Chapter 3
Setting Up the Computational Environment
"Truth in science can be defined as the working hypothesis best suited to open the way to the next better one." — Konrad Lorenz
Chapter 3 of CPVR provides a comprehensive guide to setting up a robust computational environment tailored for implementing computational physics in Rust. It begins by introducing the principles of computational physics and the unique advantages of using Rust for scientific computing. The chapter covers essential steps like installing Rust, configuring the development environment, and utilizing Cargo for project management. It dives into Rust’s ecosystem for scientific computing, offering practical examples of integrating key libraries for solving physics problems. The chapter emphasizes best practices for writing efficient Rust code, testing, benchmarking, debugging, and profiling, ensuring that readers can produce reliable and high-performance computational applications. It concludes with guidance on using version control for collaboration and deploying Rust applications to share with the broader scientific community.
3.1. Introduction to Computational Physics in Rust
Computational physics is a branch of physics that uses computational methods and numerical algorithms to solve complex physical problems that are difficult or impossible to solve analytically. It plays a critical role in scientific research, enabling simulations of physical systems across various scales—from subatomic particles to cosmological models. By translating physical theories into computational models, researchers can explore the behavior of systems under different conditions, predict outcomes, and analyze large datasets generated from experiments or simulations. Computational physics is indispensable in fields like material science, fluid dynamics, quantum mechanics, and astrophysics, where direct experimentation is either impractical or impossible.
Rust offers several unique features that make it particularly well-suited for computational physics. One of the most significant is Rust’s strong emphasis on memory safety without sacrificing performance. In scientific computing, where large datasets and complex simulations are common, avoiding memory-related bugs such as buffer overflows, null pointer dereferencing, and data races is critical. Rust’s ownership model, which enforces strict rules about how memory is accessed and modified, ensures that these errors are caught at compile time, leading to more reliable and secure code.
Another essential feature of Rust is its concurrency model. Computational physics often involves parallel processing to handle large-scale simulations efficiently. Rust’s concurrency model, based on the ownership system, allows developers to write parallel code that is safe from data races and other concurrency issues. The Send
and Sync
traits in Rust ensure that data can be safely shared and accessed across multiple threads, enabling the development of high-performance, parallelized simulations.
Additionally, Rust’s ability to provide low-level control over system resources, similar to C and C++, allows developers to optimize computationally intensive tasks. However, unlike C/C++, Rust does this while providing safety guarantees that prevent common errors. This makes Rust an excellent choice for writing high-performance scientific applications that need both speed and reliability.
Rust’s features can be effectively leveraged to implement a variety of computational physics models and simulations. Consider, for example, a simple simulation of a particle’s motion under the influence of gravity using Newton’s laws of motion. In this basic model, we represent the particle with its position, velocity, and mass, and we use Euler integration to update these properties over discrete time steps. Although elementary, this simulation serves as a stepping stone toward more complex models, such as simulating planetary systems or particle interactions within a force field. The following implementation in Rust demonstrates how to set up such a simulation in a clean and robust manner.
Here’s a sample implementation in Rust:
#[derive(Debug)]
struct Particle {
position: [f64; 3],
velocity: [f64; 3],
mass: f64,
}
impl Particle {
// Creates a new particle with a given position, velocity, and mass
fn new(position: [f64; 3], velocity: [f64; 3], mass: f64) -> Self {
if mass <= 0.0 {
panic!("Mass must be greater than zero.");
}
Self { position, velocity, mass }
}
// Applies a force to the particle, updating its velocity and position
fn apply_force(&mut self, force: [f64; 3], dt: f64) {
let acceleration: [f64; 3] = [
force[0] / self.mass,
force[1] / self.mass,
force[2] / self.mass,
];
// Update velocity based on acceleration and time step
self.velocity.iter_mut().zip(&acceleration).for_each(|(v, a)| {
*v += a * dt;
});
// Update position based on velocity and time step
self.position.iter_mut().zip(&self.velocity).for_each(|(p, v)| {
*p += v * dt;
});
}
}
fn main() {
let mut particle = Particle::new([0.0, 0.0, 0.0], [0.0, 0.0, 0.0], 1.0);
let gravity = [0.0, -9.81, 0.0];
let time_step = 0.1;
let simulation_duration = 10.0;
let steps = (simulation_duration / time_step) as usize;
println!("Starting simulation...");
for step in 0..steps {
particle.apply_force(gravity, time_step);
println!(
"Step {}: Position = ({:.2}, {:.2}, {:.2}), Velocity = ({:.2}, {:.2}, {:.2})",
step + 1,
particle.position[0],
particle.position[1],
particle.position[2],
particle.velocity[0],
particle.velocity[1],
particle.velocity[2]
);
}
println!("Simulation complete.");
}
In this implementation, the Particle
struct encapsulates the essential physical properties of a particle—its three-dimensional position, velocity, and mass. The new
method initializes a particle with specified values, while the apply_force
method calculates the particle's acceleration using the formula $a=\frac{f}{m}$. This computed acceleration is then integrated over the time step dt
to update the particle's velocity and subsequently its position, following the simple Euler integration scheme. In the main
function, a particle is instantiated at the origin with no initial velocity, and a gravitational force vector that mimics the acceleration due to gravity is defined. The simulation loop iteratively applies this gravitational force over 100 time steps, printing the updated position at each iteration. This example not only illustrates fundamental principles of numerical integration in physics simulations but also demonstrates how Rust’s safety, clarity, and performance can be harnessed to model dynamic systems. Its robust type system and memory safety guarantees make it a strong candidate for developing more complex computational models in scientific computing.
This simple example can be extended to model more complex systems, such as simulating a system of interacting particles, incorporating different forces, or modeling motion in three dimensions. Rust’s type system and memory safety features ensure that these simulations can be implemented efficiently and without common errors, while its concurrency model can be leveraged to parallelize the computations across multiple threads for performance gains.
In summary, Rust provides a powerful and safe environment for implementing computational physics models. Its unique features like memory safety, concurrency, and low-level control make it ideal for handling the demands of scientific computing. By using Rust, researchers and developers can create robust simulations that are both performant and free from the common pitfalls of traditional languages used in computational physics.
3.2. Installing Rust and Setting Up the Development Environment
Installing Rust is the first step toward building computational physics models in this powerful language. Rust is known for its performance and safety, making it an ideal choice for scientific computing. The installation process begins with Rustup
, a toolchain manager that simplifies the management of Rust versions and related tools. Rustup
is the recommended way to install Rust because it not only installs the latest stable version of Rust but also makes it easy to switch between different versions and toolchains, such as stable, beta, and nightly builds. This flexibility is crucial in scientific computing, where experimenting with the latest features or maintaining compatibility with specific versions may be necessary.
To install Rust using Rustup
, simply open a terminal or command prompt and run the following command:
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
This command downloads and runs the Rustup
installer script. During the installation process, you will be prompted to choose installation options, such as whether to add the Rust binaries to your system’s PATH
. By default, Rustup installs Rust in a user-local directory ($HOME/.cargo
on Unix-like systems and %USERPROFILE%\.cargo
on Windows), making it easy to install without requiring administrative privileges.
Once Rust is installed, you can verify the installation by running:
rustc --version
This command should display the version of Rust that has been installed, confirming that your system is ready for Rust development.
Setting up a reliable and integrated development environment (IDE) is crucial for efficient Rust development, particularly in the context of computational physics. An IDE like Visual Studio Code (VS Code) offers several advantages, including syntax highlighting, code completion, integrated debugging, and version control. These features can significantly enhance productivity and help avoid common errors, especially when working on complex simulations and models.
