incorrect results or system failures, making it crucial to design parallel systems that handle synchronization effectively.
In distributed systems, the challenges are even more pronounced. Communication between nodes must be carefully managed to ensure that data is consistent and that tasks are coordinated correctly. This requires careful design of communication protocols and data management strategies, as well as mechanisms for fault tolerance to ensure that the system can continue to operate even if some nodes fail. In computational physics, where simulations often involve large amounts of data and complex computations, these challenges are particularly significant.
Rust’s concurrency model is particularly well-suited to parallel computing due to its strong memory safety guarantees and its ability to manage threads effectively. Rust’s ownership and borrowing system ensures that data is only accessed in ways that prevent data races, a common problem in parallel computing where two threads try to access the same memory simultaneously, leading to unpredictable results. This makes Rust an ideal language for developing parallel applications in computational physics, where correctness and performance are critical.
Rust’s concurrency model is built on a foundation of memory safety and strict ownership rules. These features prevent data races and ensure that shared data is accessed only in safe, controlled ways, which is particularly beneficial in parallel computing. For instance, Rust's standard library includes constructs such as threads, while higher-level libraries like Rayon simplify data-parallelism by converting sequential iterators into parallel ones. The following code snippet demonstrates basic parallelism using Rust’s standard library to spawn multiple threads:
use std::thread;
fn main() {
let mut handles = vec![];
for i in 0..10 {
// Spawn a new thread that takes ownership of its loop variable.
let handle = thread::spawn(move || {
println!("Thread {} is running", i);
});
handles.push(handle);
}
// Wait for all threads to complete.
for handle in handles {
handle.join().unwrap();
}
}
In this code, we create multiple threads using Rust's std::thread::spawn
function. Each thread is given ownership of its loop variable i
using the move
keyword, which ensures that each thread has its own data to work with, preventing data races. The thread::spawn
function spawns a new thread for each iteration of the loop, and the join
method is called on each thread handle to ensure that the main thread waits for all spawned threads to complete. This approach is an example of data parallelism, where each thread performs a similar task on different pieces of data, making it particularly useful in scenarios where tasks can be performed concurrently without dependencies on each other.
For more complex parallelism, such as dividing a large computation across multiple threads, Rust provides several concurrency primitives, such as channels for communication between threads and mutexes for shared data access. Channels in Rust allow for safe communication between threads, enabling tasks to pass messages and synchronize their work without directly sharing memory. Mutexes, on the other hand, provide a mechanism for safely accessing shared data by ensuring that only one thread can access the data at a time. These primitives make it possible to build complex parallel systems in Rust that are both safe and efficient.
While Rust's standard library provides robust support for parallel computing on a single machine, distributed computing requires more effort. In the absence of external crates like MPI, one way to implement distributed computing in Rust is to use standard networking protocols like TCP or UDP. This involves setting up socket connections between machines and manually handling the communication protocols needed to coordinate tasks across the distributed system. Although this approach is more complex, Rust’s strong guarantees around safety and concurrency provide a solid foundation for building reliable distributed systems.
Consider a simple example of a distributed system using TCP sockets in Rust:
use std::net::{TcpListener, TcpStream};
use std::io::{Read, Write};
use std::thread;
fn handle_client(mut stream: TcpStream) {
let mut buffer = [0; 512];
stream.read(&mut buffer).unwrap();
println!("Received: {:?}", String::from_utf8_lossy(&buffer[..]));
stream.write(b"Message received").unwrap();
}
fn main() {
let listener = TcpListener::bind("127.0.0.1:7878").unwrap();
println!("Server listening on port 7878");
for stream in listener.incoming() {
match stream {
Ok(stream) => {
// Spawn a thread to handle each client connection.
thread::spawn(|| {
handle_client(stream);
});
}
Err(e) => {
eprintln!("Connection failed: {}", e);
}
}
}
}
This TCP server listens for incoming connections on port 7878. For each connection, a new thread is spawned to handle the client, ensuring that the server can manage multiple client connections concurrently. Although this implementation is elementary compared to full-fledged distributed systems, it illustrates Rust’s ability to build reliable and safe distributed applications through careful design of communication protocols and data management.
This example highlights the flexibility and power of Rust’s concurrency model, which allows developers to build parallel and distributed systems that are both safe and efficient. By leveraging Rust’s strong guarantees around memory safety and concurrency, computational physicists can develop high-performance applications that can handle the demands of modern scientific computing.
In conclusion, parallel and distributed computing are essential techniques in computational physics, enabling the efficient processing of large-scale simulations and complex data analysis tasks. Rust’s concurrency model, with its strong emphasis on memory safety and efficient thread management, provides a robust foundation for implementing parallel computing. While distributed computing requires more effort, particularly in terms of managing communication between machines, Rust’s safety guarantees and powerful concurrency primitives make it an excellent choice for developing scalable, reliable systems. By understanding the fundamental concepts and practical applications of parallel and distributed computing in Rust, computational physicists can develop efficient, scalable solutions to the challenges of modern scientific computing.
10.2. Rust’s Concurrency Model
Rust’s concurrency model is one of its most defining features, offering a unique approach to achieving safe and efficient parallelism. At the heart of Rust’s concurrency model is its ownership system, which guarantees memory safety without the need for a garbage collector. This ownership paradigm manages memory through the intertwined concepts of ownership, borrowing, and lifetimes. These mechanisms are deeply integrated into Rust’s type system, providing compile-time guarantees that prevent many of the common bugs that plague concurrent programming—such as data races.
The ownership model’s key contribution to concurrency is its strict regulation of how data is accessed and modified. In Rust, each data value has a single owner at any given time; while ownership can be transferred, data cannot be shared arbitrarily. Borrowing offers temporary access to data either mutably or immutably, but the borrowing rules ensure that no data is modified while it is being accessed elsewhere. This rigorous control over data access effectively prevents race conditions that can occur when multiple threads attempt to read from and write to the same memory concurrently.
Rust’s standard library provides several essential concurrency primitives that are foundational for building concurrent applications. The simplest among these is the thread, which can be spawned using the std::thread::spawn
function. Each thread executes independently, enabling multiple segments of a program to run concurrently. In addition to threads, Rust supports message passing through channels, which allow threads to safely communicate. Rust’s channels, implemented via the multiple producer, single consumer (mpsc) model, enable many threads to send messages to a single recipient, thereby ensuring safe data exchange without shared mutable state. Locks, particularly the Mutex
type, facilitate safe sharing of data by allowing only one thread to access a piece of data at a time.
One of the most powerful aspects of Rust’s concurrency model is its ability to share data safely between threads. This is primarily achieved through two key types: Arc
(Atomic Reference Counting) and Mutex
. The Arc
type is a thread-safe reference-counting pointer that permits multiple threads to share ownership of data by performing atomic operations on its reference count. Unlike Rc
, which is not thread-safe, Arc
can be safely shared across threads. When mutable access to shared data is necessary, Arc
is typically combined with Mutex
. The Mutex
is a mutual exclusion primitive that ensures only one thread can modify the data at any one time. By wrapping data within an Arc
, it is possible to safely share and update the data concurrently, preventing data races and maintaining consistency.