Setting up a reliable and integrated development environment (IDE) is crucial for efficient Rust development, particularly in the context of computational physics. An IDE like Visual Studio Code (VS Code) offers several advantages, including syntax highlighting, code completion, integrated debugging, and version control. Additionally, tools like Cursor, a Rust-specific code exploration and navigation tool, can further enhance the developer experience by providing intelligent code navigation, GenAI code generator, efficient refactoring capabilities, and deep insights into code structure (eq. [https://www.cursor.com](https://www.cursor.com/)). These features can significantly boost productivity, helping developers avoid common errors, especially when working on complex simulations and models.
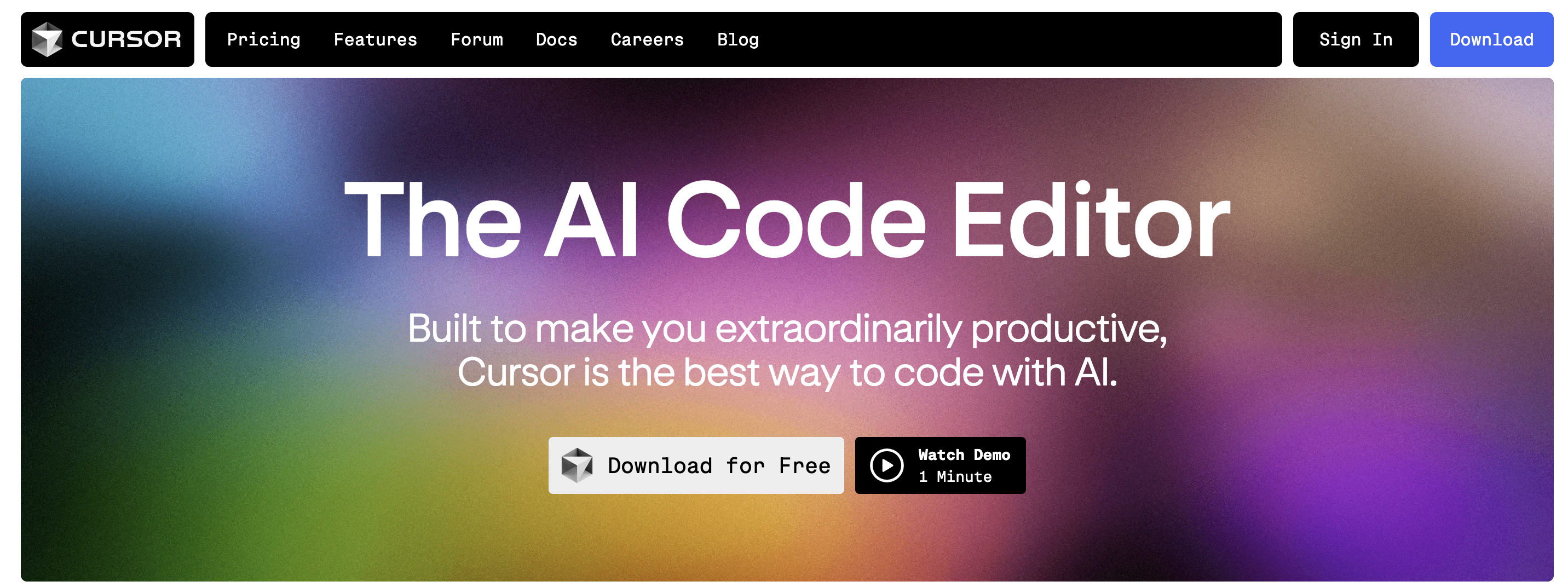
AI code editor with Cursor.
To set up VS Code for Rust development, you first need to install VS Code, which is available for Windows, macOS, and Linux. After installing VS Code, you can enhance its functionality for Rust development by installing the Rust-specific extensions. The most important extension is the "Rust Analyzer," which provides advanced language support, including real-time code analysis, error checking, and code completion.
To install Rust Analyzer in VS Code:
Open VS Code and navigate to the Extensions view by clicking on the Extensions icon in the Activity Bar on the side of the window or by pressing
Ctrl+Shift+X
.In the search box, type "Rust Analyzer" and select the extension from the list.
Click the "Install" button to add the extension to VS Code.
With Rust Analyzer installed, VS Code will automatically recognize Rust projects and provide a powerful development environment tailored to Rust. This setup is particularly beneficial for computational physics, where you might need to write, test, and debug complex Rust code that interfaces with scientific libraries and tools.
Setting up Rust on different operating systems is straightforward, thanks to Rustup’s cross-platform support. Below is a step-by-step guide for installing Rust on Windows, macOS, and Linux.
Installing Rust on Windows:
Download Rustup: Open a web browser and navigate to https://rustup.rs. Click on the "Install" button for Windows, which will download the installer.
Run the Installer: Execute the downloaded
.exe
file. Follow the prompts in the installation wizard. It will ask if you want to modify thePATH
environment variable to include Rust, which you should accept.Verify Installation: After the installation is complete, open the Command Prompt or PowerShell and run
rustc --version
to verify that Rust has been installed correctly.
Installing Rust on macOS:
Install Xcode Command Line Tools: Open a terminal and run:
xcode-select --install
This command installs the necessary tools for building Rust projects on macOS.
Install Rust with Rustup: In the terminal, run the following command to install Rust using Rustup:
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
Verify Installation: Once the installation is complete, verify it by running
rustc --version
in the terminal.
Installing Rust on Linux:
Install Required Dependencies: Depending on your Linux distribution, you might need to install some prerequisites, such as
build-essential
andcurl
. For example, on Ubuntu, you can run:
sudo apt-get update sudo apt-get install build-essential curl
Install Rust with Rustup: Run the Rustup installation command:
curl --proto '=https' --tlsv1.2 -sSf https://sh.rustup.rs | sh
Verify Installation: After installation, confirm that Rust is installed by running
rustc --version
in your terminal.
These steps provide a reliable way to set up Rust on any major operating system. Once Rust is installed, you can immediately start developing computational physics models and simulations using the robust features Rust offers. By setting up your development environment with tools like VS Code and Rust Analyzer, you ensure that your workflow is efficient, with access to powerful debugging, code completion, and real-time error detection—all of which are crucial when working with the complex and often computationally intensive code found in computational physics.
Through these installations and setup processes, Rust offers a seamless development experience across platforms, enabling scientists and engineers to focus on solving physical problems without worrying about the intricacies of managing development environments.
3.3. Understanding Cargo: Rust’s Package Manager
Cargo is the backbone of Rust’s project management and build system, streamlining the development process by automating tasks such as dependency management, compilation, testing, and documentation generation. As nearly every Rust project is managed through Cargo, it plays a pivotal role for both newcomers and seasoned developers alike. Cargo abstracts much of the complexity involved in compiling and linking code, allowing you to focus on writing and refining your computational models and simulations rather than wrestling with build intricacies. Whether you are crafting a simple script or developing a full-fledged application, Cargo ensures that your project remains organized and that all dependencies are appropriately handled, leading to smoother development cycles and more reliable software.
Cargo’s capabilities extend far beyond basic compilation; it is designed to manage dependencies, control the build process, and enforce code quality. When you create a new Rust project using Cargo, it automatically generates a well-structured directory with a manifest file (Cargo.toml) that contains crucial project metadata, configuration details, and dependency specifications. Dependencies in Rust are packaged as “crates,” and Cargo makes it exceptionally easy to add and manage these crates. For example, if your computational physics project requires advanced linear algebra operations provided by the nalgebra crate, you simply add a dependency entry to your Cargo.toml file, and Cargo takes care of downloading and integrating the library along with any necessary sub-dependencies.
Furthermore, Cargo meticulously manages the build process. It compiles only the parts of your project that have changed, thus reducing the overall build time and minimizing the risk of integration errors. Cargo also seamlessly supports testing frameworks, documentation generation, and code formatting tools such as cargo fmt and cargo clippy. These integrated tools help maintain a consistent codebase and catch common pitfalls early in the development lifecycle, ensuring high-quality, maintainable code.
To see Cargo in action, let’s walk through the process of creating a new Rust project, adding dependencies, and building a simple application. Begin by opening your terminal and creating a new project with the following command:
cargo new cpvr_project
This command creates a new directory named cpvr_project, organized as follows:
cpvr_project
├── Cargo.toml
└── src
└── main.rs
The Cargo.toml file serves as the project’s manifest, where you specify dependencies and various metadata. The src/main.rs file acts as the entry point of your application, containing the main function.
Next, let’s say you need the nalgebra crate for handling linear algebra operations in your computational physics project. To add this dependency, open the Cargo.toml file and include the following line under the [dependencies]
section:
[dependencies]
nalgebra = "0.29"
With this simple addition, Cargo will automatically download nalgebra and any of its dependent crates the next time you build your project. To compile your project, navigate to your project directory and run:
cargo build
Cargo will compile your project along with all its dependencies, producing an executable in the target/debug directory. For performance-critical applications or deployment, you can generate an optimized release build with the command:
cargo build --release
This builds an optimized executable in the target/release directory, ideally suited for intensive computations in scientific research.
To illustrate Cargo’s power further, let’s enhance our project by implementing a simple example of matrix-vector multiplication using the nalgebra crate. Modify your src/main.rs file to include the following code:
use nalgebra as na;
use na::{Matrix3, Vector3};
fn main() {
let matrix = Matrix3::new(
1.0, 2.0, 3.0,
4.0, 5.0, 6.0,
7.0, 8.0, 9.0
);
let vector = Vector3::new(1.0, 0.0, 0.0);
let result = matrix * vector;
println!("Matrix-vector multiplication result: {}", result);
}
In this example, nalgebra is used to create a 3x3 matrix and a 3D vector. The code then performs matrix-vector multiplication using the multiplication operator, which, thanks to Rust’s operator overloading, is both expressive and efficient. This demonstrates how easily external libraries can be integrated into your project, and how Cargo simplifies dependency management and the build process.
Finally, to run your project and see the output, simply execute:
cargo run
This command compiles the project (if there have been any changes) and runs the resulting executable, printing the result of the matrix-vector multiplication to your terminal.
Cargo’s integrated approach to project management in Rust not only makes setting up a new project straightforward but also ensures that your codebase remains organized, maintainable, and efficient. By automating dependency management, build orchestration, testing, and documentation, Cargo empowers developers to focus on the scientific problem at hand without getting bogged down by the complexities of the development process. This streamlined workflow is particularly beneficial in computational physics, where projects may involve intricate simulations, large-scale data processing, and the need for robust, high-performance code. Cargo stands as an indispensable tool in the Rust ecosystem, providing the foundation for building reliable and scalable scientific computing applications.
3.4. Exploring Rust’s Ecosystem for Scientific Computing
Rust’s ecosystem for scientific computing has matured significantly, offering a wide array of libraries and tools that address the diverse needs of numerical computations, linear algebra, data serialization, and random number generation. These libraries empower developers and scientists to build efficient, safe, and high-performance applications tailored to computational physics and other data-intensive disciplines. Among the most notable libraries are ndarray
, nalgebra
, serde
, and rand
. For example, ndarray
provides robust support for N-dimensional arrays, functioning similarly to Python’s NumPy for array-based computations. Meanwhile, nalgebra
serves as a comprehensive linear algebra library capable of handling complex vector and matrix operations, transformations, and decompositions. In addition, serde
facilitates the seamless serialization and deserialization of data, making it straightforward to persist and exchange simulation results in various formats (e.g., JSON or BSON). The rand
crate rounds out the ecosystem by offering efficient random number generation, which is indispensable for stochastic simulations and Monte Carlo methods.
In computational physics, tasks such as solving differential equations, performing matrix operations, and processing large datasets are everyday challenges. Libraries like ndarray
and nalgebra
prove invaluable in these scenarios: ndarray
enables the creation, manipulation, and operation of multi-dimensional arrays, which is essential for simulations that use grid-based methods or process data across multiple dimensions, while nalgebra
provides the advanced linear algebra capabilities required for efficient matrix and vector computations. Furthermore, data serialization through serde
allows simulation results to be stored and exchanged with ease, thus facilitating collaboration and the integration of Rust with other tools. The rand
crate underpins this ecosystem by generating the random values that are critical for simulations involving probabilistic models.
To illustrate how these libraries can be combined in a Rust project, consider a practical example: simulating the motion of a particle under the influence of gravity, with a twist—introducing randomness in the initial velocity and then saving the simulation results in JSON format. This example begins by setting up the project dependencies in the Cargo.toml
file:
[dependencies]
ndarray = "0.15"
nalgebra = "0.29"
serde = { version = "1.0", features = ["derive"] }
serde_json = "1.0"
rand = "0.8"
This configuration instructs Cargo to incorporate ndarray
, nalgebra
, serde
(along with its derive macros), serde_json
, and rand
into your project, enabling you to leverage their functionalities directly.
Next, implement the simulation in the src/main.rs
file. In this example, we define a Particle
struct to model a particle with three-dimensional position and velocity, along with its mass. The simulation generates a random initial velocity, then updates the particle’s state over time using a simple Euler integration method to incorporate the effects of gravitational acceleration. As the simulation runs, the position of the particle is recorded in a vector. Finally, the simulation results are serialized into JSON format and written to a file named positions.json
.
use rand::Rng;
use serde::{Serialize, Deserialize};
#[derive(Serialize, Deserialize, Debug)]
struct Particle {
position: [f64; 3], // 3D position for easy serialization
velocity: [f64; 3], // 3D velocity for easy serialization
mass: f64,
}
impl Particle {
fn new(position: [f64; 3], velocity: [f64; 3], mass: f64) -> Self {
Self { position, velocity, mass }
}
/// Updates the particle’s velocity and position based on the applied force
/// and time step (dt) using Euler integration.
fn update(&mut self, force: [f64; 3], dt: f64) {
let acceleration = [
force[0] / self.mass,
force[1] / self.mass,
force[2] / self.mass,
];
for i in 0..3 {
self.velocity[i] += acceleration[i] * dt;
self.position[i] += self.velocity[i] * dt;
}
}
}
fn main() {
let mut rng = rand::thread_rng();
let initial_velocity = [
rng.gen_range(0.0..1.0),
rng.gen_range(0.0..1.0),
0.0,
];
let mut particle = Particle::new([0.0, 0.0, 0.0], initial_velocity, 1.0);
let gravity = [0.0, -9.81, 0.0];
let time_step = 0.1;
let mut positions = Vec::new();
// Run the simulation for 100 time steps, updating the particle's state.
for _ in 0..100 {
particle.update(gravity, time_step);
positions.push(particle.position);
}
// Serialize the positions vector to a JSON string and write to a file.
let json = serde_json::to_string(&positions).unwrap();
std::fs::write("positions.json", json).expect("Unable to write file");
println!("Simulation complete. Results saved to positions.json");
}
In this code, the Particle
struct encapsulates the particle’s dynamic state, and the update
method implements Euler integration by computing the acceleration (from the applied force divided by mass) and then updating both the velocity and position over a given time step. The main function sets up a simulation by initializing a particle at the origin with a randomly generated initial velocity, applies a constant gravitational force over 100 iterations, and captures the evolving position at each time step. Finally, the positions are serialized into JSON format using serde_json
and stored in a file, showcasing Rust's robust data handling capabilities.