To illustrate Rust’s approach to avoiding common concurrency pitfalls, consider that the Send trait designates types that can be transferred safely to other threads, while the Sync trait indicates that a type can be safely shared between threads. Rust’s type system automatically implements these traits for types that are safe to transmit or share across threads, and it prohibits those that could lead to unsafe concurrent behavior from being used in such contexts. This systematic enforcement ensures that only data types deemed safe for concurrent use can be shared across threads, thereby eliminating many concurrency-related bugs.
For example, consider a situation where multiple threads need to access and modify a shared counter. Without proper synchronization, simultaneous access could lead to a race condition, resulting in an incorrect final count. In Rust, this problem is addressed with the combination of Arc
and Mutex
:
use std::sync::{Arc, Mutex};
use std::thread;
fn main() {
let counter = Arc::new(Mutex::new(0));
let mut handles = vec![];
for _ in 0..10 {
let counter = Arc::clone(&counter);
let handle = thread::spawn(move || {
let mut num = counter.lock().unwrap();
*num += 1;
});
handles.push(handle);
}
for handle in handles {
handle.join().unwrap();
}
println!("Result: {}", *counter.lock().unwrap());
}
In this example, Arc::new(Mutex::new(0))
creates an atomic reference-counted mutex that wraps an integer initialized to 0. Each thread receives a clone of this Arc
using Arc::clone
, ensuring that all threads share ownership of the same counter. When a thread needs to modify the counter, it locks the mutex with counter.lock().unwrap()
, obtaining exclusive access via a MutexGuard
. This mechanism prevents race conditions by ensuring that only one thread modifies the counter at a time. Finally, after all threads have executed, the main thread locks the counter again to print the final value (expected to be 10).
Implementing parallel tasks in Rust often begins with the std::thread
module, which allows developers to spawn new threads easily. For instance, suppose we need to perform several independent computations concurrently; this can be done with the thread::spawn
function:
use std::thread;
fn main() {
let handle1 = thread::spawn(|| {
println!("Thread 1 is running");
});
let handle2 = thread::spawn(|| {
println!("Thread 2 is running");
});
handle1.join().unwrap();
handle2.join().unwrap();
}
In this code, two threads are spawned using thread::spawn
, each executing a closure that prints a message. The join
method ensures that the main thread waits for both threads to complete before exiting, demonstrating how Rust allows simple and effective parallel execution.
Another cornerstone of Rust’s concurrency model is message passing. The std::sync::mpsc
module offers channels that facilitate communication between threads without sharing memory directly, thus mitigating the risk of data races. A channel comprises a sender and a receiver; the sender can be used by one or more threads to transmit messages, and the receiver collects these messages in a synchronized manner. Consider the following example:
use std::sync::mpsc;
use std::thread;
use std::time::Duration;
fn main() {
let (tx, rx) = mpsc::channel();
let handle = thread::spawn(move || {
let val = String::from("hello");
tx.send(val).unwrap();
thread::sleep(Duration::from_secs(1));
});
let received = rx.recv().unwrap();
println!("Received: {}", received);
handle.join().unwrap();
}
Here, a channel is created with mpsc::channel()
, yielding a sender (tx
) and a receiver (rx
). A new thread receives the sender and sends a string message; meanwhile, the main thread waits for the message using rx.recv()
, which blocks until the message is available. Message passing is especially useful in scenarios where task coordination is required without the complexities of shared mutable state.
Managing shared state among multiple threads is often necessary in advanced applications, and Rust’s combination of Arc
and Mutex
offers a robust solution for this scenario. Arc
enables safe sharing of data by reference counting, and Mutex
ensures exclusive access during mutations, thereby preserving consistency across thread operations.
In conclusion, Rust’s concurrency model is meticulously designed to combine safety with high performance. Its ownership system, along with primitives such as threads, channels, and mutexes, enables developers to build concurrent applications that are both robust and efficient. By adhering to Rust’s strict rules—reinforced by the Send and Sync traits—developers can avoid pitfalls like data races and deadlocks. These features are especially valuable in computational physics and other domains where parallel and distributed computing are critical for tackling large-scale, complex problems.
10.3. Data Parallelism in Rust
Data parallelism is a fundamental concept in computational physics that enables the efficient processing of large datasets by applying the same operation simultaneously to many data points. This approach is especially beneficial in simulations where similar calculations must be performed on extensive arrays of data, such as in particle simulations, grid-based fluid dynamics, or image processing tasks. By leveraging data parallelism, computational physicists can achieve significant performance improvements, allowing them to tackle more complex problems within a reasonable time frame.
At the core of data parallelism lies the concept of SIMD (Single Instruction, Multiple Data), which allows a single instruction to operate on multiple data points concurrently. This technique is highly effective for operations involving large arrays or matrices, such as vector arithmetic, matrix multiplication, or Fourier transforms. Rust provides support for SIMD operations through its std::simd
module, granting developers low-level access to SIMD instructions that modern CPUs offer. With these vector types and operations, the number of executed instructions is reduced dramatically, leading to substantial performance gains in numerical computations.
Rust’s std::simd
module enables efficient, fine-grained parallelism by allowing operations to be performed on vectors of data where each SIMD operation handles several elements simultaneously. While fine-grained parallelism through SIMD is ideal for independent element-wise operations like arithmetic calculations, it is also important to balance this approach with coarse-grained parallelism. Coarse-grained parallelism divides a dataset into larger chunks, which are then processed in parallel using threads or distributed computing. Although coarse-grained approaches can handle more complex tasks, they often incur higher overhead due to synchronization and communication between threads. Thus, choosing the appropriate level of parallelism depends on the specific requirements of the problem being solved.
For instance, in a physics simulation where the forces on particles are computed based on their positions, SIMD can be used to perform these calculations simultaneously for multiple particles. This not only reduces overall computation time but also improves the scalability of the simulation as the number of particles increases. Implementing SIMD operations in Rust is straightforward using the std::simd
module. Consider the following example that demonstrates how to perform simple vector addition using SIMD:
use std::simd::{f32x4, Simd};
fn main() {
let a = f32x4::from_array([1.0, 2.0, 3.0, 4.0]);
let b = f32x4::from_array([5.0, 6.0, 7.0, 8.0]);
let c = a + b;
println!("{:?}", c.to_array());
}
In this example, the f32x4
type represents a SIMD vector containing four f32
values, and the overloaded +
operator performs element-wise addition. The result is an array where each element is the sum of corresponding elements in the input arrays, processed in parallel.
For higher-level data parallelism, Rust provides the Rayon crate, which allows developers to convert standard iterators into parallel iterators with minimal effort. This approach is ideal for processing large datasets where each data element or chunk can be processed independently. Consider the following example using Rayon to perform parallel iteration over a collection:
use rayon::prelude::*;
fn main() {
let numbers: Vec<i32> = (1..1000).collect();
let sum: i32 = numbers.par_iter().map(|&x| x * x).sum();
println!("Sum of squares: {}", sum);
}
Here, par_iter()
creates a parallel iterator that squares each element of the vector concurrently before summing the results. Rayon handles the details of thread management and synchronization automatically, ensuring efficient use of multiple CPU cores.