This practical example demonstrates the powerful synergy between Rust’s ecosystem libraries—ndarray
, nalgebra
, serde
, and rand
—and illustrates their application in solving basic physics problems. By integrating these tools, you can tackle the complexities of computational physics with efficiency and ease, whether you’re conducting numerical simulations, processing large datasets, or performing advanced data transformations. Rust’s rich ecosystem not only makes it possible to develop scalable and performant scientific applications but also ensures that your code remains safe, maintainable, and aligned with best practices in software development.
3.5. Writing and Managing Rust Code for Computational Physics
In computational physics, where simulations and models can become increasingly complex, writing efficient and maintainable code is crucial. Rust, with its strong emphasis on safety, performance, and concurrency, provides several tools and practices that help developers write code that is not only fast but also easy to maintain and extend. Efficient Rust code often involves taking full advantage of Rust’s zero-cost abstractions, such as iterators and smart pointers, which allow you to write high-level, readable code without compromising on performance. Additionally, maintainability in Rust is achieved through clear and consistent coding practices, making use of Rust's powerful type system, and leveraging the borrow checker to enforce memory safety.
Writing maintainable code also involves considering the principles of modularization and code reuse. Modularization in Rust allows developers to break down complex systems into smaller, manageable components that can be developed, tested, and maintained independently. This is especially important in computational physics projects where different aspects of a simulation, such as data input/output, computation, and visualization, can be isolated into separate modules or even crates. Code reuse is encouraged through the use of Rust's crate system, which allows developers to share and reuse libraries of code across projects, promoting efficiency and consistency.
Modularization is key to managing large codebases, particularly in computational physics, where the complexity of models and simulations can grow quickly. By dividing a project into modules, you can isolate different functionalities, making it easier to develop, test, and debug each component. In Rust, a module is essentially a namespace that can contain functions, structs, enums, and other modules. Modules can be declared in the same file or spread across multiple files, allowing for a clear organization of the code.
For instance, in a computational physics project, you might have separate modules for handling vector and matrix operations, solving differential equations, and managing input/output operations. Each of these modules can be developed independently and then integrated into the main project. This modular approach not only enhances the clarity of the code but also makes it easier to test each component in isolation, leading to more reliable and maintainable software.
Code reuse is another important concept in Rust, facilitated by the extensive ecosystem of crates available through Cargo. Crates are reusable libraries or packages that can be integrated into your projects. By using crates, you can avoid reinventing the wheel and instead build upon existing, well-tested libraries. For example, in a computational physics project, you might use the nalgebra
crate for linear algebra, the ndarray
crate for N-dimensional array operations, and the serde
crate for serialization tasks. This not only speeds up development but also ensures that your project benefits from the latest optimizations and features provided by the community.
Documentation is a critical aspect of maintaining a large codebase, particularly in scientific computing, where the code may be used or modified by other researchers. Rust encourages writing documentation directly alongside the code, using comments and Rust's built-in documentation system. This approach ensures that the documentation is always up-to-date and easily accessible to anyone working on the project. Additionally, Rust’s cargo doc
command can automatically generate HTML documentation from your code comments, making it easy to share and publish detailed documentation for your projects.
To illustrate how to organize Rust code for a complex computational physics project, consider an example where we want to simulate the motion of a system of particles under various forces. This project could be organized into multiple modules, each responsible for a different aspect of the simulation.
Start by setting up the project with the following structure:
cpvr_project
├── Cargo.toml
└── src
├── main.rs
├── physics
│ ├── mod.rs
│ ├── particle.rs
│ ├── forces.rs
└── utils
├── mod.rs
└── io.rs
In this structure, the physics
module contains sub-modules for particle
and forces
, while the utils
module handles input/output operations. The main.rs
file serves as the entry point for the application, orchestrating the various components.
main.rs:
mod physics;
mod utils;
use physics::particle::Particle;
use physics::forces::apply_gravity;
use utils::io::save_positions;
fn main() {
let mut particles = vec![
Particle::new([0.0, 0.0, 0.0], [1.0, 0.0, 0.0], 1.0),
Particle::new([1.0, 0.0, 0.0], [0.0, 1.0, 0.0], 1.0),
];
let time_step = 0.1;
for _ in 0..100 {
for particle in &mut particles {
apply_gravity(particle, time_step);
}
}
save_positions(&particles, "positions.json").expect("Failed to save positions");
}
physics/mod.rs:
pub mod particle;
pub mod forces;
physics/particle.rs:
#[derive(Debug, Clone)]
pub struct Particle {
pub position: [f64; 3],
pub velocity: [f64; 3],
pub mass: f64,
}
impl Particle {
pub fn new(position: [f64; 3], velocity: [f64; 3], mass: f64) -> Self {
Self { position, velocity, mass }
}
pub fn update_position(&mut self, dt: f64) {
for i in 0..3 {
self.position[i] += self.velocity[i] * dt;
}
}
}
physics/forces.rs:
use super::particle::Particle;
pub fn apply_gravity(particle: &mut Particle, dt: f64) {
let gravity = [0.0, -9.81, 0.0];
for i in 0..3 {
particle.velocity[i] += gravity[i] * dt;
}
particle.update_position(dt);
}
utils/mod.rs:
pub mod io;
utils/io.rs:
use serde::{Serialize, Deserialize};
use std::fs::File;
use std::io::Write;
#[derive(Serialize, Deserialize)]
pub struct ParticleData {
position: [f64; 3],
}
pub fn save_positions(particles: &[super::super::physics::particle::Particle], filename: &str) -> std::io::Result<()> {
let data: Vec<ParticleData> = particles.iter().map(|p| ParticleData { position: p.position }).collect();
let json = serde_json::to_string(&data)?;
let mut file = File::create(filename)?;
file.write_all(json.as_bytes())?;
Ok(())
}
In this example, the physics
module handles all physics-related computations, with separate sub-modules for particle management (particle.rs
) and force application (forces.rs
). The utils
module manages input/output operations, such as saving particle positions to a file. The main.rs
file brings these components together, running the simulation and storing the results.
This modular approach makes the codebase easier to manage and extend. For instance, if you need to add new forces or change the way particles are updated, you can do so in the appropriate module without affecting the rest of the code. Additionally, the clear separation of concerns makes it easier to test individual components in isolation, ensuring that each part of the simulation behaves as expected.
In summary, writing and managing Rust code for computational physics involves following principles of modularization, code reuse, and documentation. By organizing your code into modules and crates, you can handle complex projects more effectively, making your codebase more maintainable and scalable. Rust’s ecosystem supports these practices, providing the tools necessary to develop robust, high-performance scientific applications. This structured approach to code organization is crucial in computational physics, where the complexity of simulations and models demands clarity, efficiency, and flexibility.
3.6. Testing and Benchmarking Computational Physics Code
In computational physics, where simulations and models can quickly become complex, writing efficient and maintainable code is paramount. Rust, with its strong emphasis on safety, performance, and concurrency, offers a range of tools and best practices that help developers produce code that is both fast and easy to maintain and extend. Rust’s zero-cost abstractions, such as iterators and smart pointers, enable you to write high-level, expressive code without sacrificing performance. Furthermore, Rust’s robust type system and borrow checker contribute to memory safety and clarity, ensuring that your code remains bug-free and easier to extend over time.
Maintaining a large codebase also involves careful attention to modularity and code reuse. In Rust, this is achieved through the module system, which allows you to break your code into logically separated units. Each module can encapsulate specific functionality—such as handling data input/output, performing complex calculations, or managing state—which makes development, testing, and debugging significantly more manageable. By organizing code into separate modules (or even independent crates), you can isolate different aspects of a simulation, making it much simpler to update or replace individual components without affecting the rest of your project.
Code reuse is further enhanced by Rust’s extensive ecosystem of crates, which promotes consistency and efficiency. Instead of reimplementing common functionalities, you can rely on well-tested libraries for tasks like linear algebra, data serialization, or random number generation. This not only speeds up development but also ensures that your project benefits from the latest optimizations and improvements available in the community.
Documentation plays a critical role as well, especially in scientific computing where code is often shared among researchers or revisited long after its initial development. Rust encourages embedding documentation directly within the code using doc comments, which can be compiled into comprehensive HTML documentation via the cargo doc
command. This practice ensures that your documentation remains up-to-date and accessible to anyone interacting with your project.
To illustrate how to organize Rust code for a complex computational physics project, consider an example where we simulate the motion of a system of particles under various forces. In this design, the project is modularized into components responsible for physics computations (such as particle dynamics and force calculations) and utilities for operations like data input/output. The following directory structure demonstrates one way to organize such a project:
cpvr_project
├── Cargo.toml
└── src
├── main.rs
├── physics
│ ├── mod.rs
│ ├── particle.rs
│ ├── forces.rs
└── utils
├── mod.rs
└── io.rs
In this structure, the physics
module encapsulates all simulation logic—dividing responsibilities into sub-modules for managing particles (particle.rs
) and applying forces (forces.rs
). The utils
module handles auxiliary tasks, such as input/output operations, while main.rs
serves as the orchestrator, tying together the individual components of the simulation.
Below is an example implementation illustrating this modular approach:
main.rs:
mod physics;
mod utils;
use physics::particle::Particle;
use physics::forces::apply_gravity;
use utils::io::save_positions;
fn main() {
// Initialize a vector of particles with different initial positions and velocities.
let mut particles = vec![
Particle::new([0.0, 0.0, 0.0], [1.0, 0.0, 0.0], 1.0),
Particle::new([1.0, 0.0, 0.0], [0.0, 1.0, 0.0], 1.0),
];
let time_step = 0.1;
// Simulate for 100 time steps, applying gravity to all particles.
for _ in 0..100 {
for particle in &mut particles {
apply_gravity(particle, time_step);
}
}
// Save the final positions of the particles to a JSON file.
save_positions(&particles, "positions.json").expect("Failed to save positions");
}
physics/mod.rs:
pub mod particle;
pub mod forces;
physics/particle.rs:
#[derive(Debug, Clone)]
pub struct Particle {
pub position: [f64; 3],
pub velocity: [f64; 3],
pub mass: f64,
}
impl Particle {
pub fn new(position: [f64; 3], velocity: [f64; 3], mass: f64) -> Self {
Self { position, velocity, mass }
}
/// Updates the particle's position using its current velocity and a given time step.
pub fn update_position(&mut self, dt: f64) {
for i in 0..3 {
self.position[i] += self.velocity[i] * dt;
}
}
}
physics/forces.rs:
use super::particle::Particle;
/// Applies gravitational force to a particle over a time step.
/// Assumes a constant gravitational acceleration in the negative y-direction.
pub fn apply_gravity(particle: &mut Particle, dt: f64) {
let gravity = [0.0, -9.81, 0.0];
for i in 0..3 {
particle.velocity[i] += gravity[i] * dt;
}
particle.update_position(dt);
}
utils/mod.rs:
pub mod io;
utils/io.rs:
use serde::{Serialize, Deserialize};
use std::fs::File;
use std::io::Write;
#[derive(Serialize, Deserialize)]
pub struct ParticleData {
position: [f64; 3],
}
/// Saves the positions of particles to a JSON file.
pub fn save_positions(particles: &[super::super::physics::particle::Particle], filename: &str) -> std::io::Result<()> {
let data: Vec<ParticleData> = particles
.iter()
.map(|p| ParticleData { position: p.position })
.collect();
let json = serde_json::to_string(&data)?;
let mut file = File::create(filename)?;
file.write_all(json.as_bytes())?;
Ok(())
}
In this example, the physics
module encapsulates the core computational logic. The particle
sub-module defines a Particle
struct along with methods to initialize it and update its position based on its velocity. The forces
sub-module provides functions to apply forces—such as gravity—to the particles. The utils
module, on the other hand, contains functions for auxiliary operations, such as saving simulation results to a JSON file.
This modular approach not only organizes your code into manageable and reusable components but also makes it easier to extend your project. For example, if you need to introduce additional forces (e.g., friction, electromagnetic forces) or modify how the particles update their positions, these changes can be made in their respective modules without affecting the overall structure of the project. Moreover, this separation of concerns facilitates targeted testing and debugging, ensuring that each component performs as expected before being integrated into the larger system.
In summary, writing and managing Rust code for computational physics involves embracing modularization, code reuse, and thorough documentation practices. By structuring your project into modules and taking advantage of Rust's powerful crate ecosystem, you can tackle complex simulations effectively while keeping your codebase maintainable and scalable. These practices are critical in computational physics, where clarity, efficiency, and flexibility are essential to successfully model and analyze complex systems.
3.7. Debugging and Profiling Rust Applications
In the realm of scientific computing, ensuring that your simulation produces correct and efficient results is paramount. The complexity of many models means that even small bugs or performance bottlenecks can lead to significant errors or slow computations. Debugging is the systematic process of identifying and fixing these errors, while profiling enables you to understand where your program spends most of its time. Together, these practices are essential for maintaining the reliability and efficiency of computational physics applications. They not only help you ensure that your code produces accurate results, but they also enable you to optimize your application for better performance when running large-scale simulations.
Rust provides a rich set of tools to assist in both debugging and profiling, making it easier to pinpoint issues and optimize performance. Traditional debuggers such as gdb (GNU Debugger) and lldb (LLVM Debugger) work well with Rust, allowing you to inspect program state, set breakpoints, and step through code interactively. In addition, Cargo commands like cargo check
can quickly identify potential errors without performing a full build, which is especially useful in the iterative process of developing complex simulations.
For profiling, Rust developers have access to tools such as perf on Linux and Instruments on macOS. These tools help identify performance bottlenecks by revealing which sections of your code consume the most time or system resources. Such insights are invaluable in scientific computing, where the performance of algorithms and simulations directly impacts the feasibility of large-scale studies.
Consider the following example where a simulation of particle motion under the influence of gravity produces unexpected results. In this code, a simple particle’s position is updated over time using a basic model, yet the position may not change as expected, indicating a possible logical error.