Performance benchmarking is essential to evaluate the benefits of data parallelism. Rust’s standard library, through the std::time
module, provides tools to measure execution times accurately. For instance, the following code snippet benchmarks a SIMD operation by timing a loop that performs vector addition repeatedly:
use std::simd::{f32x4, Simd};
use std::time::Instant;
fn main() {
let a = f32x4::from_array([1.0, 2.0, 3.0, 4.0]);
let b = f32x4::from_array([5.0, 6.0, 7.0, 8.0]);
let start = Instant::now();
for _ in 0..1_000_000 {
let _c = a + b;
}
let duration = start.elapsed();
println!("Time elapsed: {:?}", duration);
}
By comparing the execution time of SIMD-optimized code with equivalent sequential operations, developers can quantify the performance improvements achieved through data parallelism.
In summary, data parallelism is a powerful technique for accelerating computational physics applications, particularly when processing large datasets or performing numerically intensive operations. Rust’s support for low-level SIMD via the std::simd
module, along with high-level data parallelism through Rayon, equips developers with robust tools for building efficient parallel algorithms. By carefully selecting and benchmarking the appropriate level of parallelism—whether it be fine-grained SIMD or coarse-grained thread-based concurrency—developers can optimize code performance while ensuring correct and safe execution. This combination of techniques enables the practical application of data parallelism to solve complex problems in computational physics efficiently.
10.4. Task Parallelism in Rust
Task parallelism is a core concept in parallel computing, where different tasks or processes are executed concurrently to enhance efficiency and overall performance. Unlike data parallelism—where the same operation is performed on multiple data points simultaneously—task parallelism focuses on independently executing various tasks, which may be interdependent but can operate concurrently. In computational physics, task parallelism is particularly valuable when a simulation comprises multiple distinct processes such as computing physical forces, updating particle positions, managing boundary conditions, and handling I/O operations concurrently.
Effective task scheduling is critical to ensure that available CPU cores are utilized optimally, minimizing idle time and preventing bottlenecks. Load balancing is equally important; when different parts of a simulation have varying computational loads, the workload must be evenly distributed to avoid overburdening any single processing unit. Rust’s async programming model provides an excellent framework for task parallelism. Built upon futures, async/await syntax, and executors, this model allows tasks that often involve I/O or waiting for events to run concurrently without blocking the overall execution. Libraries such as Tokio and async-std supply runtime support and utilities for managing asynchronous tasks, including task spawning, handling I/O, and synchronizing work among multiple tasks.
For example, consider a physics simulation that requires simultaneously calculating particle positions, logging simulation results, and handling user input. Using the Tokio crate, one can structure these operations as independent asynchronous tasks that run concurrently. The following example demonstrates a straightforward implementation using Tokio:
use tokio::task;
#[tokio::main]
async fn main() {
// Spawn an async task for calculating positions.
let handle1 = task::spawn(async {
calculate_positions().await;
});
// Spawn an async task for logging results.
let handle2 = task::spawn(async {
log_results().await;
});
// Spawn an async task for handling user input.
let handle3 = task::spawn(async {
handle_user_input().await;
});
// Await the completion of all tasks concurrently.
handle1.await.unwrap();
handle2.await.unwrap();
handle3.await.unwrap();
}
async fn calculate_positions() {
println!("Calculating positions...");
tokio::time::sleep(tokio::time::Duration::from_secs(2)).await;
}
async fn log_results() {
println!("Logging results...");
tokio::time::sleep(tokio::time::Duration::from_secs(1)).await;
}
async fn handle_user_input() {
println!("Handling user input...");
tokio::time::sleep(tokio::time::Duration::from_secs(3)).await;
}
In this example, three asynchronous tasks are created using tokio::task::spawn
, with each task representing a separate part of the simulation. The #[tokio::main]
macro sets up an asynchronous runtime, allowing the program to await the completion of all tasks concurrently. The tokio::time::sleep
function simulates long-running operations, which might be computational tasks or I/O-bound operations in a real simulation.
For more complex simulations, tasks can be further decomposed to distribute computational load evenly. For instance, in a multi-threaded particle simulation, force calculations for different particle subsets may be performed concurrently. By breaking down large computations into smaller tasks and scheduling them asynchronously, the simulation can scale more effectively across multiple cores. This method not only improves performance by reducing overall execution time but also ensures that tasks do not block each other, leading to smoother and more responsive simulations.
Ultimately, task parallelism in Rust leverages asynchronous programming to manage independent computational tasks concurrently. The language’s powerful concurrency model—incorporating async/await, channels, and synchronization primitives—ensures that tasks are executed safely and efficiently without data races or deadlocks. This makes Rust an ideal choice for developing high-performance, scalable simulations in computational physics, where managing multiple simultaneous processes is essential.
10.5. Distributed Computing with Rust
Distributed computing is a powerful paradigm that enables the processing of large-scale computations across multiple machines. This approach is particularly advantageous in computational physics, where simulations often demand extensive computational resources that exceed what can be handled by a single machine. At its core, distributed computing involves splitting a large problem into smaller tasks that are processed concurrently on multiple nodes. These nodes communicate with one another via a network to exchange data and synchronize processes, ultimately assembling the final result from the contributions of each node.
The fundamental challenges in distributed computing include managing network communication, ensuring distributed memory consistency, and synchronizing processes to produce a coherent outcome. In a distributed system, multiple machines (or nodes) work collaboratively, with each node handling part of the computation. This cooperation requires efficient communication protocols and strategies for fault tolerance, so that the system can continue operating even if some nodes fail. For instance, different architectures in distributed computing include client-server, peer-to-peer, and hybrid models, each with its advantages and trade-offs. The client-server model is simple and centralized but can become a bottleneck, while the peer-to-peer model distributes the workload more evenly, reducing potential congestion. Hybrid models seek to combine the benefits of both approaches for enhanced flexibility and scalability.
Rust offers a variety of networking libraries that facilitate the development of distributed systems. Libraries such as Tokio, Reqwest, and Hyper provide asynchronous, high-performance networking capabilities that are essential for building scalable networked applications. Tokio, in particular, serves as an asynchronous runtime that supports TCP and UDP socket programming, connection management, and asynchronous I/O operations. These tools, combined with Rust’s strong emphasis on safety and concurrency, allow developers to write distributed systems that are both robust and efficient.
Consider, for example, a scenario where multiple nodes collaborate to compute the sum of a large dataset. Each node processes a segment of the data and then sends its partial result back to a central server, which aggregates these results to form the final sum. The following code demonstrates one approach to implementing this using Tokio:
use tokio::net::TcpListener;
use tokio::io::{AsyncReadExt, AsyncWriteExt};
use tokio::sync::Mutex; // Use tokio's Mutex for async compatibility
use std::sync::Arc;
#[tokio::main]
async fn main() {
let listener = TcpListener::bind("127.0.0.1:8080").await.unwrap();
let total_sum = Arc::new(Mutex::new(0)); // Use tokio::sync::Mutex
loop {
let (mut socket, _) = listener.accept().await.unwrap();
let total_sum = Arc::clone(&total_sum);
tokio::spawn(async move {
let mut buf = [0; 1024];
let n = match socket.read(&mut buf).await {
Ok(n) => n,
Err(_) => return,
};
let received_sum: i32 = match String::from_utf8_lossy(&buf[..n])
.trim()
.parse()
{
Ok(num) => num,
Err(_) => return,
};
let mut total = total_sum.lock().await; // Lock the async Mutex
*total += received_sum;
if let Err(_) = socket.write_all(b"Sum received").await {
return;
}
});
}
}
In this example, a TCP server listens on port 8080 for incoming connections. When a node sends a partial sum, the server reads the data, parses it, and adds it to a shared total_sum
variable protected by a Mutex
for thread safety. After processing, the server sends an acknowledgement to the client. Each node could run a similar client program that connects to the server, transmits its computed partial sum, and receives confirmation.