// src/main.rs
struct Particle {
position: f64,
velocity: f64,
mass: f64,
}
impl Particle {
fn new(position: f64, velocity: f64, mass: f64) -> Self {
Self { position, velocity, mass }
}
fn apply_gravity(&mut self, dt: f64) {
let gravity = 9.81;
let acceleration = gravity / self.mass;
self.velocity += acceleration * dt;
self.position += self.velocity * dt;
}
}
fn main() {
let mut particle = Particle::new(0.0, 0.0, 1.0);
for _ in 0..10 {
particle.apply_gravity(1.0);
println!("Position: {}", particle.position);
}
}
In this program, the expectation is that the particle’s position should increase over time as it accelerates under the force of gravity. If the printed positions do not change as expected, there is likely a logical issue in the computation of the particle’s motion. To debug this issue, you could use gdb or lldb. For example, compile the program in debug mode with:
cargo build
Then start gdb with:
gdb target/debug/cpvr_project
Set a breakpoint at the beginning of the apply_gravity
function:
break src/main.rs:10
Run the program within gdb by typing run
, and use the next
command to step through each line. Inspect variables like position
, velocity
, and acceleration
to determine if they are being updated as intended. This step-by-step debugging process can help you uncover logical errors—such as an incorrect time step or arithmetic miscalculation—that cause the simulation to behave unexpectedly.
Once logical errors have been fixed, profiling is the next critical step to ensure the simulation runs efficiently, especially in computationally intensive scenarios. For example, on a Linux system, you can profile the application using perf:
Compile an optimized version of your code with:
cargo build --release
Record the performance data with:
perf record --call-graph=dwarf ./target/release/cpvr_project
Analyze the results by running:
perf report
Profiling may reveal that a significant amount of time is consumed within the apply_gravity
function or elsewhere in your simulation loop. With this data, you can explore further optimizations such as reducing the overhead of floating-point operations or parallelizing computations using Rust’s concurrency features if the simulation warrants it.
Memory profiling is another important aspect, especially in applications that process large datasets. Tools like Valgrind or Heaptrack can help you identify memory leaks or excessive allocation, which might lead to performance degradation or even crashes.
In summary, debugging and profiling are critical components of developing high-quality, efficient scientific computing applications in Rust. With tools such as gdb, lldb, cargo check, and perf, you can systematically identify, isolate, and fix logical errors and performance bottlenecks. This rigorous approach not only ensures that your simulations produce accurate results, but also guarantees that they run efficiently at scale—an essential requirement for tackling complex, real-world scientific problems. Adopting these practices will make your computational physics projects more robust, reliable, and performant over time.
3.8. Setting Up Version Control and Collaboration
Version control is a cornerstone of modern software development, and its importance in computational physics cannot be overstated. As scientific models and simulations become increasingly complex, managing changes, tracking the evolution of code, and collaborating with others are crucial for maintaining accuracy and reproducibility. Git is the most widely used version control system, offering a distributed model that allows multiple contributors to work on a project simultaneously, each with their own copy of the entire project history. Git enables developers to track changes over time, revert to previous versions, and collaborate effectively with others, all while maintaining a detailed log of who made what changes and why. This level of control and transparency is essential in computational physics, where ensuring the integrity of the codebase directly impacts the reliability of simulation results.
In collaborative computational physics projects, where multiple researchers or developers may be working on different aspects of a simulation or model, establishing best practices for version control is critical. One of the key practices is using feature branches. Instead of working directly on the main branch, each contributor should create a new branch for their specific feature or bug fix. This approach isolates their changes from the main codebase, reducing the risk of introducing bugs or conflicts. Once the feature is complete and tested, it can be merged back into the main branch, typically through a pull request. Pull requests are an essential tool in collaborative work as they allow for code review, ensuring that multiple sets of eyes have vetted the changes before they become part of the official project.
Another best practice is writing clear and concise commit messages. Each commit should represent a logical, self-contained change, and the commit message should describe what was changed and why. This practice not only helps in understanding the project history but also makes it easier to track down issues if something goes wrong. Additionally, regular commits are encouraged, as they create a detailed history of changes, making it easier to identify when and where a problem was introduced.
Finally, incorporating continuous integration (CI) can significantly enhance collaboration in computational physics projects. CI systems automatically build and test code whenever changes are pushed to the repository, ensuring that all contributions are compatible and that no new errors have been introduced. This automated process helps maintain the stability and reliability of the codebase, even as multiple contributors work simultaneously.
To begin using Git for version control in a computational physics project, you first need to initialize a Git repository. Start by navigating to your project directory in the terminal and running the following command:
git init
This command initializes a new Git repository in your project directory, creating a hidden .git
folder that will store all the version control information. After initializing the repository, the next step is to add your project files to the repository and commit the initial state:
git add .
git commit -m "Initial commit"
The git add .
command stages all the files in your project for the next commit, while git commit -m "Initial commit"
creates a new commit with the message "Initial commit," marking the starting point of your project’s history.
If you are collaborating with others, you’ll want to push your repository to a remote server like GitHub, GitLab, or Bitbucket. Assuming you have already created a repository on GitHub, you can add it as a remote to your local repository with the following command:
git remote add origin https://github.com/username/repository.git
git push -u origin master
This command links your local repository to the remote repository on GitHub, allowing you to push your changes to the server. The git push -u origin master
command pushes your local master
branch to the remote origin
, making your project accessible to collaborators.
When working on a new feature or fix, create a new branch to keep your changes isolated from the main branch:
git checkout -b feature-branch
This command creates a new branch called feature-branch
and switches to it. You can now work on your feature independently. After completing the feature, commit your changes:
git add .
git commit -m "Implemented feature X"
To share your branch with collaborators, push it to the remote repository:
git push origin feature-branch
Once the feature is ready to be integrated into the main branch, you can open a pull request on GitHub. Pull requests are a key collaborative tool, allowing others to review your code, suggest changes, and ensure that everything works as expected before merging it into the main branch.
If you encounter conflicts when merging your branch into the main branch, Git will notify you. Resolving conflicts involves manually editing the conflicting files to decide which changes should be kept. After resolving conflicts, you can complete the merge:
git checkout master
git merge feature-branch
Finally, as the project evolves, you may need to manage different versions of the software, especially in computational physics, where maintaining the integrity of previous versions is often critical for reproducibility. Git’s tagging feature allows you to mark specific points in your project history as important, such as version releases:
git tag -a v1.0 -m "Version 1.0 release"
git push origin v1.0
Tags are immutable references to specific commits and are commonly used to indicate versions or milestones in the project. By tagging releases, you ensure that you can always go back to a specific version if needed.
In conclusion, setting up version control with Git is essential for managing and collaborating on computational physics projects. By following best practices such as using feature branches, writing clear commit messages, and leveraging CI systems, you can maintain a clean, reliable, and collaborative codebase. Git’s tools for branching, merging, and tagging provide the flexibility needed to manage complex projects effectively, ensuring that your simulations and models are developed in a structured and reproducible manner.
3.9. Deploying and Sharing Computational Physics Applications
In computational physics, the ability to share and deploy scientific software is essential for advancing research, fostering collaboration, and ensuring reproducibility. By sharing software, researchers enable others to verify their results, build upon their work, and apply the tools to different problems. Deployment refers to the process of making a software application available for use, whether by distributing it as a binary executable, publishing the source code, or hosting it on a platform where others can run the application directly. This process is critical in scientific computing, where the tools and simulations developed often need to be accessible to a broader community, including collaborators, peer reviewers, and the wider scientific community.
Rust provides several methods for packaging and sharing applications, making it easier for developers to distribute their computational physics software. One common approach is to package the application as a binary executable, which can be shared and run on different systems without requiring the end user to compile the code themselves. This method is particularly useful for ensuring that the software runs in a controlled environment, with all dependencies bundled together. Alternatively, developers can share the source code itself, allowing others to compile and modify the software. This approach is ideal for collaborative projects where transparency and flexibility are crucial.
When sharing Rust applications, Cargo, Rust’s package manager, plays a central role. Cargo can compile the code into binaries for different platforms, package the binaries, and even publish the code to crates.io, Rust’s package registry, or other platforms like GitHub. Moreover, for scientific applications that need to run on multiple operating systems, cross-compilation is a key feature, allowing developers to build executables for different platforms from a single codebase.
To deploy a Rust application, the first step is to build the project in release mode, which optimizes the code for performance. In the context of a computational physics application, this optimization is crucial, as simulations and numerical computations often require significant processing power.
For example, consider a Rust application that simulates the dynamics of a particle system. To compile this application for distribution, navigate to the project directory and run:
cargo build --release
This command generates an optimized binary in the target/release
directory. The resulting binary can be shared with others who can run the application directly, without needing to install Rust or the application’s dependencies.
If the application needs to be shared across different platforms, such as Windows, macOS, and Linux, cross-compilation is the next step. Rust’s cross-compilation capabilities allow you to build binaries for different target platforms from a single development environment. For instance, to compile the application for Windows from a Linux machine, you would install the appropriate target:
rustup target add x86_64-pc-windows-gnu
Then, build the project for that target:
cargo build --release --target x86_64-pc-windows-gnu
This command generates a Windows-compatible binary that can be shared with users on that platform.
For developers who prefer to share the source code, platforms like GitHub provide an excellent means of distributing and collaborating on Rust projects. To share a Rust project on GitHub, start by creating a new repository on the GitHub website. Then, add the repository as a remote to your local project:
git remote add origin https://github.com/username/repository.git
git push -u origin master
This process uploads the source code to GitHub, making it accessible to others who can clone the repository, contribute to the project, or compile the software themselves.
In some cases, it may be beneficial to package the Rust application as a crate and publish it on crates.io, especially if the application includes reusable components that others might find useful. To do this, ensure that your Cargo.toml
file is configured with the necessary metadata, such as the name, version, and authorship information. Then, log in to crates.io using Cargo and publish the crate:
cargo login
cargo publish
This process makes the crate available to the Rust community, where it can be easily integrated into other projects using Cargo.
In addition to distributing binaries or source code, developers might also consider using platforms like Docker to create containerized versions of their applications. Containers bundle the application and all its dependencies into a single package that can run consistently across different environments. This approach is particularly useful for ensuring that scientific software runs reliably, regardless of the underlying system configuration.
To create a Docker container for a Rust application, start by creating a Dockerfile
in the project directory:
FROM rust:latest
WORKDIR /usr/src/app
COPY . .
RUN cargo build --release
CMD ["./target/release/your_app_name"]
This Dockerfile
sets up a Rust environment, copies the project files, builds the application in release mode, and specifies the command to run the compiled binary. To build the Docker image, use the following command:
docker build -t your_app_name .
Once built, the Docker image can be shared or deployed to a container registry like Docker Hub, allowing others to pull and run the containerized application.
Finally, deploying Rust applications to cloud platforms, such as AWS, Google Cloud, or Heroku, can make the software accessible to a broader audience. These platforms provide infrastructure that can scale with demand, making them suitable for running intensive computational simulations or serving a web-based interface to your scientific application.
In summary, deploying and sharing computational physics applications developed in Rust involves several key steps, including compiling binaries, cross-compiling for different platforms, sharing source code through platforms like GitHub, publishing crates on crates.io, and using Docker for containerization. These practices ensure that the software is accessible, reliable, and easy to use across various environments, enabling researchers and developers to collaborate more effectively and advance scientific knowledge.
3.10. Conclusion
In this chapter, we have established a solid foundation for implementing computational physics using Rust. By setting up a precise and efficient computational environment, mastering essential tools like Cargo, and adopting best practices for code management and deployment, we equip ourselves to tackle complex scientific problems with confidence. This preparation ensures that our computational endeavors are not only rigorous but also ready for collaboration and sharing within the scientific community.
3.10.1. Further Learning with GenAI
Each prompt encourages learners to investigate not just the "how," but the "why," fostering a deeper understanding of Rust's features and their applications in computational physics. The goal is to challenge learners to think critically, explore advanced concepts, and develop practical skills that will enhance their ability to implement complex simulations and analyses in Rust.
Discuss the foundational principles of computational physics with a focus on the key numerical methods and algorithms commonly employed in solving complex physical problems. How does Rust’s strict type system, zero-cost abstractions, and memory safety model ensure the accurate and efficient implementation of these methods, particularly in scenarios involving parallelism, large datasets, or floating-point precision?
Compare and contrast Rust’s approach to memory management—specifically its ownership, borrowing, and lifetime models—with those of languages like C++ and Fortran, in the context of large-scale computational physics simulations. How do these differences affect the performance, safety, and reliability of long-running scientific computations, particularly when managing memory-intensive tasks such as matrix operations or finite element analysis?
Provide a detailed guide to installing Rust on different operating systems, including Windows, macOS, and Linux, with advanced configuration options for optimizing the development environment for high-performance scientific computing. What are the best practices for setting up custom compiler flags, linking against external libraries, and configuring parallel builds to maximize efficiency on each platform?
Explore the role of Rustup in managing multiple toolchains and components across different versions of Rust. How can computational physics projects benefit from using specific versions of the Rust compiler, and what are best practices for managing dependencies and ensuring compatibility across diverse environments or when switching between stable, nightly, and custom toolchains?
Examine the advanced capabilities of Cargo, Rust’s build system and package manager, focusing on features like workspaces, custom profiles, build scripts, and offline mode. How can these features be leveraged to manage complex computational physics projects with multiple dependencies and extensive customization, such as integrating third-party libraries, automating builds, and optimizing performance?
Walk through the process of creating, documenting, testing, and publishing a Rust crate designed specifically for computational physics applications. What best practices should be followed to ensure that the crate is robust, modular, well-documented, and easily integrable with other scientific libraries and tools? Provide guidance on versioning, testing strategies, and using continuous integration for scientific software development.
Analyze the performance, flexibility, and limitations of key Rust libraries such as
ndarray
,nalgebra
,serde
, andrand
within the context of scientific computing. How do these libraries compare to their counterparts in languages like Python (NumPy), C++ (Eigen), or MATLAB in terms of handling large datasets, supporting complex mathematical operations, and enabling efficient parallel computations?Investigate how Rust can be integrated with external scientific libraries and tools written in other languages (e.g., C, C++, Python) using Foreign Function Interface (FFI) or other interlanguage communication methods. Provide a detailed example of how to extend Rust's capabilities by interfacing with a high-performance C library for numerical computations, ensuring that the integration is efficient, safe, and scalable for scientific workloads.
Delve into the principles of modularization, code reuse, and documentation in Rust, particularly for structuring large computational physics projects. How can these principles be applied to create maintainable, scalable, and clear codebases that are optimized for performance and collaboration? Provide examples of how to organize code using modules, crates, and workspaces, ensuring that the project remains well-structured and accessible for both development and future extensions.
Discuss the importance of comprehensive documentation in computational physics projects and how Rust’s documentation tools, such as
rustdoc
, can be utilized to create accessible, detailed, and accurate documentation that enhances the usability and reproducibility of scientific software. What strategies should be employed to ensure that documentation keeps pace with code changes, and how can code examples, tutorials, and API references be structured to assist both novice and expert users?Explore the process of writing unit tests, integration tests, and property-based tests in Rust, specifically for validating computational physics applications. How can these tests be designed to rigorously validate the correctness, accuracy, and reliability of complex algorithms and simulations? Provide examples of testing edge cases, numerical stability, performance under high computational loads, and validation of scientific results.