Serialization and deserialization are critical for distributed computing, as data must often be transmitted between nodes in a format that preserves its structure. Rust’s serde
crate is a popular, versatile serialization framework that supports multiple formats such as JSON, BSON, and MessagePack. Using serde
, complex data structures can be easily serialized for transmission over a network and then deserialized on the receiving end. For example, the following code shows how to serialize a custom data structure to JSON and send it over a TCP connection:
use serde::{Serialize, Deserialize};
use serde_json;
use tokio::net::TcpStream;
use tokio::io::AsyncWriteExt;
#[derive(Serialize, Deserialize, Debug)]
struct DataChunk {
values: Vec<i32>,
sum: i32,
}
#[tokio::main]
async fn main() {
let data = DataChunk {
values: vec![1, 2, 3, 4, 5],
sum: 15,
};
let serialized_data = serde_json::to_string(&data).unwrap();
let mut stream = TcpStream::connect("127.0.0.1:8080").await.unwrap();
stream.write_all(serialized_data.as_bytes()).await.unwrap();
}
In this snippet, a DataChunk
struct is defined with the Serialize
and Deserialize
traits enabled via serde
. The struct is serialized into a JSON string using serde_json::to_string
, and then transmitted over a TCP connection. On the receiving end, the data can be deserialized back into the original DataChunk
structure, preserving the integrity of the data throughout the communication process.
For high-performance distributed computing, Rust can also interface with MPI (Message Passing Interface) libraries through bindings such as rsmpi
. MPI is widely used in scientific computing for managing communication between nodes in distributed systems, particularly in large-scale simulations where complex communication patterns are necessary. The following example demonstrates a basic MPI usage in Rust:
use mpi::traits::*;
use mpi::topology::SystemCommunicator;
fn main() {
let universe = mpi::initialize().unwrap();
let world = universe.world();
let rank = world.rank();
let data = if rank == 0 { vec![1, 2, 3, 4] } else { vec![0, 0, 0, 0] };
if rank == 0 {
world.process_at_rank(1).send(&data[..]).unwrap();
} else if rank == 1 {
let (received_data, _status) = world.any_process().receive_vec::<i32>().unwrap();
println!("Rank 1 received data: {:?}", received_data);
}
}
In this example, the MPI environment is initialized, and each process’s rank is determined. The process with rank 0 sends a vector to the process with rank 1, which receives and prints the data. This basic demonstration outlines the core of distributed computing using MPI in Rust.
In conclusion, distributed computing with Rust equips developers with the necessary tools to build scalable and efficient systems capable of handling large-scale computational physics problems. By leveraging Rust’s asynchronous networking libraries, robust serialization mechanisms provided by serde
, and even MPI interfaces for high-performance scenarios, one can implement distributed algorithms that are both reliable and performant. Understanding and addressing the challenges of latency, data consistency, and fault tolerance are crucial, and Rust’s strong concurrency model and memory safety guarantees provide a solid foundation for doing so. These capabilities ultimately allow computational physicists to solve complex problems that demand massive computational power, distributed seamlessly across multiple machines.
10.6. Synchronization and Communication in Rust
Rust’s approach to synchronization and communication in both parallel and distributed computing is a cornerstone of its design, ensuring that multiple threads or processes work together in a coordinated and consistent manner. Synchronization is essential for controlling access to shared resources, preventing data races, and guaranteeing that operations occur in the intended order. In distributed systems, where processes span multiple machines, synchronization becomes even more challenging, as it must coordinate actions over networks and manage data consistency across different nodes. These mechanisms are vital to avoiding issues such as data corruption, deadlocks, and inconsistent system states.
Communication between processes in distributed systems is typically achieved through message passing, shared memory, or remote procedure calls (RPC). Message passing allows different parts of the system to exchange data safely without directly sharing memory, which helps mitigate the risk of data races. Shared memory, while efficient within a single machine, requires careful synchronization, and RPC provides an abstraction that permits remote function calls as if they were local.
Rust provides several synchronization primitives in its standard library, which are essential for constructing concurrent applications. One of the simplest primitives is the thread, which enables the spawning of new threads for independent tasks. Each thread runs its code concurrently, allowing multiple tasks to execute in parallel. In addition, Rust’s channels, built on the multiple producer, single consumer (mpsc) model, permit safe message-passing between threads. Locks—particularly the Mutex
type—ensure that only one thread can access a piece of data at a time, thereby preventing data races.
One of the most powerful aspects of Rust’s concurrency model is its facility for sharing data safely among threads using types such as Arc
(Atomic Reference Counting) and Mutex
. An Arc
is a thread-safe reference-counting pointer that allows multiple threads to share ownership of data, unlike the non-thread-safe Rc
. When mutable access is required, combining Arc
with Mutex
ensures that only one thread can modify the data at any given time, maintaining data consistency and preventing race conditions.
To illustrate how Rust’s synchronization constructs work, consider this example where multiple threads safely increment a shared counter using Arc
:
use std::sync::{Arc, Mutex};
use std::thread;
fn main() {
// Create an atomic reference-counted mutex to protect the shared counter.
let counter = Arc::new(Mutex::new(0));
let mut handles = vec![];
// Spawn 10 threads, each incrementing the counter.
for _ in 0..10 {
let counter = Arc::clone(&counter); // Clone Arc to share ownership.
let handle = thread::spawn(move || {
// Lock the mutex to get exclusive access to the counter.
let mut num = counter.lock().unwrap();
*num += 1; // Increment the counter.
});
handles.push(handle);
}
// Join all threads to ensure they complete before proceeding.
for handle in handles {
handle.join().unwrap();
}
// Print the final counter value.
println!("Result: {}", *counter.lock().unwrap());
}
In this example, Arc
enables safe shared ownership of the counter among threads, while Mutex
ensures that only one thread can modify the counter at a time. Each thread locks the counter, increments it, and then releases the lock. Once all threads have finished, the main thread locks the counter again to print the final value.
Parallel task execution in Rust also frequently begins with the std::thread
module. For example, if multiple independent computations need to run concurrently, you can spawn new threads as shown below:
use std::thread;
fn main() {
// Spawn two threads that print messages concurrently.
let handle1 = thread::spawn(|| {
println!("Thread 1 is running");
});
let handle2 = thread::spawn(|| {
println!("Thread 2 is running");
});
// Wait for both threads to finish execution.
handle1.join().unwrap();
handle2.join().unwrap();
}
Here, thread::spawn
creates new threads executing closures that print messages. The join
method ensures that the main thread waits for these threads to complete, demonstrating effective parallel execution.
Message passing is another key mechanism; Rust’s mpsc (multiple producer, single consumer) module enables safe inter-thread communication through channels. For instance, the following code creates a channel, spawns a thread to send a message, and then receives the message in the main thread:
use std::sync::mpsc;
use std::thread;
use std::time::Duration;
fn main() {
// Create a channel returning a sender and a receiver.
let (tx, rx) = mpsc::channel();
// Spawn a new thread that sends a message after a short delay.
let handle = thread::spawn(move || {
let val = String::from("hello");
tx.send(val).unwrap(); // Send the message.
thread::sleep(Duration::from_secs(1)); // Simulate delay.
});
// Block until a message is received.
let received = rx.recv().unwrap();
println!("Received: {}", received);
handle.join().unwrap();
}
In this code, the sender (tx
) transfers a string to the receiver (rx
) in a separate thread. The main thread waits to receive the message using rx.recv()
, ensuring that synchronization is maintained without the need for direct shared memory, thus reducing race conditions.
Rust’s model also supports building higher-level distributed systems. Although shared memory is typically restricted to a single machine, distributed systems often use message passing over a network. Rust’s networking libraries, such as those provided by Mio or Tokio, along with frameworks for RPC, enable developers to build robust distributed applications. For example, a simple TCP server can be implemented to handle connections concurrently:
use tokio::net::TcpListener;
use tokio::io::{AsyncReadExt, AsyncWriteExt};
#[tokio::main]
async fn main() {
// Bind the TCP listener to a local address.
let listener = TcpListener::bind("127.0.0.1:8080").await.unwrap();
println!("Server listening on port 8080");
loop {
// Accept incoming connections.
let (mut socket, _) = listener.accept().await.unwrap();
// Spawn a new asynchronous task to handle each connection.
tokio::spawn(async move {
let mut buf = [0; 1024];
// Read data from the socket.
let n = socket.read(&mut buf).await.unwrap();
println!("Received: {:?}", String::from_utf8_lossy(&buf[..n]));
// Write a response back to the socket.
socket.write_all(b"Message received").await.unwrap();
});
}
}
This asynchronous TCP server example, built using Tokio, listens for connections on port 8080. Each connection is handled in its own task spawned with tokio::spawn
, which allows the server to manage multiple clients concurrently without blocking.
In conclusion, Rust’s concurrency model is designed from the ground up to provide both safety and efficiency. The integration of the ownership system with synchronization primitives like Mutex, RwLock, and channels ensures that developers can safely share and coordinate data between threads. The automatic enforcement of Send
and Sync
traits guarantees that only types safe for concurrent use are shared across threads, thereby preventing common concurrency pitfalls. Whether you are building a parallel application on a single machine or a distributed system spanning multiple nodes, Rust’s robust concurrency model offers the tools necessary to develop reliable and high-performance systems—an essential capability in computational physics and beyond.
10.7. Performance Optimization with Rust
Performance optimization is a critical aspect of developing efficient parallel and distributed systems, especially in computational physics, where simulations can be both time-consuming and resource-intensive. Bottlenecks may arise from inefficient synchronization, suboptimal memory access patterns, network latency, or contention for shared resources. Identifying and addressing these issues is essential for achieving high performance, scalability, and efficiency in such applications.
Profiling and benchmarking are fundamental tools in the optimization process. Profiling helps to analyze the runtime behavior of an application and identify hotspots—sections of code that consume an excessive amount of time or resources. Benchmarking, on the other hand, involves measuring the performance of an application under various conditions to assess the impact of optimizations. Together, these techniques provide the insight necessary to focus optimization efforts and ensure that modifications lead to tangible improvements.
When optimizing parallel code, it is crucial to minimize synchronization overhead. Excessive locking or frequent synchronization can lead to significant performance degradation. Techniques such as employing lock-free data structures, fine-grained locking, and reducing synchronization frequency can mitigate these issues. Furthermore, optimizing memory access patterns is essential—ensuring data locality, effective use of caches, and minimizing cache contention can lead to significant improvements in overall performance.
In distributed systems, network communication is a key performance factor. Network latency, bandwidth limitations, and communication protocol overhead can all impact efficiency. Techniques like data compression, batching of messages, and caching can help reduce the communication overhead and improve throughput. Moreover, understanding the trade-offs between performance, scalability, and fault tolerance is essential; for example, optimizing for maximum performance may sometimes come at the expense of reduced fault tolerance, whereas prioritizing scalability might increase communication overhead.
Rust provides powerful tools for benchmarking and profiling parallel and distributed code. The Criterion crate is a popular benchmarking tool that offers precise performance metrics, including execution times and variance, making it easier to pinpoint and address bottlenecks. Consider the following example, which uses Criterion to benchmark a simple parallel computation involving a shared counter:
use criterion::{criterion_group, criterion_main, Criterion};
use std::sync::{Arc, Mutex};
use std::thread;
/// A simple parallel computation that increments a shared counter using multiple threads.
fn parallel_computation() {
// Create an atomic reference-counted Mutex wrapping an integer counter.
let counter = Arc::new(Mutex::new(0));
let mut handles = vec![];
// Spawn 10 threads, each incrementing the counter.
for _ in 0..10 {
let counter = Arc::clone(&counter);
let handle = thread::spawn(move || {
let mut num = counter.lock().unwrap(); // Lock the counter for safe access.
*num += 1;
});
handles.push(handle);
}
// Wait for all threads to finish.
for handle in handles {
handle.join().unwrap();
}
}
fn criterion_benchmark(c: &mut Criterion) {
// Benchmark the parallel_computation function.
c.bench_function("parallel_computation", |b| b.iter(|| parallel_computation()));
}
criterion_group!(benches, criterion_benchmark);
criterion_main!(benches);
In this example, the criterion_benchmark
function defines a benchmark for the parallel_computation
function. The macros criterion_group!
and criterion_main!
generate the required scaffolding to run the benchmark, which measures the time taken by the parallel computation. This information is invaluable in guiding optimization efforts.
Profiling tools such as perf or Flamegraph further aid in performance optimization. Perf, a powerful Linux profiler, can analyze various performance metrics—such as CPU usage and cache misses—while Flamegraph visually represents where time is being spent in the code. For example, you can compile your application in release mode, run it with perf to capture performance data, and then generate a flame graph as follows:
cargo build --release
perf record -g ./target/release/your_application
perf script | ./flamegraph.pl > flamegraph.svg
These commands compile the Rust application with optimizations, profile it to capture a call graph, and then create a flame graph that visually highlights the performance hotspots, enabling targeted optimization.
Optimizing network communication in distributed systems is another key focus for performance improvements. For example, compressing data before transmission reduces the amount of data sent over the network, thereby reducing latency and improving throughput. Consider the following example that uses the flate2 crate to compress data before sending it over a TCP connection:
use flate2::write::GzEncoder;
use flate2::Compression;
use std::io::prelude::*;
use std::net::TcpStream;
fn main() {
let data = "This is some data that needs to be compressed before sending over the network.";
// Connect to a server at 127.0.0.1:8080.
let stream = TcpStream::connect("127.0.0.1:8080").unwrap();
// Create a GzEncoder to compress data with default compression.
let mut encoder = GzEncoder::new(stream, Compression::default());
// Write the data into the encoder.
encoder.write_all(data.as_bytes()).unwrap();
// Finish the compression and flush the data to the network.
encoder.finish().unwrap();
}
In this snippet, the flate2 crate compresses a text string before it is transmitted over a TCP socket. Compression reduces the data size, which is especially beneficial in distributed systems where bandwidth is at a premium.
In conclusion, performance optimization in parallel and distributed computing is a multi-faceted challenge that requires attention to synchronization overhead, memory access patterns, and network communication. Rust’s powerful profiling, benchmarking, and optimization tools—coupled with its strong type system and concurrency model—provide a robust framework for identifying and eliminating performance bottlenecks. By carefully balancing these aspects and iteratively refining the application, developers can build scalable, high-performance distributed systems capable of meeting the demanding requirements of computational physics simulations and beyond.