Discuss the importance of benchmarking in computational physics and provide a step-by-step guide to using Rust’s benchmarking tools such as Criterion and
cargo bench
to measure and optimize the performance of scientific simulations. How can detailed benchmarking be used to identify performance bottlenecks, optimize memory usage, and ensure that simulations scale effectively across multiple processing cores or large datasets?Provide an in-depth exploration of debugging tools and techniques in Rust, focusing on their application in computational physics. How can tools such as GDB, LLDB,
cargo check
, and Miri be utilized to debug complex scientific simulations, particularly in identifying memory leaks, concurrency bugs, numerical inaccuracies, or performance degradation? Provide concrete examples of troubleshooting these issues in real-world computational physics projects.Investigate profiling techniques and tools available in Rust, such as
perf
,valgrind
,cargo-profiler
, andflamegraph
. How can these tools be applied to analyze the performance of computational physics applications, especially in scenarios involving parallel processing, large-scale simulations, or complex numerical computations? What strategies should be employed to ensure that profiling data is used effectively to optimize the overall performance of the application?Examine best practices for using Git in large and complex scientific computing projects. How can advanced Git features such as submodules, large file storage (LFS), Git hooks, and automated code quality checks be employed to manage dependencies, handle large datasets, and ensure code reliability and collaboration in computational physics projects involving multiple contributors and long-term development cycles?
Provide a comprehensive guide to setting up a collaborative environment for computational physics using Git and platforms like GitHub or GitLab. Discuss advanced branching strategies, pull request workflows, continuous integration, and automated testing. How can these practices be optimized to enhance collaboration, improve code quality, and streamline project management for large-scale scientific research initiatives?
Explore various deployment strategies for Rust-based computational physics applications, including packaging binaries, creating Docker images, and deploying to cloud platforms such as AWS, Azure, or Google Cloud. How can these deployment methods be optimized for scalability, security, and performance in high-performance scientific applications, particularly when handling sensitive data or requiring real-time simulation capabilities?
Discuss the challenges and best practices for sharing scientific software with the broader research community. How can Rust-based applications be distributed effectively via crates.io, GitHub, or other platforms while ensuring reproducibility, usability, and compatibility across different environments? Provide strategies for packaging scientific applications, managing dependencies, and providing detailed documentation for installation and usage to maximize impact and accessibility.
Mastering the art of computational physics using Rust is not just about learning syntax and tools—it's about cultivating a mindset of precision, efficiency, and innovation. By diving deep into these prompts, you are not only honing your technical skills but also developing the ability to tackle complex scientific challenges with confidence and creativity. As you practice, remember that your efforts are laying the foundation for groundbreaking discoveries and advancements in science. Keep pushing your boundaries, and let your curiosity drive you to explore the limitless possibilities of what you can achieve with Rust.
3.10.2. Assignments for Practice
Here are five in-depth self-exercises based on the previous prompts that will guide readers in practicing and applying their knowledge using GenAI (ChatGPT or Gemini). These exercises are designed to encourage hands-on engagement with Rust and computational physics concepts, leveraging GenAI as a learning tool.
Exercise 4.1: Exploring Memory Safety in Computational Physics with Rust
Objective: Understand how Rust’s memory safety features (ownership, borrowing, lifetimes) can be applied in computational physics simulations to ensure correctness and efficiency.
Instructions:
Ask GenAI to explain the core concepts of ownership, borrowing, and lifetimes in Rust, with examples specifically related to handling large arrays or matrices in physics simulations.
Experiment by writing a simple Rust program that simulates a physical system (e.g., harmonic oscillator) using dynamic arrays. Implement functions that borrow and return references to data, and observe how Rust enforces memory safety.
Challenge yourself by modifying the program to introduce intentional memory errors, then ask GenAI to help debug and explain why Rust’s compiler catches these errors.
Reflect by discussing with GenAI how Rust’s memory safety features compare to other languages (e.g., C++), particularly in the context of avoiding common issues like dangling pointers and data races.
Exercise 4.2: Setting Up and Managing Rust Toolchains for Scientific Projects
Objective: Gain proficiency in using Rustup to manage toolchains and components, optimizing the development environment for computational physics tasks.
Instructions:
Ask GenAI for a detailed explanation of how Rustup manages multiple toolchains and components. Explore how different versions of Rust can be installed and used in specific projects.
Set up Rust on your machine using Rustup, then experiment with installing additional components (e.g., clippy, rustfmt) and switching between stable, beta, and nightly toolchains.
Create a sample project that requires a specific toolchain (e.g., nightly). Use Cargo to manage dependencies, and ask GenAI for advice on handling version conflicts or dependency issues.
Reflect on the experience by asking GenAI how to best manage toolchains and dependencies in large-scale scientific computing projects, ensuring stability and compatibility across different environments.
Exercise 4.3: Benchmarking and Optimizing Computational Physics Code in Rust
Objective: Learn how to benchmark and optimize the performance of computational physics applications written in Rust.
Instructions:
Start by asking GenAI for a tutorial on setting up a benchmarking framework in Rust using tools like
criterion
andcargo bench
.Write a Rust program that simulates a computationally intensive physics problem (e.g., N-body simulation). Benchmark the program’s performance using the tools discussed with GenAI.
Optimize the code by experimenting with different data structures, parallelism, or algorithmic improvements. Use GenAI to guide you through potential optimizations and best practices.
Analyze the results by comparing the before-and-after performance metrics. Discuss with GenAI the impact of each optimization and how similar techniques could be applied to other computational physics problems.
Exercise 4.4: Debugging Complex Issues in Rust for Scientific Simulations
Objective: Develop skills in debugging Rust applications, focusing on resolving issues common in scientific simulations, such as memory leaks, concurrency bugs, and numerical inaccuracies.
Instructions:
Ask GenAI for an in-depth guide to the debugging tools available in Rust, such as
gdb
,lldb
,cargo check
, andmiri
.Write a Rust program that performs a simulation prone to common issues (e.g., a multi-threaded simulation of fluid dynamics). Introduce intentional bugs, such as incorrect thread synchronization or memory leaks.
Debug the program using the tools discussed with GenAI, focusing on identifying and fixing the issues. Ask GenAI to explain why the bugs occurred and how Rust’s debugging tools help in resolving them.
Reflect by discussing with GenAI the importance of debugging in scientific computing and how Rust’s tools and features contribute to developing reliable and efficient simulations.
Exercise 4.5: Collaborating on Computational Physics Projects Using Git and Rust
Objective: Learn how to set up and manage a collaborative environment for a computational physics project using Git and Rust.
Instructions:
Ask GenAI for best practices on setting up a collaborative development workflow using Git and Rust, including branching strategies, pull requests, and continuous integration.
Create a Rust-based computational physics project and set up a Git repository for it. Use Git to manage the project, including handling dependencies and documentation.
Simulate collaboration by creating branches, making changes, and merging them. Ask GenAI for advice on resolving merge conflicts and managing large codebases.
Set up continuous integration using a platform like GitHub Actions, and ask GenAI for guidance on integrating testing and benchmarking into the CI pipeline.
Reflect by discussing with GenAI how these collaborative practices contribute to the success of large-scale scientific computing projects and how they can be applied to your future work.
These exercises are designed to immerse you in the practical aspects of using Rust for computational physics, providing hands-on experience that goes beyond theory. By engaging deeply with these exercises and using GenAI as a learning tool, you’ll develop the skills and confidence needed to tackle complex scientific challenges with Rust. The path to mastery is built through practice and exploration—embrace the opportunity to learn, experiment, and innovate.