10.8. Case Studies and Applications
Parallel and distributed computing are indispensable in computational physics, enabling researchers to solve complex, large-scale problems that would be infeasible on a single machine. These techniques are applied in diverse real-world scenarios—from simulating particle interactions in N-body systems and solving partial differential equations via parallel finite element methods to running distributed Monte Carlo simulations. Each application highlights the power and versatility of these approaches to achieve significant performance improvements and more accurate, detailed simulations.
To better understand how parallel and distributed computing are applied in Rust, one can examine case studies that illustrate the practical implementation of these techniques. Such case studies provide valuable insights into the challenges encountered during development, the performance gains realized, and the lessons learned from building large-scale, complex systems.
One case study involves implementing an N-body simulation, where the gravitational forces between a large number of particles are calculated. This problem is computationally intensive due to the $O(N^2)$ complexity inherent in calculating pairwise interactions. By parallelizing these computations, significant performance gains can be realized, which in turn enable simulations of larger systems or more detailed models.
Another example is the parallel finite element method (FEM), widely used in physics and engineering to solve partial differential equations. In parallel FEM, the computational domain is partitioned into smaller subdomains that can be solved concurrently on different processors. This not only accelerates computation but also allows for more complex and detailed models to be simulated efficiently.
Distributed Monte Carlo simulations provide yet another application, as these methods rely on repeated random sampling to estimate system properties—such as those encountered in quantum systems or statistical mechanics. Distributing the computation across multiple machines enables a vastly larger number of samples to be generated in a shorter time, thereby enhancing the accuracy of the results.
These case studies illustrate both the practical challenges and the performance enhancements associated with parallel and distributed computing in Rust. Challenges include managing synchronization across threads, optimizing memory access patterns, and addressing network latency in distributed systems. However, by leveraging Rust’s robust concurrency model, its memory safety guarantees, and powerful libraries, developers can overcome these obstacles and achieve substantial performance gains.
To illustrate these concepts in practice, consider the following detailed example of an N-body simulation implemented in Rust. In this simulation, the gravitational forces between particles are computed in parallel using the Rayon crate, which provides data parallelism via parallel iterators. The particles are stored in a vector, and their forces are calculated based on the positions of all other particles; subsequently, the velocities of the particles are updated accordingly.
use rayon::prelude::*;
use std::sync::{Arc, Mutex};
#[derive(Clone)]
struct Particle {
position: [f64; 3],
velocity: [f64; 3],
mass: f64,
}
/// Parallel computation of gravitational forces between particles.
/// Each particle's force is computed by considering the interactions with every other particle.
fn calculate_forces(particles: &mut [Particle]) {
// Wrap the particle slice in Arc and Mutex for safe concurrent access.
let particles_arc = Arc::new(Mutex::new(particles));
// Use parallel iteration to compute forces for each particle independently.
particles_arc.lock().unwrap().par_iter_mut().for_each(|p1| {
let mut force = [0.0; 3];
// Compute interaction forces with all other particles.
for p2 in particles_arc.lock().unwrap().iter() {
// Ensure p1 and p2 are not the same particle.
if p1 as *const _ != p2 as *const _ {
let direction = [
p2.position[0] - p1.position[0],
p2.position[1] - p1.position[1],
p2.position[2] - p1.position[2],
];
let distance = (direction[0].powi(2) + direction[1].powi(2) + direction[2].powi(2)).sqrt();
let force_magnitude = (p1.mass * p2.mass) / (distance * distance);
// Accumulate the contribution of the force from p2.
force[0] += force_magnitude * direction[0] / distance;
force[1] += force_magnitude * direction[1] / distance;
force[2] += force_magnitude * direction[2] / distance;
}
}
// Update the particle's velocity based on the computed force.
p1.velocity[0] += force[0] / p1.mass;
p1.velocity[1] += force[1] / p1.mass;
p1.velocity[2] += force[2] / p1.mass;
});
}
fn main() {
// Initialize a vector of particles.
let mut particles = vec![
Particle { position: [0.0, 0.0, 0.0], velocity: [0.0, 0.0, 0.0], mass: 1.0 },
Particle { position: [1.0, 0.0, 0.0], velocity: [0.0, 1.0, 0.0], mass: 1.0 },
// Add more particles as needed.
];
// Parallel computation of forces among the particles.
calculate_forces(&mut particles);
}
In this example, the calculate_forces
function is parallelized using Rayon’s par_iter_mut
method, which distributes the computation across multiple threads. Each particle’s gravitational force is computed based on its interactions with every other particle, and the velocities are then updated accordingly. By parallelizing this process, the simulation can handle more particles in less time, resulting in significant performance improvements.
Benchmarking these implementations can provide valuable comparisons with similar implementations in languages like C++ (using OpenMP) or Python (with NumPy or Dask). For instance, Rust’s criterion crate can be used for detailed performance measurements, highlighting Rust’s efficiency, memory safety, and ease of parallelization due to its robust ownership model and concurrency primitives.
Implementing such complex systems in Rust imparts valuable lessons on performance optimization, concurrency management, and the trade-offs inherent in parallel and distributed computing. Key insights include the importance of balancing workloads among threads to reduce contention and ensuring that memory access patterns are optimized to minimize cache misses.
In conclusion, practical case studies in parallel and distributed computing are indispensable for understanding how to build efficient, scalable, and robust systems in computational physics using Rust. By comparing implementations, analyzing performance gains, and reflecting on challenges—such as synchronization, memory access, and network latency—developers can harness Rust’s powerful features to solve complex problems that require the extensive computational resources provided by parallel and distributed architectures. This combination of Rust’s concurrency model, memory safety guarantees, and performance-oriented design makes it an excellent choice for tackling the demanding challenges of modern computational physics simulations.
10.9. Future Trends and Research Directions
Parallel and distributed computing are rapidly evolving fields with significant implications for computational physics. As the demand for more complex simulations and higher computational power grows, new trends are emerging that promise to reshape how computational tasks are performed. These trends include advancements in hardware, such as quantum computing; the rise of cloud and edge computing; and the development of more sophisticated algorithms that can efficiently harness these new computing paradigms. The impact of these trends on computational physics is profound, as they open up new possibilities for solving previously intractable problems and achieving unprecedented levels of performance and scalability.
Rust, as a systems programming language, is well-positioned to benefit from and contribute to these emerging trends in parallel and distributed computing. The Rust community and development team are continuously enhancing the language’s capabilities, particularly in areas relevant to parallelism and distributed computing. Several upcoming Rust features and ongoing research efforts are poised to make significant contributions to the field.
One key area of focus is the further refinement of Rust’s concurrency model. As parallel computing becomes more complex, the need for advanced synchronization mechanisms, better memory management, and more efficient task scheduling becomes critical. Upcoming features like improvements to async I/O, enhancements to the ownership model, and more robust support for lock-free data structures are likely to make Rust an even more powerful tool for parallel and distributed computing.
In the realm of distributed computing, the integration of Rust with cloud computing platforms is a growing area of interest. As cloud computing becomes the backbone of large-scale simulations and data processing tasks, Rust’s memory safety guarantees and performance advantages make it an attractive option for cloud-native applications. Furthermore, the rise of edge computing, where computational tasks are performed closer to the data source, presents new opportunities for Rust. The language’s lightweight and efficient nature make it ideal for developing applications that run on resource-constrained edge devices.
Quantum computing is another exciting area where Rust could play a significant role. Although still in its infancy, quantum computing promises to revolutionize fields like cryptography, optimization, and complex simulations. Rust’s emphasis on safety and performance could make it a strong candidate for developing quantum algorithms and interfacing with quantum hardware. As quantum computing becomes more mainstream, there may be opportunities to integrate Rust with quantum computing platforms, enabling the development of hybrid classical-quantum systems.
Current research in these areas is exploring how Rust can be leveraged to meet the demands of these new computing paradigms. Researchers are investigating ways to optimize Rust for distributed systems, including improvements to networking libraries, better support for asynchronous operations, and integration with emerging technologies like blockchain and decentralized systems. These efforts are not only advancing Rust’s capabilities but also contributing to the broader field of computational physics by providing more powerful and reliable tools for simulation and data processing.
The practical implications of these emerging trends for Rust developers are significant. As the Rust ecosystem continues to evolve, developers have the opportunity to explore new applications of Rust in cutting-edge computing environments. For example, Rust’s ongoing integration with cloud computing platforms like AWS and Azure allows developers to build scalable, cloud-native applications that can handle the massive computational demands of modern physics simulations.
Consider a scenario where a Rust-based application is deployed on a cloud platform to perform distributed Monte Carlo simulations. By leveraging cloud resources, the application can scale horizontally, adding more computing nodes as needed to handle larger simulations. Here’s a simplified example of how Rust could be used to manage cloud-based distributed computing:
use std::sync::Arc;
use tokio::net::TcpListener;
use tokio::io::{AsyncReadExt, AsyncWriteExt};
#[tokio::main]
async fn main() {
// Bind the TCP listener to a global address.
let listener = TcpListener::bind("0.0.0.0:8080").await.unwrap();
// Shared data across tasks using Arc for thread-safe reference counting.
let shared_data = Arc::new(42);
loop {
// Accept incoming connections.
let (mut socket, _) = listener.accept().await.unwrap();
let data = Arc::clone(&shared_data);
// Spawn a new asynchronous task to handle each connection.
tokio::spawn(async move {
let mut buf = [0; 1024];
// Read data from the socket.
let n = socket.read(&mut buf).await.unwrap();
let request = String::from_utf8_lossy(&buf[..n]);
// Create a response including the shared data and the received request.
let response = format!("Data: {}, Received: {}", *data, request);
// Write the response back to the socket.
socket.write_all(response.as_bytes()).await.unwrap();
});
}
}
In this example, a simple TCP server is implemented using Rust’s Tokio runtime. The server listens for incoming connections on port 8080. Each connection is handled asynchronously using tokio::spawn
, allowing the server to manage multiple clients concurrently without blocking. The shared data is safely accessed across tasks using Arc
, ensuring thread-safe reference counting. This setup could be part of a larger cloud-based distributed system where nodes communicate and share data to perform complex simulations efficiently.
As Rust continues to evolve, the ecosystem is expected to expand with new libraries, tools, and frameworks designed to support large-scale distributed computing projects. Enhanced support for distributed databases, better integration with container orchestration systems like Kubernetes, and the development of specialized libraries for quantum computing are all areas where Rust is likely to see significant growth. These advancements will further cement Rust’s role as a go-to language for high-performance, parallel, and distributed computing in computational physics and beyond.
Looking ahead, Rust has the potential to become a key player in the next generation of computing paradigms. As researchers and developers push the boundaries of what’s possible with Rust, the language’s ecosystem will likely evolve to meet the demands of emerging technologies. This includes exploring how Rust can be used in hybrid computing environments that combine classical, quantum, and edge computing, as well as investigating new approaches to scalability, fault tolerance, and performance optimization.
In conclusion, the future of parallel and distributed computing in Rust is bright, with many exciting developments on the horizon. By staying informed about emerging trends, exploring new features, and experimenting with cutting-edge applications, developers can position themselves at the forefront of this rapidly evolving field. Whether it’s through cloud computing, quantum computing, or the continued advancement of distributed systems, Rust is well-equipped to meet the challenges of the future and continue its trajectory as a leading language for computational physics and beyond.
10.10. Conclusion
This chapter offers a comprehensive introduction to parallel and distributed computing using Rust, structured to cover the fundamentals, conceptual understanding, and practical implementation. Each section is designed to equip readers with the knowledge and tools necessary to leverage Rust’s unique features in tackling complex computational physics problems. The chapter aims to demonstrate Rust’s effectiveness in building robust, efficient, and scalable parallel and distributed systems, making it an invaluable resource for both learners and practitioners in the field.
10.10.1. Further Learning with GenAI
The following prompts cover fundamental concepts, practical implementations, and advanced techniques related to concurrency, parallelism, and distributed systems.
Provide an in-depth analysis of the distinctions between parallelism and concurrency in Rust, particularly within computational physics simulations. How do Rust’s ownership and borrowing systems facilitate safe and efficient parallel computations compared to other languages? Include advanced examples illustrating both parallelism and concurrency in practice, focusing on their impact on computational performance and correctness.
Examine the fundamental differences between data parallelism and task parallelism in Rust, focusing on large-scale physics simulations. Provide detailed use cases where each technique excels, and discuss how Rust’s concurrency primitives like threads, channels, and async/await can be optimized to maximize performance. Include insights into performance tuning and avoiding common bottlenecks.
Explore the role of the Send and Sync traits in Rust, explaining how they enforce memory safety in multi-threaded environments. Provide advanced examples that highlight potential pitfalls when using these traits improperly, along with best practices for mitigating memory safety issues in high-performance parallel applications. Analyze the impact of these traits on memory access patterns in large simulations.
Investigate the Arc
> pattern for managing shared state in concurrent Rust programs. Discuss the dangers of potential deadlock scenarios, methods for detecting them in complex systems, and strategies to avoid them in high-performance parallel computing applications. Provide detailed examples of deadlock-free implementations in physics simulations, focusing on trade-offs between safety and performance. Delve into the implementation of SIMD (Single Instruction, Multiple Data) operations in Rust using the std::simd library. How can SIMD be effectively utilized to accelerate computational physics algorithms that involve heavy vector or matrix calculations? Provide an in-depth example with performance benchmarks comparing SIMD-optimized code to traditional loops, analyzing the performance gains.
Analyze the capabilities and limitations of the rayon crate for enabling data parallelism in Rust. Provide a comprehensive case study of a complex physics simulation where rayon is used to parallelize data processing tasks, and evaluate the performance improvements achieved. Discuss techniques for tuning rayon-based parallelism to achieve maximum efficiency.
Explore task scheduling in Rust’s async programming model and examine how Rust ensures efficient task execution and load balancing in asynchronous, parallel systems. Provide a detailed example of how async/await can be leveraged in computationally intensive physics applications, explaining the nuances of task management and performance optimization.
Compare and contrast the use of the tokio and async-std crates for implementing task parallelism in Rust. Provide a deep analysis of their respective strengths and weaknesses, particularly in the context of high-performance, physics-based simulations that involve both I/O-bound and CPU-bound tasks. Offer insights into selecting the right tool for specific use cases.
Perform a deep dive into message passing versus shared memory models in distributed computing. How does Rust’s support for these models compare with languages like C++ or Go? Provide an advanced example of implementing a distributed physics simulation using both models, analyzing trade-offs in terms of performance, complexity, and fault tolerance.
Investigate the use of the serde crate in Rust for efficient data serialization and deserialization in distributed systems. Provide a comprehensive example of a distributed Rust application where serde handles large, complex datasets across networked nodes. Discuss the impact of serialization performance on overall system efficiency and reliability.
Design and implement a distributed Monte Carlo simulation in Rust, addressing challenges such as managing distributed state, optimizing network communication, and ensuring fault tolerance. Include performance benchmarks and compare them to centralized implementations, analyzing the trade-offs between distributed and centralized approaches.
Examine Rust’s synchronization primitives (Mutex, RwLock, Condvar) in detail. How do these tools ensure data consistency and prevent race conditions in concurrent and parallel Rust programs? Provide examples of advanced use cases in physics simulations where synchronization is critical, discussing the impact of proper synchronization on performance and correctness.
Discuss the challenges of optimizing parallel Rust code, particularly in reducing synchronization overhead and improving memory access patterns. How can Rust’s borrow checker and lifetime annotations be leveraged to optimize performance in high-throughput computing environments? Provide advanced strategies for minimizing contention and improving parallel efficiency.
Explore the importance of profiling and benchmarking in parallel and distributed computing with Rust. Discuss the use of tools like criterion, perf, and flamegraph for identifying performance bottlenecks in Rust code. Provide a detailed example of profiling a large-scale physics simulation, including strategies for improving performance based on profiling results.
Provide an in-depth case study of implementing an N-body simulation in Rust, focusing on parallel computing techniques. Discuss the specific performance optimizations applied, the challenges faced, and how Rust’s concurrency model contributed to the overall efficiency of the simulation. Analyze how different parallelism techniques influenced the final performance outcomes.
Investigate the integration of Rust with MPI (Message Passing Interface) for implementing large-scale distributed simulations. How can Rust’s safety features be maintained while leveraging MPI’s capabilities? Provide a detailed implementation example of a distributed physics simulation using MPI in Rust, including performance comparisons and optimizations.
Explore the implementation of consensus algorithms like Raft or Paxos in Rust for ensuring consistency in distributed systems. Analyze the challenges of implementing these algorithms, particularly in handling network partitions, ensuring fault tolerance, and maintaining performance. Provide detailed examples of Rust-based implementations for distributed consensus.
Analyze potential future trends in parallel and distributed computing, focusing on how Rust is positioned to address emerging challenges. Discuss how evolving Rust features, such as async/await, WebAssembly, and low-level optimizations, might support next-generation computing paradigms like quantum computing, edge computing, or AI-driven systems.
Investigate the use of Rust’s async programming model for implementing scalable cloud computing solutions in computational physics. Discuss the benefits and challenges of using Rust for cloud-based distributed systems, particularly in managing large datasets, optimizing resource usage, and ensuring scalability in physics simulations.
Explore the design and implementation of real-time distributed physics engines in Rust. Discuss key considerations for achieving low latency, high reliability, and efficient resource management in such systems. Provide a comprehensive example showcasing how Rust’s concurrency and parallelism features can be applied to real-time distributed simulations.
Each exercise and prompt will deepen your understanding, sharpen your technical abilities, and empower you to solve intricate problems with precision and confidence. Embrace the process with curiosity and determination, knowing that your efforts will pave the way for groundbreaking achievements and advancements in the field. Let your passion for learning propel you forward, and take pride in the knowledge that you are pushing the boundaries of what is possible with Rust.
10.10.2. Assignments for Practice
Here are five in-depth self-exercises designed to help you practice parallel and distributed computing in Rust, using GenAI as a resource for guidance and feedback.
Exercise 10.1: Implementing and Comparing Concurrency Models
Objective: Understand and compare different concurrency models in Rust, including multi-threading and asynchronous programming.
Instructions:
Implementation: Write two Rust programs—one using
std::thread
for multi-threading and another usingasync
andawait
for asynchronous programming. Both programs should perform the same concurrent task, such as calculating a series of numbers in parallel.Comparison: Analyze the performance and code complexity of both implementations. Use GenAI to discuss the advantages and limitations of each concurrency model, including how Rust’s ownership model impacts their behavior.
Documentation: Document your findings, including code snippets, performance metrics, and any issues encountered. Ask GenAI for feedback on best practices and any improvements that could be made.
Exercise 10.2: Parallel Data Processing with Rayon
Objective: Implement and optimize parallel data processing using the Rayon library in Rust.
Instructions:
Implementation: Write a Rust program that uses Rayon to parallelize a data processing task, such as sorting a large dataset or performing parallel map-reduce operations.
Optimization: Experiment with different configurations and optimizations in Rayon, such as adjusting task granularity or using custom parallel iterators. Use GenAI to understand the impact of these configurations on performance.
Benchmarking: Measure the performance of your Rayon implementation and compare it with a sequential version of the task. Seek GenAI’s advice on interpreting the results and identifying potential bottlenecks.
Exercise 10.3: Building a Distributed System with Tokio
Objective: Develop a simple distributed system using the Tokio library for asynchronous programming and network communication.
Instructions:
Implementation: Create a Rust application using Tokio to implement a basic client-server model where the client sends requests to the server and receives responses. Include features such as handling multiple clients concurrently and managing network communication.
Error Handling: Implement robust error handling to manage potential issues like network failures or timeouts. Use GenAI to get insights into best practices for handling errors in distributed systems.
Documentation: Prepare a detailed report on your implementation, including code snippets, architecture diagrams, and any challenges faced. Ask GenAI for feedback on improving the system’s design and performance.
Exercise 10.4: Fault Tolerance Strategies in Distributed Systems
Objective: Explore and implement fault tolerance strategies for distributed systems in Rust.
Instructions:
Implementation: Modify your distributed system from Exercise 10.3 to include fault tolerance features such as replication, leader election, or checkpointing. Implement at least one fault tolerance strategy.
Testing: Simulate failures (e.g., by stopping server nodes or causing network partitions) and observe how your system handles these scenarios. Use GenAI to understand the effectiveness of your fault tolerance strategy and get suggestions for improvement.
Documentation: Document the fault tolerance features you’ve added, including code changes and testing results. Seek GenAI’s advice on enhancing fault tolerance and reliability.
Exercise 10.5: Analyzing Real-World Applications of Parallel Computing
Objective: Research and analyze a real-world application that leverages parallel computing with Rust.
Instructions:
Research: Identify a real-world project or case study that uses Rust for parallel computing. This could be from academic papers, industry reports, or open-source projects.
Analysis: Study the implementation details, design choices, and performance outcomes of the application. Use GenAI to help you understand the technical aspects and how Rust’s features are utilized in the project.
Reproduction: If feasible, reproduce a simplified version of the application or a similar task in Rust. Document your process and findings. Ask GenAI for feedback on your analysis and any further insights on the topic.
These exercises are designed to provide hands-on experience with parallel and distributed computing concepts using Rust, helping readers gain practical skills and a deeper understanding of these advanced topics. Engage with GenAI throughout the exercises to enhance your learning and refine your implementation techniques.